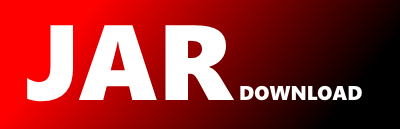
org.jboss.resteasy.client.jaxrs.internal.ResteasyClientImpl Maven / Gradle / Ivy
package org.jboss.resteasy.client.jaxrs.internal;
import java.net.URI;
import java.security.AccessController;
import java.security.PrivilegedAction;
import java.util.Map;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.ScheduledExecutorService;
import javax.net.ssl.HostnameVerifier;
import javax.net.ssl.SSLContext;
import jakarta.ws.rs.client.Invocation;
import jakarta.ws.rs.client.WebTarget;
import jakarta.ws.rs.core.Configuration;
import jakarta.ws.rs.core.Link;
import jakarta.ws.rs.core.UriBuilder;
import org.jboss.resteasy.client.jaxrs.ClientHttpEngine;
import org.jboss.resteasy.client.jaxrs.ResteasyClient;
import org.jboss.resteasy.client.jaxrs.ResteasyWebTarget;
import org.jboss.resteasy.client.jaxrs.i18n.Messages;
import org.jboss.resteasy.concurrent.ContextualExecutors;
import org.jboss.resteasy.concurrent.ContextualScheduledExecutorService;
/**
* @author Bill Burke
* @version $Revision: 1 $
*/
public class ResteasyClientImpl implements ResteasyClient {
protected final ClientHttpEngine httpEngine;
private final ExecutorService asyncInvocationExecutor;
private final ContextualScheduledExecutorService scheduledExecutorService;
protected ClientConfiguration configuration;
protected boolean closed;
protected boolean cleanupExecutor;
protected ResteasyClientImpl(final ClientHttpEngine httpEngine, final ExecutorService asyncInvocationExecutor,
final boolean cleanupExecutor,
final ScheduledExecutorService scheduledExecutorService, final ClientConfiguration configuration) {
this(httpEngine, asyncInvocationExecutor, cleanupExecutor, ContextualExecutors.wrap(scheduledExecutorService),
configuration);
}
protected ResteasyClientImpl(final ClientHttpEngine httpEngine, final ExecutorService asyncInvocationExecutor,
final boolean cleanupExecutor,
final ContextualScheduledExecutorService scheduledExecutorService, final ClientConfiguration configuration) {
this.cleanupExecutor = cleanupExecutor;
this.httpEngine = httpEngine;
this.asyncInvocationExecutor = asyncInvocationExecutor;
this.configuration = configuration;
this.scheduledExecutorService = scheduledExecutorService;
}
protected ResteasyClientImpl(final ClientHttpEngine httpEngine, final ExecutorService asyncInvocationExecutor,
final boolean cleanupExecutor, final ClientConfiguration configuration) {
this(httpEngine, asyncInvocationExecutor, cleanupExecutor, ContextualExecutors.scheduledThreadPool(), configuration);
}
public ClientHttpEngine httpEngine() {
abortIfClosed();
return httpEngine;
}
public ExecutorService asyncInvocationExecutor() {
return asyncInvocationExecutor;
}
public ScheduledExecutorService getScheduledExecutor() {
return this.scheduledExecutorService;
}
public void abortIfClosed() {
if (isClosed())
throw new IllegalStateException(Messages.MESSAGES.clientIsClosed());
}
public boolean isClosed() {
return closed;
}
@Override
public void close() {
closed = true;
try {
httpEngine.close();
if (cleanupExecutor) {
if (System.getSecurityManager() == null) {
asyncInvocationExecutor.shutdown();
} else {
AccessController.doPrivileged(new PrivilegedAction() {
@Override
public Void run() {
asyncInvocationExecutor.shutdown();
return null;
}
});
}
}
if (System.getSecurityManager() == null) {
if (scheduledExecutorService != null && !scheduledExecutorService.isManaged()) {
scheduledExecutorService.shutdown();
}
} else {
AccessController.doPrivileged((PrivilegedAction
© 2015 - 2025 Weber Informatics LLC | Privacy Policy