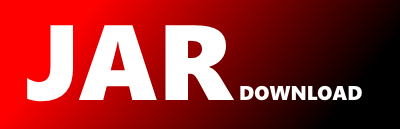
org.jasig.web.util.LRUTrackingModelPasser Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of AjaxPortletSupport Show documentation
Show all versions of AjaxPortletSupport Show documentation
Portlet and Servlet MVC classes to allow AJAX in a portlet.
/**
* Copyright 2007 The JA-SIG Collaborative. All rights reserved.
* See license distributed with this file and
* available online at http://www.uportal.org/license.html
*/
package org.jasig.web.util;
import java.util.Map;
import javax.portlet.ActionRequest;
import javax.portlet.ActionResponse;
import javax.portlet.PortletSession;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.HttpSession;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.springframework.web.portlet.util.PortletUtils;
/**
* Model passer that uses an {@link LRUMap} in the user's session to track model objects for keys. This approach
* allows a key to be re-used as long as it remains in the map. Keys are purged in LRU order once the map hits
* its maximum capacity.
*
* @author Eric Dalquist
* @version $Revision: 1.1 $
*/
public class LRUTrackingModelPasser implements ModelPasser {
private static final String MODEL_CACHE_KEY = LRUTrackingModelPasser.class.getName() + ".MODEL_CACHE_KEY";
protected final Log logger = LogFactory.getLog(this.getClass());
private int maxSize = 100;
public int getMaxSize() {
return this.maxSize;
}
/**
* The maximum size of the key cache per user session. Defaults to 100.
*/
public void setMaxSize(int maxSize) {
this.maxSize = maxSize;
}
/* (non-Javadoc)
* @see org.jasig.web.util.ModelPasser#getModelFromPortlet(javax.servlet.http.HttpServletRequest, javax.servlet.http.HttpServletResponse, java.lang.String)
*/
@SuppressWarnings("unchecked")
public Map getModelFromPortlet(HttpServletRequest request, HttpServletResponse response, String key) {
final HttpSession session = request.getSession(false);
if (session == null) {
this.logger.debug("No session available, returning null for key '" + key + "'");
return null;
}
final Map> modelCache = (Map>)session.getAttribute(MODEL_CACHE_KEY);
if (modelCache == null) {
this.logger.debug("No model cache Map in session, returning null for key '" + key + "'");
return null;
}
final Map model = modelCache.get(key);
if (this.logger.isDebugEnabled()) {
this.logger.debug("Found model '" + model + "' in HttpSession for key '" + key + "'");
}
return model;
}
/* (non-Javadoc)
* @see org.jasig.web.util.ModelPasser#passModelToServlet(javax.portlet.ActionRequest, javax.portlet.ActionResponse, java.lang.String, java.util.Map)
*/
public void passModelToServlet(ActionRequest request, ActionResponse response, String key, Map model) {
final PortletSession portletSession = request.getPortletSession();
final Map> modelCache = this.getModelCache(portletSession);
modelCache.put(key, model);
if (this.logger.isDebugEnabled()) {
this.logger.debug("Stored model '" + model + "' in cache Map for key '" + key + "'");
}
}
@SuppressWarnings("unchecked")
protected Map> getModelCache(final PortletSession portletSession) {
Map> modelCache;
synchronized (PortletUtils.getSessionMutex(portletSession)) {
modelCache = (Map>)portletSession.getAttribute(MODEL_CACHE_KEY, PortletSession.APPLICATION_SCOPE);
if (modelCache == null) {
modelCache = new LoggingLRUMap(this.maxSize);
}
//Call set on each access so the container can track session updates for replication
portletSession.setAttribute(MODEL_CACHE_KEY, modelCache, PortletSession.APPLICATION_SCOPE);
}
return modelCache;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy