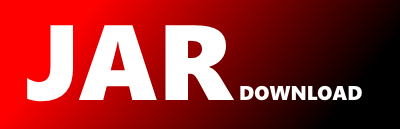
org.datafx.crud.BasicCrudService Maven / Gradle / Ivy
package org.datafx.crud;
import org.datafx.util.Call;
import org.datafx.util.EntityWithId;
import org.datafx.util.QueryParameter;
import java.util.Arrays;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class BasicCrudService, T> implements CrudService {
private Call> getAllCall;
private Call getByIdCall;
private Call deleteByIdCall;
private Call persistCall;
private Call updateCall;
private Map, List>> queries;
public BasicCrudService(Call> getAllCall, Call getByIdCall, Call deleteByIdCall, Call persistCall, Call updateCall) {
this.deleteByIdCall = deleteByIdCall;
this.getAllCall = getAllCall;
this.getByIdCall = getByIdCall;
this.persistCall = persistCall;
this.updateCall = updateCall;
queries = new HashMap<>();
}
@Override
public void delete(S entity) throws CrudException {
T id = entity.getId();
if (id == null) {
throw new CrudException("TODO");
}
try {
deleteByIdCall.call(id);
} catch (Exception e) {
throw new CrudException("TODO", e);
}
}
@Override
public S save(S entity) throws CrudException {
if (entity.getId() == null) {
try {
return persistCall.call(entity);
} catch (Exception e) {
throw new CrudException("TODO", e);
}
} else {
try {
return updateCall.call(entity);
} catch (Exception e) {
throw new CrudException("TODO", e);
}
}
}
@Override
public List getAll() throws CrudException {
try {
return getAllCall.call(null);
} catch (Exception e) {
throw new CrudException("TODO", e);
}
}
@Override
public S getById(T id) throws CrudException {
try {
return getByIdCall.call(id);
} catch (Exception e) {
throw new CrudException("TODO", e);
}
}
public void addQuery(String name, Call, List> query) {
queries.put(name, query);
}
@Override
public List query(String name, QueryParameter... params) throws CrudException {
Call, List> queryCall = queries.get(name);
if(queryCall == null) {
throw new CrudException("TODO");
}
List paramList = Arrays.asList(params);
try {
return queryCall.call(paramList);
} catch (Exception e) {
throw new CrudException("TODO", e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy