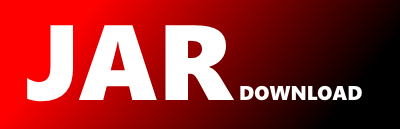
org.javastro.ivoa.entities.resource.dataservice.ServiceReference Maven / Gradle / Ivy
package org.javastro.ivoa.entities.resource.dataservice;
import java.util.HashMap;
import java.util.Map;
import javax.xml.namespace.QName;
import com.kscs.util.jaxb.Buildable;
import com.kscs.util.jaxb.Copyable;
import com.kscs.util.jaxb.PartialCopyable;
import com.kscs.util.jaxb.PropertyTree;
import com.kscs.util.jaxb.PropertyTreeUse;
import com.kscs.util.jaxb.PropertyVisitor;
import com.kscs.util.jaxb.SingleProperty;
import com.kscs.util.jaxb.SinglePropertyInfo;
import com.kscs.util.jaxb.SinglePropertyInfo;
import jakarta.annotation.Generated;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlAttribute;
import jakarta.xml.bind.annotation.XmlSchemaType;
import jakarta.xml.bind.annotation.XmlType;
import jakarta.xml.bind.annotation.XmlValue;
import org.jvnet.jaxb.lang.JAXBMergeStrategy;
import org.jvnet.jaxb.lang.JAXBToStringStrategy;
import org.jvnet.jaxb.lang.MergeFrom;
import org.jvnet.jaxb.lang.MergeStrategy;
import org.jvnet.jaxb.lang.ToString;
import org.jvnet.jaxb.lang.ToStringStrategy;
import org.jvnet.jaxb.locator.ObjectLocator;
import org.jvnet.jaxb.locator.util.LocatorUtils;
/**
* The service URL for a potentially registerd service. That is,
* if an IVOA identifier is also provided, then the service is
* described in a registry.
*
* Java class for ServiceReference complex type
.
*
* The following schema fragment specifies the expected content contained within this class.
*
* {@code
*
*
*
*
*
*
*
* }
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "ServiceReference", propOrder = {
"value"
})
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public class ServiceReference implements Cloneable, Copyable, PartialCopyable, MergeFrom, ToString
{
@XmlValue
@XmlSchemaType(name = "anyURI")
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
protected String value;
/**
* The URI form of the IVOA identifier for the service
* describing the capability refered to by this element.
*
*/
@XmlAttribute(name = "ivo-id")
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
protected String ivoId;
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
protected transient ServiceReference.Modifier __cachedModifier__;
/**
* Default no-arg constructor
*
*/
public ServiceReference() {
super();
}
/**
* Fully-initialising value constructor
*
*/
public ServiceReference(final String value, final String ivoId) {
this.value = value;
this.ivoId = ivoId;
}
/**
* Gets the value of the value property.
*
* @return
* possible object is
* {@link String }
*
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public String getValue() {
return value;
}
/**
* Sets the value of the value property.
*
* @param value
* allowed object is
* {@link String }
*
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void setValue(String value) {
this.value = value;
}
/**
* The URI form of the IVOA identifier for the service
* describing the capability refered to by this element.
*
* @return
* possible object is
* {@link String }
*
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public String getIvoId() {
return ivoId;
}
/**
* Sets the value of the ivoId property.
*
* @param value
* allowed object is
* {@link String }
*
* @see #getIvoId()
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void setIvoId(String value) {
this.ivoId = value;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public boolean equals(Object object) {
if ((object == null)||(this.getClass()!= object.getClass())) {
return false;
}
if (this == object) {
return true;
}
final ServiceReference that = ((ServiceReference) object);
{
String leftValue;
leftValue = this.getValue();
String rightValue;
rightValue = that.getValue();
if (this.value!= null) {
if (that.value!= null) {
if (!leftValue.equals(rightValue)) {
return false;
}
} else {
return false;
}
} else {
if (that.value!= null) {
return false;
}
}
}
{
String leftIvoId;
leftIvoId = this.getIvoId();
String rightIvoId;
rightIvoId = that.getIvoId();
if (this.ivoId!= null) {
if (that.ivoId!= null) {
if (!leftIvoId.equals(rightIvoId)) {
return false;
}
} else {
return false;
}
} else {
if (that.ivoId!= null) {
return false;
}
}
}
return true;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public int hashCode() {
int currentHashCode = 1;
{
currentHashCode = (currentHashCode* 31);
String theValue;
theValue = this.getValue();
if (this.value!= null) {
currentHashCode += theValue.hashCode();
}
}
{
currentHashCode = (currentHashCode* 31);
String theIvoId;
theIvoId = this.getIvoId();
if (this.ivoId!= null) {
currentHashCode += theIvoId.hashCode();
}
}
return currentHashCode;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public String toString() {
final ToStringStrategy strategy = JAXBToStringStrategy.getInstance();
final StringBuilder buffer = new StringBuilder();
append(null, buffer, strategy);
return buffer.toString();
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public StringBuilder append(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
strategy.appendStart(locator, this, buffer);
appendFields(locator, buffer, strategy);
strategy.appendEnd(locator, this, buffer);
return buffer;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public StringBuilder appendFields(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
{
String theValue;
theValue = this.getValue();
strategy.appendField(locator, this, "value", buffer, theValue, (this.value!= null));
}
{
String theIvoId;
theIvoId = this.getIvoId();
strategy.appendField(locator, this, "ivoId", buffer, theIvoId, (this.ivoId!= null));
}
return buffer;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void mergeFrom(Object left, Object right) {
final MergeStrategy strategy = JAXBMergeStrategy.getInstance();
mergeFrom(null, null, left, right, strategy);
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void mergeFrom(ObjectLocator leftLocator, ObjectLocator rightLocator, Object left, Object right, MergeStrategy strategy) {
if (right instanceof ServiceReference) {
final ServiceReference target = this;
final ServiceReference leftObject = ((ServiceReference) left);
final ServiceReference rightObject = ((ServiceReference) right);
{
Boolean valueShouldBeMergedAndSet = strategy.shouldBeMergedAndSet(leftLocator, rightLocator, (leftObject.value!= null), (rightObject.value!= null));
if (valueShouldBeMergedAndSet == Boolean.TRUE) {
String lhsValue;
lhsValue = leftObject.getValue();
String rhsValue;
rhsValue = rightObject.getValue();
String mergedValue = ((String) strategy.merge(LocatorUtils.property(leftLocator, "value", lhsValue), LocatorUtils.property(rightLocator, "value", rhsValue), lhsValue, rhsValue, (leftObject.value!= null), (rightObject.value!= null)));
target.setValue(mergedValue);
} else {
if (valueShouldBeMergedAndSet == Boolean.FALSE) {
target.value = null;
}
}
}
{
Boolean ivoIdShouldBeMergedAndSet = strategy.shouldBeMergedAndSet(leftLocator, rightLocator, (leftObject.ivoId!= null), (rightObject.ivoId!= null));
if (ivoIdShouldBeMergedAndSet == Boolean.TRUE) {
String lhsIvoId;
lhsIvoId = leftObject.getIvoId();
String rhsIvoId;
rhsIvoId = rightObject.getIvoId();
String mergedIvoId = ((String) strategy.merge(LocatorUtils.property(leftLocator, "ivoId", lhsIvoId), LocatorUtils.property(rightLocator, "ivoId", rhsIvoId), lhsIvoId, rhsIvoId, (leftObject.ivoId!= null), (rightObject.ivoId!= null)));
target.setIvoId(mergedIvoId);
} else {
if (ivoIdShouldBeMergedAndSet == Boolean.FALSE) {
target.ivoId = null;
}
}
}
}
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Object createNewInstance() {
return new ServiceReference();
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public ServiceReference clone() {
final ServiceReference _newObject;
try {
_newObject = ((ServiceReference) super.clone());
} catch (CloneNotSupportedException e) {
throw new RuntimeException(e);
}
return _newObject;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public ServiceReference createCopy() {
final ServiceReference _newObject;
try {
_newObject = ((ServiceReference) super.clone());
} catch (CloneNotSupportedException e) {
throw new RuntimeException(e);
}
_newObject.value = this.value;
_newObject.ivoId = this.ivoId;
return _newObject;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public ServiceReference createCopy(final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
final ServiceReference _newObject;
try {
_newObject = ((ServiceReference) super.clone());
} catch (CloneNotSupportedException e) {
throw new RuntimeException(e);
}
final PropertyTree valuePropertyTree = ((_propertyTree == null)?null:_propertyTree.get("value"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(valuePropertyTree!= null):((valuePropertyTree == null)||(!valuePropertyTree.isLeaf())))) {
_newObject.value = this.value;
}
final PropertyTree ivoIdPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("ivoId"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(ivoIdPropertyTree!= null):((ivoIdPropertyTree == null)||(!ivoIdPropertyTree.isLeaf())))) {
_newObject.ivoId = this.ivoId;
}
return _newObject;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public ServiceReference copyExcept(final PropertyTree _propertyTree) {
return createCopy(_propertyTree, PropertyTreeUse.EXCLUDE);
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public ServiceReference copyOnly(final PropertyTree _propertyTree) {
return createCopy(_propertyTree, PropertyTreeUse.INCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public ServiceReference.Modifier modifier() {
if (null == this.__cachedModifier__) {
this.__cachedModifier__ = new ServiceReference.Modifier();
}
return ((ServiceReference.Modifier) this.__cachedModifier__);
}
/**
* Copies all state of this object to a builder. This method is used by the copyOf
* method and should not be called directly by client code.
*
* @param _other
* A builder instance to which the state of this object will be copied.
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public<_B >void copyTo(final ServiceReference.Builder<_B> _other) {
_other.value = this.value;
_other.ivoId = this.ivoId;
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public<_B >ServiceReference.Builder<_B> newCopyBuilder(final _B _parentBuilder) {
return new ServiceReference.Builder<_B>(_parentBuilder, this, true);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public ServiceReference.Builder newCopyBuilder() {
return newCopyBuilder(null);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static ServiceReference.Builder builder() {
return new ServiceReference.Builder<>(null, null, false);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static<_B >ServiceReference.Builder<_B> copyOf(final ServiceReference _other) {
final ServiceReference.Builder<_B> _newBuilder = new ServiceReference.Builder<>(null, null, false);
_other.copyTo(_newBuilder);
return _newBuilder;
}
/**
* Copies all state of this object to a builder. This method is used by the copyOf
* method and should not be called directly by client code.
*
* @param _other
* A builder instance to which the state of this object will be copied.
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public<_B >void copyTo(final ServiceReference.Builder<_B> _other, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
final PropertyTree valuePropertyTree = ((_propertyTree == null)?null:_propertyTree.get("value"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(valuePropertyTree!= null):((valuePropertyTree == null)||(!valuePropertyTree.isLeaf())))) {
_other.value = this.value;
}
final PropertyTree ivoIdPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("ivoId"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(ivoIdPropertyTree!= null):((ivoIdPropertyTree == null)||(!ivoIdPropertyTree.isLeaf())))) {
_other.ivoId = this.ivoId;
}
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public<_B >ServiceReference.Builder<_B> newCopyBuilder(final _B _parentBuilder, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
return new ServiceReference.Builder<_B>(_parentBuilder, this, true, _propertyTree, _propertyTreeUse);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public ServiceReference.Builder newCopyBuilder(final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
return newCopyBuilder(null, _propertyTree, _propertyTreeUse);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static<_B >ServiceReference.Builder<_B> copyOf(final ServiceReference _other, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
final ServiceReference.Builder<_B> _newBuilder = new ServiceReference.Builder<>(null, null, false);
_other.copyTo(_newBuilder, _propertyTree, _propertyTreeUse);
return _newBuilder;
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static ServiceReference.Builder copyExcept(final ServiceReference _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.EXCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static ServiceReference.Builder copyOnly(final ServiceReference _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.INCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public ServiceReference visit(final PropertyVisitor _visitor_) {
_visitor_.visit(this);
_visitor_.visit(new SingleProperty<>(ServiceReference.PropInfo.VALUE, this));
_visitor_.visit(new SingleProperty<>(ServiceReference.PropInfo.IVO_ID, this));
return this;
}
public static class Builder<_B >implements Buildable
{
protected final _B _parentBuilder;
protected final ServiceReference _storedValue;
private String value;
private String ivoId;
public Builder(final _B _parentBuilder, final ServiceReference _other, final boolean _copy) {
this._parentBuilder = _parentBuilder;
if (_other!= null) {
if (_copy) {
_storedValue = null;
this.value = _other.value;
this.ivoId = _other.ivoId;
} else {
_storedValue = _other;
}
} else {
_storedValue = null;
}
}
public Builder(final _B _parentBuilder, final ServiceReference _other, final boolean _copy, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
this._parentBuilder = _parentBuilder;
if (_other!= null) {
if (_copy) {
_storedValue = null;
final PropertyTree valuePropertyTree = ((_propertyTree == null)?null:_propertyTree.get("value"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(valuePropertyTree!= null):((valuePropertyTree == null)||(!valuePropertyTree.isLeaf())))) {
this.value = _other.value;
}
final PropertyTree ivoIdPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("ivoId"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(ivoIdPropertyTree!= null):((ivoIdPropertyTree == null)||(!ivoIdPropertyTree.isLeaf())))) {
this.ivoId = _other.ivoId;
}
} else {
_storedValue = _other;
}
} else {
_storedValue = null;
}
}
public _B end() {
return this._parentBuilder;
}
protected<_P extends ServiceReference >_P init(final _P _product) {
_product.value = this.value;
_product.ivoId = this.ivoId;
return _product;
}
/**
* Sets the new value of "value" (any previous value will be replaced)
*
* @param value
* New value of the "value" property.
*/
public ServiceReference.Builder<_B> withValue(final String value) {
this.value = value;
return this;
}
/**
* Sets the new value of "ivoId" (any previous value will be replaced)
*
* @param ivoId
* New value of the "ivoId" property.
*/
public ServiceReference.Builder<_B> withIvoId(final String ivoId) {
this.ivoId = ivoId;
return this;
}
@Override
public ServiceReference build() {
if (_storedValue == null) {
return this.init(new ServiceReference());
} else {
return ((ServiceReference) _storedValue);
}
}
public ServiceReference.Builder<_B> copyOf(final ServiceReference _other) {
_other.copyTo(this);
return this;
}
public ServiceReference.Builder<_B> copyOf(final ServiceReference.Builder _other) {
return copyOf(_other.build());
}
}
public class Modifier {
public void setValue(final String value) {
ServiceReference.this.setValue(value);
}
public void setIvoId(final String ivoId) {
ServiceReference.this.setIvoId(ivoId);
}
}
public static class PropInfo {
public static final transient SinglePropertyInfo VALUE = new SinglePropertyInfo("value", ServiceReference.class, String.class, false, null, new QName("http://www.w3.org/2001/XMLSchema", "anyURI"), new QName("http://www.w3.org/2001/XMLSchema", "anyURI"), false) {
@Override
public String get(final ServiceReference _instance_) {
return ((_instance_ == null)?null:_instance_.value);
}
@Override
public void set(final ServiceReference _instance_, final String _value_) {
if (_instance_!= null) {
_instance_.value = _value_;
}
}
}
;
public static final transient SinglePropertyInfo IVO_ID = new SinglePropertyInfo("ivoId", ServiceReference.class, String.class, false, null, new QName("", "ivo-id"), new QName("http://www.ivoa.net/xml/VOResource/v1.0", "IdentifierURI"), true) {
@Override
public String get(final ServiceReference _instance_) {
return ((_instance_ == null)?null:_instance_.ivoId);
}
@Override
public void set(final ServiceReference _instance_, final String _value_) {
if (_instance_!= null) {
_instance_.ivoId = _value_;
}
}
}
;
}
public static class Select
extends ServiceReference.Selector
{
Select() {
super(null, null, null);
}
public static ServiceReference.Select _root() {
return new ServiceReference.Select();
}
}
public static class Selector , TParent >
extends com.kscs.util.jaxb.Selector
{
private com.kscs.util.jaxb.Selector> value = null;
private com.kscs.util.jaxb.Selector> ivoId = null;
public Selector(final TRoot root, final TParent parent, final String propertyName) {
super(root, parent, propertyName);
}
@Override
public Map buildChildren() {
final Map products = new HashMap<>();
products.putAll(super.buildChildren());
if (this.value!= null) {
products.put("value", this.value.init());
}
if (this.ivoId!= null) {
products.put("ivoId", this.ivoId.init());
}
return products;
}
public com.kscs.util.jaxb.Selector> value() {
return ((this.value == null)?this.value = new com.kscs.util.jaxb.Selector<>(this._root, this, "value"):this.value);
}
public com.kscs.util.jaxb.Selector> ivoId() {
return ((this.ivoId == null)?this.ivoId = new com.kscs.util.jaxb.Selector<>(this._root, this, "ivoId"):this.ivoId);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy