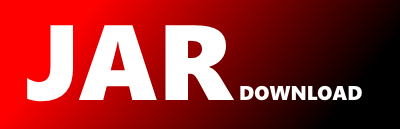
org.javastro.ivoa.entities.resource.dataservice.InputParam Maven / Gradle / Ivy
package org.javastro.ivoa.entities.resource.dataservice;
import java.util.HashMap;
import java.util.Map;
import javax.xml.namespace.QName;
import com.kscs.util.jaxb.Buildable;
import com.kscs.util.jaxb.Copyable;
import com.kscs.util.jaxb.PartialCopyable;
import com.kscs.util.jaxb.PropertyTree;
import com.kscs.util.jaxb.PropertyTreeUse;
import com.kscs.util.jaxb.PropertyVisitor;
import com.kscs.util.jaxb.SingleProperty;
import com.kscs.util.jaxb.SinglePropertyInfo;
import com.kscs.util.jaxb.SinglePropertyInfo;
import com.kscs.util.jaxb.SinglePropertyInfo;
import com.kscs.util.jaxb.SinglePropertyInfo;
import jakarta.annotation.Generated;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlAttribute;
import jakarta.xml.bind.annotation.XmlType;
import org.jvnet.jaxb.lang.JAXBMergeStrategy;
import org.jvnet.jaxb.lang.JAXBToStringStrategy;
import org.jvnet.jaxb.lang.MergeFrom;
import org.jvnet.jaxb.lang.MergeStrategy;
import org.jvnet.jaxb.lang.ToString;
import org.jvnet.jaxb.lang.ToStringStrategy;
import org.jvnet.jaxb.locator.ObjectLocator;
import org.jvnet.jaxb.locator.util.LocatorUtils;
/**
* The allowed data type names do not imply a size or precise
* format. This type is intended to be sufficient for describing
* an input parameter to a simple REST service or a function
* written in a weakly-typed language.
*
* Java class for InputParam complex type
.
*
* The following schema fragment specifies the expected content contained within this class.
*
* {@code
*
*
*
*
*
*
*
*
*
*
*
*
* }
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "InputParam", propOrder = {
"dataType"
})
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public class InputParam
extends BaseParam
implements Cloneable, Copyable, PartialCopyable, MergeFrom, ToString
{
/**
* A type of data contained in the parameter
*
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
protected SimpleDataType dataType;
/**
* An indication of whether this parameter is
* required to be provided for the application
* or service to work properly.
*
*/
@XmlAttribute(name = "use")
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
protected ParamUse use;
/**
* If true, the meaning and behavior of this parameter is
* reserved and defined by a standard interface. If
* false, it represents an implementation-specific
* parameter that effectively extends the behavior of the
* service or application.
*
*/
@XmlAttribute(name = "std")
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
protected Boolean std;
/**
* Default no-arg constructor
*
*/
public InputParam() {
super();
}
/**
* Fully-initialising value constructor
*
*/
public InputParam(final String name, final String description, final String unit, final String ucd, final String utype, final Map otherAttributes, final SimpleDataType dataType, final ParamUse use, final Boolean std) {
super(name, description, unit, ucd, utype, otherAttributes);
this.dataType = dataType;
this.use = use;
this.std = std;
}
/**
* A type of data contained in the parameter
*
* @return
* possible object is
* {@link SimpleDataType }
*
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public SimpleDataType getDataType() {
return dataType;
}
/**
* Sets the value of the dataType property.
*
* @param value
* allowed object is
* {@link SimpleDataType }
*
* @see #getDataType()
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void setDataType(SimpleDataType value) {
this.dataType = value;
}
/**
* An indication of whether this parameter is
* required to be provided for the application
* or service to work properly.
*
* @return
* possible object is
* {@link ParamUse }
*
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public ParamUse getUse() {
if (use == null) {
return ParamUse.OPTIONAL;
} else {
return use;
}
}
/**
* Sets the value of the use property.
*
* @param value
* allowed object is
* {@link ParamUse }
*
* @see #getUse()
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void setUse(ParamUse value) {
this.use = value;
}
/**
* If true, the meaning and behavior of this parameter is
* reserved and defined by a standard interface. If
* false, it represents an implementation-specific
* parameter that effectively extends the behavior of the
* service or application.
*
* @return
* possible object is
* {@link Boolean }
*
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public boolean isStd() {
if (std == null) {
return true;
} else {
return std;
}
}
/**
* Sets the value of the std property.
*
* @param value
* allowed object is
* {@link Boolean }
*
* @see #isStd()
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void setStd(Boolean value) {
this.std = value;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public boolean equals(Object object) {
if ((object == null)||(this.getClass()!= object.getClass())) {
return false;
}
if (this == object) {
return true;
}
if (!super.equals(object)) {
return false;
}
final InputParam that = ((InputParam) object);
{
SimpleDataType leftDataType;
leftDataType = this.getDataType();
SimpleDataType rightDataType;
rightDataType = that.getDataType();
if (this.dataType!= null) {
if (that.dataType!= null) {
if (!leftDataType.equals(rightDataType)) {
return false;
}
} else {
return false;
}
} else {
if (that.dataType!= null) {
return false;
}
}
}
{
ParamUse leftUse;
leftUse = this.getUse();
ParamUse rightUse;
rightUse = that.getUse();
if (this.use!= null) {
if (that.use!= null) {
if (!leftUse.equals(rightUse)) {
return false;
}
} else {
return false;
}
} else {
if (that.use!= null) {
return false;
}
}
}
{
boolean leftStd;
leftStd = ((this.std!= null)?this.isStd():true);
boolean rightStd;
rightStd = ((that.std!= null)?that.isStd():true);
if (this.std!= null) {
if (that.std!= null) {
if (leftStd!= rightStd) {
return false;
}
} else {
return false;
}
} else {
if (that.std!= null) {
return false;
}
}
}
return true;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public int hashCode() {
int currentHashCode = 1;
currentHashCode = ((currentHashCode* 31)+ super.hashCode());
{
currentHashCode = (currentHashCode* 31);
SimpleDataType theDataType;
theDataType = this.getDataType();
if (this.dataType!= null) {
currentHashCode += theDataType.hashCode();
}
}
{
currentHashCode = (currentHashCode* 31);
ParamUse theUse;
theUse = this.getUse();
if (this.use!= null) {
currentHashCode += theUse.hashCode();
}
}
{
currentHashCode = (currentHashCode* 31);
boolean theStd;
theStd = ((this.std!= null)?this.isStd():true);
if (this.std!= null) {
currentHashCode += (theStd? 1231 : 1237);
}
}
return currentHashCode;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public String toString() {
final ToStringStrategy strategy = JAXBToStringStrategy.getInstance();
final StringBuilder buffer = new StringBuilder();
append(null, buffer, strategy);
return buffer.toString();
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public StringBuilder append(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
strategy.appendStart(locator, this, buffer);
appendFields(locator, buffer, strategy);
strategy.appendEnd(locator, this, buffer);
return buffer;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public StringBuilder appendFields(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
super.appendFields(locator, buffer, strategy);
{
SimpleDataType theDataType;
theDataType = this.getDataType();
strategy.appendField(locator, this, "dataType", buffer, theDataType, (this.dataType!= null));
}
{
ParamUse theUse;
theUse = this.getUse();
strategy.appendField(locator, this, "use", buffer, theUse, (this.use!= null));
}
{
boolean theStd;
theStd = ((this.std!= null)?this.isStd():true);
strategy.appendField(locator, this, "std", buffer, theStd, (this.std!= null));
}
return buffer;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void mergeFrom(Object left, Object right) {
final MergeStrategy strategy = JAXBMergeStrategy.getInstance();
mergeFrom(null, null, left, right, strategy);
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void mergeFrom(ObjectLocator leftLocator, ObjectLocator rightLocator, Object left, Object right, MergeStrategy strategy) {
super.mergeFrom(leftLocator, rightLocator, left, right, strategy);
if (right instanceof InputParam) {
final InputParam target = this;
final InputParam leftObject = ((InputParam) left);
final InputParam rightObject = ((InputParam) right);
{
Boolean dataTypeShouldBeMergedAndSet = strategy.shouldBeMergedAndSet(leftLocator, rightLocator, (leftObject.dataType!= null), (rightObject.dataType!= null));
if (dataTypeShouldBeMergedAndSet == Boolean.TRUE) {
SimpleDataType lhsDataType;
lhsDataType = leftObject.getDataType();
SimpleDataType rhsDataType;
rhsDataType = rightObject.getDataType();
SimpleDataType mergedDataType = ((SimpleDataType) strategy.merge(LocatorUtils.property(leftLocator, "dataType", lhsDataType), LocatorUtils.property(rightLocator, "dataType", rhsDataType), lhsDataType, rhsDataType, (leftObject.dataType!= null), (rightObject.dataType!= null)));
target.setDataType(mergedDataType);
} else {
if (dataTypeShouldBeMergedAndSet == Boolean.FALSE) {
target.dataType = null;
}
}
}
{
Boolean useShouldBeMergedAndSet = strategy.shouldBeMergedAndSet(leftLocator, rightLocator, (leftObject.use!= null), (rightObject.use!= null));
if (useShouldBeMergedAndSet == Boolean.TRUE) {
ParamUse lhsUse;
lhsUse = leftObject.getUse();
ParamUse rhsUse;
rhsUse = rightObject.getUse();
ParamUse mergedUse = ((ParamUse) strategy.merge(LocatorUtils.property(leftLocator, "use", lhsUse), LocatorUtils.property(rightLocator, "use", rhsUse), lhsUse, rhsUse, (leftObject.use!= null), (rightObject.use!= null)));
target.setUse(mergedUse);
} else {
if (useShouldBeMergedAndSet == Boolean.FALSE) {
target.use = null;
}
}
}
{
Boolean stdShouldBeMergedAndSet = strategy.shouldBeMergedAndSet(leftLocator, rightLocator, (leftObject.std!= null), (rightObject.std!= null));
if (stdShouldBeMergedAndSet == Boolean.TRUE) {
boolean lhsStd;
lhsStd = ((leftObject.std!= null)?leftObject.isStd():true);
boolean rhsStd;
rhsStd = ((rightObject.std!= null)?rightObject.isStd():true);
boolean mergedStd = ((boolean) strategy.merge(LocatorUtils.property(leftLocator, "std", lhsStd), LocatorUtils.property(rightLocator, "std", rhsStd), lhsStd, rhsStd, (leftObject.std!= null), (rightObject.std!= null)));
target.setStd(mergedStd);
} else {
if (stdShouldBeMergedAndSet == Boolean.FALSE) {
target.std = null;
}
}
}
}
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Object createNewInstance() {
return new InputParam();
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public InputParam clone() {
final InputParam _newObject;
_newObject = ((InputParam) super.clone());
_newObject.dataType = ((this.dataType == null)?null:this.dataType.clone());
return _newObject;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public InputParam createCopy() {
final InputParam _newObject = ((InputParam) super.createCopy());
_newObject.dataType = ((this.dataType == null)?null:this.dataType.createCopy());
_newObject.use = this.use;
_newObject.std = this.std;
return _newObject;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public InputParam createCopy(final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
final InputParam _newObject = ((InputParam) super.createCopy(_propertyTree, _propertyTreeUse));
final PropertyTree dataTypePropertyTree = ((_propertyTree == null)?null:_propertyTree.get("dataType"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(dataTypePropertyTree!= null):((dataTypePropertyTree == null)||(!dataTypePropertyTree.isLeaf())))) {
_newObject.dataType = ((this.dataType == null)?null:this.dataType.createCopy(dataTypePropertyTree, _propertyTreeUse));
}
final PropertyTree usePropertyTree = ((_propertyTree == null)?null:_propertyTree.get("use"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(usePropertyTree!= null):((usePropertyTree == null)||(!usePropertyTree.isLeaf())))) {
_newObject.use = this.use;
}
final PropertyTree stdPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("std"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(stdPropertyTree!= null):((stdPropertyTree == null)||(!stdPropertyTree.isLeaf())))) {
_newObject.std = this.std;
}
return _newObject;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public InputParam copyExcept(final PropertyTree _propertyTree) {
return createCopy(_propertyTree, PropertyTreeUse.EXCLUDE);
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public InputParam copyOnly(final PropertyTree _propertyTree) {
return createCopy(_propertyTree, PropertyTreeUse.INCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public InputParam.Modifier modifier() {
if (null == this.__cachedModifier__) {
this.__cachedModifier__ = new InputParam.Modifier();
}
return ((InputParam.Modifier) this.__cachedModifier__);
}
/**
* Copies all state of this object to a builder. This method is used by the copyOf
* method and should not be called directly by client code.
*
* @param _other
* A builder instance to which the state of this object will be copied.
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public<_B >void copyTo(final InputParam.Builder<_B> _other) {
super.copyTo(_other);
_other.dataType = ((this.dataType == null)?null:this.dataType.newCopyBuilder(_other));
_other.use = this.use;
_other.std = this.std;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public<_B >InputParam.Builder<_B> newCopyBuilder(final _B _parentBuilder) {
return new InputParam.Builder<_B>(_parentBuilder, this, true);
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public InputParam.Builder newCopyBuilder() {
return newCopyBuilder(null);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static InputParam.Builder builder() {
return new InputParam.Builder<>(null, null, false);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static<_B >InputParam.Builder<_B> copyOf(final BaseParam _other) {
final InputParam.Builder<_B> _newBuilder = new InputParam.Builder<>(null, null, false);
_other.copyTo(_newBuilder);
return _newBuilder;
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static<_B >InputParam.Builder<_B> copyOf(final InputParam _other) {
final InputParam.Builder<_B> _newBuilder = new InputParam.Builder<>(null, null, false);
_other.copyTo(_newBuilder);
return _newBuilder;
}
/**
* Copies all state of this object to a builder. This method is used by the copyOf
* method and should not be called directly by client code.
*
* @param _other
* A builder instance to which the state of this object will be copied.
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public<_B >void copyTo(final InputParam.Builder<_B> _other, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
super.copyTo(_other, _propertyTree, _propertyTreeUse);
final PropertyTree dataTypePropertyTree = ((_propertyTree == null)?null:_propertyTree.get("dataType"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(dataTypePropertyTree!= null):((dataTypePropertyTree == null)||(!dataTypePropertyTree.isLeaf())))) {
_other.dataType = ((this.dataType == null)?null:this.dataType.newCopyBuilder(_other, dataTypePropertyTree, _propertyTreeUse));
}
final PropertyTree usePropertyTree = ((_propertyTree == null)?null:_propertyTree.get("use"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(usePropertyTree!= null):((usePropertyTree == null)||(!usePropertyTree.isLeaf())))) {
_other.use = this.use;
}
final PropertyTree stdPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("std"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(stdPropertyTree!= null):((stdPropertyTree == null)||(!stdPropertyTree.isLeaf())))) {
_other.std = this.std;
}
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public<_B >InputParam.Builder<_B> newCopyBuilder(final _B _parentBuilder, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
return new InputParam.Builder<_B>(_parentBuilder, this, true, _propertyTree, _propertyTreeUse);
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public InputParam.Builder newCopyBuilder(final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
return newCopyBuilder(null, _propertyTree, _propertyTreeUse);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static<_B >InputParam.Builder<_B> copyOf(final BaseParam _other, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
final InputParam.Builder<_B> _newBuilder = new InputParam.Builder<>(null, null, false);
_other.copyTo(_newBuilder, _propertyTree, _propertyTreeUse);
return _newBuilder;
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static<_B >InputParam.Builder<_B> copyOf(final InputParam _other, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
final InputParam.Builder<_B> _newBuilder = new InputParam.Builder<>(null, null, false);
_other.copyTo(_newBuilder, _propertyTree, _propertyTreeUse);
return _newBuilder;
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static InputParam.Builder copyExcept(final BaseParam _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.EXCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static InputParam.Builder copyExcept(final InputParam _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.EXCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static InputParam.Builder copyOnly(final BaseParam _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.INCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static InputParam.Builder copyOnly(final InputParam _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.INCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public InputParam visit(final PropertyVisitor _visitor_) {
super.visit(_visitor_);
if (_visitor_.visit(new SingleProperty<>(InputParam.PropInfo.DATA_TYPE, this))&&(this.dataType!= null)) {
this.dataType.visit(_visitor_);
}
_visitor_.visit(new SingleProperty<>(InputParam.PropInfo.USE, this));
_visitor_.visit(new SingleProperty<>(InputParam.PropInfo.STD, this));
return this;
}
public static class Builder<_B >
extends BaseParam.Builder<_B>
implements Buildable
{
private SimpleDataType.Builder> dataType;
private ParamUse use = ParamUse.OPTIONAL;
private Boolean std = true;
public Builder(final _B _parentBuilder, final InputParam _other, final boolean _copy) {
super(_parentBuilder, _other, _copy);
if (_other!= null) {
this.dataType = ((_other.dataType == null)?null:_other.dataType.newCopyBuilder(this));
this.use = _other.use;
this.std = _other.std;
}
}
public Builder(final _B _parentBuilder, final InputParam _other, final boolean _copy, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
super(_parentBuilder, _other, _copy, _propertyTree, _propertyTreeUse);
if (_other!= null) {
final PropertyTree dataTypePropertyTree = ((_propertyTree == null)?null:_propertyTree.get("dataType"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(dataTypePropertyTree!= null):((dataTypePropertyTree == null)||(!dataTypePropertyTree.isLeaf())))) {
this.dataType = ((_other.dataType == null)?null:_other.dataType.newCopyBuilder(this, dataTypePropertyTree, _propertyTreeUse));
}
final PropertyTree usePropertyTree = ((_propertyTree == null)?null:_propertyTree.get("use"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(usePropertyTree!= null):((usePropertyTree == null)||(!usePropertyTree.isLeaf())))) {
this.use = _other.use;
}
final PropertyTree stdPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("std"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(stdPropertyTree!= null):((stdPropertyTree == null)||(!stdPropertyTree.isLeaf())))) {
this.std = _other.std;
}
}
}
protected<_P extends InputParam >_P init(final _P _product) {
_product.dataType = ((this.dataType == null)?null:this.dataType.build());
_product.use = this.use;
_product.std = this.std;
return super.init(_product);
}
/**
* Sets the new value of "dataType" (any previous value will be replaced)
*
* @param dataType
* New value of the "dataType" property.
*/
public InputParam.Builder<_B> withDataType(final SimpleDataType dataType) {
this.dataType = ((dataType == null)?null:new SimpleDataType.Builder<>(this, dataType, false));
return this;
}
/**
* Returns the existing builder or a new builder to build the value of the
* "dataType" property.
* Use {@link
* org.javastro.ivoa.entities.resource.dataservice.SimpleDataType.Builder#end()} to
* return to the current builder.
*
* @return
* A new builder to build the value of the "dataType" property.
* Use {@link
* org.javastro.ivoa.entities.resource.dataservice.SimpleDataType.Builder#end()} to
* return to the current builder.
*/
public SimpleDataType.Builder extends InputParam.Builder<_B>> withDataType() {
if (this.dataType!= null) {
return this.dataType;
}
return this.dataType = new SimpleDataType.Builder<>(this, null, false);
}
/**
* Sets the new value of "use" (any previous value will be replaced)
*
* @param use
* New value of the "use" property.
*/
public InputParam.Builder<_B> withUse(final ParamUse use) {
this.use = use;
return this;
}
/**
* Sets the new value of "std" (any previous value will be replaced)
*
* @param std
* New value of the "std" property.
*/
public InputParam.Builder<_B> withStd(final Boolean std) {
this.std = std;
return this;
}
/**
* Sets the new value of "name" (any previous value will be replaced)
*
* @param name
* New value of the "name" property.
*/
@Override
public InputParam.Builder<_B> withName(final String name) {
super.withName(name);
return this;
}
/**
* Sets the new value of "description" (any previous value will be replaced)
*
* @param description
* New value of the "description" property.
*/
@Override
public InputParam.Builder<_B> withDescription(final String description) {
super.withDescription(description);
return this;
}
/**
* Sets the new value of "unit" (any previous value will be replaced)
*
* @param unit
* New value of the "unit" property.
*/
@Override
public InputParam.Builder<_B> withUnit(final String unit) {
super.withUnit(unit);
return this;
}
/**
* Sets the new value of "ucd" (any previous value will be replaced)
*
* @param ucd
* New value of the "ucd" property.
*/
@Override
public InputParam.Builder<_B> withUcd(final String ucd) {
super.withUcd(ucd);
return this;
}
/**
* Sets the new value of "utype" (any previous value will be replaced)
*
* @param utype
* New value of the "utype" property.
*/
@Override
public InputParam.Builder<_B> withUtype(final String utype) {
super.withUtype(utype);
return this;
}
@Override
public InputParam build() {
if (_storedValue == null) {
return this.init(new InputParam());
} else {
return ((InputParam) _storedValue);
}
}
public InputParam.Builder<_B> copyOf(final InputParam _other) {
_other.copyTo(this);
return this;
}
public InputParam.Builder<_B> copyOf(final InputParam.Builder _other) {
return copyOf(_other.build());
}
}
public class Modifier
extends BaseParam.Modifier
{
public void setDataType(final SimpleDataType dataType) {
InputParam.this.setDataType(dataType);
}
public void setUse(final ParamUse use) {
InputParam.this.setUse(use);
}
public void setStd(final Boolean std) {
InputParam.this.setStd(std);
}
}
public static class PropInfo {
public static final transient SinglePropertyInfo DATA_TYPE = new SinglePropertyInfo("dataType", InputParam.class, SimpleDataType.class, false, null, new QName("", "dataType"), new QName("http://www.ivoa.net/xml/VODataService/v1.1", "SimpleDataType"), false) {
@Override
public SimpleDataType get(final InputParam _instance_) {
return ((_instance_ == null)?null:_instance_.dataType);
}
@Override
public void set(final InputParam _instance_, final SimpleDataType _value_) {
if (_instance_!= null) {
_instance_.dataType = _value_;
}
}
}
;
public static final transient SinglePropertyInfo USE = new SinglePropertyInfo("use", InputParam.class, ParamUse.class, false, ParamUse.OPTIONAL, new QName("", "use"), new QName("http://www.ivoa.net/xml/VODataService/v1.1", "ParamUse"), true) {
@Override
public ParamUse get(final InputParam _instance_) {
return ((_instance_ == null)?null:_instance_.use);
}
@Override
public void set(final InputParam _instance_, final ParamUse _value_) {
if (_instance_!= null) {
_instance_.use = _value_;
}
}
}
;
public static final transient SinglePropertyInfo STD = new SinglePropertyInfo("std", InputParam.class, Boolean.class, false, true, new QName("", "std"), new QName("http://www.w3.org/2001/XMLSchema", "boolean"), true) {
@Override
public Boolean get(final InputParam _instance_) {
return ((_instance_ == null)?null:_instance_.std);
}
@Override
public void set(final InputParam _instance_, final Boolean _value_) {
if (_instance_!= null) {
_instance_.std = _value_;
}
}
}
;
}
public static class Select
extends InputParam.Selector
{
Select() {
super(null, null, null);
}
public static InputParam.Select _root() {
return new InputParam.Select();
}
}
public static class Selector , TParent >
extends BaseParam.Selector
{
private SimpleDataType.Selector> dataType = null;
private com.kscs.util.jaxb.Selector> use = null;
private com.kscs.util.jaxb.Selector> std = null;
public Selector(final TRoot root, final TParent parent, final String propertyName) {
super(root, parent, propertyName);
}
@Override
public Map buildChildren() {
final Map products = new HashMap<>();
products.putAll(super.buildChildren());
if (this.dataType!= null) {
products.put("dataType", this.dataType.init());
}
if (this.use!= null) {
products.put("use", this.use.init());
}
if (this.std!= null) {
products.put("std", this.std.init());
}
return products;
}
public SimpleDataType.Selector> dataType() {
return ((this.dataType == null)?this.dataType = new SimpleDataType.Selector<>(this._root, this, "dataType"):this.dataType);
}
public com.kscs.util.jaxb.Selector> use() {
return ((this.use == null)?this.use = new com.kscs.util.jaxb.Selector<>(this._root, this, "use"):this.use);
}
public com.kscs.util.jaxb.Selector> std() {
return ((this.std == null)?this.std = new com.kscs.util.jaxb.Selector<>(this._root, this, "std"):this.std);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy