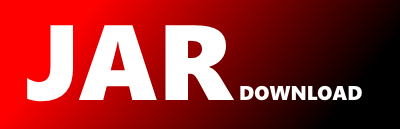
org.javastro.ivoa.entities.resource.applications.Application Maven / Gradle / Ivy
package org.javastro.ivoa.entities.resource.applications;
import java.time.LocalDateTime;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import javax.xml.namespace.QName;
import com.kscs.util.jaxb.Buildable;
import com.kscs.util.jaxb.CollectionProperty;
import com.kscs.util.jaxb.CollectionPropertyInfo;
import com.kscs.util.jaxb.CollectionPropertyInfo;
import com.kscs.util.jaxb.CollectionPropertyInfo;
import com.kscs.util.jaxb.CollectionPropertyInfo;
import com.kscs.util.jaxb.Copyable;
import com.kscs.util.jaxb.PartialCopyable;
import com.kscs.util.jaxb.PropertyTree;
import com.kscs.util.jaxb.PropertyTreeUse;
import com.kscs.util.jaxb.PropertyVisitor;
import com.kscs.util.jaxb.SingleProperty;
import com.kscs.util.jaxb.SinglePropertyInfo;
import com.kscs.util.jaxb.SinglePropertyInfo;
import com.kscs.util.jaxb.SinglePropertyInfo;
import jakarta.annotation.Generated;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlSchemaType;
import jakarta.xml.bind.annotation.XmlSeeAlso;
import jakarta.xml.bind.annotation.XmlType;
import org.javastro.ivoa.entities.resource.Content;
import org.javastro.ivoa.entities.resource.Curation;
import org.javastro.ivoa.entities.resource.Resource;
import org.javastro.ivoa.entities.resource.Validation;
import org.jvnet.jaxb.lang.JAXBMergeStrategy;
import org.jvnet.jaxb.lang.JAXBToStringStrategy;
import org.jvnet.jaxb.lang.MergeFrom;
import org.jvnet.jaxb.lang.MergeStrategy;
import org.jvnet.jaxb.lang.ToString;
import org.jvnet.jaxb.lang.ToStringStrategy;
import org.jvnet.jaxb.locator.ObjectLocator;
import org.jvnet.jaxb.locator.util.LocatorUtils;
/**
* The basic description of an application.
*
* Java class for Application complex type
.
*
* The following schema fragment specifies the expected content contained within this class.
*
* {@code
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
* }
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "Application", propOrder = {
"cost",
"licence",
"openSource",
"dataFormats",
"voStandards",
"sourceLanguages",
"sourceCodeURL"
})
@XmlSeeAlso({
DesktopApplication.class,
SoftwareLibrary.class
})
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public class Application
extends Resource
implements Cloneable, Copyable, PartialCopyable, MergeFrom, ToString
{
/**
* an indication as to what the cost of the
* application is - the string "free" or "none"
* should be used to indicate that the application
* does not cost anything. Any value for the cost
* of any non-free software should be taken to be
* an approximate cost for one license - it is
* obviously difficult to curate this value for
* non-free software so that the main use of this
* element will be to distinguish between free and
* non-free.
*
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
protected String cost;
/**
* A free text value that indicates what type of
* licence is in force. This can either be a common
* name of a license or a URL pointing to the
* license text.
*
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
protected String licence;
/**
* Is the software open source. See
* http://www.opensource.org/docs/definition.php
* for the definition of "open source"
*
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
protected Boolean openSource;
/**
* File formats that this application can read or
* write. The formats are specified by reference to
* an IVOA identifier that describes the format.
*
*/
@XmlElement(name = "dataFormat")
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
protected List dataFormats;
/**
* enumerate which standards this application is
* compliant with - *Editor note* not really too
* happy with this, as there is a certain amount of
* potential redundancy here if there is a derived
* type - e.g. a CeaApplication type will support
* CEA standard so at least one entry would have to
* be placed here...
*
*/
@XmlElement(name = "voStandard")
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
protected List voStandards;
/**
* The IVOA identifier for the principal language
* that the application is written in.
*
*/
@XmlElement(name = "sourceLanguage")
@XmlSchemaType(name = "anyURI")
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
protected List sourceLanguages;
/**
* The location where the source code can be found.
* This might be a URL to a specific archive file
* containing the source, or to the access pages
* for a source code management system.
*
*/
@XmlSchemaType(name = "anyURI")
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
protected String sourceCodeURL;
/**
* Default no-arg constructor
*
*/
public Application() {
super();
}
/**
* Fully-initialising value constructor
*
*/
public Application(final List validationLevels, final String title, final String shortName, final String identifier, final List altIdentifiers, final Curation curation, final Content content, final LocalDateTime created, final LocalDateTime updated, final String status, final String version, final String cost, final String licence, final Boolean openSource, final List dataFormats, final List voStandards, final List sourceLanguages, final String sourceCodeURL) {
super(validationLevels, title, shortName, identifier, altIdentifiers, curation, content, created, updated, status, version);
this.cost = cost;
this.licence = licence;
this.openSource = openSource;
this.dataFormats = dataFormats;
this.voStandards = voStandards;
this.sourceLanguages = sourceLanguages;
this.sourceCodeURL = sourceCodeURL;
}
/**
* an indication as to what the cost of the
* application is - the string "free" or "none"
* should be used to indicate that the application
* does not cost anything. Any value for the cost
* of any non-free software should be taken to be
* an approximate cost for one license - it is
* obviously difficult to curate this value for
* non-free software so that the main use of this
* element will be to distinguish between free and
* non-free.
*
* @return
* possible object is
* {@link String }
*
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public String getCost() {
return cost;
}
/**
* Sets the value of the cost property.
*
* @param value
* allowed object is
* {@link String }
*
* @see #getCost()
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void setCost(String value) {
this.cost = value;
}
/**
* A free text value that indicates what type of
* licence is in force. This can either be a common
* name of a license or a URL pointing to the
* license text.
*
* @return
* possible object is
* {@link String }
*
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public String getLicence() {
return licence;
}
/**
* Sets the value of the licence property.
*
* @param value
* allowed object is
* {@link String }
*
* @see #getLicence()
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void setLicence(String value) {
this.licence = value;
}
/**
* Is the software open source. See
* http://www.opensource.org/docs/definition.php
* for the definition of "open source"
*
* @return
* possible object is
* {@link Boolean }
*
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Boolean isOpenSource() {
return openSource;
}
/**
* Sets the value of the openSource property.
*
* @param value
* allowed object is
* {@link Boolean }
*
* @see #isOpenSource()
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void setOpenSource(Boolean value) {
this.openSource = value;
}
/**
* File formats that this application can read or
* write. The formats are specified by reference to
* an IVOA identifier that describes the format.
*
* Gets the value of the dataFormats property.
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the dataFormats property.
*
*
* For example, to add a new item, do as follows:
*
*
* getDataFormats().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link DataFormat }
*
*
*
* @return
* The value of the dataFormats property.
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public List getDataFormats() {
if (dataFormats == null) {
dataFormats = new ArrayList<>();
}
return this.dataFormats;
}
/**
* enumerate which standards this application is
* compliant with - *Editor note* not really too
* happy with this, as there is a certain amount of
* potential redundancy here if there is a derived
* type - e.g. a CeaApplication type will support
* CEA standard so at least one entry would have to
* be placed here...
*
* Gets the value of the voStandards property.
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the voStandards property.
*
*
* For example, to add a new item, do as follows:
*
*
* getVoStandards().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link ApplicationCapability }
*
*
*
* @return
* The value of the voStandards property.
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public List getVoStandards() {
if (voStandards == null) {
voStandards = new ArrayList<>();
}
return this.voStandards;
}
/**
* The IVOA identifier for the principal language
* that the application is written in.
*
* Gets the value of the sourceLanguages property.
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the sourceLanguages property.
*
*
* For example, to add a new item, do as follows:
*
*
* getSourceLanguages().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link String }
*
*
*
* @return
* The value of the sourceLanguages property.
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public List getSourceLanguages() {
if (sourceLanguages == null) {
sourceLanguages = new ArrayList<>();
}
return this.sourceLanguages;
}
/**
* The location where the source code can be found.
* This might be a URL to a specific archive file
* containing the source, or to the access pages
* for a source code management system.
*
* @return
* possible object is
* {@link String }
*
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public String getSourceCodeURL() {
return sourceCodeURL;
}
/**
* Sets the value of the sourceCodeURL property.
*
* @param value
* allowed object is
* {@link String }
*
* @see #getSourceCodeURL()
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void setSourceCodeURL(String value) {
this.sourceCodeURL = value;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public boolean equals(Object object) {
if ((object == null)||(this.getClass()!= object.getClass())) {
return false;
}
if (this == object) {
return true;
}
if (!super.equals(object)) {
return false;
}
final Application that = ((Application) object);
{
String leftCost;
leftCost = this.getCost();
String rightCost;
rightCost = that.getCost();
if (this.cost!= null) {
if (that.cost!= null) {
if (!leftCost.equals(rightCost)) {
return false;
}
} else {
return false;
}
} else {
if (that.cost!= null) {
return false;
}
}
}
{
String leftLicence;
leftLicence = this.getLicence();
String rightLicence;
rightLicence = that.getLicence();
if (this.licence!= null) {
if (that.licence!= null) {
if (!leftLicence.equals(rightLicence)) {
return false;
}
} else {
return false;
}
} else {
if (that.licence!= null) {
return false;
}
}
}
{
Boolean leftOpenSource;
leftOpenSource = this.isOpenSource();
Boolean rightOpenSource;
rightOpenSource = that.isOpenSource();
if (this.openSource!= null) {
if (that.openSource!= null) {
if (!leftOpenSource.equals(rightOpenSource)) {
return false;
}
} else {
return false;
}
} else {
if (that.openSource!= null) {
return false;
}
}
}
{
List leftDataFormats;
leftDataFormats = (((this.dataFormats!= null)&&(!this.dataFormats.isEmpty()))?this.getDataFormats():null);
List rightDataFormats;
rightDataFormats = (((that.dataFormats!= null)&&(!that.dataFormats.isEmpty()))?that.getDataFormats():null);
if ((this.dataFormats!= null)&&(!this.dataFormats.isEmpty())) {
if ((that.dataFormats!= null)&&(!that.dataFormats.isEmpty())) {
if (!leftDataFormats.equals(rightDataFormats)) {
return false;
}
} else {
return false;
}
} else {
if ((that.dataFormats!= null)&&(!that.dataFormats.isEmpty())) {
return false;
}
}
}
{
List leftVoStandards;
leftVoStandards = (((this.voStandards!= null)&&(!this.voStandards.isEmpty()))?this.getVoStandards():null);
List rightVoStandards;
rightVoStandards = (((that.voStandards!= null)&&(!that.voStandards.isEmpty()))?that.getVoStandards():null);
if ((this.voStandards!= null)&&(!this.voStandards.isEmpty())) {
if ((that.voStandards!= null)&&(!that.voStandards.isEmpty())) {
if (!leftVoStandards.equals(rightVoStandards)) {
return false;
}
} else {
return false;
}
} else {
if ((that.voStandards!= null)&&(!that.voStandards.isEmpty())) {
return false;
}
}
}
{
List leftSourceLanguages;
leftSourceLanguages = (((this.sourceLanguages!= null)&&(!this.sourceLanguages.isEmpty()))?this.getSourceLanguages():null);
List rightSourceLanguages;
rightSourceLanguages = (((that.sourceLanguages!= null)&&(!that.sourceLanguages.isEmpty()))?that.getSourceLanguages():null);
if ((this.sourceLanguages!= null)&&(!this.sourceLanguages.isEmpty())) {
if ((that.sourceLanguages!= null)&&(!that.sourceLanguages.isEmpty())) {
if (!leftSourceLanguages.equals(rightSourceLanguages)) {
return false;
}
} else {
return false;
}
} else {
if ((that.sourceLanguages!= null)&&(!that.sourceLanguages.isEmpty())) {
return false;
}
}
}
{
String leftSourceCodeURL;
leftSourceCodeURL = this.getSourceCodeURL();
String rightSourceCodeURL;
rightSourceCodeURL = that.getSourceCodeURL();
if (this.sourceCodeURL!= null) {
if (that.sourceCodeURL!= null) {
if (!leftSourceCodeURL.equals(rightSourceCodeURL)) {
return false;
}
} else {
return false;
}
} else {
if (that.sourceCodeURL!= null) {
return false;
}
}
}
return true;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public int hashCode() {
int currentHashCode = 1;
currentHashCode = ((currentHashCode* 31)+ super.hashCode());
{
currentHashCode = (currentHashCode* 31);
String theCost;
theCost = this.getCost();
if (this.cost!= null) {
currentHashCode += theCost.hashCode();
}
}
{
currentHashCode = (currentHashCode* 31);
String theLicence;
theLicence = this.getLicence();
if (this.licence!= null) {
currentHashCode += theLicence.hashCode();
}
}
{
currentHashCode = (currentHashCode* 31);
Boolean theOpenSource;
theOpenSource = this.isOpenSource();
if (this.openSource!= null) {
currentHashCode += theOpenSource.hashCode();
}
}
{
currentHashCode = (currentHashCode* 31);
List theDataFormats;
theDataFormats = (((this.dataFormats!= null)&&(!this.dataFormats.isEmpty()))?this.getDataFormats():null);
if ((this.dataFormats!= null)&&(!this.dataFormats.isEmpty())) {
currentHashCode += theDataFormats.hashCode();
}
}
{
currentHashCode = (currentHashCode* 31);
List theVoStandards;
theVoStandards = (((this.voStandards!= null)&&(!this.voStandards.isEmpty()))?this.getVoStandards():null);
if ((this.voStandards!= null)&&(!this.voStandards.isEmpty())) {
currentHashCode += theVoStandards.hashCode();
}
}
{
currentHashCode = (currentHashCode* 31);
List theSourceLanguages;
theSourceLanguages = (((this.sourceLanguages!= null)&&(!this.sourceLanguages.isEmpty()))?this.getSourceLanguages():null);
if ((this.sourceLanguages!= null)&&(!this.sourceLanguages.isEmpty())) {
currentHashCode += theSourceLanguages.hashCode();
}
}
{
currentHashCode = (currentHashCode* 31);
String theSourceCodeURL;
theSourceCodeURL = this.getSourceCodeURL();
if (this.sourceCodeURL!= null) {
currentHashCode += theSourceCodeURL.hashCode();
}
}
return currentHashCode;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public String toString() {
final ToStringStrategy strategy = JAXBToStringStrategy.getInstance();
final StringBuilder buffer = new StringBuilder();
append(null, buffer, strategy);
return buffer.toString();
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public StringBuilder append(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
strategy.appendStart(locator, this, buffer);
appendFields(locator, buffer, strategy);
strategy.appendEnd(locator, this, buffer);
return buffer;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public StringBuilder appendFields(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
super.appendFields(locator, buffer, strategy);
{
String theCost;
theCost = this.getCost();
strategy.appendField(locator, this, "cost", buffer, theCost, (this.cost!= null));
}
{
String theLicence;
theLicence = this.getLicence();
strategy.appendField(locator, this, "licence", buffer, theLicence, (this.licence!= null));
}
{
Boolean theOpenSource;
theOpenSource = this.isOpenSource();
strategy.appendField(locator, this, "openSource", buffer, theOpenSource, (this.openSource!= null));
}
{
List theDataFormats;
theDataFormats = (((this.dataFormats!= null)&&(!this.dataFormats.isEmpty()))?this.getDataFormats():null);
strategy.appendField(locator, this, "dataFormats", buffer, theDataFormats, ((this.dataFormats!= null)&&(!this.dataFormats.isEmpty())));
}
{
List theVoStandards;
theVoStandards = (((this.voStandards!= null)&&(!this.voStandards.isEmpty()))?this.getVoStandards():null);
strategy.appendField(locator, this, "voStandards", buffer, theVoStandards, ((this.voStandards!= null)&&(!this.voStandards.isEmpty())));
}
{
List theSourceLanguages;
theSourceLanguages = (((this.sourceLanguages!= null)&&(!this.sourceLanguages.isEmpty()))?this.getSourceLanguages():null);
strategy.appendField(locator, this, "sourceLanguages", buffer, theSourceLanguages, ((this.sourceLanguages!= null)&&(!this.sourceLanguages.isEmpty())));
}
{
String theSourceCodeURL;
theSourceCodeURL = this.getSourceCodeURL();
strategy.appendField(locator, this, "sourceCodeURL", buffer, theSourceCodeURL, (this.sourceCodeURL!= null));
}
return buffer;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void mergeFrom(Object left, Object right) {
final MergeStrategy strategy = JAXBMergeStrategy.getInstance();
mergeFrom(null, null, left, right, strategy);
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void mergeFrom(ObjectLocator leftLocator, ObjectLocator rightLocator, Object left, Object right, MergeStrategy strategy) {
super.mergeFrom(leftLocator, rightLocator, left, right, strategy);
if (right instanceof Application) {
final Application target = this;
final Application leftObject = ((Application) left);
final Application rightObject = ((Application) right);
{
Boolean costShouldBeMergedAndSet = strategy.shouldBeMergedAndSet(leftLocator, rightLocator, (leftObject.cost!= null), (rightObject.cost!= null));
if (costShouldBeMergedAndSet == Boolean.TRUE) {
String lhsCost;
lhsCost = leftObject.getCost();
String rhsCost;
rhsCost = rightObject.getCost();
String mergedCost = ((String) strategy.merge(LocatorUtils.property(leftLocator, "cost", lhsCost), LocatorUtils.property(rightLocator, "cost", rhsCost), lhsCost, rhsCost, (leftObject.cost!= null), (rightObject.cost!= null)));
target.setCost(mergedCost);
} else {
if (costShouldBeMergedAndSet == Boolean.FALSE) {
target.cost = null;
}
}
}
{
Boolean licenceShouldBeMergedAndSet = strategy.shouldBeMergedAndSet(leftLocator, rightLocator, (leftObject.licence!= null), (rightObject.licence!= null));
if (licenceShouldBeMergedAndSet == Boolean.TRUE) {
String lhsLicence;
lhsLicence = leftObject.getLicence();
String rhsLicence;
rhsLicence = rightObject.getLicence();
String mergedLicence = ((String) strategy.merge(LocatorUtils.property(leftLocator, "licence", lhsLicence), LocatorUtils.property(rightLocator, "licence", rhsLicence), lhsLicence, rhsLicence, (leftObject.licence!= null), (rightObject.licence!= null)));
target.setLicence(mergedLicence);
} else {
if (licenceShouldBeMergedAndSet == Boolean.FALSE) {
target.licence = null;
}
}
}
{
Boolean openSourceShouldBeMergedAndSet = strategy.shouldBeMergedAndSet(leftLocator, rightLocator, (leftObject.openSource!= null), (rightObject.openSource!= null));
if (openSourceShouldBeMergedAndSet == Boolean.TRUE) {
Boolean lhsOpenSource;
lhsOpenSource = leftObject.isOpenSource();
Boolean rhsOpenSource;
rhsOpenSource = rightObject.isOpenSource();
Boolean mergedOpenSource = ((Boolean) strategy.merge(LocatorUtils.property(leftLocator, "openSource", lhsOpenSource), LocatorUtils.property(rightLocator, "openSource", rhsOpenSource), lhsOpenSource, rhsOpenSource, (leftObject.openSource!= null), (rightObject.openSource!= null)));
target.setOpenSource(mergedOpenSource);
} else {
if (openSourceShouldBeMergedAndSet == Boolean.FALSE) {
target.openSource = null;
}
}
}
{
Boolean dataFormatsShouldBeMergedAndSet = strategy.shouldBeMergedAndSet(leftLocator, rightLocator, ((leftObject.dataFormats!= null)&&(!leftObject.dataFormats.isEmpty())), ((rightObject.dataFormats!= null)&&(!rightObject.dataFormats.isEmpty())));
if (dataFormatsShouldBeMergedAndSet == Boolean.TRUE) {
List lhsDataFormats;
lhsDataFormats = (((leftObject.dataFormats!= null)&&(!leftObject.dataFormats.isEmpty()))?leftObject.getDataFormats():null);
List rhsDataFormats;
rhsDataFormats = (((rightObject.dataFormats!= null)&&(!rightObject.dataFormats.isEmpty()))?rightObject.getDataFormats():null);
List mergedDataFormats = ((List ) strategy.merge(LocatorUtils.property(leftLocator, "dataFormats", lhsDataFormats), LocatorUtils.property(rightLocator, "dataFormats", rhsDataFormats), lhsDataFormats, rhsDataFormats, ((leftObject.dataFormats!= null)&&(!leftObject.dataFormats.isEmpty())), ((rightObject.dataFormats!= null)&&(!rightObject.dataFormats.isEmpty()))));
target.dataFormats = null;
if (mergedDataFormats!= null) {
List uniqueDataFormatsl = target.getDataFormats();
uniqueDataFormatsl.addAll(mergedDataFormats);
}
} else {
if (dataFormatsShouldBeMergedAndSet == Boolean.FALSE) {
target.dataFormats = null;
}
}
}
{
Boolean voStandardsShouldBeMergedAndSet = strategy.shouldBeMergedAndSet(leftLocator, rightLocator, ((leftObject.voStandards!= null)&&(!leftObject.voStandards.isEmpty())), ((rightObject.voStandards!= null)&&(!rightObject.voStandards.isEmpty())));
if (voStandardsShouldBeMergedAndSet == Boolean.TRUE) {
List lhsVoStandards;
lhsVoStandards = (((leftObject.voStandards!= null)&&(!leftObject.voStandards.isEmpty()))?leftObject.getVoStandards():null);
List rhsVoStandards;
rhsVoStandards = (((rightObject.voStandards!= null)&&(!rightObject.voStandards.isEmpty()))?rightObject.getVoStandards():null);
List mergedVoStandards = ((List ) strategy.merge(LocatorUtils.property(leftLocator, "voStandards", lhsVoStandards), LocatorUtils.property(rightLocator, "voStandards", rhsVoStandards), lhsVoStandards, rhsVoStandards, ((leftObject.voStandards!= null)&&(!leftObject.voStandards.isEmpty())), ((rightObject.voStandards!= null)&&(!rightObject.voStandards.isEmpty()))));
target.voStandards = null;
if (mergedVoStandards!= null) {
List uniqueVoStandardsl = target.getVoStandards();
uniqueVoStandardsl.addAll(mergedVoStandards);
}
} else {
if (voStandardsShouldBeMergedAndSet == Boolean.FALSE) {
target.voStandards = null;
}
}
}
{
Boolean sourceLanguagesShouldBeMergedAndSet = strategy.shouldBeMergedAndSet(leftLocator, rightLocator, ((leftObject.sourceLanguages!= null)&&(!leftObject.sourceLanguages.isEmpty())), ((rightObject.sourceLanguages!= null)&&(!rightObject.sourceLanguages.isEmpty())));
if (sourceLanguagesShouldBeMergedAndSet == Boolean.TRUE) {
List lhsSourceLanguages;
lhsSourceLanguages = (((leftObject.sourceLanguages!= null)&&(!leftObject.sourceLanguages.isEmpty()))?leftObject.getSourceLanguages():null);
List rhsSourceLanguages;
rhsSourceLanguages = (((rightObject.sourceLanguages!= null)&&(!rightObject.sourceLanguages.isEmpty()))?rightObject.getSourceLanguages():null);
List mergedSourceLanguages = ((List ) strategy.merge(LocatorUtils.property(leftLocator, "sourceLanguages", lhsSourceLanguages), LocatorUtils.property(rightLocator, "sourceLanguages", rhsSourceLanguages), lhsSourceLanguages, rhsSourceLanguages, ((leftObject.sourceLanguages!= null)&&(!leftObject.sourceLanguages.isEmpty())), ((rightObject.sourceLanguages!= null)&&(!rightObject.sourceLanguages.isEmpty()))));
target.sourceLanguages = null;
if (mergedSourceLanguages!= null) {
List uniqueSourceLanguagesl = target.getSourceLanguages();
uniqueSourceLanguagesl.addAll(mergedSourceLanguages);
}
} else {
if (sourceLanguagesShouldBeMergedAndSet == Boolean.FALSE) {
target.sourceLanguages = null;
}
}
}
{
Boolean sourceCodeURLShouldBeMergedAndSet = strategy.shouldBeMergedAndSet(leftLocator, rightLocator, (leftObject.sourceCodeURL!= null), (rightObject.sourceCodeURL!= null));
if (sourceCodeURLShouldBeMergedAndSet == Boolean.TRUE) {
String lhsSourceCodeURL;
lhsSourceCodeURL = leftObject.getSourceCodeURL();
String rhsSourceCodeURL;
rhsSourceCodeURL = rightObject.getSourceCodeURL();
String mergedSourceCodeURL = ((String) strategy.merge(LocatorUtils.property(leftLocator, "sourceCodeURL", lhsSourceCodeURL), LocatorUtils.property(rightLocator, "sourceCodeURL", rhsSourceCodeURL), lhsSourceCodeURL, rhsSourceCodeURL, (leftObject.sourceCodeURL!= null), (rightObject.sourceCodeURL!= null)));
target.setSourceCodeURL(mergedSourceCodeURL);
} else {
if (sourceCodeURLShouldBeMergedAndSet == Boolean.FALSE) {
target.sourceCodeURL = null;
}
}
}
}
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Object createNewInstance() {
return new Application();
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Application clone() {
final Application _newObject;
_newObject = ((Application) super.clone());
if (this.dataFormats == null) {
_newObject.dataFormats = null;
} else {
_newObject.dataFormats = new ArrayList<>();
for (DataFormat _item: this.dataFormats) {
_newObject.dataFormats.add(((_item == null)?null:_item.clone()));
}
}
if (this.voStandards == null) {
_newObject.voStandards = null;
} else {
_newObject.voStandards = new ArrayList<>();
for (ApplicationCapability _item: this.voStandards) {
_newObject.voStandards.add(((_item == null)?null:_item.clone()));
}
}
_newObject.sourceLanguages = ((this.sourceLanguages == null)?null:new ArrayList<>(this.sourceLanguages));
return _newObject;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Application createCopy() {
final Application _newObject = ((Application) super.createCopy());
_newObject.cost = this.cost;
_newObject.licence = this.licence;
_newObject.openSource = this.openSource;
if (this.dataFormats == null) {
_newObject.dataFormats = null;
} else {
_newObject.dataFormats = new ArrayList<>();
for (DataFormat _item: this.dataFormats) {
_newObject.dataFormats.add(((_item == null)?null:_item.createCopy()));
}
}
if (this.voStandards == null) {
_newObject.voStandards = null;
} else {
_newObject.voStandards = new ArrayList<>();
for (ApplicationCapability _item: this.voStandards) {
_newObject.voStandards.add(((_item == null)?null:_item.createCopy()));
}
}
_newObject.sourceLanguages = ((this.sourceLanguages == null)?null:new ArrayList<>(this.sourceLanguages));
_newObject.sourceCodeURL = this.sourceCodeURL;
return _newObject;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Application createCopy(final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
final Application _newObject = ((Application) super.createCopy(_propertyTree, _propertyTreeUse));
final PropertyTree costPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("cost"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(costPropertyTree!= null):((costPropertyTree == null)||(!costPropertyTree.isLeaf())))) {
_newObject.cost = this.cost;
}
final PropertyTree licencePropertyTree = ((_propertyTree == null)?null:_propertyTree.get("licence"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(licencePropertyTree!= null):((licencePropertyTree == null)||(!licencePropertyTree.isLeaf())))) {
_newObject.licence = this.licence;
}
final PropertyTree openSourcePropertyTree = ((_propertyTree == null)?null:_propertyTree.get("openSource"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(openSourcePropertyTree!= null):((openSourcePropertyTree == null)||(!openSourcePropertyTree.isLeaf())))) {
_newObject.openSource = this.openSource;
}
final PropertyTree dataFormatsPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("dataFormats"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(dataFormatsPropertyTree!= null):((dataFormatsPropertyTree == null)||(!dataFormatsPropertyTree.isLeaf())))) {
if (this.dataFormats == null) {
_newObject.dataFormats = null;
} else {
_newObject.dataFormats = new ArrayList<>();
for (DataFormat _item: this.dataFormats) {
_newObject.dataFormats.add(((_item == null)?null:_item.createCopy(dataFormatsPropertyTree, _propertyTreeUse)));
}
}
}
final PropertyTree voStandardsPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("voStandards"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(voStandardsPropertyTree!= null):((voStandardsPropertyTree == null)||(!voStandardsPropertyTree.isLeaf())))) {
if (this.voStandards == null) {
_newObject.voStandards = null;
} else {
_newObject.voStandards = new ArrayList<>();
for (ApplicationCapability _item: this.voStandards) {
_newObject.voStandards.add(((_item == null)?null:_item.createCopy(voStandardsPropertyTree, _propertyTreeUse)));
}
}
}
final PropertyTree sourceLanguagesPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("sourceLanguages"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(sourceLanguagesPropertyTree!= null):((sourceLanguagesPropertyTree == null)||(!sourceLanguagesPropertyTree.isLeaf())))) {
_newObject.sourceLanguages = ((this.sourceLanguages == null)?null:new ArrayList<>(this.sourceLanguages));
}
final PropertyTree sourceCodeURLPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("sourceCodeURL"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(sourceCodeURLPropertyTree!= null):((sourceCodeURLPropertyTree == null)||(!sourceCodeURLPropertyTree.isLeaf())))) {
_newObject.sourceCodeURL = this.sourceCodeURL;
}
return _newObject;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Application copyExcept(final PropertyTree _propertyTree) {
return createCopy(_propertyTree, PropertyTreeUse.EXCLUDE);
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Application copyOnly(final PropertyTree _propertyTree) {
return createCopy(_propertyTree, PropertyTreeUse.INCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Application.Modifier modifier() {
if (null == this.__cachedModifier__) {
this.__cachedModifier__ = new Application.Modifier();
}
return ((Application.Modifier) this.__cachedModifier__);
}
/**
* Copies all state of this object to a builder. This method is used by the copyOf
* method and should not be called directly by client code.
*
* @param _other
* A builder instance to which the state of this object will be copied.
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public<_B >void copyTo(final Application.Builder<_B> _other) {
super.copyTo(_other);
_other.cost = this.cost;
_other.licence = this.licence;
_other.openSource = this.openSource;
if (this.dataFormats == null) {
_other.dataFormats = null;
} else {
_other.dataFormats = new ArrayList<>();
for (DataFormat _item: this.dataFormats) {
_other.dataFormats.add(((_item == null)?null:_item.newCopyBuilder(_other)));
}
}
if (this.voStandards == null) {
_other.voStandards = null;
} else {
_other.voStandards = new ArrayList<>();
for (ApplicationCapability _item: this.voStandards) {
_other.voStandards.add(((_item == null)?null:_item.newCopyBuilder(_other)));
}
}
if (this.sourceLanguages == null) {
_other.sourceLanguages = null;
} else {
_other.sourceLanguages = new ArrayList<>();
for (String _item: this.sourceLanguages) {
_other.sourceLanguages.add(((_item == null)?null:new Buildable.PrimitiveBuildable(_item)));
}
}
_other.sourceCodeURL = this.sourceCodeURL;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public<_B >Application.Builder<_B> newCopyBuilder(final _B _parentBuilder) {
return new Application.Builder<_B>(_parentBuilder, this, true);
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Application.Builder newCopyBuilder() {
return newCopyBuilder(null);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static Application.Builder builder() {
return new Application.Builder<>(null, null, false);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static<_B >Application.Builder<_B> copyOf(final Resource _other) {
final Application.Builder<_B> _newBuilder = new Application.Builder<>(null, null, false);
_other.copyTo(_newBuilder);
return _newBuilder;
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static<_B >Application.Builder<_B> copyOf(final Application _other) {
final Application.Builder<_B> _newBuilder = new Application.Builder<>(null, null, false);
_other.copyTo(_newBuilder);
return _newBuilder;
}
/**
* Copies all state of this object to a builder. This method is used by the copyOf
* method and should not be called directly by client code.
*
* @param _other
* A builder instance to which the state of this object will be copied.
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public<_B >void copyTo(final Application.Builder<_B> _other, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
super.copyTo(_other, _propertyTree, _propertyTreeUse);
final PropertyTree costPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("cost"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(costPropertyTree!= null):((costPropertyTree == null)||(!costPropertyTree.isLeaf())))) {
_other.cost = this.cost;
}
final PropertyTree licencePropertyTree = ((_propertyTree == null)?null:_propertyTree.get("licence"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(licencePropertyTree!= null):((licencePropertyTree == null)||(!licencePropertyTree.isLeaf())))) {
_other.licence = this.licence;
}
final PropertyTree openSourcePropertyTree = ((_propertyTree == null)?null:_propertyTree.get("openSource"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(openSourcePropertyTree!= null):((openSourcePropertyTree == null)||(!openSourcePropertyTree.isLeaf())))) {
_other.openSource = this.openSource;
}
final PropertyTree dataFormatsPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("dataFormats"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(dataFormatsPropertyTree!= null):((dataFormatsPropertyTree == null)||(!dataFormatsPropertyTree.isLeaf())))) {
if (this.dataFormats == null) {
_other.dataFormats = null;
} else {
_other.dataFormats = new ArrayList<>();
for (DataFormat _item: this.dataFormats) {
_other.dataFormats.add(((_item == null)?null:_item.newCopyBuilder(_other, dataFormatsPropertyTree, _propertyTreeUse)));
}
}
}
final PropertyTree voStandardsPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("voStandards"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(voStandardsPropertyTree!= null):((voStandardsPropertyTree == null)||(!voStandardsPropertyTree.isLeaf())))) {
if (this.voStandards == null) {
_other.voStandards = null;
} else {
_other.voStandards = new ArrayList<>();
for (ApplicationCapability _item: this.voStandards) {
_other.voStandards.add(((_item == null)?null:_item.newCopyBuilder(_other, voStandardsPropertyTree, _propertyTreeUse)));
}
}
}
final PropertyTree sourceLanguagesPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("sourceLanguages"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(sourceLanguagesPropertyTree!= null):((sourceLanguagesPropertyTree == null)||(!sourceLanguagesPropertyTree.isLeaf())))) {
if (this.sourceLanguages == null) {
_other.sourceLanguages = null;
} else {
_other.sourceLanguages = new ArrayList<>();
for (String _item: this.sourceLanguages) {
_other.sourceLanguages.add(((_item == null)?null:new Buildable.PrimitiveBuildable(_item)));
}
}
}
final PropertyTree sourceCodeURLPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("sourceCodeURL"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(sourceCodeURLPropertyTree!= null):((sourceCodeURLPropertyTree == null)||(!sourceCodeURLPropertyTree.isLeaf())))) {
_other.sourceCodeURL = this.sourceCodeURL;
}
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public<_B >Application.Builder<_B> newCopyBuilder(final _B _parentBuilder, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
return new Application.Builder<_B>(_parentBuilder, this, true, _propertyTree, _propertyTreeUse);
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Application.Builder newCopyBuilder(final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
return newCopyBuilder(null, _propertyTree, _propertyTreeUse);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static<_B >Application.Builder<_B> copyOf(final Resource _other, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
final Application.Builder<_B> _newBuilder = new Application.Builder<>(null, null, false);
_other.copyTo(_newBuilder, _propertyTree, _propertyTreeUse);
return _newBuilder;
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static<_B >Application.Builder<_B> copyOf(final Application _other, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
final Application.Builder<_B> _newBuilder = new Application.Builder<>(null, null, false);
_other.copyTo(_newBuilder, _propertyTree, _propertyTreeUse);
return _newBuilder;
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static Application.Builder copyExcept(final Resource _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.EXCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static Application.Builder copyExcept(final Application _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.EXCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static Application.Builder copyOnly(final Resource _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.INCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static Application.Builder copyOnly(final Application _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.INCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Application visit(final PropertyVisitor _visitor_) {
super.visit(_visitor_);
_visitor_.visit(new SingleProperty<>(Application.PropInfo.COST, this));
_visitor_.visit(new SingleProperty<>(Application.PropInfo.LICENCE, this));
_visitor_.visit(new SingleProperty<>(Application.PropInfo.OPEN_SOURCE, this));
if (_visitor_.visit(new CollectionProperty<>(Application.PropInfo.DATA_FORMATS, this))&&(this.dataFormats!= null)) {
for (DataFormat _item_: this.dataFormats) {
if (_item_!= null) {
_item_.visit(_visitor_);
}
}
}
if (_visitor_.visit(new CollectionProperty<>(Application.PropInfo.VO_STANDARDS, this))&&(this.voStandards!= null)) {
for (ApplicationCapability _item_: this.voStandards) {
if (_item_!= null) {
_item_.visit(_visitor_);
}
}
}
_visitor_.visit(new CollectionProperty<>(Application.PropInfo.SOURCE_LANGUAGES, this));
_visitor_.visit(new SingleProperty<>(Application.PropInfo.SOURCE_CODE_URL, this));
return this;
}
public static class Builder<_B >
extends Resource.Builder<_B>
implements Buildable
{
private String cost;
private String licence;
private Boolean openSource;
private List>> dataFormats;
private List>> voStandards;
private List sourceLanguages;
private String sourceCodeURL;
public Builder(final _B _parentBuilder, final Application _other, final boolean _copy) {
super(_parentBuilder, _other, _copy);
if (_other!= null) {
this.cost = _other.cost;
this.licence = _other.licence;
this.openSource = _other.openSource;
if (_other.dataFormats == null) {
this.dataFormats = null;
} else {
this.dataFormats = new ArrayList<>();
for (DataFormat _item: _other.dataFormats) {
this.dataFormats.add(((_item == null)?null:_item.newCopyBuilder(this)));
}
}
if (_other.voStandards == null) {
this.voStandards = null;
} else {
this.voStandards = new ArrayList<>();
for (ApplicationCapability _item: _other.voStandards) {
this.voStandards.add(((_item == null)?null:_item.newCopyBuilder(this)));
}
}
if (_other.sourceLanguages == null) {
this.sourceLanguages = null;
} else {
this.sourceLanguages = new ArrayList<>();
for (String _item: _other.sourceLanguages) {
this.sourceLanguages.add(((_item == null)?null:new Buildable.PrimitiveBuildable(_item)));
}
}
this.sourceCodeURL = _other.sourceCodeURL;
}
}
public Builder(final _B _parentBuilder, final Application _other, final boolean _copy, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
super(_parentBuilder, _other, _copy, _propertyTree, _propertyTreeUse);
if (_other!= null) {
final PropertyTree costPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("cost"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(costPropertyTree!= null):((costPropertyTree == null)||(!costPropertyTree.isLeaf())))) {
this.cost = _other.cost;
}
final PropertyTree licencePropertyTree = ((_propertyTree == null)?null:_propertyTree.get("licence"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(licencePropertyTree!= null):((licencePropertyTree == null)||(!licencePropertyTree.isLeaf())))) {
this.licence = _other.licence;
}
final PropertyTree openSourcePropertyTree = ((_propertyTree == null)?null:_propertyTree.get("openSource"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(openSourcePropertyTree!= null):((openSourcePropertyTree == null)||(!openSourcePropertyTree.isLeaf())))) {
this.openSource = _other.openSource;
}
final PropertyTree dataFormatsPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("dataFormats"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(dataFormatsPropertyTree!= null):((dataFormatsPropertyTree == null)||(!dataFormatsPropertyTree.isLeaf())))) {
if (_other.dataFormats == null) {
this.dataFormats = null;
} else {
this.dataFormats = new ArrayList<>();
for (DataFormat _item: _other.dataFormats) {
this.dataFormats.add(((_item == null)?null:_item.newCopyBuilder(this, dataFormatsPropertyTree, _propertyTreeUse)));
}
}
}
final PropertyTree voStandardsPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("voStandards"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(voStandardsPropertyTree!= null):((voStandardsPropertyTree == null)||(!voStandardsPropertyTree.isLeaf())))) {
if (_other.voStandards == null) {
this.voStandards = null;
} else {
this.voStandards = new ArrayList<>();
for (ApplicationCapability _item: _other.voStandards) {
this.voStandards.add(((_item == null)?null:_item.newCopyBuilder(this, voStandardsPropertyTree, _propertyTreeUse)));
}
}
}
final PropertyTree sourceLanguagesPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("sourceLanguages"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(sourceLanguagesPropertyTree!= null):((sourceLanguagesPropertyTree == null)||(!sourceLanguagesPropertyTree.isLeaf())))) {
if (_other.sourceLanguages == null) {
this.sourceLanguages = null;
} else {
this.sourceLanguages = new ArrayList<>();
for (String _item: _other.sourceLanguages) {
this.sourceLanguages.add(((_item == null)?null:new Buildable.PrimitiveBuildable(_item)));
}
}
}
final PropertyTree sourceCodeURLPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("sourceCodeURL"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(sourceCodeURLPropertyTree!= null):((sourceCodeURLPropertyTree == null)||(!sourceCodeURLPropertyTree.isLeaf())))) {
this.sourceCodeURL = _other.sourceCodeURL;
}
}
}
protected<_P extends Application >_P init(final _P _product) {
_product.cost = this.cost;
_product.licence = this.licence;
_product.openSource = this.openSource;
if (this.dataFormats!= null) {
final List dataFormats = new ArrayList<>(this.dataFormats.size());
for (DataFormat.Builder> _item: this.dataFormats) {
dataFormats.add(_item.build());
}
_product.dataFormats = dataFormats;
}
if (this.voStandards!= null) {
final List voStandards = new ArrayList<>(this.voStandards.size());
for (ApplicationCapability.Builder> _item: this.voStandards) {
voStandards.add(_item.build());
}
_product.voStandards = voStandards;
}
if (this.sourceLanguages!= null) {
final List sourceLanguages = new ArrayList<>(this.sourceLanguages.size());
for (Buildable _item: this.sourceLanguages) {
sourceLanguages.add(((String) _item.build()));
}
_product.sourceLanguages = sourceLanguages;
}
_product.sourceCodeURL = this.sourceCodeURL;
return super.init(_product);
}
/**
* Sets the new value of "cost" (any previous value will be replaced)
*
* @param cost
* New value of the "cost" property.
*/
public Application.Builder<_B> withCost(final String cost) {
this.cost = cost;
return this;
}
/**
* Sets the new value of "licence" (any previous value will be replaced)
*
* @param licence
* New value of the "licence" property.
*/
public Application.Builder<_B> withLicence(final String licence) {
this.licence = licence;
return this;
}
/**
* Sets the new value of "openSource" (any previous value will be replaced)
*
* @param openSource
* New value of the "openSource" property.
*/
public Application.Builder<_B> withOpenSource(final Boolean openSource) {
this.openSource = openSource;
return this;
}
/**
* Adds the given items to the value of "dataFormats"
*
* @param dataFormats
* Items to add to the value of the "dataFormats" property
*/
public Application.Builder<_B> addDataFormats(final Iterable extends DataFormat> dataFormats) {
if (dataFormats!= null) {
if (this.dataFormats == null) {
this.dataFormats = new ArrayList<>();
}
for (DataFormat _item: dataFormats) {
this.dataFormats.add(new DataFormat.Builder<>(this, _item, false));
}
}
return this;
}
/**
* Sets the new value of "dataFormats" (any previous value will be replaced)
*
* @param dataFormats
* New value of the "dataFormats" property.
*/
public Application.Builder<_B> withDataFormats(final Iterable extends DataFormat> dataFormats) {
if (this.dataFormats!= null) {
this.dataFormats.clear();
}
return addDataFormats(dataFormats);
}
/**
* Adds the given items to the value of "dataFormats"
*
* @param dataFormats
* Items to add to the value of the "dataFormats" property
*/
public Application.Builder<_B> addDataFormats(DataFormat... dataFormats) {
addDataFormats(Arrays.asList(dataFormats));
return this;
}
/**
* Sets the new value of "dataFormats" (any previous value will be replaced)
*
* @param dataFormats
* New value of the "dataFormats" property.
*/
public Application.Builder<_B> withDataFormats(DataFormat... dataFormats) {
withDataFormats(Arrays.asList(dataFormats));
return this;
}
/**
* Returns a new builder to build an additional value of the "DataFormats"
* property.
* Use {@link
* org.javastro.ivoa.entities.resource.applications.DataFormat.Builder#end()} to
* return to the current builder.
*
* @return
* a new builder to build an additional value of the "DataFormats" property.
* Use {@link
* org.javastro.ivoa.entities.resource.applications.DataFormat.Builder#end()} to
* return to the current builder.
*/
public DataFormat.Builder extends Application.Builder<_B>> addDataFormats() {
if (this.dataFormats == null) {
this.dataFormats = new ArrayList<>();
}
final DataFormat.Builder> dataFormats_Builder = new DataFormat.Builder<>(this, null, false);
this.dataFormats.add(dataFormats_Builder);
return dataFormats_Builder;
}
/**
* Adds the given items to the value of "voStandards"
*
* @param voStandards
* Items to add to the value of the "voStandards" property
*/
public Application.Builder<_B> addVoStandards(final Iterable extends ApplicationCapability> voStandards) {
if (voStandards!= null) {
if (this.voStandards == null) {
this.voStandards = new ArrayList<>();
}
for (ApplicationCapability _item: voStandards) {
this.voStandards.add(new ApplicationCapability.Builder<>(this, _item, false));
}
}
return this;
}
/**
* Sets the new value of "voStandards" (any previous value will be replaced)
*
* @param voStandards
* New value of the "voStandards" property.
*/
public Application.Builder<_B> withVoStandards(final Iterable extends ApplicationCapability> voStandards) {
if (this.voStandards!= null) {
this.voStandards.clear();
}
return addVoStandards(voStandards);
}
/**
* Adds the given items to the value of "voStandards"
*
* @param voStandards
* Items to add to the value of the "voStandards" property
*/
public Application.Builder<_B> addVoStandards(ApplicationCapability... voStandards) {
addVoStandards(Arrays.asList(voStandards));
return this;
}
/**
* Sets the new value of "voStandards" (any previous value will be replaced)
*
* @param voStandards
* New value of the "voStandards" property.
*/
public Application.Builder<_B> withVoStandards(ApplicationCapability... voStandards) {
withVoStandards(Arrays.asList(voStandards));
return this;
}
/**
* Returns a new builder to build an additional value of the "VoStandards"
* property.
* Use {@link
* org.javastro.ivoa.entities.resource.applications.ApplicationCapability.Builder#end()}
* to return to the current builder.
*
* @return
* a new builder to build an additional value of the "VoStandards" property.
* Use {@link
* org.javastro.ivoa.entities.resource.applications.ApplicationCapability.Builder#end()}
* to return to the current builder.
*/
public ApplicationCapability.Builder extends Application.Builder<_B>> addVoStandards() {
if (this.voStandards == null) {
this.voStandards = new ArrayList<>();
}
final ApplicationCapability.Builder> voStandards_Builder = new ApplicationCapability.Builder<>(this, null, false);
this.voStandards.add(voStandards_Builder);
return voStandards_Builder;
}
/**
* Adds the given items to the value of "sourceLanguages"
*
* @param sourceLanguages
* Items to add to the value of the "sourceLanguages" property
*/
public Application.Builder<_B> addSourceLanguages(final Iterable extends String> sourceLanguages) {
if (sourceLanguages!= null) {
if (this.sourceLanguages == null) {
this.sourceLanguages = new ArrayList<>();
}
for (String _item: sourceLanguages) {
this.sourceLanguages.add(new Buildable.PrimitiveBuildable(_item));
}
}
return this;
}
/**
* Sets the new value of "sourceLanguages" (any previous value will be replaced)
*
* @param sourceLanguages
* New value of the "sourceLanguages" property.
*/
public Application.Builder<_B> withSourceLanguages(final Iterable extends String> sourceLanguages) {
if (this.sourceLanguages!= null) {
this.sourceLanguages.clear();
}
return addSourceLanguages(sourceLanguages);
}
/**
* Adds the given items to the value of "sourceLanguages"
*
* @param sourceLanguages
* Items to add to the value of the "sourceLanguages" property
*/
public Application.Builder<_B> addSourceLanguages(String... sourceLanguages) {
addSourceLanguages(Arrays.asList(sourceLanguages));
return this;
}
/**
* Sets the new value of "sourceLanguages" (any previous value will be replaced)
*
* @param sourceLanguages
* New value of the "sourceLanguages" property.
*/
public Application.Builder<_B> withSourceLanguages(String... sourceLanguages) {
withSourceLanguages(Arrays.asList(sourceLanguages));
return this;
}
/**
* Sets the new value of "sourceCodeURL" (any previous value will be replaced)
*
* @param sourceCodeURL
* New value of the "sourceCodeURL" property.
*/
public Application.Builder<_B> withSourceCodeURL(final String sourceCodeURL) {
this.sourceCodeURL = sourceCodeURL;
return this;
}
/**
* Adds the given items to the value of "validationLevels"
*
* @param validationLevels
* Items to add to the value of the "validationLevels" property
*/
@Override
public Application.Builder<_B> addValidationLevels(final Iterable extends Validation> validationLevels) {
super.addValidationLevels(validationLevels);
return this;
}
/**
* Adds the given items to the value of "validationLevels"
*
* @param validationLevels
* Items to add to the value of the "validationLevels" property
*/
@Override
public Application.Builder<_B> addValidationLevels(Validation... validationLevels) {
super.addValidationLevels(validationLevels);
return this;
}
/**
* Sets the new value of "validationLevels" (any previous value will be replaced)
*
* @param validationLevels
* New value of the "validationLevels" property.
*/
@Override
public Application.Builder<_B> withValidationLevels(final Iterable extends Validation> validationLevels) {
super.withValidationLevels(validationLevels);
return this;
}
/**
* Sets the new value of "validationLevels" (any previous value will be replaced)
*
* @param validationLevels
* New value of the "validationLevels" property.
*/
@Override
public Application.Builder<_B> withValidationLevels(Validation... validationLevels) {
super.withValidationLevels(validationLevels);
return this;
}
/**
* Sets the new value of "title" (any previous value will be replaced)
*
* @param title
* New value of the "title" property.
*/
@Override
public Application.Builder<_B> withTitle(final String title) {
super.withTitle(title);
return this;
}
/**
* Sets the new value of "shortName" (any previous value will be replaced)
*
* @param shortName
* New value of the "shortName" property.
*/
@Override
public Application.Builder<_B> withShortName(final String shortName) {
super.withShortName(shortName);
return this;
}
/**
* Sets the new value of "identifier" (any previous value will be replaced)
*
* @param identifier
* New value of the "identifier" property.
*/
@Override
public Application.Builder<_B> withIdentifier(final String identifier) {
super.withIdentifier(identifier);
return this;
}
/**
* Adds the given items to the value of "altIdentifiers"
*
* @param altIdentifiers
* Items to add to the value of the "altIdentifiers" property
*/
@Override
public Application.Builder<_B> addAltIdentifiers(final Iterable extends String> altIdentifiers) {
super.addAltIdentifiers(altIdentifiers);
return this;
}
/**
* Adds the given items to the value of "altIdentifiers"
*
* @param altIdentifiers
* Items to add to the value of the "altIdentifiers" property
*/
@Override
public Application.Builder<_B> addAltIdentifiers(String... altIdentifiers) {
super.addAltIdentifiers(altIdentifiers);
return this;
}
/**
* Sets the new value of "altIdentifiers" (any previous value will be replaced)
*
* @param altIdentifiers
* New value of the "altIdentifiers" property.
*/
@Override
public Application.Builder<_B> withAltIdentifiers(final Iterable extends String> altIdentifiers) {
super.withAltIdentifiers(altIdentifiers);
return this;
}
/**
* Sets the new value of "altIdentifiers" (any previous value will be replaced)
*
* @param altIdentifiers
* New value of the "altIdentifiers" property.
*/
@Override
public Application.Builder<_B> withAltIdentifiers(String... altIdentifiers) {
super.withAltIdentifiers(altIdentifiers);
return this;
}
/**
* Sets the new value of "curation" (any previous value will be replaced)
*
* @param curation
* New value of the "curation" property.
*/
@Override
public Application.Builder<_B> withCuration(final Curation curation) {
super.withCuration(curation);
return this;
}
/**
* Returns the existing builder or a new builder to build the value of the
* "curation" property.
* Use {@link org.javastro.ivoa.entities.resource.Curation.Builder#end()} to return
* to the current builder.
*
* @return
* A new builder to build the value of the "curation" property.
* Use {@link org.javastro.ivoa.entities.resource.Curation.Builder#end()} to return
* to the current builder.
*/
public Curation.Builder extends Application.Builder<_B>> withCuration() {
return ((Curation.Builder extends Application.Builder<_B>> ) super.withCuration());
}
/**
* Sets the new value of "content" (any previous value will be replaced)
*
* @param content
* New value of the "content" property.
*/
@Override
public Application.Builder<_B> withContent(final Content content) {
super.withContent(content);
return this;
}
/**
* Returns the existing builder or a new builder to build the value of the
* "content" property.
* Use {@link org.javastro.ivoa.entities.resource.Content.Builder#end()} to return
* to the current builder.
*
* @return
* A new builder to build the value of the "content" property.
* Use {@link org.javastro.ivoa.entities.resource.Content.Builder#end()} to return
* to the current builder.
*/
public Content.Builder extends Application.Builder<_B>> withContent() {
return ((Content.Builder extends Application.Builder<_B>> ) super.withContent());
}
/**
* Sets the new value of "created" (any previous value will be replaced)
*
* @param created
* New value of the "created" property.
*/
@Override
public Application.Builder<_B> withCreated(final LocalDateTime created) {
super.withCreated(created);
return this;
}
/**
* Sets the new value of "updated" (any previous value will be replaced)
*
* @param updated
* New value of the "updated" property.
*/
@Override
public Application.Builder<_B> withUpdated(final LocalDateTime updated) {
super.withUpdated(updated);
return this;
}
/**
* Sets the new value of "status" (any previous value will be replaced)
*
* @param status
* New value of the "status" property.
*/
@Override
public Application.Builder<_B> withStatus(final String status) {
super.withStatus(status);
return this;
}
/**
* Sets the new value of "version" (any previous value will be replaced)
*
* @param version
* New value of the "version" property.
*/
@Override
public Application.Builder<_B> withVersion(final String version) {
super.withVersion(version);
return this;
}
@Override
public Application build() {
if (_storedValue == null) {
return this.init(new Application());
} else {
return ((Application) _storedValue);
}
}
public Application.Builder<_B> copyOf(final Application _other) {
_other.copyTo(this);
return this;
}
public Application.Builder<_B> copyOf(final Application.Builder _other) {
return copyOf(_other.build());
}
}
public class Modifier
extends Resource.Modifier
{
public void setCost(final String cost) {
Application.this.setCost(cost);
}
public void setLicence(final String licence) {
Application.this.setLicence(licence);
}
public void setOpenSource(final Boolean openSource) {
Application.this.setOpenSource(openSource);
}
public List getDataFormats() {
if (Application.this.dataFormats == null) {
Application.this.dataFormats = new ArrayList<>();
}
return Application.this.dataFormats;
}
public List getVoStandards() {
if (Application.this.voStandards == null) {
Application.this.voStandards = new ArrayList<>();
}
return Application.this.voStandards;
}
public List getSourceLanguages() {
if (Application.this.sourceLanguages == null) {
Application.this.sourceLanguages = new ArrayList<>();
}
return Application.this.sourceLanguages;
}
public void setSourceCodeURL(final String sourceCodeURL) {
Application.this.setSourceCodeURL(sourceCodeURL);
}
}
public static class PropInfo {
public static final transient SinglePropertyInfo COST = new SinglePropertyInfo("cost", Application.class, String.class, false, null, new QName("", "cost"), new QName("http://www.w3.org/2001/XMLSchema", "string"), false) {
@Override
public String get(final Application _instance_) {
return ((_instance_ == null)?null:_instance_.cost);
}
@Override
public void set(final Application _instance_, final String _value_) {
if (_instance_!= null) {
_instance_.cost = _value_;
}
}
}
;
public static final transient SinglePropertyInfo LICENCE = new SinglePropertyInfo("licence", Application.class, String.class, false, null, new QName("", "licence"), new QName("http://www.w3.org/2001/XMLSchema", "string"), false) {
@Override
public String get(final Application _instance_) {
return ((_instance_ == null)?null:_instance_.licence);
}
@Override
public void set(final Application _instance_, final String _value_) {
if (_instance_!= null) {
_instance_.licence = _value_;
}
}
}
;
public static final transient SinglePropertyInfo OPEN_SOURCE = new SinglePropertyInfo("openSource", Application.class, Boolean.class, false, null, new QName("", "openSource"), new QName("http://www.w3.org/2001/XMLSchema", "boolean"), false) {
@Override
public Boolean get(final Application _instance_) {
return ((_instance_ == null)?null:_instance_.openSource);
}
@Override
public void set(final Application _instance_, final Boolean _value_) {
if (_instance_!= null) {
_instance_.openSource = _value_;
}
}
}
;
public static final transient CollectionPropertyInfo DATA_FORMATS = new CollectionPropertyInfo("dataFormats", Application.class, DataFormat.class, true, null, new QName("", "dataFormat"), new QName("http://www.ivoa.net/xml/VOApplication/v1.0rc1", "DataFormat"), false) {
@Override
public List get(final Application _instance_) {
return ((_instance_ == null)?null:_instance_.dataFormats);
}
@Override
public void set(final Application _instance_, final List _value_) {
if (_instance_!= null) {
_instance_.dataFormats = _value_;
}
}
}
;
public static final transient CollectionPropertyInfo VO_STANDARDS = new CollectionPropertyInfo("voStandards", Application.class, ApplicationCapability.class, true, null, new QName("", "voStandard"), new QName("http://www.ivoa.net/xml/VOApplication/v1.0rc1", "ApplicationCapability"), false) {
@Override
public List get(final Application _instance_) {
return ((_instance_ == null)?null:_instance_.voStandards);
}
@Override
public void set(final Application _instance_, final List _value_) {
if (_instance_!= null) {
_instance_.voStandards = _value_;
}
}
}
;
public static final transient CollectionPropertyInfo SOURCE_LANGUAGES = new CollectionPropertyInfo("sourceLanguages", Application.class, String.class, true, null, new QName("", "sourceLanguage"), new QName("http://www.ivoa.net/xml/VOApplication/v1.0rc1", "ProgrammingLanguage"), false) {
@Override
public List get(final Application _instance_) {
return ((_instance_ == null)?null:_instance_.sourceLanguages);
}
@Override
public void set(final Application _instance_, final List _value_) {
if (_instance_!= null) {
_instance_.sourceLanguages = _value_;
}
}
}
;
public static final transient SinglePropertyInfo SOURCE_CODE_URL = new SinglePropertyInfo("sourceCodeURL", Application.class, String.class, false, null, new QName("", "sourceCodeURL"), new QName("http://www.w3.org/2001/XMLSchema", "anyURI"), false) {
@Override
public String get(final Application _instance_) {
return ((_instance_ == null)?null:_instance_.sourceCodeURL);
}
@Override
public void set(final Application _instance_, final String _value_) {
if (_instance_!= null) {
_instance_.sourceCodeURL = _value_;
}
}
}
;
}
public static class Select
extends Application.Selector
{
Select() {
super(null, null, null);
}
public static Application.Select _root() {
return new Application.Select();
}
}
public static class Selector , TParent >
extends Resource.Selector
{
private com.kscs.util.jaxb.Selector> cost = null;
private com.kscs.util.jaxb.Selector> licence = null;
private com.kscs.util.jaxb.Selector> openSource = null;
private DataFormat.Selector> dataFormats = null;
private ApplicationCapability.Selector> voStandards = null;
private com.kscs.util.jaxb.Selector> sourceLanguages = null;
private com.kscs.util.jaxb.Selector> sourceCodeURL = null;
public Selector(final TRoot root, final TParent parent, final String propertyName) {
super(root, parent, propertyName);
}
@Override
public Map buildChildren() {
final Map products = new HashMap<>();
products.putAll(super.buildChildren());
if (this.cost!= null) {
products.put("cost", this.cost.init());
}
if (this.licence!= null) {
products.put("licence", this.licence.init());
}
if (this.openSource!= null) {
products.put("openSource", this.openSource.init());
}
if (this.dataFormats!= null) {
products.put("dataFormats", this.dataFormats.init());
}
if (this.voStandards!= null) {
products.put("voStandards", this.voStandards.init());
}
if (this.sourceLanguages!= null) {
products.put("sourceLanguages", this.sourceLanguages.init());
}
if (this.sourceCodeURL!= null) {
products.put("sourceCodeURL", this.sourceCodeURL.init());
}
return products;
}
public com.kscs.util.jaxb.Selector> cost() {
return ((this.cost == null)?this.cost = new com.kscs.util.jaxb.Selector<>(this._root, this, "cost"):this.cost);
}
public com.kscs.util.jaxb.Selector> licence() {
return ((this.licence == null)?this.licence = new com.kscs.util.jaxb.Selector<>(this._root, this, "licence"):this.licence);
}
public com.kscs.util.jaxb.Selector> openSource() {
return ((this.openSource == null)?this.openSource = new com.kscs.util.jaxb.Selector<>(this._root, this, "openSource"):this.openSource);
}
public DataFormat.Selector> dataFormats() {
return ((this.dataFormats == null)?this.dataFormats = new DataFormat.Selector<>(this._root, this, "dataFormats"):this.dataFormats);
}
public ApplicationCapability.Selector> voStandards() {
return ((this.voStandards == null)?this.voStandards = new ApplicationCapability.Selector<>(this._root, this, "voStandards"):this.voStandards);
}
public com.kscs.util.jaxb.Selector> sourceLanguages() {
return ((this.sourceLanguages == null)?this.sourceLanguages = new com.kscs.util.jaxb.Selector<>(this._root, this, "sourceLanguages"):this.sourceLanguages);
}
public com.kscs.util.jaxb.Selector> sourceCodeURL() {
return ((this.sourceCodeURL == null)?this.sourceCodeURL = new com.kscs.util.jaxb.Selector<>(this._root, this, "sourceCodeURL"):this.sourceCodeURL);
}
}
}