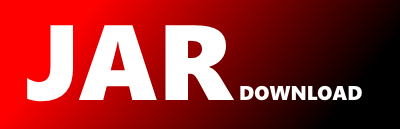
org.jasypt.hibernate3.connectionprovider.EncryptedPasswordDriverManagerConnectionProvider Maven / Gradle / Ivy
/*
* =============================================================================
*
* Copyright (c) 2007-2010, The JASYPT team (http://www.jasypt.org)
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
* =============================================================================
*/
package org.jasypt.hibernate3.connectionprovider;
import java.util.Properties;
import org.hibernate.cfg.Environment;
import org.hibernate.connection.DriverManagerConnectionProvider;
import org.jasypt.encryption.pbe.PBEStringEncryptor;
import org.jasypt.exceptions.EncryptionInitializationException;
import org.jasypt.hibernate3.encryptor.HibernatePBEEncryptorRegistry;
import org.jasypt.properties.PropertyValueEncryptionUtils;
/**
*
*
* Extension of {@link DriverManagerConnectionProvider} that allows the user
* to write the datasource configuration parameters in an encrypted manner in the
* hibernate.cfg.xml or hibernate.properties file
*
*
* The encryptable parameters are:
*
* - connection.driver_class
* - connection.url
* - connection.username
* - connection.password
*
*
*
* The name of the password encryptor (decryptor, in fact) will be set in
* property hibernate.connection.encryptor_registered_name.
* Its value must be the name of a {@link PBEStringEncryptor} object
* previously registered within {@link HibernatePBEEncryptorRegistry}.
*
*
* An example hibernate.cfg.xml file:
*
*
*
* <hibernate-configuration>
*
* <session-factory>
*
* <!-- Database connection settings -->
* <property name="connection.provider_class">org.jasypt.hibernate.connectionprovider.EncryptedPasswordDriverManagerConnectionProvider</property>
* <property name="connection.encryptor_registered_name">stringEncryptor</property>
* <property name="connection.driver_class">org.postgresql.Driver</property>
* <property name="connection.url">jdbc:postgresql://localhost/mydatabase</property>
* <property name="connection.username">myuser</property>
* <property name="connection.password">ENC(T6DAe34NasW==)</property>
* <property name="connection.pool_size">5</property>
*
* ...
*
* </session-factory>
*
* ...
*
* </hibernate-configuration>
*
*
*
* @since 1.9.0 (class existed in package
* org.jasypt.hibernate.connectionprovider since 1.4)
*
* @author Daniel Fernández
*
*/
public final class EncryptedPasswordDriverManagerConnectionProvider
extends DriverManagerConnectionProvider {
public EncryptedPasswordDriverManagerConnectionProvider() {
super();
}
public void configure(final Properties props) {
final String encryptorRegisteredName =
props.getProperty(ParameterNaming.ENCRYPTOR_REGISTERED_NAME);
final HibernatePBEEncryptorRegistry encryptorRegistry =
HibernatePBEEncryptorRegistry.getInstance();
final PBEStringEncryptor encryptor =
encryptorRegistry.getPBEStringEncryptor(encryptorRegisteredName);
if (encryptor == null) {
throw new EncryptionInitializationException(
"No string encryptor registered for hibernate " +
"with name \"" + encryptorRegisteredName + "\"");
}
// Get the original values, which may be encrypted
final String driver = props.getProperty(Environment.DRIVER);
final String url = props.getProperty(Environment.URL);
final String user = props.getProperty(Environment.USER);
final String password = props.getProperty(Environment.PASS);
// Perform decryption operations as needed and store the new values
if (PropertyValueEncryptionUtils.isEncryptedValue(driver)) {
props.setProperty(
Environment.DRIVER,
PropertyValueEncryptionUtils.decrypt(driver, encryptor));
}
if (PropertyValueEncryptionUtils.isEncryptedValue(url)) {
props.setProperty(
Environment.URL,
PropertyValueEncryptionUtils.decrypt(url, encryptor));
}
if (PropertyValueEncryptionUtils.isEncryptedValue(user)) {
props.setProperty(
Environment.USER,
PropertyValueEncryptionUtils.decrypt(user, encryptor));
}
if (PropertyValueEncryptionUtils.isEncryptedValue(password)) {
props.setProperty(
Environment.PASS,
PropertyValueEncryptionUtils.decrypt(password, encryptor));
}
// Let Hibernate process
super.configure(props);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy