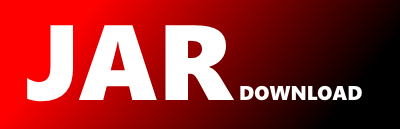
org.jboss.arquillian.testenricher.cdi.MethodParameterInjectionPoint Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of arquillian-testenricher-cdi-jakarta Show documentation
Show all versions of arquillian-testenricher-cdi-jakarta Show documentation
Jakarta CDI TestEnricher for the Arquillian Project
/*
* JBoss, Home of Professional Open Source
* Copyright 2020 Red Hat Inc. and/or its affiliates and other contributors
* by the @authors tag. See the copyright.txt in the distribution for a
* full listing of individual contributors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
* http://www.apache.org/licenses/LICENSE-2.0
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.jboss.arquillian.testenricher.cdi;
import java.lang.annotation.Annotation;
import java.lang.reflect.Member;
import java.lang.reflect.Method;
import java.lang.reflect.Type;
import java.util.Arrays;
import java.util.HashSet;
import java.util.Set;
import jakarta.enterprise.inject.Default;
import jakarta.enterprise.inject.spi.Annotated;
import jakarta.enterprise.inject.spi.AnnotatedCallable;
import jakarta.enterprise.inject.spi.AnnotatedParameter;
import jakarta.enterprise.inject.spi.Bean;
import jakarta.enterprise.inject.spi.BeanManager;
import jakarta.enterprise.inject.spi.InjectionPoint;
import jakarta.enterprise.util.AnnotationLiteral;
/**
* MethodParameterInjectionPoint
*
* @author Aslak Knutsen
* @author Pete Muir
* @version $Revision: $
*/
public class MethodParameterInjectionPoint implements InjectionPoint {
private Method method;
private int position;
private BeanManager beanManager;
public MethodParameterInjectionPoint(Method method, int position, BeanManager beanManager) {
this.method = method;
this.position = position;
this.beanManager = beanManager;
}
/* (non-Javadoc)
* @see jakarta.enterprise.inject.spi.InjectionPoint#getBean()
*/
public Bean> getBean() {
return null;
}
/* (non-Javadoc)
* @see jakarta.enterprise.inject.spi.InjectionPoint#getMember()
*/
public Member getMember() {
return method;
}
/* (non-Javadoc)
* @see jakarta.enterprise.inject.spi.InjectionPoint#getQualifiers()
*/
public Set getQualifiers() {
Set qualifiers = new HashSet();
for (Annotation annotation : method.getParameterAnnotations()[position]) {
if (beanManager.isQualifier(annotation.annotationType())) {
qualifiers.add(annotation);
}
}
if (qualifiers.size() == 0) {
qualifiers.add(new DefaultLiteral());
}
return qualifiers;
}
/* (non-Javadoc)
* @see jakarta.enterprise.inject.spi.InjectionPoint#getType()
*/
public Type getType() {
return findTypeOrGenericType();
}
/* (non-Javadoc)
* @see jakarta.enterprise.inject.spi.InjectionPoint#isDelegate()
*/
public boolean isDelegate() {
return false;
}
/* (non-Javadoc)
* @see jakarta.enterprise.inject.spi.InjectionPoint#isTransient()
*/
public boolean isTransient() {
return false;
}
/* (non-Javadoc)
* @see jakarta.enterprise.inject.spi.InjectionPoint#getAnnotated()
*/
public Annotated getAnnotated() {
return new ArgumentAnnotated();
}
private Type findTypeOrGenericType() {
if (method.getGenericParameterTypes().length > 0) {
return method.getGenericParameterTypes()[position];
}
return method.getParameterTypes()[position];
}
private static class DefaultLiteral extends AnnotationLiteral implements Default {
private static final long serialVersionUID = 1L;
}
private class ArgumentAnnotated implements AnnotatedParameter {
/* (non-Javadoc)
* @see jakarta.enterprise.inject.spi.AnnotatedParameter#getDeclaringCallable()
*/
public AnnotatedCallable getDeclaringCallable() {
return null;
}
/* (non-Javadoc)
* @see jakarta.enterprise.inject.spi.AnnotatedParameter#getPosition()
*/
public int getPosition() {
return position;
}
/* (non-Javadoc)
* @see jakarta.enterprise.inject.spi.Annotated#getAnnotation(java.lang.Class)
*/
public Y getAnnotation(Class annotationType) {
for (Annotation annotation : method.getParameterAnnotations()[position]) {
if (annotation.annotationType() == annotationType) {
return annotationType.cast(annotation);
}
}
return null;
}
/* (non-Javadoc)
* @see jakarta.enterprise.inject.spi.Annotated#getAnnotations()
*/
public Set getAnnotations() {
return new HashSet(Arrays.asList(method.getParameterAnnotations()[position]));
}
/* (non-Javadoc)
* @see jakarta.enterprise.inject.spi.Annotated#getBaseType()
*/
public Type getBaseType() {
return getType();
}
/* (non-Javadoc)
* @see jakarta.enterprise.inject.spi.Annotated#getTypeClosure()
*/
public Set getTypeClosure() {
Set types = new HashSet();
types.add(findTypeOrGenericType());
types.add(Object.class);
return types;
}
/* (non-Javadoc)
* @see jakarta.enterprise.inject.spi.Annotated#isAnnotationPresent(java.lang.Class)
*/
public boolean isAnnotationPresent(Class extends Annotation> annotationType) {
return getAnnotation(annotationType) != null;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy