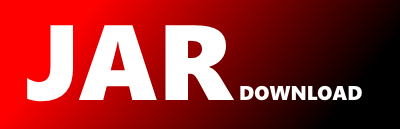
org.javastro.ivoa.entities.resource.Service Maven / Gradle / Ivy
package org.javastro.ivoa.entities.resource;
import java.time.LocalDateTime;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import javax.xml.namespace.QName;
import com.kscs.util.jaxb.Buildable;
import com.kscs.util.jaxb.CollectionProperty;
import com.kscs.util.jaxb.CollectionPropertyInfo;
import com.kscs.util.jaxb.CollectionPropertyInfo;
import com.kscs.util.jaxb.CollectionPropertyInfo;
import com.kscs.util.jaxb.Copyable;
import com.kscs.util.jaxb.PartialCopyable;
import com.kscs.util.jaxb.PropertyTree;
import com.kscs.util.jaxb.PropertyTreeUse;
import com.kscs.util.jaxb.PropertyVisitor;
import jakarta.annotation.Generated;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlSeeAlso;
import jakarta.xml.bind.annotation.XmlType;
import org.javastro.ivoa.entities.resource.dataservice.DataResource;
import org.javastro.ivoa.entities.resource.registry.Registry;
import org.jvnet.jaxb.lang.JAXBMergeStrategy;
import org.jvnet.jaxb.lang.JAXBToStringStrategy;
import org.jvnet.jaxb.lang.MergeFrom;
import org.jvnet.jaxb.lang.MergeStrategy;
import org.jvnet.jaxb.lang.ToString;
import org.jvnet.jaxb.lang.ToStringStrategy;
import org.jvnet.jaxb.locator.ObjectLocator;
import org.jvnet.jaxb.locator.util.LocatorUtils;
/**
* a resource that can be invoked by a client to perform some action
* on its behalf.
*
* Java class for Service complex type
.
*
* The following schema fragment specifies the expected content contained within this class.
*
* {@code
*
*
*
*
*
*
*
*
*
*
* }
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "Service", propOrder = {
"rights",
"capabilities"
})
@XmlSeeAlso({
DataResource.class,
Registry.class
})
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public class Service
extends Resource
implements Cloneable, Copyable, PartialCopyable, MergeFrom, ToString
{
/**
* Mainly for compatibility with DataCite, this element
* is repeatable. Resource record authors are advised
* that within the Virtual Observatory clients will
* typically only display and/or use the rights
* element occurring first and ignore later elements.
*
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
protected List rights;
/**
* A service can have many capabilities
* associated with it, each reflecting different
* aspects of the functionality it provides.
*
*/
@XmlElement(name = "capability")
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
protected List capabilities;
/**
* Default no-arg constructor
*
*/
public Service() {
super();
}
/**
* Fully-initialising value constructor
*
*/
public Service(final List validationLevels, final String title, final String shortName, final String identifier, final List altIdentifiers, final Curation curation, final Content content, final LocalDateTime created, final LocalDateTime updated, final String status, final String version, final List rights, final List capabilities) {
super(validationLevels, title, shortName, identifier, altIdentifiers, curation, content, created, updated, status, version);
this.rights = rights;
this.capabilities = capabilities;
}
/**
* Mainly for compatibility with DataCite, this element
* is repeatable. Resource record authors are advised
* that within the Virtual Observatory clients will
* typically only display and/or use the rights
* element occurring first and ignore later elements.
*
* Gets the value of the rights property.
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the rights property.
*
*
* For example, to add a new item, do as follows:
*
*
* getRights().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Rights }
*
*
*
* @return
* The value of the rights property.
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public List getRights() {
if (rights == null) {
rights = new ArrayList<>();
}
return this.rights;
}
/**
* A service can have many capabilities
* associated with it, each reflecting different
* aspects of the functionality it provides.
*
* Gets the value of the capabilities property.
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the capabilities property.
*
*
* For example, to add a new item, do as follows:
*
*
* getCapabilities().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Capability }
*
*
*
* @return
* The value of the capabilities property.
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public List getCapabilities() {
if (capabilities == null) {
capabilities = new ArrayList<>();
}
return this.capabilities;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public boolean equals(Object object) {
if ((object == null)||(this.getClass()!= object.getClass())) {
return false;
}
if (this == object) {
return true;
}
if (!super.equals(object)) {
return false;
}
final Service that = ((Service) object);
{
List leftRights;
leftRights = (((this.rights!= null)&&(!this.rights.isEmpty()))?this.getRights():null);
List rightRights;
rightRights = (((that.rights!= null)&&(!that.rights.isEmpty()))?that.getRights():null);
if ((this.rights!= null)&&(!this.rights.isEmpty())) {
if ((that.rights!= null)&&(!that.rights.isEmpty())) {
if (!leftRights.equals(rightRights)) {
return false;
}
} else {
return false;
}
} else {
if ((that.rights!= null)&&(!that.rights.isEmpty())) {
return false;
}
}
}
{
List leftCapabilities;
leftCapabilities = (((this.capabilities!= null)&&(!this.capabilities.isEmpty()))?this.getCapabilities():null);
List rightCapabilities;
rightCapabilities = (((that.capabilities!= null)&&(!that.capabilities.isEmpty()))?that.getCapabilities():null);
if ((this.capabilities!= null)&&(!this.capabilities.isEmpty())) {
if ((that.capabilities!= null)&&(!that.capabilities.isEmpty())) {
if (!leftCapabilities.equals(rightCapabilities)) {
return false;
}
} else {
return false;
}
} else {
if ((that.capabilities!= null)&&(!that.capabilities.isEmpty())) {
return false;
}
}
}
return true;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public int hashCode() {
int currentHashCode = 1;
currentHashCode = ((currentHashCode* 31)+ super.hashCode());
{
currentHashCode = (currentHashCode* 31);
List theRights;
theRights = (((this.rights!= null)&&(!this.rights.isEmpty()))?this.getRights():null);
if ((this.rights!= null)&&(!this.rights.isEmpty())) {
currentHashCode += theRights.hashCode();
}
}
{
currentHashCode = (currentHashCode* 31);
List theCapabilities;
theCapabilities = (((this.capabilities!= null)&&(!this.capabilities.isEmpty()))?this.getCapabilities():null);
if ((this.capabilities!= null)&&(!this.capabilities.isEmpty())) {
currentHashCode += theCapabilities.hashCode();
}
}
return currentHashCode;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public String toString() {
final ToStringStrategy strategy = JAXBToStringStrategy.getInstance();
final StringBuilder buffer = new StringBuilder();
append(null, buffer, strategy);
return buffer.toString();
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public StringBuilder append(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
strategy.appendStart(locator, this, buffer);
appendFields(locator, buffer, strategy);
strategy.appendEnd(locator, this, buffer);
return buffer;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public StringBuilder appendFields(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
super.appendFields(locator, buffer, strategy);
{
List theRights;
theRights = (((this.rights!= null)&&(!this.rights.isEmpty()))?this.getRights():null);
strategy.appendField(locator, this, "rights", buffer, theRights, ((this.rights!= null)&&(!this.rights.isEmpty())));
}
{
List theCapabilities;
theCapabilities = (((this.capabilities!= null)&&(!this.capabilities.isEmpty()))?this.getCapabilities():null);
strategy.appendField(locator, this, "capabilities", buffer, theCapabilities, ((this.capabilities!= null)&&(!this.capabilities.isEmpty())));
}
return buffer;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void mergeFrom(Object left, Object right) {
final MergeStrategy strategy = JAXBMergeStrategy.getInstance();
mergeFrom(null, null, left, right, strategy);
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void mergeFrom(ObjectLocator leftLocator, ObjectLocator rightLocator, Object left, Object right, MergeStrategy strategy) {
super.mergeFrom(leftLocator, rightLocator, left, right, strategy);
if (right instanceof Service) {
final Service target = this;
final Service leftObject = ((Service) left);
final Service rightObject = ((Service) right);
{
Boolean rightsShouldBeMergedAndSet = strategy.shouldBeMergedAndSet(leftLocator, rightLocator, ((leftObject.rights!= null)&&(!leftObject.rights.isEmpty())), ((rightObject.rights!= null)&&(!rightObject.rights.isEmpty())));
if (rightsShouldBeMergedAndSet == Boolean.TRUE) {
List lhsRights;
lhsRights = (((leftObject.rights!= null)&&(!leftObject.rights.isEmpty()))?leftObject.getRights():null);
List rhsRights;
rhsRights = (((rightObject.rights!= null)&&(!rightObject.rights.isEmpty()))?rightObject.getRights():null);
List mergedRights = ((List ) strategy.merge(LocatorUtils.property(leftLocator, "rights", lhsRights), LocatorUtils.property(rightLocator, "rights", rhsRights), lhsRights, rhsRights, ((leftObject.rights!= null)&&(!leftObject.rights.isEmpty())), ((rightObject.rights!= null)&&(!rightObject.rights.isEmpty()))));
target.rights = null;
if (mergedRights!= null) {
List uniqueRightsl = target.getRights();
uniqueRightsl.addAll(mergedRights);
}
} else {
if (rightsShouldBeMergedAndSet == Boolean.FALSE) {
target.rights = null;
}
}
}
{
Boolean capabilitiesShouldBeMergedAndSet = strategy.shouldBeMergedAndSet(leftLocator, rightLocator, ((leftObject.capabilities!= null)&&(!leftObject.capabilities.isEmpty())), ((rightObject.capabilities!= null)&&(!rightObject.capabilities.isEmpty())));
if (capabilitiesShouldBeMergedAndSet == Boolean.TRUE) {
List lhsCapabilities;
lhsCapabilities = (((leftObject.capabilities!= null)&&(!leftObject.capabilities.isEmpty()))?leftObject.getCapabilities():null);
List rhsCapabilities;
rhsCapabilities = (((rightObject.capabilities!= null)&&(!rightObject.capabilities.isEmpty()))?rightObject.getCapabilities():null);
List mergedCapabilities = ((List ) strategy.merge(LocatorUtils.property(leftLocator, "capabilities", lhsCapabilities), LocatorUtils.property(rightLocator, "capabilities", rhsCapabilities), lhsCapabilities, rhsCapabilities, ((leftObject.capabilities!= null)&&(!leftObject.capabilities.isEmpty())), ((rightObject.capabilities!= null)&&(!rightObject.capabilities.isEmpty()))));
target.capabilities = null;
if (mergedCapabilities!= null) {
List uniqueCapabilitiesl = target.getCapabilities();
uniqueCapabilitiesl.addAll(mergedCapabilities);
}
} else {
if (capabilitiesShouldBeMergedAndSet == Boolean.FALSE) {
target.capabilities = null;
}
}
}
}
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Object createNewInstance() {
return new Service();
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Service clone() {
final Service _newObject;
_newObject = ((Service) super.clone());
if (this.rights == null) {
_newObject.rights = null;
} else {
_newObject.rights = new ArrayList<>();
for (Rights _item: this.rights) {
_newObject.rights.add(((_item == null)?null:_item.clone()));
}
}
if (this.capabilities == null) {
_newObject.capabilities = null;
} else {
_newObject.capabilities = new ArrayList<>();
for (Capability _item: this.capabilities) {
_newObject.capabilities.add(((_item == null)?null:_item.clone()));
}
}
return _newObject;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Service createCopy() {
final Service _newObject = ((Service) super.createCopy());
if (this.rights == null) {
_newObject.rights = null;
} else {
_newObject.rights = new ArrayList<>();
for (Rights _item: this.rights) {
_newObject.rights.add(((_item == null)?null:_item.createCopy()));
}
}
if (this.capabilities == null) {
_newObject.capabilities = null;
} else {
_newObject.capabilities = new ArrayList<>();
for (Capability _item: this.capabilities) {
_newObject.capabilities.add(((_item == null)?null:_item.createCopy()));
}
}
return _newObject;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Service createCopy(final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
final Service _newObject = ((Service) super.createCopy(_propertyTree, _propertyTreeUse));
final PropertyTree rightsPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("rights"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(rightsPropertyTree!= null):((rightsPropertyTree == null)||(!rightsPropertyTree.isLeaf())))) {
if (this.rights == null) {
_newObject.rights = null;
} else {
_newObject.rights = new ArrayList<>();
for (Rights _item: this.rights) {
_newObject.rights.add(((_item == null)?null:_item.createCopy(rightsPropertyTree, _propertyTreeUse)));
}
}
}
final PropertyTree capabilitiesPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("capabilities"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(capabilitiesPropertyTree!= null):((capabilitiesPropertyTree == null)||(!capabilitiesPropertyTree.isLeaf())))) {
if (this.capabilities == null) {
_newObject.capabilities = null;
} else {
_newObject.capabilities = new ArrayList<>();
for (Capability _item: this.capabilities) {
_newObject.capabilities.add(((_item == null)?null:_item.createCopy(capabilitiesPropertyTree, _propertyTreeUse)));
}
}
}
return _newObject;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Service copyExcept(final PropertyTree _propertyTree) {
return createCopy(_propertyTree, PropertyTreeUse.EXCLUDE);
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Service copyOnly(final PropertyTree _propertyTree) {
return createCopy(_propertyTree, PropertyTreeUse.INCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Service.Modifier modifier() {
if (null == this.__cachedModifier__) {
this.__cachedModifier__ = new Service.Modifier();
}
return ((Service.Modifier) this.__cachedModifier__);
}
/**
* Copies all state of this object to a builder. This method is used by the copyOf
* method and should not be called directly by client code.
*
* @param _other
* A builder instance to which the state of this object will be copied.
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public<_B >void copyTo(final Service.Builder<_B> _other) {
super.copyTo(_other);
if (this.rights == null) {
_other.rights = null;
} else {
_other.rights = new ArrayList<>();
for (Rights _item: this.rights) {
_other.rights.add(((_item == null)?null:_item.newCopyBuilder(_other)));
}
}
if (this.capabilities == null) {
_other.capabilities = null;
} else {
_other.capabilities = new ArrayList<>();
for (Capability _item: this.capabilities) {
_other.capabilities.add(((_item == null)?null:_item.newCopyBuilder(_other)));
}
}
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public<_B >Service.Builder<_B> newCopyBuilder(final _B _parentBuilder) {
return new Service.Builder<_B>(_parentBuilder, this, true);
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Service.Builder newCopyBuilder() {
return newCopyBuilder(null);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static Service.Builder builder() {
return new Service.Builder<>(null, null, false);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static<_B >Service.Builder<_B> copyOf(final Resource _other) {
final Service.Builder<_B> _newBuilder = new Service.Builder<>(null, null, false);
_other.copyTo(_newBuilder);
return _newBuilder;
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static<_B >Service.Builder<_B> copyOf(final Service _other) {
final Service.Builder<_B> _newBuilder = new Service.Builder<>(null, null, false);
_other.copyTo(_newBuilder);
return _newBuilder;
}
/**
* Copies all state of this object to a builder. This method is used by the copyOf
* method and should not be called directly by client code.
*
* @param _other
* A builder instance to which the state of this object will be copied.
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public<_B >void copyTo(final Service.Builder<_B> _other, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
super.copyTo(_other, _propertyTree, _propertyTreeUse);
final PropertyTree rightsPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("rights"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(rightsPropertyTree!= null):((rightsPropertyTree == null)||(!rightsPropertyTree.isLeaf())))) {
if (this.rights == null) {
_other.rights = null;
} else {
_other.rights = new ArrayList<>();
for (Rights _item: this.rights) {
_other.rights.add(((_item == null)?null:_item.newCopyBuilder(_other, rightsPropertyTree, _propertyTreeUse)));
}
}
}
final PropertyTree capabilitiesPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("capabilities"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(capabilitiesPropertyTree!= null):((capabilitiesPropertyTree == null)||(!capabilitiesPropertyTree.isLeaf())))) {
if (this.capabilities == null) {
_other.capabilities = null;
} else {
_other.capabilities = new ArrayList<>();
for (Capability _item: this.capabilities) {
_other.capabilities.add(((_item == null)?null:_item.newCopyBuilder(_other, capabilitiesPropertyTree, _propertyTreeUse)));
}
}
}
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public<_B >Service.Builder<_B> newCopyBuilder(final _B _parentBuilder, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
return new Service.Builder<_B>(_parentBuilder, this, true, _propertyTree, _propertyTreeUse);
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Service.Builder newCopyBuilder(final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
return newCopyBuilder(null, _propertyTree, _propertyTreeUse);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static<_B >Service.Builder<_B> copyOf(final Resource _other, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
final Service.Builder<_B> _newBuilder = new Service.Builder<>(null, null, false);
_other.copyTo(_newBuilder, _propertyTree, _propertyTreeUse);
return _newBuilder;
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static<_B >Service.Builder<_B> copyOf(final Service _other, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
final Service.Builder<_B> _newBuilder = new Service.Builder<>(null, null, false);
_other.copyTo(_newBuilder, _propertyTree, _propertyTreeUse);
return _newBuilder;
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static Service.Builder copyExcept(final Resource _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.EXCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static Service.Builder copyExcept(final Service _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.EXCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static Service.Builder copyOnly(final Resource _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.INCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static Service.Builder copyOnly(final Service _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.INCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Service visit(final PropertyVisitor _visitor_) {
super.visit(_visitor_);
if (_visitor_.visit(new CollectionProperty<>(Service.PropInfo.RIGHTS, this))&&(this.rights!= null)) {
for (Rights _item_: this.rights) {
if (_item_!= null) {
_item_.visit(_visitor_);
}
}
}
if (_visitor_.visit(new CollectionProperty<>(Service.PropInfo.CAPABILITIES, this))&&(this.capabilities!= null)) {
for (Capability _item_: this.capabilities) {
if (_item_!= null) {
_item_.visit(_visitor_);
}
}
}
return this;
}
public static class Builder<_B >
extends Resource.Builder<_B>
implements Buildable
{
private List>> rights;
private List>> capabilities;
public Builder(final _B _parentBuilder, final Service _other, final boolean _copy) {
super(_parentBuilder, _other, _copy);
if (_other!= null) {
if (_other.rights == null) {
this.rights = null;
} else {
this.rights = new ArrayList<>();
for (Rights _item: _other.rights) {
this.rights.add(((_item == null)?null:_item.newCopyBuilder(this)));
}
}
if (_other.capabilities == null) {
this.capabilities = null;
} else {
this.capabilities = new ArrayList<>();
for (Capability _item: _other.capabilities) {
this.capabilities.add(((_item == null)?null:_item.newCopyBuilder(this)));
}
}
}
}
public Builder(final _B _parentBuilder, final Service _other, final boolean _copy, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
super(_parentBuilder, _other, _copy, _propertyTree, _propertyTreeUse);
if (_other!= null) {
final PropertyTree rightsPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("rights"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(rightsPropertyTree!= null):((rightsPropertyTree == null)||(!rightsPropertyTree.isLeaf())))) {
if (_other.rights == null) {
this.rights = null;
} else {
this.rights = new ArrayList<>();
for (Rights _item: _other.rights) {
this.rights.add(((_item == null)?null:_item.newCopyBuilder(this, rightsPropertyTree, _propertyTreeUse)));
}
}
}
final PropertyTree capabilitiesPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("capabilities"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(capabilitiesPropertyTree!= null):((capabilitiesPropertyTree == null)||(!capabilitiesPropertyTree.isLeaf())))) {
if (_other.capabilities == null) {
this.capabilities = null;
} else {
this.capabilities = new ArrayList<>();
for (Capability _item: _other.capabilities) {
this.capabilities.add(((_item == null)?null:_item.newCopyBuilder(this, capabilitiesPropertyTree, _propertyTreeUse)));
}
}
}
}
}
protected<_P extends Service >_P init(final _P _product) {
if (this.rights!= null) {
final List rights = new ArrayList<>(this.rights.size());
for (Rights.Builder> _item: this.rights) {
rights.add(_item.build());
}
_product.rights = rights;
}
if (this.capabilities!= null) {
final List capabilities = new ArrayList<>(this.capabilities.size());
for (Capability.Builder> _item: this.capabilities) {
capabilities.add(_item.build());
}
_product.capabilities = capabilities;
}
return super.init(_product);
}
/**
* Adds the given items to the value of "rights"
*
* @param rights
* Items to add to the value of the "rights" property
*/
public Service.Builder<_B> addRights(final Iterable extends Rights> rights) {
if (rights!= null) {
if (this.rights == null) {
this.rights = new ArrayList<>();
}
for (Rights _item: rights) {
this.rights.add(new Rights.Builder<>(this, _item, false));
}
}
return this;
}
/**
* Sets the new value of "rights" (any previous value will be replaced)
*
* @param rights
* New value of the "rights" property.
*/
public Service.Builder<_B> withRights(final Iterable extends Rights> rights) {
if (this.rights!= null) {
this.rights.clear();
}
return addRights(rights);
}
/**
* Adds the given items to the value of "rights"
*
* @param rights
* Items to add to the value of the "rights" property
*/
public Service.Builder<_B> addRights(Rights... rights) {
addRights(Arrays.asList(rights));
return this;
}
/**
* Sets the new value of "rights" (any previous value will be replaced)
*
* @param rights
* New value of the "rights" property.
*/
public Service.Builder<_B> withRights(Rights... rights) {
withRights(Arrays.asList(rights));
return this;
}
/**
* Returns a new builder to build an additional value of the "Rights" property.
* Use {@link org.javastro.ivoa.entities.resource.Rights.Builder#end()} to return
* to the current builder.
*
* @return
* a new builder to build an additional value of the "Rights" property.
* Use {@link org.javastro.ivoa.entities.resource.Rights.Builder#end()} to return
* to the current builder.
*/
public Rights.Builder extends Service.Builder<_B>> addRights() {
if (this.rights == null) {
this.rights = new ArrayList<>();
}
final Rights.Builder> rights_Builder = new Rights.Builder<>(this, null, false);
this.rights.add(rights_Builder);
return rights_Builder;
}
/**
* Adds the given items to the value of "capabilities"
*
* @param capabilities
* Items to add to the value of the "capabilities" property
*/
public Service.Builder<_B> addCapabilities(final Iterable extends Capability> capabilities) {
if (capabilities!= null) {
if (this.capabilities == null) {
this.capabilities = new ArrayList<>();
}
for (Capability _item: capabilities) {
this.capabilities.add(new Capability.Builder<>(this, _item, false));
}
}
return this;
}
/**
* Sets the new value of "capabilities" (any previous value will be replaced)
*
* @param capabilities
* New value of the "capabilities" property.
*/
public Service.Builder<_B> withCapabilities(final Iterable extends Capability> capabilities) {
if (this.capabilities!= null) {
this.capabilities.clear();
}
return addCapabilities(capabilities);
}
/**
* Adds the given items to the value of "capabilities"
*
* @param capabilities
* Items to add to the value of the "capabilities" property
*/
public Service.Builder<_B> addCapabilities(Capability... capabilities) {
addCapabilities(Arrays.asList(capabilities));
return this;
}
/**
* Sets the new value of "capabilities" (any previous value will be replaced)
*
* @param capabilities
* New value of the "capabilities" property.
*/
public Service.Builder<_B> withCapabilities(Capability... capabilities) {
withCapabilities(Arrays.asList(capabilities));
return this;
}
/**
* Returns a new builder to build an additional value of the "Capabilities"
* property.
* Use {@link org.javastro.ivoa.entities.resource.Capability.Builder#end()} to
* return to the current builder.
*
* @return
* a new builder to build an additional value of the "Capabilities" property.
* Use {@link org.javastro.ivoa.entities.resource.Capability.Builder#end()} to
* return to the current builder.
*/
public Capability.Builder extends Service.Builder<_B>> addCapabilities() {
if (this.capabilities == null) {
this.capabilities = new ArrayList<>();
}
final Capability.Builder> capabilities_Builder = new Capability.Builder<>(this, null, false);
this.capabilities.add(capabilities_Builder);
return capabilities_Builder;
}
/**
* Adds the given items to the value of "validationLevels"
*
* @param validationLevels
* Items to add to the value of the "validationLevels" property
*/
@Override
public Service.Builder<_B> addValidationLevels(final Iterable extends Validation> validationLevels) {
super.addValidationLevels(validationLevels);
return this;
}
/**
* Adds the given items to the value of "validationLevels"
*
* @param validationLevels
* Items to add to the value of the "validationLevels" property
*/
@Override
public Service.Builder<_B> addValidationLevels(Validation... validationLevels) {
super.addValidationLevels(validationLevels);
return this;
}
/**
* Sets the new value of "validationLevels" (any previous value will be replaced)
*
* @param validationLevels
* New value of the "validationLevels" property.
*/
@Override
public Service.Builder<_B> withValidationLevels(final Iterable extends Validation> validationLevels) {
super.withValidationLevels(validationLevels);
return this;
}
/**
* Sets the new value of "validationLevels" (any previous value will be replaced)
*
* @param validationLevels
* New value of the "validationLevels" property.
*/
@Override
public Service.Builder<_B> withValidationLevels(Validation... validationLevels) {
super.withValidationLevels(validationLevels);
return this;
}
/**
* Sets the new value of "title" (any previous value will be replaced)
*
* @param title
* New value of the "title" property.
*/
@Override
public Service.Builder<_B> withTitle(final String title) {
super.withTitle(title);
return this;
}
/**
* Sets the new value of "shortName" (any previous value will be replaced)
*
* @param shortName
* New value of the "shortName" property.
*/
@Override
public Service.Builder<_B> withShortName(final String shortName) {
super.withShortName(shortName);
return this;
}
/**
* Sets the new value of "identifier" (any previous value will be replaced)
*
* @param identifier
* New value of the "identifier" property.
*/
@Override
public Service.Builder<_B> withIdentifier(final String identifier) {
super.withIdentifier(identifier);
return this;
}
/**
* Adds the given items to the value of "altIdentifiers"
*
* @param altIdentifiers
* Items to add to the value of the "altIdentifiers" property
*/
@Override
public Service.Builder<_B> addAltIdentifiers(final Iterable extends String> altIdentifiers) {
super.addAltIdentifiers(altIdentifiers);
return this;
}
/**
* Adds the given items to the value of "altIdentifiers"
*
* @param altIdentifiers
* Items to add to the value of the "altIdentifiers" property
*/
@Override
public Service.Builder<_B> addAltIdentifiers(String... altIdentifiers) {
super.addAltIdentifiers(altIdentifiers);
return this;
}
/**
* Sets the new value of "altIdentifiers" (any previous value will be replaced)
*
* @param altIdentifiers
* New value of the "altIdentifiers" property.
*/
@Override
public Service.Builder<_B> withAltIdentifiers(final Iterable extends String> altIdentifiers) {
super.withAltIdentifiers(altIdentifiers);
return this;
}
/**
* Sets the new value of "altIdentifiers" (any previous value will be replaced)
*
* @param altIdentifiers
* New value of the "altIdentifiers" property.
*/
@Override
public Service.Builder<_B> withAltIdentifiers(String... altIdentifiers) {
super.withAltIdentifiers(altIdentifiers);
return this;
}
/**
* Sets the new value of "curation" (any previous value will be replaced)
*
* @param curation
* New value of the "curation" property.
*/
@Override
public Service.Builder<_B> withCuration(final Curation curation) {
super.withCuration(curation);
return this;
}
/**
* Returns the existing builder or a new builder to build the value of the
* "curation" property.
* Use {@link org.javastro.ivoa.entities.resource.Curation.Builder#end()} to return
* to the current builder.
*
* @return
* A new builder to build the value of the "curation" property.
* Use {@link org.javastro.ivoa.entities.resource.Curation.Builder#end()} to return
* to the current builder.
*/
public Curation.Builder extends Service.Builder<_B>> withCuration() {
return ((Curation.Builder extends Service.Builder<_B>> ) super.withCuration());
}
/**
* Sets the new value of "content" (any previous value will be replaced)
*
* @param content
* New value of the "content" property.
*/
@Override
public Service.Builder<_B> withContent(final Content content) {
super.withContent(content);
return this;
}
/**
* Returns the existing builder or a new builder to build the value of the
* "content" property.
* Use {@link org.javastro.ivoa.entities.resource.Content.Builder#end()} to return
* to the current builder.
*
* @return
* A new builder to build the value of the "content" property.
* Use {@link org.javastro.ivoa.entities.resource.Content.Builder#end()} to return
* to the current builder.
*/
public Content.Builder extends Service.Builder<_B>> withContent() {
return ((Content.Builder extends Service.Builder<_B>> ) super.withContent());
}
/**
* Sets the new value of "created" (any previous value will be replaced)
*
* @param created
* New value of the "created" property.
*/
@Override
public Service.Builder<_B> withCreated(final LocalDateTime created) {
super.withCreated(created);
return this;
}
/**
* Sets the new value of "updated" (any previous value will be replaced)
*
* @param updated
* New value of the "updated" property.
*/
@Override
public Service.Builder<_B> withUpdated(final LocalDateTime updated) {
super.withUpdated(updated);
return this;
}
/**
* Sets the new value of "status" (any previous value will be replaced)
*
* @param status
* New value of the "status" property.
*/
@Override
public Service.Builder<_B> withStatus(final String status) {
super.withStatus(status);
return this;
}
/**
* Sets the new value of "version" (any previous value will be replaced)
*
* @param version
* New value of the "version" property.
*/
@Override
public Service.Builder<_B> withVersion(final String version) {
super.withVersion(version);
return this;
}
@Override
public Service build() {
if (_storedValue == null) {
return this.init(new Service());
} else {
return ((Service) _storedValue);
}
}
public Service.Builder<_B> copyOf(final Service _other) {
_other.copyTo(this);
return this;
}
public Service.Builder<_B> copyOf(final Service.Builder _other) {
return copyOf(_other.build());
}
}
public class Modifier
extends Resource.Modifier
{
public List getRights() {
if (Service.this.rights == null) {
Service.this.rights = new ArrayList<>();
}
return Service.this.rights;
}
public List getCapabilities() {
if (Service.this.capabilities == null) {
Service.this.capabilities = new ArrayList<>();
}
return Service.this.capabilities;
}
}
public static class PropInfo {
public static final transient CollectionPropertyInfo RIGHTS = new CollectionPropertyInfo("rights", Service.class, Rights.class, true, null, new QName("", "rights"), new QName("http://www.ivoa.net/xml/VOResource/v1.0", "Rights"), false) {
@Override
public List get(final Service _instance_) {
return ((_instance_ == null)?null:_instance_.rights);
}
@Override
public void set(final Service _instance_, final List _value_) {
if (_instance_!= null) {
_instance_.rights = _value_;
}
}
}
;
public static final transient CollectionPropertyInfo CAPABILITIES = new CollectionPropertyInfo("capabilities", Service.class, Capability.class, true, null, new QName("", "capability"), new QName("http://www.ivoa.net/xml/VOResource/v1.0", "Capability"), false) {
@Override
public List get(final Service _instance_) {
return ((_instance_ == null)?null:_instance_.capabilities);
}
@Override
public void set(final Service _instance_, final List _value_) {
if (_instance_!= null) {
_instance_.capabilities = _value_;
}
}
}
;
}
public static class Select
extends Service.Selector
{
Select() {
super(null, null, null);
}
public static Service.Select _root() {
return new Service.Select();
}
}
public static class Selector , TParent >
extends Resource.Selector
{
private Rights.Selector> rights = null;
private Capability.Selector> capabilities = null;
public Selector(final TRoot root, final TParent parent, final String propertyName) {
super(root, parent, propertyName);
}
@Override
public Map buildChildren() {
final Map products = new HashMap<>();
products.putAll(super.buildChildren());
if (this.rights!= null) {
products.put("rights", this.rights.init());
}
if (this.capabilities!= null) {
products.put("capabilities", this.capabilities.init());
}
return products;
}
public Rights.Selector> rights() {
return ((this.rights == null)?this.rights = new Rights.Selector<>(this._root, this, "rights"):this.rights);
}
public Capability.Selector> capabilities() {
return ((this.capabilities == null)?this.capabilities = new Capability.Selector<>(this._root, this, "capabilities"):this.capabilities);
}
}
}