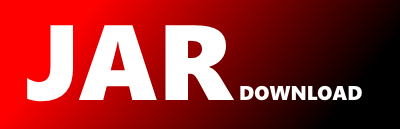
org.javastro.ivoa.entities.resource.cone.ConeSearch Maven / Gradle / Ivy
package org.javastro.ivoa.entities.resource.cone;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import javax.xml.namespace.QName;
import com.kscs.util.jaxb.Buildable;
import com.kscs.util.jaxb.Copyable;
import com.kscs.util.jaxb.PartialCopyable;
import com.kscs.util.jaxb.PropertyTree;
import com.kscs.util.jaxb.PropertyTreeUse;
import com.kscs.util.jaxb.PropertyVisitor;
import com.kscs.util.jaxb.SingleProperty;
import com.kscs.util.jaxb.SinglePropertyInfo;
import com.kscs.util.jaxb.SinglePropertyInfo;
import com.kscs.util.jaxb.SinglePropertyInfo;
import com.kscs.util.jaxb.SinglePropertyInfo;
import com.kscs.util.jaxb.SinglePropertyInfo;
import jakarta.annotation.Generated;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlType;
import org.javastro.ivoa.entities.resource.Capability;
import org.javastro.ivoa.entities.resource.ServiceInterface;
import org.javastro.ivoa.entities.resource.Validation;
import org.jvnet.jaxb.lang.JAXBMergeStrategy;
import org.jvnet.jaxb.lang.JAXBToStringStrategy;
import org.jvnet.jaxb.lang.MergeFrom;
import org.jvnet.jaxb.lang.MergeStrategy;
import org.jvnet.jaxb.lang.ToString;
import org.jvnet.jaxb.lang.ToStringStrategy;
import org.jvnet.jaxb.locator.ObjectLocator;
import org.jvnet.jaxb.locator.util.LocatorUtils;
/**
* The capabilities of a Cone Search implementation.
*
* Java class for ConeSearch complex type
.
*
* The following schema fragment specifies the expected content contained within this class.
*
* {@code
*
*
*
*
*
*
*
*
*
*
*
*
* }
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "ConeSearch", propOrder = {
"maxSR",
"maxRecords",
"verbosity",
"testQuery"
})
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public class ConeSearch
extends CSCapRestriction
implements Cloneable, Copyable, PartialCopyable, MergeFrom, ToString
{
/**
* Use 180.0 if there is no restriction.
*
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
protected float maxSR;
/**
* The largest number of records that the service will
* return.
*
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
protected int maxRecords;
/**
* True if the service supports the VERB keyword;
* false, otherwise.
*
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
protected boolean verbosity;
/**
* A query that will result in at least on
* matched record that can be used to test the
* service.
*
*/
@XmlElement(required = true)
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
protected Query testQuery;
/**
* Default no-arg constructor
*
*/
public ConeSearch() {
super();
}
/**
* Fully-initialising value constructor
*
*/
public ConeSearch(final List validationLevels, final String description, final List interfaces, final String standardID, final float maxSR, final int maxRecords, final boolean verbosity, final Query testQuery) {
super(validationLevels, description, interfaces, standardID);
this.maxSR = maxSR;
this.maxRecords = maxRecords;
this.verbosity = verbosity;
this.testQuery = testQuery;
}
/**
* Use 180.0 if there is no restriction.
*
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public float getMaxSR() {
return maxSR;
}
/**
* Sets the value of the maxSR property.
*
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void setMaxSR(float value) {
this.maxSR = value;
}
/**
* The largest number of records that the service will
* return.
*
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public int getMaxRecords() {
return maxRecords;
}
/**
* Sets the value of the maxRecords property.
*
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void setMaxRecords(int value) {
this.maxRecords = value;
}
/**
* True if the service supports the VERB keyword;
* false, otherwise.
*
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public boolean isVerbosity() {
return verbosity;
}
/**
* Sets the value of the verbosity property.
*
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void setVerbosity(boolean value) {
this.verbosity = value;
}
/**
* A query that will result in at least on
* matched record that can be used to test the
* service.
*
* @return
* possible object is
* {@link Query }
*
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Query getTestQuery() {
return testQuery;
}
/**
* Sets the value of the testQuery property.
*
* @param value
* allowed object is
* {@link Query }
*
* @see #getTestQuery()
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void setTestQuery(Query value) {
this.testQuery = value;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public boolean equals(Object object) {
if ((object == null)||(this.getClass()!= object.getClass())) {
return false;
}
if (this == object) {
return true;
}
if (!super.equals(object)) {
return false;
}
final ConeSearch that = ((ConeSearch) object);
{
float leftMaxSR;
leftMaxSR = this.getMaxSR();
float rightMaxSR;
rightMaxSR = that.getMaxSR();
if (Float.floatToIntBits(leftMaxSR)!= Float.floatToIntBits(rightMaxSR)) {
return false;
}
}
{
int leftMaxRecords;
leftMaxRecords = this.getMaxRecords();
int rightMaxRecords;
rightMaxRecords = that.getMaxRecords();
if (leftMaxRecords!= rightMaxRecords) {
return false;
}
}
{
boolean leftVerbosity;
leftVerbosity = this.isVerbosity();
boolean rightVerbosity;
rightVerbosity = that.isVerbosity();
if (leftVerbosity!= rightVerbosity) {
return false;
}
}
{
Query leftTestQuery;
leftTestQuery = this.getTestQuery();
Query rightTestQuery;
rightTestQuery = that.getTestQuery();
if (this.testQuery!= null) {
if (that.testQuery!= null) {
if (!leftTestQuery.equals(rightTestQuery)) {
return false;
}
} else {
return false;
}
} else {
if (that.testQuery!= null) {
return false;
}
}
}
return true;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public int hashCode() {
int currentHashCode = 1;
currentHashCode = ((currentHashCode* 31)+ super.hashCode());
{
currentHashCode = (currentHashCode* 31);
float theMaxSR;
theMaxSR = this.getMaxSR();
currentHashCode += Float.floatToIntBits(theMaxSR);
}
{
currentHashCode = (currentHashCode* 31);
int theMaxRecords;
theMaxRecords = this.getMaxRecords();
currentHashCode += theMaxRecords;
}
{
currentHashCode = (currentHashCode* 31);
boolean theVerbosity;
theVerbosity = this.isVerbosity();
currentHashCode += (theVerbosity? 1231 : 1237);
}
{
currentHashCode = (currentHashCode* 31);
Query theTestQuery;
theTestQuery = this.getTestQuery();
if (this.testQuery!= null) {
currentHashCode += theTestQuery.hashCode();
}
}
return currentHashCode;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public String toString() {
final ToStringStrategy strategy = JAXBToStringStrategy.getInstance();
final StringBuilder buffer = new StringBuilder();
append(null, buffer, strategy);
return buffer.toString();
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public StringBuilder append(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
strategy.appendStart(locator, this, buffer);
appendFields(locator, buffer, strategy);
strategy.appendEnd(locator, this, buffer);
return buffer;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public StringBuilder appendFields(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
super.appendFields(locator, buffer, strategy);
{
float theMaxSR;
theMaxSR = this.getMaxSR();
strategy.appendField(locator, this, "maxSR", buffer, theMaxSR, true);
}
{
int theMaxRecords;
theMaxRecords = this.getMaxRecords();
strategy.appendField(locator, this, "maxRecords", buffer, theMaxRecords, true);
}
{
boolean theVerbosity;
theVerbosity = this.isVerbosity();
strategy.appendField(locator, this, "verbosity", buffer, theVerbosity, true);
}
{
Query theTestQuery;
theTestQuery = this.getTestQuery();
strategy.appendField(locator, this, "testQuery", buffer, theTestQuery, (this.testQuery!= null));
}
return buffer;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void mergeFrom(Object left, Object right) {
final MergeStrategy strategy = JAXBMergeStrategy.getInstance();
mergeFrom(null, null, left, right, strategy);
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void mergeFrom(ObjectLocator leftLocator, ObjectLocator rightLocator, Object left, Object right, MergeStrategy strategy) {
super.mergeFrom(leftLocator, rightLocator, left, right, strategy);
if (right instanceof ConeSearch) {
final ConeSearch target = this;
final ConeSearch leftObject = ((ConeSearch) left);
final ConeSearch rightObject = ((ConeSearch) right);
{
Boolean maxSRShouldBeMergedAndSet = strategy.shouldBeMergedAndSet(leftLocator, rightLocator, true, true);
if (maxSRShouldBeMergedAndSet == Boolean.TRUE) {
float lhsMaxSR;
lhsMaxSR = leftObject.getMaxSR();
float rhsMaxSR;
rhsMaxSR = rightObject.getMaxSR();
float mergedMaxSR = ((float) strategy.merge(LocatorUtils.property(leftLocator, "maxSR", lhsMaxSR), LocatorUtils.property(rightLocator, "maxSR", rhsMaxSR), lhsMaxSR, rhsMaxSR, true, true));
target.setMaxSR(mergedMaxSR);
} else {
if (maxSRShouldBeMergedAndSet == Boolean.FALSE) {
}
}
}
{
Boolean maxRecordsShouldBeMergedAndSet = strategy.shouldBeMergedAndSet(leftLocator, rightLocator, true, true);
if (maxRecordsShouldBeMergedAndSet == Boolean.TRUE) {
int lhsMaxRecords;
lhsMaxRecords = leftObject.getMaxRecords();
int rhsMaxRecords;
rhsMaxRecords = rightObject.getMaxRecords();
int mergedMaxRecords = ((int) strategy.merge(LocatorUtils.property(leftLocator, "maxRecords", lhsMaxRecords), LocatorUtils.property(rightLocator, "maxRecords", rhsMaxRecords), lhsMaxRecords, rhsMaxRecords, true, true));
target.setMaxRecords(mergedMaxRecords);
} else {
if (maxRecordsShouldBeMergedAndSet == Boolean.FALSE) {
}
}
}
{
Boolean verbosityShouldBeMergedAndSet = strategy.shouldBeMergedAndSet(leftLocator, rightLocator, true, true);
if (verbosityShouldBeMergedAndSet == Boolean.TRUE) {
boolean lhsVerbosity;
lhsVerbosity = leftObject.isVerbosity();
boolean rhsVerbosity;
rhsVerbosity = rightObject.isVerbosity();
boolean mergedVerbosity = ((boolean) strategy.merge(LocatorUtils.property(leftLocator, "verbosity", lhsVerbosity), LocatorUtils.property(rightLocator, "verbosity", rhsVerbosity), lhsVerbosity, rhsVerbosity, true, true));
target.setVerbosity(mergedVerbosity);
} else {
if (verbosityShouldBeMergedAndSet == Boolean.FALSE) {
}
}
}
{
Boolean testQueryShouldBeMergedAndSet = strategy.shouldBeMergedAndSet(leftLocator, rightLocator, (leftObject.testQuery!= null), (rightObject.testQuery!= null));
if (testQueryShouldBeMergedAndSet == Boolean.TRUE) {
Query lhsTestQuery;
lhsTestQuery = leftObject.getTestQuery();
Query rhsTestQuery;
rhsTestQuery = rightObject.getTestQuery();
Query mergedTestQuery = ((Query) strategy.merge(LocatorUtils.property(leftLocator, "testQuery", lhsTestQuery), LocatorUtils.property(rightLocator, "testQuery", rhsTestQuery), lhsTestQuery, rhsTestQuery, (leftObject.testQuery!= null), (rightObject.testQuery!= null)));
target.setTestQuery(mergedTestQuery);
} else {
if (testQueryShouldBeMergedAndSet == Boolean.FALSE) {
target.testQuery = null;
}
}
}
}
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Object createNewInstance() {
return new ConeSearch();
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public ConeSearch clone() {
final ConeSearch _newObject;
_newObject = ((ConeSearch) super.clone());
_newObject.testQuery = ((this.testQuery == null)?null:this.testQuery.clone());
return _newObject;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public ConeSearch createCopy() {
final ConeSearch _newObject = ((ConeSearch) super.createCopy());
_newObject.maxSR = this.maxSR;
_newObject.maxRecords = this.maxRecords;
_newObject.verbosity = this.verbosity;
_newObject.testQuery = ((this.testQuery == null)?null:this.testQuery.createCopy());
return _newObject;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public ConeSearch createCopy(final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
final ConeSearch _newObject = ((ConeSearch) super.createCopy(_propertyTree, _propertyTreeUse));
final PropertyTree maxSRPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("maxSR"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(maxSRPropertyTree!= null):((maxSRPropertyTree == null)||(!maxSRPropertyTree.isLeaf())))) {
_newObject.maxSR = this.maxSR;
}
final PropertyTree maxRecordsPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("maxRecords"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(maxRecordsPropertyTree!= null):((maxRecordsPropertyTree == null)||(!maxRecordsPropertyTree.isLeaf())))) {
_newObject.maxRecords = this.maxRecords;
}
final PropertyTree verbosityPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("verbosity"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(verbosityPropertyTree!= null):((verbosityPropertyTree == null)||(!verbosityPropertyTree.isLeaf())))) {
_newObject.verbosity = this.verbosity;
}
final PropertyTree testQueryPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("testQuery"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(testQueryPropertyTree!= null):((testQueryPropertyTree == null)||(!testQueryPropertyTree.isLeaf())))) {
_newObject.testQuery = ((this.testQuery == null)?null:this.testQuery.createCopy(testQueryPropertyTree, _propertyTreeUse));
}
return _newObject;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public ConeSearch copyExcept(final PropertyTree _propertyTree) {
return createCopy(_propertyTree, PropertyTreeUse.EXCLUDE);
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public ConeSearch copyOnly(final PropertyTree _propertyTree) {
return createCopy(_propertyTree, PropertyTreeUse.INCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public ConeSearch.Modifier modifier() {
if (null == this.__cachedModifier__) {
this.__cachedModifier__ = new ConeSearch.Modifier();
}
return ((ConeSearch.Modifier) this.__cachedModifier__);
}
/**
* Copies all state of this object to a builder. This method is used by the copyOf
* method and should not be called directly by client code.
*
* @param _other
* A builder instance to which the state of this object will be copied.
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public<_B >void copyTo(final ConeSearch.Builder<_B> _other) {
super.copyTo(_other);
_other.maxSR = this.maxSR;
_other.maxRecords = this.maxRecords;
_other.verbosity = this.verbosity;
_other.testQuery = ((this.testQuery == null)?null:this.testQuery.newCopyBuilder(_other));
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public<_B >ConeSearch.Builder<_B> newCopyBuilder(final _B _parentBuilder) {
return new ConeSearch.Builder<_B>(_parentBuilder, this, true);
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public ConeSearch.Builder newCopyBuilder() {
return newCopyBuilder(null);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static ConeSearch.Builder builder() {
return new ConeSearch.Builder<>(null, null, false);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static<_B >ConeSearch.Builder<_B> copyOf(final Capability _other) {
final ConeSearch.Builder<_B> _newBuilder = new ConeSearch.Builder<>(null, null, false);
_other.copyTo(_newBuilder);
return _newBuilder;
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static<_B >ConeSearch.Builder<_B> copyOf(final CSCapRestriction _other) {
final ConeSearch.Builder<_B> _newBuilder = new ConeSearch.Builder<>(null, null, false);
_other.copyTo(_newBuilder);
return _newBuilder;
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static<_B >ConeSearch.Builder<_B> copyOf(final ConeSearch _other) {
final ConeSearch.Builder<_B> _newBuilder = new ConeSearch.Builder<>(null, null, false);
_other.copyTo(_newBuilder);
return _newBuilder;
}
/**
* Copies all state of this object to a builder. This method is used by the copyOf
* method and should not be called directly by client code.
*
* @param _other
* A builder instance to which the state of this object will be copied.
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public<_B >void copyTo(final ConeSearch.Builder<_B> _other, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
super.copyTo(_other, _propertyTree, _propertyTreeUse);
final PropertyTree maxSRPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("maxSR"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(maxSRPropertyTree!= null):((maxSRPropertyTree == null)||(!maxSRPropertyTree.isLeaf())))) {
_other.maxSR = this.maxSR;
}
final PropertyTree maxRecordsPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("maxRecords"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(maxRecordsPropertyTree!= null):((maxRecordsPropertyTree == null)||(!maxRecordsPropertyTree.isLeaf())))) {
_other.maxRecords = this.maxRecords;
}
final PropertyTree verbosityPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("verbosity"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(verbosityPropertyTree!= null):((verbosityPropertyTree == null)||(!verbosityPropertyTree.isLeaf())))) {
_other.verbosity = this.verbosity;
}
final PropertyTree testQueryPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("testQuery"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(testQueryPropertyTree!= null):((testQueryPropertyTree == null)||(!testQueryPropertyTree.isLeaf())))) {
_other.testQuery = ((this.testQuery == null)?null:this.testQuery.newCopyBuilder(_other, testQueryPropertyTree, _propertyTreeUse));
}
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public<_B >ConeSearch.Builder<_B> newCopyBuilder(final _B _parentBuilder, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
return new ConeSearch.Builder<_B>(_parentBuilder, this, true, _propertyTree, _propertyTreeUse);
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public ConeSearch.Builder newCopyBuilder(final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
return newCopyBuilder(null, _propertyTree, _propertyTreeUse);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static<_B >ConeSearch.Builder<_B> copyOf(final Capability _other, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
final ConeSearch.Builder<_B> _newBuilder = new ConeSearch.Builder<>(null, null, false);
_other.copyTo(_newBuilder, _propertyTree, _propertyTreeUse);
return _newBuilder;
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static<_B >ConeSearch.Builder<_B> copyOf(final CSCapRestriction _other, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
final ConeSearch.Builder<_B> _newBuilder = new ConeSearch.Builder<>(null, null, false);
_other.copyTo(_newBuilder, _propertyTree, _propertyTreeUse);
return _newBuilder;
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static<_B >ConeSearch.Builder<_B> copyOf(final ConeSearch _other, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
final ConeSearch.Builder<_B> _newBuilder = new ConeSearch.Builder<>(null, null, false);
_other.copyTo(_newBuilder, _propertyTree, _propertyTreeUse);
return _newBuilder;
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static ConeSearch.Builder copyExcept(final Capability _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.EXCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static ConeSearch.Builder copyExcept(final CSCapRestriction _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.EXCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static ConeSearch.Builder copyExcept(final ConeSearch _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.EXCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static ConeSearch.Builder copyOnly(final Capability _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.INCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static ConeSearch.Builder copyOnly(final CSCapRestriction _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.INCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static ConeSearch.Builder copyOnly(final ConeSearch _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.INCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public ConeSearch visit(final PropertyVisitor _visitor_) {
super.visit(_visitor_);
_visitor_.visit(new SingleProperty<>(ConeSearch.PropInfo.MAX_SR, this));
_visitor_.visit(new SingleProperty<>(ConeSearch.PropInfo.MAX_RECORDS, this));
_visitor_.visit(new SingleProperty<>(ConeSearch.PropInfo.VERBOSITY, this));
if (_visitor_.visit(new SingleProperty<>(ConeSearch.PropInfo.TEST_QUERY, this))&&(this.testQuery!= null)) {
this.testQuery.visit(_visitor_);
}
return this;
}
public static class Builder<_B >
extends CSCapRestriction.Builder<_B>
implements Buildable
{
private float maxSR;
private int maxRecords;
private boolean verbosity;
private Query.Builder> testQuery;
public Builder(final _B _parentBuilder, final ConeSearch _other, final boolean _copy) {
super(_parentBuilder, _other, _copy);
if (_other!= null) {
this.maxSR = _other.maxSR;
this.maxRecords = _other.maxRecords;
this.verbosity = _other.verbosity;
this.testQuery = ((_other.testQuery == null)?null:_other.testQuery.newCopyBuilder(this));
}
}
public Builder(final _B _parentBuilder, final ConeSearch _other, final boolean _copy, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
super(_parentBuilder, _other, _copy, _propertyTree, _propertyTreeUse);
if (_other!= null) {
final PropertyTree maxSRPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("maxSR"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(maxSRPropertyTree!= null):((maxSRPropertyTree == null)||(!maxSRPropertyTree.isLeaf())))) {
this.maxSR = _other.maxSR;
}
final PropertyTree maxRecordsPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("maxRecords"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(maxRecordsPropertyTree!= null):((maxRecordsPropertyTree == null)||(!maxRecordsPropertyTree.isLeaf())))) {
this.maxRecords = _other.maxRecords;
}
final PropertyTree verbosityPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("verbosity"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(verbosityPropertyTree!= null):((verbosityPropertyTree == null)||(!verbosityPropertyTree.isLeaf())))) {
this.verbosity = _other.verbosity;
}
final PropertyTree testQueryPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("testQuery"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(testQueryPropertyTree!= null):((testQueryPropertyTree == null)||(!testQueryPropertyTree.isLeaf())))) {
this.testQuery = ((_other.testQuery == null)?null:_other.testQuery.newCopyBuilder(this, testQueryPropertyTree, _propertyTreeUse));
}
}
}
protected<_P extends ConeSearch >_P init(final _P _product) {
_product.maxSR = this.maxSR;
_product.maxRecords = this.maxRecords;
_product.verbosity = this.verbosity;
_product.testQuery = ((this.testQuery == null)?null:this.testQuery.build());
return super.init(_product);
}
/**
* Sets the new value of "maxSR" (any previous value will be replaced)
*
* @param maxSR
* New value of the "maxSR" property.
*/
public ConeSearch.Builder<_B> withMaxSR(final float maxSR) {
this.maxSR = maxSR;
return this;
}
/**
* Sets the new value of "maxRecords" (any previous value will be replaced)
*
* @param maxRecords
* New value of the "maxRecords" property.
*/
public ConeSearch.Builder<_B> withMaxRecords(final int maxRecords) {
this.maxRecords = maxRecords;
return this;
}
/**
* Sets the new value of "verbosity" (any previous value will be replaced)
*
* @param verbosity
* New value of the "verbosity" property.
*/
public ConeSearch.Builder<_B> withVerbosity(final boolean verbosity) {
this.verbosity = verbosity;
return this;
}
/**
* Sets the new value of "testQuery" (any previous value will be replaced)
*
* @param testQuery
* New value of the "testQuery" property.
*/
public ConeSearch.Builder<_B> withTestQuery(final Query testQuery) {
this.testQuery = ((testQuery == null)?null:new Query.Builder<>(this, testQuery, false));
return this;
}
/**
* Returns the existing builder or a new builder to build the value of the
* "testQuery" property.
* Use {@link org.javastro.ivoa.entities.resource.cone.Query.Builder#end()} to
* return to the current builder.
*
* @return
* A new builder to build the value of the "testQuery" property.
* Use {@link org.javastro.ivoa.entities.resource.cone.Query.Builder#end()} to
* return to the current builder.
*/
public Query.Builder extends ConeSearch.Builder<_B>> withTestQuery() {
if (this.testQuery!= null) {
return this.testQuery;
}
return this.testQuery = new Query.Builder<>(this, null, false);
}
/**
* Adds the given items to the value of "validationLevels"
*
* @param validationLevels
* Items to add to the value of the "validationLevels" property
*/
@Override
public ConeSearch.Builder<_B> addValidationLevels(final Iterable extends Validation> validationLevels) {
super.addValidationLevels(validationLevels);
return this;
}
/**
* Adds the given items to the value of "validationLevels"
*
* @param validationLevels
* Items to add to the value of the "validationLevels" property
*/
@Override
public ConeSearch.Builder<_B> addValidationLevels(Validation... validationLevels) {
super.addValidationLevels(validationLevels);
return this;
}
/**
* Sets the new value of "validationLevels" (any previous value will be replaced)
*
* @param validationLevels
* New value of the "validationLevels" property.
*/
@Override
public ConeSearch.Builder<_B> withValidationLevels(final Iterable extends Validation> validationLevels) {
super.withValidationLevels(validationLevels);
return this;
}
/**
* Sets the new value of "validationLevels" (any previous value will be replaced)
*
* @param validationLevels
* New value of the "validationLevels" property.
*/
@Override
public ConeSearch.Builder<_B> withValidationLevels(Validation... validationLevels) {
super.withValidationLevels(validationLevels);
return this;
}
/**
* Sets the new value of "description" (any previous value will be replaced)
*
* @param description
* New value of the "description" property.
*/
@Override
public ConeSearch.Builder<_B> withDescription(final String description) {
super.withDescription(description);
return this;
}
/**
* Adds the given items to the value of "interfaces"
*
* @param interfaces
* Items to add to the value of the "interfaces" property
*/
@Override
public ConeSearch.Builder<_B> addInterfaces(final Iterable extends ServiceInterface> interfaces) {
super.addInterfaces(interfaces);
return this;
}
/**
* Adds the given items to the value of "interfaces"
*
* @param interfaces
* Items to add to the value of the "interfaces" property
*/
@Override
public ConeSearch.Builder<_B> addInterfaces(ServiceInterface... interfaces) {
super.addInterfaces(interfaces);
return this;
}
/**
* Sets the new value of "interfaces" (any previous value will be replaced)
*
* @param interfaces
* New value of the "interfaces" property.
*/
@Override
public ConeSearch.Builder<_B> withInterfaces(final Iterable extends ServiceInterface> interfaces) {
super.withInterfaces(interfaces);
return this;
}
/**
* Sets the new value of "interfaces" (any previous value will be replaced)
*
* @param interfaces
* New value of the "interfaces" property.
*/
@Override
public ConeSearch.Builder<_B> withInterfaces(ServiceInterface... interfaces) {
super.withInterfaces(interfaces);
return this;
}
/**
* Sets the new value of "standardID" (any previous value will be replaced)
*
* @param standardID
* New value of the "standardID" property.
*/
@Override
public ConeSearch.Builder<_B> withStandardID(final String standardID) {
super.withStandardID(standardID);
return this;
}
@Override
public ConeSearch build() {
if (_storedValue == null) {
return this.init(new ConeSearch());
} else {
return ((ConeSearch) _storedValue);
}
}
public ConeSearch.Builder<_B> copyOf(final ConeSearch _other) {
_other.copyTo(this);
return this;
}
public ConeSearch.Builder<_B> copyOf(final ConeSearch.Builder _other) {
return copyOf(_other.build());
}
}
public class Modifier
extends CSCapRestriction.Modifier
{
public void setMaxSR(final float maxSR) {
ConeSearch.this.setMaxSR(maxSR);
}
public void setMaxRecords(final int maxRecords) {
ConeSearch.this.setMaxRecords(maxRecords);
}
public void setVerbosity(final boolean verbosity) {
ConeSearch.this.setVerbosity(verbosity);
}
public void setTestQuery(final Query testQuery) {
ConeSearch.this.setTestQuery(testQuery);
}
}
public static class PropInfo {
public static final transient SinglePropertyInfo MAX_SR = new SinglePropertyInfo("maxSR", ConeSearch.class, Float.class, false, null, new QName("", "maxSR"), new QName("http://www.w3.org/2001/XMLSchema", "float"), false) {
@Override
public Float get(final ConeSearch _instance_) {
return ((_instance_ == null)?null:_instance_.maxSR);
}
@Override
public void set(final ConeSearch _instance_, final Float _value_) {
if (_instance_!= null) {
_instance_.maxSR = _value_;
}
}
}
;
public static final transient SinglePropertyInfo MAX_RECORDS = new SinglePropertyInfo("maxRecords", ConeSearch.class, Integer.class, false, null, new QName("", "maxRecords"), new QName("http://www.w3.org/2001/XMLSchema", "int"), false) {
@Override
public Integer get(final ConeSearch _instance_) {
return ((_instance_ == null)?null:_instance_.maxRecords);
}
@Override
public void set(final ConeSearch _instance_, final Integer _value_) {
if (_instance_!= null) {
_instance_.maxRecords = _value_;
}
}
}
;
public static final transient SinglePropertyInfo VERBOSITY = new SinglePropertyInfo("verbosity", ConeSearch.class, Boolean.class, false, null, new QName("", "verbosity"), new QName("http://www.w3.org/2001/XMLSchema", "boolean"), false) {
@Override
public Boolean get(final ConeSearch _instance_) {
return ((_instance_ == null)?null:_instance_.verbosity);
}
@Override
public void set(final ConeSearch _instance_, final Boolean _value_) {
if (_instance_!= null) {
_instance_.verbosity = _value_;
}
}
}
;
public static final transient SinglePropertyInfo TEST_QUERY = new SinglePropertyInfo("testQuery", ConeSearch.class, Query.class, false, null, new QName("", "testQuery"), new QName("http://www.ivoa.net/xml/ConeSearch/v1.0", "Query"), false) {
@Override
public Query get(final ConeSearch _instance_) {
return ((_instance_ == null)?null:_instance_.testQuery);
}
@Override
public void set(final ConeSearch _instance_, final Query _value_) {
if (_instance_!= null) {
_instance_.testQuery = _value_;
}
}
}
;
}
public static class Select
extends ConeSearch.Selector
{
Select() {
super(null, null, null);
}
public static ConeSearch.Select _root() {
return new ConeSearch.Select();
}
}
public static class Selector , TParent >
extends CSCapRestriction.Selector
{
private Query.Selector> testQuery = null;
public Selector(final TRoot root, final TParent parent, final String propertyName) {
super(root, parent, propertyName);
}
@Override
public Map buildChildren() {
final Map products = new HashMap<>();
products.putAll(super.buildChildren());
if (this.testQuery!= null) {
products.put("testQuery", this.testQuery.init());
}
return products;
}
public Query.Selector> testQuery() {
return ((this.testQuery == null)?this.testQuery = new Query.Selector<>(this._root, this, "testQuery"):this.testQuery);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy