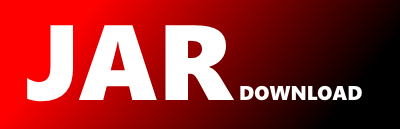
org.jbasics.collection.CollectionUtilities Maven / Gradle / Ivy
Show all versions of jbasics Show documentation
/*
* Copyright (c) 2009-2015
* IT-Consulting Stephan Schloepke (http://www.schloepke.de/)
* klemm software consulting Mirko Klemm (http://www.klemm-scs.com/)
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in
* all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
* THE SOFTWARE.
*/
package org.jbasics.collection;
import org.jbasics.annotation.ImmutableState;
import org.jbasics.annotation.Nullable;
import org.jbasics.annotation.ThreadSafe;
import org.jbasics.pattern.delegation.Delegate;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.HashSet;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import java.util.Set;
/**
* Utilities dealing with the Java Collections.
*
* @author Stephan Schloepke
* @since 1.0
*/
@ThreadSafe
@ImmutableState
public final class CollectionUtilities {
/**
* Creates an unmodifiable shallow copy of the given original {@link Map}. While the copy returns an immutable
* copy of the {@link Map} the content is not cloned in any way. Unless the content is immutable by itself the
* result is not fully immutable. In the shallow copy all references are the same as in the original {@link Map}!
*
*
* @param original The {@link Map} to copy the elements fro.
* @param The type of the key
* @param The type of the value
*
* @return Returns an immutable (unmodifiable) copy of the original {@link Map} with all the elements added but not
* cloned!
*/
public static Map createUnmodifiableShallowCopy(final Map original) {
if (original == null || original.isEmpty()) {
return Collections.emptyMap();
} else {
return Collections.unmodifiableMap(new HashMap(original));
}
}
/**
* Creates an unmodifiable shallow copy of the given original {@link Set}. While the copy returns an immutable
* copy of the {@link Set} the content is not cloned in any way. Unless the content is immutable by itself the
* result is not fully immutable. In the shallow copy all references are the same as in the original {@link Set}!
*
*
* @param original The {@link Set} to copy the elements fro.
* @param The type of the elements
*
* @return Returns an immutable (unmodifiable) copy of the original {@link Set} with all the elements added but not
* cloned!
*/
public static Set createUnmodifiableShallowCopy(final Set original) {
if (original == null || original.isEmpty()) {
return Collections.emptySet();
} else {
return Collections.unmodifiableSet(new HashSet(original));
}
}
/**
* Creates an unmodifiable shallow copy of the given original {@link List}. While the copy returns an immutable
* copy of the {@link List} the content is not cloned in any way. Unless the content is immutable by itself the
* result is not fully immutable. In the shallow copy all references are the same as in the original {@link List}!
*
*
* @param original The {@link List} to copy the elements fro.
* @param The type of the elements
*
* @return Returns an immutable (unmodifiable) copy of the original {@link List} with all the elements added but not
* cloned!
*/
public static List createUnmodifiableShallowCopy(final List original) {
if (original == null || original.isEmpty()) {
return Collections.emptyList();
} else {
return Collections.unmodifiableList(new ArrayList(original));
}
}
/**
* Dereferences the {@link Map} in the {@link Delegate} or returns an empty {@link Map} if either the given {@link
* Delegate} or delegated {@link Map} is null
. The returned {@link Map} is unmodifiable.
*
* @param The type of the {@link Map} key
* @param The type of the {@link Map} value
* @param delegate The {@link Delegate} to the {@link Map}. (Can be null
)
*
* @return An unmodifiable {@link Map} either from the {@link Delegate} or an empty one.
*/
public static Map dereferenceUnmodifiableMapDelegate(Delegate