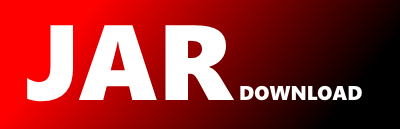
org.javastro.ivoa.entities.resource.ServiceInterface Maven / Gradle / Ivy
package org.javastro.ivoa.entities.resource;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import javax.xml.namespace.QName;
import com.kscs.util.jaxb.Buildable;
import com.kscs.util.jaxb.CollectionProperty;
import com.kscs.util.jaxb.CollectionPropertyInfo;
import com.kscs.util.jaxb.CollectionPropertyInfo;
import com.kscs.util.jaxb.CollectionPropertyInfo;
import com.kscs.util.jaxb.Copyable;
import com.kscs.util.jaxb.PartialCopyable;
import com.kscs.util.jaxb.PropertyTree;
import com.kscs.util.jaxb.PropertyTreeUse;
import com.kscs.util.jaxb.PropertyVisitor;
import com.kscs.util.jaxb.SingleProperty;
import com.kscs.util.jaxb.SinglePropertyInfo;
import com.kscs.util.jaxb.SinglePropertyInfo;
import com.kscs.util.jaxb.SinglePropertyInfo;
import jakarta.annotation.Generated;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlAttribute;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlSchemaType;
import jakarta.xml.bind.annotation.XmlSeeAlso;
import jakarta.xml.bind.annotation.XmlType;
import jakarta.xml.bind.annotation.adapters.CollapsedStringAdapter;
import jakarta.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import org.javastro.ivoa.entities.resource.dataservice.ParamHTTP;
import org.javastro.ivoa.entities.resource.registry.OAIHTTP;
import org.jvnet.jaxb.lang.JAXBMergeStrategy;
import org.jvnet.jaxb.lang.JAXBToStringStrategy;
import org.jvnet.jaxb.lang.MergeFrom;
import org.jvnet.jaxb.lang.MergeStrategy;
import org.jvnet.jaxb.lang.ToString;
import org.jvnet.jaxb.lang.ToStringStrategy;
import org.jvnet.jaxb.locator.ObjectLocator;
import org.jvnet.jaxb.locator.util.LocatorUtils;
/**
* Additional interface subtypes (beyond WebService and WebBrowser) are
* defined in the VODataService schema.
*
* Java class for Interface complex type
.
*
* The following schema fragment specifies the expected content contained within this class.
*
* {@code
*
*
*
*
*
*
*
*
*
*
*
*
*
*
* }
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "Interface", propOrder = {
"accessURLs",
"mirrorURLs",
"securityMethod",
"testQueryString"
})
@XmlSeeAlso({
WebBrowser.class,
ParamHTTP.class,
OAIHTTP.class,
WebService.class
})
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public abstract class ServiceInterface implements Cloneable, Copyable, PartialCopyable, MergeFrom, ToString
{
/**
* Although the schema allows multiple occurrences of
* accessURL, multiple accessURLs are deprecated. Each
* interface should have exactly one access URL. Where an
* interface has several mirrors, the accessURL should
* reflect the “primary” (fastest, best-connected,
* best-maintained) site, the one that non-sophisticated
* clients will go to.
*
* Additional accessURLs should be put into mirrorURLs.
* Advanced clients can retrieve the mirrorURLs and
* empirically determine interfaces closer to their
* network location.
*
*/
@XmlElement(name = "accessURL", required = true)
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
protected List accessURLs;
/**
* This is intended exclusively for true mirrors, i.e.,
* interfaces that are functionally identical to the
* original interface and that are operated by the same
* publisher. Other arrangements should be represented as
* separate services linked by mirror-of relationships.
*
*/
@XmlElement(name = "mirrorURL")
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
protected List mirrorURLs;
/**
* Services not requiring authentication must provide
* at least one interface definition without a
* securityMethod defined.
*
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
protected SecurityMethod securityMethod;
/**
* This contains data that can be passed to the interface to
* retrieve a non-empty result. This can be used by validators
* within test suites.
*
* Exactly how agents should use the data contained in
* the testQueryString depends on the concrete interface class.
* For interfaces employing the HTTP GET method, however,
* this will typically be urlencoded parameters (as for
* the application/x-www-form-urlencoded media type).
*
*/
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
@XmlSchemaType(name = "token")
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
protected String testQueryString;
/**
* The version of a standard interface specification that this
* interface complies with. Most VO standards indicate the
* version in the standardID attribute of the capability. For
* these standards, the version attribute should not be used.
*
*/
@XmlAttribute(name = "version")
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
protected String version;
/**
* For an interface complying with some registered
* standard (i.e. has a legal standardID), the role can be
* matched against interface roles enumerated in standard
* resource record. The interface descriptions in
* the standard record can provide default descriptions
* so that such details need not be repeated here.
*
*/
@XmlAttribute(name = "role")
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
@XmlSchemaType(name = "NMTOKEN")
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
protected String role;
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
protected transient ServiceInterface.Modifier __cachedModifier__;
/**
* Default no-arg constructor
*
*/
public ServiceInterface() {
super();
}
/**
* Fully-initialising value constructor
*
*/
public ServiceInterface(final List accessURLs, final List mirrorURLs, final SecurityMethod securityMethod, final String testQueryString, final String version, final String role) {
this.accessURLs = accessURLs;
this.mirrorURLs = mirrorURLs;
this.securityMethod = securityMethod;
this.testQueryString = testQueryString;
this.version = version;
this.role = role;
}
/**
* Although the schema allows multiple occurrences of
* accessURL, multiple accessURLs are deprecated. Each
* interface should have exactly one access URL. Where an
* interface has several mirrors, the accessURL should
* reflect the “primary” (fastest, best-connected,
* best-maintained) site, the one that non-sophisticated
* clients will go to.
*
* Additional accessURLs should be put into mirrorURLs.
* Advanced clients can retrieve the mirrorURLs and
* empirically determine interfaces closer to their
* network location.
*
* Gets the value of the accessURLs property.
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the accessURLs property.
*
*
* For example, to add a new item, do as follows:
*
*
* getAccessURLs().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link AccessURL }
*
*
*
* @return
* The value of the accessURLs property.
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public List getAccessURLs() {
if (accessURLs == null) {
accessURLs = new ArrayList<>();
}
return this.accessURLs;
}
/**
* This is intended exclusively for true mirrors, i.e.,
* interfaces that are functionally identical to the
* original interface and that are operated by the same
* publisher. Other arrangements should be represented as
* separate services linked by mirror-of relationships.
*
* Gets the value of the mirrorURLs property.
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the mirrorURLs property.
*
*
* For example, to add a new item, do as follows:
*
*
* getMirrorURLs().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link MirrorURL }
*
*
*
* @return
* The value of the mirrorURLs property.
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public List getMirrorURLs() {
if (mirrorURLs == null) {
mirrorURLs = new ArrayList<>();
}
return this.mirrorURLs;
}
/**
* Services not requiring authentication must provide
* at least one interface definition without a
* securityMethod defined.
*
* @return
* possible object is
* {@link SecurityMethod }
*
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public SecurityMethod getSecurityMethod() {
return securityMethod;
}
/**
* Sets the value of the securityMethod property.
*
* @param value
* allowed object is
* {@link SecurityMethod }
*
* @see #getSecurityMethod()
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void setSecurityMethod(SecurityMethod value) {
this.securityMethod = value;
}
/**
* This contains data that can be passed to the interface to
* retrieve a non-empty result. This can be used by validators
* within test suites.
*
* Exactly how agents should use the data contained in
* the testQueryString depends on the concrete interface class.
* For interfaces employing the HTTP GET method, however,
* this will typically be urlencoded parameters (as for
* the application/x-www-form-urlencoded media type).
*
* @return
* possible object is
* {@link String }
*
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public String getTestQueryString() {
return testQueryString;
}
/**
* Sets the value of the testQueryString property.
*
* @param value
* allowed object is
* {@link String }
*
* @see #getTestQueryString()
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void setTestQueryString(String value) {
this.testQueryString = value;
}
/**
* The version of a standard interface specification that this
* interface complies with. Most VO standards indicate the
* version in the standardID attribute of the capability. For
* these standards, the version attribute should not be used.
*
* @return
* possible object is
* {@link String }
*
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public String getVersion() {
return version;
}
/**
* Sets the value of the version property.
*
* @param value
* allowed object is
* {@link String }
*
* @see #getVersion()
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void setVersion(String value) {
this.version = value;
}
/**
* For an interface complying with some registered
* standard (i.e. has a legal standardID), the role can be
* matched against interface roles enumerated in standard
* resource record. The interface descriptions in
* the standard record can provide default descriptions
* so that such details need not be repeated here.
*
* @return
* possible object is
* {@link String }
*
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public String getRole() {
return role;
}
/**
* Sets the value of the role property.
*
* @param value
* allowed object is
* {@link String }
*
* @see #getRole()
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void setRole(String value) {
this.role = value;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public boolean equals(Object object) {
if ((object == null)||(this.getClass()!= object.getClass())) {
return false;
}
if (this == object) {
return true;
}
final ServiceInterface that = ((ServiceInterface) object);
{
List leftAccessURLs;
leftAccessURLs = (((this.accessURLs!= null)&&(!this.accessURLs.isEmpty()))?this.getAccessURLs():null);
List rightAccessURLs;
rightAccessURLs = (((that.accessURLs!= null)&&(!that.accessURLs.isEmpty()))?that.getAccessURLs():null);
if ((this.accessURLs!= null)&&(!this.accessURLs.isEmpty())) {
if ((that.accessURLs!= null)&&(!that.accessURLs.isEmpty())) {
if (!leftAccessURLs.equals(rightAccessURLs)) {
return false;
}
} else {
return false;
}
} else {
if ((that.accessURLs!= null)&&(!that.accessURLs.isEmpty())) {
return false;
}
}
}
{
List leftMirrorURLs;
leftMirrorURLs = (((this.mirrorURLs!= null)&&(!this.mirrorURLs.isEmpty()))?this.getMirrorURLs():null);
List rightMirrorURLs;
rightMirrorURLs = (((that.mirrorURLs!= null)&&(!that.mirrorURLs.isEmpty()))?that.getMirrorURLs():null);
if ((this.mirrorURLs!= null)&&(!this.mirrorURLs.isEmpty())) {
if ((that.mirrorURLs!= null)&&(!that.mirrorURLs.isEmpty())) {
if (!leftMirrorURLs.equals(rightMirrorURLs)) {
return false;
}
} else {
return false;
}
} else {
if ((that.mirrorURLs!= null)&&(!that.mirrorURLs.isEmpty())) {
return false;
}
}
}
{
SecurityMethod leftSecurityMethod;
leftSecurityMethod = this.getSecurityMethod();
SecurityMethod rightSecurityMethod;
rightSecurityMethod = that.getSecurityMethod();
if (this.securityMethod!= null) {
if (that.securityMethod!= null) {
if (!leftSecurityMethod.equals(rightSecurityMethod)) {
return false;
}
} else {
return false;
}
} else {
if (that.securityMethod!= null) {
return false;
}
}
}
{
String leftTestQueryString;
leftTestQueryString = this.getTestQueryString();
String rightTestQueryString;
rightTestQueryString = that.getTestQueryString();
if (this.testQueryString!= null) {
if (that.testQueryString!= null) {
if (!leftTestQueryString.equals(rightTestQueryString)) {
return false;
}
} else {
return false;
}
} else {
if (that.testQueryString!= null) {
return false;
}
}
}
{
String leftVersion;
leftVersion = this.getVersion();
String rightVersion;
rightVersion = that.getVersion();
if (this.version!= null) {
if (that.version!= null) {
if (!leftVersion.equals(rightVersion)) {
return false;
}
} else {
return false;
}
} else {
if (that.version!= null) {
return false;
}
}
}
{
String leftRole;
leftRole = this.getRole();
String rightRole;
rightRole = that.getRole();
if (this.role!= null) {
if (that.role!= null) {
if (!leftRole.equals(rightRole)) {
return false;
}
} else {
return false;
}
} else {
if (that.role!= null) {
return false;
}
}
}
return true;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public int hashCode() {
int currentHashCode = 1;
{
currentHashCode = (currentHashCode* 31);
List theAccessURLs;
theAccessURLs = (((this.accessURLs!= null)&&(!this.accessURLs.isEmpty()))?this.getAccessURLs():null);
if ((this.accessURLs!= null)&&(!this.accessURLs.isEmpty())) {
currentHashCode += theAccessURLs.hashCode();
}
}
{
currentHashCode = (currentHashCode* 31);
List theMirrorURLs;
theMirrorURLs = (((this.mirrorURLs!= null)&&(!this.mirrorURLs.isEmpty()))?this.getMirrorURLs():null);
if ((this.mirrorURLs!= null)&&(!this.mirrorURLs.isEmpty())) {
currentHashCode += theMirrorURLs.hashCode();
}
}
{
currentHashCode = (currentHashCode* 31);
SecurityMethod theSecurityMethod;
theSecurityMethod = this.getSecurityMethod();
if (this.securityMethod!= null) {
currentHashCode += theSecurityMethod.hashCode();
}
}
{
currentHashCode = (currentHashCode* 31);
String theTestQueryString;
theTestQueryString = this.getTestQueryString();
if (this.testQueryString!= null) {
currentHashCode += theTestQueryString.hashCode();
}
}
{
currentHashCode = (currentHashCode* 31);
String theVersion;
theVersion = this.getVersion();
if (this.version!= null) {
currentHashCode += theVersion.hashCode();
}
}
{
currentHashCode = (currentHashCode* 31);
String theRole;
theRole = this.getRole();
if (this.role!= null) {
currentHashCode += theRole.hashCode();
}
}
return currentHashCode;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public String toString() {
final ToStringStrategy strategy = JAXBToStringStrategy.getInstance();
final StringBuilder buffer = new StringBuilder();
append(null, buffer, strategy);
return buffer.toString();
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public StringBuilder append(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
strategy.appendStart(locator, this, buffer);
appendFields(locator, buffer, strategy);
strategy.appendEnd(locator, this, buffer);
return buffer;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public StringBuilder appendFields(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
{
List theAccessURLs;
theAccessURLs = (((this.accessURLs!= null)&&(!this.accessURLs.isEmpty()))?this.getAccessURLs():null);
strategy.appendField(locator, this, "accessURLs", buffer, theAccessURLs, ((this.accessURLs!= null)&&(!this.accessURLs.isEmpty())));
}
{
List theMirrorURLs;
theMirrorURLs = (((this.mirrorURLs!= null)&&(!this.mirrorURLs.isEmpty()))?this.getMirrorURLs():null);
strategy.appendField(locator, this, "mirrorURLs", buffer, theMirrorURLs, ((this.mirrorURLs!= null)&&(!this.mirrorURLs.isEmpty())));
}
{
SecurityMethod theSecurityMethod;
theSecurityMethod = this.getSecurityMethod();
strategy.appendField(locator, this, "securityMethod", buffer, theSecurityMethod, (this.securityMethod!= null));
}
{
String theTestQueryString;
theTestQueryString = this.getTestQueryString();
strategy.appendField(locator, this, "testQueryString", buffer, theTestQueryString, (this.testQueryString!= null));
}
{
String theVersion;
theVersion = this.getVersion();
strategy.appendField(locator, this, "version", buffer, theVersion, (this.version!= null));
}
{
String theRole;
theRole = this.getRole();
strategy.appendField(locator, this, "role", buffer, theRole, (this.role!= null));
}
return buffer;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void mergeFrom(Object left, Object right) {
final MergeStrategy strategy = JAXBMergeStrategy.getInstance();
mergeFrom(null, null, left, right, strategy);
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void mergeFrom(ObjectLocator leftLocator, ObjectLocator rightLocator, Object left, Object right, MergeStrategy strategy) {
if (right instanceof ServiceInterface) {
final ServiceInterface target = this;
final ServiceInterface leftObject = ((ServiceInterface) left);
final ServiceInterface rightObject = ((ServiceInterface) right);
{
Boolean accessURLsShouldBeMergedAndSet = strategy.shouldBeMergedAndSet(leftLocator, rightLocator, ((leftObject.accessURLs!= null)&&(!leftObject.accessURLs.isEmpty())), ((rightObject.accessURLs!= null)&&(!rightObject.accessURLs.isEmpty())));
if (accessURLsShouldBeMergedAndSet == Boolean.TRUE) {
List lhsAccessURLs;
lhsAccessURLs = (((leftObject.accessURLs!= null)&&(!leftObject.accessURLs.isEmpty()))?leftObject.getAccessURLs():null);
List rhsAccessURLs;
rhsAccessURLs = (((rightObject.accessURLs!= null)&&(!rightObject.accessURLs.isEmpty()))?rightObject.getAccessURLs():null);
List mergedAccessURLs = ((List ) strategy.merge(LocatorUtils.property(leftLocator, "accessURLs", lhsAccessURLs), LocatorUtils.property(rightLocator, "accessURLs", rhsAccessURLs), lhsAccessURLs, rhsAccessURLs, ((leftObject.accessURLs!= null)&&(!leftObject.accessURLs.isEmpty())), ((rightObject.accessURLs!= null)&&(!rightObject.accessURLs.isEmpty()))));
target.accessURLs = null;
if (mergedAccessURLs!= null) {
List uniqueAccessURLsl = target.getAccessURLs();
uniqueAccessURLsl.addAll(mergedAccessURLs);
}
} else {
if (accessURLsShouldBeMergedAndSet == Boolean.FALSE) {
target.accessURLs = null;
}
}
}
{
Boolean mirrorURLsShouldBeMergedAndSet = strategy.shouldBeMergedAndSet(leftLocator, rightLocator, ((leftObject.mirrorURLs!= null)&&(!leftObject.mirrorURLs.isEmpty())), ((rightObject.mirrorURLs!= null)&&(!rightObject.mirrorURLs.isEmpty())));
if (mirrorURLsShouldBeMergedAndSet == Boolean.TRUE) {
List lhsMirrorURLs;
lhsMirrorURLs = (((leftObject.mirrorURLs!= null)&&(!leftObject.mirrorURLs.isEmpty()))?leftObject.getMirrorURLs():null);
List rhsMirrorURLs;
rhsMirrorURLs = (((rightObject.mirrorURLs!= null)&&(!rightObject.mirrorURLs.isEmpty()))?rightObject.getMirrorURLs():null);
List mergedMirrorURLs = ((List ) strategy.merge(LocatorUtils.property(leftLocator, "mirrorURLs", lhsMirrorURLs), LocatorUtils.property(rightLocator, "mirrorURLs", rhsMirrorURLs), lhsMirrorURLs, rhsMirrorURLs, ((leftObject.mirrorURLs!= null)&&(!leftObject.mirrorURLs.isEmpty())), ((rightObject.mirrorURLs!= null)&&(!rightObject.mirrorURLs.isEmpty()))));
target.mirrorURLs = null;
if (mergedMirrorURLs!= null) {
List uniqueMirrorURLsl = target.getMirrorURLs();
uniqueMirrorURLsl.addAll(mergedMirrorURLs);
}
} else {
if (mirrorURLsShouldBeMergedAndSet == Boolean.FALSE) {
target.mirrorURLs = null;
}
}
}
{
Boolean securityMethodShouldBeMergedAndSet = strategy.shouldBeMergedAndSet(leftLocator, rightLocator, (leftObject.securityMethod!= null), (rightObject.securityMethod!= null));
if (securityMethodShouldBeMergedAndSet == Boolean.TRUE) {
SecurityMethod lhsSecurityMethod;
lhsSecurityMethod = leftObject.getSecurityMethod();
SecurityMethod rhsSecurityMethod;
rhsSecurityMethod = rightObject.getSecurityMethod();
SecurityMethod mergedSecurityMethod = ((SecurityMethod) strategy.merge(LocatorUtils.property(leftLocator, "securityMethod", lhsSecurityMethod), LocatorUtils.property(rightLocator, "securityMethod", rhsSecurityMethod), lhsSecurityMethod, rhsSecurityMethod, (leftObject.securityMethod!= null), (rightObject.securityMethod!= null)));
target.setSecurityMethod(mergedSecurityMethod);
} else {
if (securityMethodShouldBeMergedAndSet == Boolean.FALSE) {
target.securityMethod = null;
}
}
}
{
Boolean testQueryStringShouldBeMergedAndSet = strategy.shouldBeMergedAndSet(leftLocator, rightLocator, (leftObject.testQueryString!= null), (rightObject.testQueryString!= null));
if (testQueryStringShouldBeMergedAndSet == Boolean.TRUE) {
String lhsTestQueryString;
lhsTestQueryString = leftObject.getTestQueryString();
String rhsTestQueryString;
rhsTestQueryString = rightObject.getTestQueryString();
String mergedTestQueryString = ((String) strategy.merge(LocatorUtils.property(leftLocator, "testQueryString", lhsTestQueryString), LocatorUtils.property(rightLocator, "testQueryString", rhsTestQueryString), lhsTestQueryString, rhsTestQueryString, (leftObject.testQueryString!= null), (rightObject.testQueryString!= null)));
target.setTestQueryString(mergedTestQueryString);
} else {
if (testQueryStringShouldBeMergedAndSet == Boolean.FALSE) {
target.testQueryString = null;
}
}
}
{
Boolean versionShouldBeMergedAndSet = strategy.shouldBeMergedAndSet(leftLocator, rightLocator, (leftObject.version!= null), (rightObject.version!= null));
if (versionShouldBeMergedAndSet == Boolean.TRUE) {
String lhsVersion;
lhsVersion = leftObject.getVersion();
String rhsVersion;
rhsVersion = rightObject.getVersion();
String mergedVersion = ((String) strategy.merge(LocatorUtils.property(leftLocator, "version", lhsVersion), LocatorUtils.property(rightLocator, "version", rhsVersion), lhsVersion, rhsVersion, (leftObject.version!= null), (rightObject.version!= null)));
target.setVersion(mergedVersion);
} else {
if (versionShouldBeMergedAndSet == Boolean.FALSE) {
target.version = null;
}
}
}
{
Boolean roleShouldBeMergedAndSet = strategy.shouldBeMergedAndSet(leftLocator, rightLocator, (leftObject.role!= null), (rightObject.role!= null));
if (roleShouldBeMergedAndSet == Boolean.TRUE) {
String lhsRole;
lhsRole = leftObject.getRole();
String rhsRole;
rhsRole = rightObject.getRole();
String mergedRole = ((String) strategy.merge(LocatorUtils.property(leftLocator, "role", lhsRole), LocatorUtils.property(rightLocator, "role", rhsRole), lhsRole, rhsRole, (leftObject.role!= null), (rightObject.role!= null)));
target.setRole(mergedRole);
} else {
if (roleShouldBeMergedAndSet == Boolean.FALSE) {
target.role = null;
}
}
}
}
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public ServiceInterface clone() {
final ServiceInterface _newObject;
try {
_newObject = ((ServiceInterface) super.clone());
} catch (CloneNotSupportedException e) {
throw new RuntimeException(e);
}
if (this.accessURLs == null) {
_newObject.accessURLs = null;
} else {
_newObject.accessURLs = new ArrayList<>();
for (AccessURL _item: this.accessURLs) {
_newObject.accessURLs.add(((_item == null)?null:_item.clone()));
}
}
if (this.mirrorURLs == null) {
_newObject.mirrorURLs = null;
} else {
_newObject.mirrorURLs = new ArrayList<>();
for (MirrorURL _item: this.mirrorURLs) {
_newObject.mirrorURLs.add(((_item == null)?null:_item.clone()));
}
}
_newObject.securityMethod = ((this.securityMethod == null)?null:this.securityMethod.clone());
return _newObject;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public ServiceInterface createCopy() {
final ServiceInterface _newObject;
try {
_newObject = ((ServiceInterface) super.clone());
} catch (CloneNotSupportedException e) {
throw new RuntimeException(e);
}
if (this.accessURLs == null) {
_newObject.accessURLs = null;
} else {
_newObject.accessURLs = new ArrayList<>();
for (AccessURL _item: this.accessURLs) {
_newObject.accessURLs.add(((_item == null)?null:_item.createCopy()));
}
}
if (this.mirrorURLs == null) {
_newObject.mirrorURLs = null;
} else {
_newObject.mirrorURLs = new ArrayList<>();
for (MirrorURL _item: this.mirrorURLs) {
_newObject.mirrorURLs.add(((_item == null)?null:_item.createCopy()));
}
}
_newObject.securityMethod = ((this.securityMethod == null)?null:this.securityMethod.createCopy());
_newObject.testQueryString = this.testQueryString;
_newObject.version = this.version;
_newObject.role = this.role;
return _newObject;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public ServiceInterface createCopy(final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
final ServiceInterface _newObject;
try {
_newObject = ((ServiceInterface) super.clone());
} catch (CloneNotSupportedException e) {
throw new RuntimeException(e);
}
final PropertyTree accessURLsPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("accessURLs"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(accessURLsPropertyTree!= null):((accessURLsPropertyTree == null)||(!accessURLsPropertyTree.isLeaf())))) {
if (this.accessURLs == null) {
_newObject.accessURLs = null;
} else {
_newObject.accessURLs = new ArrayList<>();
for (AccessURL _item: this.accessURLs) {
_newObject.accessURLs.add(((_item == null)?null:_item.createCopy(accessURLsPropertyTree, _propertyTreeUse)));
}
}
}
final PropertyTree mirrorURLsPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("mirrorURLs"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(mirrorURLsPropertyTree!= null):((mirrorURLsPropertyTree == null)||(!mirrorURLsPropertyTree.isLeaf())))) {
if (this.mirrorURLs == null) {
_newObject.mirrorURLs = null;
} else {
_newObject.mirrorURLs = new ArrayList<>();
for (MirrorURL _item: this.mirrorURLs) {
_newObject.mirrorURLs.add(((_item == null)?null:_item.createCopy(mirrorURLsPropertyTree, _propertyTreeUse)));
}
}
}
final PropertyTree securityMethodPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("securityMethod"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(securityMethodPropertyTree!= null):((securityMethodPropertyTree == null)||(!securityMethodPropertyTree.isLeaf())))) {
_newObject.securityMethod = ((this.securityMethod == null)?null:this.securityMethod.createCopy(securityMethodPropertyTree, _propertyTreeUse));
}
final PropertyTree testQueryStringPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("testQueryString"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(testQueryStringPropertyTree!= null):((testQueryStringPropertyTree == null)||(!testQueryStringPropertyTree.isLeaf())))) {
_newObject.testQueryString = this.testQueryString;
}
final PropertyTree versionPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("version"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(versionPropertyTree!= null):((versionPropertyTree == null)||(!versionPropertyTree.isLeaf())))) {
_newObject.version = this.version;
}
final PropertyTree rolePropertyTree = ((_propertyTree == null)?null:_propertyTree.get("role"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(rolePropertyTree!= null):((rolePropertyTree == null)||(!rolePropertyTree.isLeaf())))) {
_newObject.role = this.role;
}
return _newObject;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public ServiceInterface copyExcept(final PropertyTree _propertyTree) {
return createCopy(_propertyTree, PropertyTreeUse.EXCLUDE);
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public ServiceInterface copyOnly(final PropertyTree _propertyTree) {
return createCopy(_propertyTree, PropertyTreeUse.INCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public ServiceInterface.Modifier modifier() {
if (null == this.__cachedModifier__) {
this.__cachedModifier__ = new ServiceInterface.Modifier();
}
return ((ServiceInterface.Modifier) this.__cachedModifier__);
}
/**
* Copies all state of this object to a builder. This method is used by the copyOf
* method and should not be called directly by client code.
*
* @param _other
* A builder instance to which the state of this object will be copied.
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public<_B >void copyTo(final ServiceInterface.Builder<_B> _other) {
if (this.accessURLs == null) {
_other.accessURLs = null;
} else {
_other.accessURLs = new ArrayList<>();
for (AccessURL _item: this.accessURLs) {
_other.accessURLs.add(((_item == null)?null:_item.newCopyBuilder(_other)));
}
}
if (this.mirrorURLs == null) {
_other.mirrorURLs = null;
} else {
_other.mirrorURLs = new ArrayList<>();
for (MirrorURL _item: this.mirrorURLs) {
_other.mirrorURLs.add(((_item == null)?null:_item.newCopyBuilder(_other)));
}
}
_other.securityMethod = ((this.securityMethod == null)?null:this.securityMethod.newCopyBuilder(_other));
_other.testQueryString = this.testQueryString;
_other.version = this.version;
_other.role = this.role;
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public abstract<_B >ServiceInterface.Builder<_B> newCopyBuilder(final _B _parentBuilder);
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public abstract ServiceInterface.Builder newCopyBuilder();
/**
* Copies all state of this object to a builder. This method is used by the copyOf
* method and should not be called directly by client code.
*
* @param _other
* A builder instance to which the state of this object will be copied.
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public<_B >void copyTo(final ServiceInterface.Builder<_B> _other, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
final PropertyTree accessURLsPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("accessURLs"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(accessURLsPropertyTree!= null):((accessURLsPropertyTree == null)||(!accessURLsPropertyTree.isLeaf())))) {
if (this.accessURLs == null) {
_other.accessURLs = null;
} else {
_other.accessURLs = new ArrayList<>();
for (AccessURL _item: this.accessURLs) {
_other.accessURLs.add(((_item == null)?null:_item.newCopyBuilder(_other, accessURLsPropertyTree, _propertyTreeUse)));
}
}
}
final PropertyTree mirrorURLsPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("mirrorURLs"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(mirrorURLsPropertyTree!= null):((mirrorURLsPropertyTree == null)||(!mirrorURLsPropertyTree.isLeaf())))) {
if (this.mirrorURLs == null) {
_other.mirrorURLs = null;
} else {
_other.mirrorURLs = new ArrayList<>();
for (MirrorURL _item: this.mirrorURLs) {
_other.mirrorURLs.add(((_item == null)?null:_item.newCopyBuilder(_other, mirrorURLsPropertyTree, _propertyTreeUse)));
}
}
}
final PropertyTree securityMethodPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("securityMethod"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(securityMethodPropertyTree!= null):((securityMethodPropertyTree == null)||(!securityMethodPropertyTree.isLeaf())))) {
_other.securityMethod = ((this.securityMethod == null)?null:this.securityMethod.newCopyBuilder(_other, securityMethodPropertyTree, _propertyTreeUse));
}
final PropertyTree testQueryStringPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("testQueryString"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(testQueryStringPropertyTree!= null):((testQueryStringPropertyTree == null)||(!testQueryStringPropertyTree.isLeaf())))) {
_other.testQueryString = this.testQueryString;
}
final PropertyTree versionPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("version"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(versionPropertyTree!= null):((versionPropertyTree == null)||(!versionPropertyTree.isLeaf())))) {
_other.version = this.version;
}
final PropertyTree rolePropertyTree = ((_propertyTree == null)?null:_propertyTree.get("role"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(rolePropertyTree!= null):((rolePropertyTree == null)||(!rolePropertyTree.isLeaf())))) {
_other.role = this.role;
}
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public abstract<_B >ServiceInterface.Builder<_B> newCopyBuilder(final _B _parentBuilder, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse);
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public abstract ServiceInterface.Builder newCopyBuilder(final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse);
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public ServiceInterface visit(final PropertyVisitor _visitor_) {
_visitor_.visit(this);
if (_visitor_.visit(new CollectionProperty<>(ServiceInterface.PropInfo.ACCESS_UR_LS, this))&&(this.accessURLs!= null)) {
for (AccessURL _item_: this.accessURLs) {
if (_item_!= null) {
_item_.visit(_visitor_);
}
}
}
if (_visitor_.visit(new CollectionProperty<>(ServiceInterface.PropInfo.MIRROR_UR_LS, this))&&(this.mirrorURLs!= null)) {
for (MirrorURL _item_: this.mirrorURLs) {
if (_item_!= null) {
_item_.visit(_visitor_);
}
}
}
if (_visitor_.visit(new SingleProperty<>(ServiceInterface.PropInfo.SECURITY_METHOD, this))&&(this.securityMethod!= null)) {
this.securityMethod.visit(_visitor_);
}
_visitor_.visit(new SingleProperty<>(ServiceInterface.PropInfo.TEST_QUERY_STRING, this));
_visitor_.visit(new SingleProperty<>(ServiceInterface.PropInfo.VERSION, this));
_visitor_.visit(new SingleProperty<>(ServiceInterface.PropInfo.ROLE, this));
return this;
}
public static class Builder<_B >implements Buildable
{
protected final _B _parentBuilder;
protected final ServiceInterface _storedValue;
private List>> accessURLs;
private List>> mirrorURLs;
private SecurityMethod.Builder> securityMethod;
private String testQueryString;
private String version;
private String role;
public Builder(final _B _parentBuilder, final ServiceInterface _other, final boolean _copy) {
this._parentBuilder = _parentBuilder;
if (_other!= null) {
if (_copy) {
_storedValue = null;
if (_other.accessURLs == null) {
this.accessURLs = null;
} else {
this.accessURLs = new ArrayList<>();
for (AccessURL _item: _other.accessURLs) {
this.accessURLs.add(((_item == null)?null:_item.newCopyBuilder(this)));
}
}
if (_other.mirrorURLs == null) {
this.mirrorURLs = null;
} else {
this.mirrorURLs = new ArrayList<>();
for (MirrorURL _item: _other.mirrorURLs) {
this.mirrorURLs.add(((_item == null)?null:_item.newCopyBuilder(this)));
}
}
this.securityMethod = ((_other.securityMethod == null)?null:_other.securityMethod.newCopyBuilder(this));
this.testQueryString = _other.testQueryString;
this.version = _other.version;
this.role = _other.role;
} else {
_storedValue = _other;
}
} else {
_storedValue = null;
}
}
public Builder(final _B _parentBuilder, final ServiceInterface _other, final boolean _copy, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
this._parentBuilder = _parentBuilder;
if (_other!= null) {
if (_copy) {
_storedValue = null;
final PropertyTree accessURLsPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("accessURLs"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(accessURLsPropertyTree!= null):((accessURLsPropertyTree == null)||(!accessURLsPropertyTree.isLeaf())))) {
if (_other.accessURLs == null) {
this.accessURLs = null;
} else {
this.accessURLs = new ArrayList<>();
for (AccessURL _item: _other.accessURLs) {
this.accessURLs.add(((_item == null)?null:_item.newCopyBuilder(this, accessURLsPropertyTree, _propertyTreeUse)));
}
}
}
final PropertyTree mirrorURLsPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("mirrorURLs"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(mirrorURLsPropertyTree!= null):((mirrorURLsPropertyTree == null)||(!mirrorURLsPropertyTree.isLeaf())))) {
if (_other.mirrorURLs == null) {
this.mirrorURLs = null;
} else {
this.mirrorURLs = new ArrayList<>();
for (MirrorURL _item: _other.mirrorURLs) {
this.mirrorURLs.add(((_item == null)?null:_item.newCopyBuilder(this, mirrorURLsPropertyTree, _propertyTreeUse)));
}
}
}
final PropertyTree securityMethodPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("securityMethod"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(securityMethodPropertyTree!= null):((securityMethodPropertyTree == null)||(!securityMethodPropertyTree.isLeaf())))) {
this.securityMethod = ((_other.securityMethod == null)?null:_other.securityMethod.newCopyBuilder(this, securityMethodPropertyTree, _propertyTreeUse));
}
final PropertyTree testQueryStringPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("testQueryString"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(testQueryStringPropertyTree!= null):((testQueryStringPropertyTree == null)||(!testQueryStringPropertyTree.isLeaf())))) {
this.testQueryString = _other.testQueryString;
}
final PropertyTree versionPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("version"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(versionPropertyTree!= null):((versionPropertyTree == null)||(!versionPropertyTree.isLeaf())))) {
this.version = _other.version;
}
final PropertyTree rolePropertyTree = ((_propertyTree == null)?null:_propertyTree.get("role"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(rolePropertyTree!= null):((rolePropertyTree == null)||(!rolePropertyTree.isLeaf())))) {
this.role = _other.role;
}
} else {
_storedValue = _other;
}
} else {
_storedValue = null;
}
}
public _B end() {
return this._parentBuilder;
}
protected<_P extends ServiceInterface >_P init(final _P _product) {
if (this.accessURLs!= null) {
final List accessURLs = new ArrayList<>(this.accessURLs.size());
for (AccessURL.Builder> _item: this.accessURLs) {
accessURLs.add(_item.build());
}
_product.accessURLs = accessURLs;
}
if (this.mirrorURLs!= null) {
final List mirrorURLs = new ArrayList<>(this.mirrorURLs.size());
for (MirrorURL.Builder> _item: this.mirrorURLs) {
mirrorURLs.add(_item.build());
}
_product.mirrorURLs = mirrorURLs;
}
_product.securityMethod = ((this.securityMethod == null)?null:this.securityMethod.build());
_product.testQueryString = this.testQueryString;
_product.version = this.version;
_product.role = this.role;
return _product;
}
/**
* Adds the given items to the value of "accessURLs"
*
* @param accessURLs
* Items to add to the value of the "accessURLs" property
*/
public ServiceInterface.Builder<_B> addAccessURLs(final Iterable extends AccessURL> accessURLs) {
if (accessURLs!= null) {
if (this.accessURLs == null) {
this.accessURLs = new ArrayList<>();
}
for (AccessURL _item: accessURLs) {
this.accessURLs.add(new AccessURL.Builder<>(this, _item, false));
}
}
return this;
}
/**
* Sets the new value of "accessURLs" (any previous value will be replaced)
*
* @param accessURLs
* New value of the "accessURLs" property.
*/
public ServiceInterface.Builder<_B> withAccessURLs(final Iterable extends AccessURL> accessURLs) {
if (this.accessURLs!= null) {
this.accessURLs.clear();
}
return addAccessURLs(accessURLs);
}
/**
* Adds the given items to the value of "accessURLs"
*
* @param accessURLs
* Items to add to the value of the "accessURLs" property
*/
public ServiceInterface.Builder<_B> addAccessURLs(AccessURL... accessURLs) {
addAccessURLs(Arrays.asList(accessURLs));
return this;
}
/**
* Sets the new value of "accessURLs" (any previous value will be replaced)
*
* @param accessURLs
* New value of the "accessURLs" property.
*/
public ServiceInterface.Builder<_B> withAccessURLs(AccessURL... accessURLs) {
withAccessURLs(Arrays.asList(accessURLs));
return this;
}
/**
* Returns a new builder to build an additional value of the "AccessURLs" property.
* Use {@link org.javastro.ivoa.entities.resource.AccessURL.Builder#end()} to
* return to the current builder.
*
* @return
* a new builder to build an additional value of the "AccessURLs" property.
* Use {@link org.javastro.ivoa.entities.resource.AccessURL.Builder#end()} to
* return to the current builder.
*/
public AccessURL.Builder extends ServiceInterface.Builder<_B>> addAccessURLs() {
if (this.accessURLs == null) {
this.accessURLs = new ArrayList<>();
}
final AccessURL.Builder> accessURLs_Builder = new AccessURL.Builder<>(this, null, false);
this.accessURLs.add(accessURLs_Builder);
return accessURLs_Builder;
}
/**
* Adds the given items to the value of "mirrorURLs"
*
* @param mirrorURLs
* Items to add to the value of the "mirrorURLs" property
*/
public ServiceInterface.Builder<_B> addMirrorURLs(final Iterable extends MirrorURL> mirrorURLs) {
if (mirrorURLs!= null) {
if (this.mirrorURLs == null) {
this.mirrorURLs = new ArrayList<>();
}
for (MirrorURL _item: mirrorURLs) {
this.mirrorURLs.add(new MirrorURL.Builder<>(this, _item, false));
}
}
return this;
}
/**
* Sets the new value of "mirrorURLs" (any previous value will be replaced)
*
* @param mirrorURLs
* New value of the "mirrorURLs" property.
*/
public ServiceInterface.Builder<_B> withMirrorURLs(final Iterable extends MirrorURL> mirrorURLs) {
if (this.mirrorURLs!= null) {
this.mirrorURLs.clear();
}
return addMirrorURLs(mirrorURLs);
}
/**
* Adds the given items to the value of "mirrorURLs"
*
* @param mirrorURLs
* Items to add to the value of the "mirrorURLs" property
*/
public ServiceInterface.Builder<_B> addMirrorURLs(MirrorURL... mirrorURLs) {
addMirrorURLs(Arrays.asList(mirrorURLs));
return this;
}
/**
* Sets the new value of "mirrorURLs" (any previous value will be replaced)
*
* @param mirrorURLs
* New value of the "mirrorURLs" property.
*/
public ServiceInterface.Builder<_B> withMirrorURLs(MirrorURL... mirrorURLs) {
withMirrorURLs(Arrays.asList(mirrorURLs));
return this;
}
/**
* Returns a new builder to build an additional value of the "MirrorURLs" property.
* Use {@link org.javastro.ivoa.entities.resource.MirrorURL.Builder#end()} to
* return to the current builder.
*
* @return
* a new builder to build an additional value of the "MirrorURLs" property.
* Use {@link org.javastro.ivoa.entities.resource.MirrorURL.Builder#end()} to
* return to the current builder.
*/
public MirrorURL.Builder extends ServiceInterface.Builder<_B>> addMirrorURLs() {
if (this.mirrorURLs == null) {
this.mirrorURLs = new ArrayList<>();
}
final MirrorURL.Builder> mirrorURLs_Builder = new MirrorURL.Builder<>(this, null, false);
this.mirrorURLs.add(mirrorURLs_Builder);
return mirrorURLs_Builder;
}
/**
* Sets the new value of "securityMethod" (any previous value will be replaced)
*
* @param securityMethod
* New value of the "securityMethod" property.
*/
public ServiceInterface.Builder<_B> withSecurityMethod(final SecurityMethod securityMethod) {
this.securityMethod = ((securityMethod == null)?null:new SecurityMethod.Builder<>(this, securityMethod, false));
return this;
}
/**
* Returns the existing builder or a new builder to build the value of the
* "securityMethod" property.
* Use {@link org.javastro.ivoa.entities.resource.SecurityMethod.Builder#end()} to
* return to the current builder.
*
* @return
* A new builder to build the value of the "securityMethod" property.
* Use {@link org.javastro.ivoa.entities.resource.SecurityMethod.Builder#end()} to
* return to the current builder.
*/
public SecurityMethod.Builder extends ServiceInterface.Builder<_B>> withSecurityMethod() {
if (this.securityMethod!= null) {
return this.securityMethod;
}
return this.securityMethod = new SecurityMethod.Builder<>(this, null, false);
}
/**
* Sets the new value of "testQueryString" (any previous value will be replaced)
*
* @param testQueryString
* New value of the "testQueryString" property.
*/
public ServiceInterface.Builder<_B> withTestQueryString(final String testQueryString) {
this.testQueryString = testQueryString;
return this;
}
/**
* Sets the new value of "version" (any previous value will be replaced)
*
* @param version
* New value of the "version" property.
*/
public ServiceInterface.Builder<_B> withVersion(final String version) {
this.version = version;
return this;
}
/**
* Sets the new value of "role" (any previous value will be replaced)
*
* @param role
* New value of the "role" property.
*/
public ServiceInterface.Builder<_B> withRole(final String role) {
this.role = role;
return this;
}
@Override
public ServiceInterface build() {
return ((ServiceInterface) _storedValue);
}
public ServiceInterface.Builder<_B> copyOf(final ServiceInterface _other) {
_other.copyTo(this);
return this;
}
public ServiceInterface.Builder<_B> copyOf(final ServiceInterface.Builder _other) {
return copyOf(_other.build());
}
}
public class Modifier {
public List getAccessURLs() {
if (ServiceInterface.this.accessURLs == null) {
ServiceInterface.this.accessURLs = new ArrayList<>();
}
return ServiceInterface.this.accessURLs;
}
public List getMirrorURLs() {
if (ServiceInterface.this.mirrorURLs == null) {
ServiceInterface.this.mirrorURLs = new ArrayList<>();
}
return ServiceInterface.this.mirrorURLs;
}
public void setSecurityMethod(final SecurityMethod securityMethod) {
ServiceInterface.this.setSecurityMethod(securityMethod);
}
public void setTestQueryString(final String testQueryString) {
ServiceInterface.this.setTestQueryString(testQueryString);
}
public void setVersion(final String version) {
ServiceInterface.this.setVersion(version);
}
public void setRole(final String role) {
ServiceInterface.this.setRole(role);
}
}
public static class PropInfo {
public static final transient CollectionPropertyInfo ACCESS_UR_LS = new CollectionPropertyInfo("accessURLs", ServiceInterface.class, AccessURL.class, true, null, new QName("", "accessURL"), new QName("http://www.ivoa.net/xml/VOResource/v1.0", "AccessURL"), false) {
@Override
public List get(final ServiceInterface _instance_) {
return ((_instance_ == null)?null:_instance_.accessURLs);
}
@Override
public void set(final ServiceInterface _instance_, final List _value_) {
if (_instance_!= null) {
_instance_.accessURLs = _value_;
}
}
}
;
public static final transient CollectionPropertyInfo MIRROR_UR_LS = new CollectionPropertyInfo("mirrorURLs", ServiceInterface.class, MirrorURL.class, true, null, new QName("", "mirrorURL"), new QName("http://www.ivoa.net/xml/VOResource/v1.0", "MirrorURL"), false) {
@Override
public List get(final ServiceInterface _instance_) {
return ((_instance_ == null)?null:_instance_.mirrorURLs);
}
@Override
public void set(final ServiceInterface _instance_, final List _value_) {
if (_instance_!= null) {
_instance_.mirrorURLs = _value_;
}
}
}
;
public static final transient SinglePropertyInfo SECURITY_METHOD = new SinglePropertyInfo("securityMethod", ServiceInterface.class, SecurityMethod.class, false, null, new QName("", "securityMethod"), new QName("http://www.ivoa.net/xml/VOResource/v1.0", "SecurityMethod"), false) {
@Override
public SecurityMethod get(final ServiceInterface _instance_) {
return ((_instance_ == null)?null:_instance_.securityMethod);
}
@Override
public void set(final ServiceInterface _instance_, final SecurityMethod _value_) {
if (_instance_!= null) {
_instance_.securityMethod = _value_;
}
}
}
;
public static final transient SinglePropertyInfo TEST_QUERY_STRING = new SinglePropertyInfo("testQueryString", ServiceInterface.class, String.class, false, null, new QName("", "testQueryString"), new QName("http://www.w3.org/2001/XMLSchema", "token"), false) {
@Override
public String get(final ServiceInterface _instance_) {
return ((_instance_ == null)?null:_instance_.testQueryString);
}
@Override
public void set(final ServiceInterface _instance_, final String _value_) {
if (_instance_!= null) {
_instance_.testQueryString = _value_;
}
}
}
;
public static final transient SinglePropertyInfo VERSION = new SinglePropertyInfo("version", ServiceInterface.class, String.class, false, null, new QName("", "version"), new QName("http://www.w3.org/2001/XMLSchema", "string"), true) {
@Override
public String get(final ServiceInterface _instance_) {
return ((_instance_ == null)?null:_instance_.version);
}
@Override
public void set(final ServiceInterface _instance_, final String _value_) {
if (_instance_!= null) {
_instance_.version = _value_;
}
}
}
;
public static final transient SinglePropertyInfo ROLE = new SinglePropertyInfo("role", ServiceInterface.class, String.class, false, null, new QName("", "role"), new QName("http://www.w3.org/2001/XMLSchema", "NMTOKEN"), true) {
@Override
public String get(final ServiceInterface _instance_) {
return ((_instance_ == null)?null:_instance_.role);
}
@Override
public void set(final ServiceInterface _instance_, final String _value_) {
if (_instance_!= null) {
_instance_.role = _value_;
}
}
}
;
}
public static class Select
extends ServiceInterface.Selector
{
Select() {
super(null, null, null);
}
public static ServiceInterface.Select _root() {
return new ServiceInterface.Select();
}
}
public static class Selector , TParent >
extends com.kscs.util.jaxb.Selector
{
private AccessURL.Selector> accessURLs = null;
private MirrorURL.Selector> mirrorURLs = null;
private SecurityMethod.Selector> securityMethod = null;
private com.kscs.util.jaxb.Selector> testQueryString = null;
private com.kscs.util.jaxb.Selector> version = null;
private com.kscs.util.jaxb.Selector> role = null;
public Selector(final TRoot root, final TParent parent, final String propertyName) {
super(root, parent, propertyName);
}
@Override
public Map buildChildren() {
final Map products = new HashMap<>();
products.putAll(super.buildChildren());
if (this.accessURLs!= null) {
products.put("accessURLs", this.accessURLs.init());
}
if (this.mirrorURLs!= null) {
products.put("mirrorURLs", this.mirrorURLs.init());
}
if (this.securityMethod!= null) {
products.put("securityMethod", this.securityMethod.init());
}
if (this.testQueryString!= null) {
products.put("testQueryString", this.testQueryString.init());
}
if (this.version!= null) {
products.put("version", this.version.init());
}
if (this.role!= null) {
products.put("role", this.role.init());
}
return products;
}
public AccessURL.Selector> accessURLs() {
return ((this.accessURLs == null)?this.accessURLs = new AccessURL.Selector<>(this._root, this, "accessURLs"):this.accessURLs);
}
public MirrorURL.Selector> mirrorURLs() {
return ((this.mirrorURLs == null)?this.mirrorURLs = new MirrorURL.Selector<>(this._root, this, "mirrorURLs"):this.mirrorURLs);
}
public SecurityMethod.Selector> securityMethod() {
return ((this.securityMethod == null)?this.securityMethod = new SecurityMethod.Selector<>(this._root, this, "securityMethod"):this.securityMethod);
}
public com.kscs.util.jaxb.Selector> testQueryString() {
return ((this.testQueryString == null)?this.testQueryString = new com.kscs.util.jaxb.Selector<>(this._root, this, "testQueryString"):this.testQueryString);
}
public com.kscs.util.jaxb.Selector> version() {
return ((this.version == null)?this.version = new com.kscs.util.jaxb.Selector<>(this._root, this, "version"):this.version);
}
public com.kscs.util.jaxb.Selector> role() {
return ((this.role == null)?this.role = new com.kscs.util.jaxb.Selector<>(this._root, this, "role"):this.role);
}
}
}