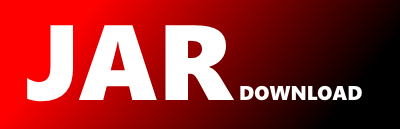
org.datafx.crud.CrudObjectProperty Maven / Gradle / Ivy
The newest version!
package org.datafx.crud;
import javafx.beans.property.SimpleObjectProperty;
import javafx.concurrent.Worker;
import org.datafx.concurrent.ConcurrentUtils;
import org.datafx.concurrent.ObservableExecutor;
import org.datafx.util.EntityWithId;
import java.util.concurrent.Executor;
public class CrudObjectProperty, T> extends SimpleObjectProperty {
private CrudService crudService;
private Executor executor;
private CrudListProperty listProperty;
public CrudObjectProperty(S entity, CrudListProperty listProperty, CrudService crudService) {
this(entity, crudService, ObservableExecutor.getDefaultInstance());
this.listProperty = listProperty;
}
public CrudObjectProperty(S entity, CrudService crudService) {
this(crudService);
set(entity);
}
public CrudObjectProperty(CrudService crudService) {
this(crudService, ObservableExecutor.getDefaultInstance());
}
public CrudObjectProperty(S entity, CrudListProperty listProperty, CrudService crudService, Executor executor) {
this(entity, crudService, executor);
this.listProperty = listProperty;
}
public CrudObjectProperty(S entity, CrudService crudService, Executor executor) {
this(crudService, executor);
set(entity);
}
private CrudObjectProperty(CrudService crudService, Executor executor) {
this.crudService = crudService;
this.executor = executor;
}
public Worker update() {
return ConcurrentUtils.executeService(executor, ConcurrentUtils.createService(() -> {
try {
T id = ConcurrentUtils.runCallableAndWait(() -> get().getId());
S updatedData = crudService.getById(id);
ConcurrentUtils.runAndWait(() -> set(updatedData));
return updatedData;
} catch (Exception e) {
throw new RuntimeException("TODO", e);
}
}));
}
public Worker save() {
return ConcurrentUtils.executeService(executor, ConcurrentUtils.createService(() -> {
try {
S updatedData = crudService.save(get());
ConcurrentUtils.runAndWait(() -> set(updatedData));
return updatedData;
} catch (Exception e) {
throw new RuntimeException("TODO", e);
}
}));
}
public Worker delete() {
return ConcurrentUtils.executeService(executor, ConcurrentUtils.createService(() -> {
try {
crudService.delete(get());
ConcurrentUtils.runAndWait(() -> {
set(null);
if (listProperty != null) {
listProperty.remove(this);
}
});
} catch (Exception e) {
throw new RuntimeException("TODO", e);
}
}));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy