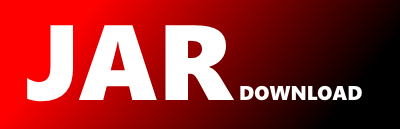
org.javastro.ivoa.entities.resource.Content Maven / Gradle / Ivy
package org.javastro.ivoa.entities.resource;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import javax.xml.namespace.QName;
import com.kscs.util.jaxb.Buildable;
import com.kscs.util.jaxb.CollectionProperty;
import com.kscs.util.jaxb.CollectionPropertyInfo;
import com.kscs.util.jaxb.CollectionPropertyInfo;
import com.kscs.util.jaxb.CollectionPropertyInfo;
import com.kscs.util.jaxb.Copyable;
import com.kscs.util.jaxb.PartialCopyable;
import com.kscs.util.jaxb.PropertyTree;
import com.kscs.util.jaxb.PropertyTreeUse;
import com.kscs.util.jaxb.PropertyVisitor;
import com.kscs.util.jaxb.SingleProperty;
import com.kscs.util.jaxb.SinglePropertyInfo;
import com.kscs.util.jaxb.SinglePropertyInfo;
import com.kscs.util.jaxb.SinglePropertyInfo;
import jakarta.annotation.Generated;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlSchemaType;
import jakarta.xml.bind.annotation.XmlType;
import jakarta.xml.bind.annotation.adapters.CollapsedStringAdapter;
import jakarta.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import org.jvnet.jaxb.lang.JAXBMergeStrategy;
import org.jvnet.jaxb.lang.JAXBToStringStrategy;
import org.jvnet.jaxb.lang.MergeFrom;
import org.jvnet.jaxb.lang.MergeStrategy;
import org.jvnet.jaxb.lang.ToString;
import org.jvnet.jaxb.lang.ToStringStrategy;
import org.jvnet.jaxb.locator.ObjectLocator;
import org.jvnet.jaxb.locator.util.LocatorUtils;
/**
* Information regarding the general content of a resource
*
* Java class for Content complex type
.
*
* The following schema fragment specifies the expected content contained within this class.
*
* {@code
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
* }
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "Content", propOrder = {
"subjects",
"description",
"source",
"referenceURL",
"types",
"contentLevels",
"relationships"
})
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public class Content implements Cloneable, Copyable, PartialCopyable, MergeFrom, ToString
{
/**
* Terms for Subject should be drawn from the Unified
* Astronomy Thesaurus (http://astrothesaurus.org).
*
*/
@XmlElement(name = "subject", required = true)
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
@XmlSchemaType(name = "token")
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
protected List subjects;
/**
* The description may include but is not limited to an abstract,
* table of contents, reference to a graphical representation of
* content or a free-text account of the content.
*
* Note that description is xs:string-typed, which means that
* whitespace is considered significant. Clients should
* render empty lines as paragraph boundaries and ideally
* refrain from reflowing material that looks formatted (i.e.,
* is broken to about 80-character lines).
*
*/
@XmlElement(required = true)
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
protected String description;
/**
* This is intended to point to an article in the published
* literature. An ADS Bibcode is recommended as a value when
* available.
*
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
protected Source source;
/**
* URL pointing to a human-readable document describing this
* resource.
*
*/
@XmlElement(required = true)
@XmlSchemaType(name = "anyURI")
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
protected String referenceURL;
/**
* Nature or genre of the content of the resource. Values for
* type should be taken from the controlled vocabulary
* http://www.ivoa.net/rdf/voresource/content_type
*
*/
@XmlElement(name = "type")
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
@XmlSchemaType(name = "token")
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
protected List types;
/**
* Description of the content level or intended audience.
* Values for contentLevel should be taken from the controlled
* vocabulary
* http://www.ivoa.net/rdf/voresource/content_level.
*
*/
@XmlElement(name = "contentLevel")
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
@XmlSchemaType(name = "token")
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
protected List contentLevels;
/**
* a description of a relationship to another resource.
*
*/
@XmlElement(name = "relationship")
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
protected List relationships;
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
protected transient Content.Modifier __cachedModifier__;
/**
* Default no-arg constructor
*
*/
public Content() {
super();
}
/**
* Fully-initialising value constructor
*
*/
public Content(final List subjects, final String description, final Source source, final String referenceURL, final List types, final List contentLevels, final List relationships) {
this.subjects = subjects;
this.description = description;
this.source = source;
this.referenceURL = referenceURL;
this.types = types;
this.contentLevels = contentLevels;
this.relationships = relationships;
}
/**
* Terms for Subject should be drawn from the Unified
* Astronomy Thesaurus (http://astrothesaurus.org).
*
* Gets the value of the subjects property.
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the subjects property.
*
*
* For example, to add a new item, do as follows:
*
*
* getSubjects().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link String }
*
*
*
* @return
* The value of the subjects property.
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public List getSubjects() {
if (subjects == null) {
subjects = new ArrayList<>();
}
return this.subjects;
}
/**
* The description may include but is not limited to an abstract,
* table of contents, reference to a graphical representation of
* content or a free-text account of the content.
*
* Note that description is xs:string-typed, which means that
* whitespace is considered significant. Clients should
* render empty lines as paragraph boundaries and ideally
* refrain from reflowing material that looks formatted (i.e.,
* is broken to about 80-character lines).
*
* @return
* possible object is
* {@link String }
*
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public String getDescription() {
return description;
}
/**
* Sets the value of the description property.
*
* @param value
* allowed object is
* {@link String }
*
* @see #getDescription()
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void setDescription(String value) {
this.description = value;
}
/**
* This is intended to point to an article in the published
* literature. An ADS Bibcode is recommended as a value when
* available.
*
* @return
* possible object is
* {@link Source }
*
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Source getSource() {
return source;
}
/**
* Sets the value of the source property.
*
* @param value
* allowed object is
* {@link Source }
*
* @see #getSource()
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void setSource(Source value) {
this.source = value;
}
/**
* URL pointing to a human-readable document describing this
* resource.
*
* @return
* possible object is
* {@link String }
*
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public String getReferenceURL() {
return referenceURL;
}
/**
* Sets the value of the referenceURL property.
*
* @param value
* allowed object is
* {@link String }
*
* @see #getReferenceURL()
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void setReferenceURL(String value) {
this.referenceURL = value;
}
/**
* Nature or genre of the content of the resource. Values for
* type should be taken from the controlled vocabulary
* http://www.ivoa.net/rdf/voresource/content_type
*
* Gets the value of the types property.
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the types property.
*
*
* For example, to add a new item, do as follows:
*
*
* getTypes().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link String }
*
*
*
* @return
* The value of the types property.
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public List getTypes() {
if (types == null) {
types = new ArrayList<>();
}
return this.types;
}
/**
* Description of the content level or intended audience.
* Values for contentLevel should be taken from the controlled
* vocabulary
* http://www.ivoa.net/rdf/voresource/content_level.
*
* Gets the value of the contentLevels property.
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the contentLevels property.
*
*
* For example, to add a new item, do as follows:
*
*
* getContentLevels().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link String }
*
*
*
* @return
* The value of the contentLevels property.
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public List getContentLevels() {
if (contentLevels == null) {
contentLevels = new ArrayList<>();
}
return this.contentLevels;
}
/**
* a description of a relationship to another resource.
*
* Gets the value of the relationships property.
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the relationships property.
*
*
* For example, to add a new item, do as follows:
*
*
* getRelationships().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Relationship }
*
*
*
* @return
* The value of the relationships property.
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public List getRelationships() {
if (relationships == null) {
relationships = new ArrayList<>();
}
return this.relationships;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public boolean equals(Object object) {
if ((object == null)||(this.getClass()!= object.getClass())) {
return false;
}
if (this == object) {
return true;
}
final Content that = ((Content) object);
{
List leftSubjects;
leftSubjects = (((this.subjects!= null)&&(!this.subjects.isEmpty()))?this.getSubjects():null);
List rightSubjects;
rightSubjects = (((that.subjects!= null)&&(!that.subjects.isEmpty()))?that.getSubjects():null);
if ((this.subjects!= null)&&(!this.subjects.isEmpty())) {
if ((that.subjects!= null)&&(!that.subjects.isEmpty())) {
if (!leftSubjects.equals(rightSubjects)) {
return false;
}
} else {
return false;
}
} else {
if ((that.subjects!= null)&&(!that.subjects.isEmpty())) {
return false;
}
}
}
{
String leftDescription;
leftDescription = this.getDescription();
String rightDescription;
rightDescription = that.getDescription();
if (this.description!= null) {
if (that.description!= null) {
if (!leftDescription.equals(rightDescription)) {
return false;
}
} else {
return false;
}
} else {
if (that.description!= null) {
return false;
}
}
}
{
Source leftSource;
leftSource = this.getSource();
Source rightSource;
rightSource = that.getSource();
if (this.source!= null) {
if (that.source!= null) {
if (!leftSource.equals(rightSource)) {
return false;
}
} else {
return false;
}
} else {
if (that.source!= null) {
return false;
}
}
}
{
String leftReferenceURL;
leftReferenceURL = this.getReferenceURL();
String rightReferenceURL;
rightReferenceURL = that.getReferenceURL();
if (this.referenceURL!= null) {
if (that.referenceURL!= null) {
if (!leftReferenceURL.equals(rightReferenceURL)) {
return false;
}
} else {
return false;
}
} else {
if (that.referenceURL!= null) {
return false;
}
}
}
{
List leftTypes;
leftTypes = (((this.types!= null)&&(!this.types.isEmpty()))?this.getTypes():null);
List rightTypes;
rightTypes = (((that.types!= null)&&(!that.types.isEmpty()))?that.getTypes():null);
if ((this.types!= null)&&(!this.types.isEmpty())) {
if ((that.types!= null)&&(!that.types.isEmpty())) {
if (!leftTypes.equals(rightTypes)) {
return false;
}
} else {
return false;
}
} else {
if ((that.types!= null)&&(!that.types.isEmpty())) {
return false;
}
}
}
{
List leftContentLevels;
leftContentLevels = (((this.contentLevels!= null)&&(!this.contentLevels.isEmpty()))?this.getContentLevels():null);
List rightContentLevels;
rightContentLevels = (((that.contentLevels!= null)&&(!that.contentLevels.isEmpty()))?that.getContentLevels():null);
if ((this.contentLevels!= null)&&(!this.contentLevels.isEmpty())) {
if ((that.contentLevels!= null)&&(!that.contentLevels.isEmpty())) {
if (!leftContentLevels.equals(rightContentLevels)) {
return false;
}
} else {
return false;
}
} else {
if ((that.contentLevels!= null)&&(!that.contentLevels.isEmpty())) {
return false;
}
}
}
{
List leftRelationships;
leftRelationships = (((this.relationships!= null)&&(!this.relationships.isEmpty()))?this.getRelationships():null);
List rightRelationships;
rightRelationships = (((that.relationships!= null)&&(!that.relationships.isEmpty()))?that.getRelationships():null);
if ((this.relationships!= null)&&(!this.relationships.isEmpty())) {
if ((that.relationships!= null)&&(!that.relationships.isEmpty())) {
if (!leftRelationships.equals(rightRelationships)) {
return false;
}
} else {
return false;
}
} else {
if ((that.relationships!= null)&&(!that.relationships.isEmpty())) {
return false;
}
}
}
return true;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public int hashCode() {
int currentHashCode = 1;
{
currentHashCode = (currentHashCode* 31);
List theSubjects;
theSubjects = (((this.subjects!= null)&&(!this.subjects.isEmpty()))?this.getSubjects():null);
if ((this.subjects!= null)&&(!this.subjects.isEmpty())) {
currentHashCode += theSubjects.hashCode();
}
}
{
currentHashCode = (currentHashCode* 31);
String theDescription;
theDescription = this.getDescription();
if (this.description!= null) {
currentHashCode += theDescription.hashCode();
}
}
{
currentHashCode = (currentHashCode* 31);
Source theSource;
theSource = this.getSource();
if (this.source!= null) {
currentHashCode += theSource.hashCode();
}
}
{
currentHashCode = (currentHashCode* 31);
String theReferenceURL;
theReferenceURL = this.getReferenceURL();
if (this.referenceURL!= null) {
currentHashCode += theReferenceURL.hashCode();
}
}
{
currentHashCode = (currentHashCode* 31);
List theTypes;
theTypes = (((this.types!= null)&&(!this.types.isEmpty()))?this.getTypes():null);
if ((this.types!= null)&&(!this.types.isEmpty())) {
currentHashCode += theTypes.hashCode();
}
}
{
currentHashCode = (currentHashCode* 31);
List theContentLevels;
theContentLevels = (((this.contentLevels!= null)&&(!this.contentLevels.isEmpty()))?this.getContentLevels():null);
if ((this.contentLevels!= null)&&(!this.contentLevels.isEmpty())) {
currentHashCode += theContentLevels.hashCode();
}
}
{
currentHashCode = (currentHashCode* 31);
List theRelationships;
theRelationships = (((this.relationships!= null)&&(!this.relationships.isEmpty()))?this.getRelationships():null);
if ((this.relationships!= null)&&(!this.relationships.isEmpty())) {
currentHashCode += theRelationships.hashCode();
}
}
return currentHashCode;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public String toString() {
final ToStringStrategy strategy = JAXBToStringStrategy.getInstance();
final StringBuilder buffer = new StringBuilder();
append(null, buffer, strategy);
return buffer.toString();
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public StringBuilder append(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
strategy.appendStart(locator, this, buffer);
appendFields(locator, buffer, strategy);
strategy.appendEnd(locator, this, buffer);
return buffer;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public StringBuilder appendFields(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
{
List theSubjects;
theSubjects = (((this.subjects!= null)&&(!this.subjects.isEmpty()))?this.getSubjects():null);
strategy.appendField(locator, this, "subjects", buffer, theSubjects, ((this.subjects!= null)&&(!this.subjects.isEmpty())));
}
{
String theDescription;
theDescription = this.getDescription();
strategy.appendField(locator, this, "description", buffer, theDescription, (this.description!= null));
}
{
Source theSource;
theSource = this.getSource();
strategy.appendField(locator, this, "source", buffer, theSource, (this.source!= null));
}
{
String theReferenceURL;
theReferenceURL = this.getReferenceURL();
strategy.appendField(locator, this, "referenceURL", buffer, theReferenceURL, (this.referenceURL!= null));
}
{
List theTypes;
theTypes = (((this.types!= null)&&(!this.types.isEmpty()))?this.getTypes():null);
strategy.appendField(locator, this, "types", buffer, theTypes, ((this.types!= null)&&(!this.types.isEmpty())));
}
{
List theContentLevels;
theContentLevels = (((this.contentLevels!= null)&&(!this.contentLevels.isEmpty()))?this.getContentLevels():null);
strategy.appendField(locator, this, "contentLevels", buffer, theContentLevels, ((this.contentLevels!= null)&&(!this.contentLevels.isEmpty())));
}
{
List theRelationships;
theRelationships = (((this.relationships!= null)&&(!this.relationships.isEmpty()))?this.getRelationships():null);
strategy.appendField(locator, this, "relationships", buffer, theRelationships, ((this.relationships!= null)&&(!this.relationships.isEmpty())));
}
return buffer;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void mergeFrom(Object left, Object right) {
final MergeStrategy strategy = JAXBMergeStrategy.getInstance();
mergeFrom(null, null, left, right, strategy);
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void mergeFrom(ObjectLocator leftLocator, ObjectLocator rightLocator, Object left, Object right, MergeStrategy strategy) {
if (right instanceof Content) {
final Content target = this;
final Content leftObject = ((Content) left);
final Content rightObject = ((Content) right);
{
Boolean subjectsShouldBeMergedAndSet = strategy.shouldBeMergedAndSet(leftLocator, rightLocator, ((leftObject.subjects!= null)&&(!leftObject.subjects.isEmpty())), ((rightObject.subjects!= null)&&(!rightObject.subjects.isEmpty())));
if (subjectsShouldBeMergedAndSet == Boolean.TRUE) {
List lhsSubjects;
lhsSubjects = (((leftObject.subjects!= null)&&(!leftObject.subjects.isEmpty()))?leftObject.getSubjects():null);
List rhsSubjects;
rhsSubjects = (((rightObject.subjects!= null)&&(!rightObject.subjects.isEmpty()))?rightObject.getSubjects():null);
List mergedSubjects = ((List ) strategy.merge(LocatorUtils.property(leftLocator, "subjects", lhsSubjects), LocatorUtils.property(rightLocator, "subjects", rhsSubjects), lhsSubjects, rhsSubjects, ((leftObject.subjects!= null)&&(!leftObject.subjects.isEmpty())), ((rightObject.subjects!= null)&&(!rightObject.subjects.isEmpty()))));
target.subjects = null;
if (mergedSubjects!= null) {
List uniqueSubjectsl = target.getSubjects();
uniqueSubjectsl.addAll(mergedSubjects);
}
} else {
if (subjectsShouldBeMergedAndSet == Boolean.FALSE) {
target.subjects = null;
}
}
}
{
Boolean descriptionShouldBeMergedAndSet = strategy.shouldBeMergedAndSet(leftLocator, rightLocator, (leftObject.description!= null), (rightObject.description!= null));
if (descriptionShouldBeMergedAndSet == Boolean.TRUE) {
String lhsDescription;
lhsDescription = leftObject.getDescription();
String rhsDescription;
rhsDescription = rightObject.getDescription();
String mergedDescription = ((String) strategy.merge(LocatorUtils.property(leftLocator, "description", lhsDescription), LocatorUtils.property(rightLocator, "description", rhsDescription), lhsDescription, rhsDescription, (leftObject.description!= null), (rightObject.description!= null)));
target.setDescription(mergedDescription);
} else {
if (descriptionShouldBeMergedAndSet == Boolean.FALSE) {
target.description = null;
}
}
}
{
Boolean sourceShouldBeMergedAndSet = strategy.shouldBeMergedAndSet(leftLocator, rightLocator, (leftObject.source!= null), (rightObject.source!= null));
if (sourceShouldBeMergedAndSet == Boolean.TRUE) {
Source lhsSource;
lhsSource = leftObject.getSource();
Source rhsSource;
rhsSource = rightObject.getSource();
Source mergedSource = ((Source) strategy.merge(LocatorUtils.property(leftLocator, "source", lhsSource), LocatorUtils.property(rightLocator, "source", rhsSource), lhsSource, rhsSource, (leftObject.source!= null), (rightObject.source!= null)));
target.setSource(mergedSource);
} else {
if (sourceShouldBeMergedAndSet == Boolean.FALSE) {
target.source = null;
}
}
}
{
Boolean referenceURLShouldBeMergedAndSet = strategy.shouldBeMergedAndSet(leftLocator, rightLocator, (leftObject.referenceURL!= null), (rightObject.referenceURL!= null));
if (referenceURLShouldBeMergedAndSet == Boolean.TRUE) {
String lhsReferenceURL;
lhsReferenceURL = leftObject.getReferenceURL();
String rhsReferenceURL;
rhsReferenceURL = rightObject.getReferenceURL();
String mergedReferenceURL = ((String) strategy.merge(LocatorUtils.property(leftLocator, "referenceURL", lhsReferenceURL), LocatorUtils.property(rightLocator, "referenceURL", rhsReferenceURL), lhsReferenceURL, rhsReferenceURL, (leftObject.referenceURL!= null), (rightObject.referenceURL!= null)));
target.setReferenceURL(mergedReferenceURL);
} else {
if (referenceURLShouldBeMergedAndSet == Boolean.FALSE) {
target.referenceURL = null;
}
}
}
{
Boolean typesShouldBeMergedAndSet = strategy.shouldBeMergedAndSet(leftLocator, rightLocator, ((leftObject.types!= null)&&(!leftObject.types.isEmpty())), ((rightObject.types!= null)&&(!rightObject.types.isEmpty())));
if (typesShouldBeMergedAndSet == Boolean.TRUE) {
List lhsTypes;
lhsTypes = (((leftObject.types!= null)&&(!leftObject.types.isEmpty()))?leftObject.getTypes():null);
List rhsTypes;
rhsTypes = (((rightObject.types!= null)&&(!rightObject.types.isEmpty()))?rightObject.getTypes():null);
List mergedTypes = ((List ) strategy.merge(LocatorUtils.property(leftLocator, "types", lhsTypes), LocatorUtils.property(rightLocator, "types", rhsTypes), lhsTypes, rhsTypes, ((leftObject.types!= null)&&(!leftObject.types.isEmpty())), ((rightObject.types!= null)&&(!rightObject.types.isEmpty()))));
target.types = null;
if (mergedTypes!= null) {
List uniqueTypesl = target.getTypes();
uniqueTypesl.addAll(mergedTypes);
}
} else {
if (typesShouldBeMergedAndSet == Boolean.FALSE) {
target.types = null;
}
}
}
{
Boolean contentLevelsShouldBeMergedAndSet = strategy.shouldBeMergedAndSet(leftLocator, rightLocator, ((leftObject.contentLevels!= null)&&(!leftObject.contentLevels.isEmpty())), ((rightObject.contentLevels!= null)&&(!rightObject.contentLevels.isEmpty())));
if (contentLevelsShouldBeMergedAndSet == Boolean.TRUE) {
List lhsContentLevels;
lhsContentLevels = (((leftObject.contentLevels!= null)&&(!leftObject.contentLevels.isEmpty()))?leftObject.getContentLevels():null);
List rhsContentLevels;
rhsContentLevels = (((rightObject.contentLevels!= null)&&(!rightObject.contentLevels.isEmpty()))?rightObject.getContentLevels():null);
List mergedContentLevels = ((List ) strategy.merge(LocatorUtils.property(leftLocator, "contentLevels", lhsContentLevels), LocatorUtils.property(rightLocator, "contentLevels", rhsContentLevels), lhsContentLevels, rhsContentLevels, ((leftObject.contentLevels!= null)&&(!leftObject.contentLevels.isEmpty())), ((rightObject.contentLevels!= null)&&(!rightObject.contentLevels.isEmpty()))));
target.contentLevels = null;
if (mergedContentLevels!= null) {
List uniqueContentLevelsl = target.getContentLevels();
uniqueContentLevelsl.addAll(mergedContentLevels);
}
} else {
if (contentLevelsShouldBeMergedAndSet == Boolean.FALSE) {
target.contentLevels = null;
}
}
}
{
Boolean relationshipsShouldBeMergedAndSet = strategy.shouldBeMergedAndSet(leftLocator, rightLocator, ((leftObject.relationships!= null)&&(!leftObject.relationships.isEmpty())), ((rightObject.relationships!= null)&&(!rightObject.relationships.isEmpty())));
if (relationshipsShouldBeMergedAndSet == Boolean.TRUE) {
List lhsRelationships;
lhsRelationships = (((leftObject.relationships!= null)&&(!leftObject.relationships.isEmpty()))?leftObject.getRelationships():null);
List rhsRelationships;
rhsRelationships = (((rightObject.relationships!= null)&&(!rightObject.relationships.isEmpty()))?rightObject.getRelationships():null);
List mergedRelationships = ((List ) strategy.merge(LocatorUtils.property(leftLocator, "relationships", lhsRelationships), LocatorUtils.property(rightLocator, "relationships", rhsRelationships), lhsRelationships, rhsRelationships, ((leftObject.relationships!= null)&&(!leftObject.relationships.isEmpty())), ((rightObject.relationships!= null)&&(!rightObject.relationships.isEmpty()))));
target.relationships = null;
if (mergedRelationships!= null) {
List uniqueRelationshipsl = target.getRelationships();
uniqueRelationshipsl.addAll(mergedRelationships);
}
} else {
if (relationshipsShouldBeMergedAndSet == Boolean.FALSE) {
target.relationships = null;
}
}
}
}
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Object createNewInstance() {
return new Content();
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Content clone() {
final Content _newObject;
try {
_newObject = ((Content) super.clone());
} catch (CloneNotSupportedException e) {
throw new RuntimeException(e);
}
_newObject.subjects = ((this.subjects == null)?null:new ArrayList<>(this.subjects));
_newObject.source = ((this.source == null)?null:this.source.clone());
_newObject.types = ((this.types == null)?null:new ArrayList<>(this.types));
_newObject.contentLevels = ((this.contentLevels == null)?null:new ArrayList<>(this.contentLevels));
if (this.relationships == null) {
_newObject.relationships = null;
} else {
_newObject.relationships = new ArrayList<>();
for (Relationship _item: this.relationships) {
_newObject.relationships.add(((_item == null)?null:_item.clone()));
}
}
return _newObject;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Content createCopy() {
final Content _newObject;
try {
_newObject = ((Content) super.clone());
} catch (CloneNotSupportedException e) {
throw new RuntimeException(e);
}
_newObject.subjects = ((this.subjects == null)?null:new ArrayList<>(this.subjects));
_newObject.description = this.description;
_newObject.source = ((this.source == null)?null:this.source.createCopy());
_newObject.referenceURL = this.referenceURL;
_newObject.types = ((this.types == null)?null:new ArrayList<>(this.types));
_newObject.contentLevels = ((this.contentLevels == null)?null:new ArrayList<>(this.contentLevels));
if (this.relationships == null) {
_newObject.relationships = null;
} else {
_newObject.relationships = new ArrayList<>();
for (Relationship _item: this.relationships) {
_newObject.relationships.add(((_item == null)?null:_item.createCopy()));
}
}
return _newObject;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Content createCopy(final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
final Content _newObject;
try {
_newObject = ((Content) super.clone());
} catch (CloneNotSupportedException e) {
throw new RuntimeException(e);
}
final PropertyTree subjectsPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("subjects"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(subjectsPropertyTree!= null):((subjectsPropertyTree == null)||(!subjectsPropertyTree.isLeaf())))) {
_newObject.subjects = ((this.subjects == null)?null:new ArrayList<>(this.subjects));
}
final PropertyTree descriptionPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("description"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(descriptionPropertyTree!= null):((descriptionPropertyTree == null)||(!descriptionPropertyTree.isLeaf())))) {
_newObject.description = this.description;
}
final PropertyTree sourcePropertyTree = ((_propertyTree == null)?null:_propertyTree.get("source"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(sourcePropertyTree!= null):((sourcePropertyTree == null)||(!sourcePropertyTree.isLeaf())))) {
_newObject.source = ((this.source == null)?null:this.source.createCopy(sourcePropertyTree, _propertyTreeUse));
}
final PropertyTree referenceURLPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("referenceURL"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(referenceURLPropertyTree!= null):((referenceURLPropertyTree == null)||(!referenceURLPropertyTree.isLeaf())))) {
_newObject.referenceURL = this.referenceURL;
}
final PropertyTree typesPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("types"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(typesPropertyTree!= null):((typesPropertyTree == null)||(!typesPropertyTree.isLeaf())))) {
_newObject.types = ((this.types == null)?null:new ArrayList<>(this.types));
}
final PropertyTree contentLevelsPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("contentLevels"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(contentLevelsPropertyTree!= null):((contentLevelsPropertyTree == null)||(!contentLevelsPropertyTree.isLeaf())))) {
_newObject.contentLevels = ((this.contentLevels == null)?null:new ArrayList<>(this.contentLevels));
}
final PropertyTree relationshipsPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("relationships"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(relationshipsPropertyTree!= null):((relationshipsPropertyTree == null)||(!relationshipsPropertyTree.isLeaf())))) {
if (this.relationships == null) {
_newObject.relationships = null;
} else {
_newObject.relationships = new ArrayList<>();
for (Relationship _item: this.relationships) {
_newObject.relationships.add(((_item == null)?null:_item.createCopy(relationshipsPropertyTree, _propertyTreeUse)));
}
}
}
return _newObject;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Content copyExcept(final PropertyTree _propertyTree) {
return createCopy(_propertyTree, PropertyTreeUse.EXCLUDE);
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Content copyOnly(final PropertyTree _propertyTree) {
return createCopy(_propertyTree, PropertyTreeUse.INCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Content.Modifier modifier() {
if (null == this.__cachedModifier__) {
this.__cachedModifier__ = new Content.Modifier();
}
return ((Content.Modifier) this.__cachedModifier__);
}
/**
* Copies all state of this object to a builder. This method is used by the copyOf
* method and should not be called directly by client code.
*
* @param _other
* A builder instance to which the state of this object will be copied.
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public<_B >void copyTo(final Content.Builder<_B> _other) {
if (this.subjects == null) {
_other.subjects = null;
} else {
_other.subjects = new ArrayList<>();
for (String _item: this.subjects) {
_other.subjects.add(((_item == null)?null:new Buildable.PrimitiveBuildable(_item)));
}
}
_other.description = this.description;
_other.source = ((this.source == null)?null:this.source.newCopyBuilder(_other));
_other.referenceURL = this.referenceURL;
if (this.types == null) {
_other.types = null;
} else {
_other.types = new ArrayList<>();
for (String _item: this.types) {
_other.types.add(((_item == null)?null:new Buildable.PrimitiveBuildable(_item)));
}
}
if (this.contentLevels == null) {
_other.contentLevels = null;
} else {
_other.contentLevels = new ArrayList<>();
for (String _item: this.contentLevels) {
_other.contentLevels.add(((_item == null)?null:new Buildable.PrimitiveBuildable(_item)));
}
}
if (this.relationships == null) {
_other.relationships = null;
} else {
_other.relationships = new ArrayList<>();
for (Relationship _item: this.relationships) {
_other.relationships.add(((_item == null)?null:_item.newCopyBuilder(_other)));
}
}
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public<_B >Content.Builder<_B> newCopyBuilder(final _B _parentBuilder) {
return new Content.Builder<_B>(_parentBuilder, this, true);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Content.Builder newCopyBuilder() {
return newCopyBuilder(null);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static Content.Builder builder() {
return new Content.Builder<>(null, null, false);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static<_B >Content.Builder<_B> copyOf(final Content _other) {
final Content.Builder<_B> _newBuilder = new Content.Builder<>(null, null, false);
_other.copyTo(_newBuilder);
return _newBuilder;
}
/**
* Copies all state of this object to a builder. This method is used by the copyOf
* method and should not be called directly by client code.
*
* @param _other
* A builder instance to which the state of this object will be copied.
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public<_B >void copyTo(final Content.Builder<_B> _other, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
final PropertyTree subjectsPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("subjects"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(subjectsPropertyTree!= null):((subjectsPropertyTree == null)||(!subjectsPropertyTree.isLeaf())))) {
if (this.subjects == null) {
_other.subjects = null;
} else {
_other.subjects = new ArrayList<>();
for (String _item: this.subjects) {
_other.subjects.add(((_item == null)?null:new Buildable.PrimitiveBuildable(_item)));
}
}
}
final PropertyTree descriptionPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("description"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(descriptionPropertyTree!= null):((descriptionPropertyTree == null)||(!descriptionPropertyTree.isLeaf())))) {
_other.description = this.description;
}
final PropertyTree sourcePropertyTree = ((_propertyTree == null)?null:_propertyTree.get("source"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(sourcePropertyTree!= null):((sourcePropertyTree == null)||(!sourcePropertyTree.isLeaf())))) {
_other.source = ((this.source == null)?null:this.source.newCopyBuilder(_other, sourcePropertyTree, _propertyTreeUse));
}
final PropertyTree referenceURLPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("referenceURL"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(referenceURLPropertyTree!= null):((referenceURLPropertyTree == null)||(!referenceURLPropertyTree.isLeaf())))) {
_other.referenceURL = this.referenceURL;
}
final PropertyTree typesPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("types"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(typesPropertyTree!= null):((typesPropertyTree == null)||(!typesPropertyTree.isLeaf())))) {
if (this.types == null) {
_other.types = null;
} else {
_other.types = new ArrayList<>();
for (String _item: this.types) {
_other.types.add(((_item == null)?null:new Buildable.PrimitiveBuildable(_item)));
}
}
}
final PropertyTree contentLevelsPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("contentLevels"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(contentLevelsPropertyTree!= null):((contentLevelsPropertyTree == null)||(!contentLevelsPropertyTree.isLeaf())))) {
if (this.contentLevels == null) {
_other.contentLevels = null;
} else {
_other.contentLevels = new ArrayList<>();
for (String _item: this.contentLevels) {
_other.contentLevels.add(((_item == null)?null:new Buildable.PrimitiveBuildable(_item)));
}
}
}
final PropertyTree relationshipsPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("relationships"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(relationshipsPropertyTree!= null):((relationshipsPropertyTree == null)||(!relationshipsPropertyTree.isLeaf())))) {
if (this.relationships == null) {
_other.relationships = null;
} else {
_other.relationships = new ArrayList<>();
for (Relationship _item: this.relationships) {
_other.relationships.add(((_item == null)?null:_item.newCopyBuilder(_other, relationshipsPropertyTree, _propertyTreeUse)));
}
}
}
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public<_B >Content.Builder<_B> newCopyBuilder(final _B _parentBuilder, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
return new Content.Builder<_B>(_parentBuilder, this, true, _propertyTree, _propertyTreeUse);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Content.Builder newCopyBuilder(final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
return newCopyBuilder(null, _propertyTree, _propertyTreeUse);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static<_B >Content.Builder<_B> copyOf(final Content _other, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
final Content.Builder<_B> _newBuilder = new Content.Builder<>(null, null, false);
_other.copyTo(_newBuilder, _propertyTree, _propertyTreeUse);
return _newBuilder;
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static Content.Builder copyExcept(final Content _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.EXCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static Content.Builder copyOnly(final Content _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.INCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Content visit(final PropertyVisitor _visitor_) {
_visitor_.visit(this);
_visitor_.visit(new CollectionProperty<>(Content.PropInfo.SUBJECTS, this));
_visitor_.visit(new SingleProperty<>(Content.PropInfo.DESCRIPTION, this));
if (_visitor_.visit(new SingleProperty<>(Content.PropInfo.SOURCE, this))&&(this.source!= null)) {
this.source.visit(_visitor_);
}
_visitor_.visit(new SingleProperty<>(Content.PropInfo.REFERENCE_URL, this));
_visitor_.visit(new CollectionProperty<>(Content.PropInfo.TYPES, this));
_visitor_.visit(new CollectionProperty<>(Content.PropInfo.CONTENT_LEVELS, this));
if (_visitor_.visit(new CollectionProperty<>(Content.PropInfo.RELATIONSHIPS, this))&&(this.relationships!= null)) {
for (Relationship _item_: this.relationships) {
if (_item_!= null) {
_item_.visit(_visitor_);
}
}
}
return this;
}
public static class Builder<_B >implements Buildable
{
protected final _B _parentBuilder;
protected final Content _storedValue;
private List subjects;
private String description;
private Source.Builder> source;
private String referenceURL;
private List types;
private List contentLevels;
private List>> relationships;
public Builder(final _B _parentBuilder, final Content _other, final boolean _copy) {
this._parentBuilder = _parentBuilder;
if (_other!= null) {
if (_copy) {
_storedValue = null;
if (_other.subjects == null) {
this.subjects = null;
} else {
this.subjects = new ArrayList<>();
for (String _item: _other.subjects) {
this.subjects.add(((_item == null)?null:new Buildable.PrimitiveBuildable(_item)));
}
}
this.description = _other.description;
this.source = ((_other.source == null)?null:_other.source.newCopyBuilder(this));
this.referenceURL = _other.referenceURL;
if (_other.types == null) {
this.types = null;
} else {
this.types = new ArrayList<>();
for (String _item: _other.types) {
this.types.add(((_item == null)?null:new Buildable.PrimitiveBuildable(_item)));
}
}
if (_other.contentLevels == null) {
this.contentLevels = null;
} else {
this.contentLevels = new ArrayList<>();
for (String _item: _other.contentLevels) {
this.contentLevels.add(((_item == null)?null:new Buildable.PrimitiveBuildable(_item)));
}
}
if (_other.relationships == null) {
this.relationships = null;
} else {
this.relationships = new ArrayList<>();
for (Relationship _item: _other.relationships) {
this.relationships.add(((_item == null)?null:_item.newCopyBuilder(this)));
}
}
} else {
_storedValue = _other;
}
} else {
_storedValue = null;
}
}
public Builder(final _B _parentBuilder, final Content _other, final boolean _copy, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
this._parentBuilder = _parentBuilder;
if (_other!= null) {
if (_copy) {
_storedValue = null;
final PropertyTree subjectsPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("subjects"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(subjectsPropertyTree!= null):((subjectsPropertyTree == null)||(!subjectsPropertyTree.isLeaf())))) {
if (_other.subjects == null) {
this.subjects = null;
} else {
this.subjects = new ArrayList<>();
for (String _item: _other.subjects) {
this.subjects.add(((_item == null)?null:new Buildable.PrimitiveBuildable(_item)));
}
}
}
final PropertyTree descriptionPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("description"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(descriptionPropertyTree!= null):((descriptionPropertyTree == null)||(!descriptionPropertyTree.isLeaf())))) {
this.description = _other.description;
}
final PropertyTree sourcePropertyTree = ((_propertyTree == null)?null:_propertyTree.get("source"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(sourcePropertyTree!= null):((sourcePropertyTree == null)||(!sourcePropertyTree.isLeaf())))) {
this.source = ((_other.source == null)?null:_other.source.newCopyBuilder(this, sourcePropertyTree, _propertyTreeUse));
}
final PropertyTree referenceURLPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("referenceURL"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(referenceURLPropertyTree!= null):((referenceURLPropertyTree == null)||(!referenceURLPropertyTree.isLeaf())))) {
this.referenceURL = _other.referenceURL;
}
final PropertyTree typesPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("types"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(typesPropertyTree!= null):((typesPropertyTree == null)||(!typesPropertyTree.isLeaf())))) {
if (_other.types == null) {
this.types = null;
} else {
this.types = new ArrayList<>();
for (String _item: _other.types) {
this.types.add(((_item == null)?null:new Buildable.PrimitiveBuildable(_item)));
}
}
}
final PropertyTree contentLevelsPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("contentLevels"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(contentLevelsPropertyTree!= null):((contentLevelsPropertyTree == null)||(!contentLevelsPropertyTree.isLeaf())))) {
if (_other.contentLevels == null) {
this.contentLevels = null;
} else {
this.contentLevels = new ArrayList<>();
for (String _item: _other.contentLevels) {
this.contentLevels.add(((_item == null)?null:new Buildable.PrimitiveBuildable(_item)));
}
}
}
final PropertyTree relationshipsPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("relationships"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(relationshipsPropertyTree!= null):((relationshipsPropertyTree == null)||(!relationshipsPropertyTree.isLeaf())))) {
if (_other.relationships == null) {
this.relationships = null;
} else {
this.relationships = new ArrayList<>();
for (Relationship _item: _other.relationships) {
this.relationships.add(((_item == null)?null:_item.newCopyBuilder(this, relationshipsPropertyTree, _propertyTreeUse)));
}
}
}
} else {
_storedValue = _other;
}
} else {
_storedValue = null;
}
}
public _B end() {
return this._parentBuilder;
}
protected<_P extends Content >_P init(final _P _product) {
if (this.subjects!= null) {
final List subjects = new ArrayList<>(this.subjects.size());
for (Buildable _item: this.subjects) {
subjects.add(((String) _item.build()));
}
_product.subjects = subjects;
}
_product.description = this.description;
_product.source = ((this.source == null)?null:this.source.build());
_product.referenceURL = this.referenceURL;
if (this.types!= null) {
final List types = new ArrayList<>(this.types.size());
for (Buildable _item: this.types) {
types.add(((String) _item.build()));
}
_product.types = types;
}
if (this.contentLevels!= null) {
final List contentLevels = new ArrayList<>(this.contentLevels.size());
for (Buildable _item: this.contentLevels) {
contentLevels.add(((String) _item.build()));
}
_product.contentLevels = contentLevels;
}
if (this.relationships!= null) {
final List relationships = new ArrayList<>(this.relationships.size());
for (Relationship.Builder> _item: this.relationships) {
relationships.add(_item.build());
}
_product.relationships = relationships;
}
return _product;
}
/**
* Adds the given items to the value of "subjects"
*
* @param subjects
* Items to add to the value of the "subjects" property
*/
public Content.Builder<_B> addSubjects(final Iterable extends String> subjects) {
if (subjects!= null) {
if (this.subjects == null) {
this.subjects = new ArrayList<>();
}
for (String _item: subjects) {
this.subjects.add(new Buildable.PrimitiveBuildable(_item));
}
}
return this;
}
/**
* Sets the new value of "subjects" (any previous value will be replaced)
*
* @param subjects
* New value of the "subjects" property.
*/
public Content.Builder<_B> withSubjects(final Iterable extends String> subjects) {
if (this.subjects!= null) {
this.subjects.clear();
}
return addSubjects(subjects);
}
/**
* Adds the given items to the value of "subjects"
*
* @param subjects
* Items to add to the value of the "subjects" property
*/
public Content.Builder<_B> addSubjects(String... subjects) {
addSubjects(Arrays.asList(subjects));
return this;
}
/**
* Sets the new value of "subjects" (any previous value will be replaced)
*
* @param subjects
* New value of the "subjects" property.
*/
public Content.Builder<_B> withSubjects(String... subjects) {
withSubjects(Arrays.asList(subjects));
return this;
}
/**
* Sets the new value of "description" (any previous value will be replaced)
*
* @param description
* New value of the "description" property.
*/
public Content.Builder<_B> withDescription(final String description) {
this.description = description;
return this;
}
/**
* Sets the new value of "source" (any previous value will be replaced)
*
* @param source
* New value of the "source" property.
*/
public Content.Builder<_B> withSource(final Source source) {
this.source = ((source == null)?null:new Source.Builder<>(this, source, false));
return this;
}
/**
* Returns the existing builder or a new builder to build the value of the "source"
* property.
* Use {@link org.javastro.ivoa.entities.resource.Source.Builder#end()} to return
* to the current builder.
*
* @return
* A new builder to build the value of the "source" property.
* Use {@link org.javastro.ivoa.entities.resource.Source.Builder#end()} to return
* to the current builder.
*/
public Source.Builder extends Content.Builder<_B>> withSource() {
if (this.source!= null) {
return this.source;
}
return this.source = new Source.Builder<>(this, null, false);
}
/**
* Sets the new value of "referenceURL" (any previous value will be replaced)
*
* @param referenceURL
* New value of the "referenceURL" property.
*/
public Content.Builder<_B> withReferenceURL(final String referenceURL) {
this.referenceURL = referenceURL;
return this;
}
/**
* Adds the given items to the value of "types"
*
* @param types
* Items to add to the value of the "types" property
*/
public Content.Builder<_B> addTypes(final Iterable extends String> types) {
if (types!= null) {
if (this.types == null) {
this.types = new ArrayList<>();
}
for (String _item: types) {
this.types.add(new Buildable.PrimitiveBuildable(_item));
}
}
return this;
}
/**
* Sets the new value of "types" (any previous value will be replaced)
*
* @param types
* New value of the "types" property.
*/
public Content.Builder<_B> withTypes(final Iterable extends String> types) {
if (this.types!= null) {
this.types.clear();
}
return addTypes(types);
}
/**
* Adds the given items to the value of "types"
*
* @param types
* Items to add to the value of the "types" property
*/
public Content.Builder<_B> addTypes(String... types) {
addTypes(Arrays.asList(types));
return this;
}
/**
* Sets the new value of "types" (any previous value will be replaced)
*
* @param types
* New value of the "types" property.
*/
public Content.Builder<_B> withTypes(String... types) {
withTypes(Arrays.asList(types));
return this;
}
/**
* Adds the given items to the value of "contentLevels"
*
* @param contentLevels
* Items to add to the value of the "contentLevels" property
*/
public Content.Builder<_B> addContentLevels(final Iterable extends String> contentLevels) {
if (contentLevels!= null) {
if (this.contentLevels == null) {
this.contentLevels = new ArrayList<>();
}
for (String _item: contentLevels) {
this.contentLevels.add(new Buildable.PrimitiveBuildable(_item));
}
}
return this;
}
/**
* Sets the new value of "contentLevels" (any previous value will be replaced)
*
* @param contentLevels
* New value of the "contentLevels" property.
*/
public Content.Builder<_B> withContentLevels(final Iterable extends String> contentLevels) {
if (this.contentLevels!= null) {
this.contentLevels.clear();
}
return addContentLevels(contentLevels);
}
/**
* Adds the given items to the value of "contentLevels"
*
* @param contentLevels
* Items to add to the value of the "contentLevels" property
*/
public Content.Builder<_B> addContentLevels(String... contentLevels) {
addContentLevels(Arrays.asList(contentLevels));
return this;
}
/**
* Sets the new value of "contentLevels" (any previous value will be replaced)
*
* @param contentLevels
* New value of the "contentLevels" property.
*/
public Content.Builder<_B> withContentLevels(String... contentLevels) {
withContentLevels(Arrays.asList(contentLevels));
return this;
}
/**
* Adds the given items to the value of "relationships"
*
* @param relationships
* Items to add to the value of the "relationships" property
*/
public Content.Builder<_B> addRelationships(final Iterable extends Relationship> relationships) {
if (relationships!= null) {
if (this.relationships == null) {
this.relationships = new ArrayList<>();
}
for (Relationship _item: relationships) {
this.relationships.add(new Relationship.Builder<>(this, _item, false));
}
}
return this;
}
/**
* Sets the new value of "relationships" (any previous value will be replaced)
*
* @param relationships
* New value of the "relationships" property.
*/
public Content.Builder<_B> withRelationships(final Iterable extends Relationship> relationships) {
if (this.relationships!= null) {
this.relationships.clear();
}
return addRelationships(relationships);
}
/**
* Adds the given items to the value of "relationships"
*
* @param relationships
* Items to add to the value of the "relationships" property
*/
public Content.Builder<_B> addRelationships(Relationship... relationships) {
addRelationships(Arrays.asList(relationships));
return this;
}
/**
* Sets the new value of "relationships" (any previous value will be replaced)
*
* @param relationships
* New value of the "relationships" property.
*/
public Content.Builder<_B> withRelationships(Relationship... relationships) {
withRelationships(Arrays.asList(relationships));
return this;
}
/**
* Returns a new builder to build an additional value of the "Relationships"
* property.
* Use {@link org.javastro.ivoa.entities.resource.Relationship.Builder#end()} to
* return to the current builder.
*
* @return
* a new builder to build an additional value of the "Relationships" property.
* Use {@link org.javastro.ivoa.entities.resource.Relationship.Builder#end()} to
* return to the current builder.
*/
public Relationship.Builder extends Content.Builder<_B>> addRelationships() {
if (this.relationships == null) {
this.relationships = new ArrayList<>();
}
final Relationship.Builder> relationships_Builder = new Relationship.Builder<>(this, null, false);
this.relationships.add(relationships_Builder);
return relationships_Builder;
}
@Override
public Content build() {
if (_storedValue == null) {
return this.init(new Content());
} else {
return ((Content) _storedValue);
}
}
public Content.Builder<_B> copyOf(final Content _other) {
_other.copyTo(this);
return this;
}
public Content.Builder<_B> copyOf(final Content.Builder _other) {
return copyOf(_other.build());
}
}
public class Modifier {
public List getSubjects() {
if (Content.this.subjects == null) {
Content.this.subjects = new ArrayList<>();
}
return Content.this.subjects;
}
public void setDescription(final String description) {
Content.this.setDescription(description);
}
public void setSource(final Source source) {
Content.this.setSource(source);
}
public void setReferenceURL(final String referenceURL) {
Content.this.setReferenceURL(referenceURL);
}
public List getTypes() {
if (Content.this.types == null) {
Content.this.types = new ArrayList<>();
}
return Content.this.types;
}
public List getContentLevels() {
if (Content.this.contentLevels == null) {
Content.this.contentLevels = new ArrayList<>();
}
return Content.this.contentLevels;
}
public List getRelationships() {
if (Content.this.relationships == null) {
Content.this.relationships = new ArrayList<>();
}
return Content.this.relationships;
}
}
public static class PropInfo {
public static final transient CollectionPropertyInfo SUBJECTS = new CollectionPropertyInfo("subjects", Content.class, String.class, true, null, new QName("", "subject"), new QName("http://www.w3.org/2001/XMLSchema", "token"), false) {
@Override
public List get(final Content _instance_) {
return ((_instance_ == null)?null:_instance_.subjects);
}
@Override
public void set(final Content _instance_, final List _value_) {
if (_instance_!= null) {
_instance_.subjects = _value_;
}
}
}
;
public static final transient SinglePropertyInfo DESCRIPTION = new SinglePropertyInfo("description", Content.class, String.class, false, null, new QName("", "description"), new QName("http://www.w3.org/2001/XMLSchema", "string"), false) {
@Override
public String get(final Content _instance_) {
return ((_instance_ == null)?null:_instance_.description);
}
@Override
public void set(final Content _instance_, final String _value_) {
if (_instance_!= null) {
_instance_.description = _value_;
}
}
}
;
public static final transient SinglePropertyInfo SOURCE = new SinglePropertyInfo("source", Content.class, Source.class, false, null, new QName("", "source"), new QName("http://www.ivoa.net/xml/VOResource/v1.0", "Source"), false) {
@Override
public Source get(final Content _instance_) {
return ((_instance_ == null)?null:_instance_.source);
}
@Override
public void set(final Content _instance_, final Source _value_) {
if (_instance_!= null) {
_instance_.source = _value_;
}
}
}
;
public static final transient SinglePropertyInfo REFERENCE_URL = new SinglePropertyInfo("referenceURL", Content.class, String.class, false, null, new QName("", "referenceURL"), new QName("http://www.w3.org/2001/XMLSchema", "anyURI"), false) {
@Override
public String get(final Content _instance_) {
return ((_instance_ == null)?null:_instance_.referenceURL);
}
@Override
public void set(final Content _instance_, final String _value_) {
if (_instance_!= null) {
_instance_.referenceURL = _value_;
}
}
}
;
public static final transient CollectionPropertyInfo TYPES = new CollectionPropertyInfo("types", Content.class, String.class, true, null, new QName("", "type"), new QName("http://www.w3.org/2001/XMLSchema", "token"), false) {
@Override
public List get(final Content _instance_) {
return ((_instance_ == null)?null:_instance_.types);
}
@Override
public void set(final Content _instance_, final List _value_) {
if (_instance_!= null) {
_instance_.types = _value_;
}
}
}
;
public static final transient CollectionPropertyInfo CONTENT_LEVELS = new CollectionPropertyInfo("contentLevels", Content.class, String.class, true, null, new QName("", "contentLevel"), new QName("http://www.w3.org/2001/XMLSchema", "token"), false) {
@Override
public List get(final Content _instance_) {
return ((_instance_ == null)?null:_instance_.contentLevels);
}
@Override
public void set(final Content _instance_, final List _value_) {
if (_instance_!= null) {
_instance_.contentLevels = _value_;
}
}
}
;
public static final transient CollectionPropertyInfo RELATIONSHIPS = new CollectionPropertyInfo("relationships", Content.class, Relationship.class, true, null, new QName("", "relationship"), new QName("http://www.ivoa.net/xml/VOResource/v1.0", "Relationship"), false) {
@Override
public List get(final Content _instance_) {
return ((_instance_ == null)?null:_instance_.relationships);
}
@Override
public void set(final Content _instance_, final List _value_) {
if (_instance_!= null) {
_instance_.relationships = _value_;
}
}
}
;
}
public static class Select
extends Content.Selector
{
Select() {
super(null, null, null);
}
public static Content.Select _root() {
return new Content.Select();
}
}
public static class Selector , TParent >
extends com.kscs.util.jaxb.Selector
{
private com.kscs.util.jaxb.Selector> subjects = null;
private com.kscs.util.jaxb.Selector> description = null;
private Source.Selector> source = null;
private com.kscs.util.jaxb.Selector> referenceURL = null;
private com.kscs.util.jaxb.Selector> types = null;
private com.kscs.util.jaxb.Selector> contentLevels = null;
private Relationship.Selector> relationships = null;
public Selector(final TRoot root, final TParent parent, final String propertyName) {
super(root, parent, propertyName);
}
@Override
public Map buildChildren() {
final Map products = new HashMap<>();
products.putAll(super.buildChildren());
if (this.subjects!= null) {
products.put("subjects", this.subjects.init());
}
if (this.description!= null) {
products.put("description", this.description.init());
}
if (this.source!= null) {
products.put("source", this.source.init());
}
if (this.referenceURL!= null) {
products.put("referenceURL", this.referenceURL.init());
}
if (this.types!= null) {
products.put("types", this.types.init());
}
if (this.contentLevels!= null) {
products.put("contentLevels", this.contentLevels.init());
}
if (this.relationships!= null) {
products.put("relationships", this.relationships.init());
}
return products;
}
public com.kscs.util.jaxb.Selector> subjects() {
return ((this.subjects == null)?this.subjects = new com.kscs.util.jaxb.Selector<>(this._root, this, "subjects"):this.subjects);
}
public com.kscs.util.jaxb.Selector> description() {
return ((this.description == null)?this.description = new com.kscs.util.jaxb.Selector<>(this._root, this, "description"):this.description);
}
public Source.Selector> source() {
return ((this.source == null)?this.source = new Source.Selector<>(this._root, this, "source"):this.source);
}
public com.kscs.util.jaxb.Selector> referenceURL() {
return ((this.referenceURL == null)?this.referenceURL = new com.kscs.util.jaxb.Selector<>(this._root, this, "referenceURL"):this.referenceURL);
}
public com.kscs.util.jaxb.Selector> types() {
return ((this.types == null)?this.types = new com.kscs.util.jaxb.Selector<>(this._root, this, "types"):this.types);
}
public com.kscs.util.jaxb.Selector> contentLevels() {
return ((this.contentLevels == null)?this.contentLevels = new com.kscs.util.jaxb.Selector<>(this._root, this, "contentLevels"):this.contentLevels);
}
public Relationship.Selector> relationships() {
return ((this.relationships == null)?this.relationships = new Relationship.Selector<>(this._root, this, "relationships"):this.relationships);
}
}
}