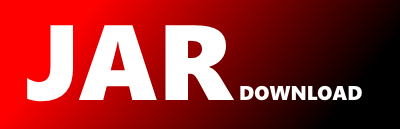
org.jbasics.net.http.HTTPHeaderConstants Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jbasics Show documentation
Show all versions of jbasics Show documentation
jBasics is a collection of useful utility classes for Java. This includes helper for XML, mathematic functions,
restful web services helper, pattern oriented programming interfaces and more. Currently Java7 and up is
supported. Version 1.0 will required at leaset Java8.
/*
* Copyright (c) 2009-2015
* IT-Consulting Stephan Schloepke (http://www.schloepke.de/)
* klemm software consulting Mirko Klemm (http://www.klemm-scs.com/)
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in
* all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
* THE SOFTWARE.
*/
package org.jbasics.net.http;
/**
* Class holding the names of the HTTP/1.1 defined header names. A deeper specification you can find at RFC2616 Section 14.
*
* @author Stephan Schloepke
* @since 1.0
*/
public final class HTTPHeaderConstants {
/**
* Accept header (see RFC Section
* 14.1).
*/
public static final String ACCEPT_HEADER = "Accept"; //$NON-NLS-1$
/**
* Accept charset header (see RFC Section
* 14.2).
*/
public static final String ACCEPT_CHARSET_HEADER = "Accept-Charset"; //$NON-NLS-1$
/**
* Accept encoding header (see RFC Section
* 14.3).
*/
public static final String ACCEPT_ENCODING_HEADER = "Accept-Encoding"; //$NON-NLS-1$
/**
* Accept language header (see RFC Section
* 14.4).
*/
public static final String ACCEPT_LANGUAGE_HEADER = "Accept-Language"; //$NON-NLS-1$
/**
* Accept ranges header (see RFC Section
* 14.5).
*/
public static final String ACCEPT_RANGES_HEADER = "Accept-Ranges"; //$NON-NLS-1$
/**
* Age header (see RFC Section 14.6).
*/
public static final String AGE_HEADER = "Age"; //$NON-NLS-1$
/**
* Allow header (see RFC Section
* 14.7).
*/
public static final String ALLOW_HEADER = "Allow"; //$NON-NLS-1$
/**
* Authorization header (see RFC Section
* 14.8).
*/
public static final String AUTHORIZATION_HEADER = "Authorization"; //$NON-NLS-1$
/**
* Cache control header (see RFC Section
* 14.9).
*/
public static final String CACHE_CONTROL_HEADER = "Cache-Control"; //$NON-NLS-1$
/**
* Connection header (see RFC Section
* 14.10).
*/
public static final String CONNECTION_HEADER = "Connection"; //$NON-NLS-1$
/**
* Content encoding header (see RFC
* Section 14.11).
*/
public static final String CONTENT_ENCODING_HEADER = "Content-Encoding"; //$NON-NLS-1$
/**
* Content language header (see RFC
* Section 14.12).
*/
public static final String CONTENT_LANGUAGE_HEADER = "Content-Language"; //$NON-NLS-1$
/**
* Content length header (see RFC Section
* 14.13).
*/
public static final String CONTENT_LENGTH_HEADER = "Content-Length"; //$NON-NLS-1$
/**
* Content location header (see RFC
* Section 14.14).
*/
public static final String CONTENT_LOCATION_HEADER = "Content-Location"; //$NON-NLS-1$
/**
* Content MD5 header (see RFC Section
* 14.15).
*/
public static final String CONTENT_MD5_HEADER = "Content-MD5"; //$NON-NLS-1$
/**
* Content range header (see RFC Section
* 14.16).
*/
public static final String CONTENT_RANGE_HEADER = "Content-Range"; //$NON-NLS-1$
/**
* Content type header (see RFC Section
* 14.17).
*/
public static final String CONTENT_TYPE_HEADER = "Content-Type"; //$NON-NLS-1$
/**
* Date header (see RFC Section
* 14.18).
*/
public static final String DATE_HEADER = "Date"; //$NON-NLS-1$
/**
* ETag header (see RFC Section
* 14.19).
*/
public static final String ETAG_HEADER = "ETag"; //$NON-NLS-1$
/**
* Expect header (see RFC Section
* 14.20).
*/
public static final String EXPECT_HEADER = "Expect"; //$NON-NLS-1$
/**
* Expires header (see RFC Section
* 14.21).
*/
public static final String EXPIRES_HEADER = "Expires"; //$NON-NLS-1$
/**
* AFrom header (see RFC Section
* 14.22).
*/
public static final String FROM_HEADER = "From"; //$NON-NLS-1$
/**
* Host header (see RFC Section
* 14.23).
*/
public static final String HOST_HEADER = "Host"; //$NON-NLS-1$
/**
* If match header (see RFC Section
* 14.24).
*/
public static final String IF_MATCH_HEADER = "If-Match"; //$NON-NLS-1$
/**
* If modified since header (see RFC
* Section 14.25).
*/
public static final String IF_MODIFIED_SINCE_HEADER = "If-Modified-Since"; //$NON-NLS-1$
/**
* If none match header (see RFC Section
* 14.26).
*/
public static final String IF_NONE_MATCH_HEADER = "If-None-Match"; //$NON-NLS-1$
/**
* If rang header (see RFC Section
* 14.27).
*/
public static final String IF_RANGE_HEADER = "If-Range"; //$NON-NLS-1$
/**
* If unmodified since header (see RFC
* Section 14.28).
*/
public static final String IF_UNMODIFIED_SINCE_HEADER = "If-Unmodified-Since"; //$NON-NLS-1$
/**
* Last modified header (see RFC Section
* 14.29).
*/
public static final String LAST_MODIFIED_HEADER = "Last-Modified"; //$NON-NLS-1$
/**
* Location header (see RFC Section
* 14.30).
*/
public static final String LOCATION_HEADER = "Location"; //$NON-NLS-1$
/**
* Accept language header.
*/
public static final String MAX_FORWARDS_HEADER = "Max-Forwards"; //$NON-NLS-1$
/**
* Accept language header.
*/
public static final String PRAGMA_HEADER = "Pragma"; //$NON-NLS-1$
/**
* Accept language header.
*/
public static final String PROXY_AUTHENTICATE_HEADER = "Proxy-Authenticate"; //$NON-NLS-1$
/**
* Accept language header.
*/
public static final String PROXY_AUTHORIZATION_HEADER = "Proxy-Authorization"; //$NON-NLS-1$
/**
* Accept language header.
*/
public static final String RANGE_HEADER = "Range"; //$NON-NLS-1$
/**
* Accept language header.
*/
public static final String REFERER_HEADER = "Referer"; //$NON-NLS-1$
/**
* Accept language header.
*/
public static final String RETRY_AFTER_HEADER = "Retry-After"; //$NON-NLS-1$
/**
* Accept language header.
*/
public static final String SERVER_HEADER = "Server"; //$NON-NLS-1$
/**
* Accept language header.
*/
public static final String TE_HEADER = "TE"; //$NON-NLS-1$
/**
* Accept language header.
*/
public static final String TRAILER_HEADER = "Trailer"; //$NON-NLS-1$
/**
* Accept language header.
*/
public static final String TRANSFER_ENCODING_HEADER = "Transfer-Encoding"; //$NON-NLS-1$
/**
* Accept language header.
*/
public static final String UPGRADE_HEADER = "Upgrade"; //$NON-NLS-1$
/**
* Accept language header.
*/
public static final String USER_AGENT_HEADER = "User-Agent"; //$NON-NLS-1$
/**
* Accept language header.
*/
public static final String VARY_HEADER = "Vary"; //$NON-NLS-1$
/**
* Accept language header.
*/
public static final String VIA_HEADER = "Via"; //$NON-NLS-1$
/**
* Accept language header.
*/
public static final String WARNING_HEADER = "Warning"; //$NON-NLS-1$
/**
* Accept language header.
*/
public static final String WWW_AUTHENTICATE_HEADER = "WWW-Authenticate"; //$NON-NLS-1$
// Most commonly used encoding format constants
/**
* The default encoding if none is specified meaning data goes in as out.
*/
public static final String IDENTITIY_ENCODING = "identity"; //$NON-NLS-1$
/**
* An encoding where the data is compressed with gzip.
*/
public static final String GZIP_ENCODING = "gzip"; //$NON-NLS-1$
/**
* An encoding where the data is compressed using the format of unix compress (adaptive lzw encoding).
*/
public static final String COMPRESS_ENCODING = "compress"; //$NON-NLS-1$
/**
* An encoding where the data is compressed by the zip / defalte method.
*/
public static final String DEFLATE_ENCODING = "deflate"; //$NON-NLS-1$
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy