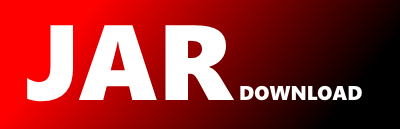
org.jberet.support.io.ExcelUserModelItemReader Maven / Gradle / Ivy
The newest version!
/*
* Copyright (c) 2014 Red Hat, Inc. and/or its affiliates.
*
* This program and the accompanying materials are made
* available under the terms of the Eclipse Public License 2.0
* which is available at https://www.eclipse.org/legal/epl-2.0/
*
* SPDX-License-Identifier: EPL-2.0
*/
package org.jberet.support.io;
import java.io.IOException;
import java.io.InputStream;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.ss.usermodel.CellType;
import org.apache.poi.ss.usermodel.DateUtil;
import org.apache.poi.ss.usermodel.FormulaEvaluator;
import org.apache.poi.ss.usermodel.Row;
import org.apache.poi.ss.usermodel.WorkbookFactory;
import org.jberet.support._private.SupportLogger;
import org.jberet.support._private.SupportMessages;
import jakarta.batch.api.BatchProperty;
import jakarta.batch.api.chunk.ItemReader;
import jakarta.enterprise.context.Dependent;
import jakarta.inject.Inject;
import jakarta.inject.Named;
/**
* An implementation of {@code jakarta.batch.api.chunk.ItemReader} for reading Excel files. Current implementation is
* based on Apache POI user model API.
*
* @see ExcelUserModelItemWriter
* @see ExcelItemReaderWriterBase
* @see ExcelEventItemReader
* @see ExcelStreamingItemReader
* @since 1.1.0
*/
@Named
@Dependent
public class ExcelUserModelItemReader extends ExcelItemReaderWriterBase implements ItemReader {
/**
* A positive integer indicating the start position in the input resource. It is optional and defaults to 0
* (starting from the 1st data item). If a header row is present, the start point should be after the header row.
*/
@Inject
@BatchProperty
protected int start;
/**
* A positive integer indicating the end position in the input resource. It is optional and defaults to
* {@code Integer.MAX_VALUE}.
*/
@Inject
@BatchProperty
protected int end;
/**
* The index (0-based) of the target sheet to read, defaults to 0.
*/
@Inject
@BatchProperty
protected int sheetIndex;
/**
* The physical row number of the header.
*/
@Inject
@BatchProperty
protected Integer headerRow;
protected InputStream inputStream;
protected FormulaEvaluator formulaEvaluator;
protected Iterator rowIterator;
protected int minColumnCount;
/**
* Mapping between column label -> header name, e.g, {A : name, B: age}, or {1: name, 2: age} for R1C1 notation.
* Only applicable when {@link #beanType} is not {@code List}.
*/
protected Map headerMapping;
@Override
public void open(final Serializable checkpoint) throws Exception {
/**
* The row number to start reading. It may be different from the injected field start. During a restart,
* we would start reading from where it ended during the last run.
*/
if (this.end == 0) {
this.end = Integer.MAX_VALUE;
}
if (headerRow == null) {
if (header == null) {
throw SupportMessages.MESSAGES.invalidReaderWriterProperty(null, null, "header | headerRow");
}
headerRow = -1;
}
if (start == headerRow) {
start += 1;
}
final int startRowNumber = checkpoint == null ? this.start : (Integer) checkpoint;
if (startRowNumber < this.start || startRowNumber > this.end
|| startRowNumber < 0 || startRowNumber <= headerRow) {
throw SupportMessages.MESSAGES.invalidStartPosition(startRowNumber, this.start, this.end);
}
inputStream = getInputStream(resource, false);
initWorkbookAndSheet(startRowNumber);
if (header != null) {
minColumnCount = header.length;
}
}
@Override
public Object readItem() throws Exception {
if (currentRowNum == this.end) {
return null;
}
Row row;
while (rowIterator.hasNext()) {
row = rowIterator.next();
currentRowNum = row.getRowNum();
final short lastCellNum = row.getLastCellNum();
if (lastCellNum == -1) { // no cell in the current row
continue;
}
final int lastColumn = Math.max(lastCellNum, minColumnCount);
if (java.util.List.class.isAssignableFrom(beanType)) {
final List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy