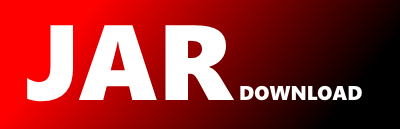
org.javastro.ivoa.entities.vodml.Multiplicity Maven / Gradle / Ivy
package org.javastro.ivoa.entities.vodml;
import java.math.BigInteger;
import java.util.HashMap;
import java.util.Map;
import javax.xml.namespace.QName;
import com.kscs.util.jaxb.Buildable;
import com.kscs.util.jaxb.Copyable;
import com.kscs.util.jaxb.PartialCopyable;
import com.kscs.util.jaxb.PropertyTree;
import com.kscs.util.jaxb.PropertyTreeUse;
import com.kscs.util.jaxb.PropertyVisitor;
import com.kscs.util.jaxb.SingleProperty;
import com.kscs.util.jaxb.SinglePropertyInfo;
import com.kscs.util.jaxb.SinglePropertyInfo;
import com.kscs.util.jaxb.SinglePropertyInfo;
import jakarta.annotation.Generated;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlSchemaType;
import jakarta.xml.bind.annotation.XmlType;
import org.jvnet.jaxb.lang.JAXBMergeStrategy;
import org.jvnet.jaxb.lang.JAXBToStringStrategy;
import org.jvnet.jaxb.lang.MergeFrom;
import org.jvnet.jaxb.lang.MergeStrategy;
import org.jvnet.jaxb.lang.ToString;
import org.jvnet.jaxb.lang.ToStringStrategy;
import org.jvnet.jaxb.locator.ObjectLocator;
import org.jvnet.jaxb.locator.util.LocatorUtils;
/**
* Also called "Cardinality". Indicates how many instances of a datatype can/must be associated to a given role.
* Unless
* Follows model in XSD, i.e. with explicit lower bound and upper bound on number of instances.
* maxOccurs must be gte minOccurs, unless it is negative, in which case it corresponds to unbounded.
*
* Java class for Multiplicity complex type
.
*
* The following schema fragment specifies the expected content contained within this class.
*
* {@code
*
*
*
*
*
*
*
*
*
*
*
*
* }
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "Multiplicity", propOrder = {
"minOccurs",
"maxOccurs"
})
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public class Multiplicity implements Cloneable, Copyable, PartialCopyable, MergeFrom, ToString
{
/**
* Lower bound on number of instances/values.
*
*/
@XmlSchemaType(name = "nonNegativeInteger")
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
protected BigInteger minOccurs;
/**
* When negative, unbounded.
*
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
protected int maxOccurs;
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
protected transient Multiplicity.Modifier __cachedModifier__;
/**
* Default no-arg constructor
*
*/
public Multiplicity() {
super();
}
/**
* Fully-initialising value constructor
*
*/
public Multiplicity(final BigInteger minOccurs, final int maxOccurs) {
this.minOccurs = minOccurs;
this.maxOccurs = maxOccurs;
}
/**
* Lower bound on number of instances/values.
*
* @return
* possible object is
* {@link BigInteger }
*
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public BigInteger getMinOccurs() {
return minOccurs;
}
/**
* Sets the value of the minOccurs property.
*
* @param value
* allowed object is
* {@link BigInteger }
*
* @see #getMinOccurs()
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void setMinOccurs(BigInteger value) {
this.minOccurs = value;
}
/**
* When negative, unbounded.
*
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public int getMaxOccurs() {
return maxOccurs;
}
/**
* Sets the value of the maxOccurs property.
*
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void setMaxOccurs(int value) {
this.maxOccurs = value;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public boolean equals(Object object) {
if ((object == null)||(this.getClass()!= object.getClass())) {
return false;
}
if (this == object) {
return true;
}
final Multiplicity that = ((Multiplicity) object);
{
BigInteger leftMinOccurs;
leftMinOccurs = this.getMinOccurs();
BigInteger rightMinOccurs;
rightMinOccurs = that.getMinOccurs();
if (this.minOccurs!= null) {
if (that.minOccurs!= null) {
if (!leftMinOccurs.equals(rightMinOccurs)) {
return false;
}
} else {
return false;
}
} else {
if (that.minOccurs!= null) {
return false;
}
}
}
{
int leftMaxOccurs;
leftMaxOccurs = this.getMaxOccurs();
int rightMaxOccurs;
rightMaxOccurs = that.getMaxOccurs();
if (leftMaxOccurs!= rightMaxOccurs) {
return false;
}
}
return true;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public int hashCode() {
int currentHashCode = 1;
{
currentHashCode = (currentHashCode* 31);
BigInteger theMinOccurs;
theMinOccurs = this.getMinOccurs();
if (this.minOccurs!= null) {
currentHashCode += theMinOccurs.hashCode();
}
}
{
currentHashCode = (currentHashCode* 31);
int theMaxOccurs;
theMaxOccurs = this.getMaxOccurs();
currentHashCode += theMaxOccurs;
}
return currentHashCode;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public String toString() {
final ToStringStrategy strategy = JAXBToStringStrategy.getInstance();
final StringBuilder buffer = new StringBuilder();
append(null, buffer, strategy);
return buffer.toString();
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public StringBuilder append(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
strategy.appendStart(locator, this, buffer);
appendFields(locator, buffer, strategy);
strategy.appendEnd(locator, this, buffer);
return buffer;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public StringBuilder appendFields(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
{
BigInteger theMinOccurs;
theMinOccurs = this.getMinOccurs();
strategy.appendField(locator, this, "minOccurs", buffer, theMinOccurs, (this.minOccurs!= null));
}
{
int theMaxOccurs;
theMaxOccurs = this.getMaxOccurs();
strategy.appendField(locator, this, "maxOccurs", buffer, theMaxOccurs, true);
}
return buffer;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void mergeFrom(Object left, Object right) {
final MergeStrategy strategy = JAXBMergeStrategy.getInstance();
mergeFrom(null, null, left, right, strategy);
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void mergeFrom(ObjectLocator leftLocator, ObjectLocator rightLocator, Object left, Object right, MergeStrategy strategy) {
if (right instanceof Multiplicity) {
final Multiplicity target = this;
final Multiplicity leftObject = ((Multiplicity) left);
final Multiplicity rightObject = ((Multiplicity) right);
{
Boolean minOccursShouldBeMergedAndSet = strategy.shouldBeMergedAndSet(leftLocator, rightLocator, (leftObject.minOccurs!= null), (rightObject.minOccurs!= null));
if (minOccursShouldBeMergedAndSet == Boolean.TRUE) {
BigInteger lhsMinOccurs;
lhsMinOccurs = leftObject.getMinOccurs();
BigInteger rhsMinOccurs;
rhsMinOccurs = rightObject.getMinOccurs();
BigInteger mergedMinOccurs = ((BigInteger) strategy.merge(LocatorUtils.property(leftLocator, "minOccurs", lhsMinOccurs), LocatorUtils.property(rightLocator, "minOccurs", rhsMinOccurs), lhsMinOccurs, rhsMinOccurs, (leftObject.minOccurs!= null), (rightObject.minOccurs!= null)));
target.setMinOccurs(mergedMinOccurs);
} else {
if (minOccursShouldBeMergedAndSet == Boolean.FALSE) {
target.minOccurs = null;
}
}
}
{
Boolean maxOccursShouldBeMergedAndSet = strategy.shouldBeMergedAndSet(leftLocator, rightLocator, true, true);
if (maxOccursShouldBeMergedAndSet == Boolean.TRUE) {
int lhsMaxOccurs;
lhsMaxOccurs = leftObject.getMaxOccurs();
int rhsMaxOccurs;
rhsMaxOccurs = rightObject.getMaxOccurs();
int mergedMaxOccurs = ((int) strategy.merge(LocatorUtils.property(leftLocator, "maxOccurs", lhsMaxOccurs), LocatorUtils.property(rightLocator, "maxOccurs", rhsMaxOccurs), lhsMaxOccurs, rhsMaxOccurs, true, true));
target.setMaxOccurs(mergedMaxOccurs);
} else {
if (maxOccursShouldBeMergedAndSet == Boolean.FALSE) {
}
}
}
}
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Object createNewInstance() {
return new Multiplicity();
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Multiplicity clone() {
final Multiplicity _newObject;
try {
_newObject = ((Multiplicity) super.clone());
} catch (CloneNotSupportedException e) {
throw new RuntimeException(e);
}
return _newObject;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Multiplicity createCopy() {
final Multiplicity _newObject;
try {
_newObject = ((Multiplicity) super.clone());
} catch (CloneNotSupportedException e) {
throw new RuntimeException(e);
}
_newObject.minOccurs = this.minOccurs;
_newObject.maxOccurs = this.maxOccurs;
return _newObject;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Multiplicity createCopy(final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
final Multiplicity _newObject;
try {
_newObject = ((Multiplicity) super.clone());
} catch (CloneNotSupportedException e) {
throw new RuntimeException(e);
}
final PropertyTree minOccursPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("minOccurs"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(minOccursPropertyTree!= null):((minOccursPropertyTree == null)||(!minOccursPropertyTree.isLeaf())))) {
_newObject.minOccurs = this.minOccurs;
}
final PropertyTree maxOccursPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("maxOccurs"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(maxOccursPropertyTree!= null):((maxOccursPropertyTree == null)||(!maxOccursPropertyTree.isLeaf())))) {
_newObject.maxOccurs = this.maxOccurs;
}
return _newObject;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Multiplicity copyExcept(final PropertyTree _propertyTree) {
return createCopy(_propertyTree, PropertyTreeUse.EXCLUDE);
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Multiplicity copyOnly(final PropertyTree _propertyTree) {
return createCopy(_propertyTree, PropertyTreeUse.INCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Multiplicity.Modifier modifier() {
if (null == this.__cachedModifier__) {
this.__cachedModifier__ = new Multiplicity.Modifier();
}
return ((Multiplicity.Modifier) this.__cachedModifier__);
}
/**
* Copies all state of this object to a builder. This method is used by the copyOf
* method and should not be called directly by client code.
*
* @param _other
* A builder instance to which the state of this object will be copied.
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public<_B >void copyTo(final Multiplicity.Builder<_B> _other) {
_other.minOccurs = this.minOccurs;
_other.maxOccurs = this.maxOccurs;
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public<_B >Multiplicity.Builder<_B> newCopyBuilder(final _B _parentBuilder) {
return new Multiplicity.Builder<_B>(_parentBuilder, this, true);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Multiplicity.Builder newCopyBuilder() {
return newCopyBuilder(null);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static Multiplicity.Builder builder() {
return new Multiplicity.Builder<>(null, null, false);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static<_B >Multiplicity.Builder<_B> copyOf(final Multiplicity _other) {
final Multiplicity.Builder<_B> _newBuilder = new Multiplicity.Builder<>(null, null, false);
_other.copyTo(_newBuilder);
return _newBuilder;
}
/**
* Copies all state of this object to a builder. This method is used by the copyOf
* method and should not be called directly by client code.
*
* @param _other
* A builder instance to which the state of this object will be copied.
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public<_B >void copyTo(final Multiplicity.Builder<_B> _other, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
final PropertyTree minOccursPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("minOccurs"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(minOccursPropertyTree!= null):((minOccursPropertyTree == null)||(!minOccursPropertyTree.isLeaf())))) {
_other.minOccurs = this.minOccurs;
}
final PropertyTree maxOccursPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("maxOccurs"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(maxOccursPropertyTree!= null):((maxOccursPropertyTree == null)||(!maxOccursPropertyTree.isLeaf())))) {
_other.maxOccurs = this.maxOccurs;
}
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public<_B >Multiplicity.Builder<_B> newCopyBuilder(final _B _parentBuilder, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
return new Multiplicity.Builder<_B>(_parentBuilder, this, true, _propertyTree, _propertyTreeUse);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Multiplicity.Builder newCopyBuilder(final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
return newCopyBuilder(null, _propertyTree, _propertyTreeUse);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static<_B >Multiplicity.Builder<_B> copyOf(final Multiplicity _other, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
final Multiplicity.Builder<_B> _newBuilder = new Multiplicity.Builder<>(null, null, false);
_other.copyTo(_newBuilder, _propertyTree, _propertyTreeUse);
return _newBuilder;
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static Multiplicity.Builder copyExcept(final Multiplicity _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.EXCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public static Multiplicity.Builder copyOnly(final Multiplicity _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.INCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Multiplicity visit(final PropertyVisitor _visitor_) {
_visitor_.visit(this);
_visitor_.visit(new SingleProperty<>(Multiplicity.PropInfo.MIN_OCCURS, this));
_visitor_.visit(new SingleProperty<>(Multiplicity.PropInfo.MAX_OCCURS, this));
return this;
}
public static class Builder<_B >implements Buildable
{
protected final _B _parentBuilder;
protected final Multiplicity _storedValue;
private BigInteger minOccurs;
private int maxOccurs;
public Builder(final _B _parentBuilder, final Multiplicity _other, final boolean _copy) {
this._parentBuilder = _parentBuilder;
if (_other!= null) {
if (_copy) {
_storedValue = null;
this.minOccurs = _other.minOccurs;
this.maxOccurs = _other.maxOccurs;
} else {
_storedValue = _other;
}
} else {
_storedValue = null;
}
}
public Builder(final _B _parentBuilder, final Multiplicity _other, final boolean _copy, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
this._parentBuilder = _parentBuilder;
if (_other!= null) {
if (_copy) {
_storedValue = null;
final PropertyTree minOccursPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("minOccurs"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(minOccursPropertyTree!= null):((minOccursPropertyTree == null)||(!minOccursPropertyTree.isLeaf())))) {
this.minOccurs = _other.minOccurs;
}
final PropertyTree maxOccursPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("maxOccurs"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(maxOccursPropertyTree!= null):((maxOccursPropertyTree == null)||(!maxOccursPropertyTree.isLeaf())))) {
this.maxOccurs = _other.maxOccurs;
}
} else {
_storedValue = _other;
}
} else {
_storedValue = null;
}
}
public _B end() {
return this._parentBuilder;
}
protected<_P extends Multiplicity >_P init(final _P _product) {
_product.minOccurs = this.minOccurs;
_product.maxOccurs = this.maxOccurs;
return _product;
}
/**
* Sets the new value of "minOccurs" (any previous value will be replaced)
*
* @param minOccurs
* New value of the "minOccurs" property.
*/
public Multiplicity.Builder<_B> withMinOccurs(final BigInteger minOccurs) {
this.minOccurs = minOccurs;
return this;
}
/**
* Sets the new value of "maxOccurs" (any previous value will be replaced)
*
* @param maxOccurs
* New value of the "maxOccurs" property.
*/
public Multiplicity.Builder<_B> withMaxOccurs(final int maxOccurs) {
this.maxOccurs = maxOccurs;
return this;
}
@Override
public Multiplicity build() {
if (_storedValue == null) {
return this.init(new Multiplicity());
} else {
return ((Multiplicity) _storedValue);
}
}
public Multiplicity.Builder<_B> copyOf(final Multiplicity _other) {
_other.copyTo(this);
return this;
}
public Multiplicity.Builder<_B> copyOf(final Multiplicity.Builder _other) {
return copyOf(_other.build());
}
}
public class Modifier {
public void setMinOccurs(final BigInteger minOccurs) {
Multiplicity.this.setMinOccurs(minOccurs);
}
public void setMaxOccurs(final int maxOccurs) {
Multiplicity.this.setMaxOccurs(maxOccurs);
}
}
public static class PropInfo {
public static final transient SinglePropertyInfo MIN_OCCURS = new SinglePropertyInfo("minOccurs", Multiplicity.class, BigInteger.class, false, null, new QName("", "minOccurs"), new QName("http://www.w3.org/2001/XMLSchema", "nonNegativeInteger"), false) {
@Override
public BigInteger get(final Multiplicity _instance_) {
return ((_instance_ == null)?null:_instance_.minOccurs);
}
@Override
public void set(final Multiplicity _instance_, final BigInteger _value_) {
if (_instance_!= null) {
_instance_.minOccurs = _value_;
}
}
}
;
public static final transient SinglePropertyInfo MAX_OCCURS = new SinglePropertyInfo("maxOccurs", Multiplicity.class, Integer.class, false, null, new QName("", "maxOccurs"), new QName("http://www.w3.org/2001/XMLSchema", "int"), false) {
@Override
public Integer get(final Multiplicity _instance_) {
return ((_instance_ == null)?null:_instance_.maxOccurs);
}
@Override
public void set(final Multiplicity _instance_, final Integer _value_) {
if (_instance_!= null) {
_instance_.maxOccurs = _value_;
}
}
}
;
}
public static class Select
extends Multiplicity.Selector
{
Select() {
super(null, null, null);
}
public static Multiplicity.Select _root() {
return new Multiplicity.Select();
}
}
public static class Selector , TParent >
extends com.kscs.util.jaxb.Selector
{
private com.kscs.util.jaxb.Selector> minOccurs = null;
public Selector(final TRoot root, final TParent parent, final String propertyName) {
super(root, parent, propertyName);
}
@Override
public Map buildChildren() {
final Map products = new HashMap<>();
products.putAll(super.buildChildren());
if (this.minOccurs!= null) {
products.put("minOccurs", this.minOccurs.init());
}
return products;
}
public com.kscs.util.jaxb.Selector> minOccurs() {
return ((this.minOccurs == null)?this.minOccurs = new com.kscs.util.jaxb.Selector<>(this._root, this, "minOccurs"):this.minOccurs);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy