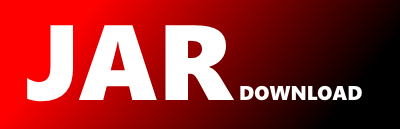
com.arjuna.ArjunaOTS._ArjunaFactoryStub Maven / Gradle / Ivy
package com.arjuna.ArjunaOTS;
/**
* Generated from IDL interface "ArjunaFactory".
*
* @author JacORB IDL compiler V 2.3.1 (JBoss patch01), 29-Jul-2009
* @version generated at Oct 2, 2012 3:07:46 PM
*/
public class _ArjunaFactoryStub
extends org.omg.CORBA.portable.ObjectImpl
implements com.arjuna.ArjunaOTS.ArjunaFactory
{
private String[] ids = {"IDL:arjuna.com/ArjunaOTS/ArjunaFactory:1.0","IDL:omg.org/CosTransactions/TransactionFactory:1.0"};
public String[] _ids()
{
return ids;
}
public final static java.lang.Class _opsClass = com.arjuna.ArjunaOTS.ArjunaFactoryOperations.class;
public org.omg.CosTransactions.Status getCurrentStatus(org.omg.CosTransactions.otid_t uid) throws org.omg.CosTransactions.NoTransaction
{
while(true)
{
if(! this._is_local())
{
org.omg.CORBA.portable.InputStream _is = null;
org.omg.CORBA.portable.OutputStream _os = null;
try
{
_os = _request( "getCurrentStatus", true);
org.omg.CosTransactions.otid_tHelper.write(_os,uid);
_is = _invoke(_os);
org.omg.CosTransactions.Status _result = org.omg.CosTransactions.StatusHelper.read(_is);
return _result;
}
catch( org.omg.CORBA.portable.RemarshalException _rx ){}
catch( org.omg.CORBA.portable.ApplicationException _ax )
{
String _id = _ax.getId();
try
{
if( _id.equals("IDL:omg.org/CosTransactions/NoTransaction:1.0"))
{
throw org.omg.CosTransactions.NoTransactionHelper.read(_ax.getInputStream());
}
else
{
throw new RuntimeException("Unexpected exception " + _id );
}
}
finally
{
try
{
_ax.getInputStream().close();
}
catch (java.io.IOException e)
{
throw new RuntimeException("Unexpected exception " + e.toString() );
}
}
}
finally
{
if (_os != null)
{
try
{
_os.close();
}
catch (java.io.IOException e)
{
throw new RuntimeException("Unexpected exception " + e.toString() );
}
}
this._releaseReply(_is);
}
}
else
{
org.omg.CORBA.portable.ServantObject _so = _servant_preinvoke( "getCurrentStatus", _opsClass );
if( _so == null )
throw new org.omg.CORBA.UNKNOWN("local invocations not supported!");
ArjunaFactoryOperations _localServant = (ArjunaFactoryOperations)_so.servant;
org.omg.CosTransactions.Status _result;
try
{
_result = _localServant.getCurrentStatus(uid);
}
finally
{
_servant_postinvoke(_so);
}
return _result;
}
}
}
public org.omg.CosTransactions.Control getTransaction(org.omg.CosTransactions.otid_t uid) throws org.omg.CosTransactions.NoTransaction
{
while(true)
{
if(! this._is_local())
{
org.omg.CORBA.portable.InputStream _is = null;
org.omg.CORBA.portable.OutputStream _os = null;
try
{
_os = _request( "getTransaction", true);
org.omg.CosTransactions.otid_tHelper.write(_os,uid);
_is = _invoke(_os);
org.omg.CosTransactions.Control _result = org.omg.CosTransactions.ControlHelper.read(_is);
return _result;
}
catch( org.omg.CORBA.portable.RemarshalException _rx ){}
catch( org.omg.CORBA.portable.ApplicationException _ax )
{
String _id = _ax.getId();
try
{
if( _id.equals("IDL:omg.org/CosTransactions/NoTransaction:1.0"))
{
throw org.omg.CosTransactions.NoTransactionHelper.read(_ax.getInputStream());
}
else
{
throw new RuntimeException("Unexpected exception " + _id );
}
}
finally
{
try
{
_ax.getInputStream().close();
}
catch (java.io.IOException e)
{
throw new RuntimeException("Unexpected exception " + e.toString() );
}
}
}
finally
{
if (_os != null)
{
try
{
_os.close();
}
catch (java.io.IOException e)
{
throw new RuntimeException("Unexpected exception " + e.toString() );
}
}
this._releaseReply(_is);
}
}
else
{
org.omg.CORBA.portable.ServantObject _so = _servant_preinvoke( "getTransaction", _opsClass );
if( _so == null )
throw new org.omg.CORBA.UNKNOWN("local invocations not supported!");
ArjunaFactoryOperations _localServant = (ArjunaFactoryOperations)_so.servant;
org.omg.CosTransactions.Control _result;
try
{
_result = _localServant.getTransaction(uid);
}
finally
{
_servant_postinvoke(_so);
}
return _result;
}
}
}
public org.omg.CosTransactions.otid_t[] numberOfTransactions(com.arjuna.ArjunaOTS.TransactionType t) throws org.omg.CosTransactions.NoTransaction,org.omg.CosTransactions.Inactive
{
while(true)
{
if(! this._is_local())
{
org.omg.CORBA.portable.InputStream _is = null;
org.omg.CORBA.portable.OutputStream _os = null;
try
{
_os = _request( "numberOfTransactions", true);
com.arjuna.ArjunaOTS.TransactionTypeHelper.write(_os,t);
_is = _invoke(_os);
org.omg.CosTransactions.otid_t[] _result = com.arjuna.ArjunaOTS.txIdsHelper.read(_is);
return _result;
}
catch( org.omg.CORBA.portable.RemarshalException _rx ){}
catch( org.omg.CORBA.portable.ApplicationException _ax )
{
String _id = _ax.getId();
try
{
if( _id.equals("IDL:omg.org/CosTransactions/NoTransaction:1.0"))
{
throw org.omg.CosTransactions.NoTransactionHelper.read(_ax.getInputStream());
}
else
if( _id.equals("IDL:omg.org/CosTransactions/Inactive:1.0"))
{
throw org.omg.CosTransactions.InactiveHelper.read(_ax.getInputStream());
}
else
{
throw new RuntimeException("Unexpected exception " + _id );
}
}
finally
{
try
{
_ax.getInputStream().close();
}
catch (java.io.IOException e)
{
throw new RuntimeException("Unexpected exception " + e.toString() );
}
}
}
finally
{
if (_os != null)
{
try
{
_os.close();
}
catch (java.io.IOException e)
{
throw new RuntimeException("Unexpected exception " + e.toString() );
}
}
this._releaseReply(_is);
}
}
else
{
org.omg.CORBA.portable.ServantObject _so = _servant_preinvoke( "numberOfTransactions", _opsClass );
if( _so == null )
throw new org.omg.CORBA.UNKNOWN("local invocations not supported!");
ArjunaFactoryOperations _localServant = (ArjunaFactoryOperations)_so.servant;
org.omg.CosTransactions.otid_t[] _result;
try
{
_result = _localServant.numberOfTransactions(t);
}
finally
{
_servant_postinvoke(_so);
}
return _result;
}
}
}
public com.arjuna.ArjunaOTS.GlobalTransactionInfo getGlobalInfo()
{
while(true)
{
if(! this._is_local())
{
org.omg.CORBA.portable.InputStream _is = null;
org.omg.CORBA.portable.OutputStream _os = null;
try
{
_os = _request( "getGlobalInfo", true);
_is = _invoke(_os);
com.arjuna.ArjunaOTS.GlobalTransactionInfo _result = com.arjuna.ArjunaOTS.GlobalTransactionInfoHelper.read(_is);
return _result;
}
catch( org.omg.CORBA.portable.RemarshalException _rx ){}
catch( org.omg.CORBA.portable.ApplicationException _ax )
{
String _id = _ax.getId();
try
{
_ax.getInputStream().close();
}
catch (java.io.IOException e)
{
throw new RuntimeException("Unexpected exception " + e.toString() );
}
throw new RuntimeException("Unexpected exception " + _id );
}
finally
{
if (_os != null)
{
try
{
_os.close();
}
catch (java.io.IOException e)
{
throw new RuntimeException("Unexpected exception " + e.toString() );
}
}
this._releaseReply(_is);
}
}
else
{
org.omg.CORBA.portable.ServantObject _so = _servant_preinvoke( "getGlobalInfo", _opsClass );
if( _so == null )
throw new org.omg.CORBA.UNKNOWN("local invocations not supported!");
ArjunaFactoryOperations _localServant = (ArjunaFactoryOperations)_so.servant;
com.arjuna.ArjunaOTS.GlobalTransactionInfo _result;
try
{
_result = _localServant.getGlobalInfo();
}
finally
{
_servant_postinvoke(_so);
}
return _result;
}
}
}
public org.omg.CosTransactions.Status getStatus(org.omg.CosTransactions.otid_t uid) throws org.omg.CosTransactions.NoTransaction
{
while(true)
{
if(! this._is_local())
{
org.omg.CORBA.portable.InputStream _is = null;
org.omg.CORBA.portable.OutputStream _os = null;
try
{
_os = _request( "getStatus", true);
org.omg.CosTransactions.otid_tHelper.write(_os,uid);
_is = _invoke(_os);
org.omg.CosTransactions.Status _result = org.omg.CosTransactions.StatusHelper.read(_is);
return _result;
}
catch( org.omg.CORBA.portable.RemarshalException _rx ){}
catch( org.omg.CORBA.portable.ApplicationException _ax )
{
String _id = _ax.getId();
try
{
if( _id.equals("IDL:omg.org/CosTransactions/NoTransaction:1.0"))
{
throw org.omg.CosTransactions.NoTransactionHelper.read(_ax.getInputStream());
}
else
{
throw new RuntimeException("Unexpected exception " + _id );
}
}
finally
{
try
{
_ax.getInputStream().close();
}
catch (java.io.IOException e)
{
throw new RuntimeException("Unexpected exception " + e.toString() );
}
}
}
finally
{
if (_os != null)
{
try
{
_os.close();
}
catch (java.io.IOException e)
{
throw new RuntimeException("Unexpected exception " + e.toString() );
}
}
this._releaseReply(_is);
}
}
else
{
org.omg.CORBA.portable.ServantObject _so = _servant_preinvoke( "getStatus", _opsClass );
if( _so == null )
throw new org.omg.CORBA.UNKNOWN("local invocations not supported!");
ArjunaFactoryOperations _localServant = (ArjunaFactoryOperations)_so.servant;
org.omg.CosTransactions.Status _result;
try
{
_result = _localServant.getStatus(uid);
}
finally
{
_servant_postinvoke(_so);
}
return _result;
}
}
}
public org.omg.CosTransactions.otid_t[] getChildTransactions(org.omg.CosTransactions.otid_t parent) throws org.omg.CosTransactions.NoTransaction,org.omg.CosTransactions.Inactive
{
while(true)
{
if(! this._is_local())
{
org.omg.CORBA.portable.InputStream _is = null;
org.omg.CORBA.portable.OutputStream _os = null;
try
{
_os = _request( "getChildTransactions", true);
org.omg.CosTransactions.otid_tHelper.write(_os,parent);
_is = _invoke(_os);
org.omg.CosTransactions.otid_t[] _result = com.arjuna.ArjunaOTS.txIdsHelper.read(_is);
return _result;
}
catch( org.omg.CORBA.portable.RemarshalException _rx ){}
catch( org.omg.CORBA.portable.ApplicationException _ax )
{
String _id = _ax.getId();
try
{
if( _id.equals("IDL:omg.org/CosTransactions/NoTransaction:1.0"))
{
throw org.omg.CosTransactions.NoTransactionHelper.read(_ax.getInputStream());
}
else
if( _id.equals("IDL:omg.org/CosTransactions/Inactive:1.0"))
{
throw org.omg.CosTransactions.InactiveHelper.read(_ax.getInputStream());
}
else
{
throw new RuntimeException("Unexpected exception " + _id );
}
}
finally
{
try
{
_ax.getInputStream().close();
}
catch (java.io.IOException e)
{
throw new RuntimeException("Unexpected exception " + e.toString() );
}
}
}
finally
{
if (_os != null)
{
try
{
_os.close();
}
catch (java.io.IOException e)
{
throw new RuntimeException("Unexpected exception " + e.toString() );
}
}
this._releaseReply(_is);
}
}
else
{
org.omg.CORBA.portable.ServantObject _so = _servant_preinvoke( "getChildTransactions", _opsClass );
if( _so == null )
throw new org.omg.CORBA.UNKNOWN("local invocations not supported!");
ArjunaFactoryOperations _localServant = (ArjunaFactoryOperations)_so.servant;
org.omg.CosTransactions.otid_t[] _result;
try
{
_result = _localServant.getChildTransactions(parent);
}
finally
{
_servant_postinvoke(_so);
}
return _result;
}
}
}
public org.omg.CosTransactions.Control recreate(org.omg.CosTransactions.PropagationContext ctx)
{
while(true)
{
if(! this._is_local())
{
org.omg.CORBA.portable.InputStream _is = null;
org.omg.CORBA.portable.OutputStream _os = null;
try
{
_os = _request( "recreate", true);
org.omg.CosTransactions.PropagationContextHelper.write(_os,ctx);
_is = _invoke(_os);
org.omg.CosTransactions.Control _result = org.omg.CosTransactions.ControlHelper.read(_is);
return _result;
}
catch( org.omg.CORBA.portable.RemarshalException _rx ){}
catch( org.omg.CORBA.portable.ApplicationException _ax )
{
String _id = _ax.getId();
try
{
_ax.getInputStream().close();
}
catch (java.io.IOException e)
{
throw new RuntimeException("Unexpected exception " + e.toString() );
}
throw new RuntimeException("Unexpected exception " + _id );
}
finally
{
if (_os != null)
{
try
{
_os.close();
}
catch (java.io.IOException e)
{
throw new RuntimeException("Unexpected exception " + e.toString() );
}
}
this._releaseReply(_is);
}
}
else
{
org.omg.CORBA.portable.ServantObject _so = _servant_preinvoke( "recreate", _opsClass );
if( _so == null )
throw new org.omg.CORBA.UNKNOWN("local invocations not supported!");
ArjunaFactoryOperations _localServant = (ArjunaFactoryOperations)_so.servant;
org.omg.CosTransactions.Control _result;
try
{
_result = _localServant.recreate(ctx);
}
finally
{
_servant_postinvoke(_so);
}
return _result;
}
}
}
public com.arjuna.ArjunaOTS.TransactionInfo getTransactionInfo(org.omg.CosTransactions.otid_t uid) throws org.omg.CosTransactions.NoTransaction
{
while(true)
{
if(! this._is_local())
{
org.omg.CORBA.portable.InputStream _is = null;
org.omg.CORBA.portable.OutputStream _os = null;
try
{
_os = _request( "getTransactionInfo", true);
org.omg.CosTransactions.otid_tHelper.write(_os,uid);
_is = _invoke(_os);
com.arjuna.ArjunaOTS.TransactionInfo _result = com.arjuna.ArjunaOTS.TransactionInfoHelper.read(_is);
return _result;
}
catch( org.omg.CORBA.portable.RemarshalException _rx ){}
catch( org.omg.CORBA.portable.ApplicationException _ax )
{
String _id = _ax.getId();
try
{
if( _id.equals("IDL:omg.org/CosTransactions/NoTransaction:1.0"))
{
throw org.omg.CosTransactions.NoTransactionHelper.read(_ax.getInputStream());
}
else
{
throw new RuntimeException("Unexpected exception " + _id );
}
}
finally
{
try
{
_ax.getInputStream().close();
}
catch (java.io.IOException e)
{
throw new RuntimeException("Unexpected exception " + e.toString() );
}
}
}
finally
{
if (_os != null)
{
try
{
_os.close();
}
catch (java.io.IOException e)
{
throw new RuntimeException("Unexpected exception " + e.toString() );
}
}
this._releaseReply(_is);
}
}
else
{
org.omg.CORBA.portable.ServantObject _so = _servant_preinvoke( "getTransactionInfo", _opsClass );
if( _so == null )
throw new org.omg.CORBA.UNKNOWN("local invocations not supported!");
ArjunaFactoryOperations _localServant = (ArjunaFactoryOperations)_so.servant;
com.arjuna.ArjunaOTS.TransactionInfo _result;
try
{
_result = _localServant.getTransactionInfo(uid);
}
finally
{
_servant_postinvoke(_so);
}
return _result;
}
}
}
public org.omg.CosTransactions.Control create(int time_out)
{
while(true)
{
if(! this._is_local())
{
org.omg.CORBA.portable.InputStream _is = null;
org.omg.CORBA.portable.OutputStream _os = null;
try
{
_os = _request( "create", true);
_os.write_ulong(time_out);
_is = _invoke(_os);
org.omg.CosTransactions.Control _result = org.omg.CosTransactions.ControlHelper.read(_is);
return _result;
}
catch( org.omg.CORBA.portable.RemarshalException _rx ){}
catch( org.omg.CORBA.portable.ApplicationException _ax )
{
String _id = _ax.getId();
try
{
_ax.getInputStream().close();
}
catch (java.io.IOException e)
{
throw new RuntimeException("Unexpected exception " + e.toString() );
}
throw new RuntimeException("Unexpected exception " + _id );
}
finally
{
if (_os != null)
{
try
{
_os.close();
}
catch (java.io.IOException e)
{
throw new RuntimeException("Unexpected exception " + e.toString() );
}
}
this._releaseReply(_is);
}
}
else
{
org.omg.CORBA.portable.ServantObject _so = _servant_preinvoke( "create", _opsClass );
if( _so == null )
throw new org.omg.CORBA.UNKNOWN("local invocations not supported!");
ArjunaFactoryOperations _localServant = (ArjunaFactoryOperations)_so.servant;
org.omg.CosTransactions.Control _result;
try
{
_result = _localServant.create(time_out);
}
finally
{
_servant_postinvoke(_so);
}
return _result;
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy