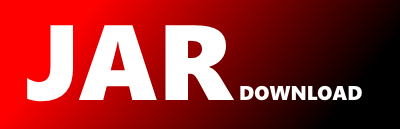
org.javastro.ivoa.entities.resource.standard.StandardKeyEnumeration Maven / Gradle / Ivy
package org.javastro.ivoa.entities.resource.standard;
import java.time.LocalDateTime;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import javax.xml.namespace.QName;
import com.kscs.util.jaxb.Buildable;
import com.kscs.util.jaxb.CollectionProperty;
import com.kscs.util.jaxb.CollectionPropertyInfo;
import com.kscs.util.jaxb.CollectionPropertyInfo;
import com.kscs.util.jaxb.Copyable;
import com.kscs.util.jaxb.PartialCopyable;
import com.kscs.util.jaxb.PropertyTree;
import com.kscs.util.jaxb.PropertyTreeUse;
import com.kscs.util.jaxb.PropertyVisitor;
import jakarta.annotation.Generated;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlType;
import org.javastro.ivoa.entities.resource.Content;
import org.javastro.ivoa.entities.resource.Curation;
import org.javastro.ivoa.entities.resource.Resource;
import org.javastro.ivoa.entities.resource.Validation;
import org.jvnet.jaxb.lang.JAXBMergeStrategy;
import org.jvnet.jaxb.lang.JAXBToStringStrategy;
import org.jvnet.jaxb.lang.MergeFrom;
import org.jvnet.jaxb.lang.MergeStrategy;
import org.jvnet.jaxb.lang.ToString;
import org.jvnet.jaxb.lang.ToStringStrategy;
import org.jvnet.jaxb.locator.ObjectLocator;
import org.jvnet.jaxb.locator.util.LocatorUtils;
/**
* A registered set of related keys. Each key can be
* uniquely identified by combining the IVOA identifier of
* this resource with the key name separated by the URI
* fragment delimiter, #, as in: ivoa-identifier#key-name
*
* Java class for StandardKeyEnumeration complex type
.
*
* The following schema fragment specifies the expected content contained within this class.
*
* {@code
*
*
*
*
*
*
*
*
*
* }
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "StandardKeyEnumeration", propOrder = {
"keies"
})
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public class StandardKeyEnumeration
extends Resource
implements Cloneable, Copyable, PartialCopyable, MergeFrom, ToString
{
/**
* the name and definition of a key--a named concept,
* feature, or property.
*
*/
@XmlElement(name = "key", required = true)
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
protected List keies;
/**
* Default no-arg constructor
*
*/
public StandardKeyEnumeration() {
super();
}
/**
* Fully-initialising value constructor
*
*/
public StandardKeyEnumeration(final List validationLevels, final String title, final String shortName, final String identifier, final List altIdentifiers, final Curation curation, final Content content, final LocalDateTime created, final LocalDateTime updated, final String status, final String version, final List keies) {
super(validationLevels, title, shortName, identifier, altIdentifiers, curation, content, created, updated, status, version);
this.keies = keies;
}
/**
* the name and definition of a key--a named concept,
* feature, or property.
*
* Gets the value of the keies property.
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the keies property.
*
*
* For example, to add a new item, do as follows:
*
*
* getKeies().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link StandardKey }
*
*
*
* @return
* The value of the keies property.
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public List getKeies() {
if (keies == null) {
keies = new ArrayList<>();
}
return this.keies;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public boolean equals(Object object) {
if ((object == null)||(this.getClass()!= object.getClass())) {
return false;
}
if (this == object) {
return true;
}
if (!super.equals(object)) {
return false;
}
final StandardKeyEnumeration that = ((StandardKeyEnumeration) object);
{
List leftKeies;
leftKeies = (((this.keies!= null)&&(!this.keies.isEmpty()))?this.getKeies():null);
List rightKeies;
rightKeies = (((that.keies!= null)&&(!that.keies.isEmpty()))?that.getKeies():null);
if ((this.keies!= null)&&(!this.keies.isEmpty())) {
if ((that.keies!= null)&&(!that.keies.isEmpty())) {
if (!leftKeies.equals(rightKeies)) {
return false;
}
} else {
return false;
}
} else {
if ((that.keies!= null)&&(!that.keies.isEmpty())) {
return false;
}
}
}
return true;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public int hashCode() {
int currentHashCode = 1;
currentHashCode = ((currentHashCode* 31)+ super.hashCode());
{
currentHashCode = (currentHashCode* 31);
List theKeies;
theKeies = (((this.keies!= null)&&(!this.keies.isEmpty()))?this.getKeies():null);
if ((this.keies!= null)&&(!this.keies.isEmpty())) {
currentHashCode += theKeies.hashCode();
}
}
return currentHashCode;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public String toString() {
final ToStringStrategy strategy = JAXBToStringStrategy.getInstance();
final StringBuilder buffer = new StringBuilder();
append(null, buffer, strategy);
return buffer.toString();
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public StringBuilder append(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
strategy.appendStart(locator, this, buffer);
appendFields(locator, buffer, strategy);
strategy.appendEnd(locator, this, buffer);
return buffer;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public StringBuilder appendFields(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
super.appendFields(locator, buffer, strategy);
{
List theKeies;
theKeies = (((this.keies!= null)&&(!this.keies.isEmpty()))?this.getKeies():null);
strategy.appendField(locator, this, "keies", buffer, theKeies, ((this.keies!= null)&&(!this.keies.isEmpty())));
}
return buffer;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void mergeFrom(Object left, Object right) {
final MergeStrategy strategy = JAXBMergeStrategy.getInstance();
mergeFrom(null, null, left, right, strategy);
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void mergeFrom(ObjectLocator leftLocator, ObjectLocator rightLocator, Object left, Object right, MergeStrategy strategy) {
super.mergeFrom(leftLocator, rightLocator, left, right, strategy);
if (right instanceof StandardKeyEnumeration) {
final StandardKeyEnumeration target = this;
final StandardKeyEnumeration leftObject = ((StandardKeyEnumeration) left);
final StandardKeyEnumeration rightObject = ((StandardKeyEnumeration) right);
{
Boolean keiesShouldBeMergedAndSet = strategy.shouldBeMergedAndSet(leftLocator, rightLocator, ((leftObject.keies!= null)&&(!leftObject.keies.isEmpty())), ((rightObject.keies!= null)&&(!rightObject.keies.isEmpty())));
if (keiesShouldBeMergedAndSet == Boolean.TRUE) {
List lhsKeies;
lhsKeies = (((leftObject.keies!= null)&&(!leftObject.keies.isEmpty()))?leftObject.getKeies():null);
List rhsKeies;
rhsKeies = (((rightObject.keies!= null)&&(!rightObject.keies.isEmpty()))?rightObject.getKeies():null);
List mergedKeies = ((List ) strategy.merge(LocatorUtils.property(leftLocator, "keies", lhsKeies), LocatorUtils.property(rightLocator, "keies", rhsKeies), lhsKeies, rhsKeies, ((leftObject.keies!= null)&&(!leftObject.keies.isEmpty())), ((rightObject.keies!= null)&&(!rightObject.keies.isEmpty()))));
target.keies = null;
if (mergedKeies!= null) {
List uniqueKeiesl = target.getKeies();
uniqueKeiesl.addAll(mergedKeies);
}
} else {
if (keiesShouldBeMergedAndSet == Boolean.FALSE) {
target.keies = null;
}
}
}
}
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Object createNewInstance() {
return new StandardKeyEnumeration();
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public StandardKeyEnumeration clone() {
final StandardKeyEnumeration _newObject;
_newObject = ((StandardKeyEnumeration) super.clone());
if (this.keies == null) {
_newObject.keies = null;
} else {
_newObject.keies = new ArrayList<>();
for (StandardKey _item: this.keies) {
_newObject.keies.add(((_item == null)?null:_item.clone()));
}
}
return _newObject;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public StandardKeyEnumeration createCopy() {
final StandardKeyEnumeration _newObject = ((StandardKeyEnumeration) super.createCopy());
if (this.keies == null) {
_newObject.keies = null;
} else {
_newObject.keies = new ArrayList<>();
for (StandardKey _item: this.keies) {
_newObject.keies.add(((_item == null)?null:_item.createCopy()));
}
}
return _newObject;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public StandardKeyEnumeration createCopy(final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
final StandardKeyEnumeration _newObject = ((StandardKeyEnumeration) super.createCopy(_propertyTree, _propertyTreeUse));
final PropertyTree keiesPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("keies"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(keiesPropertyTree!= null):((keiesPropertyTree == null)||(!keiesPropertyTree.isLeaf())))) {
if (this.keies == null) {
_newObject.keies = null;
} else {
_newObject.keies = new ArrayList<>();
for (StandardKey _item: this.keies) {
_newObject.keies.add(((_item == null)?null:_item.createCopy(keiesPropertyTree, _propertyTreeUse)));
}
}
}
return _newObject;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public StandardKeyEnumeration copyExcept(final PropertyTree _propertyTree) {
return createCopy(_propertyTree, PropertyTreeUse.EXCLUDE);
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public StandardKeyEnumeration copyOnly(final PropertyTree _propertyTree) {
return createCopy(_propertyTree, PropertyTreeUse.INCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public StandardKeyEnumeration.Modifier modifier() {
if (null == this.__cachedModifier__) {
this.__cachedModifier__ = new StandardKeyEnumeration.Modifier();
}
return ((StandardKeyEnumeration.Modifier) this.__cachedModifier__);
}
/**
* Copies all state of this object to a builder. This method is used by the copyOf
* method and should not be called directly by client code.
*
* @param _other
* A builder instance to which the state of this object will be copied.
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public<_B >void copyTo(final StandardKeyEnumeration.Builder<_B> _other) {
super.copyTo(_other);
if (this.keies == null) {
_other.keies = null;
} else {
_other.keies = new ArrayList<>();
for (StandardKey _item: this.keies) {
_other.keies.add(((_item == null)?null:_item.newCopyBuilder(_other)));
}
}
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public<_B >StandardKeyEnumeration.Builder<_B> newCopyBuilder(final _B _parentBuilder) {
return new StandardKeyEnumeration.Builder<_B>(_parentBuilder, this, true);
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public StandardKeyEnumeration.Builder newCopyBuilder() {
return newCopyBuilder(null);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static StandardKeyEnumeration.Builder builder() {
return new StandardKeyEnumeration.Builder<>(null, null, false);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static<_B >StandardKeyEnumeration.Builder<_B> copyOf(final Resource _other) {
final StandardKeyEnumeration.Builder<_B> _newBuilder = new StandardKeyEnumeration.Builder<>(null, null, false);
_other.copyTo(_newBuilder);
return _newBuilder;
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static<_B >StandardKeyEnumeration.Builder<_B> copyOf(final StandardKeyEnumeration _other) {
final StandardKeyEnumeration.Builder<_B> _newBuilder = new StandardKeyEnumeration.Builder<>(null, null, false);
_other.copyTo(_newBuilder);
return _newBuilder;
}
/**
* Copies all state of this object to a builder. This method is used by the copyOf
* method and should not be called directly by client code.
*
* @param _other
* A builder instance to which the state of this object will be copied.
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public<_B >void copyTo(final StandardKeyEnumeration.Builder<_B> _other, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
super.copyTo(_other, _propertyTree, _propertyTreeUse);
final PropertyTree keiesPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("keies"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(keiesPropertyTree!= null):((keiesPropertyTree == null)||(!keiesPropertyTree.isLeaf())))) {
if (this.keies == null) {
_other.keies = null;
} else {
_other.keies = new ArrayList<>();
for (StandardKey _item: this.keies) {
_other.keies.add(((_item == null)?null:_item.newCopyBuilder(_other, keiesPropertyTree, _propertyTreeUse)));
}
}
}
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public<_B >StandardKeyEnumeration.Builder<_B> newCopyBuilder(final _B _parentBuilder, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
return new StandardKeyEnumeration.Builder<_B>(_parentBuilder, this, true, _propertyTree, _propertyTreeUse);
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public StandardKeyEnumeration.Builder newCopyBuilder(final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
return newCopyBuilder(null, _propertyTree, _propertyTreeUse);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static<_B >StandardKeyEnumeration.Builder<_B> copyOf(final Resource _other, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
final StandardKeyEnumeration.Builder<_B> _newBuilder = new StandardKeyEnumeration.Builder<>(null, null, false);
_other.copyTo(_newBuilder, _propertyTree, _propertyTreeUse);
return _newBuilder;
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static<_B >StandardKeyEnumeration.Builder<_B> copyOf(final StandardKeyEnumeration _other, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
final StandardKeyEnumeration.Builder<_B> _newBuilder = new StandardKeyEnumeration.Builder<>(null, null, false);
_other.copyTo(_newBuilder, _propertyTree, _propertyTreeUse);
return _newBuilder;
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static StandardKeyEnumeration.Builder copyExcept(final Resource _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.EXCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static StandardKeyEnumeration.Builder copyExcept(final StandardKeyEnumeration _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.EXCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static StandardKeyEnumeration.Builder copyOnly(final Resource _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.INCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static StandardKeyEnumeration.Builder copyOnly(final StandardKeyEnumeration _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.INCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public StandardKeyEnumeration visit(final PropertyVisitor _visitor_) {
super.visit(_visitor_);
if (_visitor_.visit(new CollectionProperty<>(StandardKeyEnumeration.PropInfo.KEIES, this))&&(this.keies!= null)) {
for (StandardKey _item_: this.keies) {
if (_item_!= null) {
_item_.visit(_visitor_);
}
}
}
return this;
}
public static class Builder<_B >
extends Resource.Builder<_B>
implements Buildable
{
private List>> keies;
public Builder(final _B _parentBuilder, final StandardKeyEnumeration _other, final boolean _copy) {
super(_parentBuilder, _other, _copy);
if (_other!= null) {
if (_other.keies == null) {
this.keies = null;
} else {
this.keies = new ArrayList<>();
for (StandardKey _item: _other.keies) {
this.keies.add(((_item == null)?null:_item.newCopyBuilder(this)));
}
}
}
}
public Builder(final _B _parentBuilder, final StandardKeyEnumeration _other, final boolean _copy, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
super(_parentBuilder, _other, _copy, _propertyTree, _propertyTreeUse);
if (_other!= null) {
final PropertyTree keiesPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("keies"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(keiesPropertyTree!= null):((keiesPropertyTree == null)||(!keiesPropertyTree.isLeaf())))) {
if (_other.keies == null) {
this.keies = null;
} else {
this.keies = new ArrayList<>();
for (StandardKey _item: _other.keies) {
this.keies.add(((_item == null)?null:_item.newCopyBuilder(this, keiesPropertyTree, _propertyTreeUse)));
}
}
}
}
}
protected<_P extends StandardKeyEnumeration >_P init(final _P _product) {
if (this.keies!= null) {
final List keies = new ArrayList<>(this.keies.size());
for (StandardKey.Builder> _item: this.keies) {
keies.add(_item.build());
}
_product.keies = keies;
}
return super.init(_product);
}
/**
* Adds the given items to the value of "keies"
*
* @param keies
* Items to add to the value of the "keies" property
*/
public StandardKeyEnumeration.Builder<_B> addKeies(final Iterable extends StandardKey> keies) {
if (keies!= null) {
if (this.keies == null) {
this.keies = new ArrayList<>();
}
for (StandardKey _item: keies) {
this.keies.add(new StandardKey.Builder<>(this, _item, false));
}
}
return this;
}
/**
* Sets the new value of "keies" (any previous value will be replaced)
*
* @param keies
* New value of the "keies" property.
*/
public StandardKeyEnumeration.Builder<_B> withKeies(final Iterable extends StandardKey> keies) {
if (this.keies!= null) {
this.keies.clear();
}
return addKeies(keies);
}
/**
* Adds the given items to the value of "keies"
*
* @param keies
* Items to add to the value of the "keies" property
*/
public StandardKeyEnumeration.Builder<_B> addKeies(StandardKey... keies) {
addKeies(Arrays.asList(keies));
return this;
}
/**
* Sets the new value of "keies" (any previous value will be replaced)
*
* @param keies
* New value of the "keies" property.
*/
public StandardKeyEnumeration.Builder<_B> withKeies(StandardKey... keies) {
withKeies(Arrays.asList(keies));
return this;
}
/**
* Returns a new builder to build an additional value of the "Keies" property.
* Use {@link
* org.javastro.ivoa.entities.resource.standard.StandardKey.Builder#end()} to
* return to the current builder.
*
* @return
* a new builder to build an additional value of the "Keies" property.
* Use {@link
* org.javastro.ivoa.entities.resource.standard.StandardKey.Builder#end()} to
* return to the current builder.
*/
public StandardKey.Builder extends StandardKeyEnumeration.Builder<_B>> addKeies() {
if (this.keies == null) {
this.keies = new ArrayList<>();
}
final StandardKey.Builder> keies_Builder = new StandardKey.Builder<>(this, null, false);
this.keies.add(keies_Builder);
return keies_Builder;
}
/**
* Adds the given items to the value of "validationLevels"
*
* @param validationLevels
* Items to add to the value of the "validationLevels" property
*/
@Override
public StandardKeyEnumeration.Builder<_B> addValidationLevels(final Iterable extends Validation> validationLevels) {
super.addValidationLevels(validationLevels);
return this;
}
/**
* Adds the given items to the value of "validationLevels"
*
* @param validationLevels
* Items to add to the value of the "validationLevels" property
*/
@Override
public StandardKeyEnumeration.Builder<_B> addValidationLevels(Validation... validationLevels) {
super.addValidationLevels(validationLevels);
return this;
}
/**
* Sets the new value of "validationLevels" (any previous value will be replaced)
*
* @param validationLevels
* New value of the "validationLevels" property.
*/
@Override
public StandardKeyEnumeration.Builder<_B> withValidationLevels(final Iterable extends Validation> validationLevels) {
super.withValidationLevels(validationLevels);
return this;
}
/**
* Sets the new value of "validationLevels" (any previous value will be replaced)
*
* @param validationLevels
* New value of the "validationLevels" property.
*/
@Override
public StandardKeyEnumeration.Builder<_B> withValidationLevels(Validation... validationLevels) {
super.withValidationLevels(validationLevels);
return this;
}
/**
* Sets the new value of "title" (any previous value will be replaced)
*
* @param title
* New value of the "title" property.
*/
@Override
public StandardKeyEnumeration.Builder<_B> withTitle(final String title) {
super.withTitle(title);
return this;
}
/**
* Sets the new value of "shortName" (any previous value will be replaced)
*
* @param shortName
* New value of the "shortName" property.
*/
@Override
public StandardKeyEnumeration.Builder<_B> withShortName(final String shortName) {
super.withShortName(shortName);
return this;
}
/**
* Sets the new value of "identifier" (any previous value will be replaced)
*
* @param identifier
* New value of the "identifier" property.
*/
@Override
public StandardKeyEnumeration.Builder<_B> withIdentifier(final String identifier) {
super.withIdentifier(identifier);
return this;
}
/**
* Adds the given items to the value of "altIdentifiers"
*
* @param altIdentifiers
* Items to add to the value of the "altIdentifiers" property
*/
@Override
public StandardKeyEnumeration.Builder<_B> addAltIdentifiers(final Iterable extends String> altIdentifiers) {
super.addAltIdentifiers(altIdentifiers);
return this;
}
/**
* Adds the given items to the value of "altIdentifiers"
*
* @param altIdentifiers
* Items to add to the value of the "altIdentifiers" property
*/
@Override
public StandardKeyEnumeration.Builder<_B> addAltIdentifiers(String... altIdentifiers) {
super.addAltIdentifiers(altIdentifiers);
return this;
}
/**
* Sets the new value of "altIdentifiers" (any previous value will be replaced)
*
* @param altIdentifiers
* New value of the "altIdentifiers" property.
*/
@Override
public StandardKeyEnumeration.Builder<_B> withAltIdentifiers(final Iterable extends String> altIdentifiers) {
super.withAltIdentifiers(altIdentifiers);
return this;
}
/**
* Sets the new value of "altIdentifiers" (any previous value will be replaced)
*
* @param altIdentifiers
* New value of the "altIdentifiers" property.
*/
@Override
public StandardKeyEnumeration.Builder<_B> withAltIdentifiers(String... altIdentifiers) {
super.withAltIdentifiers(altIdentifiers);
return this;
}
/**
* Sets the new value of "curation" (any previous value will be replaced)
*
* @param curation
* New value of the "curation" property.
*/
@Override
public StandardKeyEnumeration.Builder<_B> withCuration(final Curation curation) {
super.withCuration(curation);
return this;
}
/**
* Returns the existing builder or a new builder to build the value of the
* "curation" property.
* Use {@link org.javastro.ivoa.entities.resource.Curation.Builder#end()} to return
* to the current builder.
*
* @return
* A new builder to build the value of the "curation" property.
* Use {@link org.javastro.ivoa.entities.resource.Curation.Builder#end()} to return
* to the current builder.
*/
public Curation.Builder extends StandardKeyEnumeration.Builder<_B>> withCuration() {
return ((Curation.Builder extends StandardKeyEnumeration.Builder<_B>> ) super.withCuration());
}
/**
* Sets the new value of "content" (any previous value will be replaced)
*
* @param content
* New value of the "content" property.
*/
@Override
public StandardKeyEnumeration.Builder<_B> withContent(final Content content) {
super.withContent(content);
return this;
}
/**
* Returns the existing builder or a new builder to build the value of the
* "content" property.
* Use {@link org.javastro.ivoa.entities.resource.Content.Builder#end()} to return
* to the current builder.
*
* @return
* A new builder to build the value of the "content" property.
* Use {@link org.javastro.ivoa.entities.resource.Content.Builder#end()} to return
* to the current builder.
*/
public Content.Builder extends StandardKeyEnumeration.Builder<_B>> withContent() {
return ((Content.Builder extends StandardKeyEnumeration.Builder<_B>> ) super.withContent());
}
/**
* Sets the new value of "created" (any previous value will be replaced)
*
* @param created
* New value of the "created" property.
*/
@Override
public StandardKeyEnumeration.Builder<_B> withCreated(final LocalDateTime created) {
super.withCreated(created);
return this;
}
/**
* Sets the new value of "updated" (any previous value will be replaced)
*
* @param updated
* New value of the "updated" property.
*/
@Override
public StandardKeyEnumeration.Builder<_B> withUpdated(final LocalDateTime updated) {
super.withUpdated(updated);
return this;
}
/**
* Sets the new value of "status" (any previous value will be replaced)
*
* @param status
* New value of the "status" property.
*/
@Override
public StandardKeyEnumeration.Builder<_B> withStatus(final String status) {
super.withStatus(status);
return this;
}
/**
* Sets the new value of "version" (any previous value will be replaced)
*
* @param version
* New value of the "version" property.
*/
@Override
public StandardKeyEnumeration.Builder<_B> withVersion(final String version) {
super.withVersion(version);
return this;
}
@Override
public StandardKeyEnumeration build() {
if (_storedValue == null) {
return this.init(new StandardKeyEnumeration());
} else {
return ((StandardKeyEnumeration) _storedValue);
}
}
public StandardKeyEnumeration.Builder<_B> copyOf(final StandardKeyEnumeration _other) {
_other.copyTo(this);
return this;
}
public StandardKeyEnumeration.Builder<_B> copyOf(final StandardKeyEnumeration.Builder _other) {
return copyOf(_other.build());
}
}
public class Modifier
extends Resource.Modifier
{
public List getKeies() {
if (StandardKeyEnumeration.this.keies == null) {
StandardKeyEnumeration.this.keies = new ArrayList<>();
}
return StandardKeyEnumeration.this.keies;
}
}
public static class PropInfo {
public static final transient CollectionPropertyInfo KEIES = new CollectionPropertyInfo("keies", StandardKeyEnumeration.class, StandardKey.class, true, null, new QName("", "key"), new QName("http://www.ivoa.net/xml/StandardsRegExt/v1.0", "StandardKey"), false) {
@Override
public List get(final StandardKeyEnumeration _instance_) {
return ((_instance_ == null)?null:_instance_.keies);
}
@Override
public void set(final StandardKeyEnumeration _instance_, final List _value_) {
if (_instance_!= null) {
_instance_.keies = _value_;
}
}
}
;
}
public static class Select
extends StandardKeyEnumeration.Selector
{
Select() {
super(null, null, null);
}
public static StandardKeyEnumeration.Select _root() {
return new StandardKeyEnumeration.Select();
}
}
public static class Selector , TParent >
extends Resource.Selector
{
private StandardKey.Selector> keies = null;
public Selector(final TRoot root, final TParent parent, final String propertyName) {
super(root, parent, propertyName);
}
@Override
public Map buildChildren() {
final Map products = new HashMap<>();
products.putAll(super.buildChildren());
if (this.keies!= null) {
products.put("keies", this.keies.init());
}
return products;
}
public StandardKey.Selector> keies() {
return ((this.keies == null)?this.keies = new StandardKey.Selector<>(this._root, this, "keies"):this.keies);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy