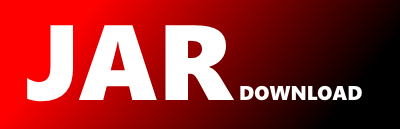
org.jboss.elemento.Elements Maven / Gradle / Ivy
/*
* Copyright 2023 Red Hat
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.jboss.elemento;
import java.util.Iterator;
import java.util.NoSuchElementException;
import java.util.function.Function;
import java.util.function.Predicate;
import java.util.function.Supplier;
import java.util.stream.Stream;
import java.util.stream.StreamSupport;
import org.gwtproject.event.shared.HandlerRegistration;
import org.gwtproject.safehtml.shared.SafeHtml;
import elemental2.core.JsArray;
import elemental2.dom.CSSStyleDeclaration;
import elemental2.dom.DOMRect;
import elemental2.dom.Element;
import elemental2.dom.HTMLAnchorElement;
import elemental2.dom.HTMLAreaElement;
import elemental2.dom.HTMLAudioElement;
import elemental2.dom.HTMLBRElement;
import elemental2.dom.HTMLBodyElement;
import elemental2.dom.HTMLButtonElement;
import elemental2.dom.HTMLCanvasElement;
import elemental2.dom.HTMLDListElement;
import elemental2.dom.HTMLDataListElement;
import elemental2.dom.HTMLDivElement;
import elemental2.dom.HTMLElement;
import elemental2.dom.HTMLEmbedElement;
import elemental2.dom.HTMLFieldSetElement;
import elemental2.dom.HTMLFormElement;
import elemental2.dom.HTMLHRElement;
import elemental2.dom.HTMLHeadingElement;
import elemental2.dom.HTMLIFrameElement;
import elemental2.dom.HTMLImageElement;
import elemental2.dom.HTMLInputElement;
import elemental2.dom.HTMLLIElement;
import elemental2.dom.HTMLLabelElement;
import elemental2.dom.HTMLLegendElement;
import elemental2.dom.HTMLMapElement;
import elemental2.dom.HTMLMeterElement;
import elemental2.dom.HTMLModElement;
import elemental2.dom.HTMLOListElement;
import elemental2.dom.HTMLObjectElement;
import elemental2.dom.HTMLOptGroupElement;
import elemental2.dom.HTMLOptionElement;
import elemental2.dom.HTMLOutputElement;
import elemental2.dom.HTMLParagraphElement;
import elemental2.dom.HTMLParamElement;
import elemental2.dom.HTMLPictureElement;
import elemental2.dom.HTMLPreElement;
import elemental2.dom.HTMLProgressElement;
import elemental2.dom.HTMLQuoteElement;
import elemental2.dom.HTMLScriptElement;
import elemental2.dom.HTMLSelectElement;
import elemental2.dom.HTMLSourceElement;
import elemental2.dom.HTMLTableCaptionElement;
import elemental2.dom.HTMLTableCellElement;
import elemental2.dom.HTMLTableColElement;
import elemental2.dom.HTMLTableElement;
import elemental2.dom.HTMLTableRowElement;
import elemental2.dom.HTMLTableSectionElement;
import elemental2.dom.HTMLTextAreaElement;
import elemental2.dom.HTMLTrackElement;
import elemental2.dom.HTMLUListElement;
import elemental2.dom.HTMLVideoElement;
import elemental2.dom.Node;
import elemental2.dom.NodeList;
import jsinterop.base.Js;
import jsinterop.base.JsArrayLike;
import static elemental2.dom.DomGlobal.document;
import static elemental2.dom.DomGlobal.window;
import static java.util.Collections.emptyIterator;
import static java.util.Collections.emptyList;
import static java.util.Spliterators.spliteratorUnknownSize;
import static jsinterop.base.Js.cast;
import static jsinterop.base.Js.isTripleEqual;
import static jsinterop.base.Js.undefined;
import static org.jboss.elemento.BodyObserver.addAttachObserver;
import static org.jboss.elemento.EventType.bind;
import static org.jboss.elemento.EventType.resize;
/**
* Builder and helper methods for working with {@link elemental2.dom.HTMLElement}s and/or {@link IsElement}.
*
* {@snippet class = ElementsDemo region = builder}
*
* @see https://developer.mozilla.org/en-US/docs/Web/HTML/Element
*/
@SuppressWarnings({"unused", "ConfusingMainMethod"})
public final class Elements {
// ------------------------------------------------------ body
/**
* Returns an HTML content builder for the document body.
*/
public static HTMLContainerBuilder body() {
return new HTMLContainerBuilder<>(document.body);
}
// ------------------------------------------------------ content sectioning
public static HTMLContainerBuilder address() {
return htmlContainer("address", HTMLElement.class);
}
public static HTMLContainerBuilder address(Element element) {
return wrapHtmlContainer(cast(element));
}
public static HTMLContainerBuilder article() {
return htmlContainer("article", HTMLElement.class);
}
public static HTMLContainerBuilder article(Element element) {
return wrapHtmlContainer(cast(element));
}
public static HTMLContainerBuilder aside() {
return htmlContainer("aside", HTMLElement.class);
}
public static HTMLContainerBuilder aside(Element element) {
return wrapHtmlContainer(cast(element));
}
public static HTMLContainerBuilder footer() {
return htmlContainer("footer", HTMLElement.class);
}
public static HTMLContainerBuilder footer(Element element) {
return wrapHtmlContainer(cast(element));
}
public static HTMLContainerBuilder h(int n) {
return htmlContainer("h" + n, HTMLHeadingElement.class);
}
public static HTMLContainerBuilder h(int n, String text) {
return h(n).textContent(text);
}
public static HTMLContainerBuilder h(Element element) {
return wrapHtmlContainer(cast(element));
}
public static HTMLContainerBuilder header() {
return htmlContainer("header", HTMLElement.class);
}
public static HTMLContainerBuilder header(Element element) {
return wrapHtmlContainer(cast(element));
}
public static HTMLContainerBuilder hgroup() {
return htmlContainer("hgroup", HTMLElement.class);
}
public static HTMLContainerBuilder hgroup(Element element) {
return wrapHtmlContainer(cast(element));
}
public static HTMLContainerBuilder nav() {
return htmlContainer("nav", HTMLElement.class);
}
public static HTMLContainerBuilder nav(Element element) {
return wrapHtmlContainer(cast(element));
}
public static HTMLContainerBuilder section() {
return htmlContainer("section", HTMLElement.class);
}
public static HTMLContainerBuilder section(Element element) {
return wrapHtmlContainer(cast(element));
}
// ------------------------------------------------------ text content
public static HTMLContainerBuilder blockquote() {
return htmlContainer("blockquote", HTMLQuoteElement.class);
}
public static HTMLContainerBuilder blockquote(Element element) {
return wrapHtmlContainer(cast(element));
}
public static HTMLContainerBuilder dd() {
return htmlContainer("dd", HTMLElement.class);
}
public static HTMLContainerBuilder dd(Element element) {
return wrapHtmlContainer(cast(element));
}
public static HTMLContainerBuilder div() {
return htmlContainer("div", HTMLDivElement.class);
}
public static HTMLContainerBuilder div(Element element) {
return wrapHtmlContainer(cast(element));
}
public static HTMLContainerBuilder dl() {
return htmlContainer("dl", HTMLDListElement.class);
}
public static HTMLContainerBuilder dl(Element element) {
return wrapHtmlContainer(cast(element));
}
public static HTMLContainerBuilder dt() {
return htmlContainer("dt", HTMLElement.class);
}
public static HTMLContainerBuilder dt(Element element) {
return wrapHtmlContainer(cast(element));
}
public static HTMLContainerBuilder figcaption() {
return htmlContainer("figcaption", HTMLElement.class);
}
public static HTMLContainerBuilder figcaption(Element element) {
return wrapHtmlContainer(cast(element));
}
public static HTMLContainerBuilder figure() {
return htmlContainer("figure", HTMLElement.class);
}
public static HTMLContainerBuilder figure(Element element) {
return wrapHtmlContainer(cast(element));
}
public static HTMLElementBuilder hr() {
return htmlElement("hr", HTMLHRElement.class);
}
public static HTMLElementBuilder hr(Element element) {
return wrapHtmlElement(cast(element));
}
public static HTMLContainerBuilder li() {
return htmlContainer("li", HTMLLIElement.class);
}
public static HTMLContainerBuilder li(Element element) {
return wrapHtmlContainer(cast(element));
}
public static HTMLContainerBuilder main() {
return htmlContainer("main", HTMLElement.class);
}
public static HTMLContainerBuilder main(Element element) {
return wrapHtmlContainer(cast(element));
}
public static HTMLContainerBuilder ol() {
return htmlContainer("ol", HTMLOListElement.class);
}
public static HTMLContainerBuilder ol(Element element) {
return wrapHtmlContainer(cast(element));
}
public static HTMLContainerBuilder p() {
return htmlContainer("p", HTMLParagraphElement.class);
}
public static HTMLContainerBuilder p(Element element) {
return wrapHtmlContainer(cast(element));
}
public static HTMLContainerBuilder pre() {
return htmlContainer("pre", HTMLPreElement.class);
}
public static HTMLContainerBuilder pre(Element element) {
return wrapHtmlContainer(cast(element));
}
public static HTMLContainerBuilder ul() {
return htmlContainer("ul", HTMLUListElement.class);
}
public static HTMLContainerBuilder ul(Element element) {
return wrapHtmlContainer(cast(element));
}
// ------------------------------------------------------ inline text semantics
public static HTMLContainerBuilder a() {
return htmlContainer("a", HTMLAnchorElement.class);
}
public static HTMLContainerBuilder a(String href) {
return a().attr("href", href);
}
public static HTMLContainerBuilder a(String href, String target) {
return a().attr("href", href).attr("target", target);
}
public static HTMLContainerBuilder a(Element element) {
return wrapHtmlContainer(cast(element));
}
public static HTMLContainerBuilder abbr() {
return htmlContainer("abbr", HTMLElement.class);
}
public static HTMLContainerBuilder abbr(String text) {
return htmlContainer("abbr", HTMLElement.class).textContent(text);
}
public static HTMLContainerBuilder abbr(Element element) {
return wrapHtmlContainer(cast(element));
}
public static HTMLContainerBuilder b() {
return htmlContainer("b", HTMLElement.class);
}
public static HTMLContainerBuilder b(String text) {
return htmlContainer("b", HTMLElement.class).textContent(text);
}
public static HTMLContainerBuilder b(Element element) {
return wrapHtmlContainer(cast(element));
}
public static HTMLElementBuilder br() {
return htmlElement("br", HTMLBRElement.class);
}
public static HTMLElementBuilder br(Element element) {
return wrapHtmlElement(cast(element));
}
public static HTMLContainerBuilder cite() {
return htmlContainer("cite", HTMLElement.class);
}
public static HTMLContainerBuilder cite(String text) {
return htmlContainer("cite", HTMLElement.class).textContent(text);
}
public static HTMLContainerBuilder cite(Element element) {
return wrapHtmlContainer(cast(element));
}
public static HTMLContainerBuilder code() {
return htmlContainer("code", HTMLElement.class);
}
public static HTMLContainerBuilder code(String text) {
return htmlContainer("code", HTMLElement.class).textContent(text);
}
public static HTMLContainerBuilder code(Element element) {
return wrapHtmlContainer(cast(element));
}
public static HTMLContainerBuilder dfn() {
return htmlContainer("dfn", HTMLElement.class);
}
public static HTMLContainerBuilder dfn(String text) {
return htmlContainer("dfn", HTMLElement.class).textContent(text);
}
public static HTMLContainerBuilder dfn(Element element) {
return wrapHtmlContainer(cast(element));
}
public static HTMLContainerBuilder em() {
return htmlContainer("em", HTMLElement.class);
}
public static HTMLContainerBuilder em(String text) {
return htmlContainer("em", HTMLElement.class).textContent(text);
}
public static HTMLContainerBuilder em(Element element) {
return wrapHtmlContainer(cast(element));
}
public static HTMLContainerBuilder i() {
return htmlContainer("i", HTMLElement.class);
}
public static HTMLContainerBuilder i(String text) {
return htmlContainer("i", HTMLElement.class).textContent(text);
}
public static HTMLContainerBuilder i(Element element) {
return wrapHtmlContainer(cast(element));
}
public static HTMLContainerBuilder kbd() {
return htmlContainer("kbd", HTMLElement.class);
}
public static HTMLContainerBuilder kbd(String text) {
return htmlContainer("kbd", HTMLElement.class).textContent(text);
}
public static HTMLContainerBuilder kbd(Element element) {
return wrapHtmlContainer(cast(element));
}
public static HTMLContainerBuilder mark() {
return htmlContainer("mark", HTMLElement.class);
}
public static HTMLContainerBuilder mark(String text) {
return htmlContainer("mark", HTMLElement.class).textContent(text);
}
public static HTMLContainerBuilder mark(Element element) {
return wrapHtmlContainer(cast(element));
}
public static HTMLContainerBuilder q() {
return htmlContainer("q", HTMLQuoteElement.class);
}
public static HTMLContainerBuilder q(String text) {
return htmlContainer("q", HTMLQuoteElement.class).textContent(text);
}
public static HTMLContainerBuilder q(Element element) {
return wrapHtmlContainer(cast(element));
}
public static HTMLContainerBuilder small() {
return htmlContainer("small", HTMLElement.class);
}
public static HTMLContainerBuilder small(String text) {
return htmlContainer("small", HTMLElement.class).textContent(text);
}
public static HTMLContainerBuilder small(Element element) {
return wrapHtmlContainer(cast(element));
}
public static HTMLContainerBuilder span() {
return htmlContainer("span", HTMLElement.class);
}
public static HTMLContainerBuilder span(Element element) {
return wrapHtmlContainer(cast(element));
}
public static HTMLContainerBuilder strong() {
return htmlContainer("strong", HTMLElement.class);
}
public static HTMLContainerBuilder strong(String text) {
return htmlContainer("strong", HTMLElement.class).textContent(text);
}
public static HTMLContainerBuilder strong(Element element) {
return wrapHtmlContainer(cast(element));
}
public static HTMLContainerBuilder sub() {
return htmlContainer("sub", HTMLElement.class);
}
public static HTMLContainerBuilder sub(String text) {
return htmlContainer("sub", HTMLElement.class).textContent(text);
}
public static HTMLContainerBuilder sub(Element element) {
return wrapHtmlContainer(cast(element));
}
public static HTMLContainerBuilder sup() {
return htmlContainer("sup", HTMLElement.class);
}
public static HTMLContainerBuilder sup(String text) {
return htmlContainer("sup", HTMLElement.class).textContent(text);
}
public static HTMLContainerBuilder sup(Element element) {
return wrapHtmlContainer(cast(element));
}
public static HTMLContainerBuilder time() {
return htmlContainer("time", HTMLElement.class);
}
public static HTMLContainerBuilder time(String text) {
return htmlContainer("time", HTMLElement.class).textContent(text);
}
public static HTMLContainerBuilder time(Element element) {
return wrapHtmlContainer(cast(element));
}
public static HTMLContainerBuilder u() {
return htmlContainer("u", HTMLElement.class);
}
public static HTMLContainerBuilder u(String text) {
return htmlContainer("u", HTMLElement.class).textContent(text);
}
public static HTMLContainerBuilder u(Element element) {
return wrapHtmlContainer(cast(element));
}
public static HTMLContainerBuilder var() {
return htmlContainer("var", HTMLElement.class);
}
public static HTMLContainerBuilder var(String text) {
return htmlContainer("var", HTMLElement.class).textContent(text);
}
public static HTMLContainerBuilder var(Element element) {
return wrapHtmlContainer(cast(element));
}
public static HTMLElementBuilder wbr() {
return htmlElement("wbr", HTMLElement.class);
}
public static HTMLElementBuilder wbr(Element element) {
return wrapHtmlElement(cast(element));
}
// ------------------------------------------------------ image and multimedia
public static HTMLElementBuilder area() {
return htmlElement("area", HTMLAreaElement.class);
}
public static HTMLElementBuilder area(Element element) {
return wrapHtmlElement(cast(element));
}
public static HTMLContainerBuilder audio() {
return htmlContainer("audio", HTMLAudioElement.class);
}
public static HTMLContainerBuilder audio(Element element) {
return wrapHtmlContainer(cast(element));
}
public static HTMLElementBuilder img() {
return htmlElement("img", HTMLImageElement.class);
}
public static HTMLElementBuilder img(String src) {
return htmlElement("img", HTMLImageElement.class).attr("src", src);
}
public static HTMLElementBuilder img(Element element) {
return wrapHtmlElement(cast(element));
}
public static HTMLContainerBuilder map() {
return htmlContainer("map", HTMLMapElement.class);
}
public static HTMLContainerBuilder map(Element element) {
return wrapHtmlContainer(cast(element));
}
public static HTMLContainerBuilder picture() {
return htmlContainer("picture", HTMLPictureElement.class);
}
public static HTMLContainerBuilder picture(Element element) {
return wrapHtmlContainer(cast(element));
}
public static HTMLElementBuilder track() {
return htmlElement("track", HTMLTrackElement.class);
}
public static HTMLElementBuilder track(Element element) {
return wrapHtmlElement(cast(element));
}
public static HTMLContainerBuilder video() {
return htmlContainer("video", HTMLVideoElement.class);
}
public static HTMLContainerBuilder video(Element element) {
return wrapHtmlContainer(cast(element));
}
// ------------------------------------------------------ embedded content
public static HTMLContainerBuilder canvas() {
return htmlContainer("canvas", HTMLCanvasElement.class);
}
public static HTMLContainerBuilder canvas(Element element) {
return wrapHtmlContainer(cast(element));
}
public static HTMLElementBuilder embed() {
return htmlElement("embed", HTMLEmbedElement.class);
}
public static HTMLElementBuilder embed(Element element) {
return wrapHtmlElement(cast(element));
}
public static HTMLContainerBuilder iframe() {
return htmlContainer("iframe", HTMLIFrameElement.class);
}
public static HTMLContainerBuilder iframe(String src) {
return iframe().attr("src", src);
}
public static HTMLContainerBuilder iframe(Element element) {
return wrapHtmlContainer(cast(element));
}
public static HTMLContainerBuilder object() {
return htmlContainer("object", HTMLObjectElement.class);
}
public static HTMLContainerBuilder object(Element element) {
return wrapHtmlContainer(cast(element));
}
public static HTMLElementBuilder param() {
return htmlElement("param", HTMLParamElement.class);
}
public static HTMLElementBuilder param(Element element) {
return wrapHtmlElement(cast(element));
}
public static HTMLElementBuilder source() {
return htmlElement("source", HTMLSourceElement.class);
}
public static HTMLElementBuilder source(Element element) {
return wrapHtmlElement(cast(element));
}
// ------------------------------------------------------ scripting
public static HTMLContainerBuilder noscript() {
return htmlContainer("noscript", HTMLElement.class);
}
public static HTMLContainerBuilder noscript(Element element) {
return wrapHtmlContainer(cast(element));
}
public static HTMLElementBuilder script() {
return htmlElement("script", HTMLScriptElement.class);
}
public static HTMLElementBuilder script(Element element) {
return wrapHtmlElement(cast(element));
}
// ------------------------------------------------------ demarcating edits
public static HTMLContainerBuilder del() {
return htmlContainer("del", HTMLModElement.class);
}
public static HTMLContainerBuilder del(String text) {
return htmlContainer("del", HTMLModElement.class).textContent(text);
}
public static HTMLContainerBuilder del(Element element) {
return wrapHtmlContainer(cast(element));
}
public static HTMLContainerBuilder ins() {
return htmlContainer("ins", HTMLModElement.class);
}
public static HTMLContainerBuilder ins(String text) {
return htmlContainer("ins", HTMLModElement.class).textContent(text);
}
public static HTMLContainerBuilder ins(Element element) {
return wrapHtmlContainer(cast(element));
}
// ------------------------------------------------------ table content
public static HTMLContainerBuilder caption() {
return htmlContainer("caption", HTMLTableCaptionElement.class);
}
public static HTMLContainerBuilder caption(Element element) {
return wrapHtmlContainer(cast(element));
}
public static HTMLElementBuilder col() {
return htmlElement("col", HTMLTableColElement.class);
}
public static HTMLElementBuilder col(Element element) {
return wrapHtmlElement(cast(element));
}
public static HTMLContainerBuilder colgroup() {
return htmlContainer("colgroup", HTMLTableColElement.class);
}
public static HTMLContainerBuilder colgroup(Element element) {
return wrapHtmlContainer(cast(element));
}
public static HTMLContainerBuilder table() {
return htmlContainer("table", HTMLTableElement.class);
}
public static HTMLContainerBuilder table(Element element) {
return wrapHtmlContainer(cast(element));
}
public static HTMLContainerBuilder tbody() {
return htmlContainer("tbody", HTMLTableSectionElement.class);
}
public static HTMLContainerBuilder tbody(Element element) {
return wrapHtmlContainer(cast(element));
}
public static HTMLContainerBuilder td() {
return htmlContainer("td", HTMLTableCellElement.class);
}
public static HTMLContainerBuilder td(Element element) {
return wrapHtmlContainer(cast(element));
}
public static HTMLContainerBuilder tfoot() {
return htmlContainer("tfoot", HTMLTableSectionElement.class);
}
public static HTMLContainerBuilder tfoot(Element element) {
return wrapHtmlContainer(cast(element));
}
public static HTMLContainerBuilder th() {
return htmlContainer("th", HTMLTableCellElement.class);
}
public static HTMLContainerBuilder th(Element element) {
return wrapHtmlContainer(cast(element));
}
public static HTMLContainerBuilder thead() {
return htmlContainer("thead", HTMLTableSectionElement.class);
}
public static HTMLContainerBuilder thead(Element element) {
return wrapHtmlContainer(cast(element));
}
public static HTMLContainerBuilder tr() {
return htmlContainer("tr", HTMLTableRowElement.class);
}
public static HTMLContainerBuilder tr(Element element) {
return wrapHtmlContainer(cast(element));
}
// ------------------------------------------------------ forms
public static HTMLContainerBuilder button() {
return htmlContainer("button", HTMLButtonElement.class);
}
public static HTMLContainerBuilder button(ButtonType type) {
HTMLButtonElement button = createHtmlElement("button", HTMLButtonElement.class);
button.type = type.name();
return new HTMLContainerBuilder<>(button);
}
public static HTMLContainerBuilder button(String text) {
return button().textContent(text);
}
public static HTMLContainerBuilder button(Element element) {
return wrapHtmlContainer(cast(element));
}
public static HTMLContainerBuilder datalist() {
return htmlContainer("datalist", HTMLDataListElement.class);
}
public static HTMLContainerBuilder datalist(Element element) {
return wrapHtmlContainer(cast(element));
}
public static HTMLContainerBuilder fieldset() {
return htmlContainer("fieldset", HTMLFieldSetElement.class);
}
public static HTMLContainerBuilder fieldset(Element element) {
return wrapHtmlContainer(cast(element));
}
public static HTMLContainerBuilder form() {
return htmlContainer("form", HTMLFormElement.class);
}
public static HTMLContainerBuilder form(Element element) {
return wrapHtmlContainer(cast(element));
}
public static InputElementBuilder input(InputType type) {
return input(type.name());
}
public static InputElementBuilder input(String type) {
return input(type, HTMLInputElement.class);
}
public static InputElementBuilder input(String type, Class jType) {
E el = createHtmlElement("input", jType);
el.type = type;
return new InputElementBuilder<>(el);
}
public static InputElementBuilder input(Element element) {
return wrapInputElement(cast(element));
}
public static HTMLContainerBuilder label() {
return htmlContainer("label", HTMLLabelElement.class);
}
public static HTMLContainerBuilder label(String text) {
return label().textContent(text);
}
public static HTMLContainerBuilder label(Element element) {
return wrapHtmlContainer(cast(element));
}
public static HTMLContainerBuilder legend() {
return htmlContainer("legend", HTMLLegendElement.class);
}
public static HTMLContainerBuilder legend(Element element) {
return wrapHtmlContainer(cast(element));
}
public static HTMLContainerBuilder meter() {
return htmlContainer("meter", HTMLMeterElement.class);
}
public static HTMLContainerBuilder meter(String text) {
return htmlContainer("meter", HTMLMeterElement.class).textContent(text);
}
public static HTMLContainerBuilder meter(Element element) {
return wrapHtmlContainer(cast(element));
}
public static HTMLContainerBuilder optgroup() {
return htmlContainer("optgroup", HTMLOptGroupElement.class);
}
public static HTMLContainerBuilder optgroup(Element element) {
return wrapHtmlContainer(cast(element));
}
public static HTMLElementBuilder option() {
return htmlElement("option", HTMLOptionElement.class);
}
public static HTMLElementBuilder option(String text) {
return option().textContent(text);
}
public static HTMLElementBuilder option(Element element) {
return wrapHtmlElement(cast(element));
}
public static HTMLContainerBuilder output() {
return htmlContainer("output", HTMLOutputElement.class);
}
public static HTMLContainerBuilder output(String text) {
return htmlContainer("output", HTMLOutputElement.class).textContent(text);
}
public static HTMLContainerBuilder output(Element element) {
return wrapHtmlContainer(cast(element));
}
public static HTMLContainerBuilder progress() {
return htmlContainer("progress", HTMLProgressElement.class);
}
public static HTMLContainerBuilder progress(Element element) {
return wrapHtmlContainer(cast(element));
}
public static HTMLContainerBuilder select() {
return htmlContainer("select", HTMLSelectElement.class);
}
public static HTMLContainerBuilder select(Element element) {
return wrapHtmlContainer(cast(element));
}
public static HTMLElementBuilder textarea() {
return htmlElement("textarea", HTMLTextAreaElement.class);
}
public static HTMLElementBuilder textarea(Element element) {
return wrapHtmlElement(cast(element));
}
// ------------------------------------------------------ factories & wrappers
/**
* Returns a builder to collect {@link HTMLElement}s.
*
* {@snippet class = ElementsBagDemo region = bag}
*/
public static ElementsBag bag() {
return new ElementsBag();
}
/**
* Returns a builder for the specified HTML element.
*/
public static HTMLElementBuilder htmlElement(String element, Class type) {
return new HTMLElementBuilder<>(createHtmlElement(element, type));
}
/**
* Returns a builder for the existing HTML element.
*/
public static HTMLElementBuilder wrapHtmlElement(E element) {
return new HTMLElementBuilder<>(element);
}
/**
* Returns a builder for the specified input element.
*/
public static InputElementBuilder inputElement(String type, Class jType) {
E input = createHtmlElement("input", jType);
input.type = type;
return new InputElementBuilder<>(input);
}
/**
* Returns a builder for the existing input element.
*/
public static InputElementBuilder wrapInputElement(E element) {
return new InputElementBuilder<>(element);
}
/**
* Returns a builder for the specified HTML element.
*/
public static HTMLContainerBuilder htmlContainer(String element, Class type) {
return new HTMLContainerBuilder<>(createHtmlElement(element, type));
}
/**
* Returns a builder for the existing HTML element.
*/
public static HTMLContainerBuilder wrapHtmlContainer(E element) {
return new HTMLContainerBuilder<>(element);
}
/**
* Creates the specified HTML element.
*/
public static E createHtmlElement(String element, Class type) {
return cast(document.createElement(element));
}
// ------------------------------------------------------ finder methods
/**
* Finds all HTML elements for the given selector.
*/
public static Iterable findAll(Node node, By selector) {
if (node != null) {
NodeList nodes = node.querySelectorAll(selector.selector());
JsArray htmlElements = new JsArray<>();
for (int i = 0; i < nodes.length; i++) {
Element element = nodes.getAt(i);
if (element instanceof HTMLElement) {
htmlElements.push((HTMLElement) element);
}
}
return () -> iterator(htmlElements);
}
return emptyList();
}
/**
* Finds all HTML elements for the given selector.
*/
public static Iterable findAll(IsElement element, By selector) {
if (element != null) {
return findAll(element.element(), selector);
}
return emptyList();
}
/**
* Finds a single HTML elements for the given selector.
*/
public static E find(Node node, By selector) {
if (node != null) {
return cast(node.querySelector(selector.selector()));
}
return null;
}
/**
* Finds a single HTML elements for the given selector.
*/
public static F find(IsElement element, By selector) {
if (element != null) {
return find(element.element(), selector);
}
return null;
}
/**
* Finds the closest HTML elements for the given selector.
*
* @see https://developer.mozilla.org/en-US/docs/Web/API/Element/closest
*/
public static E closest(Element element, By selector) {
if (element != null) {
return cast(element.closest(selector.selector()));
}
return null;
}
/**
* Finds the closest HTML elements for the given selector.
*
* @see https://developer.mozilla.org/en-US/docs/Web/API/Element/closest
*/
public static F closest(IsElement element, By selector) {
if (element != null) {
return cast(element.element().closest(selector.selector()));
}
return null;
}
// ------------------------------------------------------ iterator methods
/**
* Returns an iterator over the given array-like. The iterator does not support the
* {@link Iterator#remove()} operation.
*/
public static Iterator iterator(JsArrayLike data) {
return data != null ? new JsArrayLikeIterator<>(data) : emptyIterator();
}
/**
* Returns an iterator over the children of the given parent node. The iterator supports the {@link Iterator#remove()}
* operation, which removes the current node from its parent.
*/
public static Iterator iterator(Node parent) {
return parent != null ? new JsArrayNodeIterator(parent) : emptyIterator();
}
/**
* Returns an iterator over the children of the given parent element. The iterator supports the {@link Iterator#remove()}
* operation, which removes the current node from its parent.
*/
public static Iterator iterator(HTMLElement parent) {
return parent != null ? new JsArrayElementIterator(parent) : emptyIterator();
}
/**
* Returns an iterator over the children of the given parent element. The iterator supports the {@link Iterator#remove()}
* operation, which removes the current node from its parent.
*/
public static Iterator iterator(IsElement parent) {
return parent != null ? iterator(parent.element()) : emptyIterator();
}
// ------------------------------------------------------ iterable methods
/**
* Returns an iterable for the elements in the given array-like.
*/
public static Iterable elements(JsArrayLike nodes) {
return () -> iterator(nodes);
}
/**
* Returns an iterable for the child nodes of the given parent node.
*/
public static Iterable children(Node parent) {
return () -> iterator(parent);
}
/**
* Returns an iterable for the child elements of the given parent element.
*/
public static Iterable children(HTMLElement parent) {
return () -> iterator(parent);
}
/**
* Returns an iterable for the child elements of the given parent element.
*/
public static Iterable