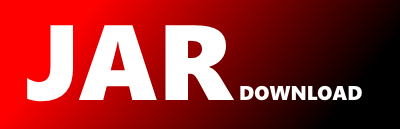
org.jastadd.ast.AST.InterfaceDecl Maven / Gradle / Ivy
/* This file was generated with JastAdd2 (http://jastadd.org) version 2.2.0 */
package org.jastadd.ast.AST;
import org.jastadd.tinytemplate.*;
import org.jastadd.Configuration;
import java.util.*;
import java.io.*;
import org.jastadd.ast.AST.*;
import org.jastadd.jrag.*;
import org.jastadd.jrag.AST.ASTCompilationUnit;
import org.jastadd.jrag.AST.ASTBlock;
import org.jastadd.JastAdd;
import java.util.Collection;
import java.util.LinkedList;
import java.util.regex.*;
import org.jastadd.jrag.AST.ASTExpression;
import java.util.Set;
import java.util.HashSet;
import java.util.ArrayList;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import org.jastadd.jrag.Unparser;
import org.jastadd.Problem;
import org.jastadd.jrag.AST.ASTAspectMethodDeclaration;
import org.jastadd.jrag.AST.ASTAspectRefineMethodDeclaration;
import org.jastadd.jrag.AST.ASTAspectFieldDeclaration;
import org.jastadd.jrag.ClassBodyObject;
import org.jastadd.ast.AST.Token;
import org.jastadd.ast.AST.SimpleNode;
import java.io.PrintStream;
/**
* @ast node
* @declaredat /home/jesper/git/jastadd2/src/jastadd/ast/Ast.ast:12
* @production InterfaceDecl : {@link TypeDecl};
*/
public class InterfaceDecl extends TypeDecl implements Cloneable {
/**
* @aspect JragCodeGen
* @declaredat /home/jesper/git/jastadd2/src/jastadd/ast/JragCodeGen.jrag:127
*/
public void emitMembers(PrintStream out) {
for (ClassBodyObject obj : classBodyDecls) {
org.jastadd.jrag.AST.SimpleNode n = obj.node;
if (n instanceof org.jastadd.jrag.AST.ASTAspectMethodDeclaration) {
try {
StringBuffer buf = new StringBuffer();
org.jastadd.jrag.AST.ASTAspectMethodDeclaration decl =
(org.jastadd.jrag.AST.ASTAspectMethodDeclaration) n;
ClassBodyDeclUnparser.unparseAbstract(decl, buf);
out.print(buf);
} catch (Exception e) {
System.err.println("Error generating " + obj.signature() + " in " + name());
e.printStackTrace();
}
} else if (n instanceof org.jastadd.jrag.AST.ASTAspectRefineMethodDeclaration) {
try {
StringBuffer buf = new StringBuffer();
org.jastadd.jrag.AST.ASTAspectRefineMethodDeclaration decl =
(org.jastadd.jrag.AST.ASTAspectRefineMethodDeclaration) n;
ClassBodyDeclUnparser.unparseAbstract(decl, buf);
out.print(buf);
} catch (Exception e) {
System.err.println("Error generating " + obj.signature() + " in " + name());
e.printStackTrace();
}
} else if (n instanceof org.jastadd.jrag.AST.ASTBlock) {
// Do not emit refined implementations.
} else {
if (!obj.comments.equals("")) {
out.print(obj.comments + " ");
}
out.print(obj.modifiers());
StringBuffer buf = new StringBuffer();
n.jjtAccept(new ClassBodyDeclUnparser(), buf);
out.print(buf.toString());
}
out.println();
}
}
/**
* @aspect JastAddCodeGen
* @declaredat /home/jesper/git/jastadd2/src/jastadd/ast/JastAddCodeGen.jadd:153
*/
public String typeDeclarationString() {
String interfaces = interfacesString();
if (interfaces.equals("")) {
return String.format("%sinterface %s%s {", modifiers(), getIdDecl().getID(), typeParameters);
} else {
return String.format("%sinterface %s%s extends %s {", modifiers(), getIdDecl().getID(),
typeParameters, interfaces);
}
}
/**
* @aspect JastAddCodeGen
* @declaredat /home/jesper/git/jastadd2/src/jastadd/ast/JastAddCodeGen.jadd:217
*/
public void jastAddGen(boolean publicModifier) {
File file = grammar().targetJavaFile(name());
try {
PrintStream stream = new PrintStream(new FileOutputStream(file));
if ( !config().license.isEmpty()) {
stream.println(config().license);
}
// TODO(joqvist): move to template.
if (!config().packageName().isEmpty()) {
stream.println("package " + config().packageName() + ";\n");
}
stream.print(grammar().genImportsList());
stream.println(docComment());
stream.println(typeDeclarationString());
emitMembers(stream);
emitAbstractSyns(stream);
emitInhDeclarations(stream);
stream.println("}");
stream.close();
} catch (FileNotFoundException f) {
System.err.println("Could not create file " + file.getName() + " in " + file.getParent());
System.exit(1);
}
}
/**
* @declaredat ASTNode:1
*/
public InterfaceDecl(int i) {
super(i);
}
/**
* @declaredat ASTNode:5
*/
public InterfaceDecl(Ast p, int i) {
this(i);
parser = p;
}
/**
* @declaredat ASTNode:10
*/
public InterfaceDecl() {
this(0);
}
/**
* Initializes the child array to the correct size.
* Initializes List and Opt nta children.
* @apilevel internal
* @ast method
* @declaredat ASTNode:19
*/
public void init$Children() {
children = new ASTNode[8];
setChild(new List(), 1);
setChild(new List(), 2);
setChild(new List(), 3);
setChild(new List(), 4);
setChild(new List(), 5);
setChild(new List(), 6);
setChild(new List(), 7);
}
/**
* @declaredat ASTNode:29
*/
public InterfaceDecl(IdDecl p0, List p1, List p2, List p3, List p4, List p5, List p6, List p7, String p8, int p9, int p10, String p11, String p12) {
setChild(p0, 0);
setChild(p1, 1);
setChild(p2, 2);
setChild(p3, 3);
setChild(p4, 4);
setChild(p5, 5);
setChild(p6, 6);
setChild(p7, 7);
setFileName(p8);
setStartLine(p9);
setEndLine(p10);
setComment(p11);
setAspectName(p12);
}
/**
* @declaredat ASTNode:44
*/
public void dumpTree(String indent, java.io.PrintStream out) {
out.print(indent + "InterfaceDecl");
out.print("\"" + getFileName() + "\"");
out.print("\"" + getStartLine() + "\"");
out.print("\"" + getEndLine() + "\"");
out.print("\"" + getComment() + "\"");
out.print("\"" + getAspectName() + "\"");
String childIndent = indent + " ";
for (int i = 0; i < getNumChild(); i++) {
getChild(i).dumpTree(childIndent, out);
}
}
/**
* @declaredat ASTNode:56
*/
public Object jjtAccept(AstVisitor visitor, Object data) {
return visitor.visit(this, data);
}
/**
* @declaredat ASTNode:59
*/
public void jjtAddChild(Node n, int i) {
checkChild(n, i);
super.jjtAddChild(n, i);
}
/**
* @declaredat ASTNode:63
*/
public void checkChild(Node n, int i) {
if (i == 0 && !(n instanceof IdDecl)) {
throw new Error("Child number 0 of TypeDecl has the type " +
n.getClass().getName() + " which is not an instance of IdDecl");
}
if (i == 1) {
if (!(n instanceof List)) {
throw new Error("Child number 1 of TypeDecl has the type " +
n.getClass().getName() + " which is not an instance of List");
}
for (int k = 0; k < ((List) n).getNumChildNoTransform(); k++) {
if (!(((List) n).getChildNoTransform(k) instanceof ClassBodyDecl)) {
throw new Error("Child number " + k + " in ClassBodyDeclList has the type " +
((List) n).getChildNoTransform(k).getClass().getName() + " which is not an instance of ClassBodyDecl");
}
}
}
if (i == 2) {
if (!(n instanceof List)) {
throw new Error("Child number 2 of TypeDecl has the type " +
n.getClass().getName() + " which is not an instance of List");
}
for (int k = 0; k < ((List) n).getNumChildNoTransform(); k++) {
if (!(((List) n).getChildNoTransform(k) instanceof SynDecl)) {
throw new Error("Child number " + k + " in SynDeclList has the type " +
((List) n).getChildNoTransform(k).getClass().getName() + " which is not an instance of SynDecl");
}
}
}
if (i == 3) {
if (!(n instanceof List)) {
throw new Error("Child number 3 of TypeDecl has the type " +
n.getClass().getName() + " which is not an instance of List");
}
for (int k = 0; k < ((List) n).getNumChildNoTransform(); k++) {
if (!(((List) n).getChildNoTransform(k) instanceof SynEq)) {
throw new Error("Child number " + k + " in SynEqList has the type " +
((List) n).getChildNoTransform(k).getClass().getName() + " which is not an instance of SynEq");
}
}
}
if (i == 4) {
if (!(n instanceof List)) {
throw new Error("Child number 4 of TypeDecl has the type " +
n.getClass().getName() + " which is not an instance of List");
}
for (int k = 0; k < ((List) n).getNumChildNoTransform(); k++) {
if (!(((List) n).getChildNoTransform(k) instanceof InhDecl)) {
throw new Error("Child number " + k + " in InhDeclList has the type " +
((List) n).getChildNoTransform(k).getClass().getName() + " which is not an instance of InhDecl");
}
}
}
if (i == 5) {
if (!(n instanceof List)) {
throw new Error("Child number 5 of TypeDecl has the type " +
n.getClass().getName() + " which is not an instance of List");
}
for (int k = 0; k < ((List) n).getNumChildNoTransform(); k++) {
if (!(((List) n).getChildNoTransform(k) instanceof InhEq)) {
throw new Error("Child number " + k + " in InhEqList has the type " +
((List) n).getChildNoTransform(k).getClass().getName() + " which is not an instance of InhEq");
}
}
}
if (i == 6) {
if (!(n instanceof List)) {
throw new Error("Child number 6 of TypeDecl has the type " +
n.getClass().getName() + " which is not an instance of List");
}
for (int k = 0; k < ((List) n).getNumChildNoTransform(); k++) {
if (!(((List) n).getChildNoTransform(k) instanceof Component)) {
throw new Error("Child number " + k + " in ComponentList has the type " +
((List) n).getChildNoTransform(k).getClass().getName() + " which is not an instance of Component");
}
}
}
if (i == 7) {
if (!(n instanceof List)) {
throw new Error("Child number 7 of TypeDecl has the type " +
n.getClass().getName() + " which is not an instance of List");
}
for (int k = 0; k < ((List) n).getNumChildNoTransform(); k++) {
if (!(((List) n).getChildNoTransform(k) instanceof CollDecl)) {
throw new Error("Child number " + k + " in CollDeclList has the type " +
((List) n).getChildNoTransform(k).getClass().getName() + " which is not an instance of CollDecl");
}
}
}
}
/** @apilevel low-level
* @declaredat ASTNode:154
*/
public int getNumChild() {
return 8;
}
/**
* @apilevel internal
* @declaredat ASTNode:160
*/
public boolean mayHaveRewrite() {
return false;
}
/** @apilevel internal
* @declaredat ASTNode:164
*/
public void flushAttrCache() {
super.flushAttrCache();
}
/** @apilevel internal
* @declaredat ASTNode:168
*/
public void flushCollectionCache() {
super.flushCollectionCache();
}
/** @apilevel internal
* @declaredat ASTNode:172
*/
public void flushRewriteCache() {
super.flushRewriteCache();
}
/** @apilevel internal
* @declaredat ASTNode:176
*/
public InterfaceDecl clone() throws CloneNotSupportedException {
InterfaceDecl node = (InterfaceDecl) super.clone();
return node;
}
/** @apilevel internal
* @declaredat ASTNode:181
*/
public InterfaceDecl copy() {
try {
InterfaceDecl node = (InterfaceDecl) clone();
node.parent = null;
if (children != null) {
node.children = (ASTNode[]) children.clone();
}
return node;
} catch (CloneNotSupportedException e) {
throw new Error("Error: clone not supported for " + getClass().getName());
}
}
/**
* Create a deep copy of the AST subtree at this node.
* The copy is dangling, i.e. has no parent.
* @return dangling copy of the subtree at this node
* @apilevel low-level
* @deprecated Please use treeCopy or treeCopyNoTransform instead
* @declaredat ASTNode:200
*/
@Deprecated
public InterfaceDecl fullCopy() {
return treeCopyNoTransform();
}
/**
* Create a deep copy of the AST subtree at this node.
* The copy is dangling, i.e. has no parent.
* @return dangling copy of the subtree at this node
* @apilevel low-level
* @declaredat ASTNode:210
*/
public InterfaceDecl treeCopyNoTransform() {
InterfaceDecl tree = (InterfaceDecl) copy();
if (children != null) {
for (int i = 0; i < children.length; ++i) {
ASTNode child = (ASTNode) children[i];
if (child != null) {
child = child.treeCopyNoTransform();
tree.setChild(child, i);
}
}
}
return tree;
}
/**
* Create a deep copy of the AST subtree at this node.
* The subtree of this node is traversed to trigger rewrites before copy.
* The copy is dangling, i.e. has no parent.
* @return dangling copy of the subtree at this node
* @apilevel low-level
* @declaredat ASTNode:230
*/
public InterfaceDecl treeCopy() {
doFullTraversal();
return treeCopyNoTransform();
}
/** @apilevel internal
* @declaredat ASTNode:235
*/
protected boolean is$Equal(ASTNode node) {
return super.is$Equal(node) && (tokenString_FileName == ((InterfaceDecl) node).tokenString_FileName) && (tokenint_StartLine == ((InterfaceDecl) node).tokenint_StartLine) && (tokenint_EndLine == ((InterfaceDecl) node).tokenint_EndLine) && (tokenString_Comment == ((InterfaceDecl) node).tokenString_Comment) && (tokenString_AspectName == ((InterfaceDecl) node).tokenString_AspectName);
}
/**
* Replaces the IdDecl child.
* @param node The new node to replace the IdDecl child.
* @apilevel high-level
*/
public void setIdDecl(IdDecl node) {
setChild(node, 0);
}
/**
* Retrieves the IdDecl child.
* @return The current node used as the IdDecl child.
* @apilevel high-level
*/
@ASTNodeAnnotation.Child(name="IdDecl")
public IdDecl getIdDecl() {
return (IdDecl) getChild(0);
}
/**
* Retrieves the IdDecl child.
* This method does not invoke AST transformations.
* @return The current node used as the IdDecl child.
* @apilevel low-level
*/
public IdDecl getIdDeclNoTransform() {
return (IdDecl) getChildNoTransform(0);
}
/**
* Replaces the ClassBodyDecl list.
* @param list The new list node to be used as the ClassBodyDecl list.
* @apilevel high-level
*/
public void setClassBodyDeclList(List list) {
setChild(list, 1);
}
/**
* Retrieves the number of children in the ClassBodyDecl list.
* @return Number of children in the ClassBodyDecl list.
* @apilevel high-level
*/
public int getNumClassBodyDecl() {
return getClassBodyDeclList().getNumChild();
}
/**
* Retrieves the number of children in the ClassBodyDecl list.
* Calling this method will not trigger rewrites.
* @return Number of children in the ClassBodyDecl list.
* @apilevel low-level
*/
public int getNumClassBodyDeclNoTransform() {
return getClassBodyDeclListNoTransform().getNumChildNoTransform();
}
/**
* Retrieves the element at index {@code i} in the ClassBodyDecl list.
* @param i Index of the element to return.
* @return The element at position {@code i} in the ClassBodyDecl list.
* @apilevel high-level
*/
public ClassBodyDecl getClassBodyDecl(int i) {
return (ClassBodyDecl) getClassBodyDeclList().getChild(i);
}
/**
* Check whether the ClassBodyDecl list has any children.
* @return {@code true} if it has at least one child, {@code false} otherwise.
* @apilevel high-level
*/
public boolean hasClassBodyDecl() {
return getClassBodyDeclList().getNumChild() != 0;
}
/**
* Append an element to the ClassBodyDecl list.
* @param node The element to append to the ClassBodyDecl list.
* @apilevel high-level
*/
public void addClassBodyDecl(ClassBodyDecl node) {
List list = (parent == null) ? getClassBodyDeclListNoTransform() : getClassBodyDeclList();
list.addChild(node);
}
/** @apilevel low-level
*/
public void addClassBodyDeclNoTransform(ClassBodyDecl node) {
List list = getClassBodyDeclListNoTransform();
list.addChild(node);
}
/**
* Replaces the ClassBodyDecl list element at index {@code i} with the new node {@code node}.
* @param node The new node to replace the old list element.
* @param i The list index of the node to be replaced.
* @apilevel high-level
*/
public void setClassBodyDecl(ClassBodyDecl node, int i) {
List list = getClassBodyDeclList();
list.setChild(node, i);
}
/**
* Retrieves the ClassBodyDecl list.
* @return The node representing the ClassBodyDecl list.
* @apilevel high-level
*/
@ASTNodeAnnotation.ListChild(name="ClassBodyDecl")
public List getClassBodyDeclList() {
List list = (List) getChild(1);
return list;
}
/**
* Retrieves the ClassBodyDecl list.
* This method does not invoke AST transformations.
* @return The node representing the ClassBodyDecl list.
* @apilevel low-level
*/
public List getClassBodyDeclListNoTransform() {
return (List) getChildNoTransform(1);
}
/**
* Retrieves the ClassBodyDecl list.
* @return The node representing the ClassBodyDecl list.
* @apilevel high-level
*/
public List getClassBodyDecls() {
return getClassBodyDeclList();
}
/**
* Retrieves the ClassBodyDecl list.
* This method does not invoke AST transformations.
* @return The node representing the ClassBodyDecl list.
* @apilevel low-level
*/
public List getClassBodyDeclsNoTransform() {
return getClassBodyDeclListNoTransform();
}
/**
* Replaces the SynDecl list.
* @param list The new list node to be used as the SynDecl list.
* @apilevel high-level
*/
public void setSynDeclList(List list) {
setChild(list, 2);
}
/**
* Retrieves the number of children in the SynDecl list.
* @return Number of children in the SynDecl list.
* @apilevel high-level
*/
public int getNumSynDecl() {
return getSynDeclList().getNumChild();
}
/**
* Retrieves the number of children in the SynDecl list.
* Calling this method will not trigger rewrites.
* @return Number of children in the SynDecl list.
* @apilevel low-level
*/
public int getNumSynDeclNoTransform() {
return getSynDeclListNoTransform().getNumChildNoTransform();
}
/**
* Retrieves the element at index {@code i} in the SynDecl list.
* @param i Index of the element to return.
* @return The element at position {@code i} in the SynDecl list.
* @apilevel high-level
*/
public SynDecl getSynDecl(int i) {
return (SynDecl) getSynDeclList().getChild(i);
}
/**
* Check whether the SynDecl list has any children.
* @return {@code true} if it has at least one child, {@code false} otherwise.
* @apilevel high-level
*/
public boolean hasSynDecl() {
return getSynDeclList().getNumChild() != 0;
}
/**
* Append an element to the SynDecl list.
* @param node The element to append to the SynDecl list.
* @apilevel high-level
*/
public void addSynDecl(SynDecl node) {
List list = (parent == null) ? getSynDeclListNoTransform() : getSynDeclList();
list.addChild(node);
}
/** @apilevel low-level
*/
public void addSynDeclNoTransform(SynDecl node) {
List list = getSynDeclListNoTransform();
list.addChild(node);
}
/**
* Replaces the SynDecl list element at index {@code i} with the new node {@code node}.
* @param node The new node to replace the old list element.
* @param i The list index of the node to be replaced.
* @apilevel high-level
*/
public void setSynDecl(SynDecl node, int i) {
List list = getSynDeclList();
list.setChild(node, i);
}
/**
* Retrieves the SynDecl list.
* @return The node representing the SynDecl list.
* @apilevel high-level
*/
@ASTNodeAnnotation.ListChild(name="SynDecl")
public List getSynDeclList() {
List list = (List) getChild(2);
return list;
}
/**
* Retrieves the SynDecl list.
* This method does not invoke AST transformations.
* @return The node representing the SynDecl list.
* @apilevel low-level
*/
public List getSynDeclListNoTransform() {
return (List) getChildNoTransform(2);
}
/**
* Retrieves the SynDecl list.
* @return The node representing the SynDecl list.
* @apilevel high-level
*/
public List getSynDecls() {
return getSynDeclList();
}
/**
* Retrieves the SynDecl list.
* This method does not invoke AST transformations.
* @return The node representing the SynDecl list.
* @apilevel low-level
*/
public List getSynDeclsNoTransform() {
return getSynDeclListNoTransform();
}
/**
* Replaces the SynEq list.
* @param list The new list node to be used as the SynEq list.
* @apilevel high-level
*/
public void setSynEqList(List list) {
setChild(list, 3);
}
/**
* Retrieves the number of children in the SynEq list.
* @return Number of children in the SynEq list.
* @apilevel high-level
*/
public int getNumSynEq() {
return getSynEqList().getNumChild();
}
/**
* Retrieves the number of children in the SynEq list.
* Calling this method will not trigger rewrites.
* @return Number of children in the SynEq list.
* @apilevel low-level
*/
public int getNumSynEqNoTransform() {
return getSynEqListNoTransform().getNumChildNoTransform();
}
/**
* Retrieves the element at index {@code i} in the SynEq list.
* @param i Index of the element to return.
* @return The element at position {@code i} in the SynEq list.
* @apilevel high-level
*/
public SynEq getSynEq(int i) {
return (SynEq) getSynEqList().getChild(i);
}
/**
* Check whether the SynEq list has any children.
* @return {@code true} if it has at least one child, {@code false} otherwise.
* @apilevel high-level
*/
public boolean hasSynEq() {
return getSynEqList().getNumChild() != 0;
}
/**
* Append an element to the SynEq list.
* @param node The element to append to the SynEq list.
* @apilevel high-level
*/
public void addSynEq(SynEq node) {
List list = (parent == null) ? getSynEqListNoTransform() : getSynEqList();
list.addChild(node);
}
/** @apilevel low-level
*/
public void addSynEqNoTransform(SynEq node) {
List list = getSynEqListNoTransform();
list.addChild(node);
}
/**
* Replaces the SynEq list element at index {@code i} with the new node {@code node}.
* @param node The new node to replace the old list element.
* @param i The list index of the node to be replaced.
* @apilevel high-level
*/
public void setSynEq(SynEq node, int i) {
List list = getSynEqList();
list.setChild(node, i);
}
/**
* Retrieves the SynEq list.
* @return The node representing the SynEq list.
* @apilevel high-level
*/
@ASTNodeAnnotation.ListChild(name="SynEq")
public List getSynEqList() {
List list = (List) getChild(3);
return list;
}
/**
* Retrieves the SynEq list.
* This method does not invoke AST transformations.
* @return The node representing the SynEq list.
* @apilevel low-level
*/
public List getSynEqListNoTransform() {
return (List) getChildNoTransform(3);
}
/**
* Retrieves the SynEq list.
* @return The node representing the SynEq list.
* @apilevel high-level
*/
public List getSynEqs() {
return getSynEqList();
}
/**
* Retrieves the SynEq list.
* This method does not invoke AST transformations.
* @return The node representing the SynEq list.
* @apilevel low-level
*/
public List getSynEqsNoTransform() {
return getSynEqListNoTransform();
}
/**
* Replaces the InhDecl list.
* @param list The new list node to be used as the InhDecl list.
* @apilevel high-level
*/
public void setInhDeclList(List list) {
setChild(list, 4);
}
/**
* Retrieves the number of children in the InhDecl list.
* @return Number of children in the InhDecl list.
* @apilevel high-level
*/
public int getNumInhDecl() {
return getInhDeclList().getNumChild();
}
/**
* Retrieves the number of children in the InhDecl list.
* Calling this method will not trigger rewrites.
* @return Number of children in the InhDecl list.
* @apilevel low-level
*/
public int getNumInhDeclNoTransform() {
return getInhDeclListNoTransform().getNumChildNoTransform();
}
/**
* Retrieves the element at index {@code i} in the InhDecl list.
* @param i Index of the element to return.
* @return The element at position {@code i} in the InhDecl list.
* @apilevel high-level
*/
public InhDecl getInhDecl(int i) {
return (InhDecl) getInhDeclList().getChild(i);
}
/**
* Check whether the InhDecl list has any children.
* @return {@code true} if it has at least one child, {@code false} otherwise.
* @apilevel high-level
*/
public boolean hasInhDecl() {
return getInhDeclList().getNumChild() != 0;
}
/**
* Append an element to the InhDecl list.
* @param node The element to append to the InhDecl list.
* @apilevel high-level
*/
public void addInhDecl(InhDecl node) {
List list = (parent == null) ? getInhDeclListNoTransform() : getInhDeclList();
list.addChild(node);
}
/** @apilevel low-level
*/
public void addInhDeclNoTransform(InhDecl node) {
List list = getInhDeclListNoTransform();
list.addChild(node);
}
/**
* Replaces the InhDecl list element at index {@code i} with the new node {@code node}.
* @param node The new node to replace the old list element.
* @param i The list index of the node to be replaced.
* @apilevel high-level
*/
public void setInhDecl(InhDecl node, int i) {
List list = getInhDeclList();
list.setChild(node, i);
}
/**
* Retrieves the InhDecl list.
* @return The node representing the InhDecl list.
* @apilevel high-level
*/
@ASTNodeAnnotation.ListChild(name="InhDecl")
public List getInhDeclList() {
List list = (List) getChild(4);
return list;
}
/**
* Retrieves the InhDecl list.
* This method does not invoke AST transformations.
* @return The node representing the InhDecl list.
* @apilevel low-level
*/
public List getInhDeclListNoTransform() {
return (List) getChildNoTransform(4);
}
/**
* Retrieves the InhDecl list.
* @return The node representing the InhDecl list.
* @apilevel high-level
*/
public List getInhDecls() {
return getInhDeclList();
}
/**
* Retrieves the InhDecl list.
* This method does not invoke AST transformations.
* @return The node representing the InhDecl list.
* @apilevel low-level
*/
public List getInhDeclsNoTransform() {
return getInhDeclListNoTransform();
}
/**
* Replaces the InhEq list.
* @param list The new list node to be used as the InhEq list.
* @apilevel high-level
*/
public void setInhEqList(List list) {
setChild(list, 5);
}
/**
* Retrieves the number of children in the InhEq list.
* @return Number of children in the InhEq list.
* @apilevel high-level
*/
public int getNumInhEq() {
return getInhEqList().getNumChild();
}
/**
* Retrieves the number of children in the InhEq list.
* Calling this method will not trigger rewrites.
* @return Number of children in the InhEq list.
* @apilevel low-level
*/
public int getNumInhEqNoTransform() {
return getInhEqListNoTransform().getNumChildNoTransform();
}
/**
* Retrieves the element at index {@code i} in the InhEq list.
* @param i Index of the element to return.
* @return The element at position {@code i} in the InhEq list.
* @apilevel high-level
*/
public InhEq getInhEq(int i) {
return (InhEq) getInhEqList().getChild(i);
}
/**
* Check whether the InhEq list has any children.
* @return {@code true} if it has at least one child, {@code false} otherwise.
* @apilevel high-level
*/
public boolean hasInhEq() {
return getInhEqList().getNumChild() != 0;
}
/**
* Append an element to the InhEq list.
* @param node The element to append to the InhEq list.
* @apilevel high-level
*/
public void addInhEq(InhEq node) {
List list = (parent == null) ? getInhEqListNoTransform() : getInhEqList();
list.addChild(node);
}
/** @apilevel low-level
*/
public void addInhEqNoTransform(InhEq node) {
List list = getInhEqListNoTransform();
list.addChild(node);
}
/**
* Replaces the InhEq list element at index {@code i} with the new node {@code node}.
* @param node The new node to replace the old list element.
* @param i The list index of the node to be replaced.
* @apilevel high-level
*/
public void setInhEq(InhEq node, int i) {
List list = getInhEqList();
list.setChild(node, i);
}
/**
* Retrieves the InhEq list.
* @return The node representing the InhEq list.
* @apilevel high-level
*/
@ASTNodeAnnotation.ListChild(name="InhEq")
public List getInhEqList() {
List list = (List) getChild(5);
return list;
}
/**
* Retrieves the InhEq list.
* This method does not invoke AST transformations.
* @return The node representing the InhEq list.
* @apilevel low-level
*/
public List getInhEqListNoTransform() {
return (List) getChildNoTransform(5);
}
/**
* Retrieves the InhEq list.
* @return The node representing the InhEq list.
* @apilevel high-level
*/
public List getInhEqs() {
return getInhEqList();
}
/**
* Retrieves the InhEq list.
* This method does not invoke AST transformations.
* @return The node representing the InhEq list.
* @apilevel low-level
*/
public List getInhEqsNoTransform() {
return getInhEqListNoTransform();
}
/**
* Replaces the Component list.
* @param list The new list node to be used as the Component list.
* @apilevel high-level
*/
public void setComponentList(List list) {
setChild(list, 6);
}
/**
* Retrieves the number of children in the Component list.
* @return Number of children in the Component list.
* @apilevel high-level
*/
public int getNumComponent() {
return getComponentList().getNumChild();
}
/**
* Retrieves the number of children in the Component list.
* Calling this method will not trigger rewrites.
* @return Number of children in the Component list.
* @apilevel low-level
*/
public int getNumComponentNoTransform() {
return getComponentListNoTransform().getNumChildNoTransform();
}
/**
* Retrieves the element at index {@code i} in the Component list.
* @param i Index of the element to return.
* @return The element at position {@code i} in the Component list.
* @apilevel high-level
*/
public Component getComponent(int i) {
return (Component) getComponentList().getChild(i);
}
/**
* Check whether the Component list has any children.
* @return {@code true} if it has at least one child, {@code false} otherwise.
* @apilevel high-level
*/
public boolean hasComponent() {
return getComponentList().getNumChild() != 0;
}
/**
* Append an element to the Component list.
* @param node The element to append to the Component list.
* @apilevel high-level
*/
public void addComponent(Component node) {
List list = (parent == null) ? getComponentListNoTransform() : getComponentList();
list.addChild(node);
}
/** @apilevel low-level
*/
public void addComponentNoTransform(Component node) {
List list = getComponentListNoTransform();
list.addChild(node);
}
/**
* Replaces the Component list element at index {@code i} with the new node {@code node}.
* @param node The new node to replace the old list element.
* @param i The list index of the node to be replaced.
* @apilevel high-level
*/
public void setComponent(Component node, int i) {
List list = getComponentList();
list.setChild(node, i);
}
/**
* Retrieves the Component list.
* @return The node representing the Component list.
* @apilevel high-level
*/
@ASTNodeAnnotation.ListChild(name="Component")
public List getComponentList() {
List list = (List) getChild(6);
return list;
}
/**
* Retrieves the Component list.
* This method does not invoke AST transformations.
* @return The node representing the Component list.
* @apilevel low-level
*/
public List getComponentListNoTransform() {
return (List) getChildNoTransform(6);
}
/**
* Retrieves the Component list.
* @return The node representing the Component list.
* @apilevel high-level
*/
public List getComponents() {
return getComponentList();
}
/**
* Retrieves the Component list.
* This method does not invoke AST transformations.
* @return The node representing the Component list.
* @apilevel low-level
*/
public List getComponentsNoTransform() {
return getComponentListNoTransform();
}
/**
* Replaces the CollDecl list.
* @param list The new list node to be used as the CollDecl list.
* @apilevel high-level
*/
public void setCollDeclList(List list) {
setChild(list, 7);
}
/**
* Retrieves the number of children in the CollDecl list.
* @return Number of children in the CollDecl list.
* @apilevel high-level
*/
public int getNumCollDecl() {
return getCollDeclList().getNumChild();
}
/**
* Retrieves the number of children in the CollDecl list.
* Calling this method will not trigger rewrites.
* @return Number of children in the CollDecl list.
* @apilevel low-level
*/
public int getNumCollDeclNoTransform() {
return getCollDeclListNoTransform().getNumChildNoTransform();
}
/**
* Retrieves the element at index {@code i} in the CollDecl list.
* @param i Index of the element to return.
* @return The element at position {@code i} in the CollDecl list.
* @apilevel high-level
*/
public CollDecl getCollDecl(int i) {
return (CollDecl) getCollDeclList().getChild(i);
}
/**
* Check whether the CollDecl list has any children.
* @return {@code true} if it has at least one child, {@code false} otherwise.
* @apilevel high-level
*/
public boolean hasCollDecl() {
return getCollDeclList().getNumChild() != 0;
}
/**
* Append an element to the CollDecl list.
* @param node The element to append to the CollDecl list.
* @apilevel high-level
*/
public void addCollDecl(CollDecl node) {
List list = (parent == null) ? getCollDeclListNoTransform() : getCollDeclList();
list.addChild(node);
}
/** @apilevel low-level
*/
public void addCollDeclNoTransform(CollDecl node) {
List list = getCollDeclListNoTransform();
list.addChild(node);
}
/**
* Replaces the CollDecl list element at index {@code i} with the new node {@code node}.
* @param node The new node to replace the old list element.
* @param i The list index of the node to be replaced.
* @apilevel high-level
*/
public void setCollDecl(CollDecl node, int i) {
List list = getCollDeclList();
list.setChild(node, i);
}
/**
* Retrieves the CollDecl list.
* @return The node representing the CollDecl list.
* @apilevel high-level
*/
@ASTNodeAnnotation.ListChild(name="CollDecl")
public List getCollDeclList() {
List list = (List) getChild(7);
return list;
}
/**
* Retrieves the CollDecl list.
* This method does not invoke AST transformations.
* @return The node representing the CollDecl list.
* @apilevel low-level
*/
public List getCollDeclListNoTransform() {
return (List) getChildNoTransform(7);
}
/**
* Retrieves the CollDecl list.
* @return The node representing the CollDecl list.
* @apilevel high-level
*/
public List getCollDecls() {
return getCollDeclList();
}
/**
* Retrieves the CollDecl list.
* This method does not invoke AST transformations.
* @return The node representing the CollDecl list.
* @apilevel low-level
*/
public List getCollDeclsNoTransform() {
return getCollDeclListNoTransform();
}
/**
* Replaces the lexeme FileName.
* @param value The new value for the lexeme FileName.
* @apilevel high-level
*/
public void setFileName(String value) {
tokenString_FileName = value;
}
/**
* Retrieves the value for the lexeme FileName.
* @return The value for the lexeme FileName.
* @apilevel high-level
*/
@ASTNodeAnnotation.Token(name="FileName")
public String getFileName() {
return tokenString_FileName != null ? tokenString_FileName : "";
}
/**
* Replaces the lexeme StartLine.
* @param value The new value for the lexeme StartLine.
* @apilevel high-level
*/
public void setStartLine(int value) {
tokenint_StartLine = value;
}
/**
* Retrieves the value for the lexeme StartLine.
* @return The value for the lexeme StartLine.
* @apilevel high-level
*/
@ASTNodeAnnotation.Token(name="StartLine")
public int getStartLine() {
return tokenint_StartLine;
}
/**
* Replaces the lexeme EndLine.
* @param value The new value for the lexeme EndLine.
* @apilevel high-level
*/
public void setEndLine(int value) {
tokenint_EndLine = value;
}
/**
* Retrieves the value for the lexeme EndLine.
* @return The value for the lexeme EndLine.
* @apilevel high-level
*/
@ASTNodeAnnotation.Token(name="EndLine")
public int getEndLine() {
return tokenint_EndLine;
}
/**
* Replaces the lexeme Comment.
* @param value The new value for the lexeme Comment.
* @apilevel high-level
*/
public void setComment(String value) {
tokenString_Comment = value;
}
/**
* Retrieves the value for the lexeme Comment.
* @return The value for the lexeme Comment.
* @apilevel high-level
*/
@ASTNodeAnnotation.Token(name="Comment")
public String getComment() {
return tokenString_Comment != null ? tokenString_Comment : "";
}
/**
* Replaces the lexeme AspectName.
* @param value The new value for the lexeme AspectName.
* @apilevel high-level
*/
public void setAspectName(String value) {
tokenString_AspectName = value;
}
/**
* Retrieves the value for the lexeme AspectName.
* @return The value for the lexeme AspectName.
* @apilevel high-level
*/
@ASTNodeAnnotation.Token(name="AspectName")
public String getAspectName() {
return tokenString_AspectName != null ? tokenString_AspectName : "";
}
/** @apilevel internal */
protected int typeDeclKind_visited = -1;
/**
* @attribute syn
* @aspect Comments
* @declaredat /home/jesper/git/jastadd2/src/jastadd/ast/Comments.jrag:163
*/
@ASTNodeAnnotation.Attribute(kind=ASTNodeAnnotation.Kind.SYN)
@ASTNodeAnnotation.Source(aspect="Comments", declaredAt="/home/jesper/git/jastadd2/src/jastadd/ast/Comments.jrag:163")
public String typeDeclKind() {
if (typeDeclKind_visited == state().boundariesCrossed) {
throw new RuntimeException("Circular definition of attribute TypeDecl.typeDeclKind().");
}
typeDeclKind_visited = state().boundariesCrossed;
String typeDeclKind_value = "interface";
typeDeclKind_visited = -1;
return typeDeclKind_value;
}
/** @apilevel internal */
protected int attributeProblems_visited = -1;
/**
* Hack to make collection only on ASTdecls that are not
* ClassDecl or InterfaceDecl.
* @attribute syn
* @aspect AttributeProblems
* @declaredat /home/jesper/git/jastadd2/src/jastadd/ast/AttributeProblems.jrag:107
*/
@ASTNodeAnnotation.Attribute(kind=ASTNodeAnnotation.Kind.SYN)
@ASTNodeAnnotation.Source(aspect="AttributeProblems", declaredAt="/home/jesper/git/jastadd2/src/jastadd/ast/AttributeProblems.jrag:107")
public Collection attributeProblems() {
if (attributeProblems_visited == state().boundariesCrossed) {
throw new RuntimeException("Circular definition of attribute InterfaceDecl.attributeProblems().");
}
attributeProblems_visited = state().boundariesCrossed;
Collection attributeProblems_value = Collections.emptyList();
attributeProblems_visited = -1;
return attributeProblems_value;
}
/** @apilevel internal */
protected java.util.Map missingSynEqs_String_visited = new java.util.HashMap(4);
/**
* @param signature the signature of the attribute
* @return the subclasses of this AST class that are missing an
* equation for for the synthesized attribute
* @attribute syn
* @aspect AttributeProblems
* @declaredat /home/jesper/git/jastadd2/src/jastadd/ast/AttributeProblems.jrag:248
*/
@ASTNodeAnnotation.Attribute(kind=ASTNodeAnnotation.Kind.SYN)
@ASTNodeAnnotation.Source(aspect="AttributeProblems", declaredAt="/home/jesper/git/jastadd2/src/jastadd/ast/AttributeProblems.jrag:248")
public Collection extends TypeDecl> missingSynEqs(String signature) {
Object _parameters = signature;
if (Integer.valueOf(state().boundariesCrossed).equals(missingSynEqs_String_visited.get(_parameters))) {
throw new RuntimeException("Circular definition of attribute TypeDecl.missingSynEqs(String).");
}
missingSynEqs_String_visited.put(_parameters, Integer.valueOf(state().boundariesCrossed));
Collection extends TypeDecl> missingSynEqs_String_value = Collections.emptyList();
missingSynEqs_String_visited.remove(_parameters);
return missingSynEqs_String_value;
}
/** @apilevel internal */
public ASTNode rewriteTo() {
return super.rewriteTo();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy