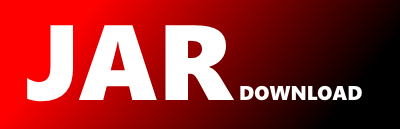
org.jboss.elemento.logger.Logger Maven / Gradle / Ivy
/*
* Copyright 2023 Red Hat
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.jboss.elemento.logger;
import java.util.HashMap;
import java.util.Map;
import elemental2.core.JsDate;
import static elemental2.dom.DomGlobal.console;
import static org.jboss.elemento.logger.Level.DEBUG;
import static org.jboss.elemento.logger.Level.ERROR;
import static org.jboss.elemento.logger.Level.INFO;
import static org.jboss.elemento.logger.Level.WARN;
/**
* Small wrapper around console.log
that uses categories, {@linkplain Level log levels}, and a predefined log
* format.
*
* The different log methods delegate to the corresponding methods in console
:
*
* - {@link #error(String, Object...)} →
console.error()
* - {@link #warn(String, Object...)} →
console.warn()
* - {@link #info(String, Object...)} →
console.info()
* - {@link #debug(String, Object...)} →
console.debug()
*
*
* To get a logger use {@link Logger#getLogger(String)}. You can use an arbitrary string as category. If you use a fully qualified class name as category the package names are shortened. In any case the category is trimmed, and right aligned. String substitutions are supported, and you can pass arbitrary parameters to the log methods.
*
* The log level is set globally for all categories using {@link Logger#setLevel(Level)}.
*
* The log format is predefined as
*
* HH:mm:ss,SSS <level> [<category>] <message>
*
*
* @see https://developer.mozilla.org/en-US/docs/Web/API/console/log_static
*/
public class Logger {
private static final int CATEGORY_LENGTH = 23;
private static final String ROOT_CATEGORY = "root";
private static final Map loggers = new HashMap<>();
private static Level level = INFO;
public static Logger getLogger(String name) {
String safeName = name == null || name.isEmpty() ? ROOT_CATEGORY : name;
Logger logger = loggers.get(safeName);
if (logger == null) {
logger = new Logger(safeName);
loggers.put(safeName, logger);
}
return logger;
}
public static void setLevel(Level level) {
Logger.level = level;
}
private final String category;
private Logger(String category) {
this.category = Category.format(category, CATEGORY_LENGTH);
}
public void debug(String message, Object... params) {
if (level.ordinal() >= DEBUG.ordinal()) {
if (params == null || params.length == 0) {
console.debug(format(DEBUG, message));
} else {
console.debug(format(DEBUG, message), params);
}
}
}
public void info(String message, Object... params) {
if (level.ordinal() >= INFO.ordinal()) {
if (params == null || params.length == 0) {
console.info(format(INFO, message));
} else {
console.info(format(INFO, message), params);
}
}
}
public void warn(String message, Object... params) {
if (level.ordinal() >= WARN.ordinal()) {
if (params == null || params.length == 0) {
console.warn(format(WARN, message));
} else {
console.warn(format(WARN, message), params);
}
}
}
public void error(String message, Object... params) {
if (params == null || params.length == 0) {
console.error(format(ERROR, message));
} else {
console.error(format(ERROR, message), params);
}
}
private String format(Level level, String message) {
String iso = new JsDate().toISOString(); // 2011-10-05T14:48:00.000Z
return iso.substring(11, 23) + " " + level.label + " [" + category + "] " + message;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy