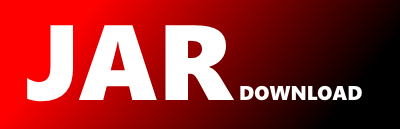
org.javastro.ivoa.entities.vodml.Role Maven / Gradle / Ivy
package org.javastro.ivoa.entities.vodml;
import java.util.HashMap;
import java.util.Map;
import javax.xml.namespace.QName;
import com.kscs.util.jaxb.Buildable;
import com.kscs.util.jaxb.Copyable;
import com.kscs.util.jaxb.PartialCopyable;
import com.kscs.util.jaxb.PropertyTree;
import com.kscs.util.jaxb.PropertyTreeUse;
import com.kscs.util.jaxb.PropertyVisitor;
import com.kscs.util.jaxb.SingleProperty;
import com.kscs.util.jaxb.SinglePropertyInfo;
import com.kscs.util.jaxb.SinglePropertyInfo;
import com.kscs.util.jaxb.SinglePropertyInfo;
import jakarta.annotation.Generated;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlSeeAlso;
import jakarta.xml.bind.annotation.XmlType;
import org.jvnet.jaxb.lang.JAXBMergeStrategy;
import org.jvnet.jaxb.lang.JAXBToStringStrategy;
import org.jvnet.jaxb.lang.MergeFrom;
import org.jvnet.jaxb.lang.MergeStrategy;
import org.jvnet.jaxb.lang.ToString;
import org.jvnet.jaxb.lang.ToStringStrategy;
import org.jvnet.jaxb.locator.ObjectLocator;
import org.jvnet.jaxb.locator.util.LocatorUtils;
/**
* Role extends ReferencableElement.
* The 'name' element that is inherited from that type must be unique in the collection of roles
* defined on the parent type.
* This uniqueness must extend over the roles available on the type by
* inheritance.
*
* Java class for Role complex type
.
*
* The following schema fragment specifies the expected content contained within this class.
*
* {@code
*
*
*
*
*
*
*
*
*
*
* }
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "Role", propOrder = {
"datatype",
"multiplicity"
})
@XmlSeeAlso({
Attribute.class,
Relation.class
})
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public abstract class Role
extends ReferableElement
implements Cloneable, Copyable, PartialCopyable, MergeFrom, ToString
{
/**
* Reference to the type that plays the role represented by this Role.
*
*/
@XmlElement(required = true)
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
protected ElementRef datatype;
/**
* The multiplicity of the role (also called cardinality) indicates whether it must have a
* value or may be without value, or possibly how many values are allowed.
*
*/
@XmlElement(required = true)
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
protected Multiplicity multiplicity;
/**
* Default no-arg constructor
*
*/
public Role() {
super();
}
/**
* Fully-initialising value constructor
*
*/
public Role(final String vodmlId, final String name, final String description, final String id, final ElementRef datatype, final Multiplicity multiplicity) {
super(vodmlId, name, description, id);
this.datatype = datatype;
this.multiplicity = multiplicity;
}
/**
* Reference to the type that plays the role represented by this Role.
*
* @return
* possible object is
* {@link ElementRef }
*
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public ElementRef getDatatype() {
return datatype;
}
/**
* Sets the value of the datatype property.
*
* @param value
* allowed object is
* {@link ElementRef }
*
* @see #getDatatype()
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void setDatatype(ElementRef value) {
this.datatype = value;
}
/**
* The multiplicity of the role (also called cardinality) indicates whether it must have a
* value or may be without value, or possibly how many values are allowed.
*
* @return
* possible object is
* {@link Multiplicity }
*
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Multiplicity getMultiplicity() {
return multiplicity;
}
/**
* Sets the value of the multiplicity property.
*
* @param value
* allowed object is
* {@link Multiplicity }
*
* @see #getMultiplicity()
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void setMultiplicity(Multiplicity value) {
this.multiplicity = value;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public boolean equals(Object object) {
if ((object == null)||(this.getClass()!= object.getClass())) {
return false;
}
if (this == object) {
return true;
}
if (!super.equals(object)) {
return false;
}
final Role that = ((Role) object);
{
ElementRef leftDatatype;
leftDatatype = this.getDatatype();
ElementRef rightDatatype;
rightDatatype = that.getDatatype();
if (this.datatype!= null) {
if (that.datatype!= null) {
if (!leftDatatype.equals(rightDatatype)) {
return false;
}
} else {
return false;
}
} else {
if (that.datatype!= null) {
return false;
}
}
}
{
Multiplicity leftMultiplicity;
leftMultiplicity = this.getMultiplicity();
Multiplicity rightMultiplicity;
rightMultiplicity = that.getMultiplicity();
if (this.multiplicity!= null) {
if (that.multiplicity!= null) {
if (!leftMultiplicity.equals(rightMultiplicity)) {
return false;
}
} else {
return false;
}
} else {
if (that.multiplicity!= null) {
return false;
}
}
}
return true;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public int hashCode() {
int currentHashCode = 1;
currentHashCode = ((currentHashCode* 31)+ super.hashCode());
{
currentHashCode = (currentHashCode* 31);
ElementRef theDatatype;
theDatatype = this.getDatatype();
if (this.datatype!= null) {
currentHashCode += theDatatype.hashCode();
}
}
{
currentHashCode = (currentHashCode* 31);
Multiplicity theMultiplicity;
theMultiplicity = this.getMultiplicity();
if (this.multiplicity!= null) {
currentHashCode += theMultiplicity.hashCode();
}
}
return currentHashCode;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public String toString() {
final ToStringStrategy strategy = JAXBToStringStrategy.getInstance();
final StringBuilder buffer = new StringBuilder();
append(null, buffer, strategy);
return buffer.toString();
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public StringBuilder append(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
strategy.appendStart(locator, this, buffer);
appendFields(locator, buffer, strategy);
strategy.appendEnd(locator, this, buffer);
return buffer;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public StringBuilder appendFields(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
super.appendFields(locator, buffer, strategy);
{
ElementRef theDatatype;
theDatatype = this.getDatatype();
strategy.appendField(locator, this, "datatype", buffer, theDatatype, (this.datatype!= null));
}
{
Multiplicity theMultiplicity;
theMultiplicity = this.getMultiplicity();
strategy.appendField(locator, this, "multiplicity", buffer, theMultiplicity, (this.multiplicity!= null));
}
return buffer;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void mergeFrom(Object left, Object right) {
final MergeStrategy strategy = JAXBMergeStrategy.getInstance();
mergeFrom(null, null, left, right, strategy);
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public void mergeFrom(ObjectLocator leftLocator, ObjectLocator rightLocator, Object left, Object right, MergeStrategy strategy) {
super.mergeFrom(leftLocator, rightLocator, left, right, strategy);
if (right instanceof Role) {
final Role target = this;
final Role leftObject = ((Role) left);
final Role rightObject = ((Role) right);
{
Boolean datatypeShouldBeMergedAndSet = strategy.shouldBeMergedAndSet(leftLocator, rightLocator, (leftObject.datatype!= null), (rightObject.datatype!= null));
if (datatypeShouldBeMergedAndSet == Boolean.TRUE) {
ElementRef lhsDatatype;
lhsDatatype = leftObject.getDatatype();
ElementRef rhsDatatype;
rhsDatatype = rightObject.getDatatype();
ElementRef mergedDatatype = ((ElementRef) strategy.merge(LocatorUtils.property(leftLocator, "datatype", lhsDatatype), LocatorUtils.property(rightLocator, "datatype", rhsDatatype), lhsDatatype, rhsDatatype, (leftObject.datatype!= null), (rightObject.datatype!= null)));
target.setDatatype(mergedDatatype);
} else {
if (datatypeShouldBeMergedAndSet == Boolean.FALSE) {
target.datatype = null;
}
}
}
{
Boolean multiplicityShouldBeMergedAndSet = strategy.shouldBeMergedAndSet(leftLocator, rightLocator, (leftObject.multiplicity!= null), (rightObject.multiplicity!= null));
if (multiplicityShouldBeMergedAndSet == Boolean.TRUE) {
Multiplicity lhsMultiplicity;
lhsMultiplicity = leftObject.getMultiplicity();
Multiplicity rhsMultiplicity;
rhsMultiplicity = rightObject.getMultiplicity();
Multiplicity mergedMultiplicity = ((Multiplicity) strategy.merge(LocatorUtils.property(leftLocator, "multiplicity", lhsMultiplicity), LocatorUtils.property(rightLocator, "multiplicity", rhsMultiplicity), lhsMultiplicity, rhsMultiplicity, (leftObject.multiplicity!= null), (rightObject.multiplicity!= null)));
target.setMultiplicity(mergedMultiplicity);
} else {
if (multiplicityShouldBeMergedAndSet == Boolean.FALSE) {
target.multiplicity = null;
}
}
}
}
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Role clone() {
final Role _newObject;
_newObject = ((Role) super.clone());
_newObject.datatype = ((this.datatype == null)?null:this.datatype.clone());
_newObject.multiplicity = ((this.multiplicity == null)?null:this.multiplicity.clone());
return _newObject;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Role createCopy() {
final Role _newObject = ((Role) super.createCopy());
_newObject.datatype = ((this.datatype == null)?null:this.datatype.createCopy());
_newObject.multiplicity = ((this.multiplicity == null)?null:this.multiplicity.createCopy());
return _newObject;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Role createCopy(final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
final Role _newObject = ((Role) super.createCopy(_propertyTree, _propertyTreeUse));
final PropertyTree datatypePropertyTree = ((_propertyTree == null)?null:_propertyTree.get("datatype"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(datatypePropertyTree!= null):((datatypePropertyTree == null)||(!datatypePropertyTree.isLeaf())))) {
_newObject.datatype = ((this.datatype == null)?null:this.datatype.createCopy(datatypePropertyTree, _propertyTreeUse));
}
final PropertyTree multiplicityPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("multiplicity"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(multiplicityPropertyTree!= null):((multiplicityPropertyTree == null)||(!multiplicityPropertyTree.isLeaf())))) {
_newObject.multiplicity = ((this.multiplicity == null)?null:this.multiplicity.createCopy(multiplicityPropertyTree, _propertyTreeUse));
}
return _newObject;
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Role copyExcept(final PropertyTree _propertyTree) {
return createCopy(_propertyTree, PropertyTreeUse.EXCLUDE);
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Role copyOnly(final PropertyTree _propertyTree) {
return createCopy(_propertyTree, PropertyTreeUse.INCLUDE);
}
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Role.Modifier modifier() {
if (null == this.__cachedModifier__) {
this.__cachedModifier__ = new Role.Modifier();
}
return ((Role.Modifier) this.__cachedModifier__);
}
/**
* Copies all state of this object to a builder. This method is used by the copyOf
* method and should not be called directly by client code.
*
* @param _other
* A builder instance to which the state of this object will be copied.
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public<_B >void copyTo(final Role.Builder<_B> _other) {
super.copyTo(_other);
_other.datatype = ((this.datatype == null)?null:this.datatype.newCopyBuilder(_other));
_other.multiplicity = ((this.multiplicity == null)?null:this.multiplicity.newCopyBuilder(_other));
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public abstract<_B >Role.Builder<_B> newCopyBuilder(final _B _parentBuilder);
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public abstract Role.Builder newCopyBuilder();
/**
* Copies all state of this object to a builder. This method is used by the copyOf
* method and should not be called directly by client code.
*
* @param _other
* A builder instance to which the state of this object will be copied.
*/
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public<_B >void copyTo(final Role.Builder<_B> _other, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
super.copyTo(_other, _propertyTree, _propertyTreeUse);
final PropertyTree datatypePropertyTree = ((_propertyTree == null)?null:_propertyTree.get("datatype"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(datatypePropertyTree!= null):((datatypePropertyTree == null)||(!datatypePropertyTree.isLeaf())))) {
_other.datatype = ((this.datatype == null)?null:this.datatype.newCopyBuilder(_other, datatypePropertyTree, _propertyTreeUse));
}
final PropertyTree multiplicityPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("multiplicity"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(multiplicityPropertyTree!= null):((multiplicityPropertyTree == null)||(!multiplicityPropertyTree.isLeaf())))) {
_other.multiplicity = ((this.multiplicity == null)?null:this.multiplicity.newCopyBuilder(_other, multiplicityPropertyTree, _propertyTreeUse));
}
}
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public abstract<_B >Role.Builder<_B> newCopyBuilder(final _B _parentBuilder, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse);
@Override
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public abstract Role.Builder newCopyBuilder(final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse);
@Generated(value = "com.sun.tools.xjc.Driver", comments = "JAXB RI v4.0.4", date = "2024-10-20T18:15:02+01:00")
public Role visit(final PropertyVisitor _visitor_) {
super.visit(_visitor_);
if (_visitor_.visit(new SingleProperty<>(Role.PropInfo.DATATYPE, this))&&(this.datatype!= null)) {
this.datatype.visit(_visitor_);
}
if (_visitor_.visit(new SingleProperty<>(Role.PropInfo.MULTIPLICITY, this))&&(this.multiplicity!= null)) {
this.multiplicity.visit(_visitor_);
}
return this;
}
public static class Builder<_B >
extends ReferableElement.Builder<_B>
implements Buildable
{
private ElementRef.Builder> datatype;
private Multiplicity.Builder> multiplicity;
public Builder(final _B _parentBuilder, final Role _other, final boolean _copy) {
super(_parentBuilder, _other, _copy);
if (_other!= null) {
this.datatype = ((_other.datatype == null)?null:_other.datatype.newCopyBuilder(this));
this.multiplicity = ((_other.multiplicity == null)?null:_other.multiplicity.newCopyBuilder(this));
}
}
public Builder(final _B _parentBuilder, final Role _other, final boolean _copy, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
super(_parentBuilder, _other, _copy, _propertyTree, _propertyTreeUse);
if (_other!= null) {
final PropertyTree datatypePropertyTree = ((_propertyTree == null)?null:_propertyTree.get("datatype"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(datatypePropertyTree!= null):((datatypePropertyTree == null)||(!datatypePropertyTree.isLeaf())))) {
this.datatype = ((_other.datatype == null)?null:_other.datatype.newCopyBuilder(this, datatypePropertyTree, _propertyTreeUse));
}
final PropertyTree multiplicityPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("multiplicity"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(multiplicityPropertyTree!= null):((multiplicityPropertyTree == null)||(!multiplicityPropertyTree.isLeaf())))) {
this.multiplicity = ((_other.multiplicity == null)?null:_other.multiplicity.newCopyBuilder(this, multiplicityPropertyTree, _propertyTreeUse));
}
}
}
protected<_P extends Role >_P init(final _P _product) {
_product.datatype = ((this.datatype == null)?null:this.datatype.build());
_product.multiplicity = ((this.multiplicity == null)?null:this.multiplicity.build());
return super.init(_product);
}
/**
* Sets the new value of "datatype" (any previous value will be replaced)
*
* @param datatype
* New value of the "datatype" property.
*/
public Role.Builder<_B> withDatatype(final ElementRef datatype) {
this.datatype = ((datatype == null)?null:new ElementRef.Builder<>(this, datatype, false));
return this;
}
/**
* Returns the existing builder or a new builder to build the value of the
* "datatype" property.
* Use {@link org.javastro.ivoa.entities.vodml.ElementRef.Builder#end()} to return
* to the current builder.
*
* @return
* A new builder to build the value of the "datatype" property.
* Use {@link org.javastro.ivoa.entities.vodml.ElementRef.Builder#end()} to return
* to the current builder.
*/
public ElementRef.Builder extends Role.Builder<_B>> withDatatype() {
if (this.datatype!= null) {
return this.datatype;
}
return this.datatype = new ElementRef.Builder<>(this, null, false);
}
/**
* Sets the new value of "multiplicity" (any previous value will be replaced)
*
* @param multiplicity
* New value of the "multiplicity" property.
*/
public Role.Builder<_B> withMultiplicity(final Multiplicity multiplicity) {
this.multiplicity = ((multiplicity == null)?null:new Multiplicity.Builder<>(this, multiplicity, false));
return this;
}
/**
* Returns the existing builder or a new builder to build the value of the
* "multiplicity" property.
* Use {@link org.javastro.ivoa.entities.vodml.Multiplicity.Builder#end()} to
* return to the current builder.
*
* @return
* A new builder to build the value of the "multiplicity" property.
* Use {@link org.javastro.ivoa.entities.vodml.Multiplicity.Builder#end()} to
* return to the current builder.
*/
public Multiplicity.Builder extends Role.Builder<_B>> withMultiplicity() {
if (this.multiplicity!= null) {
return this.multiplicity;
}
return this.multiplicity = new Multiplicity.Builder<>(this, null, false);
}
/**
* Sets the new value of "vodmlId" (any previous value will be replaced)
*
* @param vodmlId
* New value of the "vodmlId" property.
*/
@Override
public Role.Builder<_B> withVodmlId(final String vodmlId) {
super.withVodmlId(vodmlId);
return this;
}
/**
* Sets the new value of "name" (any previous value will be replaced)
*
* @param name
* New value of the "name" property.
*/
@Override
public Role.Builder<_B> withName(final String name) {
super.withName(name);
return this;
}
/**
* Sets the new value of "description" (any previous value will be replaced)
*
* @param description
* New value of the "description" property.
*/
@Override
public Role.Builder<_B> withDescription(final String description) {
super.withDescription(description);
return this;
}
/**
* Sets the new value of "id" (any previous value will be replaced)
*
* @param id
* New value of the "id" property.
*/
@Override
public Role.Builder<_B> withId(final String id) {
super.withId(id);
return this;
}
@Override
public Role build() {
return ((Role) _storedValue);
}
public Role.Builder<_B> copyOf(final Role _other) {
_other.copyTo(this);
return this;
}
public Role.Builder<_B> copyOf(final Role.Builder _other) {
return copyOf(_other.build());
}
}
public class Modifier
extends ReferableElement.Modifier
{
public void setDatatype(final ElementRef datatype) {
Role.this.setDatatype(datatype);
}
public void setMultiplicity(final Multiplicity multiplicity) {
Role.this.setMultiplicity(multiplicity);
}
}
public static class PropInfo {
public static final transient SinglePropertyInfo DATATYPE = new SinglePropertyInfo("datatype", Role.class, ElementRef.class, false, null, new QName("", "datatype"), new QName("http://www.ivoa.net/xml/VODML/v1", "ElementRef"), false) {
@Override
public ElementRef get(final Role _instance_) {
return ((_instance_ == null)?null:_instance_.datatype);
}
@Override
public void set(final Role _instance_, final ElementRef _value_) {
if (_instance_!= null) {
_instance_.datatype = _value_;
}
}
}
;
public static final transient SinglePropertyInfo MULTIPLICITY = new SinglePropertyInfo("multiplicity", Role.class, Multiplicity.class, false, null, new QName("", "multiplicity"), new QName("http://www.ivoa.net/xml/VODML/v1", "Multiplicity"), false) {
@Override
public Multiplicity get(final Role _instance_) {
return ((_instance_ == null)?null:_instance_.multiplicity);
}
@Override
public void set(final Role _instance_, final Multiplicity _value_) {
if (_instance_!= null) {
_instance_.multiplicity = _value_;
}
}
}
;
}
public static class Select
extends Role.Selector
{
Select() {
super(null, null, null);
}
public static Role.Select _root() {
return new Role.Select();
}
}
public static class Selector , TParent >
extends ReferableElement.Selector
{
private ElementRef.Selector> datatype = null;
private Multiplicity.Selector> multiplicity = null;
public Selector(final TRoot root, final TParent parent, final String propertyName) {
super(root, parent, propertyName);
}
@Override
public Map buildChildren() {
final Map products = new HashMap<>();
products.putAll(super.buildChildren());
if (this.datatype!= null) {
products.put("datatype", this.datatype.init());
}
if (this.multiplicity!= null) {
products.put("multiplicity", this.multiplicity.init());
}
return products;
}
public ElementRef.Selector> datatype() {
return ((this.datatype == null)?this.datatype = new ElementRef.Selector<>(this._root, this, "datatype"):this.datatype);
}
public Multiplicity.Selector> multiplicity() {
return ((this.multiplicity == null)?this.multiplicity = new Multiplicity.Selector<>(this._root, this, "multiplicity"):this.multiplicity);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy