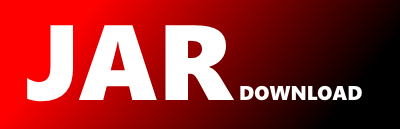
org.jbasics.math.BigDecimalMathLibrary Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jbasics Show documentation
Show all versions of jbasics Show documentation
jBasics is a collection of useful utility classes for Java. This includes helper for XML, mathematic functions,
restful web services helper, pattern oriented programming interfaces and more. Currently Java7 and up is
supported. Version 1.0 will required at leaset Java8.
/*
* Copyright (c) 2009-2015
* IT-Consulting Stephan Schloepke (http://www.schloepke.de/)
* klemm software consulting Mirko Klemm (http://www.klemm-scs.com/)
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in
* all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
* THE SOFTWARE.
*/
package org.jbasics.math;
import org.jbasics.checker.ContractCheck;
import org.jbasics.math.impl.*;
import java.math.BigDecimal;
import java.math.MathContext;
/**
* Static class holding arbitrary correct decimal functions based on BigDecimal and BigInteger.
*
* @author Stephan Schloepke
* @since 1.0
*/
public final class BigDecimalMathLibrary {
/**
* The constant value of -2 as BigDecimal.
*/
public final static BigDecimal CONSTANT_MINUS_TWO = new BigDecimal("-2");
/**
* The constant value of -1 as BigDecimal.
*/
public final static BigDecimal CONSTANT_MINUS_ONE = new BigDecimal("-1");
/**
* The constant value of -1/2 as BigDecimal.
*/
public final static BigDecimal CONSTANT_MINUS_HALF = new BigDecimal("-0.5");
/**
* The constant value of 0 as BigDecimal directly using {@link BigDecimal#ZERO}.
*/
public final static BigDecimal CONSTANT_ZERO = BigDecimal.ZERO;
/**
* The constant value of 1/4 as BigDecimal.
*/
public final static BigDecimal CONSTANT_QUARTER = new BigDecimal("0.25");
/**
* The constant value of 1/2 as BigDecimal.
*/
public final static BigDecimal CONSTANT_HALF = new BigDecimal("0.5");
/**
* The constant value of 1 as BigDecimal directly using {@link BigDecimal#ONE}.
*/
public final static BigDecimal CONSTANT_ONE = BigDecimal.ONE;
/**
* The constant value of 2 as BigDecimal.
*/
public final static BigDecimal CONSTANT_TWO = new BigDecimal("2");
/**
* The constant value of 2 as BigDecimal.
*/
public final static BigDecimal CONSTANT_THREE = new BigDecimal("3");
/**
* The constant value of 10 as BigDecimal directly using {@link BigDecimal#TEN}.
*/
public final static BigDecimal CONSTANT_TEN = BigDecimal.TEN;
/**
* The constant E as {@link IrationalNumber}.
*
* @since 1.0
*/
public final static IrationalNumber E = ExponentialIrationalNumber.E;
/**
* The constant PI as {@link IrationalNumber}.
*
* @since 1.0
*/
public final static IrationalNumber PI = PiIrationalNumber.PI;
/**
* The constant 2*PI as {@link IrationalNumber}.
*
* @since 1.0
*/
public final static IrationalNumber PI2 = PiIrationalNumber.PI2;
/**
* The constant PHI (Golden Ratio) as {@link IrationalNumber}.
*
* @since 1.0
*/
public final static IrationalNumber PHI = GoldenRatioIrationalNumber.PHI;
/**
* The constant sqrt(2) as {@link IrationalNumber}.
*
* @since 1.0
*/
public final static IrationalNumber SQRT2 = SquareRootIrationalNumber.SQUARE_ROOT_OF_2;
/**
* The constant sqrt(3) as {@link IrationalNumber}.
*
* @since 1.0
*/
public final static IrationalNumber SQRT3 = SquareRootIrationalNumber.SQUARE_ROOT_OF_3;
/**
* The constant sqrt(2*pi) as {@link IrationalNumber}.
*
* @since 1.0
*/
public final static IrationalNumber SQRT_PI2 = SquareRootIrationalNumber.SQUARE_ROOT_OF_PI2;
/**
* The constant of 1/sqrt(2) or sqrt(1/2) as {@link IrationalNumber}.
*
* @since 1.0
*/
public final static IrationalNumber SQRT_RECIPROCAL2 = SquareRootReciprocalIrationalNumber.SQUARE_ROOT_RECIPROCAL_OF_2;
/**
* Returns the {@link IrationalNumber} of the calculation e^x. In order to get the result use {@link
* IrationalNumber#valueToPrecision(MathContext)}.
*
* @param x The value for x in e^x.
*
* @return The result of e^x as {@link IrationalNumber}.
*
* @since 1.0
*/
public static IrationalNumber exp(final BigDecimal x) {
return ExponentialIrationalNumber.valueOf(x);
}
/**
* Returns x*pi as {@link IrationalNumber}. If x is one or two the constant {@link #PI} or {@link #PI2} is
* returned.
*
* @param x The factor for PI.
*
* @return The irational number of x*PI.
*
* @since 1.0
*/
public static IrationalNumber piMultiple(final BigDecimal x) {
return PiIrationalNumber.valueOf(x);
}
/**
* Returns x*PHI (the golden ratio) as {@link IrationalNumber}. If x is one the constant {@link #PHI} returned.
*
* @param x The factor for PI.
*
* @return The irational number of x*PHI.
*
* @since 1.0
*/
public static IrationalNumber goldenRatioMultiple(final BigDecimal x) {
return GoldenRatioIrationalNumber.valueOf(x);
}
/**
* Calculates the arithmetic mean of the two supplied numbers.
*
* @param a0 The first value.
* @param a1 The second value.
*
* @return The arithmetic mean of the two supplied numbers.
*
* @since 1.0
*/
public static BigDecimal arithmeticMean(final BigDecimal a0, final BigDecimal a1) {
return a0.add(a1).divide(MathImplConstants.TWO);
}
/**
* Calculates the geometric mean of the two supplied numbers.
*
* @param a0 The first value.
* @param a1 The second value.
*
* @return The geometric mean of the supplied numbers.
*
* @since 1.0
*/
public static IrationalNumber geometricMean(final BigDecimal a0, final BigDecimal a1, final MathContext mc) {
return BigDecimalMathLibrary.sqrt(ContractCheck.mustNotBeNull(a0, "a0").multiply(ContractCheck.mustNotBeNull(a1, "a1")));
}
/**
* Returns the {@link IrationalNumber} which is the square root of x.
*
* @param x The value of which to calculate the square root.
*
* @return The square root of the supplied number.
*
* @since 1.0
*/
public static IrationalNumber sqrt(final BigDecimal x) {
return SquareRootIrationalNumber.valueOf(x);
}
/**
* Calculates the arithmetic geometric mean of the supplied numbers.
*
* @param a The first number.
* @param b The second number.
*
* @return The arithmetic geometric mean of the supplied numbers.
*
* @since 1.0
*/
public static IrationalNumber arithmeticGeometricMean(final BigDecimal a, final BigDecimal b) {
return ArithmeticGeometricMeanIrationalNumber.valueOf(a, b);
}
/**
* Returns the natural logarithm of x (logex)
*
* @param x The value to calculate.
*
* @return The natural logarithm logex
*
* @since 1.0
*/
public static IrationalNumber ln(final BigDecimal x) {
return LogNaturalFunctionIrationalNumber.valueOf(x);
}
/**
* Returns the logarithm of x to base 10 (log10x)
*
* @param x The value to calculate.
*
* @return The decimal logarithm log10x
*
* @since 1.0
*/
public static IrationalNumber lg(final BigDecimal x) {
return LogIrationalNumber.valueOf(x, BigDecimal.TEN);
}
/**
* Returns the logarithm of x to base 2 (log2x)
*
* @param x The value to calculate.
*
* @return The dual or binary logarithm log2x
*
* @since 1.0
*/
public static IrationalNumber ld(final BigDecimal x) {
return LogIrationalNumber.valueOf(x, MathImplConstants.TWO);
}
/**
* Returns the logarithm of x to base b (logbx)
*
* @param x The value to calculate.
* @param b The logarithm base
*
* @return The result of logbx
*
* @since 1.0
*/
public static IrationalNumber log(final BigDecimal x, final BigDecimal b) {
return LogIrationalNumber.valueOf(x, b);
}
/**
* Calculates the power of x on a. The calculation is processed by using the natural logarithm and the e function
* (f(a,x) = a^x = x * e^ln(a)).
*
* @param a The base
* @param x The exponent
*
* @return a.pow(x) or a^x.
*
* @since 1.0
*/
public static IrationalNumber pow(final BigDecimal a, final BigDecimal x) {
return PowIrationalNumber.valueOf(a, x);
}
/**
* Returns the sine of x as {@link IrationalNumber}.
*
* @param x The value to calculate the sine for
*
* @return The sine of x
*
* @since 1.0
*/
public static IrationalNumber sin(final BigDecimal x) {
return SineIrationalNumber.valueOf(x);
}
/**
* Returns the cosine of x as {@link IrationalNumber}.
*
* @param x The value to calculate the cosine for
*
* @return The cosine of x
*
* @since 1.0
*/
public static IrationalNumber cos(final BigDecimal x) {
return CosineIrationalNumber.valueOf(x);
}
/**
* Returns the tangent of x as {@link IrationalNumber}.
*
* @param x The value to calculate the tangent for
*
* @return The tangent of x
*
* @since 1.0
*/
public static IrationalNumber tan(final BigDecimal x) {
return TangentIrationalNumber.valueOf(x);
}
/**
* Returns the arc sine of x as {@link IrationalNumber}.
*
* @param x The value to calculate the arc sine for
*
* @return The arc sine of x
*
* @since 1.0
*/
public static IrationalNumber asin(final BigDecimal x) {
return ArcSineIrationalNumber.valueOf(x);
}
/**
* Returns the arc cosine of x as {@link IrationalNumber}.
*
* @param x The value to calculate the arc cosine for
*
* @return The arc cosine of x
*
* @since 1.0
*/
public static IrationalNumber acos(final BigDecimal x) {
return ArcCosineIrationalNumber.valueOf(x);
}
/**
* Returns the arc tangent of x as {@link IrationalNumber}.
*
* @param x The value to calculate the arc tangent for
*
* @return The arc tangent of x
*
* @since 1.0
*/
public static IrationalNumber atan(final BigDecimal x) {
return ArcTangentIrationalNumber.valueOf(x);
}
/**
* Returns the hyperbolic sine of x as {@link IrationalNumber}.
*
* @param x The value to calculate the hyperbolic sine for
*
* @return The hyperbolic sine of x
*
* @since 1.0
*/
public static IrationalNumber sinh(final BigDecimal x) {
return HyperbolicSineIrationalNumber.valueOf(x);
}
/**
* Returns the hyperbolic cosine of x as {@link IrationalNumber}.
*
* @param x The value to calculate the hyperbolic cosine for
*
* @return The hyperbolic cosine of x
*
* @since 1.0
*/
public static IrationalNumber cosh(final BigDecimal x) {
return HyperbolicCosineIrationalNumber.valueOf(x);
}
/**
* Returns the hyperbolic tangent of x as {@link IrationalNumber}.
*
* @param x The value to calculate the hyperbolic tangent for
*
* @return The hyperbolic tangent of x
*
* @since 1.0
*/
public static IrationalNumber tanh(final BigDecimal x) {
return HyperbolicTangentIrationalNumber.valueOf(x);
}
/**
* Returns the gamma function of x
*
* @param x The value to calculate gamma(x)
*
* @return The gamma value of x
*/
public static IrationalNumber gamma(final BigDecimal x) {
return GammaFunctionIrationalNumber.valueOf(x);
}
/**
* Returns the incomplete gamma function.
*
* @param x
* @param alpha
*
* @return
*
* @deprecated This is going very soon since the name is incorrect
*/
@Deprecated
public static IrationalNumber gammaIncomplete(final BigDecimal x, final BigDecimal alpha) {
return GammaIncompleteIrationalNumber.valueOf(x, alpha);
}
/**
* Returns the ln(gamma) function value of x.
*
* @param x The value to calculate ln(gamma(x))
*
* @return The ln(gamma(x)) value of x
*/
public static IrationalNumber lnGamma(final BigDecimal x) {
return GammaLnFunctionIrationalNumber.valueOf(x);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy