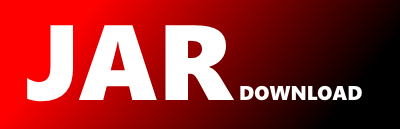
org.javasimon.proxy.DelegatingMethodInvocation Maven / Gradle / Ivy
package org.javasimon.proxy;
import java.lang.reflect.Method;
import java.util.concurrent.Callable;
/**
* Proxy method invocation.
*
* @author gquintana
*/
public class DelegatingMethodInvocation implements Delegating, Runnable, Callable
© 2015 - 2025 Weber Informatics LLC | Privacy Policy