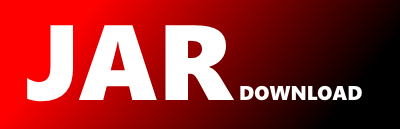
org.datafx.concurrent.ProcessChain Maven / Gradle / Ivy
The newest version!
package org.datafx.concurrent;
import javafx.concurrent.Task;
import javafx.util.Duration;
import java.util.ArrayList;
import java.util.List;
import java.util.concurrent.ExecutionException;
import java.util.concurrent.Executor;
import java.util.concurrent.Executors;
import java.util.concurrent.FutureTask;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.function.Supplier;
/**
* The class defines a chain of processes. All processes will be running in a queue and the result of a process will be used as the input parameter for the next process. A process can run
* @param Return value of the chain.
*
* @author Hendrik Ebbers
*/
public class ProcessChain {
private List> processes;
private Executor executorService;
public ProcessChain() {
this(Executors.newCachedThreadPool(), null);
}
public ProcessChain(Executor executorService) {
this(executorService, null);
}
private ProcessChain(Executor executorService, List> processes) {
this.executorService = executorService;
this.processes = new ArrayList<>();
if (processes != null) {
this.processes.addAll(processes);
}
}
public static ProcessChain create() {
return new ProcessChain<>();
}
public static ProcessChain create(Executor executorService) {
return new ProcessChain<>(executorService);
}
public ProcessChain addFunction(Function function, ThreadType type) {
processes.add(new ProcessDescription(function, type));
return new ProcessChain(executorService, processes);
}
public ProcessChain addFunctionInPlatformThread(Function function) {
return addFunction(function, ThreadType.PLATFORM);
}
public ProcessChain addFunctionInExecutor(Function function) {
return addFunction(function, ThreadType.EXECUTOR);
}
public ProcessChain addRunnable(Runnable runnable, ThreadType type) {
return addFunction((Function) (e) -> {
runnable.run();
return null;
}, type);
}
public ProcessChain addRunnableInPlatformThread(Runnable runnable) {
return addRunnable(runnable, ThreadType.PLATFORM);
}
public ProcessChain addRunnableInExecutor(Runnable runnable) {
return addRunnable(runnable, ThreadType.EXECUTOR);
}
public ProcessChain addConsumer(Consumer consumer, ThreadType type) {
return addFunction((Function) (e) -> {
consumer.accept(e);
return null;
}, type);
}
public ProcessChain addConsumerInPlatformThread(Consumer consumer) {
return addConsumer(consumer, ThreadType.PLATFORM);
}
public ProcessChain addConsumerInExecutor(Consumer consumer) {
return addConsumer(consumer, ThreadType.EXECUTOR);
}
public ProcessChain addSupplierInPlatformThread(Supplier supplier) {
return addSupplier(supplier, ThreadType.PLATFORM);
}
public ProcessChain addSupplierInExecutor(Supplier supplier) {
return addSupplier(supplier, ThreadType.EXECUTOR);
}
public ProcessChain addSupplier(Supplier supplier, ThreadType type) {
return addFunction((Function) (e) -> {
return supplier.get();
}, type);
}
@SuppressWarnings("unchecked")
private V execute(U inputParameter, ProcessDescription processDescription, Executor executorService) throws InterruptedException, ExecutionException {
if (processDescription.getThreadType().equals(ThreadType.EXECUTOR)) {
FutureTask task = new FutureTask(() -> {
return processDescription.getFunction().apply(inputParameter);
});
executorService.execute(task);
return task.get();
} else {
return ConcurrentUtils.runCallableAndWait(() -> {
return processDescription.getFunction().apply(inputParameter);
});
}
}
public Task repeatInfinite() {
return repeat(Integer.MAX_VALUE);
}
public Task repeatInfinite(Duration pauseTime) {
return repeat(Integer.MAX_VALUE, pauseTime);
}
public Task repeat(int count) {
return repeat(count, Duration.ZERO);
}
public Task repeat(int count, Duration pauseTime) {
Task task = new Task() {
@Override
protected T call() throws Exception {
Object lastResult = null;
if(count == Integer.MAX_VALUE) {
while(true) {
lastResult = null;
for (ProcessDescription, ?> processDescription : processes) {
lastResult = execute(lastResult, (ProcessDescription
© 2015 - 2025 Weber Informatics LLC | Privacy Policy