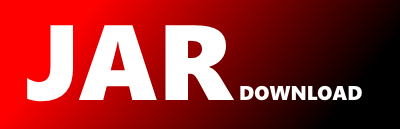
org.jboss.logmanager.JDKSpecific Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jboss-logmanager Show documentation
Show all versions of jboss-logmanager Show documentation
An implementation of java.util.logging.LogManager
The newest version!
/*
* JBoss, Home of Professional Open Source.
*
* Copyright 2022 Red Hat, Inc., and individual contributors
* as indicated by the @author tags.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.jboss.logmanager;
import static java.security.AccessController.doPrivileged;
import java.lang.module.ModuleDescriptor;
import java.security.PrivilegedAction;
import java.util.EnumSet;
import java.util.Iterator;
import java.util.Optional;
import java.util.Set;
import java.util.function.Function;
import java.util.stream.Stream;
import org.jboss.modules.Module;
import org.jboss.modules.Version;
/**
* @author David M. Lloyd
*/
final class JDKSpecific {
static final StackWalker WALKER = doPrivileged(new GetStackWalkerAction());
private JDKSpecific() {
}
private static final boolean JBOSS_MODULES;
static {
boolean jbossModules = false;
try {
//noinspection ResultOfMethodCallIgnored
Module.getStartTime();
jbossModules = true;
} catch (Throwable ignored) {
}
JBOSS_MODULES = jbossModules;
}
static Class> findCallingClass(Set rejectClassLoaders) {
final SecurityManager sm = System.getSecurityManager();
if (sm != null) {
return doPrivileged(new FindCallingClassAction(rejectClassLoaders));
} else {
return WALKER.walk(new FindFirstWalkFunction(rejectClassLoaders));
}
}
static LogContext logContextFinder(Set rejectClassLoaders,
final Function finder) {
final SecurityManager sm = System.getSecurityManager();
if (sm != null) {
return doPrivileged(new FindCallingClassesAction(rejectClassLoaders, finder));
} else {
return WALKER.walk(new FindAllWalkFunction(rejectClassLoaders, finder));
}
}
static void calculateCaller(ExtLogRecord logRecord) {
WALKER.walk(new CallerCalcFunction(logRecord));
}
private static void calculateJdkModule(final ExtLogRecord logRecord, final Class> clazz) {
final java.lang.Module module = clazz.getModule();
if (module != null) {
logRecord.setSourceModuleName(module.getName());
final ModuleDescriptor descriptor = module.getDescriptor();
if (descriptor != null) {
final Optional optional = descriptor.version();
if (optional.isPresent()) {
logRecord.setSourceModuleVersion(optional.get().toString());
} else {
logRecord.setSourceModuleVersion(null);
}
}
}
}
private static void calculateModule(final ExtLogRecord logRecord, final Class> clazz) {
final Module module = Module.forClass(clazz);
if (module != null) {
logRecord.setSourceModuleName(module.getName());
final Version version = module.getVersion();
if (version != null) {
logRecord.setSourceModuleVersion(version.toString());
} else {
logRecord.setSourceModuleVersion(null);
}
} else {
calculateJdkModule(logRecord, clazz);
}
}
private static final class CallerCalcFunction implements Function, Void> {
private final ExtLogRecord logRecord;
CallerCalcFunction(final ExtLogRecord logRecord) {
this.logRecord = logRecord;
}
public Void apply(final Stream stream) {
final String loggerClassName = logRecord.getLoggerClassName();
final Iterator iterator = stream.iterator();
boolean found = false;
while (iterator.hasNext()) {
final StackWalker.StackFrame frame = iterator.next();
final Class> clazz = frame.getDeclaringClass();
if (clazz.getName().equals(loggerClassName)) {
// next entry could be the one we want!
found = true;
} else if (found) {
logRecord.setSourceClassName(frame.getClassName());
logRecord.setSourceMethodName(frame.getMethodName());
logRecord.setSourceFileName(frame.getFileName());
logRecord.setSourceLineNumber(frame.getLineNumber());
if (JBOSS_MODULES) {
calculateModule(logRecord, clazz);
} else {
calculateJdkModule(logRecord, clazz);
}
return null;
}
}
logRecord.setUnknownCaller();
return null;
}
}
private static final class GetStackWalkerAction implements PrivilegedAction {
GetStackWalkerAction() {
}
public StackWalker run() {
return StackWalker.getInstance(EnumSet.of(StackWalker.Option.RETAIN_CLASS_REFERENCE));
}
}
private static final class FindCallingClassAction implements PrivilegedAction> {
private final Set rejectClassLoaders;
FindCallingClassAction(final Set rejectClassLoaders) {
this.rejectClassLoaders = rejectClassLoaders;
}
public Class> run() {
return WALKER.walk(new FindFirstWalkFunction(rejectClassLoaders));
}
}
private static final class FindCallingClassesAction implements PrivilegedAction {
private final Set rejectClassLoaders;
private final Function finder;
FindCallingClassesAction(final Set rejectClassLoaders,
final Function finder) {
this.rejectClassLoaders = rejectClassLoaders;
this.finder = finder;
}
public LogContext run() {
return WALKER.walk(new FindAllWalkFunction(rejectClassLoaders, finder));
}
}
private static final class FindFirstWalkFunction implements Function, Class>> {
private final Set rejectClassLoaders;
FindFirstWalkFunction(final Set rejectClassLoaders) {
this.rejectClassLoaders = rejectClassLoaders;
}
public Class> apply(final Stream stream) {
final Iterator iterator = stream.iterator();
while (iterator.hasNext()) {
final Class> clazz = iterator.next().getDeclaringClass();
final ClassLoader classLoader = clazz.getClassLoader();
if (!rejectClassLoaders.contains(classLoader)) {
return clazz;
}
}
return null;
}
}
private static final class FindAllWalkFunction implements Function, LogContext> {
private final Set rejectClassLoaders;
private final Function finder;
FindAllWalkFunction(final Set rejectClassLoaders, final Function finder) {
this.rejectClassLoaders = rejectClassLoaders;
this.finder = finder;
}
@Override
public LogContext apply(final Stream stream) {
final Iterator iterator = stream.iterator();
while (iterator.hasNext()) {
final Class> clazz = iterator.next().getDeclaringClass();
final ClassLoader classLoader = clazz.getClassLoader();
if (classLoader != null && !rejectClassLoaders.contains(classLoader)) {
final LogContext result = finder.apply(classLoader);
if (result != null) {
return result;
}
}
}
return null;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy