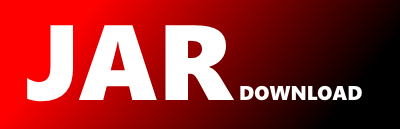
org.jasypt.hibernate.encryptor.HibernatePBEBigDecimalEncryptor Maven / Gradle / Ivy
/*
* =============================================================================
*
* Copyright (c) 2007-2010, The JASYPT team (http://www.jasypt.org)
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
* =============================================================================
*/
package org.jasypt.hibernate.encryptor;
import java.math.BigDecimal;
import org.jasypt.encryption.pbe.PBEBigDecimalEncryptor;
import org.jasypt.encryption.pbe.StandardPBEBigDecimalEncryptor;
import org.jasypt.encryption.pbe.config.PBEConfig;
import org.jasypt.exceptions.EncryptionInitializationException;
import org.jasypt.salt.SaltGenerator;
/**
*
* Placeholder class for PBEBigDecimalEncryptor objects which are
* eligible for use from Hibernate.
*
*
* This class acts as a wrapper on a PBEBigDecimalEncryptor, allowing
* to be set a registered name (see {@link #setRegisteredName(String)})
* and performing the needed registry operations against the
* {@link HibernatePBEEncryptorRegistry}.
*
*
* It is not mandatory that a PBEBigDecimalEncryptor be explicitly set
* with {@link #setEncryptor(PBEBigDecimalEncryptor)}. If not, a
* StandardPBEBigDecimalEncryptor object will be created internally
* and it will be configurable with the
* {@link #setPassword(String)}/{@link #setPasswordCharArray(char[])},
* {@link #setAlgorithm(String)}, {@link #setKeyObtentionIterations(int)},
* {@link #setSaltGenerator(SaltGenerator)}
* and {@link #setConfig(PBEConfig)} methods.
*
*
* This class is mainly intended for use from Spring Framework or some other
* IoC container (if you are not using a container of this kind, please see
* {@link HibernatePBEEncryptorRegistry}). The steps to be performed are
* the following:
*
* - Create an object of this class (declaring it).
* - Set its registeredName and, either its
* wrapped encryptor or its password,
* algorithm, keyObtentionIterations,
* saltGenerator and config properties.
* - Declare a typedef in a Hibernate mapping giving its
* encryptorRegisteredName parameter the same value specified
* to this object in registeredName.
*
*
*
* This in a Spring config file would look like:
*
*
*
* ...
* <-- Optional, as the hibernateEncryptor could be directly set an -->
* <-- algorithm and password. -->
* <bean id="bigDecimalEncryptor"
* class="org.jasypt.encryption.pbe.StandardPBEBigDecimalEncryptor">
* <property name="algorithm">
* <value>PBEWithMD5AndDES</value>
* </property>
* <property name="password">
* <value>XXXXX</value>
* </property>
* </bean>
*
* <bean id="hibernateEncryptor"
* class="org.jasypt.hibernate.encryptor.HibernatePBEBigDecimalEncryptor">
* <property name="registeredName">
* <value>myHibernateBigDecimalEncryptor</value>
* </property>
* <property name="encryptor">
* <ref bean="bigDecimalEncryptor" />
* </property>
* </bean>
* ...
*
*
*
* And then in the Hibernate mapping file:
*
*
*
* <typedef name="encrypted" class="org.jasypt.hibernate.type.EncryptedBigDecimalType">
* <param name="encryptorRegisteredName">myHibernateBigDecimalEncryptor</param>
* <param name="decimalScale">2</param>
* </typedef>
*
*
*
* An important thing to note is that, when using HibernatePBEBigDecimalEncryptor
* objects this way to wrap PBEBigDecimalEncryptors, it is not
* necessary to deal with {@link HibernatePBEEncryptorRegistry},
* because HibernatePBEBigDecimalEncryptor objects get automatically registered
* in the encryptor registry when their {@link #setRegisteredName(String)}
* method is called.
*
*
* @since 1.2
*
* @author Daniel Fernández
*
* @deprecated Will be removed in 1.11. Package org.jasypt.hibernate.connectionprovider
* has been renamed as org.jasypt.hibernate3.connectionprovider.
*
*/
public final class HibernatePBEBigDecimalEncryptor {
private String registeredName = null;
private PBEBigDecimalEncryptor encryptor = null;
private boolean encryptorSet = false;
/**
* Creates a new instance of HibernatePBEBigDecimalEncryptor It also
* creates a StandardPBEBigDecimalEncryptor for internal use, which
* can be overriden by calling setEncryptor(...).
*/
public HibernatePBEBigDecimalEncryptor() {
super();
this.encryptor = new StandardPBEBigDecimalEncryptor();
this.encryptorSet = false;
}
/*
* For internal use only, by the Registry, when a PBEBigDecimalEncryptor
* is registered programmatically.
*/
HibernatePBEBigDecimalEncryptor(final String registeredName,
final PBEBigDecimalEncryptor encryptor) {
this.encryptor = encryptor;
this.registeredName = registeredName;
this.encryptorSet = true;
}
/**
* Returns the encryptor which this object wraps.
*
* @return the encryptor.
*/
public PBEBigDecimalEncryptor getEncryptor() {
return this.encryptor;
}
/**
* Sets the PBEBigDecimalEncryptor to be held (wrapped) by this
* object. This method is optional and can be only called once.
*
* @param encryptor the encryptor.
*/
public void setEncryptor(final PBEBigDecimalEncryptor encryptor) {
if (this.encryptorSet) {
throw new EncryptionInitializationException(
"An encryptor has been already set: no " +
"further configuration possible on hibernate wrapper");
}
this.encryptor = encryptor;
this.encryptorSet = true;
}
/**
* Sets the password to be used by the internal encryptor, if a specific
* encryptor has not been set with setEncryptor(...).
*
* @param password the password to be set for the internal encryptor
*/
public void setPassword(final String password) {
if (this.encryptorSet) {
throw new EncryptionInitializationException(
"An encryptor has been already set: no " +
"further configuration possible on hibernate wrapper");
}
final StandardPBEBigDecimalEncryptor standardPBEBigDecimalEncryptor =
(StandardPBEBigDecimalEncryptor) this.encryptor;
standardPBEBigDecimalEncryptor.setPassword(password);
}
/**
* Sets the password to be used by the internal encryptor (as a char[]), if a specific
* encryptor has not been set with setEncryptor(...).
*
* @since 1.8
* @param password the password to be set for the internal encryptor
*/
public void setPasswordCharArray(final char[] password) {
if (this.encryptorSet) {
throw new EncryptionInitializationException(
"An encryptor has been already set: no " +
"further configuration possible on hibernate wrapper");
}
final StandardPBEBigDecimalEncryptor standardPBEBigDecimalEncryptor =
(StandardPBEBigDecimalEncryptor) this.encryptor;
standardPBEBigDecimalEncryptor.setPasswordCharArray(password);
}
/**
* Sets the algorithm to be used by the internal encryptor, if a specific
* encryptor has not been set with setEncryptor(...).
*
* @param algorithm the algorithm to be set for the internal encryptor
*/
public void setAlgorithm(final String algorithm) {
if (this.encryptorSet) {
throw new EncryptionInitializationException(
"An encryptor has been already set: no " +
"further configuration possible on hibernate wrapper");
}
final StandardPBEBigDecimalEncryptor standardPBEBigDecimalEncryptor =
(StandardPBEBigDecimalEncryptor) this.encryptor;
standardPBEBigDecimalEncryptor.setAlgorithm(algorithm);
}
/**
* Sets the key obtention iterations to be used by the internal encryptor,
* if a specific encryptor has not been set with setEncryptor(...).
*
* @param keyObtentionIterations to be set for the internal encryptor
*/
public void setKeyObtentionIterations(final int keyObtentionIterations) {
if (this.encryptorSet) {
throw new EncryptionInitializationException(
"An encryptor has been already set: no " +
"further configuration possible on hibernate wrapper");
}
final StandardPBEBigDecimalEncryptor standardPBEBigDecimalEncryptor =
(StandardPBEBigDecimalEncryptor) this.encryptor;
standardPBEBigDecimalEncryptor.setKeyObtentionIterations(
keyObtentionIterations);
}
/**
* Sets the salt generator to be used by the internal encryptor,
* if a specific encryptor has not been set with setEncryptor(...).
*
* @param saltGenerator the salt generator to be set for the internal
* encryptor.
*/
public void setSaltGenerator(final SaltGenerator saltGenerator) {
if (this.encryptorSet) {
throw new EncryptionInitializationException(
"An encryptor has been already set: no " +
"further configuration possible on hibernate wrapper");
}
final StandardPBEBigDecimalEncryptor standardPBEBigDecimalEncryptor =
(StandardPBEBigDecimalEncryptor) this.encryptor;
standardPBEBigDecimalEncryptor.setSaltGenerator(saltGenerator);
}
/**
* Sets the PBEConfig to be used by the internal encryptor,
* if a specific encryptor has not been set with setEncryptor(...).
*
* @param config the PBEConfig to be set for the internal encryptor
*/
public void setConfig(final PBEConfig config) {
if (this.encryptorSet) {
throw new EncryptionInitializationException(
"An encryptor has been already set: no " +
"further configuration possible on hibernate wrapper");
}
final StandardPBEBigDecimalEncryptor standardPBEBigDecimalEncryptor =
(StandardPBEBigDecimalEncryptor) this.encryptor;
standardPBEBigDecimalEncryptor.setConfig(config);
}
/**
* Encrypts a message, delegating to wrapped encryptor.
*
* @param message the message to be encrypted.
* @return the encryption result.
*/
public BigDecimal encrypt(final BigDecimal message) {
if (this.encryptor == null) {
throw new EncryptionInitializationException(
"Encryptor has not been set into Hibernate wrapper");
}
return this.encryptor.encrypt(message);
}
/**
* Decypts a message, delegating to wrapped encryptor
*
* @param encryptedMessage the message to be decrypted.
* @return the result of decryption.
*/
public BigDecimal decrypt(final BigDecimal encryptedMessage) {
if (this.encryptor == null) {
throw new EncryptionInitializationException(
"Encryptor has not been set into Hibernate wrapper");
}
return this.encryptor.decrypt(encryptedMessage);
}
/**
* Sets the registered name of the encryptor and adds it to the registry.
*
* @param registeredName the name with which the encryptor will be
* registered.
*/
public void setRegisteredName(final String registeredName) {
if (this.registeredName != null) {
// It had another name before, we have to clean
HibernatePBEEncryptorRegistry.getInstance().
unregisterHibernatePBEBigDecimalEncryptor(this.registeredName);
}
this.registeredName = registeredName;
HibernatePBEEncryptorRegistry.getInstance().
registerHibernatePBEBigDecimalEncryptor(this);
}
/**
* Returns the name with which the wrapped encryptor is registered at
* the registry.
*
* @return the registered name.
*/
public String getRegisteredName() {
return this.registeredName;
}
}