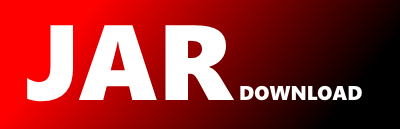
org.javastro.ivoa.entities.resource.dataservice.BaseParam Maven / Gradle / Ivy
package org.javastro.ivoa.entities.resource.dataservice;
import java.util.HashMap;
import java.util.Map;
import javax.xml.namespace.QName;
import com.kscs.util.jaxb.Buildable;
import com.kscs.util.jaxb.Copyable;
import com.kscs.util.jaxb.PartialCopyable;
import com.kscs.util.jaxb.PropertyTree;
import com.kscs.util.jaxb.PropertyTreeUse;
import com.kscs.util.jaxb.PropertyVisitor;
import com.kscs.util.jaxb.SingleProperty;
import com.kscs.util.jaxb.SinglePropertyInfo;
import com.kscs.util.jaxb.SinglePropertyInfo;
import jakarta.annotation.Generated;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlAnyAttribute;
import jakarta.xml.bind.annotation.XmlSchemaType;
import jakarta.xml.bind.annotation.XmlSeeAlso;
import jakarta.xml.bind.annotation.XmlType;
import jakarta.xml.bind.annotation.adapters.CollapsedStringAdapter;
import jakarta.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import org.jvnet.jaxb.lang.JAXBMergeStrategy;
import org.jvnet.jaxb.lang.JAXBToStringStrategy;
import org.jvnet.jaxb.lang.MergeFrom;
import org.jvnet.jaxb.lang.MergeStrategy;
import org.jvnet.jaxb.lang.ToString;
import org.jvnet.jaxb.lang.ToStringStrategy;
import org.jvnet.jaxb.locator.ObjectLocator;
import org.jvnet.jaxb.locator.util.LocatorUtils;
/**
* As the parameter's data type is usually important, schemas
* normally employ a sub-class of this type
* rather than this type directly.
*
* Java class for BaseParam complex type
.
*
* The following schema fragment specifies the expected content contained within this class.
*
* {@code
*
*
*
*
*
*
*
*
*
*
*
*
*
*
* }
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "BaseParam", propOrder = {
"name",
"description",
"unit",
"ucd",
"utype"
})
@XmlSeeAlso({
TableParam.class,
InputParam.class
})
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public class BaseParam implements Cloneable, Copyable, PartialCopyable, MergeFrom, ToString
{
/**
* The name of the parameter or column.
*
*/
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
@XmlSchemaType(name = "token")
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
protected String name;
/**
* A free-text description of a parameter's or column's
* contents.
*
*/
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
@XmlSchemaType(name = "token")
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
protected String description;
/**
* The unit associated with the values in the parameter
* or column.
*
*/
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
@XmlSchemaType(name = "token")
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
protected String unit;
/**
* There are no requirements for compliance with any
* particular UCD standard. The format of the UCD can
* be used to distinguish between UCD1, UCD1+, and
* SIA-UCD. See
* http://ivoa.net/Documents/latest/UCDlist.html
* for the latest IVOA standard set.
*
*/
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
@XmlSchemaType(name = "token")
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
protected String ucd;
/**
* The form of the utype string depends on the data
* model; common forms are sequences of dotted identifiers
* (e.g., in SSA) or URIs (e.g., in RegTAP).
*
*/
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
@XmlSchemaType(name = "token")
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
protected String utype;
@XmlAnyAttribute
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
private Map otherAttributes = new HashMap<>();
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
protected transient BaseParam.Modifier __cachedModifier__;
/**
* Default no-arg constructor
*
*/
public BaseParam() {
super();
}
/**
* Fully-initialising value constructor
*
*/
public BaseParam(final String name, final String description, final String unit, final String ucd, final String utype, final Map otherAttributes) {
this.name = name;
this.description = description;
this.unit = unit;
this.ucd = ucd;
this.utype = utype;
this.otherAttributes = otherAttributes;
}
/**
* The name of the parameter or column.
*
* @return
* possible object is
* {@link String }
*
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public String getName() {
return name;
}
/**
* Sets the value of the name property.
*
* @param value
* allowed object is
* {@link String }
*
* @see #getName()
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void setName(String value) {
this.name = value;
}
/**
* A free-text description of a parameter's or column's
* contents.
*
* @return
* possible object is
* {@link String }
*
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public String getDescription() {
return description;
}
/**
* Sets the value of the description property.
*
* @param value
* allowed object is
* {@link String }
*
* @see #getDescription()
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void setDescription(String value) {
this.description = value;
}
/**
* The unit associated with the values in the parameter
* or column.
*
* @return
* possible object is
* {@link String }
*
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public String getUnit() {
return unit;
}
/**
* Sets the value of the unit property.
*
* @param value
* allowed object is
* {@link String }
*
* @see #getUnit()
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void setUnit(String value) {
this.unit = value;
}
/**
* There are no requirements for compliance with any
* particular UCD standard. The format of the UCD can
* be used to distinguish between UCD1, UCD1+, and
* SIA-UCD. See
* http://ivoa.net/Documents/latest/UCDlist.html
* for the latest IVOA standard set.
*
* @return
* possible object is
* {@link String }
*
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public String getUcd() {
return ucd;
}
/**
* Sets the value of the ucd property.
*
* @param value
* allowed object is
* {@link String }
*
* @see #getUcd()
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void setUcd(String value) {
this.ucd = value;
}
/**
* The form of the utype string depends on the data
* model; common forms are sequences of dotted identifiers
* (e.g., in SSA) or URIs (e.g., in RegTAP).
*
* @return
* possible object is
* {@link String }
*
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public String getUtype() {
return utype;
}
/**
* Sets the value of the utype property.
*
* @param value
* allowed object is
* {@link String }
*
* @see #getUtype()
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void setUtype(String value) {
this.utype = value;
}
/**
* Gets a map that contains attributes that aren't bound to any typed property on this class.
*
*
* the map is keyed by the name of the attribute and
* the value is the string value of the attribute.
*
* the map returned by this method is live, and you can add new attribute
* by updating the map directly. Because of this design, there's no setter.
*
*
* @return
* always non-null
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Map getOtherAttributes() {
return otherAttributes;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public boolean equals(Object object) {
if ((object == null)||(this.getClass()!= object.getClass())) {
return false;
}
if (this == object) {
return true;
}
final BaseParam that = ((BaseParam) object);
{
String leftName;
leftName = this.getName();
String rightName;
rightName = that.getName();
if (this.name!= null) {
if (that.name!= null) {
if (!leftName.equals(rightName)) {
return false;
}
} else {
return false;
}
} else {
if (that.name!= null) {
return false;
}
}
}
{
String leftDescription;
leftDescription = this.getDescription();
String rightDescription;
rightDescription = that.getDescription();
if (this.description!= null) {
if (that.description!= null) {
if (!leftDescription.equals(rightDescription)) {
return false;
}
} else {
return false;
}
} else {
if (that.description!= null) {
return false;
}
}
}
{
String leftUnit;
leftUnit = this.getUnit();
String rightUnit;
rightUnit = that.getUnit();
if (this.unit!= null) {
if (that.unit!= null) {
if (!leftUnit.equals(rightUnit)) {
return false;
}
} else {
return false;
}
} else {
if (that.unit!= null) {
return false;
}
}
}
{
String leftUcd;
leftUcd = this.getUcd();
String rightUcd;
rightUcd = that.getUcd();
if (this.ucd!= null) {
if (that.ucd!= null) {
if (!leftUcd.equals(rightUcd)) {
return false;
}
} else {
return false;
}
} else {
if (that.ucd!= null) {
return false;
}
}
}
{
String leftUtype;
leftUtype = this.getUtype();
String rightUtype;
rightUtype = that.getUtype();
if (this.utype!= null) {
if (that.utype!= null) {
if (!leftUtype.equals(rightUtype)) {
return false;
}
} else {
return false;
}
} else {
if (that.utype!= null) {
return false;
}
}
}
return true;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public int hashCode() {
int currentHashCode = 1;
{
currentHashCode = (currentHashCode* 31);
String theName;
theName = this.getName();
if (this.name!= null) {
currentHashCode += theName.hashCode();
}
}
{
currentHashCode = (currentHashCode* 31);
String theDescription;
theDescription = this.getDescription();
if (this.description!= null) {
currentHashCode += theDescription.hashCode();
}
}
{
currentHashCode = (currentHashCode* 31);
String theUnit;
theUnit = this.getUnit();
if (this.unit!= null) {
currentHashCode += theUnit.hashCode();
}
}
{
currentHashCode = (currentHashCode* 31);
String theUcd;
theUcd = this.getUcd();
if (this.ucd!= null) {
currentHashCode += theUcd.hashCode();
}
}
{
currentHashCode = (currentHashCode* 31);
String theUtype;
theUtype = this.getUtype();
if (this.utype!= null) {
currentHashCode += theUtype.hashCode();
}
}
return currentHashCode;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public String toString() {
final ToStringStrategy strategy = JAXBToStringStrategy.getInstance();
final StringBuilder buffer = new StringBuilder();
append(null, buffer, strategy);
return buffer.toString();
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public StringBuilder append(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
strategy.appendStart(locator, this, buffer);
appendFields(locator, buffer, strategy);
strategy.appendEnd(locator, this, buffer);
return buffer;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public StringBuilder appendFields(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
{
String theName;
theName = this.getName();
strategy.appendField(locator, this, "name", buffer, theName, (this.name!= null));
}
{
String theDescription;
theDescription = this.getDescription();
strategy.appendField(locator, this, "description", buffer, theDescription, (this.description!= null));
}
{
String theUnit;
theUnit = this.getUnit();
strategy.appendField(locator, this, "unit", buffer, theUnit, (this.unit!= null));
}
{
String theUcd;
theUcd = this.getUcd();
strategy.appendField(locator, this, "ucd", buffer, theUcd, (this.ucd!= null));
}
{
String theUtype;
theUtype = this.getUtype();
strategy.appendField(locator, this, "utype", buffer, theUtype, (this.utype!= null));
}
return buffer;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void mergeFrom(Object left, Object right) {
final MergeStrategy strategy = JAXBMergeStrategy.getInstance();
mergeFrom(null, null, left, right, strategy);
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void mergeFrom(ObjectLocator leftLocator, ObjectLocator rightLocator, Object left, Object right, MergeStrategy strategy) {
if (right instanceof BaseParam) {
final BaseParam target = this;
final BaseParam leftObject = ((BaseParam) left);
final BaseParam rightObject = ((BaseParam) right);
{
Boolean nameShouldBeMergedAndSet = strategy.shouldBeMergedAndSet(leftLocator, rightLocator, (leftObject.name!= null), (rightObject.name!= null));
if (nameShouldBeMergedAndSet == Boolean.TRUE) {
String lhsName;
lhsName = leftObject.getName();
String rhsName;
rhsName = rightObject.getName();
String mergedName = ((String) strategy.merge(LocatorUtils.property(leftLocator, "name", lhsName), LocatorUtils.property(rightLocator, "name", rhsName), lhsName, rhsName, (leftObject.name!= null), (rightObject.name!= null)));
target.setName(mergedName);
} else {
if (nameShouldBeMergedAndSet == Boolean.FALSE) {
target.name = null;
}
}
}
{
Boolean descriptionShouldBeMergedAndSet = strategy.shouldBeMergedAndSet(leftLocator, rightLocator, (leftObject.description!= null), (rightObject.description!= null));
if (descriptionShouldBeMergedAndSet == Boolean.TRUE) {
String lhsDescription;
lhsDescription = leftObject.getDescription();
String rhsDescription;
rhsDescription = rightObject.getDescription();
String mergedDescription = ((String) strategy.merge(LocatorUtils.property(leftLocator, "description", lhsDescription), LocatorUtils.property(rightLocator, "description", rhsDescription), lhsDescription, rhsDescription, (leftObject.description!= null), (rightObject.description!= null)));
target.setDescription(mergedDescription);
} else {
if (descriptionShouldBeMergedAndSet == Boolean.FALSE) {
target.description = null;
}
}
}
{
Boolean unitShouldBeMergedAndSet = strategy.shouldBeMergedAndSet(leftLocator, rightLocator, (leftObject.unit!= null), (rightObject.unit!= null));
if (unitShouldBeMergedAndSet == Boolean.TRUE) {
String lhsUnit;
lhsUnit = leftObject.getUnit();
String rhsUnit;
rhsUnit = rightObject.getUnit();
String mergedUnit = ((String) strategy.merge(LocatorUtils.property(leftLocator, "unit", lhsUnit), LocatorUtils.property(rightLocator, "unit", rhsUnit), lhsUnit, rhsUnit, (leftObject.unit!= null), (rightObject.unit!= null)));
target.setUnit(mergedUnit);
} else {
if (unitShouldBeMergedAndSet == Boolean.FALSE) {
target.unit = null;
}
}
}
{
Boolean ucdShouldBeMergedAndSet = strategy.shouldBeMergedAndSet(leftLocator, rightLocator, (leftObject.ucd!= null), (rightObject.ucd!= null));
if (ucdShouldBeMergedAndSet == Boolean.TRUE) {
String lhsUcd;
lhsUcd = leftObject.getUcd();
String rhsUcd;
rhsUcd = rightObject.getUcd();
String mergedUcd = ((String) strategy.merge(LocatorUtils.property(leftLocator, "ucd", lhsUcd), LocatorUtils.property(rightLocator, "ucd", rhsUcd), lhsUcd, rhsUcd, (leftObject.ucd!= null), (rightObject.ucd!= null)));
target.setUcd(mergedUcd);
} else {
if (ucdShouldBeMergedAndSet == Boolean.FALSE) {
target.ucd = null;
}
}
}
{
Boolean utypeShouldBeMergedAndSet = strategy.shouldBeMergedAndSet(leftLocator, rightLocator, (leftObject.utype!= null), (rightObject.utype!= null));
if (utypeShouldBeMergedAndSet == Boolean.TRUE) {
String lhsUtype;
lhsUtype = leftObject.getUtype();
String rhsUtype;
rhsUtype = rightObject.getUtype();
String mergedUtype = ((String) strategy.merge(LocatorUtils.property(leftLocator, "utype", lhsUtype), LocatorUtils.property(rightLocator, "utype", rhsUtype), lhsUtype, rhsUtype, (leftObject.utype!= null), (rightObject.utype!= null)));
target.setUtype(mergedUtype);
} else {
if (utypeShouldBeMergedAndSet == Boolean.FALSE) {
target.utype = null;
}
}
}
}
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Object createNewInstance() {
return new BaseParam();
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public BaseParam clone() {
final BaseParam _newObject;
try {
_newObject = ((BaseParam) super.clone());
} catch (CloneNotSupportedException e) {
throw new RuntimeException(e);
}
return _newObject;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public BaseParam createCopy() {
final BaseParam _newObject;
try {
_newObject = ((BaseParam) super.clone());
} catch (CloneNotSupportedException e) {
throw new RuntimeException(e);
}
_newObject.name = this.name;
_newObject.description = this.description;
_newObject.unit = this.unit;
_newObject.ucd = this.ucd;
_newObject.utype = this.utype;
return _newObject;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public BaseParam createCopy(final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
final BaseParam _newObject;
try {
_newObject = ((BaseParam) super.clone());
} catch (CloneNotSupportedException e) {
throw new RuntimeException(e);
}
final PropertyTree namePropertyTree = ((_propertyTree == null)?null:_propertyTree.get("name"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(namePropertyTree!= null):((namePropertyTree == null)||(!namePropertyTree.isLeaf())))) {
_newObject.name = this.name;
}
final PropertyTree descriptionPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("description"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(descriptionPropertyTree!= null):((descriptionPropertyTree == null)||(!descriptionPropertyTree.isLeaf())))) {
_newObject.description = this.description;
}
final PropertyTree unitPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("unit"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(unitPropertyTree!= null):((unitPropertyTree == null)||(!unitPropertyTree.isLeaf())))) {
_newObject.unit = this.unit;
}
final PropertyTree ucdPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("ucd"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(ucdPropertyTree!= null):((ucdPropertyTree == null)||(!ucdPropertyTree.isLeaf())))) {
_newObject.ucd = this.ucd;
}
final PropertyTree utypePropertyTree = ((_propertyTree == null)?null:_propertyTree.get("utype"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(utypePropertyTree!= null):((utypePropertyTree == null)||(!utypePropertyTree.isLeaf())))) {
_newObject.utype = this.utype;
}
return _newObject;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public BaseParam copyExcept(final PropertyTree _propertyTree) {
return createCopy(_propertyTree, PropertyTreeUse.EXCLUDE);
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public BaseParam copyOnly(final PropertyTree _propertyTree) {
return createCopy(_propertyTree, PropertyTreeUse.INCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public BaseParam.Modifier modifier() {
if (null == this.__cachedModifier__) {
this.__cachedModifier__ = new BaseParam.Modifier();
}
return ((BaseParam.Modifier) this.__cachedModifier__);
}
/**
* Copies all state of this object to a builder. This method is used by the copyOf
* method and should not be called directly by client code.
*
* @param _other
* A builder instance to which the state of this object will be copied.
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public<_B >void copyTo(final BaseParam.Builder<_B> _other) {
_other.name = this.name;
_other.description = this.description;
_other.unit = this.unit;
_other.ucd = this.ucd;
_other.utype = this.utype;
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public<_B >BaseParam.Builder<_B> newCopyBuilder(final _B _parentBuilder) {
return new BaseParam.Builder<_B>(_parentBuilder, this, true);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public BaseParam.Builder newCopyBuilder() {
return newCopyBuilder(null);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static BaseParam.Builder builder() {
return new BaseParam.Builder<>(null, null, false);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static<_B >BaseParam.Builder<_B> copyOf(final BaseParam _other) {
final BaseParam.Builder<_B> _newBuilder = new BaseParam.Builder<>(null, null, false);
_other.copyTo(_newBuilder);
return _newBuilder;
}
/**
* Copies all state of this object to a builder. This method is used by the copyOf
* method and should not be called directly by client code.
*
* @param _other
* A builder instance to which the state of this object will be copied.
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public<_B >void copyTo(final BaseParam.Builder<_B> _other, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
final PropertyTree namePropertyTree = ((_propertyTree == null)?null:_propertyTree.get("name"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(namePropertyTree!= null):((namePropertyTree == null)||(!namePropertyTree.isLeaf())))) {
_other.name = this.name;
}
final PropertyTree descriptionPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("description"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(descriptionPropertyTree!= null):((descriptionPropertyTree == null)||(!descriptionPropertyTree.isLeaf())))) {
_other.description = this.description;
}
final PropertyTree unitPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("unit"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(unitPropertyTree!= null):((unitPropertyTree == null)||(!unitPropertyTree.isLeaf())))) {
_other.unit = this.unit;
}
final PropertyTree ucdPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("ucd"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(ucdPropertyTree!= null):((ucdPropertyTree == null)||(!ucdPropertyTree.isLeaf())))) {
_other.ucd = this.ucd;
}
final PropertyTree utypePropertyTree = ((_propertyTree == null)?null:_propertyTree.get("utype"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(utypePropertyTree!= null):((utypePropertyTree == null)||(!utypePropertyTree.isLeaf())))) {
_other.utype = this.utype;
}
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public<_B >BaseParam.Builder<_B> newCopyBuilder(final _B _parentBuilder, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
return new BaseParam.Builder<_B>(_parentBuilder, this, true, _propertyTree, _propertyTreeUse);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public BaseParam.Builder newCopyBuilder(final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
return newCopyBuilder(null, _propertyTree, _propertyTreeUse);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static<_B >BaseParam.Builder<_B> copyOf(final BaseParam _other, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
final BaseParam.Builder<_B> _newBuilder = new BaseParam.Builder<>(null, null, false);
_other.copyTo(_newBuilder, _propertyTree, _propertyTreeUse);
return _newBuilder;
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static BaseParam.Builder copyExcept(final BaseParam _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.EXCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static BaseParam.Builder copyOnly(final BaseParam _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.INCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public BaseParam visit(final PropertyVisitor _visitor_) {
_visitor_.visit(this);
_visitor_.visit(new SingleProperty<>(BaseParam.PropInfo.NAME, this));
_visitor_.visit(new SingleProperty<>(BaseParam.PropInfo.DESCRIPTION, this));
_visitor_.visit(new SingleProperty<>(BaseParam.PropInfo.UNIT, this));
_visitor_.visit(new SingleProperty<>(BaseParam.PropInfo.UCD, this));
_visitor_.visit(new SingleProperty<>(BaseParam.PropInfo.UTYPE, this));
return this;
}
public static class Builder<_B >implements Buildable
{
protected final _B _parentBuilder;
protected final BaseParam _storedValue;
private String name;
private String description;
private String unit;
private String ucd;
private String utype;
public Builder(final _B _parentBuilder, final BaseParam _other, final boolean _copy) {
this._parentBuilder = _parentBuilder;
if (_other!= null) {
if (_copy) {
_storedValue = null;
this.name = _other.name;
this.description = _other.description;
this.unit = _other.unit;
this.ucd = _other.ucd;
this.utype = _other.utype;
} else {
_storedValue = _other;
}
} else {
_storedValue = null;
}
}
public Builder(final _B _parentBuilder, final BaseParam _other, final boolean _copy, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
this._parentBuilder = _parentBuilder;
if (_other!= null) {
if (_copy) {
_storedValue = null;
final PropertyTree namePropertyTree = ((_propertyTree == null)?null:_propertyTree.get("name"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(namePropertyTree!= null):((namePropertyTree == null)||(!namePropertyTree.isLeaf())))) {
this.name = _other.name;
}
final PropertyTree descriptionPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("description"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(descriptionPropertyTree!= null):((descriptionPropertyTree == null)||(!descriptionPropertyTree.isLeaf())))) {
this.description = _other.description;
}
final PropertyTree unitPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("unit"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(unitPropertyTree!= null):((unitPropertyTree == null)||(!unitPropertyTree.isLeaf())))) {
this.unit = _other.unit;
}
final PropertyTree ucdPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("ucd"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(ucdPropertyTree!= null):((ucdPropertyTree == null)||(!ucdPropertyTree.isLeaf())))) {
this.ucd = _other.ucd;
}
final PropertyTree utypePropertyTree = ((_propertyTree == null)?null:_propertyTree.get("utype"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(utypePropertyTree!= null):((utypePropertyTree == null)||(!utypePropertyTree.isLeaf())))) {
this.utype = _other.utype;
}
} else {
_storedValue = _other;
}
} else {
_storedValue = null;
}
}
public _B end() {
return this._parentBuilder;
}
protected<_P extends BaseParam >_P init(final _P _product) {
_product.name = this.name;
_product.description = this.description;
_product.unit = this.unit;
_product.ucd = this.ucd;
_product.utype = this.utype;
return _product;
}
/**
* Sets the new value of "name" (any previous value will be replaced)
*
* @param name
* New value of the "name" property.
*/
public BaseParam.Builder<_B> withName(final String name) {
this.name = name;
return this;
}
/**
* Sets the new value of "description" (any previous value will be replaced)
*
* @param description
* New value of the "description" property.
*/
public BaseParam.Builder<_B> withDescription(final String description) {
this.description = description;
return this;
}
/**
* Sets the new value of "unit" (any previous value will be replaced)
*
* @param unit
* New value of the "unit" property.
*/
public BaseParam.Builder<_B> withUnit(final String unit) {
this.unit = unit;
return this;
}
/**
* Sets the new value of "ucd" (any previous value will be replaced)
*
* @param ucd
* New value of the "ucd" property.
*/
public BaseParam.Builder<_B> withUcd(final String ucd) {
this.ucd = ucd;
return this;
}
/**
* Sets the new value of "utype" (any previous value will be replaced)
*
* @param utype
* New value of the "utype" property.
*/
public BaseParam.Builder<_B> withUtype(final String utype) {
this.utype = utype;
return this;
}
@Override
public BaseParam build() {
if (_storedValue == null) {
return this.init(new BaseParam());
} else {
return ((BaseParam) _storedValue);
}
}
public BaseParam.Builder<_B> copyOf(final BaseParam _other) {
_other.copyTo(this);
return this;
}
public BaseParam.Builder<_B> copyOf(final BaseParam.Builder _other) {
return copyOf(_other.build());
}
}
public class Modifier {
public void setName(final String name) {
BaseParam.this.setName(name);
}
public void setDescription(final String description) {
BaseParam.this.setDescription(description);
}
public void setUnit(final String unit) {
BaseParam.this.setUnit(unit);
}
public void setUcd(final String ucd) {
BaseParam.this.setUcd(ucd);
}
public void setUtype(final String utype) {
BaseParam.this.setUtype(utype);
}
}
public static class PropInfo {
public static final transient SinglePropertyInfo NAME = new SinglePropertyInfo("name", BaseParam.class, String.class, false, null, new QName("", "name"), new QName("http://www.w3.org/2001/XMLSchema", "token"), false) {
@Override
public String get(final BaseParam _instance_) {
return ((_instance_ == null)?null:_instance_.name);
}
@Override
public void set(final BaseParam _instance_, final String _value_) {
if (_instance_!= null) {
_instance_.name = _value_;
}
}
}
;
public static final transient SinglePropertyInfo DESCRIPTION = new SinglePropertyInfo("description", BaseParam.class, String.class, false, null, new QName("", "description"), new QName("http://www.w3.org/2001/XMLSchema", "token"), false) {
@Override
public String get(final BaseParam _instance_) {
return ((_instance_ == null)?null:_instance_.description);
}
@Override
public void set(final BaseParam _instance_, final String _value_) {
if (_instance_!= null) {
_instance_.description = _value_;
}
}
}
;
public static final transient SinglePropertyInfo UNIT = new SinglePropertyInfo("unit", BaseParam.class, String.class, false, null, new QName("", "unit"), new QName("http://www.w3.org/2001/XMLSchema", "token"), false) {
@Override
public String get(final BaseParam _instance_) {
return ((_instance_ == null)?null:_instance_.unit);
}
@Override
public void set(final BaseParam _instance_, final String _value_) {
if (_instance_!= null) {
_instance_.unit = _value_;
}
}
}
;
public static final transient SinglePropertyInfo UCD = new SinglePropertyInfo("ucd", BaseParam.class, String.class, false, null, new QName("", "ucd"), new QName("http://www.w3.org/2001/XMLSchema", "token"), false) {
@Override
public String get(final BaseParam _instance_) {
return ((_instance_ == null)?null:_instance_.ucd);
}
@Override
public void set(final BaseParam _instance_, final String _value_) {
if (_instance_!= null) {
_instance_.ucd = _value_;
}
}
}
;
public static final transient SinglePropertyInfo UTYPE = new SinglePropertyInfo("utype", BaseParam.class, String.class, false, null, new QName("", "utype"), new QName("http://www.w3.org/2001/XMLSchema", "token"), false) {
@Override
public String get(final BaseParam _instance_) {
return ((_instance_ == null)?null:_instance_.utype);
}
@Override
public void set(final BaseParam _instance_, final String _value_) {
if (_instance_!= null) {
_instance_.utype = _value_;
}
}
}
;
}
public static class Select
extends BaseParam.Selector
{
Select() {
super(null, null, null);
}
public static BaseParam.Select _root() {
return new BaseParam.Select();
}
}
public static class Selector , TParent >
extends com.kscs.util.jaxb.Selector
{
private com.kscs.util.jaxb.Selector> name = null;
private com.kscs.util.jaxb.Selector> description = null;
private com.kscs.util.jaxb.Selector> unit = null;
private com.kscs.util.jaxb.Selector> ucd = null;
private com.kscs.util.jaxb.Selector> utype = null;
public Selector(final TRoot root, final TParent parent, final String propertyName) {
super(root, parent, propertyName);
}
@Override
public Map buildChildren() {
final Map products = new HashMap<>();
products.putAll(super.buildChildren());
if (this.name!= null) {
products.put("name", this.name.init());
}
if (this.description!= null) {
products.put("description", this.description.init());
}
if (this.unit!= null) {
products.put("unit", this.unit.init());
}
if (this.ucd!= null) {
products.put("ucd", this.ucd.init());
}
if (this.utype!= null) {
products.put("utype", this.utype.init());
}
return products;
}
public com.kscs.util.jaxb.Selector> name() {
return ((this.name == null)?this.name = new com.kscs.util.jaxb.Selector<>(this._root, this, "name"):this.name);
}
public com.kscs.util.jaxb.Selector> description() {
return ((this.description == null)?this.description = new com.kscs.util.jaxb.Selector<>(this._root, this, "description"):this.description);
}
public com.kscs.util.jaxb.Selector> unit() {
return ((this.unit == null)?this.unit = new com.kscs.util.jaxb.Selector<>(this._root, this, "unit"):this.unit);
}
public com.kscs.util.jaxb.Selector> ucd() {
return ((this.ucd == null)?this.ucd = new com.kscs.util.jaxb.Selector<>(this._root, this, "ucd"):this.ucd);
}
public com.kscs.util.jaxb.Selector> utype() {
return ((this.utype == null)?this.utype = new com.kscs.util.jaxb.Selector<>(this._root, this, "utype"):this.utype);
}
}
}