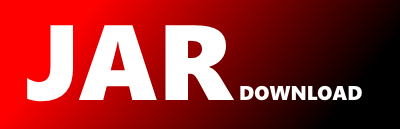
org.javastro.ivoa.entities.resource.Curation Maven / Gradle / Ivy
package org.javastro.ivoa.entities.resource;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import javax.xml.namespace.QName;
import com.kscs.util.jaxb.Buildable;
import com.kscs.util.jaxb.CollectionProperty;
import com.kscs.util.jaxb.CollectionPropertyInfo;
import com.kscs.util.jaxb.CollectionPropertyInfo;
import com.kscs.util.jaxb.CollectionPropertyInfo;
import com.kscs.util.jaxb.CollectionPropertyInfo;
import com.kscs.util.jaxb.CollectionPropertyInfo;
import com.kscs.util.jaxb.Copyable;
import com.kscs.util.jaxb.PartialCopyable;
import com.kscs.util.jaxb.PropertyTree;
import com.kscs.util.jaxb.PropertyTreeUse;
import com.kscs.util.jaxb.PropertyVisitor;
import com.kscs.util.jaxb.SingleProperty;
import com.kscs.util.jaxb.SinglePropertyInfo;
import com.kscs.util.jaxb.SinglePropertyInfo;
import com.kscs.util.jaxb.SinglePropertyInfo;
import jakarta.annotation.Generated;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlSchemaType;
import jakarta.xml.bind.annotation.XmlType;
import jakarta.xml.bind.annotation.adapters.CollapsedStringAdapter;
import jakarta.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import org.jvnet.jaxb.lang.JAXBMergeStrategy;
import org.jvnet.jaxb.lang.JAXBToStringStrategy;
import org.jvnet.jaxb.lang.MergeFrom;
import org.jvnet.jaxb.lang.MergeStrategy;
import org.jvnet.jaxb.lang.ToString;
import org.jvnet.jaxb.lang.ToStringStrategy;
import org.jvnet.jaxb.locator.ObjectLocator;
import org.jvnet.jaxb.locator.util.LocatorUtils;
/**
* Information regarding the general curation of a resource
*
* Java class for Curation complex type
.
*
* The following schema fragment specifies the expected content contained within this class.
*
* {@code
*
*
*
*
*
*
*
*
*
*
*
*
*
*
* }
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "Curation", propOrder = {
"publisher",
"creators",
"contributors",
"dates",
"version",
"contacts"
})
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public class Curation implements Cloneable, Copyable, PartialCopyable, MergeFrom, ToString
{
/**
* Entity (e.g. person or organisation) responsible for making the
* resource available
*
*/
@XmlElement(required = true)
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
protected ResourceName publisher;
/**
* This is the equivalent of the author of a publication.
*
*/
@XmlElement(name = "creator")
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
protected List creators;
/**
* Entity responsible for contributions to the content of
* the resource
*
*/
@XmlElement(name = "contributor")
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
protected List contributors;
/**
* This will typically be associated with the creation or
* availability (i.e., most recent release or version) of
* the resource. Use the role attribute to clarify.
*
*/
@XmlElement(name = "date")
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
protected List dates;
/**
* Label associated with creation or availablilty of a version of
* a resource.
*
*/
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
@XmlSchemaType(name = "token")
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
protected String version;
/**
* Information that can be used for contacting someone with
* regard to this resource.
*
*/
@XmlElement(name = "contact", required = true)
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
protected List contacts;
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
protected transient Curation.Modifier __cachedModifier__;
/**
* Default no-arg constructor
*
*/
public Curation() {
super();
}
/**
* Fully-initialising value constructor
*
*/
public Curation(final ResourceName publisher, final List creators, final List contributors, final List dates, final String version, final List contacts) {
this.publisher = publisher;
this.creators = creators;
this.contributors = contributors;
this.dates = dates;
this.version = version;
this.contacts = contacts;
}
/**
* Entity (e.g. person or organisation) responsible for making the
* resource available
*
* @return
* possible object is
* {@link ResourceName }
*
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public ResourceName getPublisher() {
return publisher;
}
/**
* Sets the value of the publisher property.
*
* @param value
* allowed object is
* {@link ResourceName }
*
* @see #getPublisher()
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void setPublisher(ResourceName value) {
this.publisher = value;
}
/**
* This is the equivalent of the author of a publication.
*
* Gets the value of the creators property.
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the creators property.
*
*
* For example, to add a new item, do as follows:
*
*
* getCreators().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Creator }
*
*
*
* @return
* The value of the creators property.
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public List getCreators() {
if (creators == null) {
creators = new ArrayList<>();
}
return this.creators;
}
/**
* Entity responsible for contributions to the content of
* the resource
*
* Gets the value of the contributors property.
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the contributors property.
*
*
* For example, to add a new item, do as follows:
*
*
* getContributors().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link ResourceName }
*
*
*
* @return
* The value of the contributors property.
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public List getContributors() {
if (contributors == null) {
contributors = new ArrayList<>();
}
return this.contributors;
}
/**
* This will typically be associated with the creation or
* availability (i.e., most recent release or version) of
* the resource. Use the role attribute to clarify.
*
* Gets the value of the dates property.
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the dates property.
*
*
* For example, to add a new item, do as follows:
*
*
* getDates().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Date }
*
*
*
* @return
* The value of the dates property.
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public List getDates() {
if (dates == null) {
dates = new ArrayList<>();
}
return this.dates;
}
/**
* Label associated with creation or availablilty of a version of
* a resource.
*
* @return
* possible object is
* {@link String }
*
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public String getVersion() {
return version;
}
/**
* Sets the value of the version property.
*
* @param value
* allowed object is
* {@link String }
*
* @see #getVersion()
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void setVersion(String value) {
this.version = value;
}
/**
* Information that can be used for contacting someone with
* regard to this resource.
*
* Gets the value of the contacts property.
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the contacts property.
*
*
* For example, to add a new item, do as follows:
*
*
* getContacts().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Contact }
*
*
*
* @return
* The value of the contacts property.
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public List getContacts() {
if (contacts == null) {
contacts = new ArrayList<>();
}
return this.contacts;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public boolean equals(Object object) {
if ((object == null)||(this.getClass()!= object.getClass())) {
return false;
}
if (this == object) {
return true;
}
final Curation that = ((Curation) object);
{
ResourceName leftPublisher;
leftPublisher = this.getPublisher();
ResourceName rightPublisher;
rightPublisher = that.getPublisher();
if (this.publisher!= null) {
if (that.publisher!= null) {
if (!leftPublisher.equals(rightPublisher)) {
return false;
}
} else {
return false;
}
} else {
if (that.publisher!= null) {
return false;
}
}
}
{
List leftCreators;
leftCreators = (((this.creators!= null)&&(!this.creators.isEmpty()))?this.getCreators():null);
List rightCreators;
rightCreators = (((that.creators!= null)&&(!that.creators.isEmpty()))?that.getCreators():null);
if ((this.creators!= null)&&(!this.creators.isEmpty())) {
if ((that.creators!= null)&&(!that.creators.isEmpty())) {
if (!leftCreators.equals(rightCreators)) {
return false;
}
} else {
return false;
}
} else {
if ((that.creators!= null)&&(!that.creators.isEmpty())) {
return false;
}
}
}
{
List leftContributors;
leftContributors = (((this.contributors!= null)&&(!this.contributors.isEmpty()))?this.getContributors():null);
List rightContributors;
rightContributors = (((that.contributors!= null)&&(!that.contributors.isEmpty()))?that.getContributors():null);
if ((this.contributors!= null)&&(!this.contributors.isEmpty())) {
if ((that.contributors!= null)&&(!that.contributors.isEmpty())) {
if (!leftContributors.equals(rightContributors)) {
return false;
}
} else {
return false;
}
} else {
if ((that.contributors!= null)&&(!that.contributors.isEmpty())) {
return false;
}
}
}
{
List leftDates;
leftDates = (((this.dates!= null)&&(!this.dates.isEmpty()))?this.getDates():null);
List rightDates;
rightDates = (((that.dates!= null)&&(!that.dates.isEmpty()))?that.getDates():null);
if ((this.dates!= null)&&(!this.dates.isEmpty())) {
if ((that.dates!= null)&&(!that.dates.isEmpty())) {
if (!leftDates.equals(rightDates)) {
return false;
}
} else {
return false;
}
} else {
if ((that.dates!= null)&&(!that.dates.isEmpty())) {
return false;
}
}
}
{
String leftVersion;
leftVersion = this.getVersion();
String rightVersion;
rightVersion = that.getVersion();
if (this.version!= null) {
if (that.version!= null) {
if (!leftVersion.equals(rightVersion)) {
return false;
}
} else {
return false;
}
} else {
if (that.version!= null) {
return false;
}
}
}
{
List leftContacts;
leftContacts = (((this.contacts!= null)&&(!this.contacts.isEmpty()))?this.getContacts():null);
List rightContacts;
rightContacts = (((that.contacts!= null)&&(!that.contacts.isEmpty()))?that.getContacts():null);
if ((this.contacts!= null)&&(!this.contacts.isEmpty())) {
if ((that.contacts!= null)&&(!that.contacts.isEmpty())) {
if (!leftContacts.equals(rightContacts)) {
return false;
}
} else {
return false;
}
} else {
if ((that.contacts!= null)&&(!that.contacts.isEmpty())) {
return false;
}
}
}
return true;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public int hashCode() {
int currentHashCode = 1;
{
currentHashCode = (currentHashCode* 31);
ResourceName thePublisher;
thePublisher = this.getPublisher();
if (this.publisher!= null) {
currentHashCode += thePublisher.hashCode();
}
}
{
currentHashCode = (currentHashCode* 31);
List theCreators;
theCreators = (((this.creators!= null)&&(!this.creators.isEmpty()))?this.getCreators():null);
if ((this.creators!= null)&&(!this.creators.isEmpty())) {
currentHashCode += theCreators.hashCode();
}
}
{
currentHashCode = (currentHashCode* 31);
List theContributors;
theContributors = (((this.contributors!= null)&&(!this.contributors.isEmpty()))?this.getContributors():null);
if ((this.contributors!= null)&&(!this.contributors.isEmpty())) {
currentHashCode += theContributors.hashCode();
}
}
{
currentHashCode = (currentHashCode* 31);
List theDates;
theDates = (((this.dates!= null)&&(!this.dates.isEmpty()))?this.getDates():null);
if ((this.dates!= null)&&(!this.dates.isEmpty())) {
currentHashCode += theDates.hashCode();
}
}
{
currentHashCode = (currentHashCode* 31);
String theVersion;
theVersion = this.getVersion();
if (this.version!= null) {
currentHashCode += theVersion.hashCode();
}
}
{
currentHashCode = (currentHashCode* 31);
List theContacts;
theContacts = (((this.contacts!= null)&&(!this.contacts.isEmpty()))?this.getContacts():null);
if ((this.contacts!= null)&&(!this.contacts.isEmpty())) {
currentHashCode += theContacts.hashCode();
}
}
return currentHashCode;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public String toString() {
final ToStringStrategy strategy = JAXBToStringStrategy.getInstance();
final StringBuilder buffer = new StringBuilder();
append(null, buffer, strategy);
return buffer.toString();
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public StringBuilder append(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
strategy.appendStart(locator, this, buffer);
appendFields(locator, buffer, strategy);
strategy.appendEnd(locator, this, buffer);
return buffer;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public StringBuilder appendFields(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
{
ResourceName thePublisher;
thePublisher = this.getPublisher();
strategy.appendField(locator, this, "publisher", buffer, thePublisher, (this.publisher!= null));
}
{
List theCreators;
theCreators = (((this.creators!= null)&&(!this.creators.isEmpty()))?this.getCreators():null);
strategy.appendField(locator, this, "creators", buffer, theCreators, ((this.creators!= null)&&(!this.creators.isEmpty())));
}
{
List theContributors;
theContributors = (((this.contributors!= null)&&(!this.contributors.isEmpty()))?this.getContributors():null);
strategy.appendField(locator, this, "contributors", buffer, theContributors, ((this.contributors!= null)&&(!this.contributors.isEmpty())));
}
{
List theDates;
theDates = (((this.dates!= null)&&(!this.dates.isEmpty()))?this.getDates():null);
strategy.appendField(locator, this, "dates", buffer, theDates, ((this.dates!= null)&&(!this.dates.isEmpty())));
}
{
String theVersion;
theVersion = this.getVersion();
strategy.appendField(locator, this, "version", buffer, theVersion, (this.version!= null));
}
{
List theContacts;
theContacts = (((this.contacts!= null)&&(!this.contacts.isEmpty()))?this.getContacts():null);
strategy.appendField(locator, this, "contacts", buffer, theContacts, ((this.contacts!= null)&&(!this.contacts.isEmpty())));
}
return buffer;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void mergeFrom(Object left, Object right) {
final MergeStrategy strategy = JAXBMergeStrategy.getInstance();
mergeFrom(null, null, left, right, strategy);
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void mergeFrom(ObjectLocator leftLocator, ObjectLocator rightLocator, Object left, Object right, MergeStrategy strategy) {
if (right instanceof Curation) {
final Curation target = this;
final Curation leftObject = ((Curation) left);
final Curation rightObject = ((Curation) right);
{
Boolean publisherShouldBeMergedAndSet = strategy.shouldBeMergedAndSet(leftLocator, rightLocator, (leftObject.publisher!= null), (rightObject.publisher!= null));
if (publisherShouldBeMergedAndSet == Boolean.TRUE) {
ResourceName lhsPublisher;
lhsPublisher = leftObject.getPublisher();
ResourceName rhsPublisher;
rhsPublisher = rightObject.getPublisher();
ResourceName mergedPublisher = ((ResourceName) strategy.merge(LocatorUtils.property(leftLocator, "publisher", lhsPublisher), LocatorUtils.property(rightLocator, "publisher", rhsPublisher), lhsPublisher, rhsPublisher, (leftObject.publisher!= null), (rightObject.publisher!= null)));
target.setPublisher(mergedPublisher);
} else {
if (publisherShouldBeMergedAndSet == Boolean.FALSE) {
target.publisher = null;
}
}
}
{
Boolean creatorsShouldBeMergedAndSet = strategy.shouldBeMergedAndSet(leftLocator, rightLocator, ((leftObject.creators!= null)&&(!leftObject.creators.isEmpty())), ((rightObject.creators!= null)&&(!rightObject.creators.isEmpty())));
if (creatorsShouldBeMergedAndSet == Boolean.TRUE) {
List lhsCreators;
lhsCreators = (((leftObject.creators!= null)&&(!leftObject.creators.isEmpty()))?leftObject.getCreators():null);
List rhsCreators;
rhsCreators = (((rightObject.creators!= null)&&(!rightObject.creators.isEmpty()))?rightObject.getCreators():null);
List mergedCreators = ((List ) strategy.merge(LocatorUtils.property(leftLocator, "creators", lhsCreators), LocatorUtils.property(rightLocator, "creators", rhsCreators), lhsCreators, rhsCreators, ((leftObject.creators!= null)&&(!leftObject.creators.isEmpty())), ((rightObject.creators!= null)&&(!rightObject.creators.isEmpty()))));
target.creators = null;
if (mergedCreators!= null) {
List uniqueCreatorsl = target.getCreators();
uniqueCreatorsl.addAll(mergedCreators);
}
} else {
if (creatorsShouldBeMergedAndSet == Boolean.FALSE) {
target.creators = null;
}
}
}
{
Boolean contributorsShouldBeMergedAndSet = strategy.shouldBeMergedAndSet(leftLocator, rightLocator, ((leftObject.contributors!= null)&&(!leftObject.contributors.isEmpty())), ((rightObject.contributors!= null)&&(!rightObject.contributors.isEmpty())));
if (contributorsShouldBeMergedAndSet == Boolean.TRUE) {
List lhsContributors;
lhsContributors = (((leftObject.contributors!= null)&&(!leftObject.contributors.isEmpty()))?leftObject.getContributors():null);
List rhsContributors;
rhsContributors = (((rightObject.contributors!= null)&&(!rightObject.contributors.isEmpty()))?rightObject.getContributors():null);
List mergedContributors = ((List ) strategy.merge(LocatorUtils.property(leftLocator, "contributors", lhsContributors), LocatorUtils.property(rightLocator, "contributors", rhsContributors), lhsContributors, rhsContributors, ((leftObject.contributors!= null)&&(!leftObject.contributors.isEmpty())), ((rightObject.contributors!= null)&&(!rightObject.contributors.isEmpty()))));
target.contributors = null;
if (mergedContributors!= null) {
List uniqueContributorsl = target.getContributors();
uniqueContributorsl.addAll(mergedContributors);
}
} else {
if (contributorsShouldBeMergedAndSet == Boolean.FALSE) {
target.contributors = null;
}
}
}
{
Boolean datesShouldBeMergedAndSet = strategy.shouldBeMergedAndSet(leftLocator, rightLocator, ((leftObject.dates!= null)&&(!leftObject.dates.isEmpty())), ((rightObject.dates!= null)&&(!rightObject.dates.isEmpty())));
if (datesShouldBeMergedAndSet == Boolean.TRUE) {
List lhsDates;
lhsDates = (((leftObject.dates!= null)&&(!leftObject.dates.isEmpty()))?leftObject.getDates():null);
List rhsDates;
rhsDates = (((rightObject.dates!= null)&&(!rightObject.dates.isEmpty()))?rightObject.getDates():null);
List mergedDates = ((List ) strategy.merge(LocatorUtils.property(leftLocator, "dates", lhsDates), LocatorUtils.property(rightLocator, "dates", rhsDates), lhsDates, rhsDates, ((leftObject.dates!= null)&&(!leftObject.dates.isEmpty())), ((rightObject.dates!= null)&&(!rightObject.dates.isEmpty()))));
target.dates = null;
if (mergedDates!= null) {
List uniqueDatesl = target.getDates();
uniqueDatesl.addAll(mergedDates);
}
} else {
if (datesShouldBeMergedAndSet == Boolean.FALSE) {
target.dates = null;
}
}
}
{
Boolean versionShouldBeMergedAndSet = strategy.shouldBeMergedAndSet(leftLocator, rightLocator, (leftObject.version!= null), (rightObject.version!= null));
if (versionShouldBeMergedAndSet == Boolean.TRUE) {
String lhsVersion;
lhsVersion = leftObject.getVersion();
String rhsVersion;
rhsVersion = rightObject.getVersion();
String mergedVersion = ((String) strategy.merge(LocatorUtils.property(leftLocator, "version", lhsVersion), LocatorUtils.property(rightLocator, "version", rhsVersion), lhsVersion, rhsVersion, (leftObject.version!= null), (rightObject.version!= null)));
target.setVersion(mergedVersion);
} else {
if (versionShouldBeMergedAndSet == Boolean.FALSE) {
target.version = null;
}
}
}
{
Boolean contactsShouldBeMergedAndSet = strategy.shouldBeMergedAndSet(leftLocator, rightLocator, ((leftObject.contacts!= null)&&(!leftObject.contacts.isEmpty())), ((rightObject.contacts!= null)&&(!rightObject.contacts.isEmpty())));
if (contactsShouldBeMergedAndSet == Boolean.TRUE) {
List lhsContacts;
lhsContacts = (((leftObject.contacts!= null)&&(!leftObject.contacts.isEmpty()))?leftObject.getContacts():null);
List rhsContacts;
rhsContacts = (((rightObject.contacts!= null)&&(!rightObject.contacts.isEmpty()))?rightObject.getContacts():null);
List mergedContacts = ((List ) strategy.merge(LocatorUtils.property(leftLocator, "contacts", lhsContacts), LocatorUtils.property(rightLocator, "contacts", rhsContacts), lhsContacts, rhsContacts, ((leftObject.contacts!= null)&&(!leftObject.contacts.isEmpty())), ((rightObject.contacts!= null)&&(!rightObject.contacts.isEmpty()))));
target.contacts = null;
if (mergedContacts!= null) {
List uniqueContactsl = target.getContacts();
uniqueContactsl.addAll(mergedContacts);
}
} else {
if (contactsShouldBeMergedAndSet == Boolean.FALSE) {
target.contacts = null;
}
}
}
}
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Object createNewInstance() {
return new Curation();
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Curation clone() {
final Curation _newObject;
try {
_newObject = ((Curation) super.clone());
} catch (CloneNotSupportedException e) {
throw new RuntimeException(e);
}
_newObject.publisher = ((this.publisher == null)?null:this.publisher.clone());
if (this.creators == null) {
_newObject.creators = null;
} else {
_newObject.creators = new ArrayList<>();
for (Creator _item: this.creators) {
_newObject.creators.add(((_item == null)?null:_item.clone()));
}
}
if (this.contributors == null) {
_newObject.contributors = null;
} else {
_newObject.contributors = new ArrayList<>();
for (ResourceName _item: this.contributors) {
_newObject.contributors.add(((_item == null)?null:_item.clone()));
}
}
if (this.dates == null) {
_newObject.dates = null;
} else {
_newObject.dates = new ArrayList<>();
for (Date _item: this.dates) {
_newObject.dates.add(((_item == null)?null:_item.clone()));
}
}
if (this.contacts == null) {
_newObject.contacts = null;
} else {
_newObject.contacts = new ArrayList<>();
for (Contact _item: this.contacts) {
_newObject.contacts.add(((_item == null)?null:_item.clone()));
}
}
return _newObject;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Curation createCopy() {
final Curation _newObject;
try {
_newObject = ((Curation) super.clone());
} catch (CloneNotSupportedException e) {
throw new RuntimeException(e);
}
_newObject.publisher = ((this.publisher == null)?null:this.publisher.createCopy());
if (this.creators == null) {
_newObject.creators = null;
} else {
_newObject.creators = new ArrayList<>();
for (Creator _item: this.creators) {
_newObject.creators.add(((_item == null)?null:_item.createCopy()));
}
}
if (this.contributors == null) {
_newObject.contributors = null;
} else {
_newObject.contributors = new ArrayList<>();
for (ResourceName _item: this.contributors) {
_newObject.contributors.add(((_item == null)?null:_item.createCopy()));
}
}
if (this.dates == null) {
_newObject.dates = null;
} else {
_newObject.dates = new ArrayList<>();
for (Date _item: this.dates) {
_newObject.dates.add(((_item == null)?null:_item.createCopy()));
}
}
_newObject.version = this.version;
if (this.contacts == null) {
_newObject.contacts = null;
} else {
_newObject.contacts = new ArrayList<>();
for (Contact _item: this.contacts) {
_newObject.contacts.add(((_item == null)?null:_item.createCopy()));
}
}
return _newObject;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Curation createCopy(final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
final Curation _newObject;
try {
_newObject = ((Curation) super.clone());
} catch (CloneNotSupportedException e) {
throw new RuntimeException(e);
}
final PropertyTree publisherPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("publisher"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(publisherPropertyTree!= null):((publisherPropertyTree == null)||(!publisherPropertyTree.isLeaf())))) {
_newObject.publisher = ((this.publisher == null)?null:this.publisher.createCopy(publisherPropertyTree, _propertyTreeUse));
}
final PropertyTree creatorsPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("creators"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(creatorsPropertyTree!= null):((creatorsPropertyTree == null)||(!creatorsPropertyTree.isLeaf())))) {
if (this.creators == null) {
_newObject.creators = null;
} else {
_newObject.creators = new ArrayList<>();
for (Creator _item: this.creators) {
_newObject.creators.add(((_item == null)?null:_item.createCopy(creatorsPropertyTree, _propertyTreeUse)));
}
}
}
final PropertyTree contributorsPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("contributors"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(contributorsPropertyTree!= null):((contributorsPropertyTree == null)||(!contributorsPropertyTree.isLeaf())))) {
if (this.contributors == null) {
_newObject.contributors = null;
} else {
_newObject.contributors = new ArrayList<>();
for (ResourceName _item: this.contributors) {
_newObject.contributors.add(((_item == null)?null:_item.createCopy(contributorsPropertyTree, _propertyTreeUse)));
}
}
}
final PropertyTree datesPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("dates"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(datesPropertyTree!= null):((datesPropertyTree == null)||(!datesPropertyTree.isLeaf())))) {
if (this.dates == null) {
_newObject.dates = null;
} else {
_newObject.dates = new ArrayList<>();
for (Date _item: this.dates) {
_newObject.dates.add(((_item == null)?null:_item.createCopy(datesPropertyTree, _propertyTreeUse)));
}
}
}
final PropertyTree versionPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("version"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(versionPropertyTree!= null):((versionPropertyTree == null)||(!versionPropertyTree.isLeaf())))) {
_newObject.version = this.version;
}
final PropertyTree contactsPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("contacts"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(contactsPropertyTree!= null):((contactsPropertyTree == null)||(!contactsPropertyTree.isLeaf())))) {
if (this.contacts == null) {
_newObject.contacts = null;
} else {
_newObject.contacts = new ArrayList<>();
for (Contact _item: this.contacts) {
_newObject.contacts.add(((_item == null)?null:_item.createCopy(contactsPropertyTree, _propertyTreeUse)));
}
}
}
return _newObject;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Curation copyExcept(final PropertyTree _propertyTree) {
return createCopy(_propertyTree, PropertyTreeUse.EXCLUDE);
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Curation copyOnly(final PropertyTree _propertyTree) {
return createCopy(_propertyTree, PropertyTreeUse.INCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Curation.Modifier modifier() {
if (null == this.__cachedModifier__) {
this.__cachedModifier__ = new Curation.Modifier();
}
return ((Curation.Modifier) this.__cachedModifier__);
}
/**
* Copies all state of this object to a builder. This method is used by the copyOf
* method and should not be called directly by client code.
*
* @param _other
* A builder instance to which the state of this object will be copied.
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public<_B >void copyTo(final Curation.Builder<_B> _other) {
_other.publisher = ((this.publisher == null)?null:this.publisher.newCopyBuilder(_other));
if (this.creators == null) {
_other.creators = null;
} else {
_other.creators = new ArrayList<>();
for (Creator _item: this.creators) {
_other.creators.add(((_item == null)?null:_item.newCopyBuilder(_other)));
}
}
if (this.contributors == null) {
_other.contributors = null;
} else {
_other.contributors = new ArrayList<>();
for (ResourceName _item: this.contributors) {
_other.contributors.add(((_item == null)?null:_item.newCopyBuilder(_other)));
}
}
if (this.dates == null) {
_other.dates = null;
} else {
_other.dates = new ArrayList<>();
for (Date _item: this.dates) {
_other.dates.add(((_item == null)?null:_item.newCopyBuilder(_other)));
}
}
_other.version = this.version;
if (this.contacts == null) {
_other.contacts = null;
} else {
_other.contacts = new ArrayList<>();
for (Contact _item: this.contacts) {
_other.contacts.add(((_item == null)?null:_item.newCopyBuilder(_other)));
}
}
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public<_B >Curation.Builder<_B> newCopyBuilder(final _B _parentBuilder) {
return new Curation.Builder<_B>(_parentBuilder, this, true);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Curation.Builder newCopyBuilder() {
return newCopyBuilder(null);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static Curation.Builder builder() {
return new Curation.Builder<>(null, null, false);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static<_B >Curation.Builder<_B> copyOf(final Curation _other) {
final Curation.Builder<_B> _newBuilder = new Curation.Builder<>(null, null, false);
_other.copyTo(_newBuilder);
return _newBuilder;
}
/**
* Copies all state of this object to a builder. This method is used by the copyOf
* method and should not be called directly by client code.
*
* @param _other
* A builder instance to which the state of this object will be copied.
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public<_B >void copyTo(final Curation.Builder<_B> _other, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
final PropertyTree publisherPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("publisher"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(publisherPropertyTree!= null):((publisherPropertyTree == null)||(!publisherPropertyTree.isLeaf())))) {
_other.publisher = ((this.publisher == null)?null:this.publisher.newCopyBuilder(_other, publisherPropertyTree, _propertyTreeUse));
}
final PropertyTree creatorsPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("creators"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(creatorsPropertyTree!= null):((creatorsPropertyTree == null)||(!creatorsPropertyTree.isLeaf())))) {
if (this.creators == null) {
_other.creators = null;
} else {
_other.creators = new ArrayList<>();
for (Creator _item: this.creators) {
_other.creators.add(((_item == null)?null:_item.newCopyBuilder(_other, creatorsPropertyTree, _propertyTreeUse)));
}
}
}
final PropertyTree contributorsPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("contributors"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(contributorsPropertyTree!= null):((contributorsPropertyTree == null)||(!contributorsPropertyTree.isLeaf())))) {
if (this.contributors == null) {
_other.contributors = null;
} else {
_other.contributors = new ArrayList<>();
for (ResourceName _item: this.contributors) {
_other.contributors.add(((_item == null)?null:_item.newCopyBuilder(_other, contributorsPropertyTree, _propertyTreeUse)));
}
}
}
final PropertyTree datesPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("dates"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(datesPropertyTree!= null):((datesPropertyTree == null)||(!datesPropertyTree.isLeaf())))) {
if (this.dates == null) {
_other.dates = null;
} else {
_other.dates = new ArrayList<>();
for (Date _item: this.dates) {
_other.dates.add(((_item == null)?null:_item.newCopyBuilder(_other, datesPropertyTree, _propertyTreeUse)));
}
}
}
final PropertyTree versionPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("version"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(versionPropertyTree!= null):((versionPropertyTree == null)||(!versionPropertyTree.isLeaf())))) {
_other.version = this.version;
}
final PropertyTree contactsPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("contacts"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(contactsPropertyTree!= null):((contactsPropertyTree == null)||(!contactsPropertyTree.isLeaf())))) {
if (this.contacts == null) {
_other.contacts = null;
} else {
_other.contacts = new ArrayList<>();
for (Contact _item: this.contacts) {
_other.contacts.add(((_item == null)?null:_item.newCopyBuilder(_other, contactsPropertyTree, _propertyTreeUse)));
}
}
}
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public<_B >Curation.Builder<_B> newCopyBuilder(final _B _parentBuilder, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
return new Curation.Builder<_B>(_parentBuilder, this, true, _propertyTree, _propertyTreeUse);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Curation.Builder newCopyBuilder(final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
return newCopyBuilder(null, _propertyTree, _propertyTreeUse);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static<_B >Curation.Builder<_B> copyOf(final Curation _other, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
final Curation.Builder<_B> _newBuilder = new Curation.Builder<>(null, null, false);
_other.copyTo(_newBuilder, _propertyTree, _propertyTreeUse);
return _newBuilder;
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static Curation.Builder copyExcept(final Curation _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.EXCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static Curation.Builder copyOnly(final Curation _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.INCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Curation visit(final PropertyVisitor _visitor_) {
_visitor_.visit(this);
if (_visitor_.visit(new SingleProperty<>(Curation.PropInfo.PUBLISHER, this))&&(this.publisher!= null)) {
this.publisher.visit(_visitor_);
}
if (_visitor_.visit(new CollectionProperty<>(Curation.PropInfo.CREATORS, this))&&(this.creators!= null)) {
for (Creator _item_: this.creators) {
if (_item_!= null) {
_item_.visit(_visitor_);
}
}
}
if (_visitor_.visit(new CollectionProperty<>(Curation.PropInfo.CONTRIBUTORS, this))&&(this.contributors!= null)) {
for (ResourceName _item_: this.contributors) {
if (_item_!= null) {
_item_.visit(_visitor_);
}
}
}
if (_visitor_.visit(new CollectionProperty<>(Curation.PropInfo.DATES, this))&&(this.dates!= null)) {
for (Date _item_: this.dates) {
if (_item_!= null) {
_item_.visit(_visitor_);
}
}
}
_visitor_.visit(new SingleProperty<>(Curation.PropInfo.VERSION, this));
if (_visitor_.visit(new CollectionProperty<>(Curation.PropInfo.CONTACTS, this))&&(this.contacts!= null)) {
for (Contact _item_: this.contacts) {
if (_item_!= null) {
_item_.visit(_visitor_);
}
}
}
return this;
}
public static class Builder<_B >implements Buildable
{
protected final _B _parentBuilder;
protected final Curation _storedValue;
private ResourceName.Builder> publisher;
private List>> creators;
private List>> contributors;
private List>> dates;
private String version;
private List>> contacts;
public Builder(final _B _parentBuilder, final Curation _other, final boolean _copy) {
this._parentBuilder = _parentBuilder;
if (_other!= null) {
if (_copy) {
_storedValue = null;
this.publisher = ((_other.publisher == null)?null:_other.publisher.newCopyBuilder(this));
if (_other.creators == null) {
this.creators = null;
} else {
this.creators = new ArrayList<>();
for (Creator _item: _other.creators) {
this.creators.add(((_item == null)?null:_item.newCopyBuilder(this)));
}
}
if (_other.contributors == null) {
this.contributors = null;
} else {
this.contributors = new ArrayList<>();
for (ResourceName _item: _other.contributors) {
this.contributors.add(((_item == null)?null:_item.newCopyBuilder(this)));
}
}
if (_other.dates == null) {
this.dates = null;
} else {
this.dates = new ArrayList<>();
for (Date _item: _other.dates) {
this.dates.add(((_item == null)?null:_item.newCopyBuilder(this)));
}
}
this.version = _other.version;
if (_other.contacts == null) {
this.contacts = null;
} else {
this.contacts = new ArrayList<>();
for (Contact _item: _other.contacts) {
this.contacts.add(((_item == null)?null:_item.newCopyBuilder(this)));
}
}
} else {
_storedValue = _other;
}
} else {
_storedValue = null;
}
}
public Builder(final _B _parentBuilder, final Curation _other, final boolean _copy, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
this._parentBuilder = _parentBuilder;
if (_other!= null) {
if (_copy) {
_storedValue = null;
final PropertyTree publisherPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("publisher"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(publisherPropertyTree!= null):((publisherPropertyTree == null)||(!publisherPropertyTree.isLeaf())))) {
this.publisher = ((_other.publisher == null)?null:_other.publisher.newCopyBuilder(this, publisherPropertyTree, _propertyTreeUse));
}
final PropertyTree creatorsPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("creators"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(creatorsPropertyTree!= null):((creatorsPropertyTree == null)||(!creatorsPropertyTree.isLeaf())))) {
if (_other.creators == null) {
this.creators = null;
} else {
this.creators = new ArrayList<>();
for (Creator _item: _other.creators) {
this.creators.add(((_item == null)?null:_item.newCopyBuilder(this, creatorsPropertyTree, _propertyTreeUse)));
}
}
}
final PropertyTree contributorsPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("contributors"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(contributorsPropertyTree!= null):((contributorsPropertyTree == null)||(!contributorsPropertyTree.isLeaf())))) {
if (_other.contributors == null) {
this.contributors = null;
} else {
this.contributors = new ArrayList<>();
for (ResourceName _item: _other.contributors) {
this.contributors.add(((_item == null)?null:_item.newCopyBuilder(this, contributorsPropertyTree, _propertyTreeUse)));
}
}
}
final PropertyTree datesPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("dates"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(datesPropertyTree!= null):((datesPropertyTree == null)||(!datesPropertyTree.isLeaf())))) {
if (_other.dates == null) {
this.dates = null;
} else {
this.dates = new ArrayList<>();
for (Date _item: _other.dates) {
this.dates.add(((_item == null)?null:_item.newCopyBuilder(this, datesPropertyTree, _propertyTreeUse)));
}
}
}
final PropertyTree versionPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("version"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(versionPropertyTree!= null):((versionPropertyTree == null)||(!versionPropertyTree.isLeaf())))) {
this.version = _other.version;
}
final PropertyTree contactsPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("contacts"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(contactsPropertyTree!= null):((contactsPropertyTree == null)||(!contactsPropertyTree.isLeaf())))) {
if (_other.contacts == null) {
this.contacts = null;
} else {
this.contacts = new ArrayList<>();
for (Contact _item: _other.contacts) {
this.contacts.add(((_item == null)?null:_item.newCopyBuilder(this, contactsPropertyTree, _propertyTreeUse)));
}
}
}
} else {
_storedValue = _other;
}
} else {
_storedValue = null;
}
}
public _B end() {
return this._parentBuilder;
}
protected<_P extends Curation >_P init(final _P _product) {
_product.publisher = ((this.publisher == null)?null:this.publisher.build());
if (this.creators!= null) {
final List creators = new ArrayList<>(this.creators.size());
for (Creator.Builder> _item: this.creators) {
creators.add(_item.build());
}
_product.creators = creators;
}
if (this.contributors!= null) {
final List contributors = new ArrayList<>(this.contributors.size());
for (ResourceName.Builder> _item: this.contributors) {
contributors.add(_item.build());
}
_product.contributors = contributors;
}
if (this.dates!= null) {
final List dates = new ArrayList<>(this.dates.size());
for (Date.Builder> _item: this.dates) {
dates.add(_item.build());
}
_product.dates = dates;
}
_product.version = this.version;
if (this.contacts!= null) {
final List contacts = new ArrayList<>(this.contacts.size());
for (Contact.Builder> _item: this.contacts) {
contacts.add(_item.build());
}
_product.contacts = contacts;
}
return _product;
}
/**
* Sets the new value of "publisher" (any previous value will be replaced)
*
* @param publisher
* New value of the "publisher" property.
*/
public Curation.Builder<_B> withPublisher(final ResourceName publisher) {
this.publisher = ((publisher == null)?null:new ResourceName.Builder<>(this, publisher, false));
return this;
}
/**
* Returns the existing builder or a new builder to build the value of the
* "publisher" property.
* Use {@link org.javastro.ivoa.entities.resource.ResourceName.Builder#end()} to
* return to the current builder.
*
* @return
* A new builder to build the value of the "publisher" property.
* Use {@link org.javastro.ivoa.entities.resource.ResourceName.Builder#end()} to
* return to the current builder.
*/
public ResourceName.Builder extends Curation.Builder<_B>> withPublisher() {
if (this.publisher!= null) {
return this.publisher;
}
return this.publisher = new ResourceName.Builder<>(this, null, false);
}
/**
* Adds the given items to the value of "creators"
*
* @param creators
* Items to add to the value of the "creators" property
*/
public Curation.Builder<_B> addCreators(final Iterable extends Creator> creators) {
if (creators!= null) {
if (this.creators == null) {
this.creators = new ArrayList<>();
}
for (Creator _item: creators) {
this.creators.add(new Creator.Builder<>(this, _item, false));
}
}
return this;
}
/**
* Sets the new value of "creators" (any previous value will be replaced)
*
* @param creators
* New value of the "creators" property.
*/
public Curation.Builder<_B> withCreators(final Iterable extends Creator> creators) {
if (this.creators!= null) {
this.creators.clear();
}
return addCreators(creators);
}
/**
* Adds the given items to the value of "creators"
*
* @param creators
* Items to add to the value of the "creators" property
*/
public Curation.Builder<_B> addCreators(Creator... creators) {
addCreators(Arrays.asList(creators));
return this;
}
/**
* Sets the new value of "creators" (any previous value will be replaced)
*
* @param creators
* New value of the "creators" property.
*/
public Curation.Builder<_B> withCreators(Creator... creators) {
withCreators(Arrays.asList(creators));
return this;
}
/**
* Returns a new builder to build an additional value of the "Creators" property.
* Use {@link org.javastro.ivoa.entities.resource.Creator.Builder#end()} to return
* to the current builder.
*
* @return
* a new builder to build an additional value of the "Creators" property.
* Use {@link org.javastro.ivoa.entities.resource.Creator.Builder#end()} to return
* to the current builder.
*/
public Creator.Builder extends Curation.Builder<_B>> addCreators() {
if (this.creators == null) {
this.creators = new ArrayList<>();
}
final Creator.Builder> creators_Builder = new Creator.Builder<>(this, null, false);
this.creators.add(creators_Builder);
return creators_Builder;
}
/**
* Adds the given items to the value of "contributors"
*
* @param contributors
* Items to add to the value of the "contributors" property
*/
public Curation.Builder<_B> addContributors(final Iterable extends ResourceName> contributors) {
if (contributors!= null) {
if (this.contributors == null) {
this.contributors = new ArrayList<>();
}
for (ResourceName _item: contributors) {
this.contributors.add(new ResourceName.Builder<>(this, _item, false));
}
}
return this;
}
/**
* Sets the new value of "contributors" (any previous value will be replaced)
*
* @param contributors
* New value of the "contributors" property.
*/
public Curation.Builder<_B> withContributors(final Iterable extends ResourceName> contributors) {
if (this.contributors!= null) {
this.contributors.clear();
}
return addContributors(contributors);
}
/**
* Adds the given items to the value of "contributors"
*
* @param contributors
* Items to add to the value of the "contributors" property
*/
public Curation.Builder<_B> addContributors(ResourceName... contributors) {
addContributors(Arrays.asList(contributors));
return this;
}
/**
* Sets the new value of "contributors" (any previous value will be replaced)
*
* @param contributors
* New value of the "contributors" property.
*/
public Curation.Builder<_B> withContributors(ResourceName... contributors) {
withContributors(Arrays.asList(contributors));
return this;
}
/**
* Returns a new builder to build an additional value of the "Contributors"
* property.
* Use {@link org.javastro.ivoa.entities.resource.ResourceName.Builder#end()} to
* return to the current builder.
*
* @return
* a new builder to build an additional value of the "Contributors" property.
* Use {@link org.javastro.ivoa.entities.resource.ResourceName.Builder#end()} to
* return to the current builder.
*/
public ResourceName.Builder extends Curation.Builder<_B>> addContributors() {
if (this.contributors == null) {
this.contributors = new ArrayList<>();
}
final ResourceName.Builder> contributors_Builder = new ResourceName.Builder<>(this, null, false);
this.contributors.add(contributors_Builder);
return contributors_Builder;
}
/**
* Adds the given items to the value of "dates"
*
* @param dates
* Items to add to the value of the "dates" property
*/
public Curation.Builder<_B> addDates(final Iterable extends Date> dates) {
if (dates!= null) {
if (this.dates == null) {
this.dates = new ArrayList<>();
}
for (Date _item: dates) {
this.dates.add(new Date.Builder<>(this, _item, false));
}
}
return this;
}
/**
* Sets the new value of "dates" (any previous value will be replaced)
*
* @param dates
* New value of the "dates" property.
*/
public Curation.Builder<_B> withDates(final Iterable extends Date> dates) {
if (this.dates!= null) {
this.dates.clear();
}
return addDates(dates);
}
/**
* Adds the given items to the value of "dates"
*
* @param dates
* Items to add to the value of the "dates" property
*/
public Curation.Builder<_B> addDates(Date... dates) {
addDates(Arrays.asList(dates));
return this;
}
/**
* Sets the new value of "dates" (any previous value will be replaced)
*
* @param dates
* New value of the "dates" property.
*/
public Curation.Builder<_B> withDates(Date... dates) {
withDates(Arrays.asList(dates));
return this;
}
/**
* Returns a new builder to build an additional value of the "Dates" property.
* Use {@link org.javastro.ivoa.entities.resource.Date.Builder#end()} to return to
* the current builder.
*
* @return
* a new builder to build an additional value of the "Dates" property.
* Use {@link org.javastro.ivoa.entities.resource.Date.Builder#end()} to return to
* the current builder.
*/
public Date.Builder extends Curation.Builder<_B>> addDates() {
if (this.dates == null) {
this.dates = new ArrayList<>();
}
final Date.Builder> dates_Builder = new Date.Builder<>(this, null, false);
this.dates.add(dates_Builder);
return dates_Builder;
}
/**
* Sets the new value of "version" (any previous value will be replaced)
*
* @param version
* New value of the "version" property.
*/
public Curation.Builder<_B> withVersion(final String version) {
this.version = version;
return this;
}
/**
* Adds the given items to the value of "contacts"
*
* @param contacts
* Items to add to the value of the "contacts" property
*/
public Curation.Builder<_B> addContacts(final Iterable extends Contact> contacts) {
if (contacts!= null) {
if (this.contacts == null) {
this.contacts = new ArrayList<>();
}
for (Contact _item: contacts) {
this.contacts.add(new Contact.Builder<>(this, _item, false));
}
}
return this;
}
/**
* Sets the new value of "contacts" (any previous value will be replaced)
*
* @param contacts
* New value of the "contacts" property.
*/
public Curation.Builder<_B> withContacts(final Iterable extends Contact> contacts) {
if (this.contacts!= null) {
this.contacts.clear();
}
return addContacts(contacts);
}
/**
* Adds the given items to the value of "contacts"
*
* @param contacts
* Items to add to the value of the "contacts" property
*/
public Curation.Builder<_B> addContacts(Contact... contacts) {
addContacts(Arrays.asList(contacts));
return this;
}
/**
* Sets the new value of "contacts" (any previous value will be replaced)
*
* @param contacts
* New value of the "contacts" property.
*/
public Curation.Builder<_B> withContacts(Contact... contacts) {
withContacts(Arrays.asList(contacts));
return this;
}
/**
* Returns a new builder to build an additional value of the "Contacts" property.
* Use {@link org.javastro.ivoa.entities.resource.Contact.Builder#end()} to return
* to the current builder.
*
* @return
* a new builder to build an additional value of the "Contacts" property.
* Use {@link org.javastro.ivoa.entities.resource.Contact.Builder#end()} to return
* to the current builder.
*/
public Contact.Builder extends Curation.Builder<_B>> addContacts() {
if (this.contacts == null) {
this.contacts = new ArrayList<>();
}
final Contact.Builder> contacts_Builder = new Contact.Builder<>(this, null, false);
this.contacts.add(contacts_Builder);
return contacts_Builder;
}
@Override
public Curation build() {
if (_storedValue == null) {
return this.init(new Curation());
} else {
return ((Curation) _storedValue);
}
}
public Curation.Builder<_B> copyOf(final Curation _other) {
_other.copyTo(this);
return this;
}
public Curation.Builder<_B> copyOf(final Curation.Builder _other) {
return copyOf(_other.build());
}
}
public class Modifier {
public void setPublisher(final ResourceName publisher) {
Curation.this.setPublisher(publisher);
}
public List getCreators() {
if (Curation.this.creators == null) {
Curation.this.creators = new ArrayList<>();
}
return Curation.this.creators;
}
public List getContributors() {
if (Curation.this.contributors == null) {
Curation.this.contributors = new ArrayList<>();
}
return Curation.this.contributors;
}
public List getDates() {
if (Curation.this.dates == null) {
Curation.this.dates = new ArrayList<>();
}
return Curation.this.dates;
}
public void setVersion(final String version) {
Curation.this.setVersion(version);
}
public List getContacts() {
if (Curation.this.contacts == null) {
Curation.this.contacts = new ArrayList<>();
}
return Curation.this.contacts;
}
}
public static class PropInfo {
public static final transient SinglePropertyInfo PUBLISHER = new SinglePropertyInfo("publisher", Curation.class, ResourceName.class, false, null, new QName("", "publisher"), new QName("http://www.ivoa.net/xml/VOResource/v1.0", "ResourceName"), false) {
@Override
public ResourceName get(final Curation _instance_) {
return ((_instance_ == null)?null:_instance_.publisher);
}
@Override
public void set(final Curation _instance_, final ResourceName _value_) {
if (_instance_!= null) {
_instance_.publisher = _value_;
}
}
}
;
public static final transient CollectionPropertyInfo CREATORS = new CollectionPropertyInfo("creators", Curation.class, Creator.class, true, null, new QName("", "creator"), new QName("http://www.ivoa.net/xml/VOResource/v1.0", "Creator"), false) {
@Override
public List get(final Curation _instance_) {
return ((_instance_ == null)?null:_instance_.creators);
}
@Override
public void set(final Curation _instance_, final List _value_) {
if (_instance_!= null) {
_instance_.creators = _value_;
}
}
}
;
public static final transient CollectionPropertyInfo CONTRIBUTORS = new CollectionPropertyInfo("contributors", Curation.class, ResourceName.class, true, null, new QName("", "contributor"), new QName("http://www.ivoa.net/xml/VOResource/v1.0", "ResourceName"), false) {
@Override
public List get(final Curation _instance_) {
return ((_instance_ == null)?null:_instance_.contributors);
}
@Override
public void set(final Curation _instance_, final List _value_) {
if (_instance_!= null) {
_instance_.contributors = _value_;
}
}
}
;
public static final transient CollectionPropertyInfo DATES = new CollectionPropertyInfo("dates", Curation.class, Date.class, true, null, new QName("", "date"), new QName("http://www.ivoa.net/xml/VOResource/v1.0", "Date"), false) {
@Override
public List get(final Curation _instance_) {
return ((_instance_ == null)?null:_instance_.dates);
}
@Override
public void set(final Curation _instance_, final List _value_) {
if (_instance_!= null) {
_instance_.dates = _value_;
}
}
}
;
public static final transient SinglePropertyInfo VERSION = new SinglePropertyInfo("version", Curation.class, String.class, false, null, new QName("", "version"), new QName("http://www.w3.org/2001/XMLSchema", "token"), false) {
@Override
public String get(final Curation _instance_) {
return ((_instance_ == null)?null:_instance_.version);
}
@Override
public void set(final Curation _instance_, final String _value_) {
if (_instance_!= null) {
_instance_.version = _value_;
}
}
}
;
public static final transient CollectionPropertyInfo CONTACTS = new CollectionPropertyInfo("contacts", Curation.class, Contact.class, true, null, new QName("", "contact"), new QName("http://www.ivoa.net/xml/VOResource/v1.0", "Contact"), false) {
@Override
public List get(final Curation _instance_) {
return ((_instance_ == null)?null:_instance_.contacts);
}
@Override
public void set(final Curation _instance_, final List _value_) {
if (_instance_!= null) {
_instance_.contacts = _value_;
}
}
}
;
}
public static class Select
extends Curation.Selector
{
Select() {
super(null, null, null);
}
public static Curation.Select _root() {
return new Curation.Select();
}
}
public static class Selector , TParent >
extends com.kscs.util.jaxb.Selector
{
private ResourceName.Selector> publisher = null;
private Creator.Selector> creators = null;
private ResourceName.Selector> contributors = null;
private Date.Selector> dates = null;
private com.kscs.util.jaxb.Selector> version = null;
private Contact.Selector> contacts = null;
public Selector(final TRoot root, final TParent parent, final String propertyName) {
super(root, parent, propertyName);
}
@Override
public Map buildChildren() {
final Map products = new HashMap<>();
products.putAll(super.buildChildren());
if (this.publisher!= null) {
products.put("publisher", this.publisher.init());
}
if (this.creators!= null) {
products.put("creators", this.creators.init());
}
if (this.contributors!= null) {
products.put("contributors", this.contributors.init());
}
if (this.dates!= null) {
products.put("dates", this.dates.init());
}
if (this.version!= null) {
products.put("version", this.version.init());
}
if (this.contacts!= null) {
products.put("contacts", this.contacts.init());
}
return products;
}
public ResourceName.Selector> publisher() {
return ((this.publisher == null)?this.publisher = new ResourceName.Selector<>(this._root, this, "publisher"):this.publisher);
}
public Creator.Selector> creators() {
return ((this.creators == null)?this.creators = new Creator.Selector<>(this._root, this, "creators"):this.creators);
}
public ResourceName.Selector> contributors() {
return ((this.contributors == null)?this.contributors = new ResourceName.Selector<>(this._root, this, "contributors"):this.contributors);
}
public Date.Selector> dates() {
return ((this.dates == null)?this.dates = new Date.Selector<>(this._root, this, "dates"):this.dates);
}
public com.kscs.util.jaxb.Selector> version() {
return ((this.version == null)?this.version = new com.kscs.util.jaxb.Selector<>(this._root, this, "version"):this.version);
}
public Contact.Selector> contacts() {
return ((this.contacts == null)?this.contacts = new Contact.Selector<>(this._root, this, "contacts"):this.contacts);
}
}
}