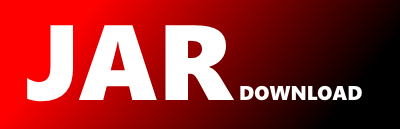
org.javabits.yar.guice.DefaultRegistryAnnotatedBindingBuilderImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of yar-guice Show documentation
Show all versions of yar-guice Show documentation
Yar Guice: provide a implementation / integration base on guice
/*
* Copyright (c) 4/30/15 8:53 PM Romain Gilles
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.javabits.yar.guice;
import com.google.common.collect.ImmutableList;
import com.google.inject.Binder;
import com.google.inject.Key;
import com.google.inject.TypeLiteral;
import com.google.inject.util.Types;
import org.javabits.yar.BlockingSupplier;
import org.javabits.yar.Supplier;
import java.lang.reflect.ParameterizedType;
import java.util.concurrent.TimeUnit;
/**
* This binding builder will handle the classic binding to and from the registry.
* This binding builder will produce 3 types of binding when {@link #toRegistry()} is executed.
*
* Let say we want to get an implementation of the {@code Hello} interface from the registry.
* Therefore you will add the following binding to your module:
*
* bind(Hello.class).toRegistry();
*
* This binding will automatically amply the following available injections:
*
* - @Inject Hello hello;
* - @Inject com.google.common.base.Supplier>Hello< hello;
* - @Inject org.javabits.yar.Supplier>Hello< hello;
* - @Inject org.javabits.yar.BlockingSupplier>Hello< hello;
*
*
* @author Romain Gilles
*/
class DefaultRegistryAnnotatedBindingBuilderImpl extends RegistryAnnotatedBindingBuilderImpl {
DefaultRegistryAnnotatedBindingBuilderImpl(Binder binder, Key key) {
super(binder, key);
}
DefaultRegistryAnnotatedBindingBuilderImpl(Binder binder, TypeLiteral typeLiteral) {
super(binder, typeLiteral);
}
DefaultRegistryAnnotatedBindingBuilderImpl(Binder binder, Class clazz) {
super(binder, clazz);
}
@Override
public void toRegistry(long timeout, TimeUnit unit) {
linkedBindingBuilder().toProvider(newRegistryProvider(timeout, unit));
bindSuppliers();
}
@Override
Iterable> doToRegistry() {
RegistryProvider registryProvider = newRegistryProvider();
linkedBindingBuilder().toProvider(registryProvider);
RegistryProvider> blockingSupplierRegistryProvider = bindSuppliers();
return ImmutableList.of(registryProvider, blockingSupplierRegistryProvider);
}
private RegistryProvider> bindSuppliers() {
Key> blockingSupplierKey = newBlockingSupplierKey();
BlockingSupplierRegistryProvider blockingSupplierRegistryProvider = BlockingSupplierRegistryProvider.newProviderOf(key());
binder().bind(blockingSupplierKey).toProvider(blockingSupplierRegistryProvider);
Key> yarSupplierKey = newYarSupplierKey();
binder().bind(yarSupplierKey).to(blockingSupplierKey);
binder().bind(newGuavaSupplierKey()).to(yarSupplierKey);
binder().bind(newJavaSupplierKey()).to(yarSupplierKey);
return blockingSupplierRegistryProvider;
}
private Key> newGuavaSupplierKey() {
ParameterizedType guavaSupplierType = Types.newParameterizedType(com.google.common.base.Supplier.class, key().getTypeLiteral().getType());
return Keys.of(guavaSupplierType, key());
}
private Key> newJavaSupplierKey() {
ParameterizedType guavaSupplierType = Types.newParameterizedType(java.util.function.Supplier.class, key().getTypeLiteral().getType());
return Keys.of(guavaSupplierType, key());
}
private Key> newYarSupplierKey() {
ParameterizedType yarSupplierType = Types.newParameterizedType(Supplier.class, key().getTypeLiteral().getType());
return Keys.of(yarSupplierType, key());
}
private Key> newBlockingSupplierKey() {
ParameterizedType blockingSupplierType = Types.newParameterizedType(BlockingSupplier.class, key().getTypeLiteral().getType());
return Keys.of(blockingSupplierType, key());
}
private RegistryProvider extends T> newRegistryProvider(long timeout, TimeUnit unit) {
return new RegistryProviderImpl<>(key(), timeout, unit);
}
private RegistryProvider newRegistryProvider() {
return new RegistryProviderImpl<>(key());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy