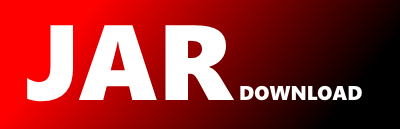
org.jboss.aerogear.unifiedpush.message.jms.CdiJmsBridge Maven / Gradle / Ivy
/**
* JBoss, Home of Professional Open Source
* Copyright Red Hat, Inc., and individual contributors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.jboss.aerogear.unifiedpush.message.jms;
import javax.annotation.Resource;
import javax.enterprise.event.Observes;
import javax.inject.Inject;
import javax.jms.Queue;
import javax.jms.Topic;
import org.jboss.aerogear.unifiedpush.message.MetricsCollector;
import org.jboss.aerogear.unifiedpush.message.event.AllBatchesLoadedEvent;
import org.jboss.aerogear.unifiedpush.message.event.BatchLoadedEvent;
import org.jboss.aerogear.unifiedpush.message.event.MetricsProcessingStartedEvent;
import org.jboss.aerogear.unifiedpush.message.event.TriggerMetricCollectionEvent;
import org.jboss.aerogear.unifiedpush.message.event.TriggerVariantMetricCollectionEvent;
import org.jboss.aerogear.unifiedpush.message.util.JmsClient;
/**
* A CDI-to-JMS bridge takes selected CDI events with {@link DispatchToQueue} stereotype and passes them to JMS queue or topic so that they can be handled asynchronously.
*
* @see MetricsCollector
* @see MetricCollectionTrigger
*/
public class CdiJmsBridge {
@Resource(mappedName = "java:/queue/AllBatchesLoadedQueue")
private Queue allBatchesLoadedQueue;
@Resource(mappedName = "java:/queue/BatchLoadedQueue")
private Queue batchLoadedQueue;
@Resource(mappedName = "java:/queue/TriggerMetricCollectionQueue")
private Queue triggerMetricCollectionQueue;
@Resource(mappedName = "java:/queue/TriggerVariantMetricCollectionQueue")
private Queue triggerVariantMetricCollectionQueue;
@Resource(mappedName = "java:/topic/MetricsProcessingStartedTopic")
private Topic metricsProcessingStartedTopic;
@Inject
private JmsClient jmsClient;
/**
* Listens to {@link AllBatchesLoadedEvent} event and passes it to JMS queue /queue/AllBatchesLoadedQueue with variantID as a correlation identifier.
*
* @param event indicates that all batches for given variant were loaded
*/
public void queueMessage(@Observes @DispatchToQueue AllBatchesLoadedEvent event) {
jmsClient.send(event)
.inTransaction()
.withProperty("variantID", event.getVariantID())
.to(allBatchesLoadedQueue);
}
/**
* Listens to {@link BatchLoadedEvent} event and passes it to JMS queue /queue/BatchLoadedQueue with variantID as a correlation identifier.
*
* @param event indicates that batch of tokens was loaded
*/
public void queueMessage(@Observes @DispatchToQueue BatchLoadedEvent event) {
jmsClient.send(event)
.inTransaction()
.withProperty("variantID", event.getVariantID())
.to(batchLoadedQueue);
}
/**
* Listens to {@link TriggerMetricCollectionEvent} event and passes it to JMS queue /queue/TriggerMetricCollectionQueue for internal processing.
*
* Duplicate message detection is performed via pushMessageInformationId so that we ensure only one message for given push message is fired,
* as we want don't want multiple workers processing metrics for given push message.
*
* The delivery of this message is delayed so that some metrics are generated by it first start to poll respective queues.
*
* @param event indicates that push message metric collection should be started
*/
public void queueMessage(@Observes @DispatchToQueue TriggerMetricCollectionEvent event) {
jmsClient.send(event)
.withDuplicateDetectionId(event.getPushMessageInformationId())
.withDelayedDelivery(500L)
.to(triggerMetricCollectionQueue);
}
/**
* Listens to {@link TriggerVariantMetricCollectionEvent} event and passes it to JMS queue /queue/TriggerVariantMetricCollectionQueue for internal processing.
*
* @param event indicates that variant metric collection should be started
*/
public void queueMessage(@Observes @DispatchToQueue TriggerVariantMetricCollectionEvent event) {
jmsClient.send(event)
.to(triggerVariantMetricCollectionQueue);
}
/**
* Listens to {@link MetricsProcessingStartedEvent} to deliver it to the JMS topic for internal processing
*
* @param event indicator that metrics processing has started
*/
public void broadcastMessage(@Observes @DispatchToQueue MetricsProcessingStartedEvent event) {
jmsClient.send(event)
.to(metricsProcessingStartedTopic);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy