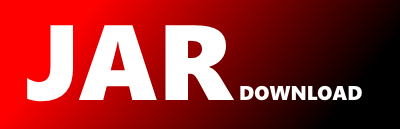
org.javabits.yar.guice.GuiceWatcherRegistration Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of yar-guice Show documentation
Show all versions of yar-guice Show documentation
Yar Guice: provide a implementation / integration base on guice
/*
* Copyright 2013 Romain Gilles
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.javabits.yar.guice;
import com.google.inject.Inject;
import com.google.inject.Injector;
import com.google.inject.Key;
import com.google.inject.matcher.Matcher;
import org.javabits.yar.Id;
import org.javabits.yar.IdMatcher;
import org.javabits.yar.Watcher;
import static org.javabits.yar.guice.Matchers.getId;
/**
* TODO comment
* Date: 3/17/13
*
* @author Romain Gilles
*/
abstract class GuiceWatcherRegistration {
private final Matcher> matcher;
GuiceWatcherRegistration(Matcher> matcher) {
this.matcher = matcher;
}
public static GuiceWatcherRegistration get(Matcher> matcher, Key extends RegistryListener super T>> key) {
return new KeyGuiceWatcherRegistration<>(matcher, key);
}
public static GuiceWatcherRegistration get(Matcher> matcher, RegistryListener super T> listener) {
return new InstanceGuiceWatcherRegistration<>(matcher, listener);
}
public abstract Watcher watcher();
public IdMatcher matcher() {
return newKeyMatcher(matcher);
}
private static IdMatcher newKeyMatcher(final Matcher> keyMatcher) {
return new IdMatcherWrapper<>(keyMatcher);
}
static class KeyGuiceWatcherRegistration extends GuiceWatcherRegistration {
private final Key extends RegistryListener super T>> key;
private Injector injector;
KeyGuiceWatcherRegistration(Matcher> matcher, Key extends RegistryListener super T>> key) {
super(matcher);
this.key = key;
}
@Override
public Watcher watcher() {
return newWatcherAdapter(injector.getInstance(key));
}
@Inject
public void setInjector(Injector injector) {
this.injector = injector;
}
}
static class InstanceGuiceWatcherRegistration extends GuiceWatcherRegistration {
private final RegistryListener super T> listener;
InstanceGuiceWatcherRegistration(Matcher> matcher, RegistryListener super T> listener) {
super(matcher);
this.listener = listener;
}
@Override
public Watcher watcher() {
return newWatcherAdapter(listener);
}
}
@SuppressWarnings("unchecked")
private static Watcher newWatcherAdapter(final RegistryListener listener) {
return listener;
}
private static class IdMatcherWrapper implements IdMatcher {
private final Matcher> matcher;
private final Id id;
public IdMatcherWrapper(Matcher> keyMatcher) {
matcher = keyMatcher;
id = getId(matcher);
}
@Override
public boolean matches(Id otherId) {
return matcher.matches(getKey(otherId));
}
@SuppressWarnings("unchecked")
private Key getKey(Id otherId) {
Key otherGuiceKey;
if (otherId.annotation() != null)
otherGuiceKey = (Key) Key.get(otherId.type(), otherId.annotation());
else if (otherId.annotationType() != null) {
otherGuiceKey = (Key) Key.get(otherId.type(), otherId.annotationType());
} else {
otherGuiceKey = (Key) Key.get(otherId.type());
}
return otherGuiceKey;
}
@Override
public Id id() {
return id;
}
@Override
public String toString() {
return "IdMatcherWrapper{" +
"id=" + id +
'}';
}
}
@Override
public String toString() {
return "GuiceWatcherRegistration{" +
"matcher=" + matcher +
'}';
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy