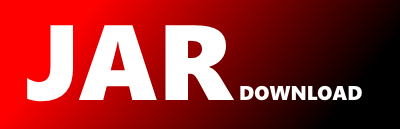
javax.portlet.faces.preference.Preference Maven / Gradle / Ivy
/*
* JBoss, Home of Professional Open Source.
* Copyright 2012, Red Hat, Inc., and individual contributors
* as indicated by the @author tags. See the copyright.txt file in the
* distribution for a full listing of individual contributors.
*
* This is free software; you can redistribute it and/or modify it
* under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation; either version 2.1 of
* the License, or (at your option) any later version.
*
* This software is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this software; if not, write to the Free
* Software Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA
* 02110-1301 USA, or see the FSF site: http://www.fsf.org.
*/
package javax.portlet.faces.preference;
import java.util.List;
import javax.portlet.ReadOnlyException;
/**
* The Preference
interface allows one to access each PortletPreferences
as a discrete object.
* This allows one to more easily access a preference via EL. Operations made on a Preference
object are
* immediately reflected in the underlying PortletPreferences
. As usual, changes aren't committed until
* PortletPreferences.store
is called.
*/
public interface Preference {
/**
* Sets the name of this preference.
*
* @param name
* the new name for this preference.
*/
void setName(String name);
/**
* Returns the name of this preference.
*
* @return the name of this preference.
*/
String getName();
/**
* Associates the specified String value with this preference.
*
* null
values for the value parameter are allowed.
*
* @param value
* value to be associated with the specified key.
*
* @exception ReadOnlyException
* if this preference cannot be modified for this request
*
* @see #setValues(String[])
*/
void setValue(String value) throws ReadOnlyException;
/**
* Returns the first String value associated with this preference. If there is one or more values associated with
* this preference it returns the first associated value. If there are no values associated with this preference, or
* the backing preference database is unavailable, it returns null.
*
* @return the first value associated with this preference, or null
if there isn't an associated value
* or the backing store is inaccessible.
*
*
* @see #getValues()
*/
String getValue();
/**
* Associates the specified String array value with this preference.
*
* null
values in the values parameter are allowed.
*
* @param values
* values to be associated with key
*
* @exception ReadOnlyException
* if this preference cannot be modified for this request
*
* @see #setValue(String)
*/
void setValues(String[] values) throws ReadOnlyException;
/**
* Returns a List
of values associated with this preference.
*
*
* Returns the null
if there aren't any values, or if the backing store is inaccessible.
*
*
* If the implementation supports stored defaults and such a default exists and is accessible, they are
* returned in a situation where null otherwise would have been returned.
*
*
*
* @return the List associated with this preference, or null
if the associated value does not exist.
*
* @see #getValue()
*/
List getValues();
/**
* Returns true, if the value of this preference cannot be modified by the user.
*
* Modifiable preferences can be changed by the portlet in any standard portlet mode (EDIT, HELP, VIEW
* ). Per default every preference is modifiable.
*
* Read-only preferences cannot be changed by the portlet in any standard portlet mode, but inside of custom modes
* it may be allowed changing them. Preferences are read-only, if they are defined in the deployment descriptor with
* read-only
set to true
, or if the portlet container restricts write access.
*
* @return false, if the value of this preference can be changed
*
*/
boolean isReadOnly();
/**
* Resets or removes the value(s) of this preference.
*
* If this implementation supports stored defaults, and there is such a default for the specified preference, the
* preference will be reset to the stored default.
*
* If there is no default available the preference will be removed from the underyling system.
*
* @exception ReadOnlyException
* if this preference cannot be modified for this request
*/
void reset() throws ReadOnlyException;
}