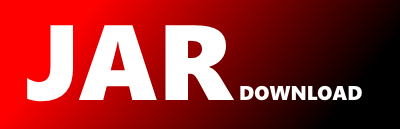
org.javastro.ivoa.entities.resource.Contact Maven / Gradle / Ivy
package org.javastro.ivoa.entities.resource;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import javax.xml.namespace.QName;
import com.kscs.util.jaxb.Buildable;
import com.kscs.util.jaxb.CollectionProperty;
import com.kscs.util.jaxb.CollectionPropertyInfo;
import com.kscs.util.jaxb.CollectionPropertyInfo;
import com.kscs.util.jaxb.Copyable;
import com.kscs.util.jaxb.PartialCopyable;
import com.kscs.util.jaxb.PropertyTree;
import com.kscs.util.jaxb.PropertyTreeUse;
import com.kscs.util.jaxb.PropertyVisitor;
import com.kscs.util.jaxb.SingleProperty;
import com.kscs.util.jaxb.SinglePropertyInfo;
import com.kscs.util.jaxb.SinglePropertyInfo;
import com.kscs.util.jaxb.SinglePropertyInfo;
import jakarta.annotation.Generated;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlAttribute;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlSchemaType;
import jakarta.xml.bind.annotation.XmlType;
import jakarta.xml.bind.annotation.adapters.CollapsedStringAdapter;
import jakarta.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import org.jvnet.jaxb.lang.JAXBMergeStrategy;
import org.jvnet.jaxb.lang.JAXBToStringStrategy;
import org.jvnet.jaxb.lang.MergeFrom;
import org.jvnet.jaxb.lang.MergeStrategy;
import org.jvnet.jaxb.lang.ToString;
import org.jvnet.jaxb.lang.ToStringStrategy;
import org.jvnet.jaxb.locator.ObjectLocator;
import org.jvnet.jaxb.locator.util.LocatorUtils;
/**
* Information allowing establishing contact, e.g., for purposes
* of support.
*
* Java class for Contact complex type
.
*
* The following schema fragment specifies the expected content contained within this class.
*
* {@code
*
*
*
*
*
*
*
*
*
*
*
*
*
*
* }
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "Contact", propOrder = {
"name",
"address",
"email",
"telephone",
"altIdentifiers"
})
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public class Contact implements Cloneable, Copyable, PartialCopyable, MergeFrom, ToString
{
/**
* This can be a person's name, e.g. “John P. Jones” or
* a group, “Archive Support Team”.
*
*/
@XmlElement(required = true)
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
protected ResourceName name;
/**
* All components of the mailing address are given in one
* string, e.g. “3700 San Martin Drive, Baltimore, MD 21218 USA”.
*
*/
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
@XmlSchemaType(name = "token")
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
protected String address;
/**
* the contact email address
*
*/
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
@XmlSchemaType(name = "token")
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
protected String email;
/**
* Complete international dialing codes should be given, e.g.
* “+1-410-338-1234”.
*
*/
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
@XmlSchemaType(name = "token")
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
protected String telephone;
/**
* A reference to this entitiy in a non-IVOA identifier
* scheme, e.g., orcid. Always use a URI form including
* a scheme here.
*
*/
@XmlElement(name = "altIdentifier")
@XmlSchemaType(name = "anyURI")
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
protected List altIdentifiers;
/**
* An IVOA identifier for the contact (typically when it is
* an organization).
*
*/
@XmlAttribute(name = "ivo-id")
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
protected String ivoId;
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
protected transient Contact.Modifier __cachedModifier__;
/**
* Default no-arg constructor
*
*/
public Contact() {
super();
}
/**
* Fully-initialising value constructor
*
*/
public Contact(final ResourceName name, final String address, final String email, final String telephone, final List altIdentifiers, final String ivoId) {
this.name = name;
this.address = address;
this.email = email;
this.telephone = telephone;
this.altIdentifiers = altIdentifiers;
this.ivoId = ivoId;
}
/**
* This can be a person's name, e.g. “John P. Jones” or
* a group, “Archive Support Team”.
*
* @return
* possible object is
* {@link ResourceName }
*
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public ResourceName getName() {
return name;
}
/**
* Sets the value of the name property.
*
* @param value
* allowed object is
* {@link ResourceName }
*
* @see #getName()
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void setName(ResourceName value) {
this.name = value;
}
/**
* All components of the mailing address are given in one
* string, e.g. “3700 San Martin Drive, Baltimore, MD 21218 USA”.
*
* @return
* possible object is
* {@link String }
*
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public String getAddress() {
return address;
}
/**
* Sets the value of the address property.
*
* @param value
* allowed object is
* {@link String }
*
* @see #getAddress()
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void setAddress(String value) {
this.address = value;
}
/**
* the contact email address
*
* @return
* possible object is
* {@link String }
*
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public String getEmail() {
return email;
}
/**
* Sets the value of the email property.
*
* @param value
* allowed object is
* {@link String }
*
* @see #getEmail()
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void setEmail(String value) {
this.email = value;
}
/**
* Complete international dialing codes should be given, e.g.
* “+1-410-338-1234”.
*
* @return
* possible object is
* {@link String }
*
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public String getTelephone() {
return telephone;
}
/**
* Sets the value of the telephone property.
*
* @param value
* allowed object is
* {@link String }
*
* @see #getTelephone()
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void setTelephone(String value) {
this.telephone = value;
}
/**
* A reference to this entitiy in a non-IVOA identifier
* scheme, e.g., orcid. Always use a URI form including
* a scheme here.
*
* Gets the value of the altIdentifiers property.
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the altIdentifiers property.
*
*
* For example, to add a new item, do as follows:
*
*
* getAltIdentifiers().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link String }
*
*
*
* @return
* The value of the altIdentifiers property.
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public List getAltIdentifiers() {
if (altIdentifiers == null) {
altIdentifiers = new ArrayList<>();
}
return this.altIdentifiers;
}
/**
* An IVOA identifier for the contact (typically when it is
* an organization).
*
* @return
* possible object is
* {@link String }
*
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public String getIvoId() {
return ivoId;
}
/**
* Sets the value of the ivoId property.
*
* @param value
* allowed object is
* {@link String }
*
* @see #getIvoId()
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void setIvoId(String value) {
this.ivoId = value;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public boolean equals(Object object) {
if ((object == null)||(this.getClass()!= object.getClass())) {
return false;
}
if (this == object) {
return true;
}
final Contact that = ((Contact) object);
{
ResourceName leftName;
leftName = this.getName();
ResourceName rightName;
rightName = that.getName();
if (this.name!= null) {
if (that.name!= null) {
if (!leftName.equals(rightName)) {
return false;
}
} else {
return false;
}
} else {
if (that.name!= null) {
return false;
}
}
}
{
String leftAddress;
leftAddress = this.getAddress();
String rightAddress;
rightAddress = that.getAddress();
if (this.address!= null) {
if (that.address!= null) {
if (!leftAddress.equals(rightAddress)) {
return false;
}
} else {
return false;
}
} else {
if (that.address!= null) {
return false;
}
}
}
{
String leftEmail;
leftEmail = this.getEmail();
String rightEmail;
rightEmail = that.getEmail();
if (this.email!= null) {
if (that.email!= null) {
if (!leftEmail.equals(rightEmail)) {
return false;
}
} else {
return false;
}
} else {
if (that.email!= null) {
return false;
}
}
}
{
String leftTelephone;
leftTelephone = this.getTelephone();
String rightTelephone;
rightTelephone = that.getTelephone();
if (this.telephone!= null) {
if (that.telephone!= null) {
if (!leftTelephone.equals(rightTelephone)) {
return false;
}
} else {
return false;
}
} else {
if (that.telephone!= null) {
return false;
}
}
}
{
List leftAltIdentifiers;
leftAltIdentifiers = (((this.altIdentifiers!= null)&&(!this.altIdentifiers.isEmpty()))?this.getAltIdentifiers():null);
List rightAltIdentifiers;
rightAltIdentifiers = (((that.altIdentifiers!= null)&&(!that.altIdentifiers.isEmpty()))?that.getAltIdentifiers():null);
if ((this.altIdentifiers!= null)&&(!this.altIdentifiers.isEmpty())) {
if ((that.altIdentifiers!= null)&&(!that.altIdentifiers.isEmpty())) {
if (!leftAltIdentifiers.equals(rightAltIdentifiers)) {
return false;
}
} else {
return false;
}
} else {
if ((that.altIdentifiers!= null)&&(!that.altIdentifiers.isEmpty())) {
return false;
}
}
}
{
String leftIvoId;
leftIvoId = this.getIvoId();
String rightIvoId;
rightIvoId = that.getIvoId();
if (this.ivoId!= null) {
if (that.ivoId!= null) {
if (!leftIvoId.equals(rightIvoId)) {
return false;
}
} else {
return false;
}
} else {
if (that.ivoId!= null) {
return false;
}
}
}
return true;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public int hashCode() {
int currentHashCode = 1;
{
currentHashCode = (currentHashCode* 31);
ResourceName theName;
theName = this.getName();
if (this.name!= null) {
currentHashCode += theName.hashCode();
}
}
{
currentHashCode = (currentHashCode* 31);
String theAddress;
theAddress = this.getAddress();
if (this.address!= null) {
currentHashCode += theAddress.hashCode();
}
}
{
currentHashCode = (currentHashCode* 31);
String theEmail;
theEmail = this.getEmail();
if (this.email!= null) {
currentHashCode += theEmail.hashCode();
}
}
{
currentHashCode = (currentHashCode* 31);
String theTelephone;
theTelephone = this.getTelephone();
if (this.telephone!= null) {
currentHashCode += theTelephone.hashCode();
}
}
{
currentHashCode = (currentHashCode* 31);
List theAltIdentifiers;
theAltIdentifiers = (((this.altIdentifiers!= null)&&(!this.altIdentifiers.isEmpty()))?this.getAltIdentifiers():null);
if ((this.altIdentifiers!= null)&&(!this.altIdentifiers.isEmpty())) {
currentHashCode += theAltIdentifiers.hashCode();
}
}
{
currentHashCode = (currentHashCode* 31);
String theIvoId;
theIvoId = this.getIvoId();
if (this.ivoId!= null) {
currentHashCode += theIvoId.hashCode();
}
}
return currentHashCode;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public String toString() {
final ToStringStrategy strategy = JAXBToStringStrategy.getInstance();
final StringBuilder buffer = new StringBuilder();
append(null, buffer, strategy);
return buffer.toString();
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public StringBuilder append(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
strategy.appendStart(locator, this, buffer);
appendFields(locator, buffer, strategy);
strategy.appendEnd(locator, this, buffer);
return buffer;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public StringBuilder appendFields(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
{
ResourceName theName;
theName = this.getName();
strategy.appendField(locator, this, "name", buffer, theName, (this.name!= null));
}
{
String theAddress;
theAddress = this.getAddress();
strategy.appendField(locator, this, "address", buffer, theAddress, (this.address!= null));
}
{
String theEmail;
theEmail = this.getEmail();
strategy.appendField(locator, this, "email", buffer, theEmail, (this.email!= null));
}
{
String theTelephone;
theTelephone = this.getTelephone();
strategy.appendField(locator, this, "telephone", buffer, theTelephone, (this.telephone!= null));
}
{
List theAltIdentifiers;
theAltIdentifiers = (((this.altIdentifiers!= null)&&(!this.altIdentifiers.isEmpty()))?this.getAltIdentifiers():null);
strategy.appendField(locator, this, "altIdentifiers", buffer, theAltIdentifiers, ((this.altIdentifiers!= null)&&(!this.altIdentifiers.isEmpty())));
}
{
String theIvoId;
theIvoId = this.getIvoId();
strategy.appendField(locator, this, "ivoId", buffer, theIvoId, (this.ivoId!= null));
}
return buffer;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void mergeFrom(Object left, Object right) {
final MergeStrategy strategy = JAXBMergeStrategy.getInstance();
mergeFrom(null, null, left, right, strategy);
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void mergeFrom(ObjectLocator leftLocator, ObjectLocator rightLocator, Object left, Object right, MergeStrategy strategy) {
if (right instanceof Contact) {
final Contact target = this;
final Contact leftObject = ((Contact) left);
final Contact rightObject = ((Contact) right);
{
Boolean nameShouldBeMergedAndSet = strategy.shouldBeMergedAndSet(leftLocator, rightLocator, (leftObject.name!= null), (rightObject.name!= null));
if (nameShouldBeMergedAndSet == Boolean.TRUE) {
ResourceName lhsName;
lhsName = leftObject.getName();
ResourceName rhsName;
rhsName = rightObject.getName();
ResourceName mergedName = ((ResourceName) strategy.merge(LocatorUtils.property(leftLocator, "name", lhsName), LocatorUtils.property(rightLocator, "name", rhsName), lhsName, rhsName, (leftObject.name!= null), (rightObject.name!= null)));
target.setName(mergedName);
} else {
if (nameShouldBeMergedAndSet == Boolean.FALSE) {
target.name = null;
}
}
}
{
Boolean addressShouldBeMergedAndSet = strategy.shouldBeMergedAndSet(leftLocator, rightLocator, (leftObject.address!= null), (rightObject.address!= null));
if (addressShouldBeMergedAndSet == Boolean.TRUE) {
String lhsAddress;
lhsAddress = leftObject.getAddress();
String rhsAddress;
rhsAddress = rightObject.getAddress();
String mergedAddress = ((String) strategy.merge(LocatorUtils.property(leftLocator, "address", lhsAddress), LocatorUtils.property(rightLocator, "address", rhsAddress), lhsAddress, rhsAddress, (leftObject.address!= null), (rightObject.address!= null)));
target.setAddress(mergedAddress);
} else {
if (addressShouldBeMergedAndSet == Boolean.FALSE) {
target.address = null;
}
}
}
{
Boolean emailShouldBeMergedAndSet = strategy.shouldBeMergedAndSet(leftLocator, rightLocator, (leftObject.email!= null), (rightObject.email!= null));
if (emailShouldBeMergedAndSet == Boolean.TRUE) {
String lhsEmail;
lhsEmail = leftObject.getEmail();
String rhsEmail;
rhsEmail = rightObject.getEmail();
String mergedEmail = ((String) strategy.merge(LocatorUtils.property(leftLocator, "email", lhsEmail), LocatorUtils.property(rightLocator, "email", rhsEmail), lhsEmail, rhsEmail, (leftObject.email!= null), (rightObject.email!= null)));
target.setEmail(mergedEmail);
} else {
if (emailShouldBeMergedAndSet == Boolean.FALSE) {
target.email = null;
}
}
}
{
Boolean telephoneShouldBeMergedAndSet = strategy.shouldBeMergedAndSet(leftLocator, rightLocator, (leftObject.telephone!= null), (rightObject.telephone!= null));
if (telephoneShouldBeMergedAndSet == Boolean.TRUE) {
String lhsTelephone;
lhsTelephone = leftObject.getTelephone();
String rhsTelephone;
rhsTelephone = rightObject.getTelephone();
String mergedTelephone = ((String) strategy.merge(LocatorUtils.property(leftLocator, "telephone", lhsTelephone), LocatorUtils.property(rightLocator, "telephone", rhsTelephone), lhsTelephone, rhsTelephone, (leftObject.telephone!= null), (rightObject.telephone!= null)));
target.setTelephone(mergedTelephone);
} else {
if (telephoneShouldBeMergedAndSet == Boolean.FALSE) {
target.telephone = null;
}
}
}
{
Boolean altIdentifiersShouldBeMergedAndSet = strategy.shouldBeMergedAndSet(leftLocator, rightLocator, ((leftObject.altIdentifiers!= null)&&(!leftObject.altIdentifiers.isEmpty())), ((rightObject.altIdentifiers!= null)&&(!rightObject.altIdentifiers.isEmpty())));
if (altIdentifiersShouldBeMergedAndSet == Boolean.TRUE) {
List lhsAltIdentifiers;
lhsAltIdentifiers = (((leftObject.altIdentifiers!= null)&&(!leftObject.altIdentifiers.isEmpty()))?leftObject.getAltIdentifiers():null);
List rhsAltIdentifiers;
rhsAltIdentifiers = (((rightObject.altIdentifiers!= null)&&(!rightObject.altIdentifiers.isEmpty()))?rightObject.getAltIdentifiers():null);
List mergedAltIdentifiers = ((List ) strategy.merge(LocatorUtils.property(leftLocator, "altIdentifiers", lhsAltIdentifiers), LocatorUtils.property(rightLocator, "altIdentifiers", rhsAltIdentifiers), lhsAltIdentifiers, rhsAltIdentifiers, ((leftObject.altIdentifiers!= null)&&(!leftObject.altIdentifiers.isEmpty())), ((rightObject.altIdentifiers!= null)&&(!rightObject.altIdentifiers.isEmpty()))));
target.altIdentifiers = null;
if (mergedAltIdentifiers!= null) {
List uniqueAltIdentifiersl = target.getAltIdentifiers();
uniqueAltIdentifiersl.addAll(mergedAltIdentifiers);
}
} else {
if (altIdentifiersShouldBeMergedAndSet == Boolean.FALSE) {
target.altIdentifiers = null;
}
}
}
{
Boolean ivoIdShouldBeMergedAndSet = strategy.shouldBeMergedAndSet(leftLocator, rightLocator, (leftObject.ivoId!= null), (rightObject.ivoId!= null));
if (ivoIdShouldBeMergedAndSet == Boolean.TRUE) {
String lhsIvoId;
lhsIvoId = leftObject.getIvoId();
String rhsIvoId;
rhsIvoId = rightObject.getIvoId();
String mergedIvoId = ((String) strategy.merge(LocatorUtils.property(leftLocator, "ivoId", lhsIvoId), LocatorUtils.property(rightLocator, "ivoId", rhsIvoId), lhsIvoId, rhsIvoId, (leftObject.ivoId!= null), (rightObject.ivoId!= null)));
target.setIvoId(mergedIvoId);
} else {
if (ivoIdShouldBeMergedAndSet == Boolean.FALSE) {
target.ivoId = null;
}
}
}
}
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Object createNewInstance() {
return new Contact();
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Contact clone() {
final Contact _newObject;
try {
_newObject = ((Contact) super.clone());
} catch (CloneNotSupportedException e) {
throw new RuntimeException(e);
}
_newObject.name = ((this.name == null)?null:this.name.clone());
_newObject.altIdentifiers = ((this.altIdentifiers == null)?null:new ArrayList<>(this.altIdentifiers));
return _newObject;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Contact createCopy() {
final Contact _newObject;
try {
_newObject = ((Contact) super.clone());
} catch (CloneNotSupportedException e) {
throw new RuntimeException(e);
}
_newObject.name = ((this.name == null)?null:this.name.createCopy());
_newObject.address = this.address;
_newObject.email = this.email;
_newObject.telephone = this.telephone;
_newObject.altIdentifiers = ((this.altIdentifiers == null)?null:new ArrayList<>(this.altIdentifiers));
_newObject.ivoId = this.ivoId;
return _newObject;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Contact createCopy(final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
final Contact _newObject;
try {
_newObject = ((Contact) super.clone());
} catch (CloneNotSupportedException e) {
throw new RuntimeException(e);
}
final PropertyTree namePropertyTree = ((_propertyTree == null)?null:_propertyTree.get("name"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(namePropertyTree!= null):((namePropertyTree == null)||(!namePropertyTree.isLeaf())))) {
_newObject.name = ((this.name == null)?null:this.name.createCopy(namePropertyTree, _propertyTreeUse));
}
final PropertyTree addressPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("address"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(addressPropertyTree!= null):((addressPropertyTree == null)||(!addressPropertyTree.isLeaf())))) {
_newObject.address = this.address;
}
final PropertyTree emailPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("email"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(emailPropertyTree!= null):((emailPropertyTree == null)||(!emailPropertyTree.isLeaf())))) {
_newObject.email = this.email;
}
final PropertyTree telephonePropertyTree = ((_propertyTree == null)?null:_propertyTree.get("telephone"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(telephonePropertyTree!= null):((telephonePropertyTree == null)||(!telephonePropertyTree.isLeaf())))) {
_newObject.telephone = this.telephone;
}
final PropertyTree altIdentifiersPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("altIdentifiers"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(altIdentifiersPropertyTree!= null):((altIdentifiersPropertyTree == null)||(!altIdentifiersPropertyTree.isLeaf())))) {
_newObject.altIdentifiers = ((this.altIdentifiers == null)?null:new ArrayList<>(this.altIdentifiers));
}
final PropertyTree ivoIdPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("ivoId"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(ivoIdPropertyTree!= null):((ivoIdPropertyTree == null)||(!ivoIdPropertyTree.isLeaf())))) {
_newObject.ivoId = this.ivoId;
}
return _newObject;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Contact copyExcept(final PropertyTree _propertyTree) {
return createCopy(_propertyTree, PropertyTreeUse.EXCLUDE);
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Contact copyOnly(final PropertyTree _propertyTree) {
return createCopy(_propertyTree, PropertyTreeUse.INCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Contact.Modifier modifier() {
if (null == this.__cachedModifier__) {
this.__cachedModifier__ = new Contact.Modifier();
}
return ((Contact.Modifier) this.__cachedModifier__);
}
/**
* Copies all state of this object to a builder. This method is used by the copyOf
* method and should not be called directly by client code.
*
* @param _other
* A builder instance to which the state of this object will be copied.
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public<_B >void copyTo(final Contact.Builder<_B> _other) {
_other.name = ((this.name == null)?null:this.name.newCopyBuilder(_other));
_other.address = this.address;
_other.email = this.email;
_other.telephone = this.telephone;
if (this.altIdentifiers == null) {
_other.altIdentifiers = null;
} else {
_other.altIdentifiers = new ArrayList<>();
for (String _item: this.altIdentifiers) {
_other.altIdentifiers.add(((_item == null)?null:new Buildable.PrimitiveBuildable(_item)));
}
}
_other.ivoId = this.ivoId;
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public<_B >Contact.Builder<_B> newCopyBuilder(final _B _parentBuilder) {
return new Contact.Builder<_B>(_parentBuilder, this, true);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Contact.Builder newCopyBuilder() {
return newCopyBuilder(null);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static Contact.Builder builder() {
return new Contact.Builder<>(null, null, false);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static<_B >Contact.Builder<_B> copyOf(final Contact _other) {
final Contact.Builder<_B> _newBuilder = new Contact.Builder<>(null, null, false);
_other.copyTo(_newBuilder);
return _newBuilder;
}
/**
* Copies all state of this object to a builder. This method is used by the copyOf
* method and should not be called directly by client code.
*
* @param _other
* A builder instance to which the state of this object will be copied.
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public<_B >void copyTo(final Contact.Builder<_B> _other, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
final PropertyTree namePropertyTree = ((_propertyTree == null)?null:_propertyTree.get("name"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(namePropertyTree!= null):((namePropertyTree == null)||(!namePropertyTree.isLeaf())))) {
_other.name = ((this.name == null)?null:this.name.newCopyBuilder(_other, namePropertyTree, _propertyTreeUse));
}
final PropertyTree addressPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("address"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(addressPropertyTree!= null):((addressPropertyTree == null)||(!addressPropertyTree.isLeaf())))) {
_other.address = this.address;
}
final PropertyTree emailPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("email"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(emailPropertyTree!= null):((emailPropertyTree == null)||(!emailPropertyTree.isLeaf())))) {
_other.email = this.email;
}
final PropertyTree telephonePropertyTree = ((_propertyTree == null)?null:_propertyTree.get("telephone"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(telephonePropertyTree!= null):((telephonePropertyTree == null)||(!telephonePropertyTree.isLeaf())))) {
_other.telephone = this.telephone;
}
final PropertyTree altIdentifiersPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("altIdentifiers"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(altIdentifiersPropertyTree!= null):((altIdentifiersPropertyTree == null)||(!altIdentifiersPropertyTree.isLeaf())))) {
if (this.altIdentifiers == null) {
_other.altIdentifiers = null;
} else {
_other.altIdentifiers = new ArrayList<>();
for (String _item: this.altIdentifiers) {
_other.altIdentifiers.add(((_item == null)?null:new Buildable.PrimitiveBuildable(_item)));
}
}
}
final PropertyTree ivoIdPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("ivoId"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(ivoIdPropertyTree!= null):((ivoIdPropertyTree == null)||(!ivoIdPropertyTree.isLeaf())))) {
_other.ivoId = this.ivoId;
}
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public<_B >Contact.Builder<_B> newCopyBuilder(final _B _parentBuilder, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
return new Contact.Builder<_B>(_parentBuilder, this, true, _propertyTree, _propertyTreeUse);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Contact.Builder newCopyBuilder(final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
return newCopyBuilder(null, _propertyTree, _propertyTreeUse);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static<_B >Contact.Builder<_B> copyOf(final Contact _other, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
final Contact.Builder<_B> _newBuilder = new Contact.Builder<>(null, null, false);
_other.copyTo(_newBuilder, _propertyTree, _propertyTreeUse);
return _newBuilder;
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static Contact.Builder copyExcept(final Contact _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.EXCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static Contact.Builder copyOnly(final Contact _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.INCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Contact visit(final PropertyVisitor _visitor_) {
_visitor_.visit(this);
if (_visitor_.visit(new SingleProperty<>(Contact.PropInfo.NAME, this))&&(this.name!= null)) {
this.name.visit(_visitor_);
}
_visitor_.visit(new SingleProperty<>(Contact.PropInfo.ADDRESS, this));
_visitor_.visit(new SingleProperty<>(Contact.PropInfo.EMAIL, this));
_visitor_.visit(new SingleProperty<>(Contact.PropInfo.TELEPHONE, this));
_visitor_.visit(new CollectionProperty<>(Contact.PropInfo.ALT_IDENTIFIERS, this));
_visitor_.visit(new SingleProperty<>(Contact.PropInfo.IVO_ID, this));
return this;
}
public static class Builder<_B >implements Buildable
{
protected final _B _parentBuilder;
protected final Contact _storedValue;
private ResourceName.Builder> name;
private String address;
private String email;
private String telephone;
private List altIdentifiers;
private String ivoId;
public Builder(final _B _parentBuilder, final Contact _other, final boolean _copy) {
this._parentBuilder = _parentBuilder;
if (_other!= null) {
if (_copy) {
_storedValue = null;
this.name = ((_other.name == null)?null:_other.name.newCopyBuilder(this));
this.address = _other.address;
this.email = _other.email;
this.telephone = _other.telephone;
if (_other.altIdentifiers == null) {
this.altIdentifiers = null;
} else {
this.altIdentifiers = new ArrayList<>();
for (String _item: _other.altIdentifiers) {
this.altIdentifiers.add(((_item == null)?null:new Buildable.PrimitiveBuildable(_item)));
}
}
this.ivoId = _other.ivoId;
} else {
_storedValue = _other;
}
} else {
_storedValue = null;
}
}
public Builder(final _B _parentBuilder, final Contact _other, final boolean _copy, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
this._parentBuilder = _parentBuilder;
if (_other!= null) {
if (_copy) {
_storedValue = null;
final PropertyTree namePropertyTree = ((_propertyTree == null)?null:_propertyTree.get("name"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(namePropertyTree!= null):((namePropertyTree == null)||(!namePropertyTree.isLeaf())))) {
this.name = ((_other.name == null)?null:_other.name.newCopyBuilder(this, namePropertyTree, _propertyTreeUse));
}
final PropertyTree addressPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("address"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(addressPropertyTree!= null):((addressPropertyTree == null)||(!addressPropertyTree.isLeaf())))) {
this.address = _other.address;
}
final PropertyTree emailPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("email"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(emailPropertyTree!= null):((emailPropertyTree == null)||(!emailPropertyTree.isLeaf())))) {
this.email = _other.email;
}
final PropertyTree telephonePropertyTree = ((_propertyTree == null)?null:_propertyTree.get("telephone"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(telephonePropertyTree!= null):((telephonePropertyTree == null)||(!telephonePropertyTree.isLeaf())))) {
this.telephone = _other.telephone;
}
final PropertyTree altIdentifiersPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("altIdentifiers"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(altIdentifiersPropertyTree!= null):((altIdentifiersPropertyTree == null)||(!altIdentifiersPropertyTree.isLeaf())))) {
if (_other.altIdentifiers == null) {
this.altIdentifiers = null;
} else {
this.altIdentifiers = new ArrayList<>();
for (String _item: _other.altIdentifiers) {
this.altIdentifiers.add(((_item == null)?null:new Buildable.PrimitiveBuildable(_item)));
}
}
}
final PropertyTree ivoIdPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("ivoId"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(ivoIdPropertyTree!= null):((ivoIdPropertyTree == null)||(!ivoIdPropertyTree.isLeaf())))) {
this.ivoId = _other.ivoId;
}
} else {
_storedValue = _other;
}
} else {
_storedValue = null;
}
}
public _B end() {
return this._parentBuilder;
}
protected<_P extends Contact >_P init(final _P _product) {
_product.name = ((this.name == null)?null:this.name.build());
_product.address = this.address;
_product.email = this.email;
_product.telephone = this.telephone;
if (this.altIdentifiers!= null) {
final List altIdentifiers = new ArrayList<>(this.altIdentifiers.size());
for (Buildable _item: this.altIdentifiers) {
altIdentifiers.add(((String) _item.build()));
}
_product.altIdentifiers = altIdentifiers;
}
_product.ivoId = this.ivoId;
return _product;
}
/**
* Sets the new value of "name" (any previous value will be replaced)
*
* @param name
* New value of the "name" property.
*/
public Contact.Builder<_B> withName(final ResourceName name) {
this.name = ((name == null)?null:new ResourceName.Builder<>(this, name, false));
return this;
}
/**
* Returns the existing builder or a new builder to build the value of the "name"
* property.
* Use {@link org.javastro.ivoa.entities.resource.ResourceName.Builder#end()} to
* return to the current builder.
*
* @return
* A new builder to build the value of the "name" property.
* Use {@link org.javastro.ivoa.entities.resource.ResourceName.Builder#end()} to
* return to the current builder.
*/
public ResourceName.Builder extends Contact.Builder<_B>> withName() {
if (this.name!= null) {
return this.name;
}
return this.name = new ResourceName.Builder<>(this, null, false);
}
/**
* Sets the new value of "address" (any previous value will be replaced)
*
* @param address
* New value of the "address" property.
*/
public Contact.Builder<_B> withAddress(final String address) {
this.address = address;
return this;
}
/**
* Sets the new value of "email" (any previous value will be replaced)
*
* @param email
* New value of the "email" property.
*/
public Contact.Builder<_B> withEmail(final String email) {
this.email = email;
return this;
}
/**
* Sets the new value of "telephone" (any previous value will be replaced)
*
* @param telephone
* New value of the "telephone" property.
*/
public Contact.Builder<_B> withTelephone(final String telephone) {
this.telephone = telephone;
return this;
}
/**
* Adds the given items to the value of "altIdentifiers"
*
* @param altIdentifiers
* Items to add to the value of the "altIdentifiers" property
*/
public Contact.Builder<_B> addAltIdentifiers(final Iterable extends String> altIdentifiers) {
if (altIdentifiers!= null) {
if (this.altIdentifiers == null) {
this.altIdentifiers = new ArrayList<>();
}
for (String _item: altIdentifiers) {
this.altIdentifiers.add(new Buildable.PrimitiveBuildable(_item));
}
}
return this;
}
/**
* Sets the new value of "altIdentifiers" (any previous value will be replaced)
*
* @param altIdentifiers
* New value of the "altIdentifiers" property.
*/
public Contact.Builder<_B> withAltIdentifiers(final Iterable extends String> altIdentifiers) {
if (this.altIdentifiers!= null) {
this.altIdentifiers.clear();
}
return addAltIdentifiers(altIdentifiers);
}
/**
* Adds the given items to the value of "altIdentifiers"
*
* @param altIdentifiers
* Items to add to the value of the "altIdentifiers" property
*/
public Contact.Builder<_B> addAltIdentifiers(String... altIdentifiers) {
addAltIdentifiers(Arrays.asList(altIdentifiers));
return this;
}
/**
* Sets the new value of "altIdentifiers" (any previous value will be replaced)
*
* @param altIdentifiers
* New value of the "altIdentifiers" property.
*/
public Contact.Builder<_B> withAltIdentifiers(String... altIdentifiers) {
withAltIdentifiers(Arrays.asList(altIdentifiers));
return this;
}
/**
* Sets the new value of "ivoId" (any previous value will be replaced)
*
* @param ivoId
* New value of the "ivoId" property.
*/
public Contact.Builder<_B> withIvoId(final String ivoId) {
this.ivoId = ivoId;
return this;
}
@Override
public Contact build() {
if (_storedValue == null) {
return this.init(new Contact());
} else {
return ((Contact) _storedValue);
}
}
public Contact.Builder<_B> copyOf(final Contact _other) {
_other.copyTo(this);
return this;
}
public Contact.Builder<_B> copyOf(final Contact.Builder _other) {
return copyOf(_other.build());
}
}
public class Modifier {
public void setName(final ResourceName name) {
Contact.this.setName(name);
}
public void setAddress(final String address) {
Contact.this.setAddress(address);
}
public void setEmail(final String email) {
Contact.this.setEmail(email);
}
public void setTelephone(final String telephone) {
Contact.this.setTelephone(telephone);
}
public List getAltIdentifiers() {
if (Contact.this.altIdentifiers == null) {
Contact.this.altIdentifiers = new ArrayList<>();
}
return Contact.this.altIdentifiers;
}
public void setIvoId(final String ivoId) {
Contact.this.setIvoId(ivoId);
}
}
public static class PropInfo {
public static final transient SinglePropertyInfo NAME = new SinglePropertyInfo("name", Contact.class, ResourceName.class, false, null, new QName("", "name"), new QName("http://www.ivoa.net/xml/VOResource/v1.0", "ResourceName"), false) {
@Override
public ResourceName get(final Contact _instance_) {
return ((_instance_ == null)?null:_instance_.name);
}
@Override
public void set(final Contact _instance_, final ResourceName _value_) {
if (_instance_!= null) {
_instance_.name = _value_;
}
}
}
;
public static final transient SinglePropertyInfo ADDRESS = new SinglePropertyInfo("address", Contact.class, String.class, false, null, new QName("", "address"), new QName("http://www.w3.org/2001/XMLSchema", "token"), false) {
@Override
public String get(final Contact _instance_) {
return ((_instance_ == null)?null:_instance_.address);
}
@Override
public void set(final Contact _instance_, final String _value_) {
if (_instance_!= null) {
_instance_.address = _value_;
}
}
}
;
public static final transient SinglePropertyInfo EMAIL = new SinglePropertyInfo("email", Contact.class, String.class, false, null, new QName("", "email"), new QName("http://www.w3.org/2001/XMLSchema", "token"), false) {
@Override
public String get(final Contact _instance_) {
return ((_instance_ == null)?null:_instance_.email);
}
@Override
public void set(final Contact _instance_, final String _value_) {
if (_instance_!= null) {
_instance_.email = _value_;
}
}
}
;
public static final transient SinglePropertyInfo TELEPHONE = new SinglePropertyInfo("telephone", Contact.class, String.class, false, null, new QName("", "telephone"), new QName("http://www.w3.org/2001/XMLSchema", "token"), false) {
@Override
public String get(final Contact _instance_) {
return ((_instance_ == null)?null:_instance_.telephone);
}
@Override
public void set(final Contact _instance_, final String _value_) {
if (_instance_!= null) {
_instance_.telephone = _value_;
}
}
}
;
public static final transient CollectionPropertyInfo ALT_IDENTIFIERS = new CollectionPropertyInfo("altIdentifiers", Contact.class, String.class, true, null, new QName("", "altIdentifier"), new QName("http://www.w3.org/2001/XMLSchema", "anyURI"), false) {
@Override
public List get(final Contact _instance_) {
return ((_instance_ == null)?null:_instance_.altIdentifiers);
}
@Override
public void set(final Contact _instance_, final List _value_) {
if (_instance_!= null) {
_instance_.altIdentifiers = _value_;
}
}
}
;
public static final transient SinglePropertyInfo IVO_ID = new SinglePropertyInfo("ivoId", Contact.class, String.class, false, null, new QName("", "ivo-id"), new QName("http://www.ivoa.net/xml/VOResource/v1.0", "IdentifierURI"), true) {
@Override
public String get(final Contact _instance_) {
return ((_instance_ == null)?null:_instance_.ivoId);
}
@Override
public void set(final Contact _instance_, final String _value_) {
if (_instance_!= null) {
_instance_.ivoId = _value_;
}
}
}
;
}
public static class Select
extends Contact.Selector
{
Select() {
super(null, null, null);
}
public static Contact.Select _root() {
return new Contact.Select();
}
}
public static class Selector , TParent >
extends com.kscs.util.jaxb.Selector
{
private ResourceName.Selector> name = null;
private com.kscs.util.jaxb.Selector> address = null;
private com.kscs.util.jaxb.Selector> email = null;
private com.kscs.util.jaxb.Selector> telephone = null;
private com.kscs.util.jaxb.Selector> altIdentifiers = null;
private com.kscs.util.jaxb.Selector> ivoId = null;
public Selector(final TRoot root, final TParent parent, final String propertyName) {
super(root, parent, propertyName);
}
@Override
public Map buildChildren() {
final Map products = new HashMap<>();
products.putAll(super.buildChildren());
if (this.name!= null) {
products.put("name", this.name.init());
}
if (this.address!= null) {
products.put("address", this.address.init());
}
if (this.email!= null) {
products.put("email", this.email.init());
}
if (this.telephone!= null) {
products.put("telephone", this.telephone.init());
}
if (this.altIdentifiers!= null) {
products.put("altIdentifiers", this.altIdentifiers.init());
}
if (this.ivoId!= null) {
products.put("ivoId", this.ivoId.init());
}
return products;
}
public ResourceName.Selector> name() {
return ((this.name == null)?this.name = new ResourceName.Selector<>(this._root, this, "name"):this.name);
}
public com.kscs.util.jaxb.Selector> address() {
return ((this.address == null)?this.address = new com.kscs.util.jaxb.Selector<>(this._root, this, "address"):this.address);
}
public com.kscs.util.jaxb.Selector> email() {
return ((this.email == null)?this.email = new com.kscs.util.jaxb.Selector<>(this._root, this, "email"):this.email);
}
public com.kscs.util.jaxb.Selector> telephone() {
return ((this.telephone == null)?this.telephone = new com.kscs.util.jaxb.Selector<>(this._root, this, "telephone"):this.telephone);
}
public com.kscs.util.jaxb.Selector> altIdentifiers() {
return ((this.altIdentifiers == null)?this.altIdentifiers = new com.kscs.util.jaxb.Selector<>(this._root, this, "altIdentifiers"):this.altIdentifiers);
}
public com.kscs.util.jaxb.Selector> ivoId() {
return ((this.ivoId == null)?this.ivoId = new com.kscs.util.jaxb.Selector<>(this._root, this, "ivoId"):this.ivoId);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy