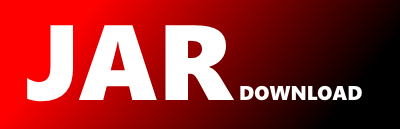
org.datafx.crud.rest.RestCall Maven / Gradle / Ivy
package org.datafx.crud.rest;
import org.datafx.util.Call;
import org.datafx.util.HttpMethods;
import java.io.IOException;
import java.io.InputStream;
import java.net.HttpURLConnection;
import java.net.URL;
import java.util.function.Function;
public class RestCall implements Call {
private Function urlFactory;
private HttpMethods httpMethod;
private RequestDataHandler requestDataProvider;
private Function responseDataHandler;
public RestCall(Function urlFactory, HttpMethods httpMethod, RequestDataHandler requestDataProvider, Function responseDataHandler) {
this.httpMethod = httpMethod;
this.requestDataProvider = requestDataProvider;
this.responseDataHandler = responseDataHandler;
this.urlFactory = urlFactory;
}
public T call(S input) throws IOException {
HttpURLConnection connection = (HttpURLConnection) urlFactory.apply(input).openConnection();
connection.setRequestMethod(httpMethod.toString());
if(requestDataProvider != null) {
requestDataProvider.writeData(input, connection.getOutputStream());
}
if(responseDataHandler != null) {
return responseDataHandler.apply(connection.getInputStream());
}
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy