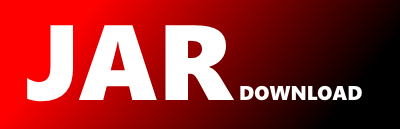
org.jboss.netty.handler.codec.http.HttpVersion Maven / Gradle / Ivy
Go to download
The Netty project is an effort to provide an asynchronous event-driven
network application framework and tools for rapid development of
maintainable high performance and high scalability protocol servers and
clients. In other words, Netty is a NIO client server framework which
enables quick and easy development of network applications such as protocol
servers and clients. It greatly simplifies and streamlines network
programming such as TCP and UDP socket server.
/*
* JBoss, Home of Professional Open Source
* Copyright 2005-2008, Red Hat Middleware LLC, and individual contributors
* by the @authors tag. See the copyright.txt in the distribution for a
* full listing of individual contributors.
*
* This is free software; you can redistribute it and/or modify it
* under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation; either version 2.1 of
* the License, or (at your option) any later version.
*
* This software is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this software; if not, write to the Free
* Software Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA
* 02110-1301 USA, or see the FSF site: http://www.fsf.org.
*/
package org.jboss.netty.handler.codec.http;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
/**
* The protocols we support;
*
* @author The Netty Project ([email protected])
* @author Andy Taylor ([email protected])
* @author Trustin Lee ([email protected])
* @version $Rev: 838 $, $Date: 2009-02-12 14:02:22 +0900 (Thu, 12 Feb 2009) $
*
* @apiviz.exclude
*/
public class HttpVersion implements Comparable {
private static final Pattern VERSION_PATTERN =
Pattern.compile("(\\S+)/(\\d+)\\.(\\d+)");
public static final HttpVersion HTTP_1_0 = new HttpVersion("HTTP", 1, 0);
public static final HttpVersion HTTP_1_1 = new HttpVersion("HTTP", 1, 1);
public static HttpVersion valueOf(String text) {
if (text == null) {
throw new NullPointerException("text");
}
text = text.trim().toUpperCase();
if (text.equals("HTTP/1.1")) {
return HTTP_1_1;
}
if (text.equals("HTTP/1.0")) {
return HTTP_1_0;
}
return new HttpVersion(text);
}
private final String protocolName;
private final int majorVersion;
private final int minorVersion;
private final String text;
public HttpVersion(String text) {
if (text == null) {
throw new NullPointerException("text");
}
text = text.trim().toUpperCase();
if (text.length() == 0) {
throw new IllegalArgumentException("empty text");
}
Matcher m = VERSION_PATTERN.matcher(text);
if (!m.matches()) {
throw new IllegalArgumentException("invalid version format: " + text);
}
protocolName = m.group(1);
majorVersion = Integer.parseInt(m.group(2));
minorVersion = Integer.parseInt(m.group(3));
this.text = protocolName + '/' + majorVersion + '.' + minorVersion;
}
public HttpVersion(
String protocolName, int majorVersion, int minorVersion) {
if (protocolName == null) {
throw new NullPointerException("protocolName");
}
protocolName = protocolName.trim().toUpperCase();
if (protocolName.length() == 0) {
throw new IllegalArgumentException("empty protocolName");
}
for (int i = 0; i < protocolName.length(); i ++) {
if (Character.isISOControl(protocolName.charAt(i)) ||
Character.isWhitespace(protocolName.charAt(i))) {
throw new IllegalArgumentException("invalid character in protocolName");
}
}
if (majorVersion < 0) {
throw new IllegalArgumentException("negative majorVersion");
}
if (minorVersion < 0) {
throw new IllegalArgumentException("negative minorVersion");
}
this.protocolName = protocolName;
this.majorVersion = majorVersion;
this.minorVersion = minorVersion;
text = protocolName + '/' + majorVersion + '.' + minorVersion;
}
public String getProtocolName() {
return protocolName;
}
public int getMajorVersion() {
return majorVersion;
}
public int getMinorVersion() {
return minorVersion;
}
public String getText() {
return text;
}
@Override
public String toString() {
return getText();
}
@Override
public int hashCode() {
return (getProtocolName().hashCode() * 31 + getMajorVersion()) * 31 +
getMinorVersion();
}
@Override
public boolean equals(Object o) {
if (!(o instanceof HttpVersion)) {
return false;
}
HttpVersion that = (HttpVersion) o;
return getMinorVersion() == that.getMinorVersion() &&
getMajorVersion() == that.getMajorVersion() &&
getProtocolName().equals(that.getProtocolName());
}
public int compareTo(HttpVersion o) {
int v = getProtocolName().compareTo(o.getProtocolName());
if (v != 0) {
return v;
}
v = getMajorVersion() - o.getMajorVersion();
if (v != 0) {
return v;
}
return getMinorVersion() - o.getMinorVersion();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy