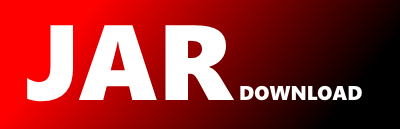
org.javastro.ivoa.entities.resource.Relationship Maven / Gradle / Ivy
package org.javastro.ivoa.entities.resource;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import javax.xml.namespace.QName;
import com.kscs.util.jaxb.Buildable;
import com.kscs.util.jaxb.CollectionProperty;
import com.kscs.util.jaxb.CollectionPropertyInfo;
import com.kscs.util.jaxb.CollectionPropertyInfo;
import com.kscs.util.jaxb.Copyable;
import com.kscs.util.jaxb.PartialCopyable;
import com.kscs.util.jaxb.PropertyTree;
import com.kscs.util.jaxb.PropertyTreeUse;
import com.kscs.util.jaxb.PropertyVisitor;
import com.kscs.util.jaxb.SingleProperty;
import com.kscs.util.jaxb.SinglePropertyInfo;
import com.kscs.util.jaxb.SinglePropertyInfo;
import jakarta.annotation.Generated;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlSchemaType;
import jakarta.xml.bind.annotation.XmlType;
import jakarta.xml.bind.annotation.adapters.CollapsedStringAdapter;
import jakarta.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import org.jvnet.jaxb.lang.JAXBMergeStrategy;
import org.jvnet.jaxb.lang.JAXBToStringStrategy;
import org.jvnet.jaxb.lang.MergeFrom;
import org.jvnet.jaxb.lang.MergeStrategy;
import org.jvnet.jaxb.lang.ToString;
import org.jvnet.jaxb.lang.ToStringStrategy;
import org.jvnet.jaxb.locator.ObjectLocator;
import org.jvnet.jaxb.locator.util.LocatorUtils;
/**
* A description of the relationship between one resource and one or
* more other resources.
*
* Java class for Relationship complex type
.
*
* The following schema fragment specifies the expected content contained within this class.
*
* {@code
*
*
*
*
*
*
*
*
*
*
* }
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "Relationship", propOrder = {
"relationshipType",
"relatedResources"
})
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public class Relationship implements Cloneable, Copyable, PartialCopyable, MergeFrom, ToString
{
/**
* The value of relationshipType should be taken from the
* vocabulary at
* http://www.ivoa.net/rdf/voresource/relationship_type.
*
*/
@XmlElement(required = true)
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
@XmlSchemaType(name = "token")
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
protected String relationshipType;
/**
* the name of resource that this resource is related to.
*
*/
@XmlElement(name = "relatedResource", required = true)
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
protected List relatedResources;
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
protected transient Relationship.Modifier __cachedModifier__;
/**
* Default no-arg constructor
*
*/
public Relationship() {
super();
}
/**
* Fully-initialising value constructor
*
*/
public Relationship(final String relationshipType, final List relatedResources) {
this.relationshipType = relationshipType;
this.relatedResources = relatedResources;
}
/**
* The value of relationshipType should be taken from the
* vocabulary at
* http://www.ivoa.net/rdf/voresource/relationship_type.
*
* @return
* possible object is
* {@link String }
*
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public String getRelationshipType() {
return relationshipType;
}
/**
* Sets the value of the relationshipType property.
*
* @param value
* allowed object is
* {@link String }
*
* @see #getRelationshipType()
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void setRelationshipType(String value) {
this.relationshipType = value;
}
/**
* the name of resource that this resource is related to.
*
* Gets the value of the relatedResources property.
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the relatedResources property.
*
*
* For example, to add a new item, do as follows:
*
*
* getRelatedResources().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link ResourceName }
*
*
*
* @return
* The value of the relatedResources property.
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public List getRelatedResources() {
if (relatedResources == null) {
relatedResources = new ArrayList<>();
}
return this.relatedResources;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public boolean equals(Object object) {
if ((object == null)||(this.getClass()!= object.getClass())) {
return false;
}
if (this == object) {
return true;
}
final Relationship that = ((Relationship) object);
{
String leftRelationshipType;
leftRelationshipType = this.getRelationshipType();
String rightRelationshipType;
rightRelationshipType = that.getRelationshipType();
if (this.relationshipType!= null) {
if (that.relationshipType!= null) {
if (!leftRelationshipType.equals(rightRelationshipType)) {
return false;
}
} else {
return false;
}
} else {
if (that.relationshipType!= null) {
return false;
}
}
}
{
List leftRelatedResources;
leftRelatedResources = (((this.relatedResources!= null)&&(!this.relatedResources.isEmpty()))?this.getRelatedResources():null);
List rightRelatedResources;
rightRelatedResources = (((that.relatedResources!= null)&&(!that.relatedResources.isEmpty()))?that.getRelatedResources():null);
if ((this.relatedResources!= null)&&(!this.relatedResources.isEmpty())) {
if ((that.relatedResources!= null)&&(!that.relatedResources.isEmpty())) {
if (!leftRelatedResources.equals(rightRelatedResources)) {
return false;
}
} else {
return false;
}
} else {
if ((that.relatedResources!= null)&&(!that.relatedResources.isEmpty())) {
return false;
}
}
}
return true;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public int hashCode() {
int currentHashCode = 1;
{
currentHashCode = (currentHashCode* 31);
String theRelationshipType;
theRelationshipType = this.getRelationshipType();
if (this.relationshipType!= null) {
currentHashCode += theRelationshipType.hashCode();
}
}
{
currentHashCode = (currentHashCode* 31);
List theRelatedResources;
theRelatedResources = (((this.relatedResources!= null)&&(!this.relatedResources.isEmpty()))?this.getRelatedResources():null);
if ((this.relatedResources!= null)&&(!this.relatedResources.isEmpty())) {
currentHashCode += theRelatedResources.hashCode();
}
}
return currentHashCode;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public String toString() {
final ToStringStrategy strategy = JAXBToStringStrategy.getInstance();
final StringBuilder buffer = new StringBuilder();
append(null, buffer, strategy);
return buffer.toString();
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public StringBuilder append(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
strategy.appendStart(locator, this, buffer);
appendFields(locator, buffer, strategy);
strategy.appendEnd(locator, this, buffer);
return buffer;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public StringBuilder appendFields(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
{
String theRelationshipType;
theRelationshipType = this.getRelationshipType();
strategy.appendField(locator, this, "relationshipType", buffer, theRelationshipType, (this.relationshipType!= null));
}
{
List theRelatedResources;
theRelatedResources = (((this.relatedResources!= null)&&(!this.relatedResources.isEmpty()))?this.getRelatedResources():null);
strategy.appendField(locator, this, "relatedResources", buffer, theRelatedResources, ((this.relatedResources!= null)&&(!this.relatedResources.isEmpty())));
}
return buffer;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void mergeFrom(Object left, Object right) {
final MergeStrategy strategy = JAXBMergeStrategy.getInstance();
mergeFrom(null, null, left, right, strategy);
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void mergeFrom(ObjectLocator leftLocator, ObjectLocator rightLocator, Object left, Object right, MergeStrategy strategy) {
if (right instanceof Relationship) {
final Relationship target = this;
final Relationship leftObject = ((Relationship) left);
final Relationship rightObject = ((Relationship) right);
{
Boolean relationshipTypeShouldBeMergedAndSet = strategy.shouldBeMergedAndSet(leftLocator, rightLocator, (leftObject.relationshipType!= null), (rightObject.relationshipType!= null));
if (relationshipTypeShouldBeMergedAndSet == Boolean.TRUE) {
String lhsRelationshipType;
lhsRelationshipType = leftObject.getRelationshipType();
String rhsRelationshipType;
rhsRelationshipType = rightObject.getRelationshipType();
String mergedRelationshipType = ((String) strategy.merge(LocatorUtils.property(leftLocator, "relationshipType", lhsRelationshipType), LocatorUtils.property(rightLocator, "relationshipType", rhsRelationshipType), lhsRelationshipType, rhsRelationshipType, (leftObject.relationshipType!= null), (rightObject.relationshipType!= null)));
target.setRelationshipType(mergedRelationshipType);
} else {
if (relationshipTypeShouldBeMergedAndSet == Boolean.FALSE) {
target.relationshipType = null;
}
}
}
{
Boolean relatedResourcesShouldBeMergedAndSet = strategy.shouldBeMergedAndSet(leftLocator, rightLocator, ((leftObject.relatedResources!= null)&&(!leftObject.relatedResources.isEmpty())), ((rightObject.relatedResources!= null)&&(!rightObject.relatedResources.isEmpty())));
if (relatedResourcesShouldBeMergedAndSet == Boolean.TRUE) {
List lhsRelatedResources;
lhsRelatedResources = (((leftObject.relatedResources!= null)&&(!leftObject.relatedResources.isEmpty()))?leftObject.getRelatedResources():null);
List rhsRelatedResources;
rhsRelatedResources = (((rightObject.relatedResources!= null)&&(!rightObject.relatedResources.isEmpty()))?rightObject.getRelatedResources():null);
List mergedRelatedResources = ((List ) strategy.merge(LocatorUtils.property(leftLocator, "relatedResources", lhsRelatedResources), LocatorUtils.property(rightLocator, "relatedResources", rhsRelatedResources), lhsRelatedResources, rhsRelatedResources, ((leftObject.relatedResources!= null)&&(!leftObject.relatedResources.isEmpty())), ((rightObject.relatedResources!= null)&&(!rightObject.relatedResources.isEmpty()))));
target.relatedResources = null;
if (mergedRelatedResources!= null) {
List uniqueRelatedResourcesl = target.getRelatedResources();
uniqueRelatedResourcesl.addAll(mergedRelatedResources);
}
} else {
if (relatedResourcesShouldBeMergedAndSet == Boolean.FALSE) {
target.relatedResources = null;
}
}
}
}
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Object createNewInstance() {
return new Relationship();
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Relationship clone() {
final Relationship _newObject;
try {
_newObject = ((Relationship) super.clone());
} catch (CloneNotSupportedException e) {
throw new RuntimeException(e);
}
if (this.relatedResources == null) {
_newObject.relatedResources = null;
} else {
_newObject.relatedResources = new ArrayList<>();
for (ResourceName _item: this.relatedResources) {
_newObject.relatedResources.add(((_item == null)?null:_item.clone()));
}
}
return _newObject;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Relationship createCopy() {
final Relationship _newObject;
try {
_newObject = ((Relationship) super.clone());
} catch (CloneNotSupportedException e) {
throw new RuntimeException(e);
}
_newObject.relationshipType = this.relationshipType;
if (this.relatedResources == null) {
_newObject.relatedResources = null;
} else {
_newObject.relatedResources = new ArrayList<>();
for (ResourceName _item: this.relatedResources) {
_newObject.relatedResources.add(((_item == null)?null:_item.createCopy()));
}
}
return _newObject;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Relationship createCopy(final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
final Relationship _newObject;
try {
_newObject = ((Relationship) super.clone());
} catch (CloneNotSupportedException e) {
throw new RuntimeException(e);
}
final PropertyTree relationshipTypePropertyTree = ((_propertyTree == null)?null:_propertyTree.get("relationshipType"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(relationshipTypePropertyTree!= null):((relationshipTypePropertyTree == null)||(!relationshipTypePropertyTree.isLeaf())))) {
_newObject.relationshipType = this.relationshipType;
}
final PropertyTree relatedResourcesPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("relatedResources"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(relatedResourcesPropertyTree!= null):((relatedResourcesPropertyTree == null)||(!relatedResourcesPropertyTree.isLeaf())))) {
if (this.relatedResources == null) {
_newObject.relatedResources = null;
} else {
_newObject.relatedResources = new ArrayList<>();
for (ResourceName _item: this.relatedResources) {
_newObject.relatedResources.add(((_item == null)?null:_item.createCopy(relatedResourcesPropertyTree, _propertyTreeUse)));
}
}
}
return _newObject;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Relationship copyExcept(final PropertyTree _propertyTree) {
return createCopy(_propertyTree, PropertyTreeUse.EXCLUDE);
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Relationship copyOnly(final PropertyTree _propertyTree) {
return createCopy(_propertyTree, PropertyTreeUse.INCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Relationship.Modifier modifier() {
if (null == this.__cachedModifier__) {
this.__cachedModifier__ = new Relationship.Modifier();
}
return ((Relationship.Modifier) this.__cachedModifier__);
}
/**
* Copies all state of this object to a builder. This method is used by the copyOf
* method and should not be called directly by client code.
*
* @param _other
* A builder instance to which the state of this object will be copied.
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public<_B >void copyTo(final Relationship.Builder<_B> _other) {
_other.relationshipType = this.relationshipType;
if (this.relatedResources == null) {
_other.relatedResources = null;
} else {
_other.relatedResources = new ArrayList<>();
for (ResourceName _item: this.relatedResources) {
_other.relatedResources.add(((_item == null)?null:_item.newCopyBuilder(_other)));
}
}
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public<_B >Relationship.Builder<_B> newCopyBuilder(final _B _parentBuilder) {
return new Relationship.Builder<_B>(_parentBuilder, this, true);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Relationship.Builder newCopyBuilder() {
return newCopyBuilder(null);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static Relationship.Builder builder() {
return new Relationship.Builder<>(null, null, false);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static<_B >Relationship.Builder<_B> copyOf(final Relationship _other) {
final Relationship.Builder<_B> _newBuilder = new Relationship.Builder<>(null, null, false);
_other.copyTo(_newBuilder);
return _newBuilder;
}
/**
* Copies all state of this object to a builder. This method is used by the copyOf
* method and should not be called directly by client code.
*
* @param _other
* A builder instance to which the state of this object will be copied.
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public<_B >void copyTo(final Relationship.Builder<_B> _other, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
final PropertyTree relationshipTypePropertyTree = ((_propertyTree == null)?null:_propertyTree.get("relationshipType"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(relationshipTypePropertyTree!= null):((relationshipTypePropertyTree == null)||(!relationshipTypePropertyTree.isLeaf())))) {
_other.relationshipType = this.relationshipType;
}
final PropertyTree relatedResourcesPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("relatedResources"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(relatedResourcesPropertyTree!= null):((relatedResourcesPropertyTree == null)||(!relatedResourcesPropertyTree.isLeaf())))) {
if (this.relatedResources == null) {
_other.relatedResources = null;
} else {
_other.relatedResources = new ArrayList<>();
for (ResourceName _item: this.relatedResources) {
_other.relatedResources.add(((_item == null)?null:_item.newCopyBuilder(_other, relatedResourcesPropertyTree, _propertyTreeUse)));
}
}
}
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public<_B >Relationship.Builder<_B> newCopyBuilder(final _B _parentBuilder, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
return new Relationship.Builder<_B>(_parentBuilder, this, true, _propertyTree, _propertyTreeUse);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Relationship.Builder newCopyBuilder(final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
return newCopyBuilder(null, _propertyTree, _propertyTreeUse);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static<_B >Relationship.Builder<_B> copyOf(final Relationship _other, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
final Relationship.Builder<_B> _newBuilder = new Relationship.Builder<>(null, null, false);
_other.copyTo(_newBuilder, _propertyTree, _propertyTreeUse);
return _newBuilder;
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static Relationship.Builder copyExcept(final Relationship _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.EXCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static Relationship.Builder copyOnly(final Relationship _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.INCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Relationship visit(final PropertyVisitor _visitor_) {
_visitor_.visit(this);
_visitor_.visit(new SingleProperty<>(Relationship.PropInfo.RELATIONSHIP_TYPE, this));
if (_visitor_.visit(new CollectionProperty<>(Relationship.PropInfo.RELATED_RESOURCES, this))&&(this.relatedResources!= null)) {
for (ResourceName _item_: this.relatedResources) {
if (_item_!= null) {
_item_.visit(_visitor_);
}
}
}
return this;
}
public static class Builder<_B >implements Buildable
{
protected final _B _parentBuilder;
protected final Relationship _storedValue;
private String relationshipType;
private List>> relatedResources;
public Builder(final _B _parentBuilder, final Relationship _other, final boolean _copy) {
this._parentBuilder = _parentBuilder;
if (_other!= null) {
if (_copy) {
_storedValue = null;
this.relationshipType = _other.relationshipType;
if (_other.relatedResources == null) {
this.relatedResources = null;
} else {
this.relatedResources = new ArrayList<>();
for (ResourceName _item: _other.relatedResources) {
this.relatedResources.add(((_item == null)?null:_item.newCopyBuilder(this)));
}
}
} else {
_storedValue = _other;
}
} else {
_storedValue = null;
}
}
public Builder(final _B _parentBuilder, final Relationship _other, final boolean _copy, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
this._parentBuilder = _parentBuilder;
if (_other!= null) {
if (_copy) {
_storedValue = null;
final PropertyTree relationshipTypePropertyTree = ((_propertyTree == null)?null:_propertyTree.get("relationshipType"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(relationshipTypePropertyTree!= null):((relationshipTypePropertyTree == null)||(!relationshipTypePropertyTree.isLeaf())))) {
this.relationshipType = _other.relationshipType;
}
final PropertyTree relatedResourcesPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("relatedResources"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(relatedResourcesPropertyTree!= null):((relatedResourcesPropertyTree == null)||(!relatedResourcesPropertyTree.isLeaf())))) {
if (_other.relatedResources == null) {
this.relatedResources = null;
} else {
this.relatedResources = new ArrayList<>();
for (ResourceName _item: _other.relatedResources) {
this.relatedResources.add(((_item == null)?null:_item.newCopyBuilder(this, relatedResourcesPropertyTree, _propertyTreeUse)));
}
}
}
} else {
_storedValue = _other;
}
} else {
_storedValue = null;
}
}
public _B end() {
return this._parentBuilder;
}
protected<_P extends Relationship >_P init(final _P _product) {
_product.relationshipType = this.relationshipType;
if (this.relatedResources!= null) {
final List relatedResources = new ArrayList<>(this.relatedResources.size());
for (ResourceName.Builder> _item: this.relatedResources) {
relatedResources.add(_item.build());
}
_product.relatedResources = relatedResources;
}
return _product;
}
/**
* Sets the new value of "relationshipType" (any previous value will be replaced)
*
* @param relationshipType
* New value of the "relationshipType" property.
*/
public Relationship.Builder<_B> withRelationshipType(final String relationshipType) {
this.relationshipType = relationshipType;
return this;
}
/**
* Adds the given items to the value of "relatedResources"
*
* @param relatedResources
* Items to add to the value of the "relatedResources" property
*/
public Relationship.Builder<_B> addRelatedResources(final Iterable extends ResourceName> relatedResources) {
if (relatedResources!= null) {
if (this.relatedResources == null) {
this.relatedResources = new ArrayList<>();
}
for (ResourceName _item: relatedResources) {
this.relatedResources.add(new ResourceName.Builder<>(this, _item, false));
}
}
return this;
}
/**
* Sets the new value of "relatedResources" (any previous value will be replaced)
*
* @param relatedResources
* New value of the "relatedResources" property.
*/
public Relationship.Builder<_B> withRelatedResources(final Iterable extends ResourceName> relatedResources) {
if (this.relatedResources!= null) {
this.relatedResources.clear();
}
return addRelatedResources(relatedResources);
}
/**
* Adds the given items to the value of "relatedResources"
*
* @param relatedResources
* Items to add to the value of the "relatedResources" property
*/
public Relationship.Builder<_B> addRelatedResources(ResourceName... relatedResources) {
addRelatedResources(Arrays.asList(relatedResources));
return this;
}
/**
* Sets the new value of "relatedResources" (any previous value will be replaced)
*
* @param relatedResources
* New value of the "relatedResources" property.
*/
public Relationship.Builder<_B> withRelatedResources(ResourceName... relatedResources) {
withRelatedResources(Arrays.asList(relatedResources));
return this;
}
/**
* Returns a new builder to build an additional value of the "RelatedResources"
* property.
* Use {@link org.javastro.ivoa.entities.resource.ResourceName.Builder#end()} to
* return to the current builder.
*
* @return
* a new builder to build an additional value of the "RelatedResources" property.
* Use {@link org.javastro.ivoa.entities.resource.ResourceName.Builder#end()} to
* return to the current builder.
*/
public ResourceName.Builder extends Relationship.Builder<_B>> addRelatedResources() {
if (this.relatedResources == null) {
this.relatedResources = new ArrayList<>();
}
final ResourceName.Builder> relatedResources_Builder = new ResourceName.Builder<>(this, null, false);
this.relatedResources.add(relatedResources_Builder);
return relatedResources_Builder;
}
@Override
public Relationship build() {
if (_storedValue == null) {
return this.init(new Relationship());
} else {
return ((Relationship) _storedValue);
}
}
public Relationship.Builder<_B> copyOf(final Relationship _other) {
_other.copyTo(this);
return this;
}
public Relationship.Builder<_B> copyOf(final Relationship.Builder _other) {
return copyOf(_other.build());
}
}
public class Modifier {
public void setRelationshipType(final String relationshipType) {
Relationship.this.setRelationshipType(relationshipType);
}
public List getRelatedResources() {
if (Relationship.this.relatedResources == null) {
Relationship.this.relatedResources = new ArrayList<>();
}
return Relationship.this.relatedResources;
}
}
public static class PropInfo {
public static final transient SinglePropertyInfo RELATIONSHIP_TYPE = new SinglePropertyInfo("relationshipType", Relationship.class, String.class, false, null, new QName("", "relationshipType"), new QName("http://www.w3.org/2001/XMLSchema", "token"), false) {
@Override
public String get(final Relationship _instance_) {
return ((_instance_ == null)?null:_instance_.relationshipType);
}
@Override
public void set(final Relationship _instance_, final String _value_) {
if (_instance_!= null) {
_instance_.relationshipType = _value_;
}
}
}
;
public static final transient CollectionPropertyInfo RELATED_RESOURCES = new CollectionPropertyInfo("relatedResources", Relationship.class, ResourceName.class, true, null, new QName("", "relatedResource"), new QName("http://www.ivoa.net/xml/VOResource/v1.0", "ResourceName"), false) {
@Override
public List get(final Relationship _instance_) {
return ((_instance_ == null)?null:_instance_.relatedResources);
}
@Override
public void set(final Relationship _instance_, final List _value_) {
if (_instance_!= null) {
_instance_.relatedResources = _value_;
}
}
}
;
}
public static class Select
extends Relationship.Selector
{
Select() {
super(null, null, null);
}
public static Relationship.Select _root() {
return new Relationship.Select();
}
}
public static class Selector , TParent >
extends com.kscs.util.jaxb.Selector
{
private com.kscs.util.jaxb.Selector> relationshipType = null;
private ResourceName.Selector> relatedResources = null;
public Selector(final TRoot root, final TParent parent, final String propertyName) {
super(root, parent, propertyName);
}
@Override
public Map buildChildren() {
final Map products = new HashMap<>();
products.putAll(super.buildChildren());
if (this.relationshipType!= null) {
products.put("relationshipType", this.relationshipType.init());
}
if (this.relatedResources!= null) {
products.put("relatedResources", this.relatedResources.init());
}
return products;
}
public com.kscs.util.jaxb.Selector> relationshipType() {
return ((this.relationshipType == null)?this.relationshipType = new com.kscs.util.jaxb.Selector<>(this._root, this, "relationshipType"):this.relationshipType);
}
public ResourceName.Selector> relatedResources() {
return ((this.relatedResources == null)?this.relatedResources = new ResourceName.Selector<>(this._root, this, "relatedResources"):this.relatedResources);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy