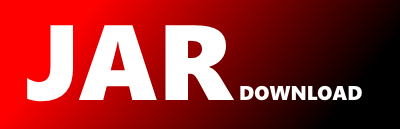
io.narayana.lra.arquillian.deployment.scenario.ScenarioGeneratorBase Maven / Gradle / Ivy
/*
Copyright The Narayana Authors
SPDX-License-Identifier: Apache-2.0
*/
package io.narayana.lra.arquillian.deployment.scenario;
import io.narayana.lra.arquillian.deployment.Deployment;
import org.jboss.arquillian.config.descriptor.api.ArquillianDescriptor;
import org.jboss.arquillian.config.descriptor.api.ContainerDef;
import org.jboss.arquillian.config.descriptor.api.ExtensionDef;
import org.jboss.arquillian.config.descriptor.api.GroupDef;
import org.jboss.arquillian.core.api.Instance;
import org.jboss.arquillian.core.api.annotation.Inject;
import org.jboss.logging.Logger;
import java.lang.reflect.Method;
import java.util.Arrays;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import java.util.stream.Collectors;
/**
*
* This class is composed by a collection of methods useful to classes implementing
* the interface DeploymentScenarioGenerator. In particular, this class represents
* a main point of control when it comes to classes that use the "extension" section
* in the Arquillian.xml file. In fact, this class supplies basic methods to identify
* a particular extension section and handle properties defined in it.
*
*
* The concept behind this class comes from the project deploymentscenario
, which can be found
* among the showcases of Arquillian.
*
* @see Arquillian Deployment Scenario
*/
public class ScenarioGeneratorBase {
static final Logger log = Logger.getLogger(ScenarioGeneratorBase.class);
@Inject
Instance arquillianDescriptorInstance;
/**
* This method return a {@link Map} representing the properties defined in the extension
* section and identifiable with the parameter extensionName
* @return {@link Map} representing a set of properties
*/
Map getExtensionProperties() {
ArquillianDescriptor arquillianDescriptor = arquillianDescriptorInstance.get();
Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy