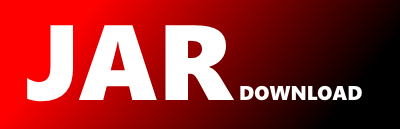
org.javastro.ivoa.entities.uws.ErrorSummary Maven / Gradle / Ivy
package org.javastro.ivoa.entities.uws;
import java.util.HashMap;
import java.util.Map;
import javax.xml.namespace.QName;
import com.kscs.util.jaxb.Buildable;
import com.kscs.util.jaxb.Copyable;
import com.kscs.util.jaxb.PartialCopyable;
import com.kscs.util.jaxb.PropertyTree;
import com.kscs.util.jaxb.PropertyTreeUse;
import com.kscs.util.jaxb.PropertyVisitor;
import com.kscs.util.jaxb.SingleProperty;
import com.kscs.util.jaxb.SinglePropertyInfo;
import com.kscs.util.jaxb.SinglePropertyInfo;
import com.kscs.util.jaxb.SinglePropertyInfo;
import com.kscs.util.jaxb.SinglePropertyInfo;
import jakarta.annotation.Generated;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlAttribute;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlType;
import org.jvnet.jaxb.lang.JAXBMergeStrategy;
import org.jvnet.jaxb.lang.JAXBToStringStrategy;
import org.jvnet.jaxb.lang.MergeFrom;
import org.jvnet.jaxb.lang.MergeStrategy;
import org.jvnet.jaxb.lang.ToString;
import org.jvnet.jaxb.lang.ToStringStrategy;
import org.jvnet.jaxb.locator.ObjectLocator;
import org.jvnet.jaxb.locator.util.LocatorUtils;
/**
* A short summary of an error - a fuller representation of the
* error may be retrieved from /{jobs}/{job-id}/error
*
* Java class for ErrorSummary complex type
.
*
* The following schema fragment specifies the expected content contained within this class.
*
* {@code
*
*
*
*
*
*
*
*
*
*
*
* }
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "ErrorSummary", propOrder = {
"message"
})
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public class ErrorSummary implements Cloneable, Copyable, PartialCopyable, MergeFrom, ToString
{
@XmlElement(required = true)
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
protected String message;
/**
* characterization of the type of the error
*
*/
@XmlAttribute(name = "type", required = true)
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
protected ErrorType type;
/**
* if true then there is a more detailed error message available at /{jobs}/{job-id}/error
*
*/
@XmlAttribute(name = "hasDetail", required = true)
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
protected boolean hasDetail;
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
protected transient ErrorSummary.Modifier __cachedModifier__;
/**
* Default no-arg constructor
*
*/
public ErrorSummary() {
super();
}
/**
* Fully-initialising value constructor
*
*/
public ErrorSummary(final String message, final ErrorType type, final boolean hasDetail) {
this.message = message;
this.type = type;
this.hasDetail = hasDetail;
}
/**
* Gets the value of the message property.
*
* @return
* possible object is
* {@link String }
*
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public String getMessage() {
return message;
}
/**
* Sets the value of the message property.
*
* @param value
* allowed object is
* {@link String }
*
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void setMessage(String value) {
this.message = value;
}
/**
* characterization of the type of the error
*
* @return
* possible object is
* {@link ErrorType }
*
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public ErrorType getType() {
return type;
}
/**
* Sets the value of the type property.
*
* @param value
* allowed object is
* {@link ErrorType }
*
* @see #getType()
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void setType(ErrorType value) {
this.type = value;
}
/**
* if true then there is a more detailed error message available at /{jobs}/{job-id}/error
*
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public boolean isHasDetail() {
return hasDetail;
}
/**
* Sets the value of the hasDetail property.
*
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void setHasDetail(boolean value) {
this.hasDetail = value;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public boolean equals(Object object) {
if ((object == null)||(this.getClass()!= object.getClass())) {
return false;
}
if (this == object) {
return true;
}
final ErrorSummary that = ((ErrorSummary) object);
{
String leftMessage;
leftMessage = this.getMessage();
String rightMessage;
rightMessage = that.getMessage();
if (this.message!= null) {
if (that.message!= null) {
if (!leftMessage.equals(rightMessage)) {
return false;
}
} else {
return false;
}
} else {
if (that.message!= null) {
return false;
}
}
}
{
ErrorType leftType;
leftType = this.getType();
ErrorType rightType;
rightType = that.getType();
if (this.type!= null) {
if (that.type!= null) {
if (!leftType.equals(rightType)) {
return false;
}
} else {
return false;
}
} else {
if (that.type!= null) {
return false;
}
}
}
{
boolean leftHasDetail;
leftHasDetail = this.isHasDetail();
boolean rightHasDetail;
rightHasDetail = that.isHasDetail();
if (leftHasDetail!= rightHasDetail) {
return false;
}
}
return true;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public int hashCode() {
int currentHashCode = 1;
{
currentHashCode = (currentHashCode* 31);
String theMessage;
theMessage = this.getMessage();
if (this.message!= null) {
currentHashCode += theMessage.hashCode();
}
}
{
currentHashCode = (currentHashCode* 31);
ErrorType theType;
theType = this.getType();
if (this.type!= null) {
currentHashCode += theType.hashCode();
}
}
{
currentHashCode = (currentHashCode* 31);
boolean theHasDetail;
theHasDetail = this.isHasDetail();
currentHashCode += (theHasDetail? 1231 : 1237);
}
return currentHashCode;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public String toString() {
final ToStringStrategy strategy = JAXBToStringStrategy.getInstance();
final StringBuilder buffer = new StringBuilder();
append(null, buffer, strategy);
return buffer.toString();
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public StringBuilder append(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
strategy.appendStart(locator, this, buffer);
appendFields(locator, buffer, strategy);
strategy.appendEnd(locator, this, buffer);
return buffer;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public StringBuilder appendFields(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
{
String theMessage;
theMessage = this.getMessage();
strategy.appendField(locator, this, "message", buffer, theMessage, (this.message!= null));
}
{
ErrorType theType;
theType = this.getType();
strategy.appendField(locator, this, "type", buffer, theType, (this.type!= null));
}
{
boolean theHasDetail;
theHasDetail = this.isHasDetail();
strategy.appendField(locator, this, "hasDetail", buffer, theHasDetail, true);
}
return buffer;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void mergeFrom(Object left, Object right) {
final MergeStrategy strategy = JAXBMergeStrategy.getInstance();
mergeFrom(null, null, left, right, strategy);
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void mergeFrom(ObjectLocator leftLocator, ObjectLocator rightLocator, Object left, Object right, MergeStrategy strategy) {
if (right instanceof ErrorSummary) {
final ErrorSummary target = this;
final ErrorSummary leftObject = ((ErrorSummary) left);
final ErrorSummary rightObject = ((ErrorSummary) right);
{
Boolean messageShouldBeMergedAndSet = strategy.shouldBeMergedAndSet(leftLocator, rightLocator, (leftObject.message!= null), (rightObject.message!= null));
if (messageShouldBeMergedAndSet == Boolean.TRUE) {
String lhsMessage;
lhsMessage = leftObject.getMessage();
String rhsMessage;
rhsMessage = rightObject.getMessage();
String mergedMessage = ((String) strategy.merge(LocatorUtils.property(leftLocator, "message", lhsMessage), LocatorUtils.property(rightLocator, "message", rhsMessage), lhsMessage, rhsMessage, (leftObject.message!= null), (rightObject.message!= null)));
target.setMessage(mergedMessage);
} else {
if (messageShouldBeMergedAndSet == Boolean.FALSE) {
target.message = null;
}
}
}
{
Boolean typeShouldBeMergedAndSet = strategy.shouldBeMergedAndSet(leftLocator, rightLocator, (leftObject.type!= null), (rightObject.type!= null));
if (typeShouldBeMergedAndSet == Boolean.TRUE) {
ErrorType lhsType;
lhsType = leftObject.getType();
ErrorType rhsType;
rhsType = rightObject.getType();
ErrorType mergedType = ((ErrorType) strategy.merge(LocatorUtils.property(leftLocator, "type", lhsType), LocatorUtils.property(rightLocator, "type", rhsType), lhsType, rhsType, (leftObject.type!= null), (rightObject.type!= null)));
target.setType(mergedType);
} else {
if (typeShouldBeMergedAndSet == Boolean.FALSE) {
target.type = null;
}
}
}
{
Boolean hasDetailShouldBeMergedAndSet = strategy.shouldBeMergedAndSet(leftLocator, rightLocator, true, true);
if (hasDetailShouldBeMergedAndSet == Boolean.TRUE) {
boolean lhsHasDetail;
lhsHasDetail = leftObject.isHasDetail();
boolean rhsHasDetail;
rhsHasDetail = rightObject.isHasDetail();
boolean mergedHasDetail = ((boolean) strategy.merge(LocatorUtils.property(leftLocator, "hasDetail", lhsHasDetail), LocatorUtils.property(rightLocator, "hasDetail", rhsHasDetail), lhsHasDetail, rhsHasDetail, true, true));
target.setHasDetail(mergedHasDetail);
} else {
if (hasDetailShouldBeMergedAndSet == Boolean.FALSE) {
}
}
}
}
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Object createNewInstance() {
return new ErrorSummary();
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public ErrorSummary clone() {
final ErrorSummary _newObject;
try {
_newObject = ((ErrorSummary) super.clone());
} catch (CloneNotSupportedException e) {
throw new RuntimeException(e);
}
return _newObject;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public ErrorSummary createCopy() {
final ErrorSummary _newObject;
try {
_newObject = ((ErrorSummary) super.clone());
} catch (CloneNotSupportedException e) {
throw new RuntimeException(e);
}
_newObject.message = this.message;
_newObject.type = this.type;
_newObject.hasDetail = this.hasDetail;
return _newObject;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public ErrorSummary createCopy(final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
final ErrorSummary _newObject;
try {
_newObject = ((ErrorSummary) super.clone());
} catch (CloneNotSupportedException e) {
throw new RuntimeException(e);
}
final PropertyTree messagePropertyTree = ((_propertyTree == null)?null:_propertyTree.get("message"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(messagePropertyTree!= null):((messagePropertyTree == null)||(!messagePropertyTree.isLeaf())))) {
_newObject.message = this.message;
}
final PropertyTree typePropertyTree = ((_propertyTree == null)?null:_propertyTree.get("type"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(typePropertyTree!= null):((typePropertyTree == null)||(!typePropertyTree.isLeaf())))) {
_newObject.type = this.type;
}
final PropertyTree hasDetailPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("hasDetail"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(hasDetailPropertyTree!= null):((hasDetailPropertyTree == null)||(!hasDetailPropertyTree.isLeaf())))) {
_newObject.hasDetail = this.hasDetail;
}
return _newObject;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public ErrorSummary copyExcept(final PropertyTree _propertyTree) {
return createCopy(_propertyTree, PropertyTreeUse.EXCLUDE);
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public ErrorSummary copyOnly(final PropertyTree _propertyTree) {
return createCopy(_propertyTree, PropertyTreeUse.INCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public ErrorSummary.Modifier modifier() {
if (null == this.__cachedModifier__) {
this.__cachedModifier__ = new ErrorSummary.Modifier();
}
return ((ErrorSummary.Modifier) this.__cachedModifier__);
}
/**
* Copies all state of this object to a builder. This method is used by the copyOf
* method and should not be called directly by client code.
*
* @param _other
* A builder instance to which the state of this object will be copied.
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public<_B >void copyTo(final ErrorSummary.Builder<_B> _other) {
_other.message = this.message;
_other.type = this.type;
_other.hasDetail = this.hasDetail;
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public<_B >ErrorSummary.Builder<_B> newCopyBuilder(final _B _parentBuilder) {
return new ErrorSummary.Builder<_B>(_parentBuilder, this, true);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public ErrorSummary.Builder newCopyBuilder() {
return newCopyBuilder(null);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static ErrorSummary.Builder builder() {
return new ErrorSummary.Builder<>(null, null, false);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static<_B >ErrorSummary.Builder<_B> copyOf(final ErrorSummary _other) {
final ErrorSummary.Builder<_B> _newBuilder = new ErrorSummary.Builder<>(null, null, false);
_other.copyTo(_newBuilder);
return _newBuilder;
}
/**
* Copies all state of this object to a builder. This method is used by the copyOf
* method and should not be called directly by client code.
*
* @param _other
* A builder instance to which the state of this object will be copied.
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public<_B >void copyTo(final ErrorSummary.Builder<_B> _other, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
final PropertyTree messagePropertyTree = ((_propertyTree == null)?null:_propertyTree.get("message"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(messagePropertyTree!= null):((messagePropertyTree == null)||(!messagePropertyTree.isLeaf())))) {
_other.message = this.message;
}
final PropertyTree typePropertyTree = ((_propertyTree == null)?null:_propertyTree.get("type"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(typePropertyTree!= null):((typePropertyTree == null)||(!typePropertyTree.isLeaf())))) {
_other.type = this.type;
}
final PropertyTree hasDetailPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("hasDetail"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(hasDetailPropertyTree!= null):((hasDetailPropertyTree == null)||(!hasDetailPropertyTree.isLeaf())))) {
_other.hasDetail = this.hasDetail;
}
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public<_B >ErrorSummary.Builder<_B> newCopyBuilder(final _B _parentBuilder, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
return new ErrorSummary.Builder<_B>(_parentBuilder, this, true, _propertyTree, _propertyTreeUse);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public ErrorSummary.Builder newCopyBuilder(final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
return newCopyBuilder(null, _propertyTree, _propertyTreeUse);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static<_B >ErrorSummary.Builder<_B> copyOf(final ErrorSummary _other, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
final ErrorSummary.Builder<_B> _newBuilder = new ErrorSummary.Builder<>(null, null, false);
_other.copyTo(_newBuilder, _propertyTree, _propertyTreeUse);
return _newBuilder;
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static ErrorSummary.Builder copyExcept(final ErrorSummary _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.EXCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static ErrorSummary.Builder copyOnly(final ErrorSummary _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.INCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public ErrorSummary visit(final PropertyVisitor _visitor_) {
_visitor_.visit(this);
_visitor_.visit(new SingleProperty<>(ErrorSummary.PropInfo.MESSAGE, this));
_visitor_.visit(new SingleProperty<>(ErrorSummary.PropInfo.TYPE, this));
_visitor_.visit(new SingleProperty<>(ErrorSummary.PropInfo.HAS_DETAIL, this));
return this;
}
public static class Builder<_B >implements Buildable
{
protected final _B _parentBuilder;
protected final ErrorSummary _storedValue;
private String message;
private ErrorType type;
private boolean hasDetail;
public Builder(final _B _parentBuilder, final ErrorSummary _other, final boolean _copy) {
this._parentBuilder = _parentBuilder;
if (_other!= null) {
if (_copy) {
_storedValue = null;
this.message = _other.message;
this.type = _other.type;
this.hasDetail = _other.hasDetail;
} else {
_storedValue = _other;
}
} else {
_storedValue = null;
}
}
public Builder(final _B _parentBuilder, final ErrorSummary _other, final boolean _copy, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
this._parentBuilder = _parentBuilder;
if (_other!= null) {
if (_copy) {
_storedValue = null;
final PropertyTree messagePropertyTree = ((_propertyTree == null)?null:_propertyTree.get("message"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(messagePropertyTree!= null):((messagePropertyTree == null)||(!messagePropertyTree.isLeaf())))) {
this.message = _other.message;
}
final PropertyTree typePropertyTree = ((_propertyTree == null)?null:_propertyTree.get("type"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(typePropertyTree!= null):((typePropertyTree == null)||(!typePropertyTree.isLeaf())))) {
this.type = _other.type;
}
final PropertyTree hasDetailPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("hasDetail"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(hasDetailPropertyTree!= null):((hasDetailPropertyTree == null)||(!hasDetailPropertyTree.isLeaf())))) {
this.hasDetail = _other.hasDetail;
}
} else {
_storedValue = _other;
}
} else {
_storedValue = null;
}
}
public _B end() {
return this._parentBuilder;
}
protected<_P extends ErrorSummary >_P init(final _P _product) {
_product.message = this.message;
_product.type = this.type;
_product.hasDetail = this.hasDetail;
return _product;
}
/**
* Sets the new value of "message" (any previous value will be replaced)
*
* @param message
* New value of the "message" property.
*/
public ErrorSummary.Builder<_B> withMessage(final String message) {
this.message = message;
return this;
}
/**
* Sets the new value of "type" (any previous value will be replaced)
*
* @param type
* New value of the "type" property.
*/
public ErrorSummary.Builder<_B> withType(final ErrorType type) {
this.type = type;
return this;
}
/**
* Sets the new value of "hasDetail" (any previous value will be replaced)
*
* @param hasDetail
* New value of the "hasDetail" property.
*/
public ErrorSummary.Builder<_B> withHasDetail(final boolean hasDetail) {
this.hasDetail = hasDetail;
return this;
}
@Override
public ErrorSummary build() {
if (_storedValue == null) {
return this.init(new ErrorSummary());
} else {
return ((ErrorSummary) _storedValue);
}
}
public ErrorSummary.Builder<_B> copyOf(final ErrorSummary _other) {
_other.copyTo(this);
return this;
}
public ErrorSummary.Builder<_B> copyOf(final ErrorSummary.Builder _other) {
return copyOf(_other.build());
}
}
public class Modifier {
public void setMessage(final String message) {
ErrorSummary.this.setMessage(message);
}
public void setType(final ErrorType type) {
ErrorSummary.this.setType(type);
}
public void setHasDetail(final boolean hasDetail) {
ErrorSummary.this.setHasDetail(hasDetail);
}
}
public static class PropInfo {
public static final transient SinglePropertyInfo MESSAGE = new SinglePropertyInfo("message", ErrorSummary.class, String.class, false, null, new QName("http://www.ivoa.net/xml/UWS/v1.0", "message"), new QName("http://www.w3.org/2001/XMLSchema", "string"), false) {
@Override
public String get(final ErrorSummary _instance_) {
return ((_instance_ == null)?null:_instance_.message);
}
@Override
public void set(final ErrorSummary _instance_, final String _value_) {
if (_instance_!= null) {
_instance_.message = _value_;
}
}
}
;
public static final transient SinglePropertyInfo TYPE = new SinglePropertyInfo("type", ErrorSummary.class, ErrorType.class, false, null, new QName("", "type"), new QName("http://www.ivoa.net/xml/UWS/v1.0", "ErrorType"), true) {
@Override
public ErrorType get(final ErrorSummary _instance_) {
return ((_instance_ == null)?null:_instance_.type);
}
@Override
public void set(final ErrorSummary _instance_, final ErrorType _value_) {
if (_instance_!= null) {
_instance_.type = _value_;
}
}
}
;
public static final transient SinglePropertyInfo HAS_DETAIL = new SinglePropertyInfo("hasDetail", ErrorSummary.class, Boolean.class, false, null, new QName("", "hasDetail"), new QName("http://www.w3.org/2001/XMLSchema", "boolean"), true) {
@Override
public Boolean get(final ErrorSummary _instance_) {
return ((_instance_ == null)?null:_instance_.hasDetail);
}
@Override
public void set(final ErrorSummary _instance_, final Boolean _value_) {
if (_instance_!= null) {
_instance_.hasDetail = _value_;
}
}
}
;
}
public static class Select
extends ErrorSummary.Selector
{
Select() {
super(null, null, null);
}
public static ErrorSummary.Select _root() {
return new ErrorSummary.Select();
}
}
public static class Selector , TParent >
extends com.kscs.util.jaxb.Selector
{
private com.kscs.util.jaxb.Selector> message = null;
private com.kscs.util.jaxb.Selector> type = null;
public Selector(final TRoot root, final TParent parent, final String propertyName) {
super(root, parent, propertyName);
}
@Override
public Map buildChildren() {
final Map products = new HashMap<>();
products.putAll(super.buildChildren());
if (this.message!= null) {
products.put("message", this.message.init());
}
if (this.type!= null) {
products.put("type", this.type.init());
}
return products;
}
public com.kscs.util.jaxb.Selector> message() {
return ((this.message == null)?this.message = new com.kscs.util.jaxb.Selector<>(this._root, this, "message"):this.message);
}
public com.kscs.util.jaxb.Selector> type() {
return ((this.type == null)?this.type = new com.kscs.util.jaxb.Selector<>(this._root, this, "type"):this.type);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy