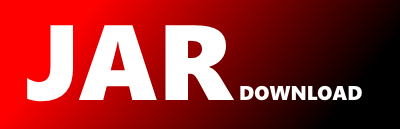
org.jboss.pressgang.ccms.wrapper.DBContentSpecWrapper Maven / Gradle / Ivy
package org.jboss.pressgang.ccms.wrapper;
import java.util.Collection;
import java.util.List;
import java.util.Set;
import org.jboss.pressgang.ccms.model.Tag;
import org.jboss.pressgang.ccms.model.contentspec.CSNode;
import org.jboss.pressgang.ccms.model.contentspec.ContentSpec;
import org.jboss.pressgang.ccms.model.contentspec.TranslatedContentSpec;
import org.jboss.pressgang.ccms.provider.DBProviderFactory;
import org.jboss.pressgang.ccms.wrapper.base.DBBaseContentSpecWrapper;
import org.jboss.pressgang.ccms.wrapper.collection.CollectionWrapper;
import org.jboss.pressgang.ccms.wrapper.collection.DBCSNodeCollectionWrapper;
import org.jboss.pressgang.ccms.wrapper.collection.DBTagCollectionWrapper;
import org.jboss.pressgang.ccms.wrapper.collection.UpdateableCollectionWrapper;
import org.jboss.pressgang.ccms.wrapper.collection.handler.DBCSBookTagCollectionHandler;
import org.jboss.pressgang.ccms.wrapper.collection.handler.DBCSNodeCollectionHandler;
public class DBContentSpecWrapper extends DBBaseContentSpecWrapper implements ContentSpecWrapper {
private final DBCSNodeCollectionHandler csNodeCollectionHandler;
private final DBCSBookTagCollectionHandler bookTagCollectionHandler;
public DBContentSpecWrapper(final DBProviderFactory providerFactory, final ContentSpec contentSpec, boolean isRevision) {
super(providerFactory, contentSpec, isRevision);
csNodeCollectionHandler = new DBCSNodeCollectionHandler(contentSpec);
bookTagCollectionHandler = new DBCSBookTagCollectionHandler(contentSpec);
}
@Override
public CollectionWrapper getBookTags() {
return getWrapperFactory().createCollection(getEntity().getBookTags(), Tag.class, isRevisionEntity(), bookTagCollectionHandler);
}
@Override
public void setBookTags(CollectionWrapper bookTags) {
if (bookTags == null) return;
final DBTagCollectionWrapper dbTags = (DBTagCollectionWrapper) bookTags;
dbTags.setHandler(bookTagCollectionHandler);
// Since book tags in a content spec are generated from a set and not cached, there is no way to see if this collection is the same
// as the collection passed. So just process all the tags anyway.
// Add new tags and skip any existing tags
final List currentBookTags = getEntity().getBookTags();
final Collection newBookTags = dbTags.unwrap();
for (final Tag bookTag : newBookTags) {
if (currentBookTags.contains(bookTag)) {
currentBookTags.remove(bookTag);
continue;
} else {
getEntity().addBookTag(bookTag);
}
}
// Remove tags that should no longer exist in the collection
for (final Tag removeBookTag : currentBookTags) {
getEntity().removeBookTag(removeBookTag);
}
}
@Override
public UpdateableCollectionWrapper getChildren() {
final CollectionWrapper collection = getWrapperFactory().createCollection(getEntity().getChildrenList(), CSNode.class,
isRevisionEntity(), csNodeCollectionHandler);
return (UpdateableCollectionWrapper) collection;
}
@Override
public void setChildren(UpdateableCollectionWrapper nodes) {
if (nodes == null) return;
final DBCSNodeCollectionWrapper dbNodes = (DBCSNodeCollectionWrapper) nodes;
dbNodes.setHandler(csNodeCollectionHandler);
// Since children nodes in a content spec are generated from a set and not cached, there is no way to see if this collection is
// the same as the collection passed. So just process all the nodes anyway.
// Add new tags and skip any existing tags
final Set currentChildren = getEntity().getChildren();
final Collection newChildren = dbNodes.unwrap();
for (final CSNode child : newChildren) {
if (currentChildren.contains(child)) {
currentChildren.remove(child);
continue;
} else {
getEntity().addChild(child);
}
}
// Remove tags that should no longer exist in the collection
for (final CSNode removeChild : currentChildren) {
getEntity().removeChild(removeChild);
}
}
@Override
public CollectionWrapper getTranslatedContentSpecs() {
return getWrapperFactory().createCollection(getEntity().getTranslatedContentSpecs(getEntityManager(), getRevision()),
TranslatedContentSpec.class, isRevisionEntity());
}
@Override
public void setType(Integer typeId) {
getEntity().setContentSpecType(typeId);
}
@Override
public String getCondition() {
return getEntity().getCondition();
}
@Override
public void setCondition(String condition) {
getEntity().setCondition(condition);
}
@Override
public CSNodeWrapper getMetaData(String metaDataTitle) {
return getWrapperFactory().create(getEntity().getMetaData(metaDataTitle), isRevisionEntity());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy