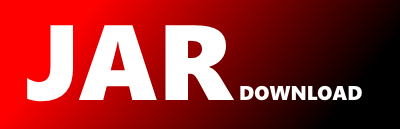
org.datafx.concurrent.ProcessChain Maven / Gradle / Ivy
package org.datafx.concurrent;
import javafx.concurrent.Task;
import java.util.ArrayList;
import java.util.List;
import java.util.concurrent.*;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.function.Supplier;
/**
* The class defines a chain of processes. All processes will be running in a queue and the result of a process will be used as the input parameter for the next process. A process can run
* @param Return value of the chain.
*
* @author Hendrik Ebbers
*/
public class ProcessChain {
private List> processes;
private Executor executorService;
public ProcessChain() {
this(Executors.newCachedThreadPool(), null);
}
public ProcessChain(Executor executorService) {
this(executorService, null);
}
private ProcessChain(Executor executorService, List> processes) {
this.executorService = executorService;
this.processes = new ArrayList<>();
if (processes != null) {
this.processes.addAll(processes);
}
}
public static ProcessChain create() {
return new ProcessChain<>();
}
public static ProcessChain create(Executor executorService) {
return new ProcessChain<>(executorService);
}
public ProcessChain inPlatformThread(Function function) {
processes.add(new ProcessDescription(function, ThreadType.PLATFORM));
return new ProcessChain(executorService, processes);
}
public ProcessChain inExecutor(Function function) {
processes.add(new ProcessDescription(function, ThreadType.EXECUTOR));
return new ProcessChain(executorService, processes);
}
public ProcessChain inPlatformThread(Runnable runnable) {
return inPlatformThread((Function) (e) -> {
runnable.run();
return null;
});
}
public ProcessChain inExecutor(Runnable runnable) {
return inExecutor((Function) (e) -> {
runnable.run();
return null;
});
}
public ProcessChain inPlatformThread(Consumer consumer) {
return inPlatformThread((Function) (e) -> {
consumer.accept(e);
return null;
});
}
public ProcessChain inExecutor(Consumer consumer) {
return inExecutor((Function) (e) -> {
consumer.accept(e);
return null;
});
}
public ProcessChain inPlatformThread(Supplier supplier) {
return inPlatformThread((Function) (e) -> {
return supplier.get();
});
}
public ProcessChain inExecutor(Supplier supplier) {
return inExecutor((Function) (e) -> {
return supplier.get();
});
}
@SuppressWarnings("unchecked")
private V execute(U inputParameter, ProcessDescription processDescription, Executor executorService) throws InterruptedException, ExecutionException {
if (processDescription.getThreadType().equals(ThreadType.EXECUTOR)) {
FutureTask task = new FutureTask(() -> {
return processDescription.getFunction().apply(inputParameter);
});
executorService.execute(task);
return task.get();
} else {
return ConcurrentUtils.runAndWait(() -> {
return processDescription.getFunction().apply(inputParameter);
});
}
}
public Task run() {
Task task = new Task() {
@Override
protected T call() throws Exception {
Object lastResult = null;
for (ProcessDescription, ?> processDescription : processes) {
lastResult = execute(lastResult, (ProcessDescription
© 2015 - 2025 Weber Informatics LLC | Privacy Policy