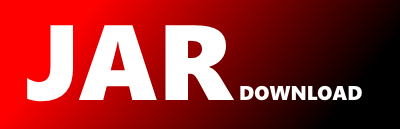
org.jboss.logging.log4j.Log4jLoggerPlugin Maven / Gradle / Ivy
/*
* JBoss, Home of Professional Open Source
* Copyright 2005, JBoss Inc., and individual contributors as indicated
* by the @authors tag. See the copyright.txt in the distribution for a
* full listing of individual contributors.
*
* This is free software; you can redistribute it and/or modify it
* under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation; either version 2.1 of
* the License, or (at your option) any later version.
*
* This software is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this software; if not, write to the Free
* Software Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA
* 02110-1301 USA, or see the FSF site: http://www.fsf.org.
*/
package org.jboss.logging.log4j;
import org.jboss.logging.LoggerPlugin;
import org.jboss.logging.MDCProvider;
import org.jboss.logging.MDCSupport;
import org.jboss.logging.NDCProvider;
import org.jboss.logging.NDCSupport;
import org.apache.log4j.Category;
import org.apache.log4j.Level;
import org.apache.log4j.LogManager;
import org.apache.log4j.Priority;
/**
* Delegate for org.jboss.logging.Logger logging to log4j. Body of implementation
* mainly copied from old Logger implementation.
*
* @see org.jboss.logging.Logger
* @see org.jboss.logging.LoggerPlugin
*
* @author Sacha Labourey.
* @author Jason T. Greene
* @version $Revision: 3150 $
*/
public class Log4jLoggerPlugin implements LoggerPlugin, NDCSupport, MDCSupport
{
// Constants -----------------------------------------------------
/**
* Fully qualified classname for this class so Log4J locationinfo will be
* correct
*/
private static final String FQCN = Log4jLoggerPlugin.class.getName();
// Attributes ----------------------------------------------------
/** The Log4j delegate logger. */
private transient org.apache.log4j.Logger log;
// Static --------------------------------------------------------
// Constructors --------------------------------------------------
public Log4jLoggerPlugin () { }
public void init (String name)
{
log = LogManager.getLogger(name);
}
// Public --------------------------------------------------------
public Category getCategory()
{
return log;
}
/**
* Exposes the delegate Log4j Logger.
*
* @return the underlying logger
*/
public org.apache.log4j.Logger getLogger()
{
return log;
}
// LoggerPlugin implementation ----------------------------------------------
public boolean isTraceEnabled()
{
Level l = Level.TRACE;
if (log.isEnabledFor(l) == false)
return false;
return l.isGreaterOrEqual(log.getEffectiveLevel());
}
/**
* Issue a log msg with a level of TRACE.
* Invokes log.log(Level.TRACE, message);
*/
public void trace(Object message)
{
log.log(FQCN, Level.TRACE, message, null);
}
/**
* Issue a log msg and throwable with a level of TRACE.
* Invokes log.log(Level.TRACE, message, t);
*/
public void trace(Object message, Throwable t)
{
log.log(FQCN, Level.TRACE, message, t);
}
/** {@inheritDoc} */
public void trace(final String loggerFcqn, final Object message, final Throwable t)
{
log.log(loggerFcqn, Level.TRACE, message, t);
}
/**
* Check to see if the TRACE level is enabled for this logger.
*
* @return true if a {@link #trace(Object)} method invocation would pass
* the msg to the configured appenders, false otherwise.
*/
@Deprecated
public boolean isDebugEnabled()
{
Level l = Level.DEBUG;
if (log.isEnabledFor(l) == false)
return false;
return l.isGreaterOrEqual(log.getEffectiveLevel());
}
/**
* Issue a log msg with a level of DEBUG.
* Invokes log.log(Level.DEBUG, message);
*/
public void debug(Object message)
{
log.log(FQCN, Level.DEBUG, message, null);
}
/**
* Issue a log msg and throwable with a level of DEBUG.
* Invokes log.log(Level.DEBUG, message, t);
*/
public void debug(Object message, Throwable t)
{
log.log(FQCN, Level.DEBUG, message, t);
}
/** {@inheritDoc} */
public void debug(final String loggerFcqn, final Object message, final Throwable t)
{
log.log(loggerFcqn, Level.DEBUG, message, t);
}
/**
* Check to see if the INFO level is enabled for this logger.
*
* @return true if a {@link #info(Object)} method invocation would pass
* the msg to the configured appenders, false otherwise.
*/
@Deprecated
public boolean isInfoEnabled()
{
Level l = Level.INFO;
if (log.isEnabledFor(l) == false)
return false;
return l.isGreaterOrEqual(log.getEffectiveLevel());
}
/**
* Issue a log msg with a level of INFO.
* Invokes log.log(Level.INFO, message);
*/
public void info(Object message)
{
log.log(FQCN, Level.INFO, message, null);
}
/**
* Issue a log msg and throwable with a level of INFO.
* Invokes log.log(Level.INFO, message, t);
*/
public void info(Object message, Throwable t)
{
log.log(FQCN, Level.INFO, message, t);
}
/** {@inheritDoc} */
public void info(final String loggerFcqn, final Object message, final Throwable t)
{
log.log(loggerFcqn, Level.INFO, message, t);
}
/**
* Issue a log msg with a level of WARN.
* Invokes log.log(Level.WARN, message);
*/
public void warn(Object message)
{
log.log(FQCN, Level.WARN, message, null);
}
/**
* Issue a log msg and throwable with a level of WARN.
* Invokes log.log(Level.WARN, message, t);
*/
public void warn(Object message, Throwable t)
{
log.log(FQCN, Level.WARN, message, t);
}
/** {@inheritDoc} */
public void warn(final String loggerFcqn, final Object message, final Throwable t)
{
log.log(loggerFcqn, Level.WARN, message, t);
}
/**
* Issue a log msg with a level of ERROR.
* Invokes log.log(Level.ERROR, message);
*/
public void error(Object message)
{
log.log(FQCN, Level.ERROR, message, null);
}
/**
* Issue a log msg and throwable with a level of ERROR.
* Invokes log.log(Level.ERROR, message, t);
*/
public void error(Object message, Throwable t)
{
log.log(FQCN, Level.ERROR, message, t);
}
/** {@inheritDoc} */
public void error(final String loggerFcqn, final Object message, final Throwable t)
{
log.log(loggerFcqn, Level.ERROR, message, t);
}
/**
* Issue a log msg with a level of FATAL.
* Invokes log.log(Level.FATAL, message);
*/
public void fatal(Object message)
{
log.log(FQCN, Level.FATAL, message, null);
}
/**
* Issue a log msg and throwable with a level of FATAL.
* Invokes log.log(Level.FATAL, message, t);
*/
public void fatal(Object message, Throwable t)
{
log.log(FQCN, Level.FATAL, message, t);
}
/** {@inheritDoc} */
public void fatal(final String loggerFcqn, final Object message, final Throwable t)
{
log.log(loggerFcqn, Level.FATAL, message, t);
}
/**
* Issue a log msg with the given level.
* Invokes log.log(p, message);
*
* @param p the priority
* @param message the message
* @deprecated Use Level versions.
*/
public void log(Priority p, Object message)
{
log.log(FQCN, p, message, null);
}
/**
* Issue a log msg with the given priority.
* Invokes log.log(p, message, t);
*
*
* @param p the priority
* @param message the message
* @param t the throwable
* @deprecated Use Level versions.
*/
public void log(Priority p, Object message, Throwable t)
{
log.log(FQCN, p, message, t);
}
/**
* Issue a log msg with the given level.
* Invokes log.log(l, message);
*
* @param l the level
* @param message the message
*/
public void log(Level l, Object message)
{
log.log(FQCN, l, message, null);
}
/**
* Issue a log msg with the given level.
* Invokes log.log(l, message, t);
*
* @param l the level
* @param message the message
* @param t the throwable
*/
public void log(Level l, Object message, Throwable t)
{
log.log(FQCN, l, message, t);
}
public NDCProvider getNDCProvider()
{
return new Log4jNDCProvider();
}
public MDCProvider getMDCProvider()
{
return new Log4jMDCProvider();
}
// Y overrides ---------------------------------------------------
// Package protected ---------------------------------------------
// Protected -----------------------------------------------------
// Private -------------------------------------------------------
// Inner classes -------------------------------------------------
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy