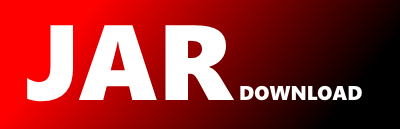
com.arjuna.orbportability.common.ant.IDLCompiler Maven / Gradle / Ivy
The newest version!
/*
* JBoss, Home of Professional Open Source
* Copyright 2006, Red Hat Middleware LLC, and individual contributors
* as indicated by the @author tags.
* See the copyright.txt in the distribution for a full listing
* of individual contributors.
* This copyrighted material is made available to anyone wishing to use,
* modify, copy, or redistribute it subject to the terms and conditions
* of the GNU Lesser General Public License, v. 2.1.
* This program is distributed in the hope that it will be useful, but WITHOUT A
* WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS FOR A
* PARTICULAR PURPOSE. See the GNU Lesser General Public License for more details.
* You should have received a copy of the GNU Lesser General Public License,
* v.2.1 along with this distribution; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston,
* MA 02110-1301, USA.
*
* (C) 2005-2006,
* @author JBoss Inc.
*/
/*
* Copyright (C) 2002
*
* Arjuna Solutions Limited,
* Newcastle upon Tyne,
* Tyne and Wear,
* UK.
*
* $Id: IDLCompiler.java 2342 2006-03-30 13:06:17Z $
*/
package com.arjuna.orbportability.common.ant;
import java.io.File;
import java.io.FileOutputStream;
import java.io.InputStream;
import java.util.ArrayList;
import java.util.Enumeration;
import java.util.HashSet;
import java.util.Hashtable;
import java.util.StringTokenizer;
import javax.xml.parsers.DocumentBuilder;
import javax.xml.parsers.DocumentBuilderFactory;
import org.apache.tools.ant.BuildException;
import org.apache.tools.ant.Task;
import org.apache.tools.ant.types.FileSet;
import org.w3c.dom.Document;
import org.w3c.dom.Element;
import org.w3c.dom.NamedNodeMap;
import org.w3c.dom.Node;
import org.w3c.dom.NodeList;
/**
* Ant task to compile IDL across multiple ORB's.
*
* This task uses a definitions file (idl-compiler-definitions.xml) which defines how to call each of the supported IDL compilers.
* For each compiler you specify a number of parameters and whether they are required for this IDL compiler. You then
* call this ant task and pass it a comma separated list of parameters which it then maps to the IDL compiler specific parameters.
*
* @author Richard A. Begg
*/
public class IDLCompiler extends Task
{
private final static String NAME_ELEMENT = "name";
private final static String VALUE_ELEMENT = "value";
protected String _parameters = null;
protected String _orb = null;
protected Hashtable _idlCompilerDefinitions = null;
protected String _filename = null;
protected String _destinationDirectory = null;
protected String _packageName = null;
protected String _buildList = null;
protected boolean _debugOn = false;
protected boolean _verbose = false;
protected FileSet _fileSet = null;
public IDLCompiler()
{
parseIDLCompilerDefinitionsFile();
}
public final void setDebug(String debug)
{
_debugOn = new Boolean(debug).booleanValue();
}
public final void setVerbose(String verbose)
{
_verbose = new Boolean(verbose).booleanValue();
}
public final void setDestdir(String destDir)
{
_destinationDirectory = destDir;
}
public void setBuildlist(String buildList)
{
_buildList = buildList;
}
public final void setPackage(String packageName)
{
_packageName = packageName;
}
public final void setParameters(String parameters)
{
_parameters = parameters;
}
public final void setFilename(String filename)
{
_filename = filename;
}
public final void setOrb(String orb)
{
_orb = orb;
}
protected boolean childrenContainsSubParam(Node n)
{
NodeList children = n.getChildNodes();
boolean found = false;
for (int count=0;(count 0))
{
throw new BuildException("The IDL build list is invalid!");
}
while (blp.getNextElement())
{
String source = blp.getSource();
String packageName = blp.getPackage();
String mappings = blp.getMappings();
if (!processIDL(idlCompiler, source, packageName, _destinationDirectory, mappings))
{
throw new BuildException("Failed to compile '" + source + "' file");
}
}
}
else
{
if (_filename == null)
{
throw new BuildException("No source filename has been given");
}
if (_packageName == null)
{
throw new BuildException("No package name has been given");
}
log("Processing '" + _filename + "'");
if (!processIDL(idlCompiler, _filename, _packageName, _destinationDirectory))
{
throw new BuildException("Failed to compile '" + _filename + "' file");
}
}
}
public boolean processIDL(IDLCompilerDetails idlCompiler, String filename, String packageName, String destDir)
{
return processIDL(idlCompiler, filename, packageName, "");
}
public boolean processIDL(IDLCompilerDetails idlCompiler, String filename, String packageName, String destDir, String mappings)
{
boolean returnValue = false;
log("Processing for " + idlCompiler.getORBName() + " '" + filename + "'");
if (_verbose)
{
log("Package: " + packageName);
log("Parameters: " + _parameters);
log("Mappings: " + mappings);
log("Destination Directory: " + destDir);
}
try
{
String[] idlParameters = idlCompiler.parse(filename, destDir, packageName, _parameters, mappings);
String[] idlExec = createExecArray(idlCompiler.getCompilerExecutable());
String[] execParameters = new String[idlExec.length + idlParameters.length];
System.arraycopy(idlExec, 0, execParameters, 0, idlExec.length);
System.arraycopy(idlParameters, 0, execParameters, idlExec.length, idlParameters.length);
Runtime rt = Runtime.getRuntime();
if (_debugOn)
{
for (int count = 0; count < execParameters.length; count++)
log("Param[" + count + "] = '" + execParameters[count] + "'");
}
Process idlProc = rt.exec(execParameters);
if (idlProc == null)
{
throw new BuildException("Failed to run IDL compiler");
}
new InputStreamFileWriter(idlProc.getInputStream(), "idl-compiler.out");
new InputStreamFileWriter(idlProc.getErrorStream(), "idl-compiler.err");
returnValue = (idlProc.waitFor() == 0);
}
catch (Exception e)
{
e.printStackTrace();
throw new BuildException("The following exception occurred while executing the IDL compiler: " + e);
}
return (returnValue);
}
public class IDLCompilerDetails
{
protected String _orbName;
protected String _compilerExe;
protected Hashtable _inParameters;
protected ArrayList _outParameters;
protected Hashtable _parameterValueMap = new Hashtable();
protected Hashtable _forEachMapping = new Hashtable();
public IDLCompilerDetails()
{
_inParameters = new Hashtable();
_outParameters = new ArrayList();
}
public void setIDLExecutable(String idlExe)
{
_compilerExe = idlExe;
}
public void setORBName(String name)
{
_orbName = name;
}
public String getORBName()
{
return (_orbName);
}
public String getCompilerExecutable() throws Exception
{
return (replaceVariables(_compilerExe, _parameterValueMap, new HashSet()));
}
public void addInParameter(String param, boolean required, String delimiter, boolean isClasspath, String replace)
{
_inParameters.put(param, new InParameterDetails(param, required, delimiter, isClasspath, replace));
}
public InParameterDetails getInParameter(String param)
{
return ((InParameterDetails) _inParameters.get(param));
}
public void addOutParameter(String param)
{
_outParameters.add(param);
}
public void addOutParameter(String param, String forEach)
{
_outParameters.add(param);
setForEach(param, forEach);
}
public void setForEach(String param, String forEach)
{
_forEachMapping.put(param, forEach);
}
public void addOutParameter(ArrayList params)
{
_outParameters.add(params);
}
public void addOutParameter(ArrayList params, String forEach)
{
_outParameters.add(params);
_forEachMapping.put(params, forEach);
}
protected InParameterDetails setAndFlagParameter(String param, String value, Hashtable map)
{
if (value != null)
{
InParameterDetails inParamDef = getInParameter(param);
if (inParamDef == null)
{
throw new BuildException("The parameter '" + param + "' used in the parameter attribute is not defined for this ORB");
}
if (inParamDef.hasDelimiter())
{
StringTokenizer st = new StringTokenizer(value,inParamDef.getDelimiter());
ArrayList values = new ArrayList();
while (st.hasMoreTokens())
{
values.add(st.nextToken());
}
map.put(param, values);
}
else
{
map.put(param, value);
}
return (inParamDef);
}
else
{
map.put(param, value);
}
return (null);
}
/**
* Passed the parameters line from the ANT build file, e.g.
*
* parameter='value',parameter2='value2',...
*/
public String[] parse(String filename, String destDir, String packageName, String parameters, String mappings) throws BuildException
{
HashSet usedParameters = new HashSet();
ArrayList outputParameters = new ArrayList();
String parameterPair;
String parameter;
String value;
/**
* Ensure filename is valid and contains data
*/
if ( (filename != null) && (filename.length() > 0) )
{
usedParameters.add(setAndFlagParameter("filename", filename, _parameterValueMap));
}
else
{
/**
* If the filename wasn't valid or didn't contain data then throw a buildexception
*/
throw new BuildException("The filename specified was invalid");
}
/**
* Ensure destDir is valid and contains data
*/
if ( (destDir != null) && (destDir.length() > 0) )
{
usedParameters.add(setAndFlagParameter("destdir", destDir, _parameterValueMap));
}
/**
* Ensure packageName is valid and contains data
*/
if ( (packageName != null) && (packageName.length() > 0) )
{
usedParameters.add(setAndFlagParameter("package", packageName, _parameterValueMap));
}
/**
* Ensure mappings is valid and contains data
*/
if ( (mappings != null) && (mappings.length() > 0) )
{
usedParameters.add(setAndFlagParameter("mapping", mappings, _parameterValueMap));
}
if (parameters != null)
{
/**
* Parse passed in parameters
*/
while (parameters.indexOf(',') != -1)
{
parameterPair = parameters.substring(0, parameters.indexOf(','));
parameter = parameterPair.substring(0, parameterPair.indexOf('='));
value = parameterPair.substring(parameterPair.indexOf('='));
value = value.substring(value.indexOf('\'') + 1);
value = value.substring(0, value.indexOf('\''));
InParameterDetails inParamDef = getInParameter(parameter);
if (inParamDef == null)
{
throw new BuildException("The parameter '" + parameter + "' used in the parameter attribute is not defined for this ORB");
}
if (usedParameters.contains(inParamDef))
{
throw new BuildException("The parameter '" + parameter + "' has been defined twice");
}
/**
* Add parameter to usedParameters array
*/
usedParameters.add(inParamDef);
ArrayList values = new ArrayList();
_parameterValueMap.put(parameter, values);
if (inParamDef.hasDelimiter())
{
StringTokenizer st = new StringTokenizer(value,inParamDef.getDelimiter());
while (st.hasMoreTokens())
{
values.add(st.nextToken());
}
}
else
{
values.add(value);
}
parameters = parameters.substring(parameters.indexOf(',') + 1);
}
parameter = parameters.substring(0, parameters.indexOf('='));
value = parameters.substring(parameters.indexOf('='));
value = value.substring(value.indexOf('\'') + 1);
value = value.substring(0, value.indexOf('\''));
InParameterDetails inParamDef = getInParameter(parameter);
if (inParamDef == null)
{
throw new BuildException("The parameter '" + parameter + "' used in the parameter attribute is not defined for this ORB");
}
if (usedParameters.contains(inParamDef))
{
throw new BuildException("The parameter '" + parameter + "' has been defined twice");
}
usedParameters.add(inParamDef);
ArrayList values = new ArrayList();
_parameterValueMap.put(parameter, values);
if ( ( inParamDef.hasDelimiter() ) && ( !inParamDef.isClasspath() ) )
{
StringTokenizer st = new StringTokenizer(value,inParamDef.getDelimiter());
while (st.hasMoreTokens())
{
values.add(st.nextToken());
}
}
else
{
if ( inParamDef.isClasspath() )
{
if ( !inParamDef.hasDelimiter() )
{
throw new BuildException("Param defined as classpath but delimiter defined");
}
value = createClasspath( value, inParamDef.getDelimiter() );
}
if ( inParamDef.getReplaceString() != null )
{
String replaceString = inParamDef.getReplaceString();
String find = replaceString.substring( 0, replaceString.indexOf(',') );
String replaceWith = replaceString.substring( replaceString.indexOf(',') + 1 );
while ( value.indexOf(find) != -1 )
{
value = value.substring(0, value.indexOf(find)) + replaceWith + value.substring( value.indexOf(find) + find.length() );
}
}
values.add(value);
}
}
for (Enumeration e = _inParameters.elements(); e.hasMoreElements();)
{
InParameterDetails ipd = (InParameterDetails) e.nextElement();
if (ipd.isRequired() && !usedParameters.contains(ipd))
{
throw new BuildException("The parameter '" + ipd.getParameter() + "' is defined as being required but has not been specified");
}
}
for (int count = 0; count < _outParameters.size(); count++)
{
if ( _outParameters.get(count) instanceof ArrayList )
{
ArrayList subParams = (ArrayList) _outParameters.get(count);
ArrayList multiParam = new ArrayList();
try
{
generateOutputParameters(subParams, usedParameters, multiParam);
outputParameters.addAll(multiParam);
}
catch (BuildException e)
{
throw e;
}
catch (Exception e)
{
// If one of the parameters in the multi-param block is not defined
// then ignore this block
}
}
else
{
try
{
generateOutputParameters(_outParameters.get(count), usedParameters, outputParameters);
}
catch (BuildException e)
{
throw e;
}
catch (Exception e)
{
// If one of the parameters in the multi-param block is not defined
// then ignore this block
}
}
}
String[] returnArray = new String[outputParameters.size()];
System.arraycopy(outputParameters.toArray(), 0, returnArray, 0, outputParameters.size());
return (returnArray);
}
private String createClasspath( String value, String delimiter )
{
String returnValue = "";
StringTokenizer st = new StringTokenizer(value, delimiter);
while ( st.hasMoreTokens() )
{
returnValue += (String)st.nextToken();
if ( st.hasMoreTokens() )
{
returnValue += File.pathSeparatorChar;
}
}
return returnValue;
}
private void generateOutputParameters(Object forEachKey, HashSet usedParameters, ArrayList outputParameters) throws Exception
{
String forEach = (String)_forEachMapping.get( forEachKey );
if (forEach == null)
{
if ( forEachKey instanceof ArrayList )
{
ArrayList al = (ArrayList)forEachKey;
for (int count=0;count');
}
public boolean isValid()
{
return (_valid);
}
private boolean getNextElement()
{
boolean returnValue = false;
if ((_valid) && (_buildList.indexOf('<') != -1))
{
String element = _buildList.substring(_buildList.indexOf('<') + 1);
element = element.substring(0, element.indexOf('>'));
_currentSrc = element.substring(element.indexOf('\'') + 1);
_currentSrc = _currentSrc.substring(0, _currentSrc.indexOf('\''));
element = element.substring(element.indexOf(',') + 1);
_currentPackage = element.substring(element.indexOf('\'') + 1);
_currentPackage = _currentPackage.substring(0, _currentPackage.indexOf('\''));
element = element.substring(element.indexOf(',') + 1);
_currentMapping = element.substring(element.indexOf('\'') + 1);
_currentMapping = _currentMapping.substring(0, _currentMapping.indexOf('\''));
_buildList = _buildList.substring(1).substring(_buildList.indexOf('>'));
returnValue = true;
}
return (returnValue);
}
public String getSource()
{
return (_currentSrc);
}
public String getPackage()
{
return (_currentPackage);
}
public String getMappings()
{
return (_currentMapping);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy