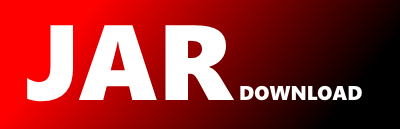
org.javastro.ivoa.entities.resource.Creator Maven / Gradle / Ivy
package org.javastro.ivoa.entities.resource;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import javax.xml.namespace.QName;
import com.kscs.util.jaxb.Buildable;
import com.kscs.util.jaxb.CollectionProperty;
import com.kscs.util.jaxb.CollectionPropertyInfo;
import com.kscs.util.jaxb.CollectionPropertyInfo;
import com.kscs.util.jaxb.Copyable;
import com.kscs.util.jaxb.PartialCopyable;
import com.kscs.util.jaxb.PropertyTree;
import com.kscs.util.jaxb.PropertyTreeUse;
import com.kscs.util.jaxb.PropertyVisitor;
import com.kscs.util.jaxb.SingleProperty;
import com.kscs.util.jaxb.SinglePropertyInfo;
import com.kscs.util.jaxb.SinglePropertyInfo;
import com.kscs.util.jaxb.SinglePropertyInfo;
import jakarta.annotation.Generated;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlAttribute;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlSchemaType;
import jakarta.xml.bind.annotation.XmlType;
import org.jvnet.jaxb.lang.JAXBMergeStrategy;
import org.jvnet.jaxb.lang.JAXBToStringStrategy;
import org.jvnet.jaxb.lang.MergeFrom;
import org.jvnet.jaxb.lang.MergeStrategy;
import org.jvnet.jaxb.lang.ToString;
import org.jvnet.jaxb.lang.ToStringStrategy;
import org.jvnet.jaxb.locator.ObjectLocator;
import org.jvnet.jaxb.locator.util.LocatorUtils;
/**
* The entity (e.g. person or organisation) primarily responsible
* for creating something
*
* Java class for Creator complex type
.
*
* The following schema fragment specifies the expected content contained within this class.
*
* {@code
*
*
*
*
*
*
*
*
*
*
*
*
* }
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "Creator", propOrder = {
"name",
"logo",
"altIdentifiers"
})
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public class Creator implements Cloneable, Copyable, PartialCopyable, MergeFrom, ToString
{
/**
* Users of the creation should use this name in
* subsequent credits and acknowledgements.
*
* This should be exactly one name, preferably last name
* first (as in "van der Waals, Johannes Diderik").
*
*/
@XmlElement(required = true)
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
protected ResourceName name;
/**
* A logo needs only be provided for the first occurrence.
* When multiple logos are supplied via multiple creator
* elements, the application is free to choose which to
* use.
*
*/
@XmlSchemaType(name = "anyURI")
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
protected String logo;
/**
* A reference to this entitiy in a non-IVOA identifier
* scheme, e.g., orcid. Always use a URI form including
* a scheme here.
*
*/
@XmlElement(name = "altIdentifier")
@XmlSchemaType(name = "anyURI")
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
protected List altIdentifiers;
/**
* An IVOA identifier for the creator (typically when it is
* an organization).
*
*/
@XmlAttribute(name = "ivo-id")
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
protected String ivoId;
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
protected transient Creator.Modifier __cachedModifier__;
/**
* Default no-arg constructor
*
*/
public Creator() {
super();
}
/**
* Fully-initialising value constructor
*
*/
public Creator(final ResourceName name, final String logo, final List altIdentifiers, final String ivoId) {
this.name = name;
this.logo = logo;
this.altIdentifiers = altIdentifiers;
this.ivoId = ivoId;
}
/**
* Users of the creation should use this name in
* subsequent credits and acknowledgements.
*
* This should be exactly one name, preferably last name
* first (as in "van der Waals, Johannes Diderik").
*
* @return
* possible object is
* {@link ResourceName }
*
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public ResourceName getName() {
return name;
}
/**
* Sets the value of the name property.
*
* @param value
* allowed object is
* {@link ResourceName }
*
* @see #getName()
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void setName(ResourceName value) {
this.name = value;
}
/**
* A logo needs only be provided for the first occurrence.
* When multiple logos are supplied via multiple creator
* elements, the application is free to choose which to
* use.
*
* @return
* possible object is
* {@link String }
*
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public String getLogo() {
return logo;
}
/**
* Sets the value of the logo property.
*
* @param value
* allowed object is
* {@link String }
*
* @see #getLogo()
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void setLogo(String value) {
this.logo = value;
}
/**
* A reference to this entitiy in a non-IVOA identifier
* scheme, e.g., orcid. Always use a URI form including
* a scheme here.
*
* Gets the value of the altIdentifiers property.
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the altIdentifiers property.
*
*
* For example, to add a new item, do as follows:
*
*
* getAltIdentifiers().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link String }
*
*
*
* @return
* The value of the altIdentifiers property.
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public List getAltIdentifiers() {
if (altIdentifiers == null) {
altIdentifiers = new ArrayList<>();
}
return this.altIdentifiers;
}
/**
* An IVOA identifier for the creator (typically when it is
* an organization).
*
* @return
* possible object is
* {@link String }
*
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public String getIvoId() {
return ivoId;
}
/**
* Sets the value of the ivoId property.
*
* @param value
* allowed object is
* {@link String }
*
* @see #getIvoId()
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void setIvoId(String value) {
this.ivoId = value;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public boolean equals(Object object) {
if ((object == null)||(this.getClass()!= object.getClass())) {
return false;
}
if (this == object) {
return true;
}
final Creator that = ((Creator) object);
{
ResourceName leftName;
leftName = this.getName();
ResourceName rightName;
rightName = that.getName();
if (this.name!= null) {
if (that.name!= null) {
if (!leftName.equals(rightName)) {
return false;
}
} else {
return false;
}
} else {
if (that.name!= null) {
return false;
}
}
}
{
String leftLogo;
leftLogo = this.getLogo();
String rightLogo;
rightLogo = that.getLogo();
if (this.logo!= null) {
if (that.logo!= null) {
if (!leftLogo.equals(rightLogo)) {
return false;
}
} else {
return false;
}
} else {
if (that.logo!= null) {
return false;
}
}
}
{
List leftAltIdentifiers;
leftAltIdentifiers = (((this.altIdentifiers!= null)&&(!this.altIdentifiers.isEmpty()))?this.getAltIdentifiers():null);
List rightAltIdentifiers;
rightAltIdentifiers = (((that.altIdentifiers!= null)&&(!that.altIdentifiers.isEmpty()))?that.getAltIdentifiers():null);
if ((this.altIdentifiers!= null)&&(!this.altIdentifiers.isEmpty())) {
if ((that.altIdentifiers!= null)&&(!that.altIdentifiers.isEmpty())) {
if (!leftAltIdentifiers.equals(rightAltIdentifiers)) {
return false;
}
} else {
return false;
}
} else {
if ((that.altIdentifiers!= null)&&(!that.altIdentifiers.isEmpty())) {
return false;
}
}
}
{
String leftIvoId;
leftIvoId = this.getIvoId();
String rightIvoId;
rightIvoId = that.getIvoId();
if (this.ivoId!= null) {
if (that.ivoId!= null) {
if (!leftIvoId.equals(rightIvoId)) {
return false;
}
} else {
return false;
}
} else {
if (that.ivoId!= null) {
return false;
}
}
}
return true;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public int hashCode() {
int currentHashCode = 1;
{
currentHashCode = (currentHashCode* 31);
ResourceName theName;
theName = this.getName();
if (this.name!= null) {
currentHashCode += theName.hashCode();
}
}
{
currentHashCode = (currentHashCode* 31);
String theLogo;
theLogo = this.getLogo();
if (this.logo!= null) {
currentHashCode += theLogo.hashCode();
}
}
{
currentHashCode = (currentHashCode* 31);
List theAltIdentifiers;
theAltIdentifiers = (((this.altIdentifiers!= null)&&(!this.altIdentifiers.isEmpty()))?this.getAltIdentifiers():null);
if ((this.altIdentifiers!= null)&&(!this.altIdentifiers.isEmpty())) {
currentHashCode += theAltIdentifiers.hashCode();
}
}
{
currentHashCode = (currentHashCode* 31);
String theIvoId;
theIvoId = this.getIvoId();
if (this.ivoId!= null) {
currentHashCode += theIvoId.hashCode();
}
}
return currentHashCode;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public String toString() {
final ToStringStrategy strategy = JAXBToStringStrategy.getInstance();
final StringBuilder buffer = new StringBuilder();
append(null, buffer, strategy);
return buffer.toString();
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public StringBuilder append(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
strategy.appendStart(locator, this, buffer);
appendFields(locator, buffer, strategy);
strategy.appendEnd(locator, this, buffer);
return buffer;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public StringBuilder appendFields(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
{
ResourceName theName;
theName = this.getName();
strategy.appendField(locator, this, "name", buffer, theName, (this.name!= null));
}
{
String theLogo;
theLogo = this.getLogo();
strategy.appendField(locator, this, "logo", buffer, theLogo, (this.logo!= null));
}
{
List theAltIdentifiers;
theAltIdentifiers = (((this.altIdentifiers!= null)&&(!this.altIdentifiers.isEmpty()))?this.getAltIdentifiers():null);
strategy.appendField(locator, this, "altIdentifiers", buffer, theAltIdentifiers, ((this.altIdentifiers!= null)&&(!this.altIdentifiers.isEmpty())));
}
{
String theIvoId;
theIvoId = this.getIvoId();
strategy.appendField(locator, this, "ivoId", buffer, theIvoId, (this.ivoId!= null));
}
return buffer;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void mergeFrom(Object left, Object right) {
final MergeStrategy strategy = JAXBMergeStrategy.getInstance();
mergeFrom(null, null, left, right, strategy);
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void mergeFrom(ObjectLocator leftLocator, ObjectLocator rightLocator, Object left, Object right, MergeStrategy strategy) {
if (right instanceof Creator) {
final Creator target = this;
final Creator leftObject = ((Creator) left);
final Creator rightObject = ((Creator) right);
{
Boolean nameShouldBeMergedAndSet = strategy.shouldBeMergedAndSet(leftLocator, rightLocator, (leftObject.name!= null), (rightObject.name!= null));
if (nameShouldBeMergedAndSet == Boolean.TRUE) {
ResourceName lhsName;
lhsName = leftObject.getName();
ResourceName rhsName;
rhsName = rightObject.getName();
ResourceName mergedName = ((ResourceName) strategy.merge(LocatorUtils.property(leftLocator, "name", lhsName), LocatorUtils.property(rightLocator, "name", rhsName), lhsName, rhsName, (leftObject.name!= null), (rightObject.name!= null)));
target.setName(mergedName);
} else {
if (nameShouldBeMergedAndSet == Boolean.FALSE) {
target.name = null;
}
}
}
{
Boolean logoShouldBeMergedAndSet = strategy.shouldBeMergedAndSet(leftLocator, rightLocator, (leftObject.logo!= null), (rightObject.logo!= null));
if (logoShouldBeMergedAndSet == Boolean.TRUE) {
String lhsLogo;
lhsLogo = leftObject.getLogo();
String rhsLogo;
rhsLogo = rightObject.getLogo();
String mergedLogo = ((String) strategy.merge(LocatorUtils.property(leftLocator, "logo", lhsLogo), LocatorUtils.property(rightLocator, "logo", rhsLogo), lhsLogo, rhsLogo, (leftObject.logo!= null), (rightObject.logo!= null)));
target.setLogo(mergedLogo);
} else {
if (logoShouldBeMergedAndSet == Boolean.FALSE) {
target.logo = null;
}
}
}
{
Boolean altIdentifiersShouldBeMergedAndSet = strategy.shouldBeMergedAndSet(leftLocator, rightLocator, ((leftObject.altIdentifiers!= null)&&(!leftObject.altIdentifiers.isEmpty())), ((rightObject.altIdentifiers!= null)&&(!rightObject.altIdentifiers.isEmpty())));
if (altIdentifiersShouldBeMergedAndSet == Boolean.TRUE) {
List lhsAltIdentifiers;
lhsAltIdentifiers = (((leftObject.altIdentifiers!= null)&&(!leftObject.altIdentifiers.isEmpty()))?leftObject.getAltIdentifiers():null);
List rhsAltIdentifiers;
rhsAltIdentifiers = (((rightObject.altIdentifiers!= null)&&(!rightObject.altIdentifiers.isEmpty()))?rightObject.getAltIdentifiers():null);
List mergedAltIdentifiers = ((List ) strategy.merge(LocatorUtils.property(leftLocator, "altIdentifiers", lhsAltIdentifiers), LocatorUtils.property(rightLocator, "altIdentifiers", rhsAltIdentifiers), lhsAltIdentifiers, rhsAltIdentifiers, ((leftObject.altIdentifiers!= null)&&(!leftObject.altIdentifiers.isEmpty())), ((rightObject.altIdentifiers!= null)&&(!rightObject.altIdentifiers.isEmpty()))));
target.altIdentifiers = null;
if (mergedAltIdentifiers!= null) {
List uniqueAltIdentifiersl = target.getAltIdentifiers();
uniqueAltIdentifiersl.addAll(mergedAltIdentifiers);
}
} else {
if (altIdentifiersShouldBeMergedAndSet == Boolean.FALSE) {
target.altIdentifiers = null;
}
}
}
{
Boolean ivoIdShouldBeMergedAndSet = strategy.shouldBeMergedAndSet(leftLocator, rightLocator, (leftObject.ivoId!= null), (rightObject.ivoId!= null));
if (ivoIdShouldBeMergedAndSet == Boolean.TRUE) {
String lhsIvoId;
lhsIvoId = leftObject.getIvoId();
String rhsIvoId;
rhsIvoId = rightObject.getIvoId();
String mergedIvoId = ((String) strategy.merge(LocatorUtils.property(leftLocator, "ivoId", lhsIvoId), LocatorUtils.property(rightLocator, "ivoId", rhsIvoId), lhsIvoId, rhsIvoId, (leftObject.ivoId!= null), (rightObject.ivoId!= null)));
target.setIvoId(mergedIvoId);
} else {
if (ivoIdShouldBeMergedAndSet == Boolean.FALSE) {
target.ivoId = null;
}
}
}
}
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Object createNewInstance() {
return new Creator();
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Creator clone() {
final Creator _newObject;
try {
_newObject = ((Creator) super.clone());
} catch (CloneNotSupportedException e) {
throw new RuntimeException(e);
}
_newObject.name = ((this.name == null)?null:this.name.clone());
_newObject.altIdentifiers = ((this.altIdentifiers == null)?null:new ArrayList<>(this.altIdentifiers));
return _newObject;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Creator createCopy() {
final Creator _newObject;
try {
_newObject = ((Creator) super.clone());
} catch (CloneNotSupportedException e) {
throw new RuntimeException(e);
}
_newObject.name = ((this.name == null)?null:this.name.createCopy());
_newObject.logo = this.logo;
_newObject.altIdentifiers = ((this.altIdentifiers == null)?null:new ArrayList<>(this.altIdentifiers));
_newObject.ivoId = this.ivoId;
return _newObject;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Creator createCopy(final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
final Creator _newObject;
try {
_newObject = ((Creator) super.clone());
} catch (CloneNotSupportedException e) {
throw new RuntimeException(e);
}
final PropertyTree namePropertyTree = ((_propertyTree == null)?null:_propertyTree.get("name"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(namePropertyTree!= null):((namePropertyTree == null)||(!namePropertyTree.isLeaf())))) {
_newObject.name = ((this.name == null)?null:this.name.createCopy(namePropertyTree, _propertyTreeUse));
}
final PropertyTree logoPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("logo"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(logoPropertyTree!= null):((logoPropertyTree == null)||(!logoPropertyTree.isLeaf())))) {
_newObject.logo = this.logo;
}
final PropertyTree altIdentifiersPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("altIdentifiers"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(altIdentifiersPropertyTree!= null):((altIdentifiersPropertyTree == null)||(!altIdentifiersPropertyTree.isLeaf())))) {
_newObject.altIdentifiers = ((this.altIdentifiers == null)?null:new ArrayList<>(this.altIdentifiers));
}
final PropertyTree ivoIdPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("ivoId"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(ivoIdPropertyTree!= null):((ivoIdPropertyTree == null)||(!ivoIdPropertyTree.isLeaf())))) {
_newObject.ivoId = this.ivoId;
}
return _newObject;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Creator copyExcept(final PropertyTree _propertyTree) {
return createCopy(_propertyTree, PropertyTreeUse.EXCLUDE);
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Creator copyOnly(final PropertyTree _propertyTree) {
return createCopy(_propertyTree, PropertyTreeUse.INCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Creator.Modifier modifier() {
if (null == this.__cachedModifier__) {
this.__cachedModifier__ = new Creator.Modifier();
}
return ((Creator.Modifier) this.__cachedModifier__);
}
/**
* Copies all state of this object to a builder. This method is used by the copyOf
* method and should not be called directly by client code.
*
* @param _other
* A builder instance to which the state of this object will be copied.
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public<_B >void copyTo(final Creator.Builder<_B> _other) {
_other.name = ((this.name == null)?null:this.name.newCopyBuilder(_other));
_other.logo = this.logo;
if (this.altIdentifiers == null) {
_other.altIdentifiers = null;
} else {
_other.altIdentifiers = new ArrayList<>();
for (String _item: this.altIdentifiers) {
_other.altIdentifiers.add(((_item == null)?null:new Buildable.PrimitiveBuildable(_item)));
}
}
_other.ivoId = this.ivoId;
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public<_B >Creator.Builder<_B> newCopyBuilder(final _B _parentBuilder) {
return new Creator.Builder<_B>(_parentBuilder, this, true);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Creator.Builder newCopyBuilder() {
return newCopyBuilder(null);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static Creator.Builder builder() {
return new Creator.Builder<>(null, null, false);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static<_B >Creator.Builder<_B> copyOf(final Creator _other) {
final Creator.Builder<_B> _newBuilder = new Creator.Builder<>(null, null, false);
_other.copyTo(_newBuilder);
return _newBuilder;
}
/**
* Copies all state of this object to a builder. This method is used by the copyOf
* method and should not be called directly by client code.
*
* @param _other
* A builder instance to which the state of this object will be copied.
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public<_B >void copyTo(final Creator.Builder<_B> _other, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
final PropertyTree namePropertyTree = ((_propertyTree == null)?null:_propertyTree.get("name"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(namePropertyTree!= null):((namePropertyTree == null)||(!namePropertyTree.isLeaf())))) {
_other.name = ((this.name == null)?null:this.name.newCopyBuilder(_other, namePropertyTree, _propertyTreeUse));
}
final PropertyTree logoPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("logo"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(logoPropertyTree!= null):((logoPropertyTree == null)||(!logoPropertyTree.isLeaf())))) {
_other.logo = this.logo;
}
final PropertyTree altIdentifiersPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("altIdentifiers"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(altIdentifiersPropertyTree!= null):((altIdentifiersPropertyTree == null)||(!altIdentifiersPropertyTree.isLeaf())))) {
if (this.altIdentifiers == null) {
_other.altIdentifiers = null;
} else {
_other.altIdentifiers = new ArrayList<>();
for (String _item: this.altIdentifiers) {
_other.altIdentifiers.add(((_item == null)?null:new Buildable.PrimitiveBuildable(_item)));
}
}
}
final PropertyTree ivoIdPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("ivoId"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(ivoIdPropertyTree!= null):((ivoIdPropertyTree == null)||(!ivoIdPropertyTree.isLeaf())))) {
_other.ivoId = this.ivoId;
}
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public<_B >Creator.Builder<_B> newCopyBuilder(final _B _parentBuilder, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
return new Creator.Builder<_B>(_parentBuilder, this, true, _propertyTree, _propertyTreeUse);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Creator.Builder newCopyBuilder(final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
return newCopyBuilder(null, _propertyTree, _propertyTreeUse);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static<_B >Creator.Builder<_B> copyOf(final Creator _other, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
final Creator.Builder<_B> _newBuilder = new Creator.Builder<>(null, null, false);
_other.copyTo(_newBuilder, _propertyTree, _propertyTreeUse);
return _newBuilder;
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static Creator.Builder copyExcept(final Creator _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.EXCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static Creator.Builder copyOnly(final Creator _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.INCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Creator visit(final PropertyVisitor _visitor_) {
_visitor_.visit(this);
if (_visitor_.visit(new SingleProperty<>(Creator.PropInfo.NAME, this))&&(this.name!= null)) {
this.name.visit(_visitor_);
}
_visitor_.visit(new SingleProperty<>(Creator.PropInfo.LOGO, this));
_visitor_.visit(new CollectionProperty<>(Creator.PropInfo.ALT_IDENTIFIERS, this));
_visitor_.visit(new SingleProperty<>(Creator.PropInfo.IVO_ID, this));
return this;
}
public static class Builder<_B >implements Buildable
{
protected final _B _parentBuilder;
protected final Creator _storedValue;
private ResourceName.Builder> name;
private String logo;
private List altIdentifiers;
private String ivoId;
public Builder(final _B _parentBuilder, final Creator _other, final boolean _copy) {
this._parentBuilder = _parentBuilder;
if (_other!= null) {
if (_copy) {
_storedValue = null;
this.name = ((_other.name == null)?null:_other.name.newCopyBuilder(this));
this.logo = _other.logo;
if (_other.altIdentifiers == null) {
this.altIdentifiers = null;
} else {
this.altIdentifiers = new ArrayList<>();
for (String _item: _other.altIdentifiers) {
this.altIdentifiers.add(((_item == null)?null:new Buildable.PrimitiveBuildable(_item)));
}
}
this.ivoId = _other.ivoId;
} else {
_storedValue = _other;
}
} else {
_storedValue = null;
}
}
public Builder(final _B _parentBuilder, final Creator _other, final boolean _copy, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
this._parentBuilder = _parentBuilder;
if (_other!= null) {
if (_copy) {
_storedValue = null;
final PropertyTree namePropertyTree = ((_propertyTree == null)?null:_propertyTree.get("name"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(namePropertyTree!= null):((namePropertyTree == null)||(!namePropertyTree.isLeaf())))) {
this.name = ((_other.name == null)?null:_other.name.newCopyBuilder(this, namePropertyTree, _propertyTreeUse));
}
final PropertyTree logoPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("logo"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(logoPropertyTree!= null):((logoPropertyTree == null)||(!logoPropertyTree.isLeaf())))) {
this.logo = _other.logo;
}
final PropertyTree altIdentifiersPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("altIdentifiers"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(altIdentifiersPropertyTree!= null):((altIdentifiersPropertyTree == null)||(!altIdentifiersPropertyTree.isLeaf())))) {
if (_other.altIdentifiers == null) {
this.altIdentifiers = null;
} else {
this.altIdentifiers = new ArrayList<>();
for (String _item: _other.altIdentifiers) {
this.altIdentifiers.add(((_item == null)?null:new Buildable.PrimitiveBuildable(_item)));
}
}
}
final PropertyTree ivoIdPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("ivoId"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(ivoIdPropertyTree!= null):((ivoIdPropertyTree == null)||(!ivoIdPropertyTree.isLeaf())))) {
this.ivoId = _other.ivoId;
}
} else {
_storedValue = _other;
}
} else {
_storedValue = null;
}
}
public _B end() {
return this._parentBuilder;
}
protected<_P extends Creator >_P init(final _P _product) {
_product.name = ((this.name == null)?null:this.name.build());
_product.logo = this.logo;
if (this.altIdentifiers!= null) {
final List altIdentifiers = new ArrayList<>(this.altIdentifiers.size());
for (Buildable _item: this.altIdentifiers) {
altIdentifiers.add(((String) _item.build()));
}
_product.altIdentifiers = altIdentifiers;
}
_product.ivoId = this.ivoId;
return _product;
}
/**
* Sets the new value of "name" (any previous value will be replaced)
*
* @param name
* New value of the "name" property.
*/
public Creator.Builder<_B> withName(final ResourceName name) {
this.name = ((name == null)?null:new ResourceName.Builder<>(this, name, false));
return this;
}
/**
* Returns the existing builder or a new builder to build the value of the "name"
* property.
* Use {@link org.javastro.ivoa.entities.resource.ResourceName.Builder#end()} to
* return to the current builder.
*
* @return
* A new builder to build the value of the "name" property.
* Use {@link org.javastro.ivoa.entities.resource.ResourceName.Builder#end()} to
* return to the current builder.
*/
public ResourceName.Builder extends Creator.Builder<_B>> withName() {
if (this.name!= null) {
return this.name;
}
return this.name = new ResourceName.Builder<>(this, null, false);
}
/**
* Sets the new value of "logo" (any previous value will be replaced)
*
* @param logo
* New value of the "logo" property.
*/
public Creator.Builder<_B> withLogo(final String logo) {
this.logo = logo;
return this;
}
/**
* Adds the given items to the value of "altIdentifiers"
*
* @param altIdentifiers
* Items to add to the value of the "altIdentifiers" property
*/
public Creator.Builder<_B> addAltIdentifiers(final Iterable extends String> altIdentifiers) {
if (altIdentifiers!= null) {
if (this.altIdentifiers == null) {
this.altIdentifiers = new ArrayList<>();
}
for (String _item: altIdentifiers) {
this.altIdentifiers.add(new Buildable.PrimitiveBuildable(_item));
}
}
return this;
}
/**
* Sets the new value of "altIdentifiers" (any previous value will be replaced)
*
* @param altIdentifiers
* New value of the "altIdentifiers" property.
*/
public Creator.Builder<_B> withAltIdentifiers(final Iterable extends String> altIdentifiers) {
if (this.altIdentifiers!= null) {
this.altIdentifiers.clear();
}
return addAltIdentifiers(altIdentifiers);
}
/**
* Adds the given items to the value of "altIdentifiers"
*
* @param altIdentifiers
* Items to add to the value of the "altIdentifiers" property
*/
public Creator.Builder<_B> addAltIdentifiers(String... altIdentifiers) {
addAltIdentifiers(Arrays.asList(altIdentifiers));
return this;
}
/**
* Sets the new value of "altIdentifiers" (any previous value will be replaced)
*
* @param altIdentifiers
* New value of the "altIdentifiers" property.
*/
public Creator.Builder<_B> withAltIdentifiers(String... altIdentifiers) {
withAltIdentifiers(Arrays.asList(altIdentifiers));
return this;
}
/**
* Sets the new value of "ivoId" (any previous value will be replaced)
*
* @param ivoId
* New value of the "ivoId" property.
*/
public Creator.Builder<_B> withIvoId(final String ivoId) {
this.ivoId = ivoId;
return this;
}
@Override
public Creator build() {
if (_storedValue == null) {
return this.init(new Creator());
} else {
return ((Creator) _storedValue);
}
}
public Creator.Builder<_B> copyOf(final Creator _other) {
_other.copyTo(this);
return this;
}
public Creator.Builder<_B> copyOf(final Creator.Builder _other) {
return copyOf(_other.build());
}
}
public class Modifier {
public void setName(final ResourceName name) {
Creator.this.setName(name);
}
public void setLogo(final String logo) {
Creator.this.setLogo(logo);
}
public List getAltIdentifiers() {
if (Creator.this.altIdentifiers == null) {
Creator.this.altIdentifiers = new ArrayList<>();
}
return Creator.this.altIdentifiers;
}
public void setIvoId(final String ivoId) {
Creator.this.setIvoId(ivoId);
}
}
public static class PropInfo {
public static final transient SinglePropertyInfo NAME = new SinglePropertyInfo("name", Creator.class, ResourceName.class, false, null, new QName("", "name"), new QName("http://www.ivoa.net/xml/VOResource/v1.0", "ResourceName"), false) {
@Override
public ResourceName get(final Creator _instance_) {
return ((_instance_ == null)?null:_instance_.name);
}
@Override
public void set(final Creator _instance_, final ResourceName _value_) {
if (_instance_!= null) {
_instance_.name = _value_;
}
}
}
;
public static final transient SinglePropertyInfo LOGO = new SinglePropertyInfo("logo", Creator.class, String.class, false, null, new QName("", "logo"), new QName("http://www.w3.org/2001/XMLSchema", "anyURI"), false) {
@Override
public String get(final Creator _instance_) {
return ((_instance_ == null)?null:_instance_.logo);
}
@Override
public void set(final Creator _instance_, final String _value_) {
if (_instance_!= null) {
_instance_.logo = _value_;
}
}
}
;
public static final transient CollectionPropertyInfo ALT_IDENTIFIERS = new CollectionPropertyInfo("altIdentifiers", Creator.class, String.class, true, null, new QName("", "altIdentifier"), new QName("http://www.w3.org/2001/XMLSchema", "anyURI"), false) {
@Override
public List get(final Creator _instance_) {
return ((_instance_ == null)?null:_instance_.altIdentifiers);
}
@Override
public void set(final Creator _instance_, final List _value_) {
if (_instance_!= null) {
_instance_.altIdentifiers = _value_;
}
}
}
;
public static final transient SinglePropertyInfo IVO_ID = new SinglePropertyInfo("ivoId", Creator.class, String.class, false, null, new QName("", "ivo-id"), new QName("http://www.ivoa.net/xml/VOResource/v1.0", "IdentifierURI"), true) {
@Override
public String get(final Creator _instance_) {
return ((_instance_ == null)?null:_instance_.ivoId);
}
@Override
public void set(final Creator _instance_, final String _value_) {
if (_instance_!= null) {
_instance_.ivoId = _value_;
}
}
}
;
}
public static class Select
extends Creator.Selector
{
Select() {
super(null, null, null);
}
public static Creator.Select _root() {
return new Creator.Select();
}
}
public static class Selector , TParent >
extends com.kscs.util.jaxb.Selector
{
private ResourceName.Selector> name = null;
private com.kscs.util.jaxb.Selector> logo = null;
private com.kscs.util.jaxb.Selector> altIdentifiers = null;
private com.kscs.util.jaxb.Selector> ivoId = null;
public Selector(final TRoot root, final TParent parent, final String propertyName) {
super(root, parent, propertyName);
}
@Override
public Map buildChildren() {
final Map products = new HashMap<>();
products.putAll(super.buildChildren());
if (this.name!= null) {
products.put("name", this.name.init());
}
if (this.logo!= null) {
products.put("logo", this.logo.init());
}
if (this.altIdentifiers!= null) {
products.put("altIdentifiers", this.altIdentifiers.init());
}
if (this.ivoId!= null) {
products.put("ivoId", this.ivoId.init());
}
return products;
}
public ResourceName.Selector> name() {
return ((this.name == null)?this.name = new ResourceName.Selector<>(this._root, this, "name"):this.name);
}
public com.kscs.util.jaxb.Selector> logo() {
return ((this.logo == null)?this.logo = new com.kscs.util.jaxb.Selector<>(this._root, this, "logo"):this.logo);
}
public com.kscs.util.jaxb.Selector> altIdentifiers() {
return ((this.altIdentifiers == null)?this.altIdentifiers = new com.kscs.util.jaxb.Selector<>(this._root, this, "altIdentifiers"):this.altIdentifiers);
}
public com.kscs.util.jaxb.Selector> ivoId() {
return ((this.ivoId == null)?this.ivoId = new com.kscs.util.jaxb.Selector<>(this._root, this, "ivoId"):this.ivoId);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy