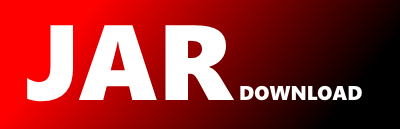
org.javastro.ivoa.entities.vodml.Enumeration Maven / Gradle / Ivy
package org.javastro.ivoa.entities.vodml;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import javax.xml.namespace.QName;
import com.kscs.util.jaxb.Buildable;
import com.kscs.util.jaxb.CollectionProperty;
import com.kscs.util.jaxb.CollectionPropertyInfo;
import com.kscs.util.jaxb.CollectionPropertyInfo;
import com.kscs.util.jaxb.Copyable;
import com.kscs.util.jaxb.PartialCopyable;
import com.kscs.util.jaxb.PropertyTree;
import com.kscs.util.jaxb.PropertyTreeUse;
import com.kscs.util.jaxb.PropertyVisitor;
import jakarta.annotation.Generated;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlType;
import org.jvnet.jaxb.lang.JAXBMergeStrategy;
import org.jvnet.jaxb.lang.JAXBToStringStrategy;
import org.jvnet.jaxb.lang.MergeFrom;
import org.jvnet.jaxb.lang.MergeStrategy;
import org.jvnet.jaxb.lang.ToString;
import org.jvnet.jaxb.lang.ToStringStrategy;
import org.jvnet.jaxb.locator.ObjectLocator;
import org.jvnet.jaxb.locator.util.LocatorUtils;
/**
* A primitive type with a limited, discrete set of values.
* May explicitly extend a PrimitiveType. Its values must be compatible with that type then.
* TBD Should define what it
* might mean for an enumeraiton to extend another enumeration.
* Should it restrict the possible values further? Or should it add to the values? Or ...?
*
* Java class for Enumeration complex type
.
*
* The following schema fragment specifies the expected content contained within this class.
*
* {@code
*
*
*
*
*
*
*
*
*
* }
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "Enumeration", propOrder = {
"literals"
})
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public class Enumeration
extends PrimitiveType
implements Cloneable, Copyable, PartialCopyable, MergeFrom, ToString
{
/**
* TODO
*
*/
@XmlElement(name = "literal", required = true)
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
protected List literals;
/**
* Default no-arg constructor
*
*/
public Enumeration() {
super();
}
/**
* Fully-initialising value constructor
*
*/
public Enumeration(final String vodmlId, final String name, final String description, final String id, final ElementRef _extends, final List constraints, final Boolean _abstract, final List literals) {
super(vodmlId, name, description, id, _extends, constraints, _abstract);
this.literals = literals;
}
/**
* TODO
*
* Gets the value of the literals property.
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the literals property.
*
*
* For example, to add a new item, do as follows:
*
*
* getLiterals().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link EnumLiteral }
*
*
*
* @return
* The value of the literals property.
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public List getLiterals() {
if (literals == null) {
literals = new ArrayList<>();
}
return this.literals;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public boolean equals(Object object) {
if ((object == null)||(this.getClass()!= object.getClass())) {
return false;
}
if (this == object) {
return true;
}
if (!super.equals(object)) {
return false;
}
final Enumeration that = ((Enumeration) object);
{
List leftLiterals;
leftLiterals = (((this.literals!= null)&&(!this.literals.isEmpty()))?this.getLiterals():null);
List rightLiterals;
rightLiterals = (((that.literals!= null)&&(!that.literals.isEmpty()))?that.getLiterals():null);
if ((this.literals!= null)&&(!this.literals.isEmpty())) {
if ((that.literals!= null)&&(!that.literals.isEmpty())) {
if (!leftLiterals.equals(rightLiterals)) {
return false;
}
} else {
return false;
}
} else {
if ((that.literals!= null)&&(!that.literals.isEmpty())) {
return false;
}
}
}
return true;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public int hashCode() {
int currentHashCode = 1;
currentHashCode = ((currentHashCode* 31)+ super.hashCode());
{
currentHashCode = (currentHashCode* 31);
List theLiterals;
theLiterals = (((this.literals!= null)&&(!this.literals.isEmpty()))?this.getLiterals():null);
if ((this.literals!= null)&&(!this.literals.isEmpty())) {
currentHashCode += theLiterals.hashCode();
}
}
return currentHashCode;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public String toString() {
final ToStringStrategy strategy = JAXBToStringStrategy.getInstance();
final StringBuilder buffer = new StringBuilder();
append(null, buffer, strategy);
return buffer.toString();
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public StringBuilder append(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
strategy.appendStart(locator, this, buffer);
appendFields(locator, buffer, strategy);
strategy.appendEnd(locator, this, buffer);
return buffer;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public StringBuilder appendFields(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
super.appendFields(locator, buffer, strategy);
{
List theLiterals;
theLiterals = (((this.literals!= null)&&(!this.literals.isEmpty()))?this.getLiterals():null);
strategy.appendField(locator, this, "literals", buffer, theLiterals, ((this.literals!= null)&&(!this.literals.isEmpty())));
}
return buffer;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void mergeFrom(Object left, Object right) {
final MergeStrategy strategy = JAXBMergeStrategy.getInstance();
mergeFrom(null, null, left, right, strategy);
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void mergeFrom(ObjectLocator leftLocator, ObjectLocator rightLocator, Object left, Object right, MergeStrategy strategy) {
super.mergeFrom(leftLocator, rightLocator, left, right, strategy);
if (right instanceof Enumeration) {
final Enumeration target = this;
final Enumeration leftObject = ((Enumeration) left);
final Enumeration rightObject = ((Enumeration) right);
{
Boolean literalsShouldBeMergedAndSet = strategy.shouldBeMergedAndSet(leftLocator, rightLocator, ((leftObject.literals!= null)&&(!leftObject.literals.isEmpty())), ((rightObject.literals!= null)&&(!rightObject.literals.isEmpty())));
if (literalsShouldBeMergedAndSet == Boolean.TRUE) {
List lhsLiterals;
lhsLiterals = (((leftObject.literals!= null)&&(!leftObject.literals.isEmpty()))?leftObject.getLiterals():null);
List rhsLiterals;
rhsLiterals = (((rightObject.literals!= null)&&(!rightObject.literals.isEmpty()))?rightObject.getLiterals():null);
List mergedLiterals = ((List ) strategy.merge(LocatorUtils.property(leftLocator, "literals", lhsLiterals), LocatorUtils.property(rightLocator, "literals", rhsLiterals), lhsLiterals, rhsLiterals, ((leftObject.literals!= null)&&(!leftObject.literals.isEmpty())), ((rightObject.literals!= null)&&(!rightObject.literals.isEmpty()))));
target.literals = null;
if (mergedLiterals!= null) {
List uniqueLiteralsl = target.getLiterals();
uniqueLiteralsl.addAll(mergedLiterals);
}
} else {
if (literalsShouldBeMergedAndSet == Boolean.FALSE) {
target.literals = null;
}
}
}
}
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Object createNewInstance() {
return new Enumeration();
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Enumeration clone() {
final Enumeration _newObject;
_newObject = ((Enumeration) super.clone());
if (this.literals == null) {
_newObject.literals = null;
} else {
_newObject.literals = new ArrayList<>();
for (EnumLiteral _item: this.literals) {
_newObject.literals.add(((_item == null)?null:_item.clone()));
}
}
return _newObject;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Enumeration createCopy() {
final Enumeration _newObject = ((Enumeration) super.createCopy());
if (this.literals == null) {
_newObject.literals = null;
} else {
_newObject.literals = new ArrayList<>();
for (EnumLiteral _item: this.literals) {
_newObject.literals.add(((_item == null)?null:_item.createCopy()));
}
}
return _newObject;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Enumeration createCopy(final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
final Enumeration _newObject = ((Enumeration) super.createCopy(_propertyTree, _propertyTreeUse));
final PropertyTree literalsPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("literals"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(literalsPropertyTree!= null):((literalsPropertyTree == null)||(!literalsPropertyTree.isLeaf())))) {
if (this.literals == null) {
_newObject.literals = null;
} else {
_newObject.literals = new ArrayList<>();
for (EnumLiteral _item: this.literals) {
_newObject.literals.add(((_item == null)?null:_item.createCopy(literalsPropertyTree, _propertyTreeUse)));
}
}
}
return _newObject;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Enumeration copyExcept(final PropertyTree _propertyTree) {
return createCopy(_propertyTree, PropertyTreeUse.EXCLUDE);
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Enumeration copyOnly(final PropertyTree _propertyTree) {
return createCopy(_propertyTree, PropertyTreeUse.INCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Enumeration.Modifier modifier() {
if (null == this.__cachedModifier__) {
this.__cachedModifier__ = new Enumeration.Modifier();
}
return ((Enumeration.Modifier) this.__cachedModifier__);
}
/**
* Copies all state of this object to a builder. This method is used by the copyOf
* method and should not be called directly by client code.
*
* @param _other
* A builder instance to which the state of this object will be copied.
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public<_B >void copyTo(final Enumeration.Builder<_B> _other) {
super.copyTo(_other);
if (this.literals == null) {
_other.literals = null;
} else {
_other.literals = new ArrayList<>();
for (EnumLiteral _item: this.literals) {
_other.literals.add(((_item == null)?null:_item.newCopyBuilder(_other)));
}
}
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public<_B >Enumeration.Builder<_B> newCopyBuilder(final _B _parentBuilder) {
return new Enumeration.Builder<_B>(_parentBuilder, this, true);
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Enumeration.Builder newCopyBuilder() {
return newCopyBuilder(null);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static Enumeration.Builder builder() {
return new Enumeration.Builder<>(null, null, false);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static<_B >Enumeration.Builder<_B> copyOf(final ReferableElement _other) {
final Enumeration.Builder<_B> _newBuilder = new Enumeration.Builder<>(null, null, false);
_other.copyTo(_newBuilder);
return _newBuilder;
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static<_B >Enumeration.Builder<_B> copyOf(final Type _other) {
final Enumeration.Builder<_B> _newBuilder = new Enumeration.Builder<>(null, null, false);
_other.copyTo(_newBuilder);
return _newBuilder;
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static<_B >Enumeration.Builder<_B> copyOf(final ValueType _other) {
final Enumeration.Builder<_B> _newBuilder = new Enumeration.Builder<>(null, null, false);
_other.copyTo(_newBuilder);
return _newBuilder;
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static<_B >Enumeration.Builder<_B> copyOf(final PrimitiveType _other) {
final Enumeration.Builder<_B> _newBuilder = new Enumeration.Builder<>(null, null, false);
_other.copyTo(_newBuilder);
return _newBuilder;
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static<_B >Enumeration.Builder<_B> copyOf(final Enumeration _other) {
final Enumeration.Builder<_B> _newBuilder = new Enumeration.Builder<>(null, null, false);
_other.copyTo(_newBuilder);
return _newBuilder;
}
/**
* Copies all state of this object to a builder. This method is used by the copyOf
* method and should not be called directly by client code.
*
* @param _other
* A builder instance to which the state of this object will be copied.
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public<_B >void copyTo(final Enumeration.Builder<_B> _other, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
super.copyTo(_other, _propertyTree, _propertyTreeUse);
final PropertyTree literalsPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("literals"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(literalsPropertyTree!= null):((literalsPropertyTree == null)||(!literalsPropertyTree.isLeaf())))) {
if (this.literals == null) {
_other.literals = null;
} else {
_other.literals = new ArrayList<>();
for (EnumLiteral _item: this.literals) {
_other.literals.add(((_item == null)?null:_item.newCopyBuilder(_other, literalsPropertyTree, _propertyTreeUse)));
}
}
}
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public<_B >Enumeration.Builder<_B> newCopyBuilder(final _B _parentBuilder, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
return new Enumeration.Builder<_B>(_parentBuilder, this, true, _propertyTree, _propertyTreeUse);
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Enumeration.Builder newCopyBuilder(final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
return newCopyBuilder(null, _propertyTree, _propertyTreeUse);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static<_B >Enumeration.Builder<_B> copyOf(final ReferableElement _other, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
final Enumeration.Builder<_B> _newBuilder = new Enumeration.Builder<>(null, null, false);
_other.copyTo(_newBuilder, _propertyTree, _propertyTreeUse);
return _newBuilder;
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static<_B >Enumeration.Builder<_B> copyOf(final Type _other, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
final Enumeration.Builder<_B> _newBuilder = new Enumeration.Builder<>(null, null, false);
_other.copyTo(_newBuilder, _propertyTree, _propertyTreeUse);
return _newBuilder;
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static<_B >Enumeration.Builder<_B> copyOf(final ValueType _other, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
final Enumeration.Builder<_B> _newBuilder = new Enumeration.Builder<>(null, null, false);
_other.copyTo(_newBuilder, _propertyTree, _propertyTreeUse);
return _newBuilder;
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static<_B >Enumeration.Builder<_B> copyOf(final PrimitiveType _other, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
final Enumeration.Builder<_B> _newBuilder = new Enumeration.Builder<>(null, null, false);
_other.copyTo(_newBuilder, _propertyTree, _propertyTreeUse);
return _newBuilder;
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static<_B >Enumeration.Builder<_B> copyOf(final Enumeration _other, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
final Enumeration.Builder<_B> _newBuilder = new Enumeration.Builder<>(null, null, false);
_other.copyTo(_newBuilder, _propertyTree, _propertyTreeUse);
return _newBuilder;
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static Enumeration.Builder copyExcept(final ReferableElement _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.EXCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static Enumeration.Builder copyExcept(final Type _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.EXCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static Enumeration.Builder copyExcept(final ValueType _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.EXCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static Enumeration.Builder copyExcept(final PrimitiveType _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.EXCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static Enumeration.Builder copyExcept(final Enumeration _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.EXCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static Enumeration.Builder copyOnly(final ReferableElement _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.INCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static Enumeration.Builder copyOnly(final Type _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.INCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static Enumeration.Builder copyOnly(final ValueType _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.INCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static Enumeration.Builder copyOnly(final PrimitiveType _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.INCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static Enumeration.Builder copyOnly(final Enumeration _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.INCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Enumeration visit(final PropertyVisitor _visitor_) {
super.visit(_visitor_);
if (_visitor_.visit(new CollectionProperty<>(Enumeration.PropInfo.LITERALS, this))&&(this.literals!= null)) {
for (EnumLiteral _item_: this.literals) {
if (_item_!= null) {
_item_.visit(_visitor_);
}
}
}
return this;
}
public static class Builder<_B >
extends PrimitiveType.Builder<_B>
implements Buildable
{
private List>> literals;
public Builder(final _B _parentBuilder, final Enumeration _other, final boolean _copy) {
super(_parentBuilder, _other, _copy);
if (_other!= null) {
if (_other.literals == null) {
this.literals = null;
} else {
this.literals = new ArrayList<>();
for (EnumLiteral _item: _other.literals) {
this.literals.add(((_item == null)?null:_item.newCopyBuilder(this)));
}
}
}
}
public Builder(final _B _parentBuilder, final Enumeration _other, final boolean _copy, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
super(_parentBuilder, _other, _copy, _propertyTree, _propertyTreeUse);
if (_other!= null) {
final PropertyTree literalsPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("literals"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(literalsPropertyTree!= null):((literalsPropertyTree == null)||(!literalsPropertyTree.isLeaf())))) {
if (_other.literals == null) {
this.literals = null;
} else {
this.literals = new ArrayList<>();
for (EnumLiteral _item: _other.literals) {
this.literals.add(((_item == null)?null:_item.newCopyBuilder(this, literalsPropertyTree, _propertyTreeUse)));
}
}
}
}
}
protected<_P extends Enumeration >_P init(final _P _product) {
if (this.literals!= null) {
final List literals = new ArrayList<>(this.literals.size());
for (EnumLiteral.Builder> _item: this.literals) {
literals.add(_item.build());
}
_product.literals = literals;
}
return super.init(_product);
}
/**
* Adds the given items to the value of "literals"
*
* @param literals
* Items to add to the value of the "literals" property
*/
public Enumeration.Builder<_B> addLiterals(final Iterable extends EnumLiteral> literals) {
if (literals!= null) {
if (this.literals == null) {
this.literals = new ArrayList<>();
}
for (EnumLiteral _item: literals) {
this.literals.add(new EnumLiteral.Builder<>(this, _item, false));
}
}
return this;
}
/**
* Sets the new value of "literals" (any previous value will be replaced)
*
* @param literals
* New value of the "literals" property.
*/
public Enumeration.Builder<_B> withLiterals(final Iterable extends EnumLiteral> literals) {
if (this.literals!= null) {
this.literals.clear();
}
return addLiterals(literals);
}
/**
* Adds the given items to the value of "literals"
*
* @param literals
* Items to add to the value of the "literals" property
*/
public Enumeration.Builder<_B> addLiterals(EnumLiteral... literals) {
addLiterals(Arrays.asList(literals));
return this;
}
/**
* Sets the new value of "literals" (any previous value will be replaced)
*
* @param literals
* New value of the "literals" property.
*/
public Enumeration.Builder<_B> withLiterals(EnumLiteral... literals) {
withLiterals(Arrays.asList(literals));
return this;
}
/**
* Returns a new builder to build an additional value of the "Literals" property.
* Use {@link org.javastro.ivoa.entities.vodml.EnumLiteral.Builder#end()} to return
* to the current builder.
*
* @return
* a new builder to build an additional value of the "Literals" property.
* Use {@link org.javastro.ivoa.entities.vodml.EnumLiteral.Builder#end()} to return
* to the current builder.
*/
public EnumLiteral.Builder extends Enumeration.Builder<_B>> addLiterals() {
if (this.literals == null) {
this.literals = new ArrayList<>();
}
final EnumLiteral.Builder> literals_Builder = new EnumLiteral.Builder<>(this, null, false);
this.literals.add(literals_Builder);
return literals_Builder;
}
/**
* Sets the new value of "_extends" (any previous value will be replaced)
*
* @param _extends
* New value of the "_extends" property.
*/
@Override
public Enumeration.Builder<_B> withExtends(final ElementRef _extends) {
super.withExtends(_extends);
return this;
}
/**
* Returns the existing builder or a new builder to build the value of the
* "_extends" property.
* Use {@link org.javastro.ivoa.entities.vodml.ElementRef.Builder#end()} to return
* to the current builder.
*
* @return
* A new builder to build the value of the "_extends" property.
* Use {@link org.javastro.ivoa.entities.vodml.ElementRef.Builder#end()} to return
* to the current builder.
*/
public ElementRef.Builder extends Enumeration.Builder<_B>> withExtends() {
return ((ElementRef.Builder extends Enumeration.Builder<_B>> ) super.withExtends());
}
/**
* Adds the given items to the value of "constraints"
*
* @param constraints
* Items to add to the value of the "constraints" property
*/
@Override
public Enumeration.Builder<_B> addConstraints(final Iterable extends Constraint> constraints) {
super.addConstraints(constraints);
return this;
}
/**
* Adds the given items to the value of "constraints"
*
* @param constraints
* Items to add to the value of the "constraints" property
*/
@Override
public Enumeration.Builder<_B> addConstraints(Constraint... constraints) {
super.addConstraints(constraints);
return this;
}
/**
* Sets the new value of "constraints" (any previous value will be replaced)
*
* @param constraints
* New value of the "constraints" property.
*/
@Override
public Enumeration.Builder<_B> withConstraints(final Iterable extends Constraint> constraints) {
super.withConstraints(constraints);
return this;
}
/**
* Sets the new value of "constraints" (any previous value will be replaced)
*
* @param constraints
* New value of the "constraints" property.
*/
@Override
public Enumeration.Builder<_B> withConstraints(Constraint... constraints) {
super.withConstraints(constraints);
return this;
}
/**
* Sets the new value of "_abstract" (any previous value will be replaced)
*
* @param _abstract
* New value of the "_abstract" property.
*/
@Override
public Enumeration.Builder<_B> withAbstract(final Boolean _abstract) {
super.withAbstract(_abstract);
return this;
}
/**
* Sets the new value of "vodmlId" (any previous value will be replaced)
*
* @param vodmlId
* New value of the "vodmlId" property.
*/
@Override
public Enumeration.Builder<_B> withVodmlId(final String vodmlId) {
super.withVodmlId(vodmlId);
return this;
}
/**
* Sets the new value of "name" (any previous value will be replaced)
*
* @param name
* New value of the "name" property.
*/
@Override
public Enumeration.Builder<_B> withName(final String name) {
super.withName(name);
return this;
}
/**
* Sets the new value of "description" (any previous value will be replaced)
*
* @param description
* New value of the "description" property.
*/
@Override
public Enumeration.Builder<_B> withDescription(final String description) {
super.withDescription(description);
return this;
}
/**
* Sets the new value of "id" (any previous value will be replaced)
*
* @param id
* New value of the "id" property.
*/
@Override
public Enumeration.Builder<_B> withId(final String id) {
super.withId(id);
return this;
}
@Override
public Enumeration build() {
if (_storedValue == null) {
return this.init(new Enumeration());
} else {
return ((Enumeration) _storedValue);
}
}
public Enumeration.Builder<_B> copyOf(final Enumeration _other) {
_other.copyTo(this);
return this;
}
public Enumeration.Builder<_B> copyOf(final Enumeration.Builder _other) {
return copyOf(_other.build());
}
}
public class Modifier
extends PrimitiveType.Modifier
{
public List getLiterals() {
if (Enumeration.this.literals == null) {
Enumeration.this.literals = new ArrayList<>();
}
return Enumeration.this.literals;
}
}
public static class PropInfo {
public static final transient CollectionPropertyInfo LITERALS = new CollectionPropertyInfo("literals", Enumeration.class, EnumLiteral.class, true, null, new QName("", "literal"), new QName("http://www.ivoa.net/xml/VODML/v1", "EnumLiteral"), false) {
@Override
public List get(final Enumeration _instance_) {
return ((_instance_ == null)?null:_instance_.literals);
}
@Override
public void set(final Enumeration _instance_, final List _value_) {
if (_instance_!= null) {
_instance_.literals = _value_;
}
}
}
;
}
public static class Select
extends Enumeration.Selector
{
Select() {
super(null, null, null);
}
public static Enumeration.Select _root() {
return new Enumeration.Select();
}
}
public static class Selector , TParent >
extends PrimitiveType.Selector
{
private EnumLiteral.Selector> literals = null;
public Selector(final TRoot root, final TParent parent, final String propertyName) {
super(root, parent, propertyName);
}
@Override
public Map buildChildren() {
final Map products = new HashMap<>();
products.putAll(super.buildChildren());
if (this.literals!= null) {
products.put("literals", this.literals.init());
}
return products;
}
public EnumLiteral.Selector> literals() {
return ((this.literals == null)?this.literals = new EnumLiteral.Selector<>(this._root, this, "literals"):this.literals);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy