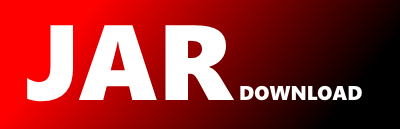
org.javastro.ivoa.entities.resource.dataservice.DataService Maven / Gradle / Ivy
package org.javastro.ivoa.entities.resource.dataservice;
import java.time.LocalDateTime;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import com.kscs.util.jaxb.Buildable;
import com.kscs.util.jaxb.Copyable;
import com.kscs.util.jaxb.PartialCopyable;
import com.kscs.util.jaxb.PropertyTree;
import com.kscs.util.jaxb.PropertyTreeUse;
import com.kscs.util.jaxb.PropertyVisitor;
import jakarta.annotation.Generated;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlType;
import org.javastro.ivoa.entities.resource.Capability;
import org.javastro.ivoa.entities.resource.Content;
import org.javastro.ivoa.entities.resource.Curation;
import org.javastro.ivoa.entities.resource.Resource;
import org.javastro.ivoa.entities.resource.ResourceName;
import org.javastro.ivoa.entities.resource.Rights;
import org.javastro.ivoa.entities.resource.Service;
import org.javastro.ivoa.entities.resource.Validation;
import org.jvnet.jaxb.lang.JAXBMergeStrategy;
import org.jvnet.jaxb.lang.JAXBToStringStrategy;
import org.jvnet.jaxb.lang.MergeFrom;
import org.jvnet.jaxb.lang.MergeStrategy;
import org.jvnet.jaxb.lang.ToString;
import org.jvnet.jaxb.lang.ToStringStrategy;
import org.jvnet.jaxb.locator.ObjectLocator;
/**
* This resource type should only be used if the service has no
* common underlying tabular schema (e.g., a storage service) or
* if it is not explicitly accessible (e.g., an ftp server with
* images). Use CatalogService otherwise.
*
* Java class for DataService complex type
.
*
* The following schema fragment specifies the expected content contained within this class.
*
* {@code
*
*
*
*
*
*
* }
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "DataService")
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public class DataService
extends DataResource
implements Cloneable, Copyable, PartialCopyable, MergeFrom, ToString
{
/**
* Default no-arg constructor
*
*/
public DataService() {
super();
}
/**
* Fully-initialising value constructor
*
*/
public DataService(final List validationLevels, final String title, final String shortName, final String identifier, final List altIdentifiers, final Curation curation, final Content content, final LocalDateTime created, final LocalDateTime updated, final String status, final String version, final List rights, final List capabilities, final List facilities, final List instruments, final Coverage coverage) {
super(validationLevels, title, shortName, identifier, altIdentifiers, curation, content, created, updated, status, version, rights, capabilities, facilities, instruments, coverage);
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public boolean equals(Object object) {
if ((object == null)||(this.getClass()!= object.getClass())) {
return false;
}
if (this == object) {
return true;
}
if (!super.equals(object)) {
return false;
}
return true;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public int hashCode() {
int currentHashCode = 1;
currentHashCode = ((currentHashCode* 31)+ super.hashCode());
return currentHashCode;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public String toString() {
final ToStringStrategy strategy = JAXBToStringStrategy.getInstance();
final StringBuilder buffer = new StringBuilder();
append(null, buffer, strategy);
return buffer.toString();
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public StringBuilder append(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
strategy.appendStart(locator, this, buffer);
appendFields(locator, buffer, strategy);
strategy.appendEnd(locator, this, buffer);
return buffer;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public StringBuilder appendFields(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
super.appendFields(locator, buffer, strategy);
return buffer;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void mergeFrom(Object left, Object right) {
final MergeStrategy strategy = JAXBMergeStrategy.getInstance();
mergeFrom(null, null, left, right, strategy);
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void mergeFrom(ObjectLocator leftLocator, ObjectLocator rightLocator, Object left, Object right, MergeStrategy strategy) {
super.mergeFrom(leftLocator, rightLocator, left, right, strategy);
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Object createNewInstance() {
return new DataService();
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public DataService clone() {
final DataService _newObject;
_newObject = ((DataService) super.clone());
return _newObject;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public DataService createCopy() {
final DataService _newObject = ((DataService) super.createCopy());
return _newObject;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public DataService createCopy(final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
final DataService _newObject = ((DataService) super.createCopy(_propertyTree, _propertyTreeUse));
return _newObject;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public DataService copyExcept(final PropertyTree _propertyTree) {
return createCopy(_propertyTree, PropertyTreeUse.EXCLUDE);
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public DataService copyOnly(final PropertyTree _propertyTree) {
return createCopy(_propertyTree, PropertyTreeUse.INCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public DataService.Modifier modifier() {
if (null == this.__cachedModifier__) {
this.__cachedModifier__ = new DataService.Modifier();
}
return ((DataService.Modifier) this.__cachedModifier__);
}
/**
* Copies all state of this object to a builder. This method is used by the copyOf
* method and should not be called directly by client code.
*
* @param _other
* A builder instance to which the state of this object will be copied.
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public<_B >void copyTo(final DataService.Builder<_B> _other) {
super.copyTo(_other);
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public<_B >DataService.Builder<_B> newCopyBuilder(final _B _parentBuilder) {
return new DataService.Builder<_B>(_parentBuilder, this, true);
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public DataService.Builder newCopyBuilder() {
return newCopyBuilder(null);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static DataService.Builder builder() {
return new DataService.Builder<>(null, null, false);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static<_B >DataService.Builder<_B> copyOf(final Resource _other) {
final DataService.Builder<_B> _newBuilder = new DataService.Builder<>(null, null, false);
_other.copyTo(_newBuilder);
return _newBuilder;
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static<_B >DataService.Builder<_B> copyOf(final Service _other) {
final DataService.Builder<_B> _newBuilder = new DataService.Builder<>(null, null, false);
_other.copyTo(_newBuilder);
return _newBuilder;
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static<_B >DataService.Builder<_B> copyOf(final DataResource _other) {
final DataService.Builder<_B> _newBuilder = new DataService.Builder<>(null, null, false);
_other.copyTo(_newBuilder);
return _newBuilder;
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static<_B >DataService.Builder<_B> copyOf(final DataService _other) {
final DataService.Builder<_B> _newBuilder = new DataService.Builder<>(null, null, false);
_other.copyTo(_newBuilder);
return _newBuilder;
}
/**
* Copies all state of this object to a builder. This method is used by the copyOf
* method and should not be called directly by client code.
*
* @param _other
* A builder instance to which the state of this object will be copied.
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public<_B >void copyTo(final DataService.Builder<_B> _other, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
super.copyTo(_other, _propertyTree, _propertyTreeUse);
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public<_B >DataService.Builder<_B> newCopyBuilder(final _B _parentBuilder, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
return new DataService.Builder<_B>(_parentBuilder, this, true, _propertyTree, _propertyTreeUse);
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public DataService.Builder newCopyBuilder(final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
return newCopyBuilder(null, _propertyTree, _propertyTreeUse);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static<_B >DataService.Builder<_B> copyOf(final Resource _other, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
final DataService.Builder<_B> _newBuilder = new DataService.Builder<>(null, null, false);
_other.copyTo(_newBuilder, _propertyTree, _propertyTreeUse);
return _newBuilder;
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static<_B >DataService.Builder<_B> copyOf(final Service _other, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
final DataService.Builder<_B> _newBuilder = new DataService.Builder<>(null, null, false);
_other.copyTo(_newBuilder, _propertyTree, _propertyTreeUse);
return _newBuilder;
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static<_B >DataService.Builder<_B> copyOf(final DataResource _other, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
final DataService.Builder<_B> _newBuilder = new DataService.Builder<>(null, null, false);
_other.copyTo(_newBuilder, _propertyTree, _propertyTreeUse);
return _newBuilder;
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static<_B >DataService.Builder<_B> copyOf(final DataService _other, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
final DataService.Builder<_B> _newBuilder = new DataService.Builder<>(null, null, false);
_other.copyTo(_newBuilder, _propertyTree, _propertyTreeUse);
return _newBuilder;
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static DataService.Builder copyExcept(final Resource _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.EXCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static DataService.Builder copyExcept(final Service _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.EXCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static DataService.Builder copyExcept(final DataResource _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.EXCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static DataService.Builder copyExcept(final DataService _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.EXCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static DataService.Builder copyOnly(final Resource _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.INCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static DataService.Builder copyOnly(final Service _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.INCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static DataService.Builder copyOnly(final DataResource _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.INCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static DataService.Builder copyOnly(final DataService _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.INCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public DataService visit(final PropertyVisitor _visitor_) {
super.visit(_visitor_);
return this;
}
public static class Builder<_B >
extends DataResource.Builder<_B>
implements Buildable
{
public Builder(final _B _parentBuilder, final DataService _other, final boolean _copy) {
super(_parentBuilder, _other, _copy);
if (_other!= null) {
}
}
public Builder(final _B _parentBuilder, final DataService _other, final boolean _copy, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
super(_parentBuilder, _other, _copy, _propertyTree, _propertyTreeUse);
if (_other!= null) {
}
}
protected<_P extends DataService >_P init(final _P _product) {
return super.init(_product);
}
/**
* Adds the given items to the value of "facilities"
*
* @param facilities
* Items to add to the value of the "facilities" property
*/
@Override
public DataService.Builder<_B> addFacilities(final Iterable extends ResourceName> facilities) {
super.addFacilities(facilities);
return this;
}
/**
* Adds the given items to the value of "facilities"
*
* @param facilities
* Items to add to the value of the "facilities" property
*/
@Override
public DataService.Builder<_B> addFacilities(ResourceName... facilities) {
super.addFacilities(facilities);
return this;
}
/**
* Sets the new value of "facilities" (any previous value will be replaced)
*
* @param facilities
* New value of the "facilities" property.
*/
@Override
public DataService.Builder<_B> withFacilities(final Iterable extends ResourceName> facilities) {
super.withFacilities(facilities);
return this;
}
/**
* Sets the new value of "facilities" (any previous value will be replaced)
*
* @param facilities
* New value of the "facilities" property.
*/
@Override
public DataService.Builder<_B> withFacilities(ResourceName... facilities) {
super.withFacilities(facilities);
return this;
}
/**
* Adds the given items to the value of "instruments"
*
* @param instruments
* Items to add to the value of the "instruments" property
*/
@Override
public DataService.Builder<_B> addInstruments(final Iterable extends ResourceName> instruments) {
super.addInstruments(instruments);
return this;
}
/**
* Adds the given items to the value of "instruments"
*
* @param instruments
* Items to add to the value of the "instruments" property
*/
@Override
public DataService.Builder<_B> addInstruments(ResourceName... instruments) {
super.addInstruments(instruments);
return this;
}
/**
* Sets the new value of "instruments" (any previous value will be replaced)
*
* @param instruments
* New value of the "instruments" property.
*/
@Override
public DataService.Builder<_B> withInstruments(final Iterable extends ResourceName> instruments) {
super.withInstruments(instruments);
return this;
}
/**
* Sets the new value of "instruments" (any previous value will be replaced)
*
* @param instruments
* New value of the "instruments" property.
*/
@Override
public DataService.Builder<_B> withInstruments(ResourceName... instruments) {
super.withInstruments(instruments);
return this;
}
/**
* Sets the new value of "coverage" (any previous value will be replaced)
*
* @param coverage
* New value of the "coverage" property.
*/
@Override
public DataService.Builder<_B> withCoverage(final Coverage coverage) {
super.withCoverage(coverage);
return this;
}
/**
* Returns the existing builder or a new builder to build the value of the
* "coverage" property.
* Use {@link
* org.javastro.ivoa.entities.resource.dataservice.Coverage.Builder#end()} to
* return to the current builder.
*
* @return
* A new builder to build the value of the "coverage" property.
* Use {@link
* org.javastro.ivoa.entities.resource.dataservice.Coverage.Builder#end()} to
* return to the current builder.
*/
public Coverage.Builder extends DataService.Builder<_B>> withCoverage() {
return ((Coverage.Builder extends DataService.Builder<_B>> ) super.withCoverage());
}
/**
* Adds the given items to the value of "rights"
*
* @param rights
* Items to add to the value of the "rights" property
*/
@Override
public DataService.Builder<_B> addRights(final Iterable extends Rights> rights) {
super.addRights(rights);
return this;
}
/**
* Adds the given items to the value of "rights"
*
* @param rights
* Items to add to the value of the "rights" property
*/
@Override
public DataService.Builder<_B> addRights(Rights... rights) {
super.addRights(rights);
return this;
}
/**
* Sets the new value of "rights" (any previous value will be replaced)
*
* @param rights
* New value of the "rights" property.
*/
@Override
public DataService.Builder<_B> withRights(final Iterable extends Rights> rights) {
super.withRights(rights);
return this;
}
/**
* Sets the new value of "rights" (any previous value will be replaced)
*
* @param rights
* New value of the "rights" property.
*/
@Override
public DataService.Builder<_B> withRights(Rights... rights) {
super.withRights(rights);
return this;
}
/**
* Adds the given items to the value of "capabilities"
*
* @param capabilities
* Items to add to the value of the "capabilities" property
*/
@Override
public DataService.Builder<_B> addCapabilities(final Iterable extends Capability> capabilities) {
super.addCapabilities(capabilities);
return this;
}
/**
* Adds the given items to the value of "capabilities"
*
* @param capabilities
* Items to add to the value of the "capabilities" property
*/
@Override
public DataService.Builder<_B> addCapabilities(Capability... capabilities) {
super.addCapabilities(capabilities);
return this;
}
/**
* Sets the new value of "capabilities" (any previous value will be replaced)
*
* @param capabilities
* New value of the "capabilities" property.
*/
@Override
public DataService.Builder<_B> withCapabilities(final Iterable extends Capability> capabilities) {
super.withCapabilities(capabilities);
return this;
}
/**
* Sets the new value of "capabilities" (any previous value will be replaced)
*
* @param capabilities
* New value of the "capabilities" property.
*/
@Override
public DataService.Builder<_B> withCapabilities(Capability... capabilities) {
super.withCapabilities(capabilities);
return this;
}
/**
* Adds the given items to the value of "validationLevels"
*
* @param validationLevels
* Items to add to the value of the "validationLevels" property
*/
@Override
public DataService.Builder<_B> addValidationLevels(final Iterable extends Validation> validationLevels) {
super.addValidationLevels(validationLevels);
return this;
}
/**
* Adds the given items to the value of "validationLevels"
*
* @param validationLevels
* Items to add to the value of the "validationLevels" property
*/
@Override
public DataService.Builder<_B> addValidationLevels(Validation... validationLevels) {
super.addValidationLevels(validationLevels);
return this;
}
/**
* Sets the new value of "validationLevels" (any previous value will be replaced)
*
* @param validationLevels
* New value of the "validationLevels" property.
*/
@Override
public DataService.Builder<_B> withValidationLevels(final Iterable extends Validation> validationLevels) {
super.withValidationLevels(validationLevels);
return this;
}
/**
* Sets the new value of "validationLevels" (any previous value will be replaced)
*
* @param validationLevels
* New value of the "validationLevels" property.
*/
@Override
public DataService.Builder<_B> withValidationLevels(Validation... validationLevels) {
super.withValidationLevels(validationLevels);
return this;
}
/**
* Sets the new value of "title" (any previous value will be replaced)
*
* @param title
* New value of the "title" property.
*/
@Override
public DataService.Builder<_B> withTitle(final String title) {
super.withTitle(title);
return this;
}
/**
* Sets the new value of "shortName" (any previous value will be replaced)
*
* @param shortName
* New value of the "shortName" property.
*/
@Override
public DataService.Builder<_B> withShortName(final String shortName) {
super.withShortName(shortName);
return this;
}
/**
* Sets the new value of "identifier" (any previous value will be replaced)
*
* @param identifier
* New value of the "identifier" property.
*/
@Override
public DataService.Builder<_B> withIdentifier(final String identifier) {
super.withIdentifier(identifier);
return this;
}
/**
* Adds the given items to the value of "altIdentifiers"
*
* @param altIdentifiers
* Items to add to the value of the "altIdentifiers" property
*/
@Override
public DataService.Builder<_B> addAltIdentifiers(final Iterable extends String> altIdentifiers) {
super.addAltIdentifiers(altIdentifiers);
return this;
}
/**
* Adds the given items to the value of "altIdentifiers"
*
* @param altIdentifiers
* Items to add to the value of the "altIdentifiers" property
*/
@Override
public DataService.Builder<_B> addAltIdentifiers(String... altIdentifiers) {
super.addAltIdentifiers(altIdentifiers);
return this;
}
/**
* Sets the new value of "altIdentifiers" (any previous value will be replaced)
*
* @param altIdentifiers
* New value of the "altIdentifiers" property.
*/
@Override
public DataService.Builder<_B> withAltIdentifiers(final Iterable extends String> altIdentifiers) {
super.withAltIdentifiers(altIdentifiers);
return this;
}
/**
* Sets the new value of "altIdentifiers" (any previous value will be replaced)
*
* @param altIdentifiers
* New value of the "altIdentifiers" property.
*/
@Override
public DataService.Builder<_B> withAltIdentifiers(String... altIdentifiers) {
super.withAltIdentifiers(altIdentifiers);
return this;
}
/**
* Sets the new value of "curation" (any previous value will be replaced)
*
* @param curation
* New value of the "curation" property.
*/
@Override
public DataService.Builder<_B> withCuration(final Curation curation) {
super.withCuration(curation);
return this;
}
/**
* Returns the existing builder or a new builder to build the value of the
* "curation" property.
* Use {@link org.javastro.ivoa.entities.resource.Curation.Builder#end()} to return
* to the current builder.
*
* @return
* A new builder to build the value of the "curation" property.
* Use {@link org.javastro.ivoa.entities.resource.Curation.Builder#end()} to return
* to the current builder.
*/
public Curation.Builder extends DataService.Builder<_B>> withCuration() {
return ((Curation.Builder extends DataService.Builder<_B>> ) super.withCuration());
}
/**
* Sets the new value of "content" (any previous value will be replaced)
*
* @param content
* New value of the "content" property.
*/
@Override
public DataService.Builder<_B> withContent(final Content content) {
super.withContent(content);
return this;
}
/**
* Returns the existing builder or a new builder to build the value of the
* "content" property.
* Use {@link org.javastro.ivoa.entities.resource.Content.Builder#end()} to return
* to the current builder.
*
* @return
* A new builder to build the value of the "content" property.
* Use {@link org.javastro.ivoa.entities.resource.Content.Builder#end()} to return
* to the current builder.
*/
public Content.Builder extends DataService.Builder<_B>> withContent() {
return ((Content.Builder extends DataService.Builder<_B>> ) super.withContent());
}
/**
* Sets the new value of "created" (any previous value will be replaced)
*
* @param created
* New value of the "created" property.
*/
@Override
public DataService.Builder<_B> withCreated(final LocalDateTime created) {
super.withCreated(created);
return this;
}
/**
* Sets the new value of "updated" (any previous value will be replaced)
*
* @param updated
* New value of the "updated" property.
*/
@Override
public DataService.Builder<_B> withUpdated(final LocalDateTime updated) {
super.withUpdated(updated);
return this;
}
/**
* Sets the new value of "status" (any previous value will be replaced)
*
* @param status
* New value of the "status" property.
*/
@Override
public DataService.Builder<_B> withStatus(final String status) {
super.withStatus(status);
return this;
}
/**
* Sets the new value of "version" (any previous value will be replaced)
*
* @param version
* New value of the "version" property.
*/
@Override
public DataService.Builder<_B> withVersion(final String version) {
super.withVersion(version);
return this;
}
@Override
public DataService build() {
if (_storedValue == null) {
return this.init(new DataService());
} else {
return ((DataService) _storedValue);
}
}
public DataService.Builder<_B> copyOf(final DataService _other) {
_other.copyTo(this);
return this;
}
public DataService.Builder<_B> copyOf(final DataService.Builder _other) {
return copyOf(_other.build());
}
}
public class Modifier
extends DataResource.Modifier
{
}
public static class PropInfo {
}
public static class Select
extends DataService.Selector
{
Select() {
super(null, null, null);
}
public static DataService.Select _root() {
return new DataService.Select();
}
}
public static class Selector , TParent >
extends DataResource.Selector
{
public Selector(final TRoot root, final TParent parent, final String propertyName) {
super(root, parent, propertyName);
}
@Override
public Map buildChildren() {
final Map products = new HashMap<>();
products.putAll(super.buildChildren());
return products;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy