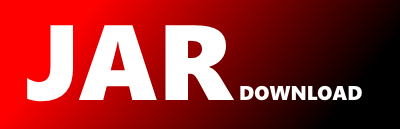
org.javastro.ivoa.entities.resource.dataservice.DataResource Maven / Gradle / Ivy
package org.javastro.ivoa.entities.resource.dataservice;
import java.time.LocalDateTime;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import javax.xml.namespace.QName;
import com.kscs.util.jaxb.Buildable;
import com.kscs.util.jaxb.CollectionProperty;
import com.kscs.util.jaxb.CollectionPropertyInfo;
import com.kscs.util.jaxb.CollectionPropertyInfo;
import com.kscs.util.jaxb.Copyable;
import com.kscs.util.jaxb.PartialCopyable;
import com.kscs.util.jaxb.PropertyTree;
import com.kscs.util.jaxb.PropertyTreeUse;
import com.kscs.util.jaxb.PropertyVisitor;
import com.kscs.util.jaxb.SingleProperty;
import com.kscs.util.jaxb.SinglePropertyInfo;
import com.kscs.util.jaxb.SinglePropertyInfo;
import jakarta.annotation.Generated;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlSeeAlso;
import jakarta.xml.bind.annotation.XmlType;
import org.javastro.ivoa.entities.resource.Capability;
import org.javastro.ivoa.entities.resource.Content;
import org.javastro.ivoa.entities.resource.Curation;
import org.javastro.ivoa.entities.resource.Resource;
import org.javastro.ivoa.entities.resource.ResourceName;
import org.javastro.ivoa.entities.resource.Rights;
import org.javastro.ivoa.entities.resource.Service;
import org.javastro.ivoa.entities.resource.Validation;
import org.jvnet.jaxb.lang.JAXBMergeStrategy;
import org.jvnet.jaxb.lang.JAXBToStringStrategy;
import org.jvnet.jaxb.lang.MergeFrom;
import org.jvnet.jaxb.lang.MergeStrategy;
import org.jvnet.jaxb.lang.ToString;
import org.jvnet.jaxb.lang.ToStringStrategy;
import org.jvnet.jaxb.locator.ObjectLocator;
import org.jvnet.jaxb.locator.util.LocatorUtils;
/**
* This resource type should only be used if the resource has no
* common underlying tabular schema (e.g., an inhomogeneous archive).
* Use CatalogResource otherwise.
*
* Java class for DataResource complex type
.
*
* The following schema fragment specifies the expected content contained within this class.
*
* {@code
*
*
*
*
*
*
*
*
*
*
*
* }
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "DataResource", propOrder = {
"facilities",
"instruments",
"coverage"
})
@XmlSeeAlso({
DataService.class,
CatalogResource.class
})
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public class DataResource
extends Service
implements Cloneable, Copyable, PartialCopyable, MergeFrom, ToString
{
/**
* The observatory or facility used to collect the data
* contained or managed by this resource.
*
*/
@XmlElement(name = "facility")
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
protected List facilities;
/**
* The instrument used to collect the data contain or
* managed by a resource.
*
*/
@XmlElement(name = "instrument")
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
protected List instruments;
/**
* Extent of the content of the resource over space, time,
* and frequency.
*
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
protected Coverage coverage;
/**
* Default no-arg constructor
*
*/
public DataResource() {
super();
}
/**
* Fully-initialising value constructor
*
*/
public DataResource(final List validationLevels, final String title, final String shortName, final String identifier, final List altIdentifiers, final Curation curation, final Content content, final LocalDateTime created, final LocalDateTime updated, final String status, final String version, final List rights, final List capabilities, final List facilities, final List instruments, final Coverage coverage) {
super(validationLevels, title, shortName, identifier, altIdentifiers, curation, content, created, updated, status, version, rights, capabilities);
this.facilities = facilities;
this.instruments = instruments;
this.coverage = coverage;
}
/**
* The observatory or facility used to collect the data
* contained or managed by this resource.
*
* Gets the value of the facilities property.
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the facilities property.
*
*
* For example, to add a new item, do as follows:
*
*
* getFacilities().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link ResourceName }
*
*
*
* @return
* The value of the facilities property.
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public List getFacilities() {
if (facilities == null) {
facilities = new ArrayList<>();
}
return this.facilities;
}
/**
* The instrument used to collect the data contain or
* managed by a resource.
*
* Gets the value of the instruments property.
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the instruments property.
*
*
* For example, to add a new item, do as follows:
*
*
* getInstruments().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link ResourceName }
*
*
*
* @return
* The value of the instruments property.
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public List getInstruments() {
if (instruments == null) {
instruments = new ArrayList<>();
}
return this.instruments;
}
/**
* Extent of the content of the resource over space, time,
* and frequency.
*
* @return
* possible object is
* {@link Coverage }
*
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Coverage getCoverage() {
return coverage;
}
/**
* Sets the value of the coverage property.
*
* @param value
* allowed object is
* {@link Coverage }
*
* @see #getCoverage()
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void setCoverage(Coverage value) {
this.coverage = value;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public boolean equals(Object object) {
if ((object == null)||(this.getClass()!= object.getClass())) {
return false;
}
if (this == object) {
return true;
}
if (!super.equals(object)) {
return false;
}
final DataResource that = ((DataResource) object);
{
List leftFacilities;
leftFacilities = (((this.facilities!= null)&&(!this.facilities.isEmpty()))?this.getFacilities():null);
List rightFacilities;
rightFacilities = (((that.facilities!= null)&&(!that.facilities.isEmpty()))?that.getFacilities():null);
if ((this.facilities!= null)&&(!this.facilities.isEmpty())) {
if ((that.facilities!= null)&&(!that.facilities.isEmpty())) {
if (!leftFacilities.equals(rightFacilities)) {
return false;
}
} else {
return false;
}
} else {
if ((that.facilities!= null)&&(!that.facilities.isEmpty())) {
return false;
}
}
}
{
List leftInstruments;
leftInstruments = (((this.instruments!= null)&&(!this.instruments.isEmpty()))?this.getInstruments():null);
List rightInstruments;
rightInstruments = (((that.instruments!= null)&&(!that.instruments.isEmpty()))?that.getInstruments():null);
if ((this.instruments!= null)&&(!this.instruments.isEmpty())) {
if ((that.instruments!= null)&&(!that.instruments.isEmpty())) {
if (!leftInstruments.equals(rightInstruments)) {
return false;
}
} else {
return false;
}
} else {
if ((that.instruments!= null)&&(!that.instruments.isEmpty())) {
return false;
}
}
}
{
Coverage leftCoverage;
leftCoverage = this.getCoverage();
Coverage rightCoverage;
rightCoverage = that.getCoverage();
if (this.coverage!= null) {
if (that.coverage!= null) {
if (!leftCoverage.equals(rightCoverage)) {
return false;
}
} else {
return false;
}
} else {
if (that.coverage!= null) {
return false;
}
}
}
return true;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public int hashCode() {
int currentHashCode = 1;
currentHashCode = ((currentHashCode* 31)+ super.hashCode());
{
currentHashCode = (currentHashCode* 31);
List theFacilities;
theFacilities = (((this.facilities!= null)&&(!this.facilities.isEmpty()))?this.getFacilities():null);
if ((this.facilities!= null)&&(!this.facilities.isEmpty())) {
currentHashCode += theFacilities.hashCode();
}
}
{
currentHashCode = (currentHashCode* 31);
List theInstruments;
theInstruments = (((this.instruments!= null)&&(!this.instruments.isEmpty()))?this.getInstruments():null);
if ((this.instruments!= null)&&(!this.instruments.isEmpty())) {
currentHashCode += theInstruments.hashCode();
}
}
{
currentHashCode = (currentHashCode* 31);
Coverage theCoverage;
theCoverage = this.getCoverage();
if (this.coverage!= null) {
currentHashCode += theCoverage.hashCode();
}
}
return currentHashCode;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public String toString() {
final ToStringStrategy strategy = JAXBToStringStrategy.getInstance();
final StringBuilder buffer = new StringBuilder();
append(null, buffer, strategy);
return buffer.toString();
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public StringBuilder append(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
strategy.appendStart(locator, this, buffer);
appendFields(locator, buffer, strategy);
strategy.appendEnd(locator, this, buffer);
return buffer;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public StringBuilder appendFields(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
super.appendFields(locator, buffer, strategy);
{
List theFacilities;
theFacilities = (((this.facilities!= null)&&(!this.facilities.isEmpty()))?this.getFacilities():null);
strategy.appendField(locator, this, "facilities", buffer, theFacilities, ((this.facilities!= null)&&(!this.facilities.isEmpty())));
}
{
List theInstruments;
theInstruments = (((this.instruments!= null)&&(!this.instruments.isEmpty()))?this.getInstruments():null);
strategy.appendField(locator, this, "instruments", buffer, theInstruments, ((this.instruments!= null)&&(!this.instruments.isEmpty())));
}
{
Coverage theCoverage;
theCoverage = this.getCoverage();
strategy.appendField(locator, this, "coverage", buffer, theCoverage, (this.coverage!= null));
}
return buffer;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void mergeFrom(Object left, Object right) {
final MergeStrategy strategy = JAXBMergeStrategy.getInstance();
mergeFrom(null, null, left, right, strategy);
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void mergeFrom(ObjectLocator leftLocator, ObjectLocator rightLocator, Object left, Object right, MergeStrategy strategy) {
super.mergeFrom(leftLocator, rightLocator, left, right, strategy);
if (right instanceof DataResource) {
final DataResource target = this;
final DataResource leftObject = ((DataResource) left);
final DataResource rightObject = ((DataResource) right);
{
Boolean facilitiesShouldBeMergedAndSet = strategy.shouldBeMergedAndSet(leftLocator, rightLocator, ((leftObject.facilities!= null)&&(!leftObject.facilities.isEmpty())), ((rightObject.facilities!= null)&&(!rightObject.facilities.isEmpty())));
if (facilitiesShouldBeMergedAndSet == Boolean.TRUE) {
List lhsFacilities;
lhsFacilities = (((leftObject.facilities!= null)&&(!leftObject.facilities.isEmpty()))?leftObject.getFacilities():null);
List rhsFacilities;
rhsFacilities = (((rightObject.facilities!= null)&&(!rightObject.facilities.isEmpty()))?rightObject.getFacilities():null);
List mergedFacilities = ((List ) strategy.merge(LocatorUtils.property(leftLocator, "facilities", lhsFacilities), LocatorUtils.property(rightLocator, "facilities", rhsFacilities), lhsFacilities, rhsFacilities, ((leftObject.facilities!= null)&&(!leftObject.facilities.isEmpty())), ((rightObject.facilities!= null)&&(!rightObject.facilities.isEmpty()))));
target.facilities = null;
if (mergedFacilities!= null) {
List uniqueFacilitiesl = target.getFacilities();
uniqueFacilitiesl.addAll(mergedFacilities);
}
} else {
if (facilitiesShouldBeMergedAndSet == Boolean.FALSE) {
target.facilities = null;
}
}
}
{
Boolean instrumentsShouldBeMergedAndSet = strategy.shouldBeMergedAndSet(leftLocator, rightLocator, ((leftObject.instruments!= null)&&(!leftObject.instruments.isEmpty())), ((rightObject.instruments!= null)&&(!rightObject.instruments.isEmpty())));
if (instrumentsShouldBeMergedAndSet == Boolean.TRUE) {
List lhsInstruments;
lhsInstruments = (((leftObject.instruments!= null)&&(!leftObject.instruments.isEmpty()))?leftObject.getInstruments():null);
List rhsInstruments;
rhsInstruments = (((rightObject.instruments!= null)&&(!rightObject.instruments.isEmpty()))?rightObject.getInstruments():null);
List mergedInstruments = ((List ) strategy.merge(LocatorUtils.property(leftLocator, "instruments", lhsInstruments), LocatorUtils.property(rightLocator, "instruments", rhsInstruments), lhsInstruments, rhsInstruments, ((leftObject.instruments!= null)&&(!leftObject.instruments.isEmpty())), ((rightObject.instruments!= null)&&(!rightObject.instruments.isEmpty()))));
target.instruments = null;
if (mergedInstruments!= null) {
List uniqueInstrumentsl = target.getInstruments();
uniqueInstrumentsl.addAll(mergedInstruments);
}
} else {
if (instrumentsShouldBeMergedAndSet == Boolean.FALSE) {
target.instruments = null;
}
}
}
{
Boolean coverageShouldBeMergedAndSet = strategy.shouldBeMergedAndSet(leftLocator, rightLocator, (leftObject.coverage!= null), (rightObject.coverage!= null));
if (coverageShouldBeMergedAndSet == Boolean.TRUE) {
Coverage lhsCoverage;
lhsCoverage = leftObject.getCoverage();
Coverage rhsCoverage;
rhsCoverage = rightObject.getCoverage();
Coverage mergedCoverage = ((Coverage) strategy.merge(LocatorUtils.property(leftLocator, "coverage", lhsCoverage), LocatorUtils.property(rightLocator, "coverage", rhsCoverage), lhsCoverage, rhsCoverage, (leftObject.coverage!= null), (rightObject.coverage!= null)));
target.setCoverage(mergedCoverage);
} else {
if (coverageShouldBeMergedAndSet == Boolean.FALSE) {
target.coverage = null;
}
}
}
}
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Object createNewInstance() {
return new DataResource();
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public DataResource clone() {
final DataResource _newObject;
_newObject = ((DataResource) super.clone());
if (this.facilities == null) {
_newObject.facilities = null;
} else {
_newObject.facilities = new ArrayList<>();
for (ResourceName _item: this.facilities) {
_newObject.facilities.add(((_item == null)?null:_item.clone()));
}
}
if (this.instruments == null) {
_newObject.instruments = null;
} else {
_newObject.instruments = new ArrayList<>();
for (ResourceName _item: this.instruments) {
_newObject.instruments.add(((_item == null)?null:_item.clone()));
}
}
_newObject.coverage = ((this.coverage == null)?null:this.coverage.clone());
return _newObject;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public DataResource createCopy() {
final DataResource _newObject = ((DataResource) super.createCopy());
if (this.facilities == null) {
_newObject.facilities = null;
} else {
_newObject.facilities = new ArrayList<>();
for (ResourceName _item: this.facilities) {
_newObject.facilities.add(((_item == null)?null:_item.createCopy()));
}
}
if (this.instruments == null) {
_newObject.instruments = null;
} else {
_newObject.instruments = new ArrayList<>();
for (ResourceName _item: this.instruments) {
_newObject.instruments.add(((_item == null)?null:_item.createCopy()));
}
}
_newObject.coverage = ((this.coverage == null)?null:this.coverage.createCopy());
return _newObject;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public DataResource createCopy(final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
final DataResource _newObject = ((DataResource) super.createCopy(_propertyTree, _propertyTreeUse));
final PropertyTree facilitiesPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("facilities"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(facilitiesPropertyTree!= null):((facilitiesPropertyTree == null)||(!facilitiesPropertyTree.isLeaf())))) {
if (this.facilities == null) {
_newObject.facilities = null;
} else {
_newObject.facilities = new ArrayList<>();
for (ResourceName _item: this.facilities) {
_newObject.facilities.add(((_item == null)?null:_item.createCopy(facilitiesPropertyTree, _propertyTreeUse)));
}
}
}
final PropertyTree instrumentsPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("instruments"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(instrumentsPropertyTree!= null):((instrumentsPropertyTree == null)||(!instrumentsPropertyTree.isLeaf())))) {
if (this.instruments == null) {
_newObject.instruments = null;
} else {
_newObject.instruments = new ArrayList<>();
for (ResourceName _item: this.instruments) {
_newObject.instruments.add(((_item == null)?null:_item.createCopy(instrumentsPropertyTree, _propertyTreeUse)));
}
}
}
final PropertyTree coveragePropertyTree = ((_propertyTree == null)?null:_propertyTree.get("coverage"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(coveragePropertyTree!= null):((coveragePropertyTree == null)||(!coveragePropertyTree.isLeaf())))) {
_newObject.coverage = ((this.coverage == null)?null:this.coverage.createCopy(coveragePropertyTree, _propertyTreeUse));
}
return _newObject;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public DataResource copyExcept(final PropertyTree _propertyTree) {
return createCopy(_propertyTree, PropertyTreeUse.EXCLUDE);
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public DataResource copyOnly(final PropertyTree _propertyTree) {
return createCopy(_propertyTree, PropertyTreeUse.INCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public DataResource.Modifier modifier() {
if (null == this.__cachedModifier__) {
this.__cachedModifier__ = new DataResource.Modifier();
}
return ((DataResource.Modifier) this.__cachedModifier__);
}
/**
* Copies all state of this object to a builder. This method is used by the copyOf
* method and should not be called directly by client code.
*
* @param _other
* A builder instance to which the state of this object will be copied.
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public<_B >void copyTo(final DataResource.Builder<_B> _other) {
super.copyTo(_other);
if (this.facilities == null) {
_other.facilities = null;
} else {
_other.facilities = new ArrayList<>();
for (ResourceName _item: this.facilities) {
_other.facilities.add(((_item == null)?null:_item.newCopyBuilder(_other)));
}
}
if (this.instruments == null) {
_other.instruments = null;
} else {
_other.instruments = new ArrayList<>();
for (ResourceName _item: this.instruments) {
_other.instruments.add(((_item == null)?null:_item.newCopyBuilder(_other)));
}
}
_other.coverage = ((this.coverage == null)?null:this.coverage.newCopyBuilder(_other));
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public<_B >DataResource.Builder<_B> newCopyBuilder(final _B _parentBuilder) {
return new DataResource.Builder<_B>(_parentBuilder, this, true);
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public DataResource.Builder newCopyBuilder() {
return newCopyBuilder(null);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static DataResource.Builder builder() {
return new DataResource.Builder<>(null, null, false);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static<_B >DataResource.Builder<_B> copyOf(final Resource _other) {
final DataResource.Builder<_B> _newBuilder = new DataResource.Builder<>(null, null, false);
_other.copyTo(_newBuilder);
return _newBuilder;
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static<_B >DataResource.Builder<_B> copyOf(final Service _other) {
final DataResource.Builder<_B> _newBuilder = new DataResource.Builder<>(null, null, false);
_other.copyTo(_newBuilder);
return _newBuilder;
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static<_B >DataResource.Builder<_B> copyOf(final DataResource _other) {
final DataResource.Builder<_B> _newBuilder = new DataResource.Builder<>(null, null, false);
_other.copyTo(_newBuilder);
return _newBuilder;
}
/**
* Copies all state of this object to a builder. This method is used by the copyOf
* method and should not be called directly by client code.
*
* @param _other
* A builder instance to which the state of this object will be copied.
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public<_B >void copyTo(final DataResource.Builder<_B> _other, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
super.copyTo(_other, _propertyTree, _propertyTreeUse);
final PropertyTree facilitiesPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("facilities"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(facilitiesPropertyTree!= null):((facilitiesPropertyTree == null)||(!facilitiesPropertyTree.isLeaf())))) {
if (this.facilities == null) {
_other.facilities = null;
} else {
_other.facilities = new ArrayList<>();
for (ResourceName _item: this.facilities) {
_other.facilities.add(((_item == null)?null:_item.newCopyBuilder(_other, facilitiesPropertyTree, _propertyTreeUse)));
}
}
}
final PropertyTree instrumentsPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("instruments"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(instrumentsPropertyTree!= null):((instrumentsPropertyTree == null)||(!instrumentsPropertyTree.isLeaf())))) {
if (this.instruments == null) {
_other.instruments = null;
} else {
_other.instruments = new ArrayList<>();
for (ResourceName _item: this.instruments) {
_other.instruments.add(((_item == null)?null:_item.newCopyBuilder(_other, instrumentsPropertyTree, _propertyTreeUse)));
}
}
}
final PropertyTree coveragePropertyTree = ((_propertyTree == null)?null:_propertyTree.get("coverage"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(coveragePropertyTree!= null):((coveragePropertyTree == null)||(!coveragePropertyTree.isLeaf())))) {
_other.coverage = ((this.coverage == null)?null:this.coverage.newCopyBuilder(_other, coveragePropertyTree, _propertyTreeUse));
}
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public<_B >DataResource.Builder<_B> newCopyBuilder(final _B _parentBuilder, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
return new DataResource.Builder<_B>(_parentBuilder, this, true, _propertyTree, _propertyTreeUse);
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public DataResource.Builder newCopyBuilder(final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
return newCopyBuilder(null, _propertyTree, _propertyTreeUse);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static<_B >DataResource.Builder<_B> copyOf(final Resource _other, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
final DataResource.Builder<_B> _newBuilder = new DataResource.Builder<>(null, null, false);
_other.copyTo(_newBuilder, _propertyTree, _propertyTreeUse);
return _newBuilder;
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static<_B >DataResource.Builder<_B> copyOf(final Service _other, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
final DataResource.Builder<_B> _newBuilder = new DataResource.Builder<>(null, null, false);
_other.copyTo(_newBuilder, _propertyTree, _propertyTreeUse);
return _newBuilder;
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static<_B >DataResource.Builder<_B> copyOf(final DataResource _other, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
final DataResource.Builder<_B> _newBuilder = new DataResource.Builder<>(null, null, false);
_other.copyTo(_newBuilder, _propertyTree, _propertyTreeUse);
return _newBuilder;
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static DataResource.Builder copyExcept(final Resource _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.EXCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static DataResource.Builder copyExcept(final Service _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.EXCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static DataResource.Builder copyExcept(final DataResource _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.EXCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static DataResource.Builder copyOnly(final Resource _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.INCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static DataResource.Builder copyOnly(final Service _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.INCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static DataResource.Builder copyOnly(final DataResource _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.INCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public DataResource visit(final PropertyVisitor _visitor_) {
super.visit(_visitor_);
if (_visitor_.visit(new CollectionProperty<>(DataResource.PropInfo.FACILITIES, this))&&(this.facilities!= null)) {
for (ResourceName _item_: this.facilities) {
if (_item_!= null) {
_item_.visit(_visitor_);
}
}
}
if (_visitor_.visit(new CollectionProperty<>(DataResource.PropInfo.INSTRUMENTS, this))&&(this.instruments!= null)) {
for (ResourceName _item_: this.instruments) {
if (_item_!= null) {
_item_.visit(_visitor_);
}
}
}
if (_visitor_.visit(new SingleProperty<>(DataResource.PropInfo.COVERAGE, this))&&(this.coverage!= null)) {
this.coverage.visit(_visitor_);
}
return this;
}
public static class Builder<_B >
extends Service.Builder<_B>
implements Buildable
{
private List>> facilities;
private List>> instruments;
private Coverage.Builder> coverage;
public Builder(final _B _parentBuilder, final DataResource _other, final boolean _copy) {
super(_parentBuilder, _other, _copy);
if (_other!= null) {
if (_other.facilities == null) {
this.facilities = null;
} else {
this.facilities = new ArrayList<>();
for (ResourceName _item: _other.facilities) {
this.facilities.add(((_item == null)?null:_item.newCopyBuilder(this)));
}
}
if (_other.instruments == null) {
this.instruments = null;
} else {
this.instruments = new ArrayList<>();
for (ResourceName _item: _other.instruments) {
this.instruments.add(((_item == null)?null:_item.newCopyBuilder(this)));
}
}
this.coverage = ((_other.coverage == null)?null:_other.coverage.newCopyBuilder(this));
}
}
public Builder(final _B _parentBuilder, final DataResource _other, final boolean _copy, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
super(_parentBuilder, _other, _copy, _propertyTree, _propertyTreeUse);
if (_other!= null) {
final PropertyTree facilitiesPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("facilities"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(facilitiesPropertyTree!= null):((facilitiesPropertyTree == null)||(!facilitiesPropertyTree.isLeaf())))) {
if (_other.facilities == null) {
this.facilities = null;
} else {
this.facilities = new ArrayList<>();
for (ResourceName _item: _other.facilities) {
this.facilities.add(((_item == null)?null:_item.newCopyBuilder(this, facilitiesPropertyTree, _propertyTreeUse)));
}
}
}
final PropertyTree instrumentsPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("instruments"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(instrumentsPropertyTree!= null):((instrumentsPropertyTree == null)||(!instrumentsPropertyTree.isLeaf())))) {
if (_other.instruments == null) {
this.instruments = null;
} else {
this.instruments = new ArrayList<>();
for (ResourceName _item: _other.instruments) {
this.instruments.add(((_item == null)?null:_item.newCopyBuilder(this, instrumentsPropertyTree, _propertyTreeUse)));
}
}
}
final PropertyTree coveragePropertyTree = ((_propertyTree == null)?null:_propertyTree.get("coverage"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(coveragePropertyTree!= null):((coveragePropertyTree == null)||(!coveragePropertyTree.isLeaf())))) {
this.coverage = ((_other.coverage == null)?null:_other.coverage.newCopyBuilder(this, coveragePropertyTree, _propertyTreeUse));
}
}
}
protected<_P extends DataResource >_P init(final _P _product) {
if (this.facilities!= null) {
final List facilities = new ArrayList<>(this.facilities.size());
for (ResourceName.Builder> _item: this.facilities) {
facilities.add(_item.build());
}
_product.facilities = facilities;
}
if (this.instruments!= null) {
final List instruments = new ArrayList<>(this.instruments.size());
for (ResourceName.Builder> _item: this.instruments) {
instruments.add(_item.build());
}
_product.instruments = instruments;
}
_product.coverage = ((this.coverage == null)?null:this.coverage.build());
return super.init(_product);
}
/**
* Adds the given items to the value of "facilities"
*
* @param facilities
* Items to add to the value of the "facilities" property
*/
public DataResource.Builder<_B> addFacilities(final Iterable extends ResourceName> facilities) {
if (facilities!= null) {
if (this.facilities == null) {
this.facilities = new ArrayList<>();
}
for (ResourceName _item: facilities) {
this.facilities.add(new ResourceName.Builder<>(this, _item, false));
}
}
return this;
}
/**
* Sets the new value of "facilities" (any previous value will be replaced)
*
* @param facilities
* New value of the "facilities" property.
*/
public DataResource.Builder<_B> withFacilities(final Iterable extends ResourceName> facilities) {
if (this.facilities!= null) {
this.facilities.clear();
}
return addFacilities(facilities);
}
/**
* Adds the given items to the value of "facilities"
*
* @param facilities
* Items to add to the value of the "facilities" property
*/
public DataResource.Builder<_B> addFacilities(ResourceName... facilities) {
addFacilities(Arrays.asList(facilities));
return this;
}
/**
* Sets the new value of "facilities" (any previous value will be replaced)
*
* @param facilities
* New value of the "facilities" property.
*/
public DataResource.Builder<_B> withFacilities(ResourceName... facilities) {
withFacilities(Arrays.asList(facilities));
return this;
}
/**
* Returns a new builder to build an additional value of the "Facilities" property.
* Use {@link org.javastro.ivoa.entities.resource.ResourceName.Builder#end()} to
* return to the current builder.
*
* @return
* a new builder to build an additional value of the "Facilities" property.
* Use {@link org.javastro.ivoa.entities.resource.ResourceName.Builder#end()} to
* return to the current builder.
*/
public ResourceName.Builder extends DataResource.Builder<_B>> addFacilities() {
if (this.facilities == null) {
this.facilities = new ArrayList<>();
}
final ResourceName.Builder> facilities_Builder = new ResourceName.Builder<>(this, null, false);
this.facilities.add(facilities_Builder);
return facilities_Builder;
}
/**
* Adds the given items to the value of "instruments"
*
* @param instruments
* Items to add to the value of the "instruments" property
*/
public DataResource.Builder<_B> addInstruments(final Iterable extends ResourceName> instruments) {
if (instruments!= null) {
if (this.instruments == null) {
this.instruments = new ArrayList<>();
}
for (ResourceName _item: instruments) {
this.instruments.add(new ResourceName.Builder<>(this, _item, false));
}
}
return this;
}
/**
* Sets the new value of "instruments" (any previous value will be replaced)
*
* @param instruments
* New value of the "instruments" property.
*/
public DataResource.Builder<_B> withInstruments(final Iterable extends ResourceName> instruments) {
if (this.instruments!= null) {
this.instruments.clear();
}
return addInstruments(instruments);
}
/**
* Adds the given items to the value of "instruments"
*
* @param instruments
* Items to add to the value of the "instruments" property
*/
public DataResource.Builder<_B> addInstruments(ResourceName... instruments) {
addInstruments(Arrays.asList(instruments));
return this;
}
/**
* Sets the new value of "instruments" (any previous value will be replaced)
*
* @param instruments
* New value of the "instruments" property.
*/
public DataResource.Builder<_B> withInstruments(ResourceName... instruments) {
withInstruments(Arrays.asList(instruments));
return this;
}
/**
* Returns a new builder to build an additional value of the "Instruments"
* property.
* Use {@link org.javastro.ivoa.entities.resource.ResourceName.Builder#end()} to
* return to the current builder.
*
* @return
* a new builder to build an additional value of the "Instruments" property.
* Use {@link org.javastro.ivoa.entities.resource.ResourceName.Builder#end()} to
* return to the current builder.
*/
public ResourceName.Builder extends DataResource.Builder<_B>> addInstruments() {
if (this.instruments == null) {
this.instruments = new ArrayList<>();
}
final ResourceName.Builder> instruments_Builder = new ResourceName.Builder<>(this, null, false);
this.instruments.add(instruments_Builder);
return instruments_Builder;
}
/**
* Sets the new value of "coverage" (any previous value will be replaced)
*
* @param coverage
* New value of the "coverage" property.
*/
public DataResource.Builder<_B> withCoverage(final Coverage coverage) {
this.coverage = ((coverage == null)?null:new Coverage.Builder<>(this, coverage, false));
return this;
}
/**
* Returns the existing builder or a new builder to build the value of the
* "coverage" property.
* Use {@link
* org.javastro.ivoa.entities.resource.dataservice.Coverage.Builder#end()} to
* return to the current builder.
*
* @return
* A new builder to build the value of the "coverage" property.
* Use {@link
* org.javastro.ivoa.entities.resource.dataservice.Coverage.Builder#end()} to
* return to the current builder.
*/
public Coverage.Builder extends DataResource.Builder<_B>> withCoverage() {
if (this.coverage!= null) {
return this.coverage;
}
return this.coverage = new Coverage.Builder<>(this, null, false);
}
/**
* Adds the given items to the value of "rights"
*
* @param rights
* Items to add to the value of the "rights" property
*/
@Override
public DataResource.Builder<_B> addRights(final Iterable extends Rights> rights) {
super.addRights(rights);
return this;
}
/**
* Adds the given items to the value of "rights"
*
* @param rights
* Items to add to the value of the "rights" property
*/
@Override
public DataResource.Builder<_B> addRights(Rights... rights) {
super.addRights(rights);
return this;
}
/**
* Sets the new value of "rights" (any previous value will be replaced)
*
* @param rights
* New value of the "rights" property.
*/
@Override
public DataResource.Builder<_B> withRights(final Iterable extends Rights> rights) {
super.withRights(rights);
return this;
}
/**
* Sets the new value of "rights" (any previous value will be replaced)
*
* @param rights
* New value of the "rights" property.
*/
@Override
public DataResource.Builder<_B> withRights(Rights... rights) {
super.withRights(rights);
return this;
}
/**
* Adds the given items to the value of "capabilities"
*
* @param capabilities
* Items to add to the value of the "capabilities" property
*/
@Override
public DataResource.Builder<_B> addCapabilities(final Iterable extends Capability> capabilities) {
super.addCapabilities(capabilities);
return this;
}
/**
* Adds the given items to the value of "capabilities"
*
* @param capabilities
* Items to add to the value of the "capabilities" property
*/
@Override
public DataResource.Builder<_B> addCapabilities(Capability... capabilities) {
super.addCapabilities(capabilities);
return this;
}
/**
* Sets the new value of "capabilities" (any previous value will be replaced)
*
* @param capabilities
* New value of the "capabilities" property.
*/
@Override
public DataResource.Builder<_B> withCapabilities(final Iterable extends Capability> capabilities) {
super.withCapabilities(capabilities);
return this;
}
/**
* Sets the new value of "capabilities" (any previous value will be replaced)
*
* @param capabilities
* New value of the "capabilities" property.
*/
@Override
public DataResource.Builder<_B> withCapabilities(Capability... capabilities) {
super.withCapabilities(capabilities);
return this;
}
/**
* Adds the given items to the value of "validationLevels"
*
* @param validationLevels
* Items to add to the value of the "validationLevels" property
*/
@Override
public DataResource.Builder<_B> addValidationLevels(final Iterable extends Validation> validationLevels) {
super.addValidationLevels(validationLevels);
return this;
}
/**
* Adds the given items to the value of "validationLevels"
*
* @param validationLevels
* Items to add to the value of the "validationLevels" property
*/
@Override
public DataResource.Builder<_B> addValidationLevels(Validation... validationLevels) {
super.addValidationLevels(validationLevels);
return this;
}
/**
* Sets the new value of "validationLevels" (any previous value will be replaced)
*
* @param validationLevels
* New value of the "validationLevels" property.
*/
@Override
public DataResource.Builder<_B> withValidationLevels(final Iterable extends Validation> validationLevels) {
super.withValidationLevels(validationLevels);
return this;
}
/**
* Sets the new value of "validationLevels" (any previous value will be replaced)
*
* @param validationLevels
* New value of the "validationLevels" property.
*/
@Override
public DataResource.Builder<_B> withValidationLevels(Validation... validationLevels) {
super.withValidationLevels(validationLevels);
return this;
}
/**
* Sets the new value of "title" (any previous value will be replaced)
*
* @param title
* New value of the "title" property.
*/
@Override
public DataResource.Builder<_B> withTitle(final String title) {
super.withTitle(title);
return this;
}
/**
* Sets the new value of "shortName" (any previous value will be replaced)
*
* @param shortName
* New value of the "shortName" property.
*/
@Override
public DataResource.Builder<_B> withShortName(final String shortName) {
super.withShortName(shortName);
return this;
}
/**
* Sets the new value of "identifier" (any previous value will be replaced)
*
* @param identifier
* New value of the "identifier" property.
*/
@Override
public DataResource.Builder<_B> withIdentifier(final String identifier) {
super.withIdentifier(identifier);
return this;
}
/**
* Adds the given items to the value of "altIdentifiers"
*
* @param altIdentifiers
* Items to add to the value of the "altIdentifiers" property
*/
@Override
public DataResource.Builder<_B> addAltIdentifiers(final Iterable extends String> altIdentifiers) {
super.addAltIdentifiers(altIdentifiers);
return this;
}
/**
* Adds the given items to the value of "altIdentifiers"
*
* @param altIdentifiers
* Items to add to the value of the "altIdentifiers" property
*/
@Override
public DataResource.Builder<_B> addAltIdentifiers(String... altIdentifiers) {
super.addAltIdentifiers(altIdentifiers);
return this;
}
/**
* Sets the new value of "altIdentifiers" (any previous value will be replaced)
*
* @param altIdentifiers
* New value of the "altIdentifiers" property.
*/
@Override
public DataResource.Builder<_B> withAltIdentifiers(final Iterable extends String> altIdentifiers) {
super.withAltIdentifiers(altIdentifiers);
return this;
}
/**
* Sets the new value of "altIdentifiers" (any previous value will be replaced)
*
* @param altIdentifiers
* New value of the "altIdentifiers" property.
*/
@Override
public DataResource.Builder<_B> withAltIdentifiers(String... altIdentifiers) {
super.withAltIdentifiers(altIdentifiers);
return this;
}
/**
* Sets the new value of "curation" (any previous value will be replaced)
*
* @param curation
* New value of the "curation" property.
*/
@Override
public DataResource.Builder<_B> withCuration(final Curation curation) {
super.withCuration(curation);
return this;
}
/**
* Returns the existing builder or a new builder to build the value of the
* "curation" property.
* Use {@link org.javastro.ivoa.entities.resource.Curation.Builder#end()} to return
* to the current builder.
*
* @return
* A new builder to build the value of the "curation" property.
* Use {@link org.javastro.ivoa.entities.resource.Curation.Builder#end()} to return
* to the current builder.
*/
public Curation.Builder extends DataResource.Builder<_B>> withCuration() {
return ((Curation.Builder extends DataResource.Builder<_B>> ) super.withCuration());
}
/**
* Sets the new value of "content" (any previous value will be replaced)
*
* @param content
* New value of the "content" property.
*/
@Override
public DataResource.Builder<_B> withContent(final Content content) {
super.withContent(content);
return this;
}
/**
* Returns the existing builder or a new builder to build the value of the
* "content" property.
* Use {@link org.javastro.ivoa.entities.resource.Content.Builder#end()} to return
* to the current builder.
*
* @return
* A new builder to build the value of the "content" property.
* Use {@link org.javastro.ivoa.entities.resource.Content.Builder#end()} to return
* to the current builder.
*/
public Content.Builder extends DataResource.Builder<_B>> withContent() {
return ((Content.Builder extends DataResource.Builder<_B>> ) super.withContent());
}
/**
* Sets the new value of "created" (any previous value will be replaced)
*
* @param created
* New value of the "created" property.
*/
@Override
public DataResource.Builder<_B> withCreated(final LocalDateTime created) {
super.withCreated(created);
return this;
}
/**
* Sets the new value of "updated" (any previous value will be replaced)
*
* @param updated
* New value of the "updated" property.
*/
@Override
public DataResource.Builder<_B> withUpdated(final LocalDateTime updated) {
super.withUpdated(updated);
return this;
}
/**
* Sets the new value of "status" (any previous value will be replaced)
*
* @param status
* New value of the "status" property.
*/
@Override
public DataResource.Builder<_B> withStatus(final String status) {
super.withStatus(status);
return this;
}
/**
* Sets the new value of "version" (any previous value will be replaced)
*
* @param version
* New value of the "version" property.
*/
@Override
public DataResource.Builder<_B> withVersion(final String version) {
super.withVersion(version);
return this;
}
@Override
public DataResource build() {
if (_storedValue == null) {
return this.init(new DataResource());
} else {
return ((DataResource) _storedValue);
}
}
public DataResource.Builder<_B> copyOf(final DataResource _other) {
_other.copyTo(this);
return this;
}
public DataResource.Builder<_B> copyOf(final DataResource.Builder _other) {
return copyOf(_other.build());
}
}
public class Modifier
extends Service.Modifier
{
public List getFacilities() {
if (DataResource.this.facilities == null) {
DataResource.this.facilities = new ArrayList<>();
}
return DataResource.this.facilities;
}
public List getInstruments() {
if (DataResource.this.instruments == null) {
DataResource.this.instruments = new ArrayList<>();
}
return DataResource.this.instruments;
}
public void setCoverage(final Coverage coverage) {
DataResource.this.setCoverage(coverage);
}
}
public static class PropInfo {
public static final transient CollectionPropertyInfo FACILITIES = new CollectionPropertyInfo("facilities", DataResource.class, ResourceName.class, true, null, new QName("", "facility"), new QName("http://www.ivoa.net/xml/VOResource/v1.0", "ResourceName"), false) {
@Override
public List get(final DataResource _instance_) {
return ((_instance_ == null)?null:_instance_.facilities);
}
@Override
public void set(final DataResource _instance_, final List _value_) {
if (_instance_!= null) {
_instance_.facilities = _value_;
}
}
}
;
public static final transient CollectionPropertyInfo INSTRUMENTS = new CollectionPropertyInfo("instruments", DataResource.class, ResourceName.class, true, null, new QName("", "instrument"), new QName("http://www.ivoa.net/xml/VOResource/v1.0", "ResourceName"), false) {
@Override
public List get(final DataResource _instance_) {
return ((_instance_ == null)?null:_instance_.instruments);
}
@Override
public void set(final DataResource _instance_, final List _value_) {
if (_instance_!= null) {
_instance_.instruments = _value_;
}
}
}
;
public static final transient SinglePropertyInfo COVERAGE = new SinglePropertyInfo("coverage", DataResource.class, Coverage.class, false, null, new QName("", "coverage"), new QName("http://www.ivoa.net/xml/VODataService/v1.1", "Coverage"), false) {
@Override
public Coverage get(final DataResource _instance_) {
return ((_instance_ == null)?null:_instance_.coverage);
}
@Override
public void set(final DataResource _instance_, final Coverage _value_) {
if (_instance_!= null) {
_instance_.coverage = _value_;
}
}
}
;
}
public static class Select
extends DataResource.Selector
{
Select() {
super(null, null, null);
}
public static DataResource.Select _root() {
return new DataResource.Select();
}
}
public static class Selector , TParent >
extends Service.Selector
{
private ResourceName.Selector> facilities = null;
private ResourceName.Selector> instruments = null;
private Coverage.Selector> coverage = null;
public Selector(final TRoot root, final TParent parent, final String propertyName) {
super(root, parent, propertyName);
}
@Override
public Map buildChildren() {
final Map products = new HashMap<>();
products.putAll(super.buildChildren());
if (this.facilities!= null) {
products.put("facilities", this.facilities.init());
}
if (this.instruments!= null) {
products.put("instruments", this.instruments.init());
}
if (this.coverage!= null) {
products.put("coverage", this.coverage.init());
}
return products;
}
public ResourceName.Selector> facilities() {
return ((this.facilities == null)?this.facilities = new ResourceName.Selector<>(this._root, this, "facilities"):this.facilities);
}
public ResourceName.Selector> instruments() {
return ((this.instruments == null)?this.instruments = new ResourceName.Selector<>(this._root, this, "instruments"):this.instruments);
}
public Coverage.Selector> coverage() {
return ((this.coverage == null)?this.coverage = new Coverage.Selector<>(this._root, this, "coverage"):this.coverage);
}
}
}