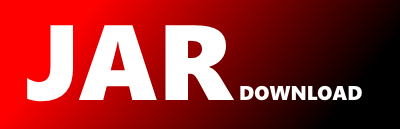
org.javastro.ivoa.entities.resource.dataservice.TableParam Maven / Gradle / Ivy
package org.javastro.ivoa.entities.resource.dataservice;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import javax.xml.namespace.QName;
import com.kscs.util.jaxb.Buildable;
import com.kscs.util.jaxb.CollectionProperty;
import com.kscs.util.jaxb.CollectionPropertyInfo;
import com.kscs.util.jaxb.CollectionPropertyInfo;
import com.kscs.util.jaxb.Copyable;
import com.kscs.util.jaxb.PartialCopyable;
import com.kscs.util.jaxb.PropertyTree;
import com.kscs.util.jaxb.PropertyTreeUse;
import com.kscs.util.jaxb.PropertyVisitor;
import com.kscs.util.jaxb.SingleProperty;
import com.kscs.util.jaxb.SinglePropertyInfo;
import com.kscs.util.jaxb.SinglePropertyInfo;
import com.kscs.util.jaxb.SinglePropertyInfo;
import jakarta.annotation.Generated;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlAttribute;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlSchemaType;
import jakarta.xml.bind.annotation.XmlType;
import jakarta.xml.bind.annotation.adapters.CollapsedStringAdapter;
import jakarta.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import org.jvnet.jaxb.lang.JAXBMergeStrategy;
import org.jvnet.jaxb.lang.JAXBToStringStrategy;
import org.jvnet.jaxb.lang.MergeFrom;
import org.jvnet.jaxb.lang.MergeStrategy;
import org.jvnet.jaxb.lang.ToString;
import org.jvnet.jaxb.lang.ToStringStrategy;
import org.jvnet.jaxb.locator.ObjectLocator;
import org.jvnet.jaxb.locator.util.LocatorUtils;
/**
* A description of a table parameter having a fixed data type.
*
* Java class for TableParam complex type
.
*
* The following schema fragment specifies the expected content contained within this class.
*
* {@code
*
*
*
*
*
*
*
*
*
*
*
*
* }
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "TableParam", propOrder = {
"dataType",
"flags"
})
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public class TableParam
extends BaseParam
implements Cloneable, Copyable, PartialCopyable, MergeFrom, ToString
{
/**
* A type of data contained in the column
*
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
protected TableDataType dataType;
/**
* See the specification document for definitions
* of recognized keywords.
*
*/
@XmlElement(name = "flag")
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
@XmlSchemaType(name = "token")
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
protected List flags;
/**
* If true, the meaning and use of this parameter is
* reserved and defined by a standard model. If false,
* it represents a parameter specific to the data described
* If not provided, then the value is unknown.
*
*/
@XmlAttribute(name = "std")
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
protected Boolean std;
/**
* Default no-arg constructor
*
*/
public TableParam() {
super();
}
/**
* Fully-initialising value constructor
*
*/
public TableParam(final String name, final String description, final String unit, final String ucd, final String utype, final Map otherAttributes, final TableDataType dataType, final List flags, final Boolean std) {
super(name, description, unit, ucd, utype, otherAttributes);
this.dataType = dataType;
this.flags = flags;
this.std = std;
}
/**
* A type of data contained in the column
*
* @return
* possible object is
* {@link TableDataType }
*
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public TableDataType getDataType() {
return dataType;
}
/**
* Sets the value of the dataType property.
*
* @param value
* allowed object is
* {@link TableDataType }
*
* @see #getDataType()
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void setDataType(TableDataType value) {
this.dataType = value;
}
/**
* See the specification document for definitions
* of recognized keywords.
*
* Gets the value of the flags property.
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the flags property.
*
*
* For example, to add a new item, do as follows:
*
*
* getFlags().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link String }
*
*
*
* @return
* The value of the flags property.
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public List getFlags() {
if (flags == null) {
flags = new ArrayList<>();
}
return this.flags;
}
/**
* If true, the meaning and use of this parameter is
* reserved and defined by a standard model. If false,
* it represents a parameter specific to the data described
* If not provided, then the value is unknown.
*
* @return
* possible object is
* {@link Boolean }
*
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Boolean isStd() {
return std;
}
/**
* Sets the value of the std property.
*
* @param value
* allowed object is
* {@link Boolean }
*
* @see #isStd()
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void setStd(Boolean value) {
this.std = value;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public boolean equals(Object object) {
if ((object == null)||(this.getClass()!= object.getClass())) {
return false;
}
if (this == object) {
return true;
}
if (!super.equals(object)) {
return false;
}
final TableParam that = ((TableParam) object);
{
TableDataType leftDataType;
leftDataType = this.getDataType();
TableDataType rightDataType;
rightDataType = that.getDataType();
if (this.dataType!= null) {
if (that.dataType!= null) {
if (!leftDataType.equals(rightDataType)) {
return false;
}
} else {
return false;
}
} else {
if (that.dataType!= null) {
return false;
}
}
}
{
List leftFlags;
leftFlags = (((this.flags!= null)&&(!this.flags.isEmpty()))?this.getFlags():null);
List rightFlags;
rightFlags = (((that.flags!= null)&&(!that.flags.isEmpty()))?that.getFlags():null);
if ((this.flags!= null)&&(!this.flags.isEmpty())) {
if ((that.flags!= null)&&(!that.flags.isEmpty())) {
if (!leftFlags.equals(rightFlags)) {
return false;
}
} else {
return false;
}
} else {
if ((that.flags!= null)&&(!that.flags.isEmpty())) {
return false;
}
}
}
{
Boolean leftStd;
leftStd = this.isStd();
Boolean rightStd;
rightStd = that.isStd();
if (this.std!= null) {
if (that.std!= null) {
if (!leftStd.equals(rightStd)) {
return false;
}
} else {
return false;
}
} else {
if (that.std!= null) {
return false;
}
}
}
return true;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public int hashCode() {
int currentHashCode = 1;
currentHashCode = ((currentHashCode* 31)+ super.hashCode());
{
currentHashCode = (currentHashCode* 31);
TableDataType theDataType;
theDataType = this.getDataType();
if (this.dataType!= null) {
currentHashCode += theDataType.hashCode();
}
}
{
currentHashCode = (currentHashCode* 31);
List theFlags;
theFlags = (((this.flags!= null)&&(!this.flags.isEmpty()))?this.getFlags():null);
if ((this.flags!= null)&&(!this.flags.isEmpty())) {
currentHashCode += theFlags.hashCode();
}
}
{
currentHashCode = (currentHashCode* 31);
Boolean theStd;
theStd = this.isStd();
if (this.std!= null) {
currentHashCode += theStd.hashCode();
}
}
return currentHashCode;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public String toString() {
final ToStringStrategy strategy = JAXBToStringStrategy.getInstance();
final StringBuilder buffer = new StringBuilder();
append(null, buffer, strategy);
return buffer.toString();
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public StringBuilder append(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
strategy.appendStart(locator, this, buffer);
appendFields(locator, buffer, strategy);
strategy.appendEnd(locator, this, buffer);
return buffer;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public StringBuilder appendFields(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
super.appendFields(locator, buffer, strategy);
{
TableDataType theDataType;
theDataType = this.getDataType();
strategy.appendField(locator, this, "dataType", buffer, theDataType, (this.dataType!= null));
}
{
List theFlags;
theFlags = (((this.flags!= null)&&(!this.flags.isEmpty()))?this.getFlags():null);
strategy.appendField(locator, this, "flags", buffer, theFlags, ((this.flags!= null)&&(!this.flags.isEmpty())));
}
{
Boolean theStd;
theStd = this.isStd();
strategy.appendField(locator, this, "std", buffer, theStd, (this.std!= null));
}
return buffer;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void mergeFrom(Object left, Object right) {
final MergeStrategy strategy = JAXBMergeStrategy.getInstance();
mergeFrom(null, null, left, right, strategy);
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void mergeFrom(ObjectLocator leftLocator, ObjectLocator rightLocator, Object left, Object right, MergeStrategy strategy) {
super.mergeFrom(leftLocator, rightLocator, left, right, strategy);
if (right instanceof TableParam) {
final TableParam target = this;
final TableParam leftObject = ((TableParam) left);
final TableParam rightObject = ((TableParam) right);
{
Boolean dataTypeShouldBeMergedAndSet = strategy.shouldBeMergedAndSet(leftLocator, rightLocator, (leftObject.dataType!= null), (rightObject.dataType!= null));
if (dataTypeShouldBeMergedAndSet == Boolean.TRUE) {
TableDataType lhsDataType;
lhsDataType = leftObject.getDataType();
TableDataType rhsDataType;
rhsDataType = rightObject.getDataType();
TableDataType mergedDataType = ((TableDataType) strategy.merge(LocatorUtils.property(leftLocator, "dataType", lhsDataType), LocatorUtils.property(rightLocator, "dataType", rhsDataType), lhsDataType, rhsDataType, (leftObject.dataType!= null), (rightObject.dataType!= null)));
target.setDataType(mergedDataType);
} else {
if (dataTypeShouldBeMergedAndSet == Boolean.FALSE) {
target.dataType = null;
}
}
}
{
Boolean flagsShouldBeMergedAndSet = strategy.shouldBeMergedAndSet(leftLocator, rightLocator, ((leftObject.flags!= null)&&(!leftObject.flags.isEmpty())), ((rightObject.flags!= null)&&(!rightObject.flags.isEmpty())));
if (flagsShouldBeMergedAndSet == Boolean.TRUE) {
List lhsFlags;
lhsFlags = (((leftObject.flags!= null)&&(!leftObject.flags.isEmpty()))?leftObject.getFlags():null);
List rhsFlags;
rhsFlags = (((rightObject.flags!= null)&&(!rightObject.flags.isEmpty()))?rightObject.getFlags():null);
List mergedFlags = ((List ) strategy.merge(LocatorUtils.property(leftLocator, "flags", lhsFlags), LocatorUtils.property(rightLocator, "flags", rhsFlags), lhsFlags, rhsFlags, ((leftObject.flags!= null)&&(!leftObject.flags.isEmpty())), ((rightObject.flags!= null)&&(!rightObject.flags.isEmpty()))));
target.flags = null;
if (mergedFlags!= null) {
List uniqueFlagsl = target.getFlags();
uniqueFlagsl.addAll(mergedFlags);
}
} else {
if (flagsShouldBeMergedAndSet == Boolean.FALSE) {
target.flags = null;
}
}
}
{
Boolean stdShouldBeMergedAndSet = strategy.shouldBeMergedAndSet(leftLocator, rightLocator, (leftObject.std!= null), (rightObject.std!= null));
if (stdShouldBeMergedAndSet == Boolean.TRUE) {
Boolean lhsStd;
lhsStd = leftObject.isStd();
Boolean rhsStd;
rhsStd = rightObject.isStd();
Boolean mergedStd = ((Boolean) strategy.merge(LocatorUtils.property(leftLocator, "std", lhsStd), LocatorUtils.property(rightLocator, "std", rhsStd), lhsStd, rhsStd, (leftObject.std!= null), (rightObject.std!= null)));
target.setStd(mergedStd);
} else {
if (stdShouldBeMergedAndSet == Boolean.FALSE) {
target.std = null;
}
}
}
}
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Object createNewInstance() {
return new TableParam();
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public TableParam clone() {
final TableParam _newObject;
_newObject = ((TableParam) super.clone());
_newObject.dataType = ((this.dataType == null)?null:this.dataType.clone());
_newObject.flags = ((this.flags == null)?null:new ArrayList<>(this.flags));
return _newObject;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public TableParam createCopy() {
final TableParam _newObject = ((TableParam) super.createCopy());
_newObject.dataType = ((this.dataType == null)?null:this.dataType.createCopy());
_newObject.flags = ((this.flags == null)?null:new ArrayList<>(this.flags));
_newObject.std = this.std;
return _newObject;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public TableParam createCopy(final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
final TableParam _newObject = ((TableParam) super.createCopy(_propertyTree, _propertyTreeUse));
final PropertyTree dataTypePropertyTree = ((_propertyTree == null)?null:_propertyTree.get("dataType"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(dataTypePropertyTree!= null):((dataTypePropertyTree == null)||(!dataTypePropertyTree.isLeaf())))) {
_newObject.dataType = ((this.dataType == null)?null:this.dataType.createCopy(dataTypePropertyTree, _propertyTreeUse));
}
final PropertyTree flagsPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("flags"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(flagsPropertyTree!= null):((flagsPropertyTree == null)||(!flagsPropertyTree.isLeaf())))) {
_newObject.flags = ((this.flags == null)?null:new ArrayList<>(this.flags));
}
final PropertyTree stdPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("std"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(stdPropertyTree!= null):((stdPropertyTree == null)||(!stdPropertyTree.isLeaf())))) {
_newObject.std = this.std;
}
return _newObject;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public TableParam copyExcept(final PropertyTree _propertyTree) {
return createCopy(_propertyTree, PropertyTreeUse.EXCLUDE);
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public TableParam copyOnly(final PropertyTree _propertyTree) {
return createCopy(_propertyTree, PropertyTreeUse.INCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public TableParam.Modifier modifier() {
if (null == this.__cachedModifier__) {
this.__cachedModifier__ = new TableParam.Modifier();
}
return ((TableParam.Modifier) this.__cachedModifier__);
}
/**
* Copies all state of this object to a builder. This method is used by the copyOf
* method and should not be called directly by client code.
*
* @param _other
* A builder instance to which the state of this object will be copied.
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public<_B >void copyTo(final TableParam.Builder<_B> _other) {
super.copyTo(_other);
_other.dataType = ((this.dataType == null)?null:this.dataType.newCopyBuilder(_other));
if (this.flags == null) {
_other.flags = null;
} else {
_other.flags = new ArrayList<>();
for (String _item: this.flags) {
_other.flags.add(((_item == null)?null:new Buildable.PrimitiveBuildable(_item)));
}
}
_other.std = this.std;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public<_B >TableParam.Builder<_B> newCopyBuilder(final _B _parentBuilder) {
return new TableParam.Builder<_B>(_parentBuilder, this, true);
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public TableParam.Builder newCopyBuilder() {
return newCopyBuilder(null);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static TableParam.Builder builder() {
return new TableParam.Builder<>(null, null, false);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static<_B >TableParam.Builder<_B> copyOf(final BaseParam _other) {
final TableParam.Builder<_B> _newBuilder = new TableParam.Builder<>(null, null, false);
_other.copyTo(_newBuilder);
return _newBuilder;
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static<_B >TableParam.Builder<_B> copyOf(final TableParam _other) {
final TableParam.Builder<_B> _newBuilder = new TableParam.Builder<>(null, null, false);
_other.copyTo(_newBuilder);
return _newBuilder;
}
/**
* Copies all state of this object to a builder. This method is used by the copyOf
* method and should not be called directly by client code.
*
* @param _other
* A builder instance to which the state of this object will be copied.
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public<_B >void copyTo(final TableParam.Builder<_B> _other, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
super.copyTo(_other, _propertyTree, _propertyTreeUse);
final PropertyTree dataTypePropertyTree = ((_propertyTree == null)?null:_propertyTree.get("dataType"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(dataTypePropertyTree!= null):((dataTypePropertyTree == null)||(!dataTypePropertyTree.isLeaf())))) {
_other.dataType = ((this.dataType == null)?null:this.dataType.newCopyBuilder(_other, dataTypePropertyTree, _propertyTreeUse));
}
final PropertyTree flagsPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("flags"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(flagsPropertyTree!= null):((flagsPropertyTree == null)||(!flagsPropertyTree.isLeaf())))) {
if (this.flags == null) {
_other.flags = null;
} else {
_other.flags = new ArrayList<>();
for (String _item: this.flags) {
_other.flags.add(((_item == null)?null:new Buildable.PrimitiveBuildable(_item)));
}
}
}
final PropertyTree stdPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("std"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(stdPropertyTree!= null):((stdPropertyTree == null)||(!stdPropertyTree.isLeaf())))) {
_other.std = this.std;
}
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public<_B >TableParam.Builder<_B> newCopyBuilder(final _B _parentBuilder, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
return new TableParam.Builder<_B>(_parentBuilder, this, true, _propertyTree, _propertyTreeUse);
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public TableParam.Builder newCopyBuilder(final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
return newCopyBuilder(null, _propertyTree, _propertyTreeUse);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static<_B >TableParam.Builder<_B> copyOf(final BaseParam _other, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
final TableParam.Builder<_B> _newBuilder = new TableParam.Builder<>(null, null, false);
_other.copyTo(_newBuilder, _propertyTree, _propertyTreeUse);
return _newBuilder;
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static<_B >TableParam.Builder<_B> copyOf(final TableParam _other, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
final TableParam.Builder<_B> _newBuilder = new TableParam.Builder<>(null, null, false);
_other.copyTo(_newBuilder, _propertyTree, _propertyTreeUse);
return _newBuilder;
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static TableParam.Builder copyExcept(final BaseParam _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.EXCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static TableParam.Builder copyExcept(final TableParam _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.EXCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static TableParam.Builder copyOnly(final BaseParam _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.INCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static TableParam.Builder copyOnly(final TableParam _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.INCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public TableParam visit(final PropertyVisitor _visitor_) {
super.visit(_visitor_);
if (_visitor_.visit(new SingleProperty<>(TableParam.PropInfo.DATA_TYPE, this))&&(this.dataType!= null)) {
this.dataType.visit(_visitor_);
}
_visitor_.visit(new CollectionProperty<>(TableParam.PropInfo.FLAGS, this));
_visitor_.visit(new SingleProperty<>(TableParam.PropInfo.STD, this));
return this;
}
public static class Builder<_B >
extends BaseParam.Builder<_B>
implements Buildable
{
private TableDataType.Builder> dataType;
private List flags;
private Boolean std;
public Builder(final _B _parentBuilder, final TableParam _other, final boolean _copy) {
super(_parentBuilder, _other, _copy);
if (_other!= null) {
this.dataType = ((_other.dataType == null)?null:_other.dataType.newCopyBuilder(this));
if (_other.flags == null) {
this.flags = null;
} else {
this.flags = new ArrayList<>();
for (String _item: _other.flags) {
this.flags.add(((_item == null)?null:new Buildable.PrimitiveBuildable(_item)));
}
}
this.std = _other.std;
}
}
public Builder(final _B _parentBuilder, final TableParam _other, final boolean _copy, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
super(_parentBuilder, _other, _copy, _propertyTree, _propertyTreeUse);
if (_other!= null) {
final PropertyTree dataTypePropertyTree = ((_propertyTree == null)?null:_propertyTree.get("dataType"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(dataTypePropertyTree!= null):((dataTypePropertyTree == null)||(!dataTypePropertyTree.isLeaf())))) {
this.dataType = ((_other.dataType == null)?null:_other.dataType.newCopyBuilder(this, dataTypePropertyTree, _propertyTreeUse));
}
final PropertyTree flagsPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("flags"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(flagsPropertyTree!= null):((flagsPropertyTree == null)||(!flagsPropertyTree.isLeaf())))) {
if (_other.flags == null) {
this.flags = null;
} else {
this.flags = new ArrayList<>();
for (String _item: _other.flags) {
this.flags.add(((_item == null)?null:new Buildable.PrimitiveBuildable(_item)));
}
}
}
final PropertyTree stdPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("std"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(stdPropertyTree!= null):((stdPropertyTree == null)||(!stdPropertyTree.isLeaf())))) {
this.std = _other.std;
}
}
}
protected<_P extends TableParam >_P init(final _P _product) {
_product.dataType = ((this.dataType == null)?null:this.dataType.build());
if (this.flags!= null) {
final List flags = new ArrayList<>(this.flags.size());
for (Buildable _item: this.flags) {
flags.add(((String) _item.build()));
}
_product.flags = flags;
}
_product.std = this.std;
return super.init(_product);
}
/**
* Sets the new value of "dataType" (any previous value will be replaced)
*
* @param dataType
* New value of the "dataType" property.
*/
public TableParam.Builder<_B> withDataType(final TableDataType dataType) {
this.dataType = ((dataType == null)?null:new TableDataType.Builder<>(this, dataType, false));
return this;
}
/**
* Adds the given items to the value of "flags"
*
* @param flags
* Items to add to the value of the "flags" property
*/
public TableParam.Builder<_B> addFlags(final Iterable extends String> flags) {
if (flags!= null) {
if (this.flags == null) {
this.flags = new ArrayList<>();
}
for (String _item: flags) {
this.flags.add(new Buildable.PrimitiveBuildable(_item));
}
}
return this;
}
/**
* Sets the new value of "flags" (any previous value will be replaced)
*
* @param flags
* New value of the "flags" property.
*/
public TableParam.Builder<_B> withFlags(final Iterable extends String> flags) {
if (this.flags!= null) {
this.flags.clear();
}
return addFlags(flags);
}
/**
* Adds the given items to the value of "flags"
*
* @param flags
* Items to add to the value of the "flags" property
*/
public TableParam.Builder<_B> addFlags(String... flags) {
addFlags(Arrays.asList(flags));
return this;
}
/**
* Sets the new value of "flags" (any previous value will be replaced)
*
* @param flags
* New value of the "flags" property.
*/
public TableParam.Builder<_B> withFlags(String... flags) {
withFlags(Arrays.asList(flags));
return this;
}
/**
* Sets the new value of "std" (any previous value will be replaced)
*
* @param std
* New value of the "std" property.
*/
public TableParam.Builder<_B> withStd(final Boolean std) {
this.std = std;
return this;
}
/**
* Sets the new value of "name" (any previous value will be replaced)
*
* @param name
* New value of the "name" property.
*/
@Override
public TableParam.Builder<_B> withName(final String name) {
super.withName(name);
return this;
}
/**
* Sets the new value of "description" (any previous value will be replaced)
*
* @param description
* New value of the "description" property.
*/
@Override
public TableParam.Builder<_B> withDescription(final String description) {
super.withDescription(description);
return this;
}
/**
* Sets the new value of "unit" (any previous value will be replaced)
*
* @param unit
* New value of the "unit" property.
*/
@Override
public TableParam.Builder<_B> withUnit(final String unit) {
super.withUnit(unit);
return this;
}
/**
* Sets the new value of "ucd" (any previous value will be replaced)
*
* @param ucd
* New value of the "ucd" property.
*/
@Override
public TableParam.Builder<_B> withUcd(final String ucd) {
super.withUcd(ucd);
return this;
}
/**
* Sets the new value of "utype" (any previous value will be replaced)
*
* @param utype
* New value of the "utype" property.
*/
@Override
public TableParam.Builder<_B> withUtype(final String utype) {
super.withUtype(utype);
return this;
}
@Override
public TableParam build() {
if (_storedValue == null) {
return this.init(new TableParam());
} else {
return ((TableParam) _storedValue);
}
}
public TableParam.Builder<_B> copyOf(final TableParam _other) {
_other.copyTo(this);
return this;
}
public TableParam.Builder<_B> copyOf(final TableParam.Builder _other) {
return copyOf(_other.build());
}
}
public class Modifier
extends BaseParam.Modifier
{
public void setDataType(final TableDataType dataType) {
TableParam.this.setDataType(dataType);
}
public List getFlags() {
if (TableParam.this.flags == null) {
TableParam.this.flags = new ArrayList<>();
}
return TableParam.this.flags;
}
public void setStd(final Boolean std) {
TableParam.this.setStd(std);
}
}
public static class PropInfo {
public static final transient SinglePropertyInfo DATA_TYPE = new SinglePropertyInfo("dataType", TableParam.class, TableDataType.class, false, null, new QName("", "dataType"), new QName("http://www.ivoa.net/xml/VODataService/v1.1", "TableDataType"), false) {
@Override
public TableDataType get(final TableParam _instance_) {
return ((_instance_ == null)?null:_instance_.dataType);
}
@Override
public void set(final TableParam _instance_, final TableDataType _value_) {
if (_instance_!= null) {
_instance_.dataType = _value_;
}
}
}
;
public static final transient CollectionPropertyInfo FLAGS = new CollectionPropertyInfo("flags", TableParam.class, String.class, true, null, new QName("", "flag"), new QName("http://www.w3.org/2001/XMLSchema", "token"), false) {
@Override
public List get(final TableParam _instance_) {
return ((_instance_ == null)?null:_instance_.flags);
}
@Override
public void set(final TableParam _instance_, final List _value_) {
if (_instance_!= null) {
_instance_.flags = _value_;
}
}
}
;
public static final transient SinglePropertyInfo STD = new SinglePropertyInfo("std", TableParam.class, Boolean.class, false, null, new QName("", "std"), new QName("http://www.w3.org/2001/XMLSchema", "boolean"), true) {
@Override
public Boolean get(final TableParam _instance_) {
return ((_instance_ == null)?null:_instance_.std);
}
@Override
public void set(final TableParam _instance_, final Boolean _value_) {
if (_instance_!= null) {
_instance_.std = _value_;
}
}
}
;
}
public static class Select
extends TableParam.Selector
{
Select() {
super(null, null, null);
}
public static TableParam.Select _root() {
return new TableParam.Select();
}
}
public static class Selector , TParent >
extends BaseParam.Selector
{
private TableDataType.Selector> dataType = null;
private com.kscs.util.jaxb.Selector> flags = null;
private com.kscs.util.jaxb.Selector> std = null;
public Selector(final TRoot root, final TParent parent, final String propertyName) {
super(root, parent, propertyName);
}
@Override
public Map buildChildren() {
final Map products = new HashMap<>();
products.putAll(super.buildChildren());
if (this.dataType!= null) {
products.put("dataType", this.dataType.init());
}
if (this.flags!= null) {
products.put("flags", this.flags.init());
}
if (this.std!= null) {
products.put("std", this.std.init());
}
return products;
}
public TableDataType.Selector> dataType() {
return ((this.dataType == null)?this.dataType = new TableDataType.Selector<>(this._root, this, "dataType"):this.dataType);
}
public com.kscs.util.jaxb.Selector> flags() {
return ((this.flags == null)?this.flags = new com.kscs.util.jaxb.Selector<>(this._root, this, "flags"):this.flags);
}
public com.kscs.util.jaxb.Selector> std() {
return ((this.std == null)?this.std = new com.kscs.util.jaxb.Selector<>(this._root, this, "std"):this.std);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy