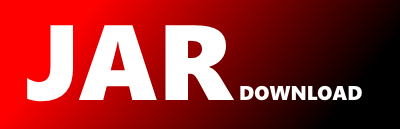
org.javastro.ivoa.entities.resource.applications.ExecutionEnvironment Maven / Gradle / Ivy
package org.javastro.ivoa.entities.resource.applications;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import javax.xml.namespace.QName;
import com.kscs.util.jaxb.Buildable;
import com.kscs.util.jaxb.CollectionProperty;
import com.kscs.util.jaxb.CollectionPropertyInfo;
import com.kscs.util.jaxb.CollectionPropertyInfo;
import com.kscs.util.jaxb.Copyable;
import com.kscs.util.jaxb.PartialCopyable;
import com.kscs.util.jaxb.PropertyTree;
import com.kscs.util.jaxb.PropertyTreeUse;
import com.kscs.util.jaxb.PropertyVisitor;
import com.kscs.util.jaxb.SingleProperty;
import com.kscs.util.jaxb.SinglePropertyInfo;
import com.kscs.util.jaxb.SinglePropertyInfo;
import jakarta.annotation.Generated;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlSchemaType;
import jakarta.xml.bind.annotation.XmlType;
import org.jvnet.jaxb.lang.JAXBMergeStrategy;
import org.jvnet.jaxb.lang.JAXBToStringStrategy;
import org.jvnet.jaxb.lang.MergeFrom;
import org.jvnet.jaxb.lang.MergeStrategy;
import org.jvnet.jaxb.lang.ToString;
import org.jvnet.jaxb.lang.ToStringStrategy;
import org.jvnet.jaxb.locator.ObjectLocator;
import org.jvnet.jaxb.locator.util.LocatorUtils;
/**
* Description of the full execution environment. Where
* possible this description should be sufficient to download
* and run the application.
*
* Java class for ExecutionEnvironment complex type
.
*
* The following schema fragment specifies the expected content contained within this class.
*
* {@code
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
* }
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "ExecutionEnvironment", propOrder = {
"platform",
"architecture",
"subtype",
"minVersion",
"maxVersion",
"downloads",
"path"
})
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public class ExecutionEnvironment implements Cloneable, Copyable, PartialCopyable, MergeFrom, ToString
{
/**
* The major classification of the execution environment.
* This should be an ivoa identifier for the platform
* name;
*
*/
@XmlElement(required = true)
@XmlSchemaType(name = "anyURI")
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
protected String platform;
/**
* The hardware architecture that the software is
* compiled for, if relevant.
*
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
protected String architecture;
/**
* this is used to specify exactly the specific kind of
* the environment - e.g. in the case of unix/linux it
* would be nice if the output of uname would suffice
* here - however the most common
*
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
protected String subtype;
/**
* the minimum version of the environment that the code
* will run in -
*
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
protected String minVersion;
/**
* The highest version of the environment that this
* applies to.
*
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
protected String maxVersion;
/**
* This can actually point to different types of entity
* depending on the platform e.g. for the "unix" platform
* this would typically point to a compiled executable
* image (though it might point to an archive or
* "packaging" file that contains the executable amongst
* other files). For the "Java Webstart" platform this
* element will point to a ".jnlp" file that contains all
* of the information necessary for the Java Webstart
* technology to download and run the application.
*
*/
@XmlElement(name = "download", required = true)
@XmlSchemaType(name = "anyURI")
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
protected List downloads;
/**
* If the software image is packaged within some form of
* archive format (e.g. tar or zip format) then this
* element can be used to indicate the exact location of
* the software image within the archive file.
*
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
protected String path;
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
protected transient ExecutionEnvironment.Modifier __cachedModifier__;
/**
* Default no-arg constructor
*
*/
public ExecutionEnvironment() {
super();
}
/**
* Fully-initialising value constructor
*
*/
public ExecutionEnvironment(final String platform, final String architecture, final String subtype, final String minVersion, final String maxVersion, final List downloads, final String path) {
this.platform = platform;
this.architecture = architecture;
this.subtype = subtype;
this.minVersion = minVersion;
this.maxVersion = maxVersion;
this.downloads = downloads;
this.path = path;
}
/**
* The major classification of the execution environment.
* This should be an ivoa identifier for the platform
* name;
*
* @return
* possible object is
* {@link String }
*
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public String getPlatform() {
return platform;
}
/**
* Sets the value of the platform property.
*
* @param value
* allowed object is
* {@link String }
*
* @see #getPlatform()
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void setPlatform(String value) {
this.platform = value;
}
/**
* The hardware architecture that the software is
* compiled for, if relevant.
*
* @return
* possible object is
* {@link String }
*
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public String getArchitecture() {
return architecture;
}
/**
* Sets the value of the architecture property.
*
* @param value
* allowed object is
* {@link String }
*
* @see #getArchitecture()
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void setArchitecture(String value) {
this.architecture = value;
}
/**
* this is used to specify exactly the specific kind of
* the environment - e.g. in the case of unix/linux it
* would be nice if the output of uname would suffice
* here - however the most common
*
* @return
* possible object is
* {@link String }
*
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public String getSubtype() {
return subtype;
}
/**
* Sets the value of the subtype property.
*
* @param value
* allowed object is
* {@link String }
*
* @see #getSubtype()
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void setSubtype(String value) {
this.subtype = value;
}
/**
* the minimum version of the environment that the code
* will run in -
*
* @return
* possible object is
* {@link String }
*
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public String getMinVersion() {
return minVersion;
}
/**
* Sets the value of the minVersion property.
*
* @param value
* allowed object is
* {@link String }
*
* @see #getMinVersion()
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void setMinVersion(String value) {
this.minVersion = value;
}
/**
* The highest version of the environment that this
* applies to.
*
* @return
* possible object is
* {@link String }
*
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public String getMaxVersion() {
return maxVersion;
}
/**
* Sets the value of the maxVersion property.
*
* @param value
* allowed object is
* {@link String }
*
* @see #getMaxVersion()
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void setMaxVersion(String value) {
this.maxVersion = value;
}
/**
* This can actually point to different types of entity
* depending on the platform e.g. for the "unix" platform
* this would typically point to a compiled executable
* image (though it might point to an archive or
* "packaging" file that contains the executable amongst
* other files). For the "Java Webstart" platform this
* element will point to a ".jnlp" file that contains all
* of the information necessary for the Java Webstart
* technology to download and run the application.
*
* Gets the value of the downloads property.
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the downloads property.
*
*
* For example, to add a new item, do as follows:
*
*
* getDownloads().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link String }
*
*
*
* @return
* The value of the downloads property.
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public List getDownloads() {
if (downloads == null) {
downloads = new ArrayList<>();
}
return this.downloads;
}
/**
* If the software image is packaged within some form of
* archive format (e.g. tar or zip format) then this
* element can be used to indicate the exact location of
* the software image within the archive file.
*
* @return
* possible object is
* {@link String }
*
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public String getPath() {
return path;
}
/**
* Sets the value of the path property.
*
* @param value
* allowed object is
* {@link String }
*
* @see #getPath()
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void setPath(String value) {
this.path = value;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public boolean equals(Object object) {
if ((object == null)||(this.getClass()!= object.getClass())) {
return false;
}
if (this == object) {
return true;
}
final ExecutionEnvironment that = ((ExecutionEnvironment) object);
{
String leftPlatform;
leftPlatform = this.getPlatform();
String rightPlatform;
rightPlatform = that.getPlatform();
if (this.platform!= null) {
if (that.platform!= null) {
if (!leftPlatform.equals(rightPlatform)) {
return false;
}
} else {
return false;
}
} else {
if (that.platform!= null) {
return false;
}
}
}
{
String leftArchitecture;
leftArchitecture = this.getArchitecture();
String rightArchitecture;
rightArchitecture = that.getArchitecture();
if (this.architecture!= null) {
if (that.architecture!= null) {
if (!leftArchitecture.equals(rightArchitecture)) {
return false;
}
} else {
return false;
}
} else {
if (that.architecture!= null) {
return false;
}
}
}
{
String leftSubtype;
leftSubtype = this.getSubtype();
String rightSubtype;
rightSubtype = that.getSubtype();
if (this.subtype!= null) {
if (that.subtype!= null) {
if (!leftSubtype.equals(rightSubtype)) {
return false;
}
} else {
return false;
}
} else {
if (that.subtype!= null) {
return false;
}
}
}
{
String leftMinVersion;
leftMinVersion = this.getMinVersion();
String rightMinVersion;
rightMinVersion = that.getMinVersion();
if (this.minVersion!= null) {
if (that.minVersion!= null) {
if (!leftMinVersion.equals(rightMinVersion)) {
return false;
}
} else {
return false;
}
} else {
if (that.minVersion!= null) {
return false;
}
}
}
{
String leftMaxVersion;
leftMaxVersion = this.getMaxVersion();
String rightMaxVersion;
rightMaxVersion = that.getMaxVersion();
if (this.maxVersion!= null) {
if (that.maxVersion!= null) {
if (!leftMaxVersion.equals(rightMaxVersion)) {
return false;
}
} else {
return false;
}
} else {
if (that.maxVersion!= null) {
return false;
}
}
}
{
List leftDownloads;
leftDownloads = (((this.downloads!= null)&&(!this.downloads.isEmpty()))?this.getDownloads():null);
List rightDownloads;
rightDownloads = (((that.downloads!= null)&&(!that.downloads.isEmpty()))?that.getDownloads():null);
if ((this.downloads!= null)&&(!this.downloads.isEmpty())) {
if ((that.downloads!= null)&&(!that.downloads.isEmpty())) {
if (!leftDownloads.equals(rightDownloads)) {
return false;
}
} else {
return false;
}
} else {
if ((that.downloads!= null)&&(!that.downloads.isEmpty())) {
return false;
}
}
}
{
String leftPath;
leftPath = this.getPath();
String rightPath;
rightPath = that.getPath();
if (this.path!= null) {
if (that.path!= null) {
if (!leftPath.equals(rightPath)) {
return false;
}
} else {
return false;
}
} else {
if (that.path!= null) {
return false;
}
}
}
return true;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public int hashCode() {
int currentHashCode = 1;
{
currentHashCode = (currentHashCode* 31);
String thePlatform;
thePlatform = this.getPlatform();
if (this.platform!= null) {
currentHashCode += thePlatform.hashCode();
}
}
{
currentHashCode = (currentHashCode* 31);
String theArchitecture;
theArchitecture = this.getArchitecture();
if (this.architecture!= null) {
currentHashCode += theArchitecture.hashCode();
}
}
{
currentHashCode = (currentHashCode* 31);
String theSubtype;
theSubtype = this.getSubtype();
if (this.subtype!= null) {
currentHashCode += theSubtype.hashCode();
}
}
{
currentHashCode = (currentHashCode* 31);
String theMinVersion;
theMinVersion = this.getMinVersion();
if (this.minVersion!= null) {
currentHashCode += theMinVersion.hashCode();
}
}
{
currentHashCode = (currentHashCode* 31);
String theMaxVersion;
theMaxVersion = this.getMaxVersion();
if (this.maxVersion!= null) {
currentHashCode += theMaxVersion.hashCode();
}
}
{
currentHashCode = (currentHashCode* 31);
List theDownloads;
theDownloads = (((this.downloads!= null)&&(!this.downloads.isEmpty()))?this.getDownloads():null);
if ((this.downloads!= null)&&(!this.downloads.isEmpty())) {
currentHashCode += theDownloads.hashCode();
}
}
{
currentHashCode = (currentHashCode* 31);
String thePath;
thePath = this.getPath();
if (this.path!= null) {
currentHashCode += thePath.hashCode();
}
}
return currentHashCode;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public String toString() {
final ToStringStrategy strategy = JAXBToStringStrategy.getInstance();
final StringBuilder buffer = new StringBuilder();
append(null, buffer, strategy);
return buffer.toString();
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public StringBuilder append(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
strategy.appendStart(locator, this, buffer);
appendFields(locator, buffer, strategy);
strategy.appendEnd(locator, this, buffer);
return buffer;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public StringBuilder appendFields(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
{
String thePlatform;
thePlatform = this.getPlatform();
strategy.appendField(locator, this, "platform", buffer, thePlatform, (this.platform!= null));
}
{
String theArchitecture;
theArchitecture = this.getArchitecture();
strategy.appendField(locator, this, "architecture", buffer, theArchitecture, (this.architecture!= null));
}
{
String theSubtype;
theSubtype = this.getSubtype();
strategy.appendField(locator, this, "subtype", buffer, theSubtype, (this.subtype!= null));
}
{
String theMinVersion;
theMinVersion = this.getMinVersion();
strategy.appendField(locator, this, "minVersion", buffer, theMinVersion, (this.minVersion!= null));
}
{
String theMaxVersion;
theMaxVersion = this.getMaxVersion();
strategy.appendField(locator, this, "maxVersion", buffer, theMaxVersion, (this.maxVersion!= null));
}
{
List theDownloads;
theDownloads = (((this.downloads!= null)&&(!this.downloads.isEmpty()))?this.getDownloads():null);
strategy.appendField(locator, this, "downloads", buffer, theDownloads, ((this.downloads!= null)&&(!this.downloads.isEmpty())));
}
{
String thePath;
thePath = this.getPath();
strategy.appendField(locator, this, "path", buffer, thePath, (this.path!= null));
}
return buffer;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void mergeFrom(Object left, Object right) {
final MergeStrategy strategy = JAXBMergeStrategy.getInstance();
mergeFrom(null, null, left, right, strategy);
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void mergeFrom(ObjectLocator leftLocator, ObjectLocator rightLocator, Object left, Object right, MergeStrategy strategy) {
if (right instanceof ExecutionEnvironment) {
final ExecutionEnvironment target = this;
final ExecutionEnvironment leftObject = ((ExecutionEnvironment) left);
final ExecutionEnvironment rightObject = ((ExecutionEnvironment) right);
{
Boolean platformShouldBeMergedAndSet = strategy.shouldBeMergedAndSet(leftLocator, rightLocator, (leftObject.platform!= null), (rightObject.platform!= null));
if (platformShouldBeMergedAndSet == Boolean.TRUE) {
String lhsPlatform;
lhsPlatform = leftObject.getPlatform();
String rhsPlatform;
rhsPlatform = rightObject.getPlatform();
String mergedPlatform = ((String) strategy.merge(LocatorUtils.property(leftLocator, "platform", lhsPlatform), LocatorUtils.property(rightLocator, "platform", rhsPlatform), lhsPlatform, rhsPlatform, (leftObject.platform!= null), (rightObject.platform!= null)));
target.setPlatform(mergedPlatform);
} else {
if (platformShouldBeMergedAndSet == Boolean.FALSE) {
target.platform = null;
}
}
}
{
Boolean architectureShouldBeMergedAndSet = strategy.shouldBeMergedAndSet(leftLocator, rightLocator, (leftObject.architecture!= null), (rightObject.architecture!= null));
if (architectureShouldBeMergedAndSet == Boolean.TRUE) {
String lhsArchitecture;
lhsArchitecture = leftObject.getArchitecture();
String rhsArchitecture;
rhsArchitecture = rightObject.getArchitecture();
String mergedArchitecture = ((String) strategy.merge(LocatorUtils.property(leftLocator, "architecture", lhsArchitecture), LocatorUtils.property(rightLocator, "architecture", rhsArchitecture), lhsArchitecture, rhsArchitecture, (leftObject.architecture!= null), (rightObject.architecture!= null)));
target.setArchitecture(mergedArchitecture);
} else {
if (architectureShouldBeMergedAndSet == Boolean.FALSE) {
target.architecture = null;
}
}
}
{
Boolean subtypeShouldBeMergedAndSet = strategy.shouldBeMergedAndSet(leftLocator, rightLocator, (leftObject.subtype!= null), (rightObject.subtype!= null));
if (subtypeShouldBeMergedAndSet == Boolean.TRUE) {
String lhsSubtype;
lhsSubtype = leftObject.getSubtype();
String rhsSubtype;
rhsSubtype = rightObject.getSubtype();
String mergedSubtype = ((String) strategy.merge(LocatorUtils.property(leftLocator, "subtype", lhsSubtype), LocatorUtils.property(rightLocator, "subtype", rhsSubtype), lhsSubtype, rhsSubtype, (leftObject.subtype!= null), (rightObject.subtype!= null)));
target.setSubtype(mergedSubtype);
} else {
if (subtypeShouldBeMergedAndSet == Boolean.FALSE) {
target.subtype = null;
}
}
}
{
Boolean minVersionShouldBeMergedAndSet = strategy.shouldBeMergedAndSet(leftLocator, rightLocator, (leftObject.minVersion!= null), (rightObject.minVersion!= null));
if (minVersionShouldBeMergedAndSet == Boolean.TRUE) {
String lhsMinVersion;
lhsMinVersion = leftObject.getMinVersion();
String rhsMinVersion;
rhsMinVersion = rightObject.getMinVersion();
String mergedMinVersion = ((String) strategy.merge(LocatorUtils.property(leftLocator, "minVersion", lhsMinVersion), LocatorUtils.property(rightLocator, "minVersion", rhsMinVersion), lhsMinVersion, rhsMinVersion, (leftObject.minVersion!= null), (rightObject.minVersion!= null)));
target.setMinVersion(mergedMinVersion);
} else {
if (minVersionShouldBeMergedAndSet == Boolean.FALSE) {
target.minVersion = null;
}
}
}
{
Boolean maxVersionShouldBeMergedAndSet = strategy.shouldBeMergedAndSet(leftLocator, rightLocator, (leftObject.maxVersion!= null), (rightObject.maxVersion!= null));
if (maxVersionShouldBeMergedAndSet == Boolean.TRUE) {
String lhsMaxVersion;
lhsMaxVersion = leftObject.getMaxVersion();
String rhsMaxVersion;
rhsMaxVersion = rightObject.getMaxVersion();
String mergedMaxVersion = ((String) strategy.merge(LocatorUtils.property(leftLocator, "maxVersion", lhsMaxVersion), LocatorUtils.property(rightLocator, "maxVersion", rhsMaxVersion), lhsMaxVersion, rhsMaxVersion, (leftObject.maxVersion!= null), (rightObject.maxVersion!= null)));
target.setMaxVersion(mergedMaxVersion);
} else {
if (maxVersionShouldBeMergedAndSet == Boolean.FALSE) {
target.maxVersion = null;
}
}
}
{
Boolean downloadsShouldBeMergedAndSet = strategy.shouldBeMergedAndSet(leftLocator, rightLocator, ((leftObject.downloads!= null)&&(!leftObject.downloads.isEmpty())), ((rightObject.downloads!= null)&&(!rightObject.downloads.isEmpty())));
if (downloadsShouldBeMergedAndSet == Boolean.TRUE) {
List lhsDownloads;
lhsDownloads = (((leftObject.downloads!= null)&&(!leftObject.downloads.isEmpty()))?leftObject.getDownloads():null);
List rhsDownloads;
rhsDownloads = (((rightObject.downloads!= null)&&(!rightObject.downloads.isEmpty()))?rightObject.getDownloads():null);
List mergedDownloads = ((List ) strategy.merge(LocatorUtils.property(leftLocator, "downloads", lhsDownloads), LocatorUtils.property(rightLocator, "downloads", rhsDownloads), lhsDownloads, rhsDownloads, ((leftObject.downloads!= null)&&(!leftObject.downloads.isEmpty())), ((rightObject.downloads!= null)&&(!rightObject.downloads.isEmpty()))));
target.downloads = null;
if (mergedDownloads!= null) {
List uniqueDownloadsl = target.getDownloads();
uniqueDownloadsl.addAll(mergedDownloads);
}
} else {
if (downloadsShouldBeMergedAndSet == Boolean.FALSE) {
target.downloads = null;
}
}
}
{
Boolean pathShouldBeMergedAndSet = strategy.shouldBeMergedAndSet(leftLocator, rightLocator, (leftObject.path!= null), (rightObject.path!= null));
if (pathShouldBeMergedAndSet == Boolean.TRUE) {
String lhsPath;
lhsPath = leftObject.getPath();
String rhsPath;
rhsPath = rightObject.getPath();
String mergedPath = ((String) strategy.merge(LocatorUtils.property(leftLocator, "path", lhsPath), LocatorUtils.property(rightLocator, "path", rhsPath), lhsPath, rhsPath, (leftObject.path!= null), (rightObject.path!= null)));
target.setPath(mergedPath);
} else {
if (pathShouldBeMergedAndSet == Boolean.FALSE) {
target.path = null;
}
}
}
}
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Object createNewInstance() {
return new ExecutionEnvironment();
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public ExecutionEnvironment clone() {
final ExecutionEnvironment _newObject;
try {
_newObject = ((ExecutionEnvironment) super.clone());
} catch (CloneNotSupportedException e) {
throw new RuntimeException(e);
}
_newObject.downloads = ((this.downloads == null)?null:new ArrayList<>(this.downloads));
return _newObject;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public ExecutionEnvironment createCopy() {
final ExecutionEnvironment _newObject;
try {
_newObject = ((ExecutionEnvironment) super.clone());
} catch (CloneNotSupportedException e) {
throw new RuntimeException(e);
}
_newObject.platform = this.platform;
_newObject.architecture = this.architecture;
_newObject.subtype = this.subtype;
_newObject.minVersion = this.minVersion;
_newObject.maxVersion = this.maxVersion;
_newObject.downloads = ((this.downloads == null)?null:new ArrayList<>(this.downloads));
_newObject.path = this.path;
return _newObject;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public ExecutionEnvironment createCopy(final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
final ExecutionEnvironment _newObject;
try {
_newObject = ((ExecutionEnvironment) super.clone());
} catch (CloneNotSupportedException e) {
throw new RuntimeException(e);
}
final PropertyTree platformPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("platform"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(platformPropertyTree!= null):((platformPropertyTree == null)||(!platformPropertyTree.isLeaf())))) {
_newObject.platform = this.platform;
}
final PropertyTree architecturePropertyTree = ((_propertyTree == null)?null:_propertyTree.get("architecture"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(architecturePropertyTree!= null):((architecturePropertyTree == null)||(!architecturePropertyTree.isLeaf())))) {
_newObject.architecture = this.architecture;
}
final PropertyTree subtypePropertyTree = ((_propertyTree == null)?null:_propertyTree.get("subtype"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(subtypePropertyTree!= null):((subtypePropertyTree == null)||(!subtypePropertyTree.isLeaf())))) {
_newObject.subtype = this.subtype;
}
final PropertyTree minVersionPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("minVersion"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(minVersionPropertyTree!= null):((minVersionPropertyTree == null)||(!minVersionPropertyTree.isLeaf())))) {
_newObject.minVersion = this.minVersion;
}
final PropertyTree maxVersionPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("maxVersion"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(maxVersionPropertyTree!= null):((maxVersionPropertyTree == null)||(!maxVersionPropertyTree.isLeaf())))) {
_newObject.maxVersion = this.maxVersion;
}
final PropertyTree downloadsPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("downloads"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(downloadsPropertyTree!= null):((downloadsPropertyTree == null)||(!downloadsPropertyTree.isLeaf())))) {
_newObject.downloads = ((this.downloads == null)?null:new ArrayList<>(this.downloads));
}
final PropertyTree pathPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("path"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(pathPropertyTree!= null):((pathPropertyTree == null)||(!pathPropertyTree.isLeaf())))) {
_newObject.path = this.path;
}
return _newObject;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public ExecutionEnvironment copyExcept(final PropertyTree _propertyTree) {
return createCopy(_propertyTree, PropertyTreeUse.EXCLUDE);
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public ExecutionEnvironment copyOnly(final PropertyTree _propertyTree) {
return createCopy(_propertyTree, PropertyTreeUse.INCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public ExecutionEnvironment.Modifier modifier() {
if (null == this.__cachedModifier__) {
this.__cachedModifier__ = new ExecutionEnvironment.Modifier();
}
return ((ExecutionEnvironment.Modifier) this.__cachedModifier__);
}
/**
* Copies all state of this object to a builder. This method is used by the copyOf
* method and should not be called directly by client code.
*
* @param _other
* A builder instance to which the state of this object will be copied.
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public<_B >void copyTo(final ExecutionEnvironment.Builder<_B> _other) {
_other.platform = this.platform;
_other.architecture = this.architecture;
_other.subtype = this.subtype;
_other.minVersion = this.minVersion;
_other.maxVersion = this.maxVersion;
if (this.downloads == null) {
_other.downloads = null;
} else {
_other.downloads = new ArrayList<>();
for (String _item: this.downloads) {
_other.downloads.add(((_item == null)?null:new Buildable.PrimitiveBuildable(_item)));
}
}
_other.path = this.path;
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public<_B >ExecutionEnvironment.Builder<_B> newCopyBuilder(final _B _parentBuilder) {
return new ExecutionEnvironment.Builder<_B>(_parentBuilder, this, true);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public ExecutionEnvironment.Builder newCopyBuilder() {
return newCopyBuilder(null);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static ExecutionEnvironment.Builder builder() {
return new ExecutionEnvironment.Builder<>(null, null, false);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static<_B >ExecutionEnvironment.Builder<_B> copyOf(final ExecutionEnvironment _other) {
final ExecutionEnvironment.Builder<_B> _newBuilder = new ExecutionEnvironment.Builder<>(null, null, false);
_other.copyTo(_newBuilder);
return _newBuilder;
}
/**
* Copies all state of this object to a builder. This method is used by the copyOf
* method and should not be called directly by client code.
*
* @param _other
* A builder instance to which the state of this object will be copied.
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public<_B >void copyTo(final ExecutionEnvironment.Builder<_B> _other, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
final PropertyTree platformPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("platform"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(platformPropertyTree!= null):((platformPropertyTree == null)||(!platformPropertyTree.isLeaf())))) {
_other.platform = this.platform;
}
final PropertyTree architecturePropertyTree = ((_propertyTree == null)?null:_propertyTree.get("architecture"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(architecturePropertyTree!= null):((architecturePropertyTree == null)||(!architecturePropertyTree.isLeaf())))) {
_other.architecture = this.architecture;
}
final PropertyTree subtypePropertyTree = ((_propertyTree == null)?null:_propertyTree.get("subtype"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(subtypePropertyTree!= null):((subtypePropertyTree == null)||(!subtypePropertyTree.isLeaf())))) {
_other.subtype = this.subtype;
}
final PropertyTree minVersionPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("minVersion"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(minVersionPropertyTree!= null):((minVersionPropertyTree == null)||(!minVersionPropertyTree.isLeaf())))) {
_other.minVersion = this.minVersion;
}
final PropertyTree maxVersionPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("maxVersion"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(maxVersionPropertyTree!= null):((maxVersionPropertyTree == null)||(!maxVersionPropertyTree.isLeaf())))) {
_other.maxVersion = this.maxVersion;
}
final PropertyTree downloadsPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("downloads"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(downloadsPropertyTree!= null):((downloadsPropertyTree == null)||(!downloadsPropertyTree.isLeaf())))) {
if (this.downloads == null) {
_other.downloads = null;
} else {
_other.downloads = new ArrayList<>();
for (String _item: this.downloads) {
_other.downloads.add(((_item == null)?null:new Buildable.PrimitiveBuildable(_item)));
}
}
}
final PropertyTree pathPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("path"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(pathPropertyTree!= null):((pathPropertyTree == null)||(!pathPropertyTree.isLeaf())))) {
_other.path = this.path;
}
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public<_B >ExecutionEnvironment.Builder<_B> newCopyBuilder(final _B _parentBuilder, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
return new ExecutionEnvironment.Builder<_B>(_parentBuilder, this, true, _propertyTree, _propertyTreeUse);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public ExecutionEnvironment.Builder newCopyBuilder(final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
return newCopyBuilder(null, _propertyTree, _propertyTreeUse);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static<_B >ExecutionEnvironment.Builder<_B> copyOf(final ExecutionEnvironment _other, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
final ExecutionEnvironment.Builder<_B> _newBuilder = new ExecutionEnvironment.Builder<>(null, null, false);
_other.copyTo(_newBuilder, _propertyTree, _propertyTreeUse);
return _newBuilder;
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static ExecutionEnvironment.Builder copyExcept(final ExecutionEnvironment _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.EXCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static ExecutionEnvironment.Builder copyOnly(final ExecutionEnvironment _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.INCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public ExecutionEnvironment visit(final PropertyVisitor _visitor_) {
_visitor_.visit(this);
_visitor_.visit(new SingleProperty<>(ExecutionEnvironment.PropInfo.PLATFORM, this));
_visitor_.visit(new SingleProperty<>(ExecutionEnvironment.PropInfo.ARCHITECTURE, this));
_visitor_.visit(new SingleProperty<>(ExecutionEnvironment.PropInfo.SUBTYPE, this));
_visitor_.visit(new SingleProperty<>(ExecutionEnvironment.PropInfo.MIN_VERSION, this));
_visitor_.visit(new SingleProperty<>(ExecutionEnvironment.PropInfo.MAX_VERSION, this));
_visitor_.visit(new CollectionProperty<>(ExecutionEnvironment.PropInfo.DOWNLOADS, this));
_visitor_.visit(new SingleProperty<>(ExecutionEnvironment.PropInfo.PATH, this));
return this;
}
public static class Builder<_B >implements Buildable
{
protected final _B _parentBuilder;
protected final ExecutionEnvironment _storedValue;
private String platform;
private String architecture;
private String subtype;
private String minVersion;
private String maxVersion;
private List downloads;
private String path;
public Builder(final _B _parentBuilder, final ExecutionEnvironment _other, final boolean _copy) {
this._parentBuilder = _parentBuilder;
if (_other!= null) {
if (_copy) {
_storedValue = null;
this.platform = _other.platform;
this.architecture = _other.architecture;
this.subtype = _other.subtype;
this.minVersion = _other.minVersion;
this.maxVersion = _other.maxVersion;
if (_other.downloads == null) {
this.downloads = null;
} else {
this.downloads = new ArrayList<>();
for (String _item: _other.downloads) {
this.downloads.add(((_item == null)?null:new Buildable.PrimitiveBuildable(_item)));
}
}
this.path = _other.path;
} else {
_storedValue = _other;
}
} else {
_storedValue = null;
}
}
public Builder(final _B _parentBuilder, final ExecutionEnvironment _other, final boolean _copy, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
this._parentBuilder = _parentBuilder;
if (_other!= null) {
if (_copy) {
_storedValue = null;
final PropertyTree platformPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("platform"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(platformPropertyTree!= null):((platformPropertyTree == null)||(!platformPropertyTree.isLeaf())))) {
this.platform = _other.platform;
}
final PropertyTree architecturePropertyTree = ((_propertyTree == null)?null:_propertyTree.get("architecture"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(architecturePropertyTree!= null):((architecturePropertyTree == null)||(!architecturePropertyTree.isLeaf())))) {
this.architecture = _other.architecture;
}
final PropertyTree subtypePropertyTree = ((_propertyTree == null)?null:_propertyTree.get("subtype"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(subtypePropertyTree!= null):((subtypePropertyTree == null)||(!subtypePropertyTree.isLeaf())))) {
this.subtype = _other.subtype;
}
final PropertyTree minVersionPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("minVersion"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(minVersionPropertyTree!= null):((minVersionPropertyTree == null)||(!minVersionPropertyTree.isLeaf())))) {
this.minVersion = _other.minVersion;
}
final PropertyTree maxVersionPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("maxVersion"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(maxVersionPropertyTree!= null):((maxVersionPropertyTree == null)||(!maxVersionPropertyTree.isLeaf())))) {
this.maxVersion = _other.maxVersion;
}
final PropertyTree downloadsPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("downloads"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(downloadsPropertyTree!= null):((downloadsPropertyTree == null)||(!downloadsPropertyTree.isLeaf())))) {
if (_other.downloads == null) {
this.downloads = null;
} else {
this.downloads = new ArrayList<>();
for (String _item: _other.downloads) {
this.downloads.add(((_item == null)?null:new Buildable.PrimitiveBuildable(_item)));
}
}
}
final PropertyTree pathPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("path"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(pathPropertyTree!= null):((pathPropertyTree == null)||(!pathPropertyTree.isLeaf())))) {
this.path = _other.path;
}
} else {
_storedValue = _other;
}
} else {
_storedValue = null;
}
}
public _B end() {
return this._parentBuilder;
}
protected<_P extends ExecutionEnvironment >_P init(final _P _product) {
_product.platform = this.platform;
_product.architecture = this.architecture;
_product.subtype = this.subtype;
_product.minVersion = this.minVersion;
_product.maxVersion = this.maxVersion;
if (this.downloads!= null) {
final List downloads = new ArrayList<>(this.downloads.size());
for (Buildable _item: this.downloads) {
downloads.add(((String) _item.build()));
}
_product.downloads = downloads;
}
_product.path = this.path;
return _product;
}
/**
* Sets the new value of "platform" (any previous value will be replaced)
*
* @param platform
* New value of the "platform" property.
*/
public ExecutionEnvironment.Builder<_B> withPlatform(final String platform) {
this.platform = platform;
return this;
}
/**
* Sets the new value of "architecture" (any previous value will be replaced)
*
* @param architecture
* New value of the "architecture" property.
*/
public ExecutionEnvironment.Builder<_B> withArchitecture(final String architecture) {
this.architecture = architecture;
return this;
}
/**
* Sets the new value of "subtype" (any previous value will be replaced)
*
* @param subtype
* New value of the "subtype" property.
*/
public ExecutionEnvironment.Builder<_B> withSubtype(final String subtype) {
this.subtype = subtype;
return this;
}
/**
* Sets the new value of "minVersion" (any previous value will be replaced)
*
* @param minVersion
* New value of the "minVersion" property.
*/
public ExecutionEnvironment.Builder<_B> withMinVersion(final String minVersion) {
this.minVersion = minVersion;
return this;
}
/**
* Sets the new value of "maxVersion" (any previous value will be replaced)
*
* @param maxVersion
* New value of the "maxVersion" property.
*/
public ExecutionEnvironment.Builder<_B> withMaxVersion(final String maxVersion) {
this.maxVersion = maxVersion;
return this;
}
/**
* Adds the given items to the value of "downloads"
*
* @param downloads
* Items to add to the value of the "downloads" property
*/
public ExecutionEnvironment.Builder<_B> addDownloads(final Iterable extends String> downloads) {
if (downloads!= null) {
if (this.downloads == null) {
this.downloads = new ArrayList<>();
}
for (String _item: downloads) {
this.downloads.add(new Buildable.PrimitiveBuildable(_item));
}
}
return this;
}
/**
* Sets the new value of "downloads" (any previous value will be replaced)
*
* @param downloads
* New value of the "downloads" property.
*/
public ExecutionEnvironment.Builder<_B> withDownloads(final Iterable extends String> downloads) {
if (this.downloads!= null) {
this.downloads.clear();
}
return addDownloads(downloads);
}
/**
* Adds the given items to the value of "downloads"
*
* @param downloads
* Items to add to the value of the "downloads" property
*/
public ExecutionEnvironment.Builder<_B> addDownloads(String... downloads) {
addDownloads(Arrays.asList(downloads));
return this;
}
/**
* Sets the new value of "downloads" (any previous value will be replaced)
*
* @param downloads
* New value of the "downloads" property.
*/
public ExecutionEnvironment.Builder<_B> withDownloads(String... downloads) {
withDownloads(Arrays.asList(downloads));
return this;
}
/**
* Sets the new value of "path" (any previous value will be replaced)
*
* @param path
* New value of the "path" property.
*/
public ExecutionEnvironment.Builder<_B> withPath(final String path) {
this.path = path;
return this;
}
@Override
public ExecutionEnvironment build() {
if (_storedValue == null) {
return this.init(new ExecutionEnvironment());
} else {
return ((ExecutionEnvironment) _storedValue);
}
}
public ExecutionEnvironment.Builder<_B> copyOf(final ExecutionEnvironment _other) {
_other.copyTo(this);
return this;
}
public ExecutionEnvironment.Builder<_B> copyOf(final ExecutionEnvironment.Builder _other) {
return copyOf(_other.build());
}
}
public class Modifier {
public void setPlatform(final String platform) {
ExecutionEnvironment.this.setPlatform(platform);
}
public void setArchitecture(final String architecture) {
ExecutionEnvironment.this.setArchitecture(architecture);
}
public void setSubtype(final String subtype) {
ExecutionEnvironment.this.setSubtype(subtype);
}
public void setMinVersion(final String minVersion) {
ExecutionEnvironment.this.setMinVersion(minVersion);
}
public void setMaxVersion(final String maxVersion) {
ExecutionEnvironment.this.setMaxVersion(maxVersion);
}
public List getDownloads() {
if (ExecutionEnvironment.this.downloads == null) {
ExecutionEnvironment.this.downloads = new ArrayList<>();
}
return ExecutionEnvironment.this.downloads;
}
public void setPath(final String path) {
ExecutionEnvironment.this.setPath(path);
}
}
public static class PropInfo {
public static final transient SinglePropertyInfo PLATFORM = new SinglePropertyInfo("platform", ExecutionEnvironment.class, String.class, false, null, new QName("", "platform"), new QName("http://www.ivoa.net/xml/VOApplication/v1.0rc1", "Platform"), false) {
@Override
public String get(final ExecutionEnvironment _instance_) {
return ((_instance_ == null)?null:_instance_.platform);
}
@Override
public void set(final ExecutionEnvironment _instance_, final String _value_) {
if (_instance_!= null) {
_instance_.platform = _value_;
}
}
}
;
public static final transient SinglePropertyInfo ARCHITECTURE = new SinglePropertyInfo("architecture", ExecutionEnvironment.class, String.class, false, null, new QName("", "architecture"), new QName("http://www.w3.org/2001/XMLSchema", "string"), false) {
@Override
public String get(final ExecutionEnvironment _instance_) {
return ((_instance_ == null)?null:_instance_.architecture);
}
@Override
public void set(final ExecutionEnvironment _instance_, final String _value_) {
if (_instance_!= null) {
_instance_.architecture = _value_;
}
}
}
;
public static final transient SinglePropertyInfo SUBTYPE = new SinglePropertyInfo("subtype", ExecutionEnvironment.class, String.class, false, null, new QName("", "subtype"), new QName("http://www.w3.org/2001/XMLSchema", "string"), false) {
@Override
public String get(final ExecutionEnvironment _instance_) {
return ((_instance_ == null)?null:_instance_.subtype);
}
@Override
public void set(final ExecutionEnvironment _instance_, final String _value_) {
if (_instance_!= null) {
_instance_.subtype = _value_;
}
}
}
;
public static final transient SinglePropertyInfo MIN_VERSION = new SinglePropertyInfo("minVersion", ExecutionEnvironment.class, String.class, false, null, new QName("", "minVersion"), new QName("http://www.w3.org/2001/XMLSchema", "string"), false) {
@Override
public String get(final ExecutionEnvironment _instance_) {
return ((_instance_ == null)?null:_instance_.minVersion);
}
@Override
public void set(final ExecutionEnvironment _instance_, final String _value_) {
if (_instance_!= null) {
_instance_.minVersion = _value_;
}
}
}
;
public static final transient SinglePropertyInfo MAX_VERSION = new SinglePropertyInfo("maxVersion", ExecutionEnvironment.class, String.class, false, null, new QName("", "maxVersion"), new QName("http://www.w3.org/2001/XMLSchema", "string"), false) {
@Override
public String get(final ExecutionEnvironment _instance_) {
return ((_instance_ == null)?null:_instance_.maxVersion);
}
@Override
public void set(final ExecutionEnvironment _instance_, final String _value_) {
if (_instance_!= null) {
_instance_.maxVersion = _value_;
}
}
}
;
public static final transient CollectionPropertyInfo DOWNLOADS = new CollectionPropertyInfo("downloads", ExecutionEnvironment.class, String.class, true, null, new QName("", "download"), new QName("http://www.w3.org/2001/XMLSchema", "anyURI"), false) {
@Override
public List get(final ExecutionEnvironment _instance_) {
return ((_instance_ == null)?null:_instance_.downloads);
}
@Override
public void set(final ExecutionEnvironment _instance_, final List _value_) {
if (_instance_!= null) {
_instance_.downloads = _value_;
}
}
}
;
public static final transient SinglePropertyInfo PATH = new SinglePropertyInfo("path", ExecutionEnvironment.class, String.class, false, null, new QName("", "path"), new QName("http://www.w3.org/2001/XMLSchema", "string"), false) {
@Override
public String get(final ExecutionEnvironment _instance_) {
return ((_instance_ == null)?null:_instance_.path);
}
@Override
public void set(final ExecutionEnvironment _instance_, final String _value_) {
if (_instance_!= null) {
_instance_.path = _value_;
}
}
}
;
}
public static class Select
extends ExecutionEnvironment.Selector
{
Select() {
super(null, null, null);
}
public static ExecutionEnvironment.Select _root() {
return new ExecutionEnvironment.Select();
}
}
public static class Selector , TParent >
extends com.kscs.util.jaxb.Selector
{
private com.kscs.util.jaxb.Selector> platform = null;
private com.kscs.util.jaxb.Selector> architecture = null;
private com.kscs.util.jaxb.Selector> subtype = null;
private com.kscs.util.jaxb.Selector> minVersion = null;
private com.kscs.util.jaxb.Selector> maxVersion = null;
private com.kscs.util.jaxb.Selector> downloads = null;
private com.kscs.util.jaxb.Selector> path = null;
public Selector(final TRoot root, final TParent parent, final String propertyName) {
super(root, parent, propertyName);
}
@Override
public Map buildChildren() {
final Map products = new HashMap<>();
products.putAll(super.buildChildren());
if (this.platform!= null) {
products.put("platform", this.platform.init());
}
if (this.architecture!= null) {
products.put("architecture", this.architecture.init());
}
if (this.subtype!= null) {
products.put("subtype", this.subtype.init());
}
if (this.minVersion!= null) {
products.put("minVersion", this.minVersion.init());
}
if (this.maxVersion!= null) {
products.put("maxVersion", this.maxVersion.init());
}
if (this.downloads!= null) {
products.put("downloads", this.downloads.init());
}
if (this.path!= null) {
products.put("path", this.path.init());
}
return products;
}
public com.kscs.util.jaxb.Selector> platform() {
return ((this.platform == null)?this.platform = new com.kscs.util.jaxb.Selector<>(this._root, this, "platform"):this.platform);
}
public com.kscs.util.jaxb.Selector> architecture() {
return ((this.architecture == null)?this.architecture = new com.kscs.util.jaxb.Selector<>(this._root, this, "architecture"):this.architecture);
}
public com.kscs.util.jaxb.Selector> subtype() {
return ((this.subtype == null)?this.subtype = new com.kscs.util.jaxb.Selector<>(this._root, this, "subtype"):this.subtype);
}
public com.kscs.util.jaxb.Selector> minVersion() {
return ((this.minVersion == null)?this.minVersion = new com.kscs.util.jaxb.Selector<>(this._root, this, "minVersion"):this.minVersion);
}
public com.kscs.util.jaxb.Selector> maxVersion() {
return ((this.maxVersion == null)?this.maxVersion = new com.kscs.util.jaxb.Selector<>(this._root, this, "maxVersion"):this.maxVersion);
}
public com.kscs.util.jaxb.Selector> downloads() {
return ((this.downloads == null)?this.downloads = new com.kscs.util.jaxb.Selector<>(this._root, this, "downloads"):this.downloads);
}
public com.kscs.util.jaxb.Selector> path() {
return ((this.path == null)?this.path = new com.kscs.util.jaxb.Selector<>(this._root, this, "path"):this.path);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy